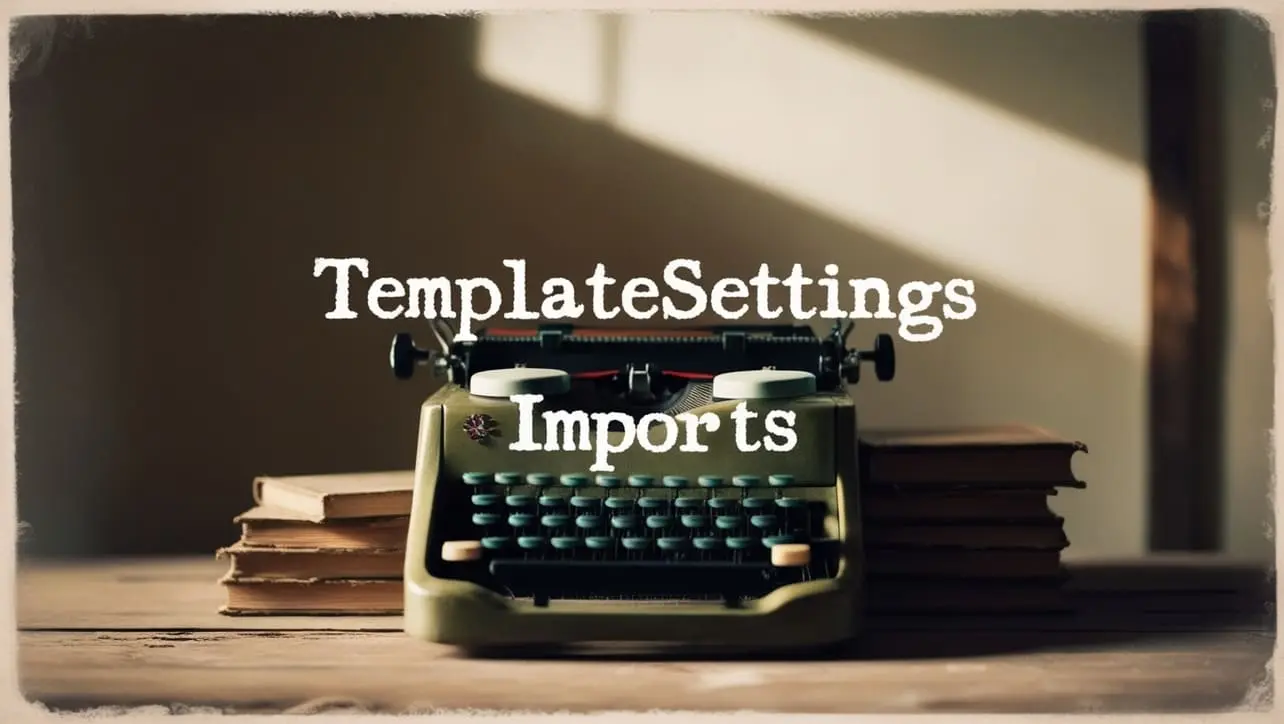
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.methodOf() Util Method
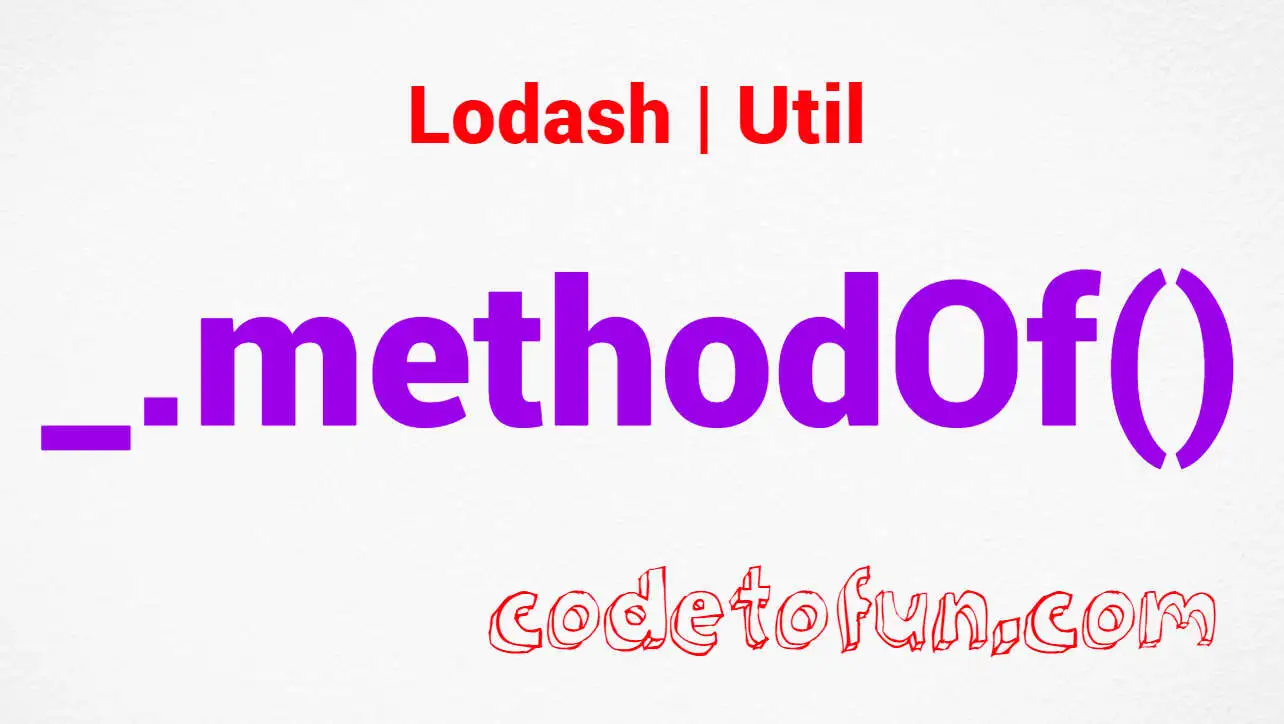
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript development, efficient manipulation of objects and functions is essential. Lodash, a comprehensive utility library, offers a plethora of functions to simplify common tasks. Among these functions is _.methodOf()
, a versatile utility method that allows for dynamic invocation of object methods.
This method provides flexibility and convenience in handling object-oriented programming paradigms.
🧠 Understanding _.methodOf() Method
The _.methodOf()
method in Lodash facilitates the dynamic invocation of methods belonging to an object. By specifying the object and method name as arguments, developers can execute functions within the context of the provided object. This enables streamlined access to object methods, enhancing code modularity and readability.
💡 Syntax
The syntax for the _.methodOf()
method is straightforward:
_.methodOf(object, methodName)
- object: The object containing the method to invoke.
- methodName: The name of the method to invoke.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.methodOf()
method:
const _ = require('lodash');
const user = {
name: 'John',
greet() {
return `Hello, ${this.name}!`;
}
};
const greetingFunction = _.methodOf(user, 'greet');
const greeting = greetingFunction();
console.log(greeting);
// Output: Hello, John!
In this example, _.methodOf()
is used to dynamically invoke the greet() method belonging to the user object, resulting in the expected greeting message.
🏆 Best Practices
When working with the _.methodOf()
method, consider the following best practices:
Validate Input Objects:
Before using
_.methodOf()
, ensure that the input object contains the desired method. Performing validation checks can prevent runtime errors and enhance code robustness.example.jsCopiedconst car = { brand: 'Toyota', startEngine() { console.log('Engine started.'); } }; if (_.has(car, 'startEngine')) { const startCar = _.methodOf(car, 'startEngine'); startCar(); } else { console.error('Method not found.'); }
Handle Context Appropriately:
Consider the context in which the method will be executed. Ensure that the context (this binding) is preserved or set appropriately to avoid unexpected behavior.
example.jsCopiedconst calculator = { value: 0, add(num) { this.value += num; return this.value; } }; const addFive = _.methodOf(calculator, 'add'); // Explicitly set context to calculator object const result = addFive.call(calculator, 5); console.log(result); // Output: 5
Encapsulate Logic:
Encapsulate complex or repetitive logic within methods of objects.
_.methodOf()
can then be used to invoke these methods dynamically, promoting code reusability and maintainability.example.jsCopiedconst mathUtils = { square(num) { return num * num; }, cube(num) { return num * num * num; } }; const calculate = (operation, num) => { const method = _.methodOf(mathUtils, operation); return method(num); }; console.log(calculate('square', 3)); // Output: 9 console.log(calculate('cube', 3)); // Output: 27
📚 Use Cases
Dynamic Function Execution:
_.methodOf()
is particularly useful for scenarios where the method to be executed is determined dynamically at runtime. This enables flexible and dynamic function invocation.example.jsCopiedconst objectWithMethods = { method1() { console.log('Method 1 executed.'); }, method2() { console.log('Method 2 executed.'); } }; const methodName = /* ...dynamically determine method name... */ ; const dynamicMethod = _.methodOf(objectWithMethods, methodName); dynamicMethod();
Function Composition:
In function composition patterns,
_.methodOf()
can be employed to compose complex behaviors by dynamically invoking individual functions within the composition.example.jsCopiedconst composeFunctions = (f, g) => _.flow(_.methodOf(window, f), _.methodOf(window, g)); const composedFunction = composeFunctions('alert', 'confirm'); composedFunction('Hello');
Method Delegation:
When delegating method calls between objects or modules,
_.methodOf()
can facilitate the seamless invocation of methods within different contexts.example.jsCopiedconst moduleA = { methodA() { console.log('Method A executed.'); } }; const moduleB = { methodB() { console.log('Method B executed.'); } }; const delegateMethod = (moduleName, methodName) => { const module = /* ...retrieve module dynamically... */ ; const method = _.methodOf(module, methodName); return method.call(module); }; delegateMethod('moduleA', 'methodA'); delegateMethod('moduleB', 'methodB');
🎉 Conclusion
The _.methodOf()
method in Lodash offers a convenient solution for dynamically invoking object methods. Whether you're dealing with dynamic function execution, function composition, or method delegation, this utility method provides flexibility and versatility in JavaScript programming.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.methodOf()
method in your Lodash projects.
👨💻 Join our Community:
Author
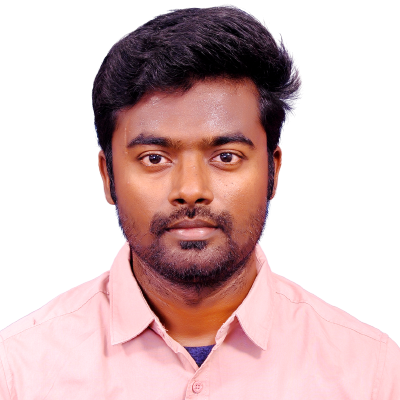
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
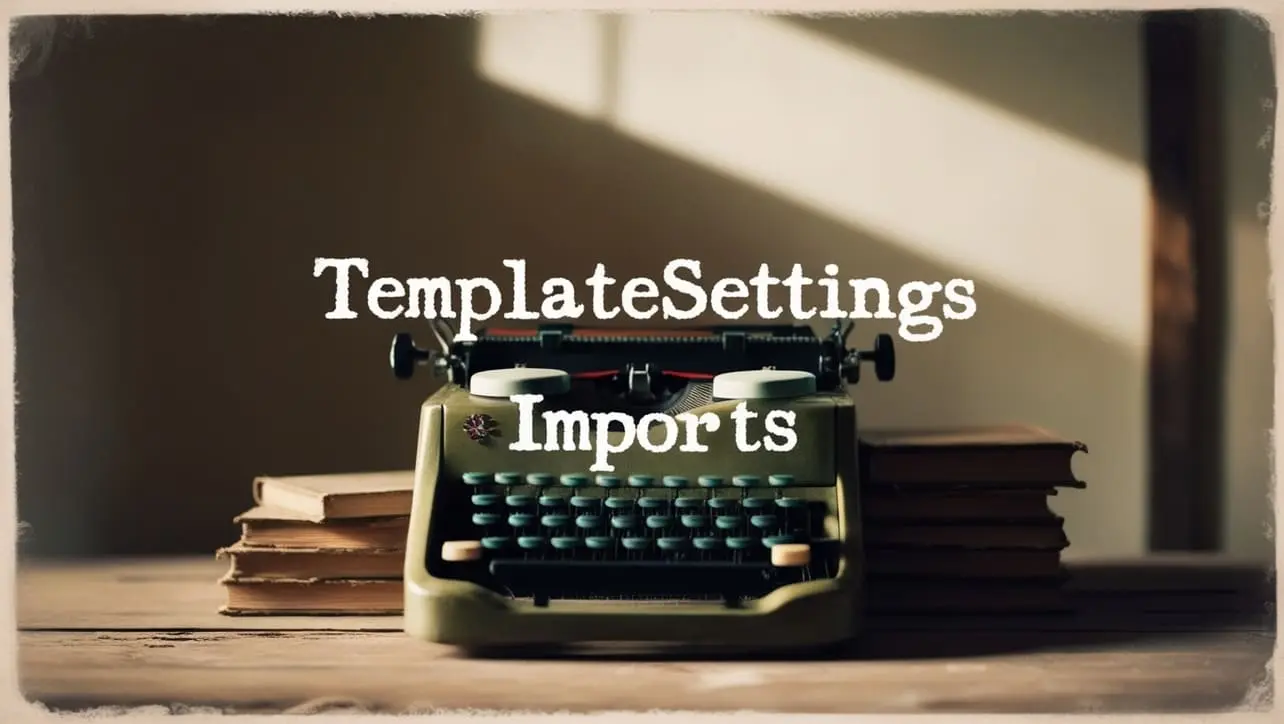
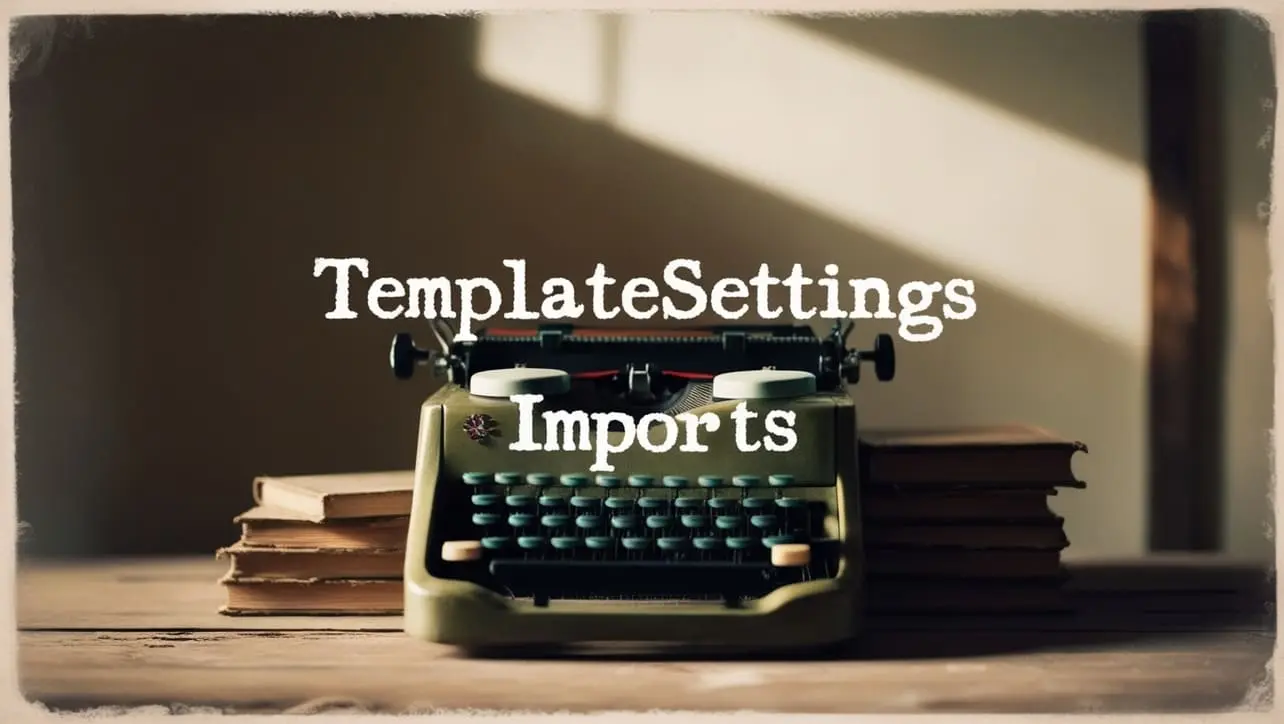
Lodash _.templateSettings.imports Property
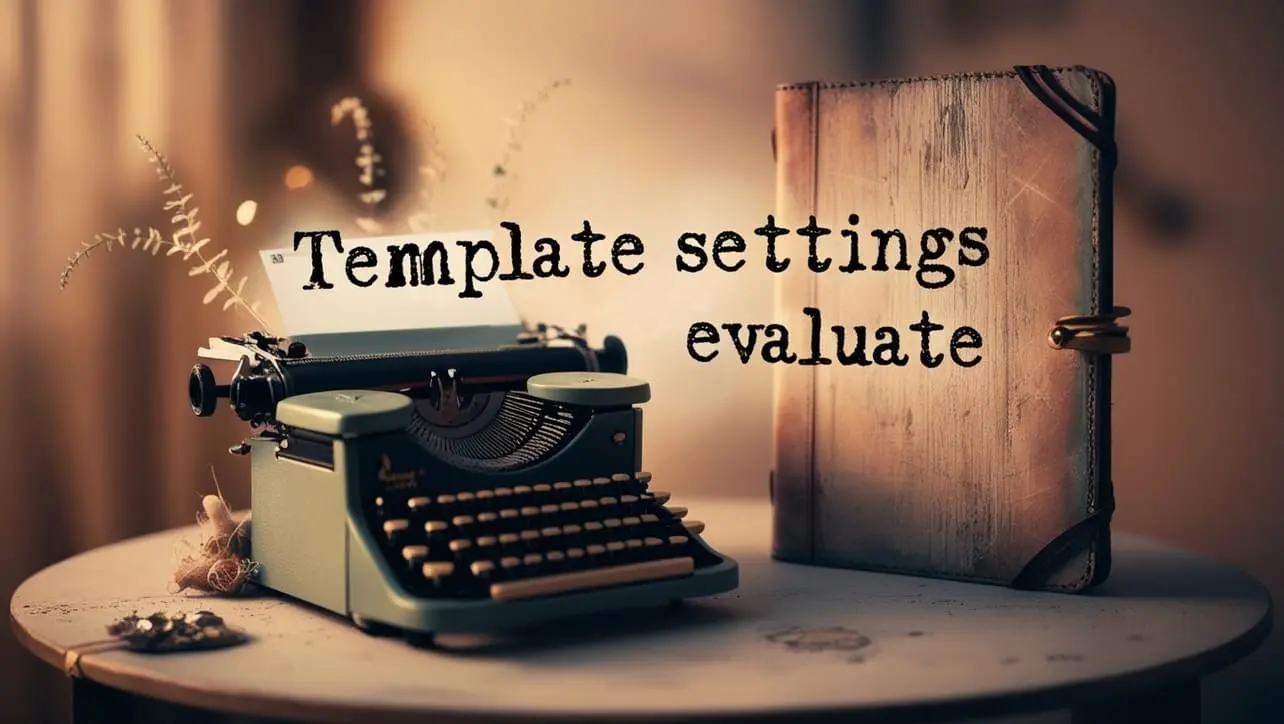
Lodash _.templateSettings.evaluate Property
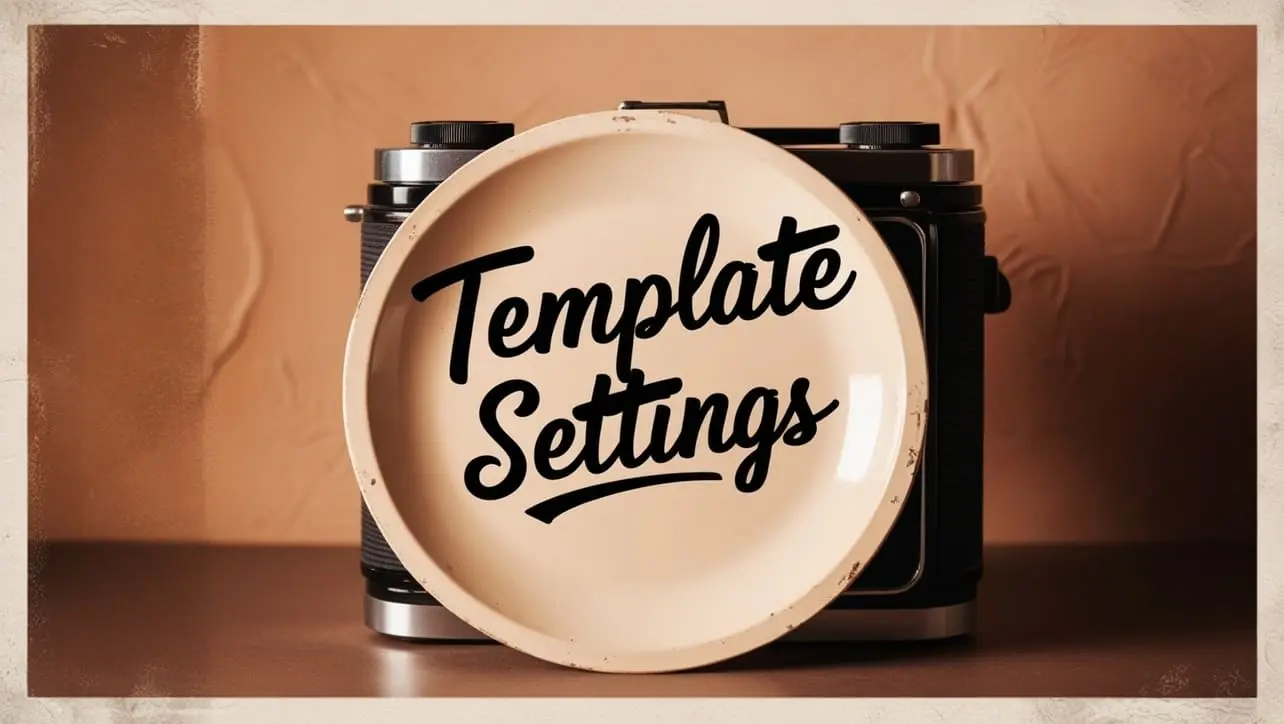
Lodash _.templateSettings Property
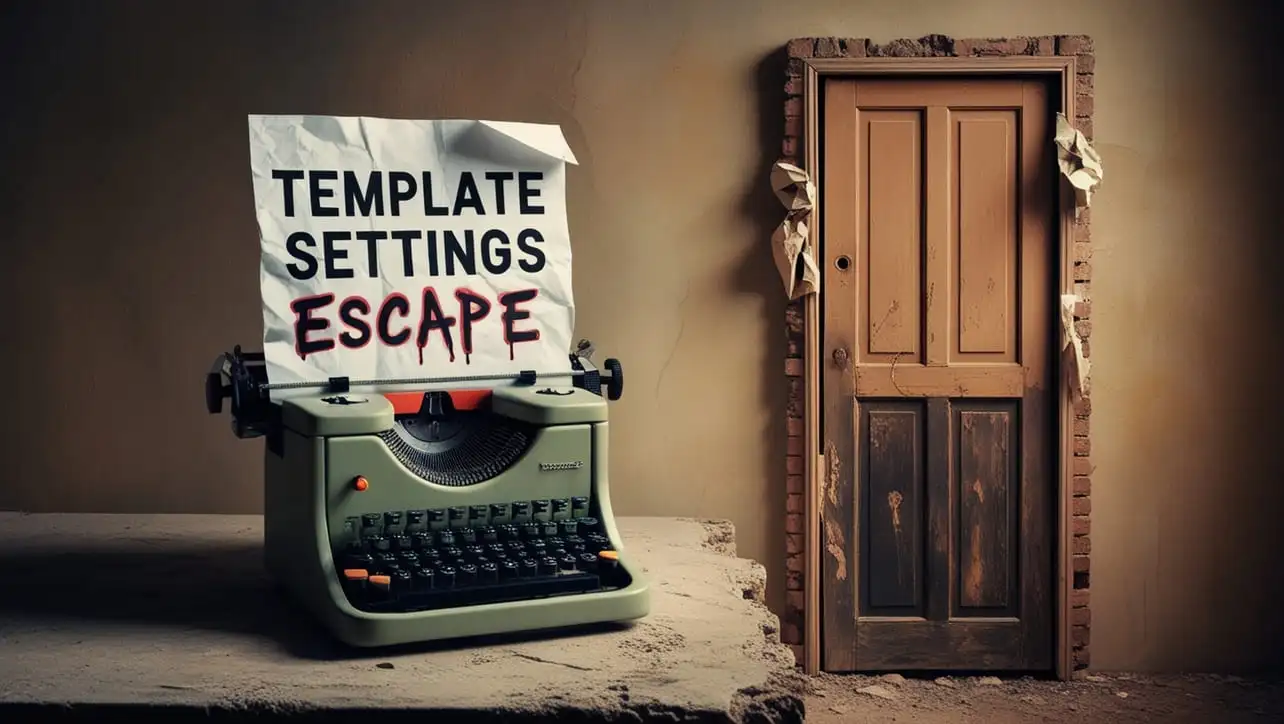
Lodash _.templateSettings.escape Property
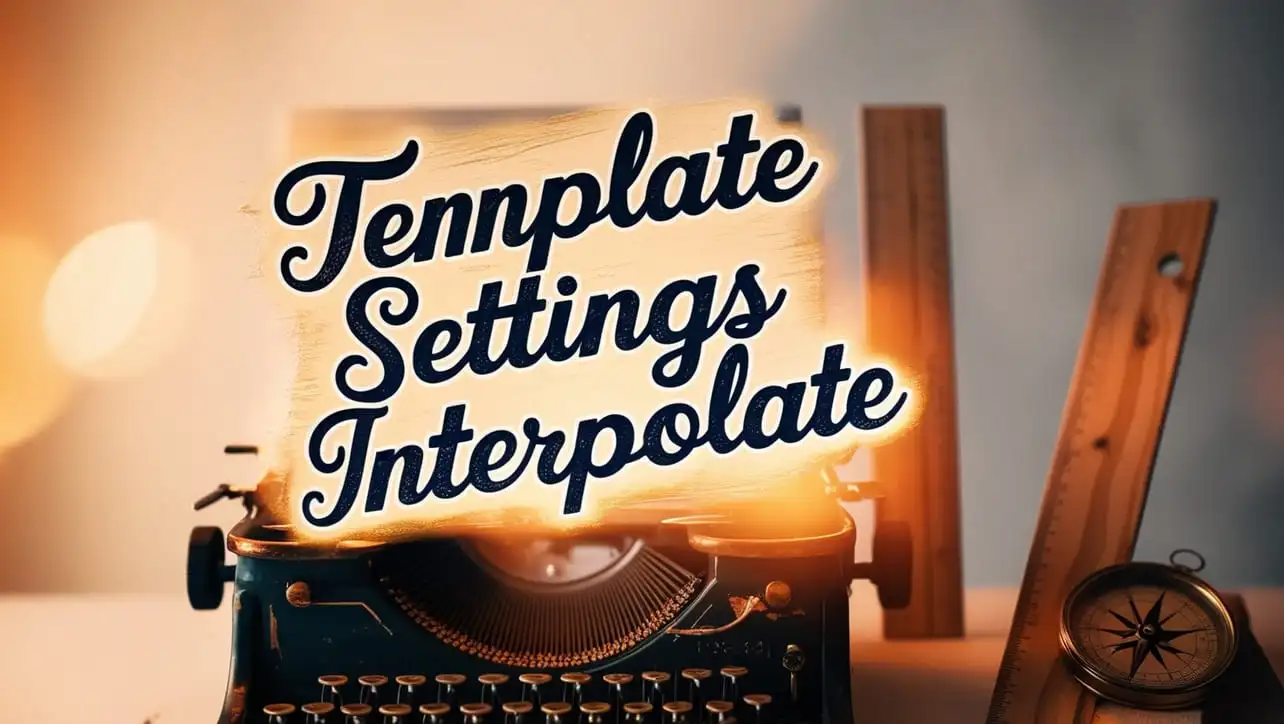
Lodash _.templateSettings.interpolate Property
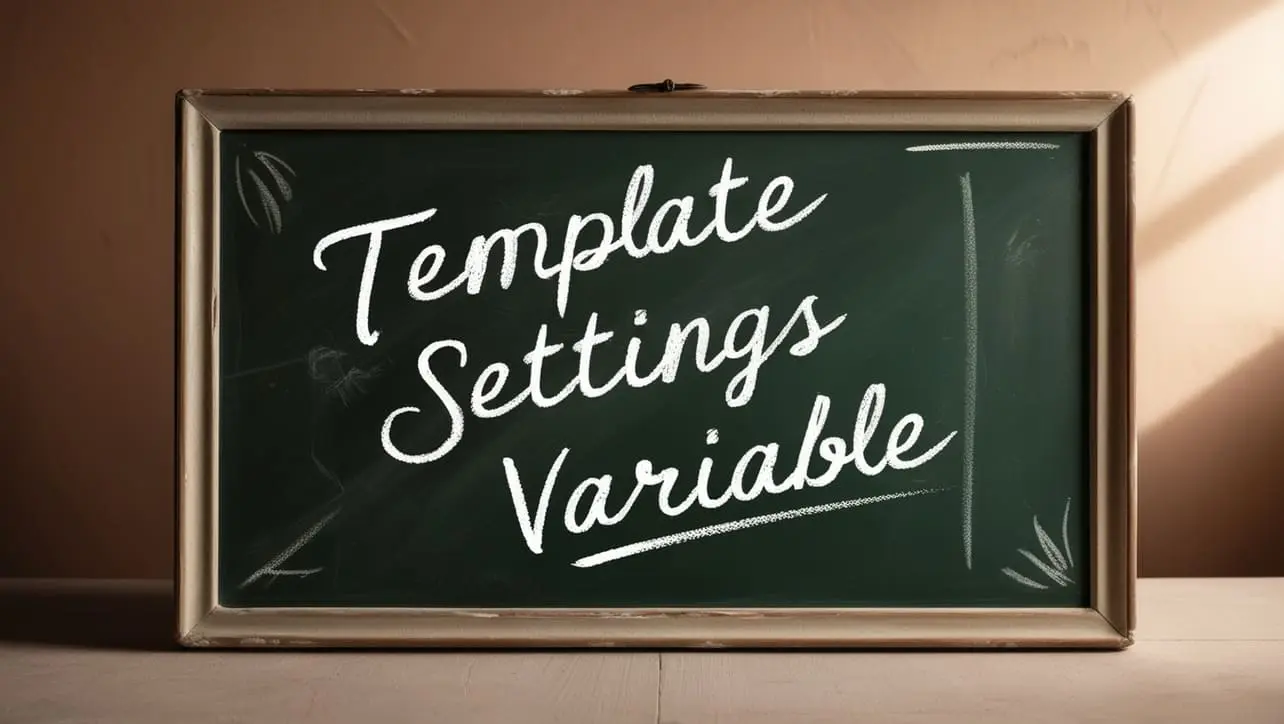
If you have any doubts regarding this article (Lodash _.methodOf() Util Method), please comment here. I will help you immediately.