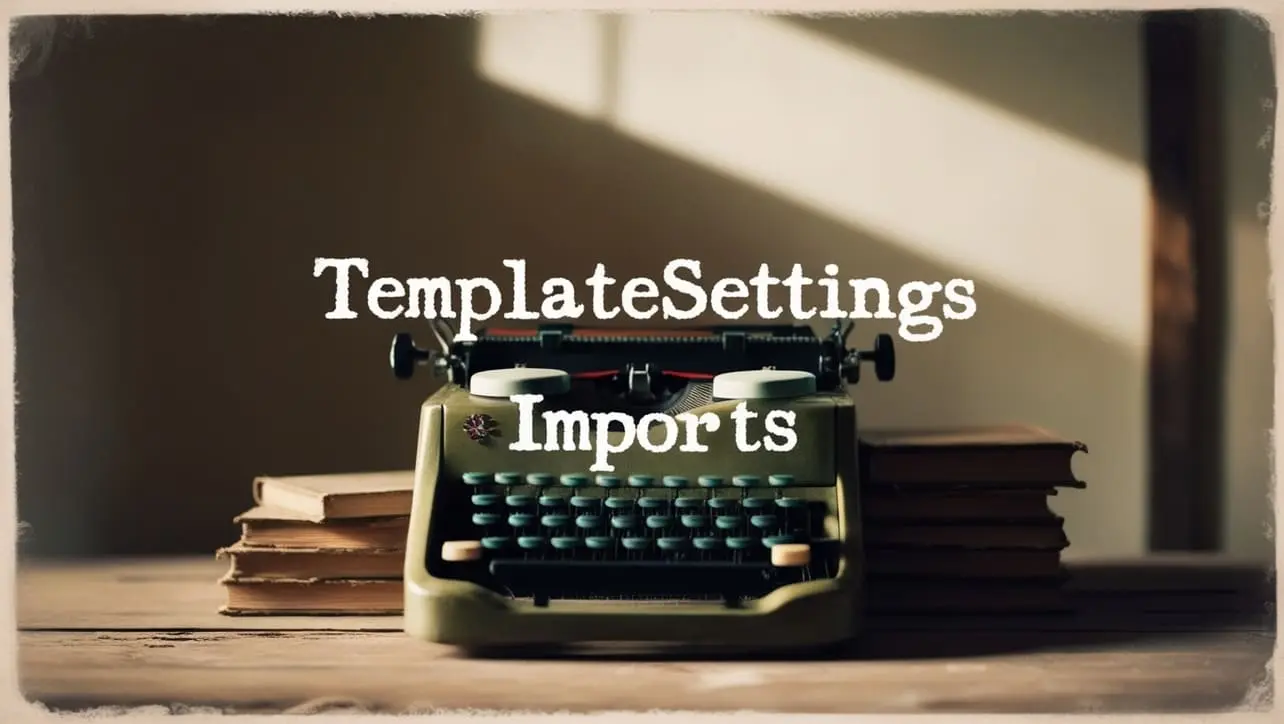
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.mixin() Util Method
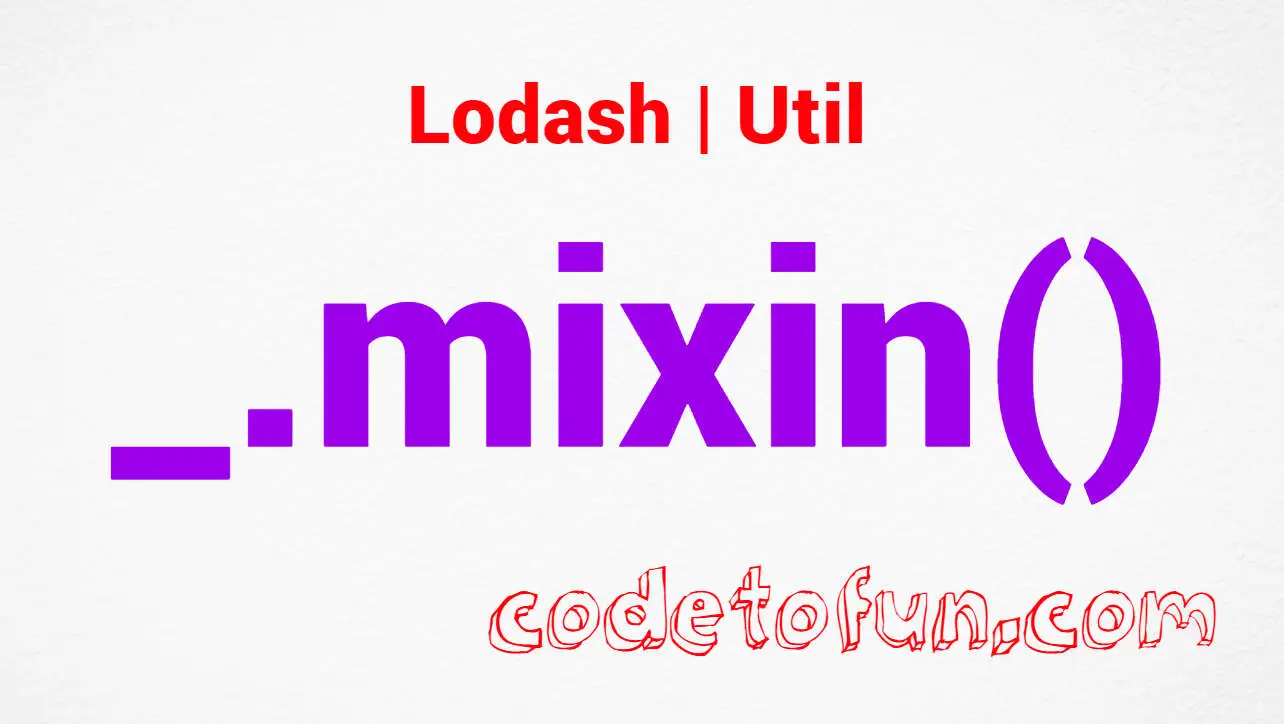
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, code reusability and modularity are key principles for writing clean and efficient code. Lodash, a popular utility library, provides a plethora of functions to simplify common programming tasks. Among its arsenal of features is the _.mixin()
method, which enables developers to extend Lodash with custom utility functions.
This empowers developers to create their own utility functions or integrate third-party libraries seamlessly into their projects.
🧠 Understanding _.mixin() Method
The _.mixin()
method in Lodash allows developers to add custom utility functions to the Lodash library, making them accessible alongside built-in Lodash functions. This facilitates code organization, promotes reusability, and enhances collaboration among developers by providing a centralized location for utility functions.
💡 Syntax
The syntax for the _.mixin()
method is straightforward:
_.mixin([object=lodash], source, [options])
- object (Optional): The object to extend.
- source: The source object containing the functions to merge.
- options (Optional): The options object.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.mixin()
method:
const _ = require('lodash');
_.mixin({
// Custom utility function to calculate the average of an array
average: function(array) {
if(!Array.isArray(array) || array.length === 0) return NaN;
return _.sum(array) / array.length;
}
});
const numbers = [5, 10, 15, 20];
const avg = _.average(numbers);
console.log(avg);
// Output: 12.5
In this example, we extend Lodash with a custom utility function called average, which calculates the average of an array. This function becomes available for use alongside other Lodash functions.
🏆 Best Practices
When working with the _.mixin()
method, consider the following best practices:
Modularity and Encapsulation:
Encapsulate related utility functions within a single source object when using
_.mixin()
. This promotes modularity and ensures that functions are organized logically for easy maintenance and understanding.example.jsCopied_.mixin({ math: { // Custom math utility functions square: function(x) { return x * x; }, cube: function(x) { return x * x * x; } }, string: { // Custom string utility functions capitalize: function(str) { return str.charAt(0).toUpperCase() + str.slice(1); } } });
Avoid Overwriting Existing Functions:
Exercise caution to avoid overwriting existing functions in Lodash or conflicting with functions from other libraries. Use descriptive function names and namespace your custom functions appropriately to minimize the risk of naming collisions.
example.jsCopied_.mixin({ // Avoid overwriting existing Lodash functions customFilter: function(array, predicate) { // Implementation... } });
Document Your Custom Functions:
Provide clear documentation and usage examples for your custom utility functions to facilitate collaboration and ensure ease of use for other developers working with your codebase.
example.jsCopied/** * Calculates the average of an array. * @param {Array} array The array of numbers. * @returns {number} The average value. */ _.mixin({ average: function(array) { if(!Array.isArray(array) || array.length === 0) return NaN; return _.sum(array) / array.length; } });
📚 Use Cases
Domain-Specific Utility Functions:
Create custom utility functions tailored to specific domains or business requirements. These functions can encapsulate domain logic and simplify complex operations within your application.
example.jsCopied_.mixin({ // Custom utility functions for financial calculations financial: { calculateInterest: function(principal, rate, time) { // Implementation... }, calculateROI: function(initialInvestment, finalValue) { // Implementation... } } });
Integration with Third-Party Libraries:
Integrate utility functions from third-party libraries seamlessly into your Lodash ecosystem using
_.mixin()
. This allows you to leverage the functionality of external libraries alongside native Lodash functions.example.jsCopiedconst moment = require('moment'); _.mixin({ date: { // Custom utility functions for date manipulation using Moment.js formatDate: function(date, format) { return moment(date).format(format); }, // More custom date functions... } });
Standardization of Common Operations:
Standardize common operations or algorithms across your codebase by defining them as custom utility functions with
_.mixin()
. This promotes consistency and reduces code duplication.example.jsCopied_.mixin({ algorithms: { // Custom utility functions for common algorithms binarySearch: function(array, target) { // Implementation... }, // More custom algorithm functions... } });
🎉 Conclusion
The _.mixin()
method in Lodash serves as a powerful mechanism for extending the functionality of the library with custom utility functions. By leveraging _.mixin()
, developers can promote code reusability, encapsulation, and collaboration within their projects. Whether creating domain-specific utilities, integrating third-party libraries, or standardizing common operations, _.mixin()
empowers developers to enhance the capabilities of Lodash to suit their unique requirements.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.mixin()
method in your Lodash projects.
👨💻 Join our Community:
Author
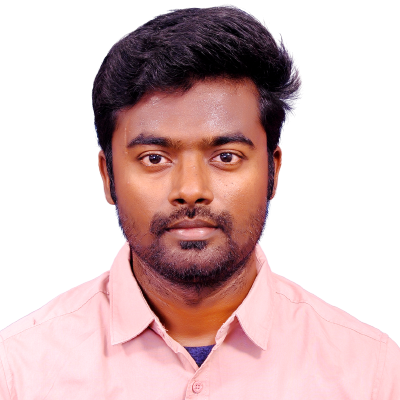
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
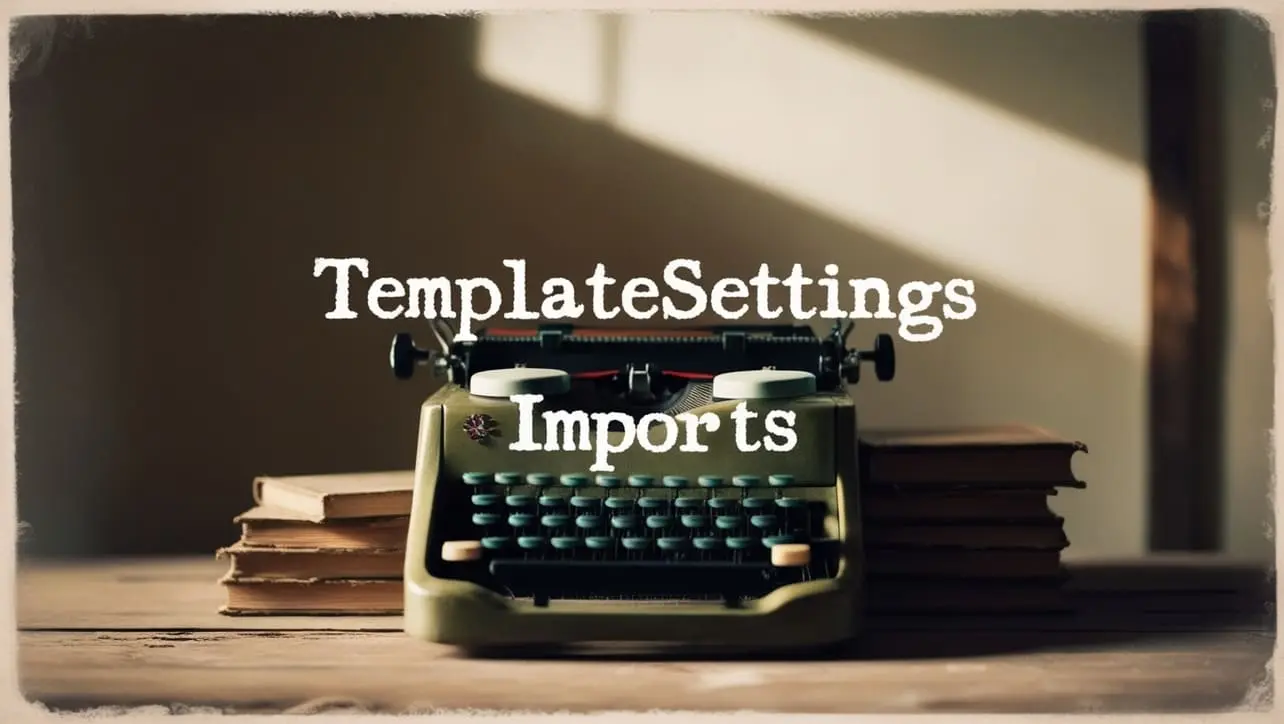
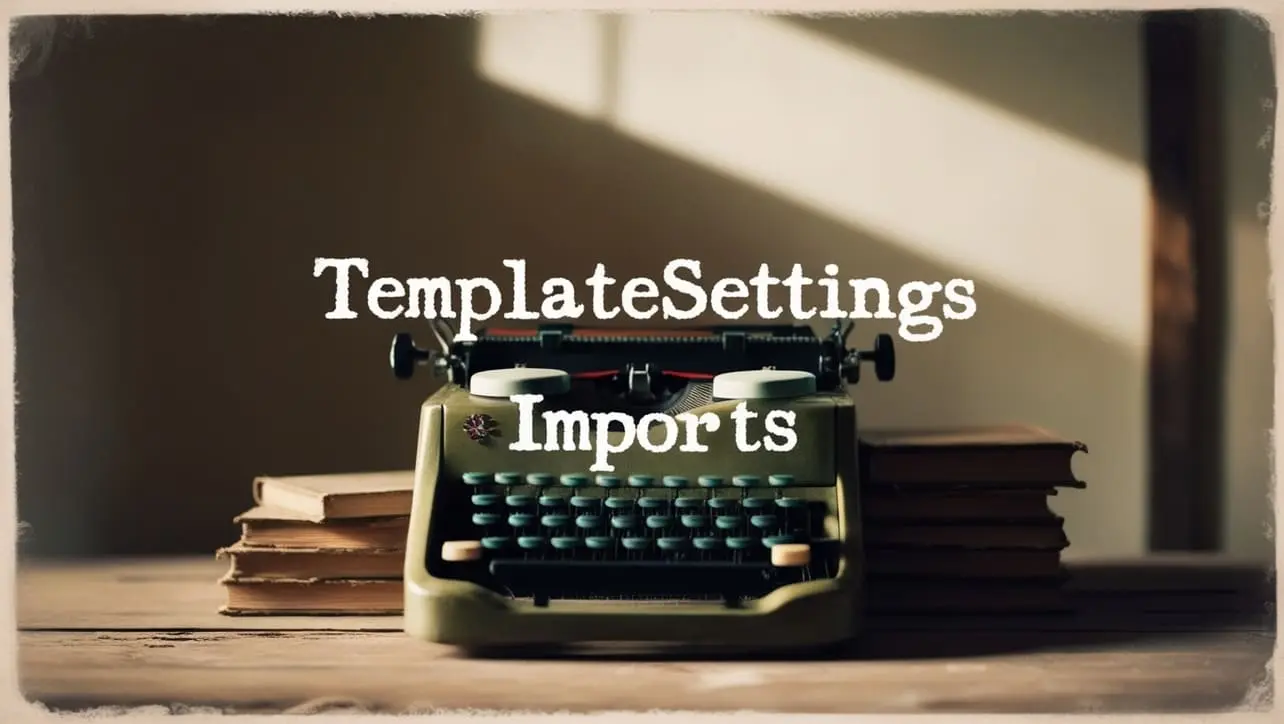
Lodash _.templateSettings.imports Property
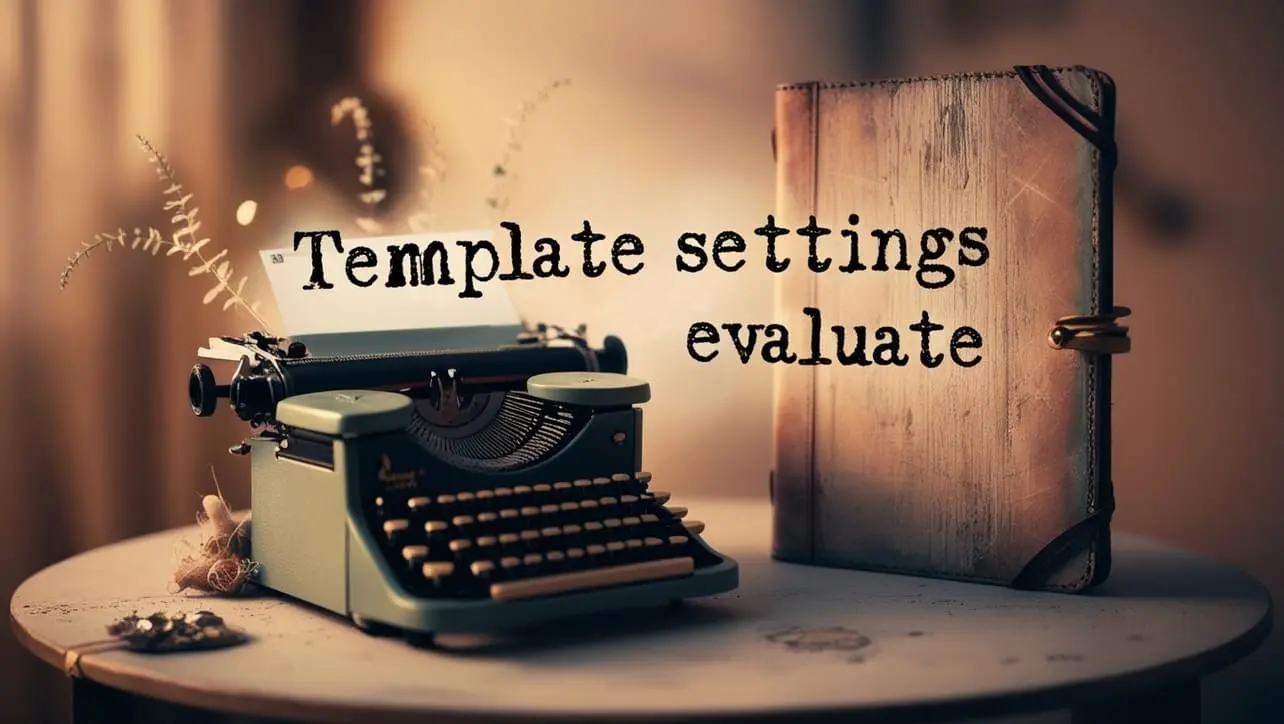
Lodash _.templateSettings.evaluate Property
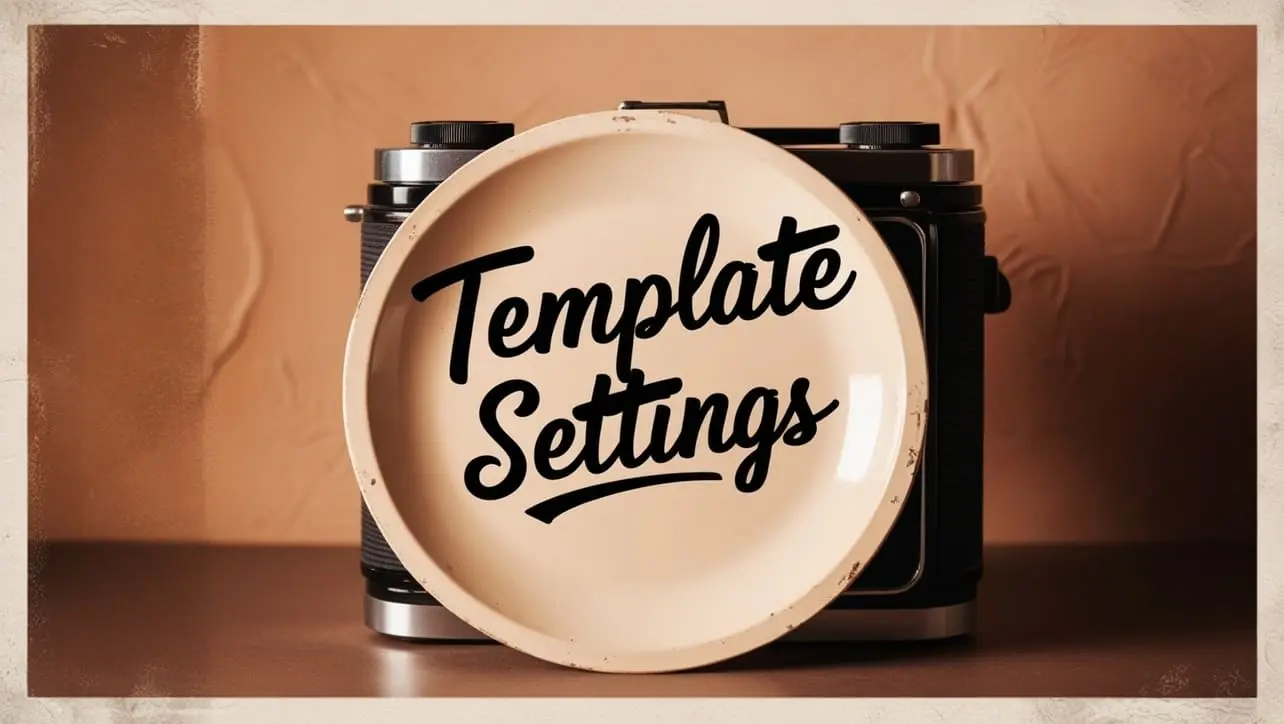
Lodash _.templateSettings Property
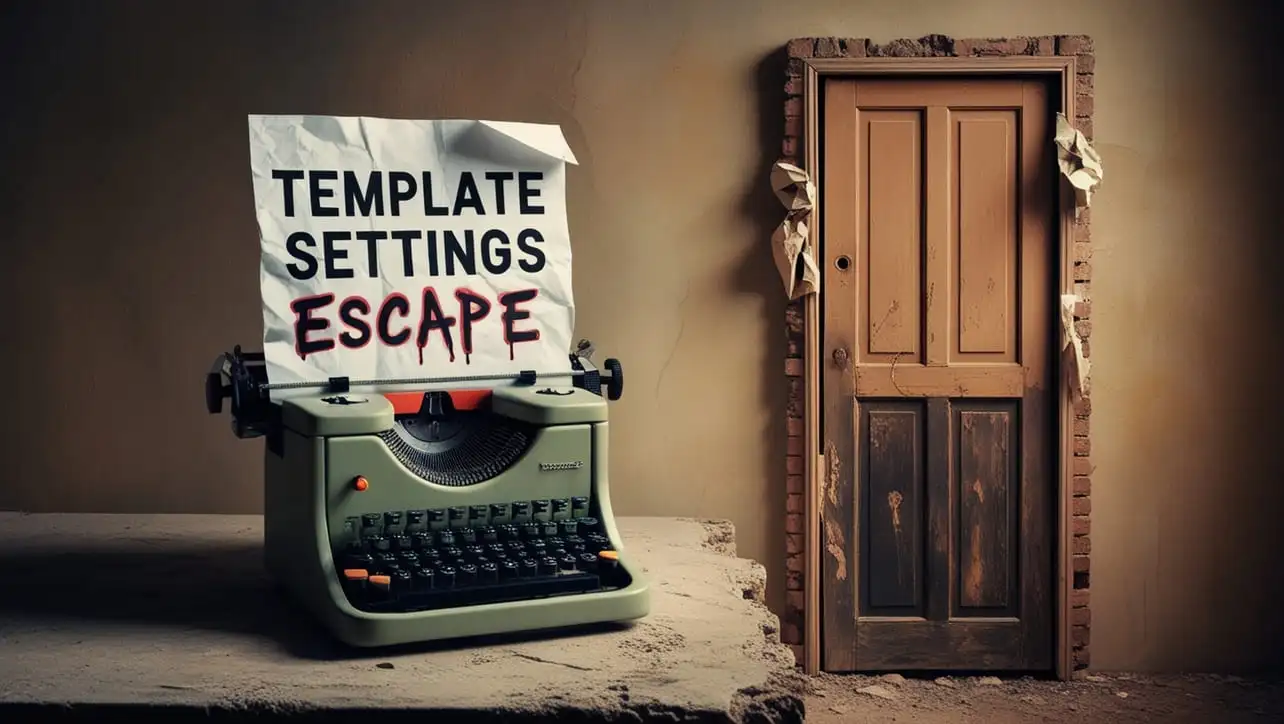
Lodash _.templateSettings.escape Property
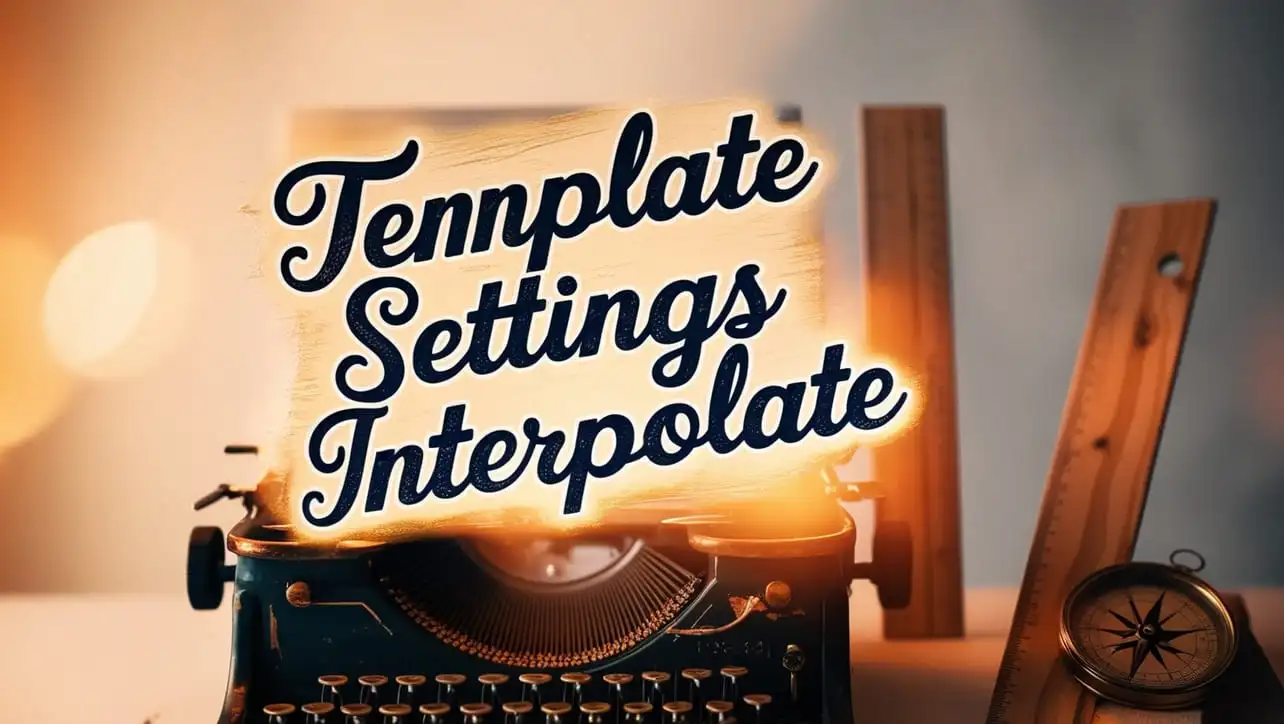
Lodash _.templateSettings.interpolate Property
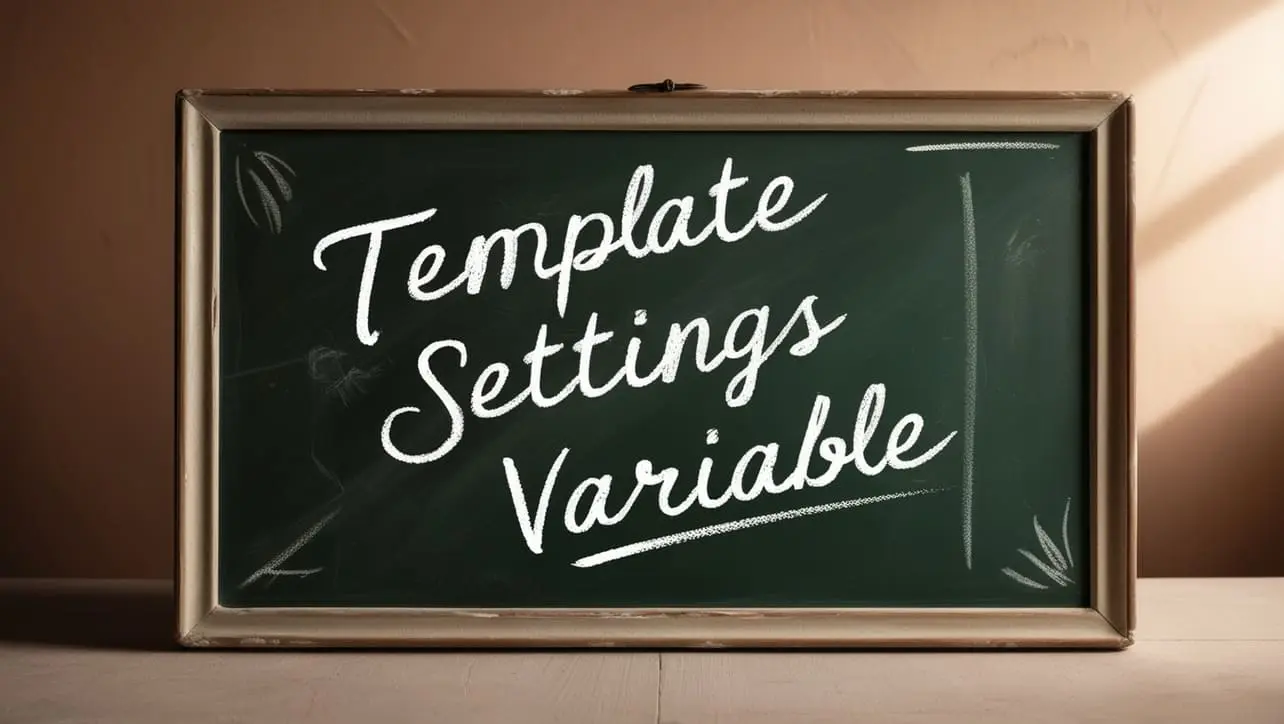
If you have any doubts regarding this article (Lodash _.mixin() Util Method), please comment here. I will help you immediately.