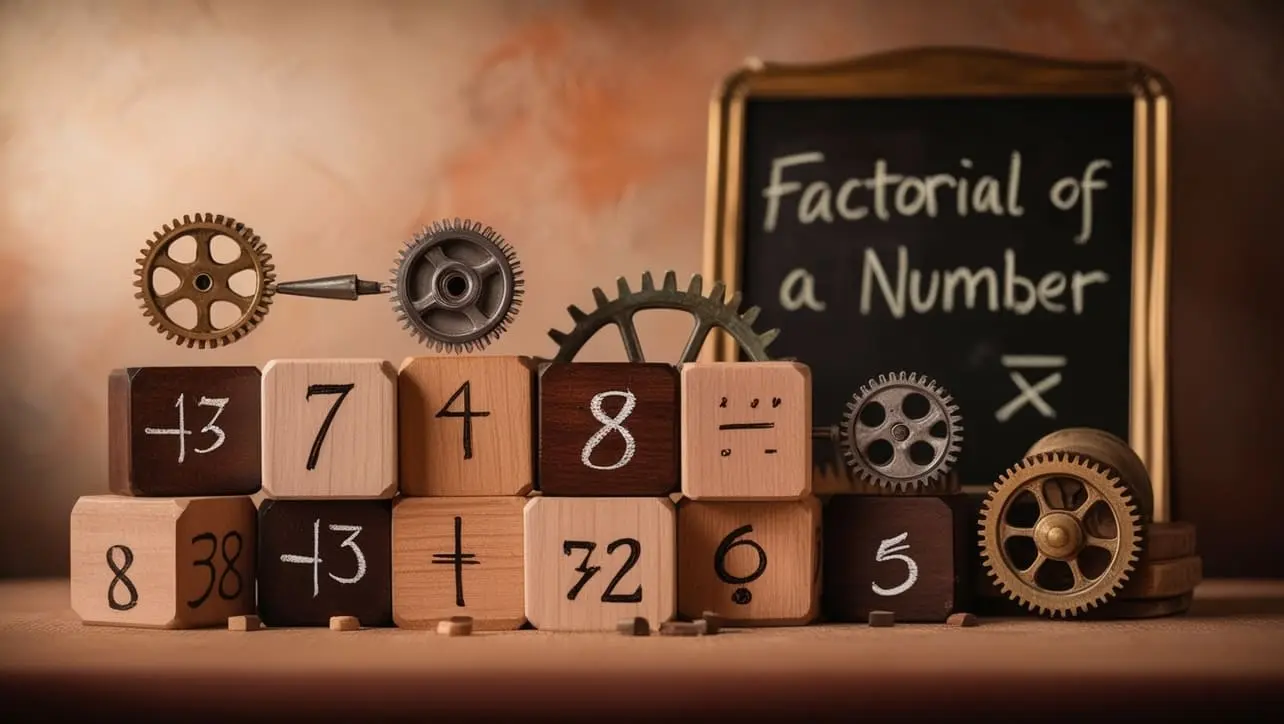
C Program to find Factorial of a Number
The factorial of a number is a mathematical operation that denotes the product of all positive integers from 1 to that number. It is denoted by the exclamation mark (!)
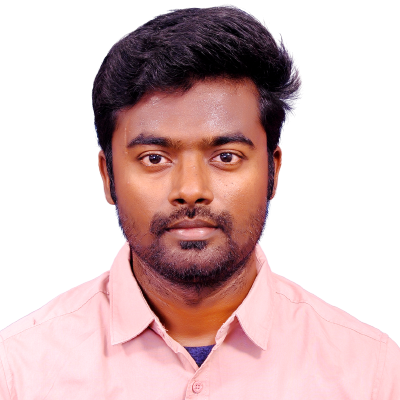
The factorial of a number is a mathematical operation that denotes the product of all positive integers from 1 to that number. It is denoted by the exclamation mark (!)
Condensing a Number refers to summing its digits until a single-digit number is obtained.
An even number is an integer that is divisible by 2, meaning it leaves no remainder when divided by 2. In other words, an even number can be divided into two equal parts.
An odd number is an integer that is not divisible by 2, meaning it does not leave a remainder of 0 when divided by 2. In other words, an odd number cannot be evenly divided into two equal parts.
Prime factors are the prime numbers that divide a given number evenly, without leaving a remainder. In other words, they are the prime numbers that are factors of a given number.
A prime number is a natural number greater than 1 that has no positive divisors other than 1 and itself. In other words, a prime number is a number that is only divisible by 1 and itself, with no other factors.
An Armstrong number, also known as a narcissistic number, is a number that is equal to the sum of its own digits, each raised to the power of the number of digits.
Swapping two numbers means exchanging their values. In other words, if you have two variables A and B, swapping them would involve interchanging their values so that the initial value of A becomes the value of B, and vice versa.
C interview programs refer to programming questions or problems that are commonly asked in job interviews for positions that require knowledge of the C programming language. These programs are designed to test a candidate’s understanding of C concepts, problem-solving skills, and ability to write efficient and correct code.
The cos() function is a standard library function in C that is used to calculate the cosine of a specified angle.
The cosh() function is a standard library function in C that is used to calculate the hyperbolic cosine of a specified number.
The ceil() function is a standard library function in C that is used to round a specified floating-point number up to the nearest integer greater than or equal to the given value.
The cbrt() function is a standard library function in C that is used to calculate the cube root of a specified number.
The atanh() function is a standard library function in C that is used to calculate the inverse hyperbolic tangent (artanh) of a given argument.
The atan() function is a standard library function in C that is used to calculate the arctangent of a specified number.
The atan2() function is a standard library function in C that is used to calculate the arctangent of the ratio of two specified numbers.
The asinh() function is a standard library function in C that is used to calculate the inverse hyperbolic sine (arcsinh) of a given argument.
The ldexp() function is a standard library function in C that is used to multiply a given floating-point value by a specified power of 2.