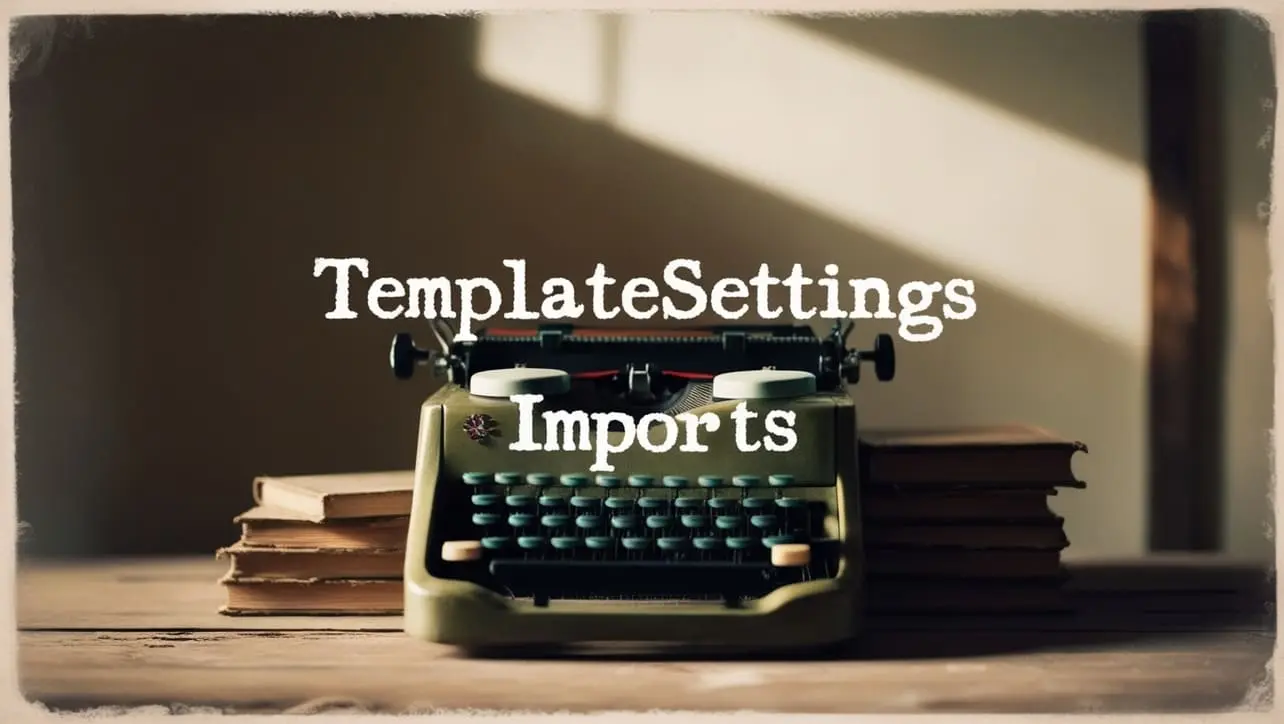
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.toPath() Util Method
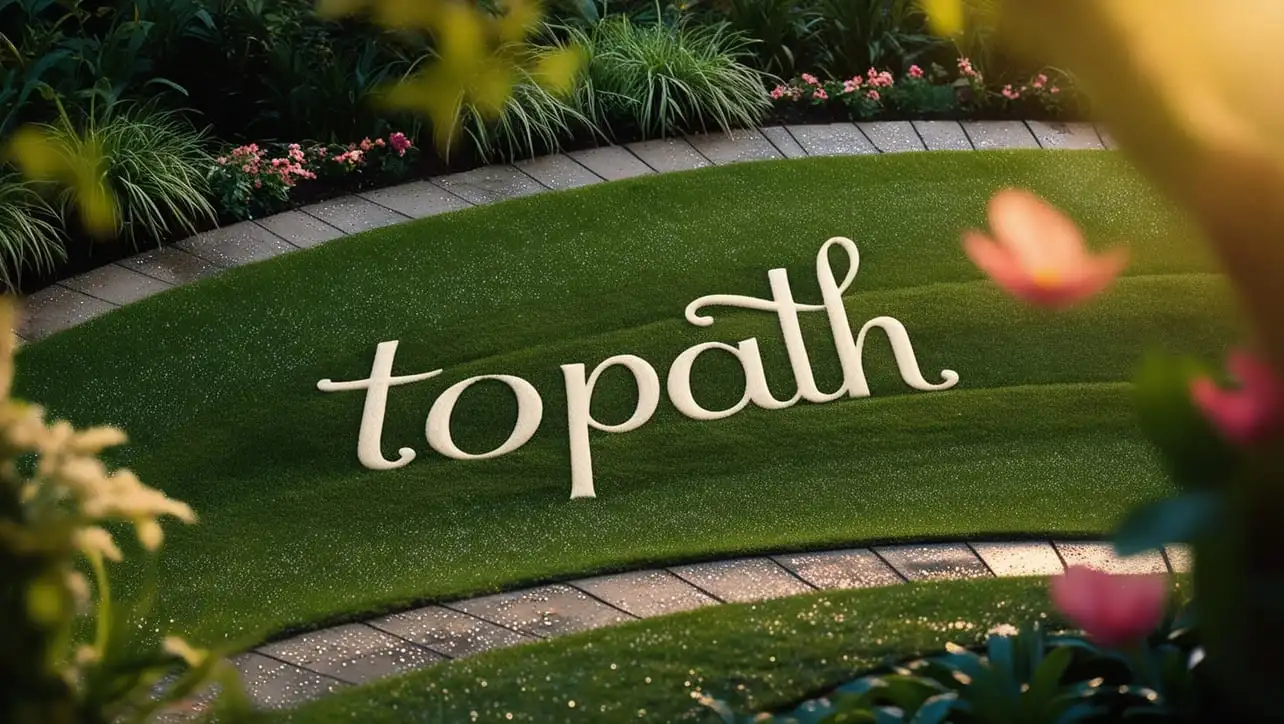
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript programming, navigating complex nested objects or accessing deeply nested properties can be a challenging task. Fortunately, Lodash provides a versatile utility function called _.toPath()
to simplify this process.
The _.toPath()
method converts a string representation of a property path into an array of property names or keys, making it easier to traverse nested structures and access specific values within objects.
🧠 Understanding _.toPath() Method
The _.toPath()
method in Lodash serves as a bridge between string-based property paths and the corresponding array-based representation. This conversion facilitates dynamic property access and navigation within complex objects or JSON structures.
💡 Syntax
The syntax for the _.toPath()
method is straightforward:
_.toPath(value)
- value: The property path string to convert.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.toPath()
method:
const _ = require('lodash');
const pathString = 'a.b[0].c.d';
const pathArray = _.toPath(pathString);
console.log(pathArray);
// Output: ['a', 'b', '0', 'c', 'd']
In this example, the pathString representing a nested property path is converted into an array of property names and array indices using _.toPath()
.
🏆 Best Practices
When working with the _.toPath()
method, consider the following best practices:
Error Handling:
Ensure robust error handling when using
_.toPath()
to handle invalid or malformed property paths gracefully.example.jsCopiedconst invalidPathString = 'a.b..c[0]'; const pathArray = _.toPath(invalidPathString); if(pathArray.length === 0) { console.error('Invalid property path:', invalidPathString); } else { console.log('Valid property path:', pathArray); }
Dynamically Accessing Properties:
Utilize
_.toPath()
in scenarios where you need to dynamically access nested properties within objects based on user input or configuration data.example.jsCopiedconst userConfig = { 'user': { 'name': 'John', 'address': { 'street': '123 Main St', 'city': 'Anytown' } } }; const propertyPath = 'user.address.city'; const pathArray = _.toPath(propertyPath); const city = _.get(userConfig, pathArray); console.log('User city:', city);
Data Transformation:
Leverage
_.toPath()
to transform string-based property paths into array representations for further manipulation or processing.example.jsCopiedconst propertyPaths = ['user.name', 'user.address.city', 'user.address.postalCode']; const transformedPaths = propertyPaths.map(_.toPath); console.log('Transformed property paths:', transformedPaths);
📚 Use Cases
Accessing Nested Properties:
_.toPath()
is invaluable when working with complex JSON data structures and needing to access deeply nested properties dynamically.example.jsCopiedconst nestedObject = { 'a': { 'b': { 'c': { 'd': 'Nested Value' } } } }; const propertyPath = 'a.b.c.d'; const pathArray = _.toPath(propertyPath); const nestedValue = _.get(nestedObject, pathArray); console.log('Nested value:', nestedValue);
Forming Property Paths Dynamically:
When constructing property paths dynamically,
_.toPath()
can be used to convert string representations into arrays for subsequent manipulation or property access.example.jsCopiedconst parentObject = {}; const propertyKeys = ['user', 'address', 'city']; const propertyPath = propertyKeys.join('.'); const pathArray = _.toPath(propertyPath); _.set(parentObject, pathArray, 'Anytown'); console.log('Updated parent object:', parentObject);
Validation and Sanitization:
In scenarios where validation or sanitization of property paths is required,
_.toPath()
can be used to transform and validate user-provided input.example.jsCopiedconst userInput = 'user.address.city'; const sanitizedPath = _.toPath(userInput); console.log('Sanitized property path:', sanitizedPath);
🎉 Conclusion
The _.toPath()
utility method in Lodash provides a convenient way to convert string-based property paths into array representations, facilitating dynamic property access and traversal within nested objects. Whether you're accessing deeply nested properties, dynamically constructing property paths, or validating user input, _.toPath()
serves as a valuable tool in your JavaScript toolkit.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.toPath()
method in your Lodash projects.
👨💻 Join our Community:
Author
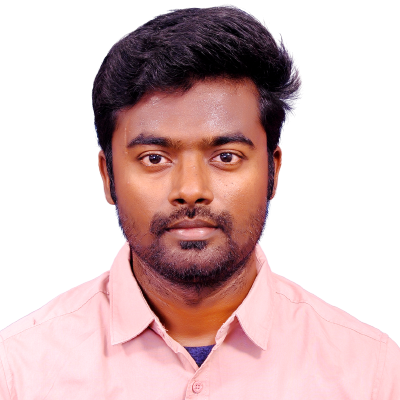
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
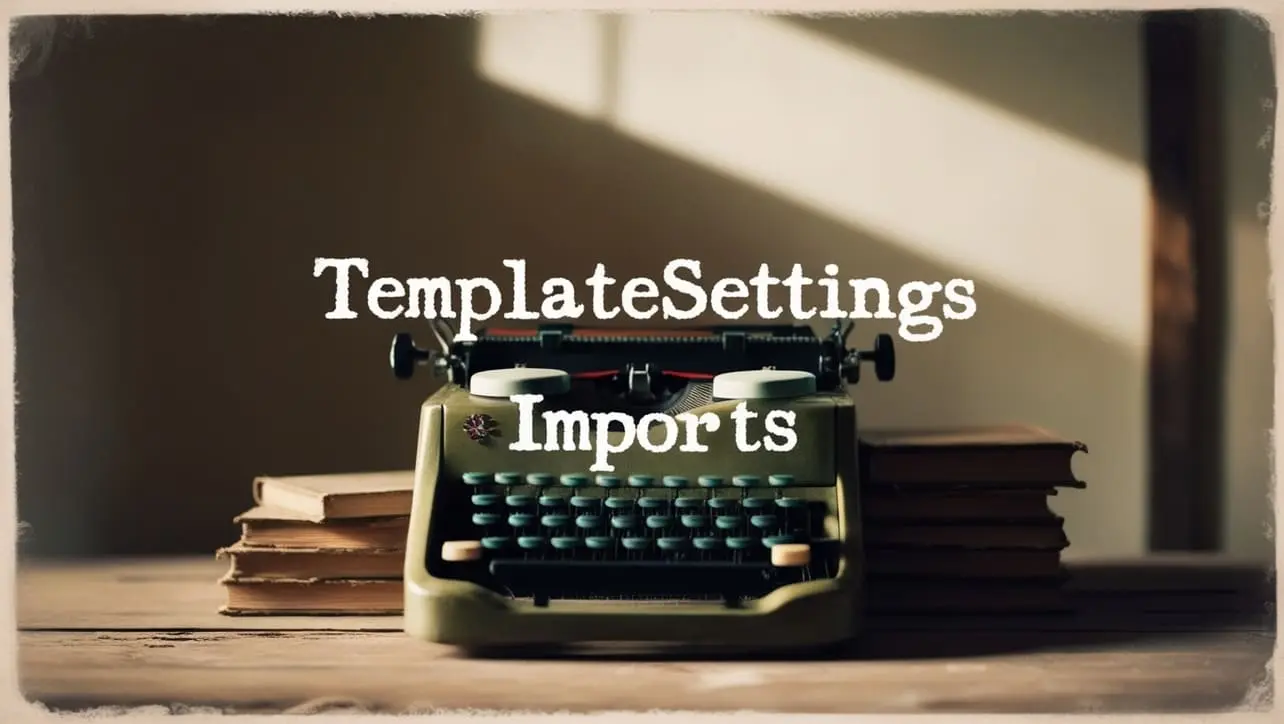
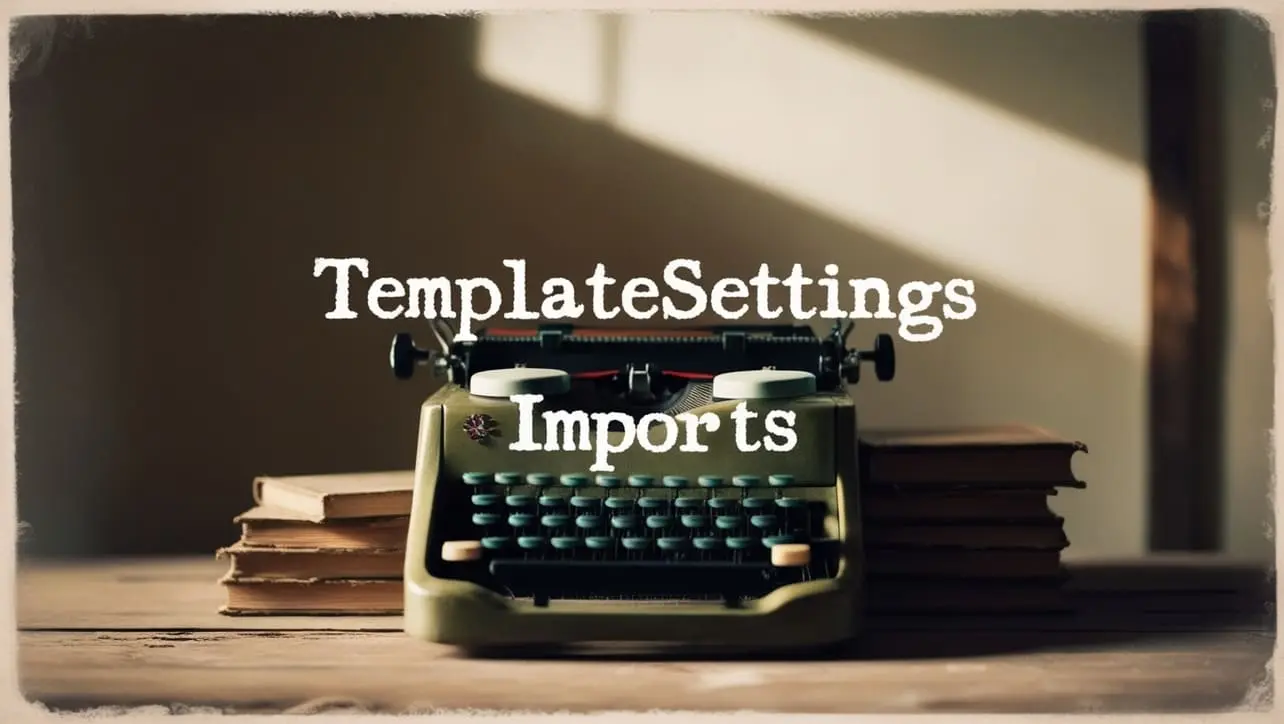
Lodash _.templateSettings.imports Property
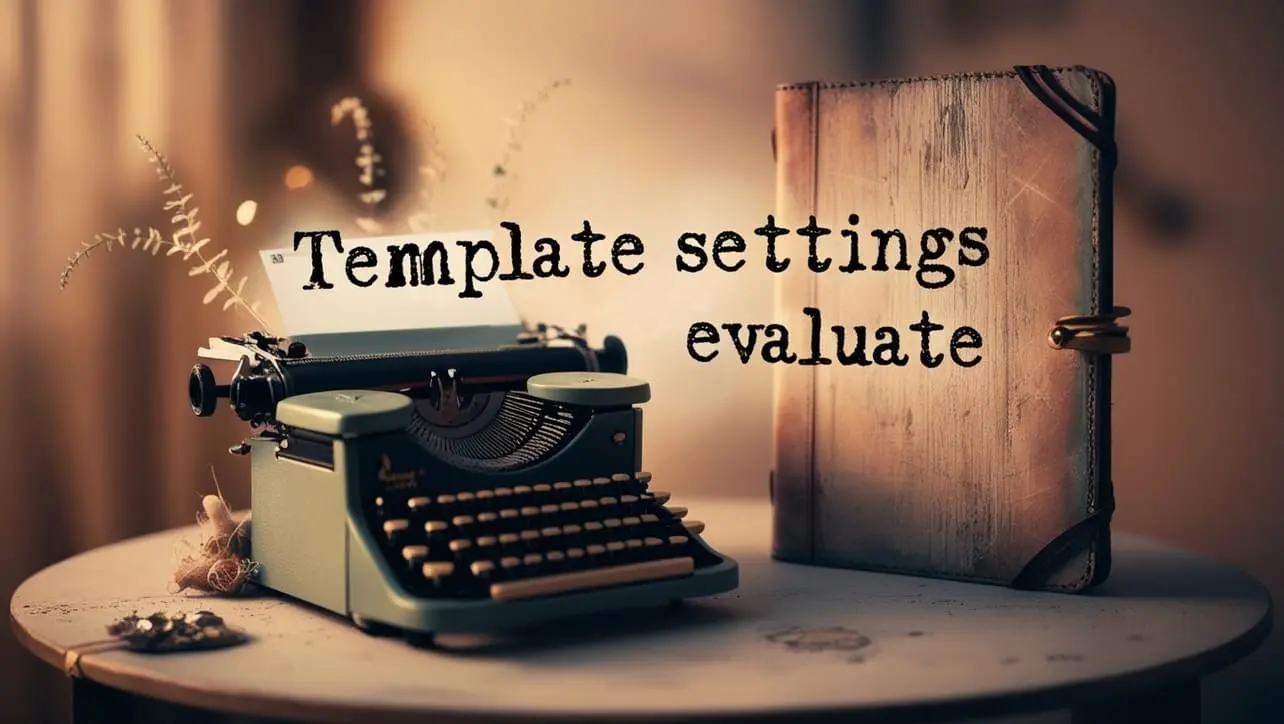
Lodash _.templateSettings.evaluate Property
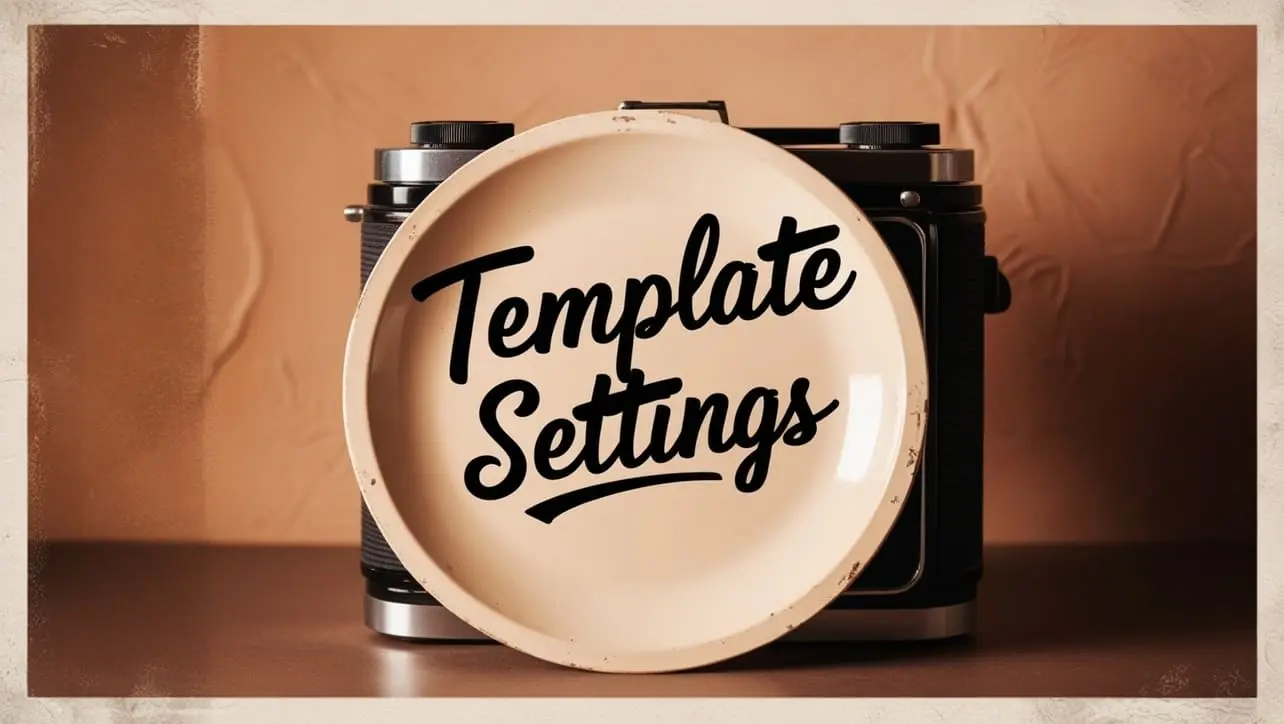
Lodash _.templateSettings Property
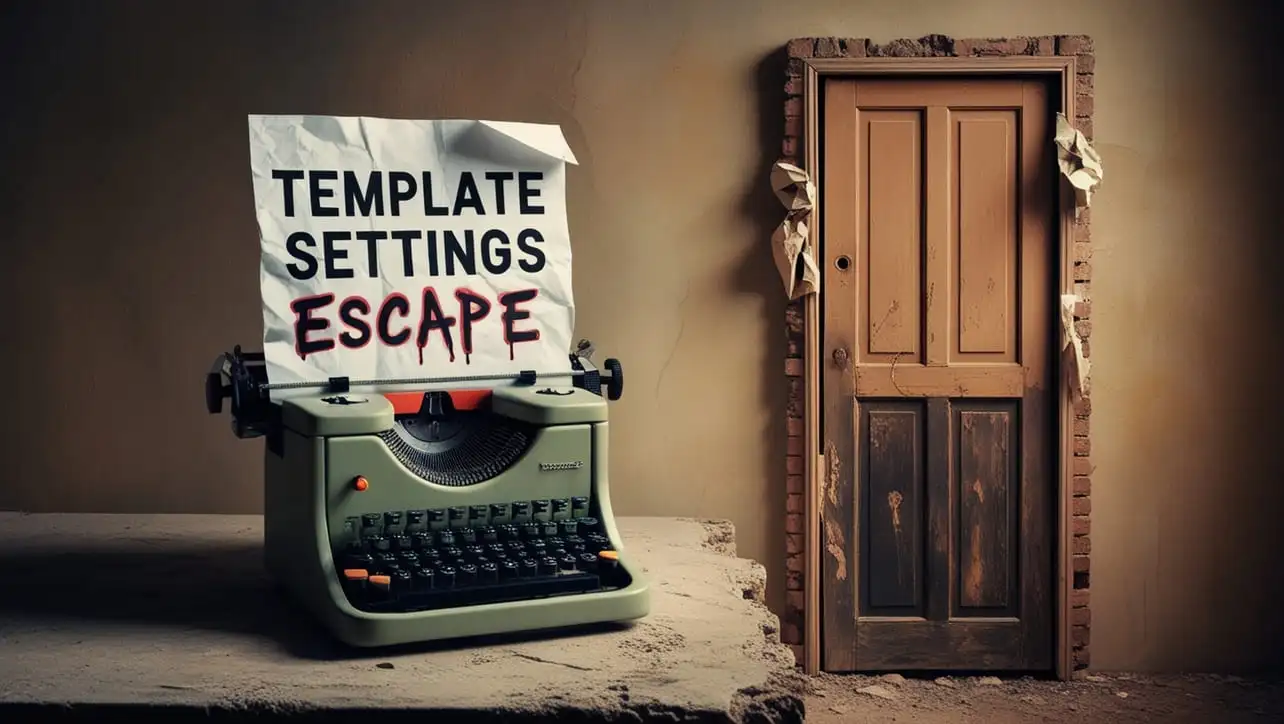
Lodash _.templateSettings.escape Property
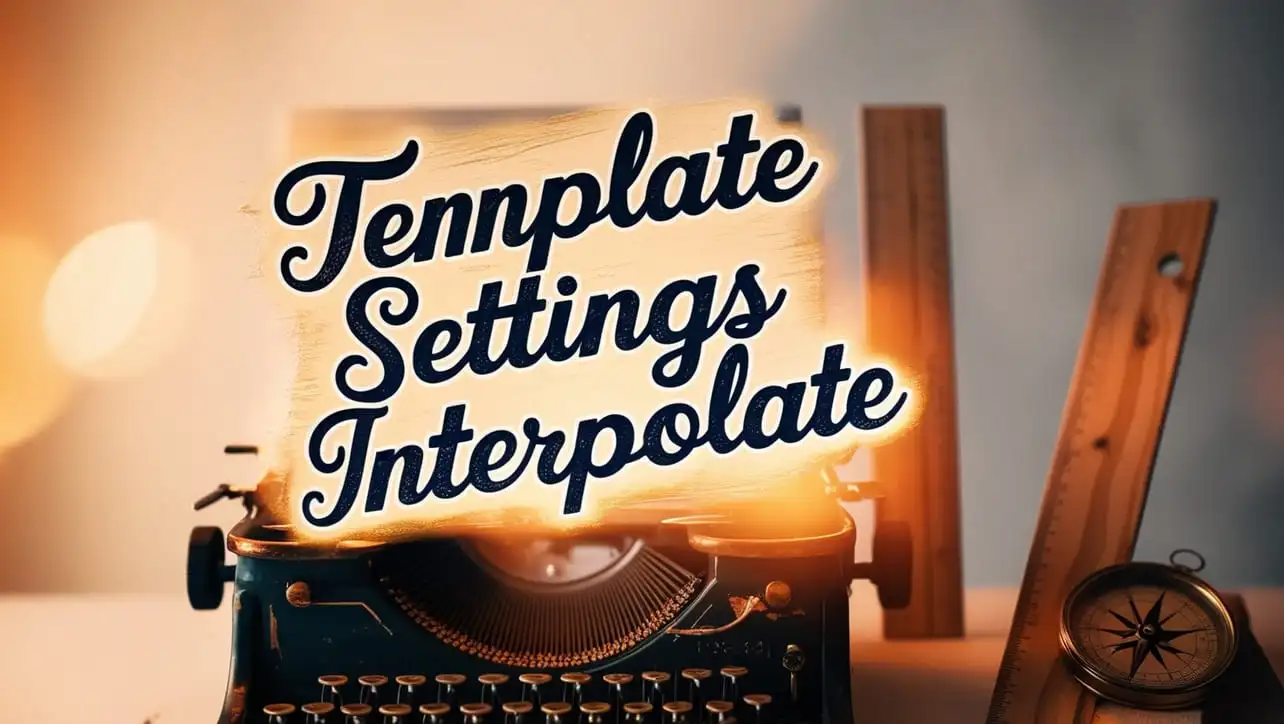
Lodash _.templateSettings.interpolate Property
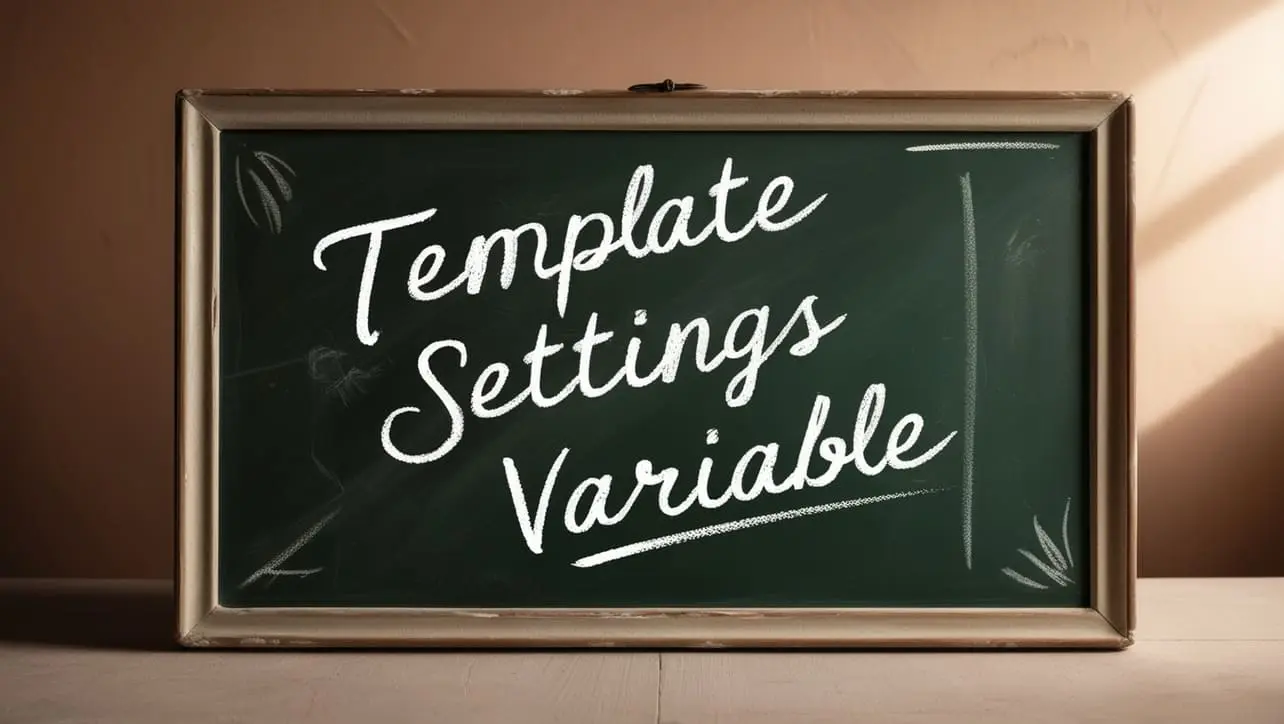
If you have any doubts regarding this article (Lodash _.toPath() Util Method), please comment here. I will help you immediately.