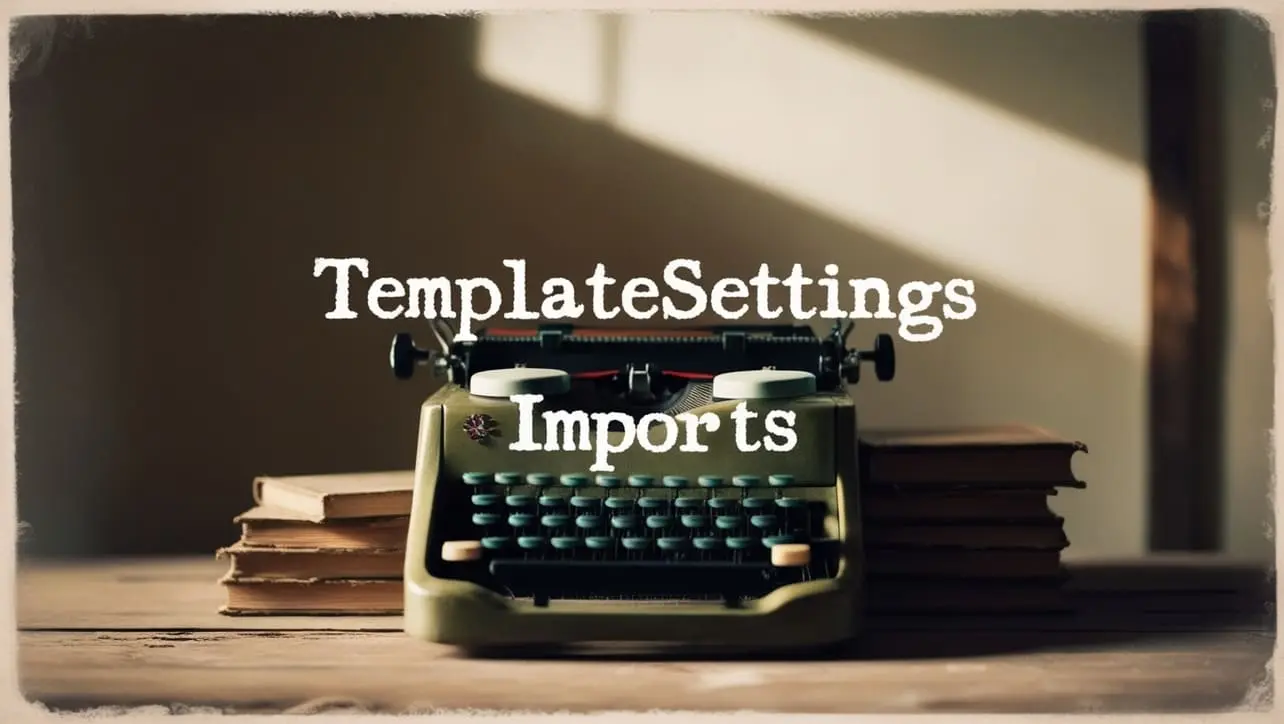
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.propertyOf() Util Method
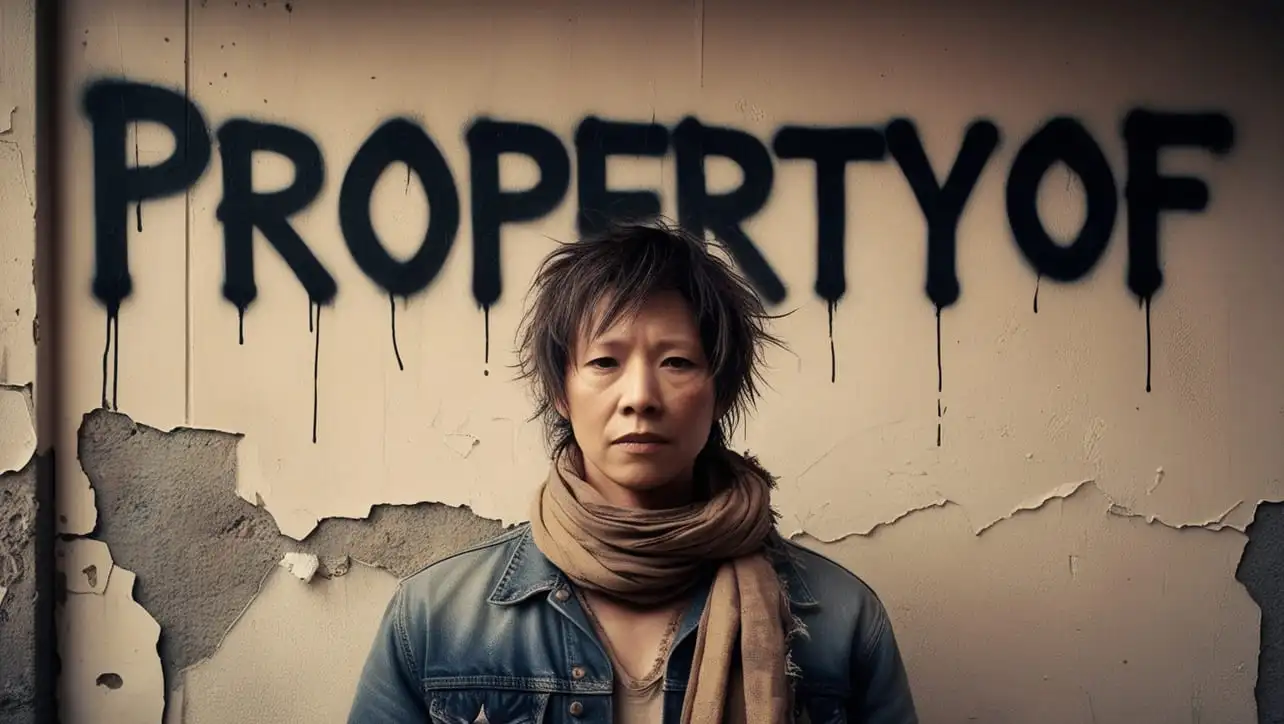
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript programming, accessing nested properties of objects can sometimes be cumbersome and error-prone. Lodash, a popular utility library, offers a variety of functions to simplify common programming tasks. One such function is _.propertyOf()
, which provides a convenient way to access nested properties of objects using a string path.
This method enhances code readability and reduces the complexity of property access operations.
🧠 Understanding _.propertyOf() Method
The _.propertyOf()
method in Lodash creates a function that returns the value of a property at the given path of an object. This allows developers to access nested properties in a concise and readable manner, improving code maintainability and efficiency.
💡 Syntax
The syntax for the _.propertyOf()
method is straightforward:
_.propertyOf(object)
- object: The object to query.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.propertyOf()
method:
const _ = require('lodash');
const user = {
name: 'John Doe',
address: {
city: 'New York',
zipCode: '10001'
}
};
const getAddress = _.propertyOf(user);
console.log(getAddress('address.city'));
// Output: 'New York'
console.log(getAddress('address.zipCode'));
// Output: '10001'
In this example, _.propertyOf(user) creates a function getAddress that can be used to retrieve the values of nested properties of the user object.
🏆 Best Practices
When working with the _.propertyOf()
method, consider the following best practices:
Error Handling:
Ensure proper error handling when using
_.propertyOf()
, especially when accessing nested properties. Handle cases where the specified property path does not exist to prevent unexpected errors.example.jsCopiedconst getUserAddress = _.propertyOf(user); const city = getUserAddress('address.city'); const country = getUserAddress('address.country'); // Error: Cannot read property 'country' of undefined if(country === undefined) { console.log('Country not available'); } else { console.log(country); }
Dynamic Property Access:
Utilize
_.propertyOf()
for dynamic property access, allowing flexibility in property retrieval based on runtime conditions or user input.example.jsCopiedconst getProperty = _.propertyOf(user); const propertyPath = 'address.city'; console.log(getProperty(propertyPath)); // Output: 'New York' // Dynamically change property path const newPropertyPath = 'name'; console.log(getProperty(newPropertyPath)); // Output: 'John Doe'
Performance Considerations:
Be mindful of performance implications when using
_.propertyOf()
, especially in performance-critical applications. Avoid unnecessary property accesses within loops or high-frequency operations.example.jsCopiedconst getUserProperty = _.propertyOf(user); // Performance test console.time('propertyAccess'); for (let i = 0; i < 1000000; i++) { getUserProperty('address.city'); } console.timeEnd('propertyAccess');
📚 Use Cases
Data Transformation:
_.propertyOf()
is useful for data transformation tasks where nested properties need to be extracted from objects for further processing or display.example.jsCopiedconst users = [ { id: 1, name: 'Alice', address: { city: 'New York' } }, { id: 2, name: 'Bob', address: { city: 'Los Angeles' } }, { id: 3, name: 'Charlie', address: { city: 'Chicago' } } ]; const getCity = _.propertyOf('address.city'); const cities = users.map(user => getCity(user)); console.log(cities); // Output: ['New York', 'Los Angeles', 'Chicago']
Dynamic UI Rendering:
In dynamic UI rendering scenarios,
_.propertyOf()
can be used to access nested properties of objects based on dynamic data requirements, simplifying the rendering process.example.jsCopiedconst userData = { name: 'John Doe', profile: { email: 'john@example.com', phone: '+1234567890' } }; const property = 'profile.email'; const getValue = _.propertyOf(userData); console.log(getValue(property)); // Output: 'john@example.com'
Configuration Management:
When managing configurations with nested properties,
_.propertyOf()
can facilitate easy access to specific configuration values based on predefined paths.example.jsCopiedconst config = { app: { name: 'MyApp', version: '1.0', settings: { theme: 'dark', language: 'en' } } }; const getTheme = _.propertyOf(config); console.log(getTheme('app.settings.theme')); // Output: 'dark'
🎉 Conclusion
The _.propertyOf()
method in Lodash provides a convenient and efficient way to access nested properties of objects using string paths. Whether you're extracting data, rendering dynamic UI elements, or managing configurations, this method offers versatility and simplicity in property access operations.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.propertyOf()
method in your Lodash projects.
👨💻 Join our Community:
Author
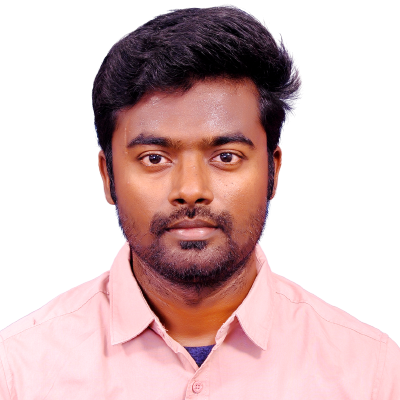
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
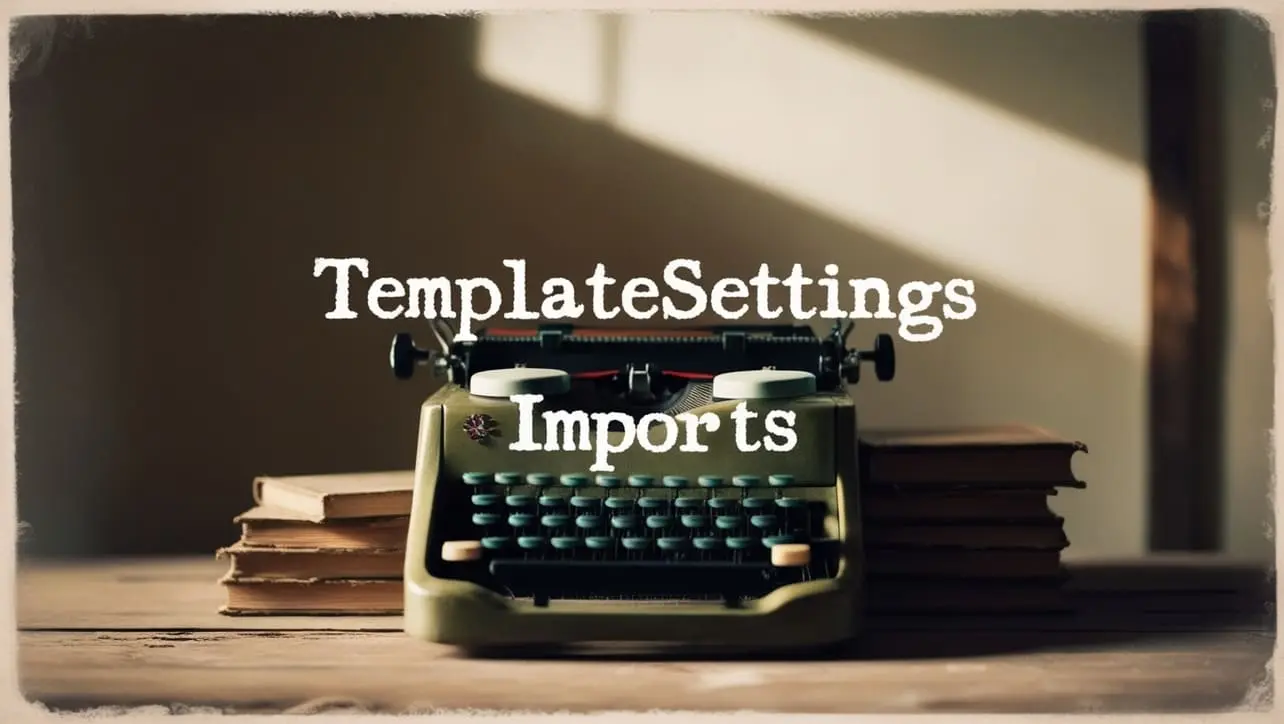
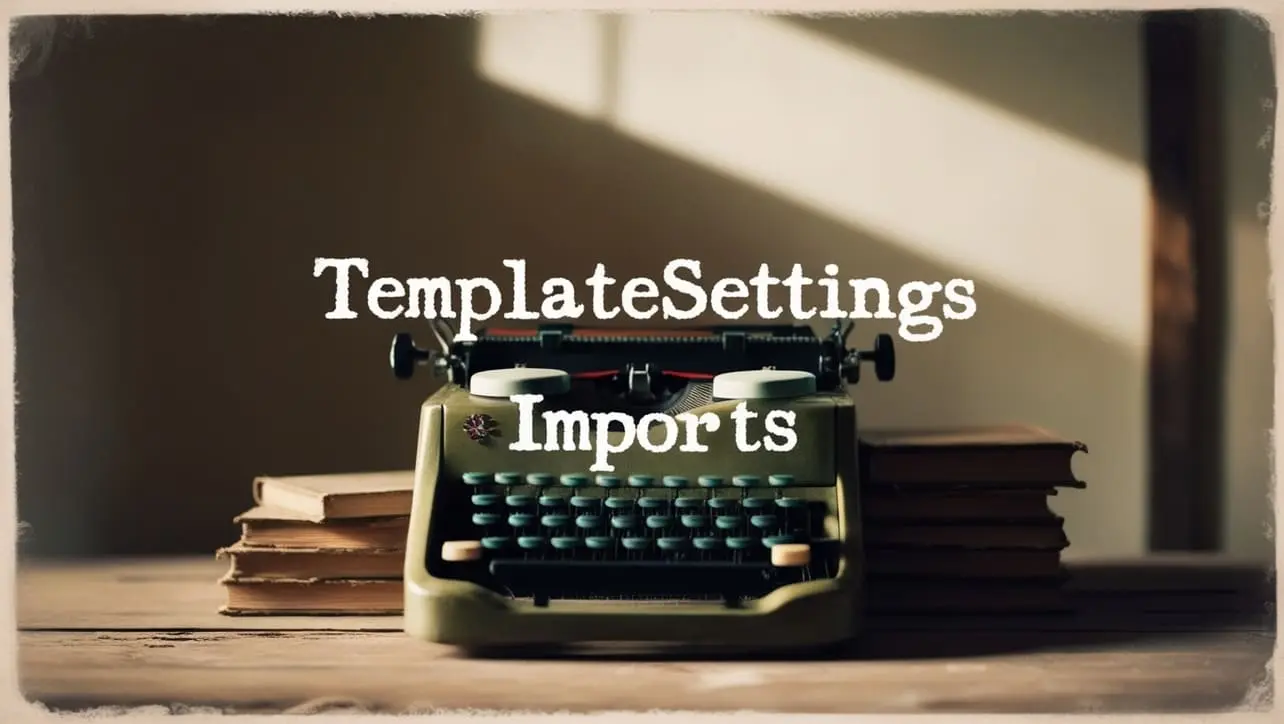
Lodash _.templateSettings.imports Property
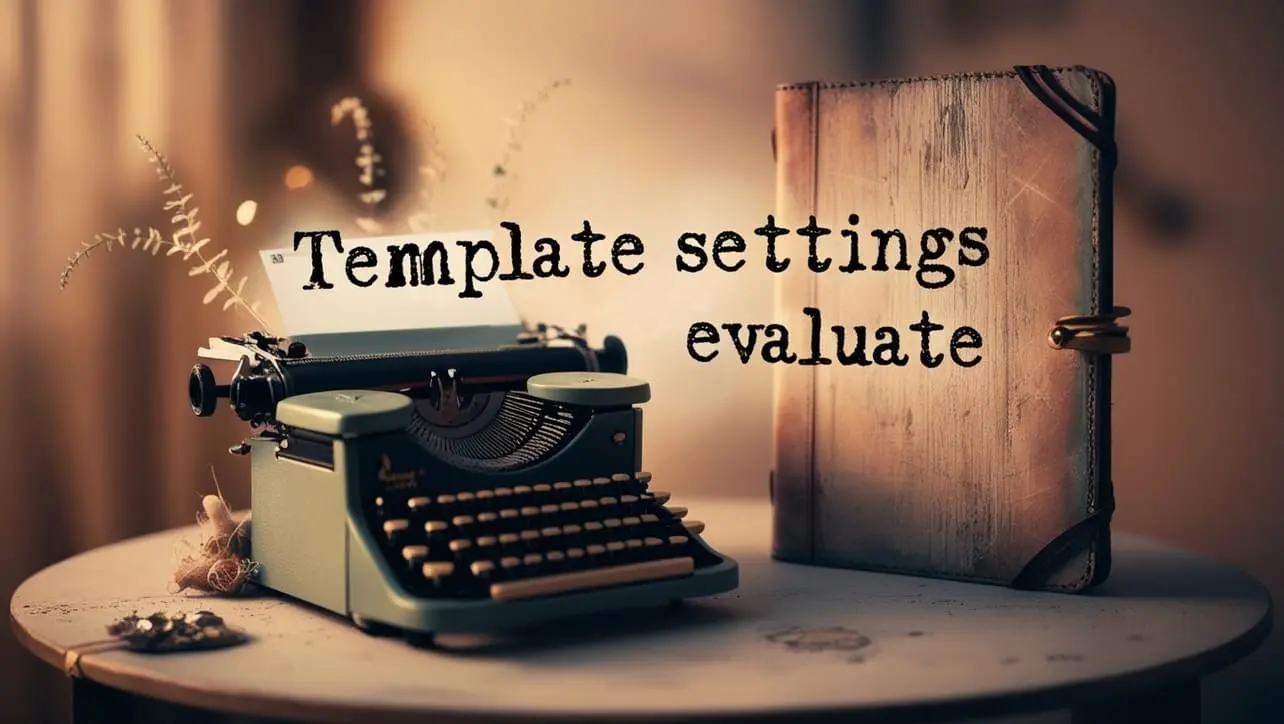
Lodash _.templateSettings.evaluate Property
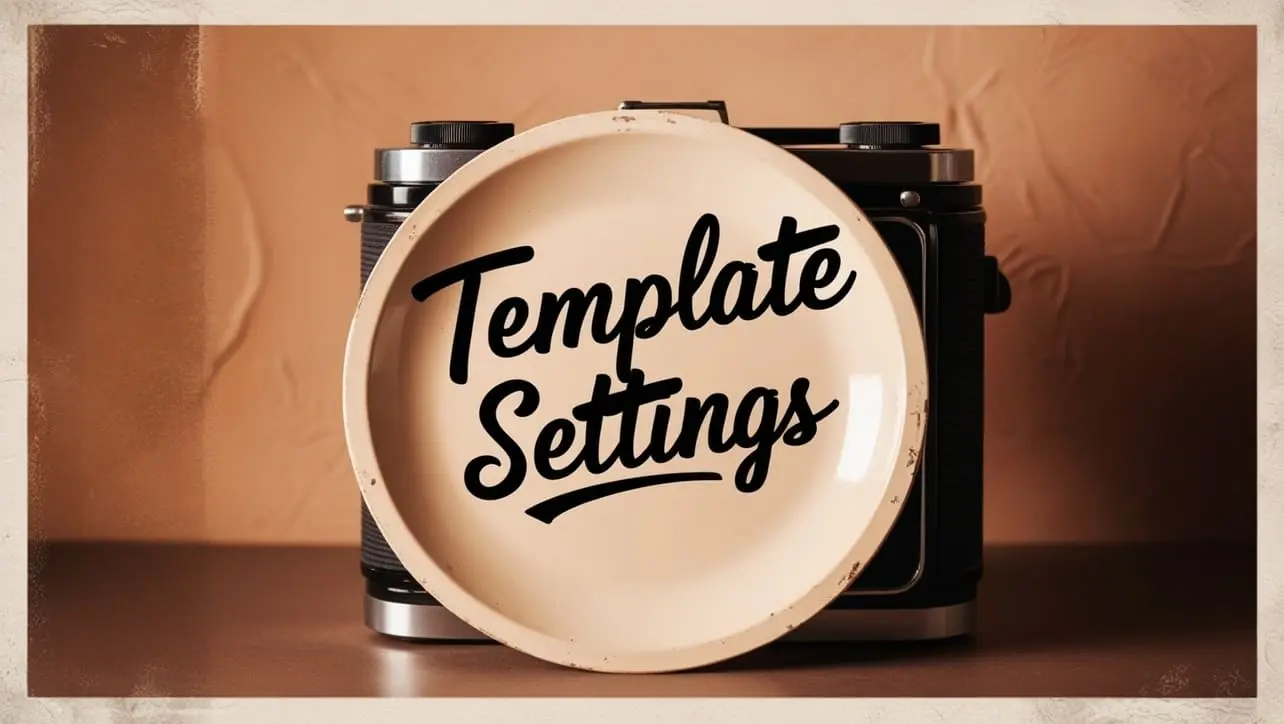
Lodash _.templateSettings Property
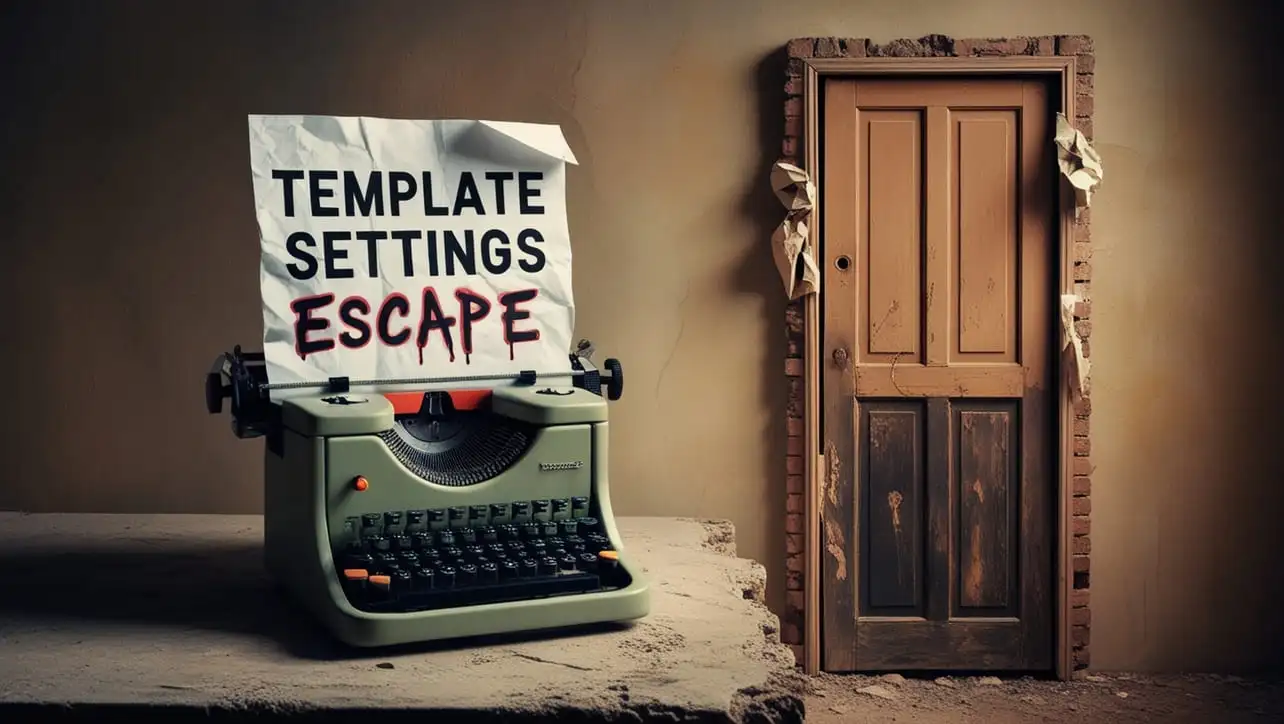
Lodash _.templateSettings.escape Property
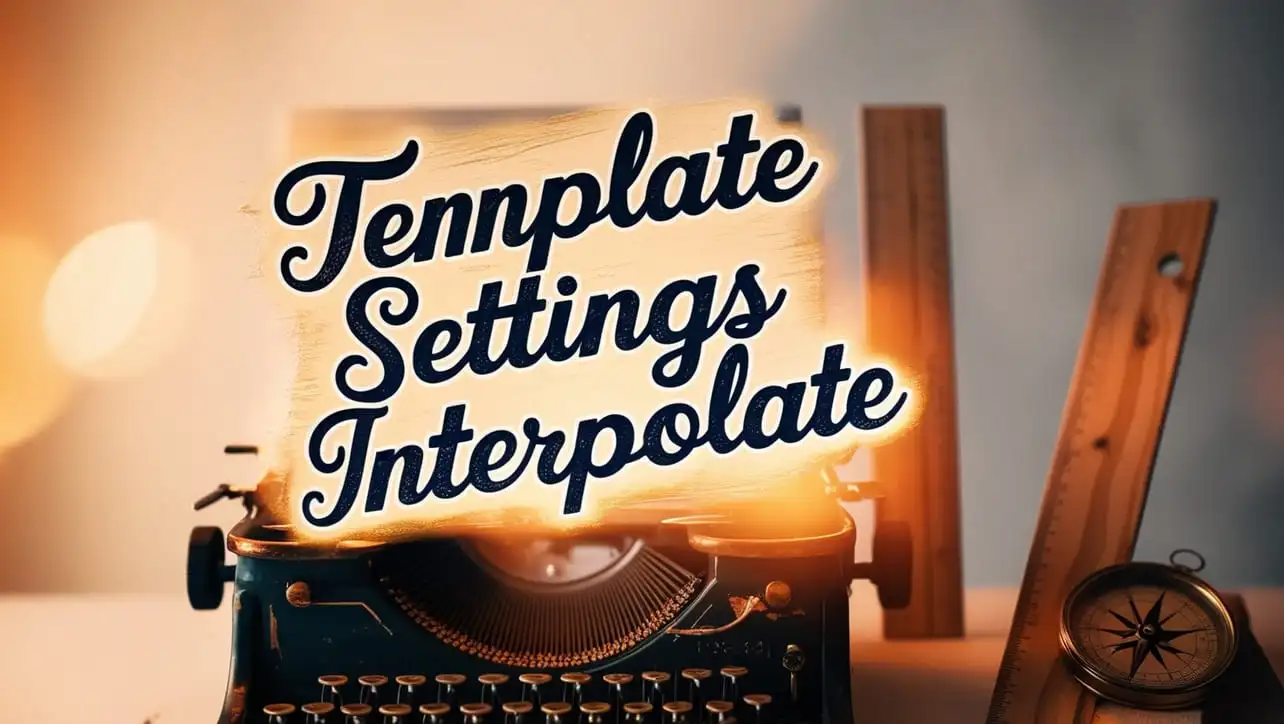
Lodash _.templateSettings.interpolate Property
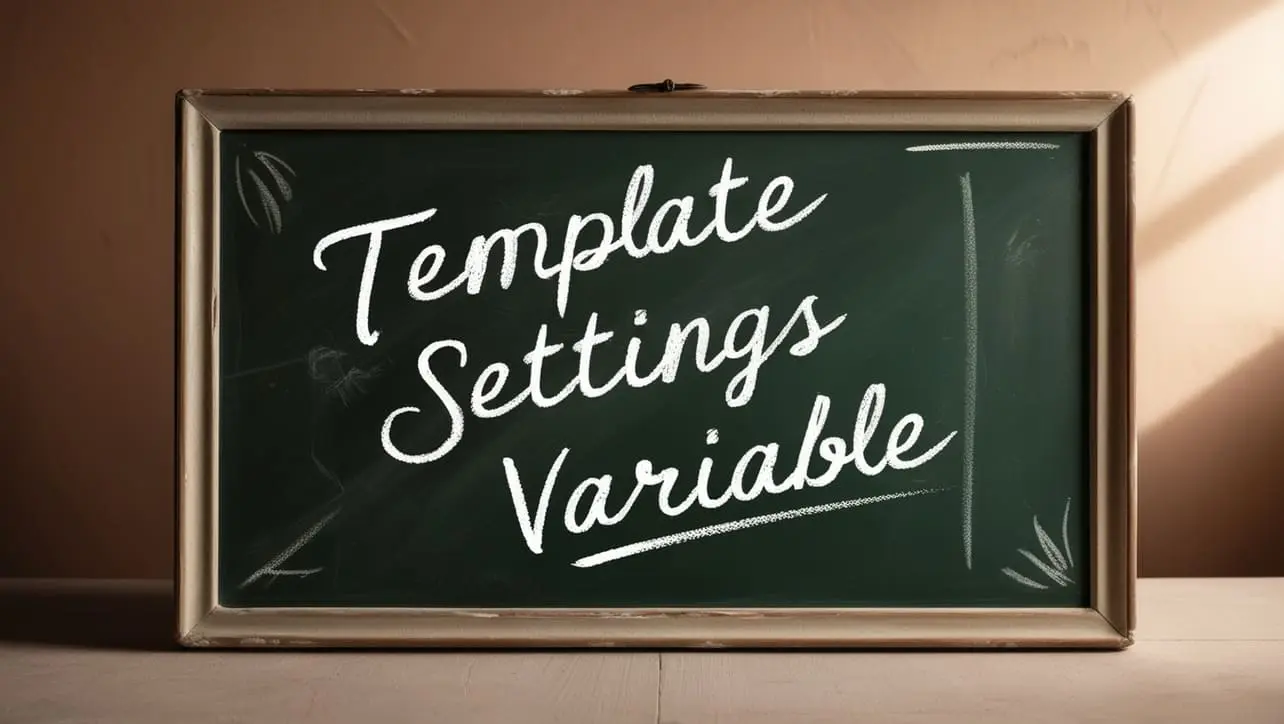
If you have any doubts regarding this article (Lodash _.propertyOf() Util Method), please comment here. I will help you immediately.