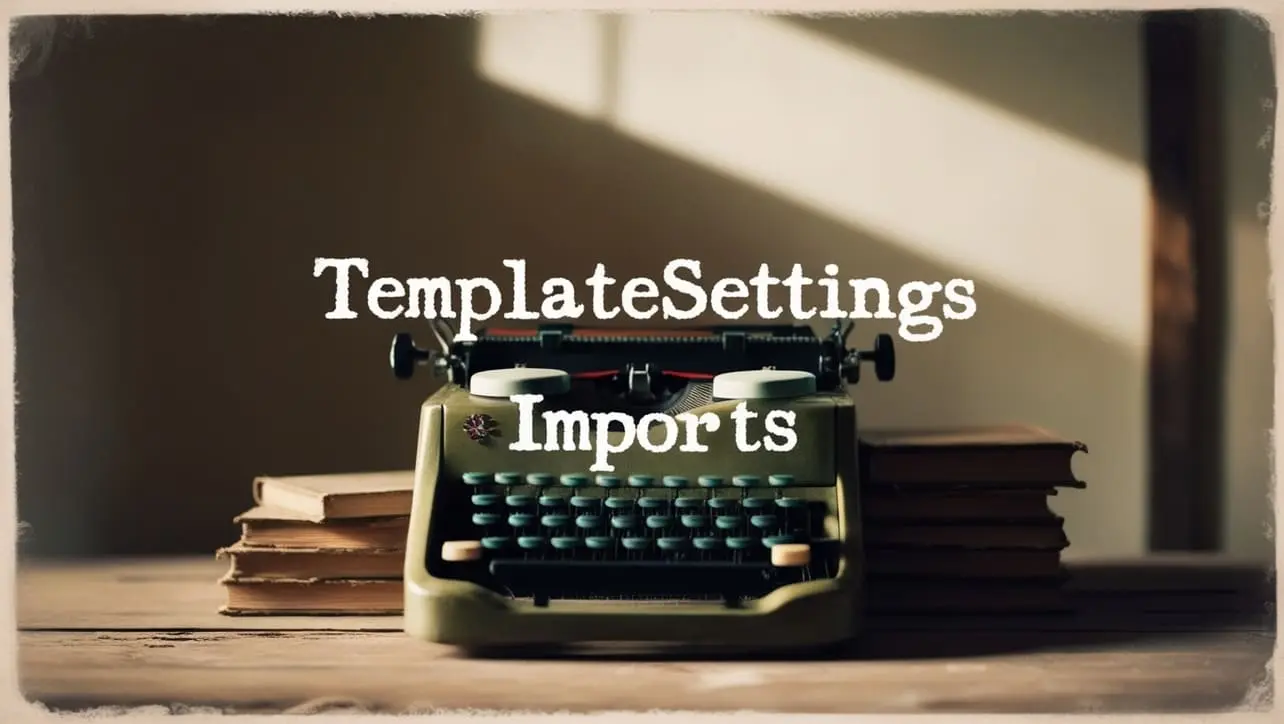
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.method() Util Method
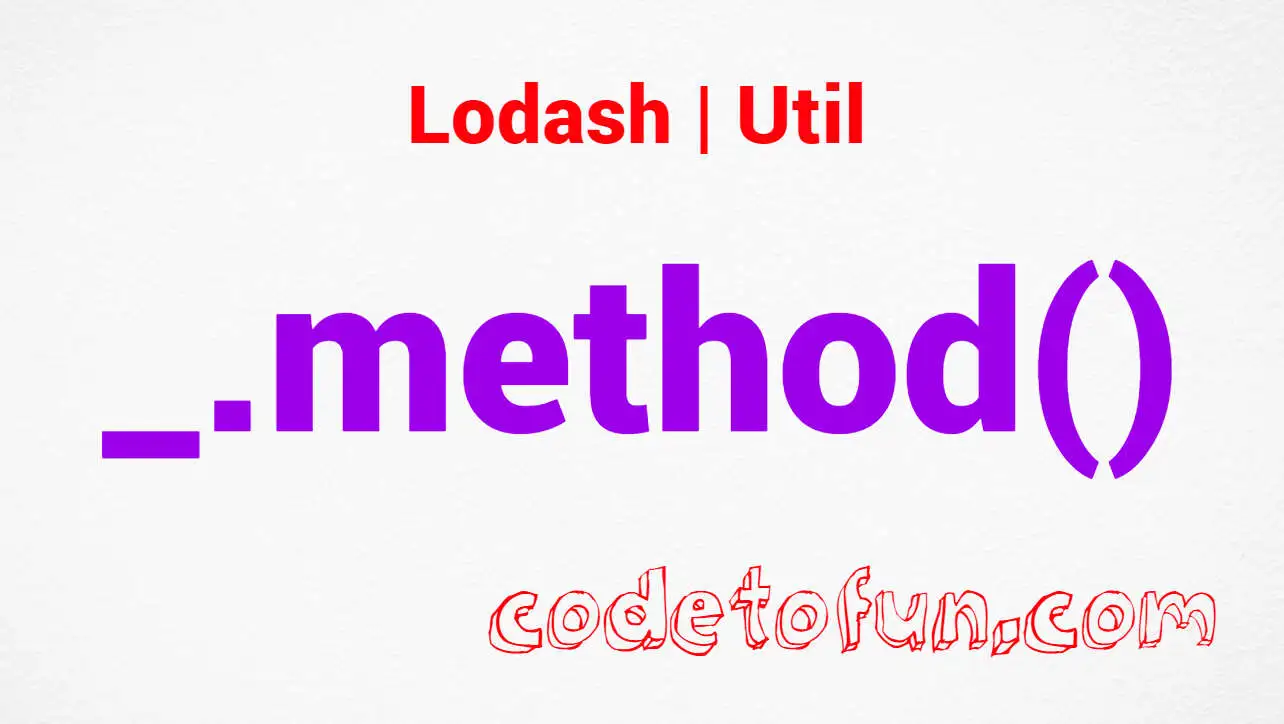
Photo Credit to CodeToFun
🙋 Introduction
Lodash is renowned for its comprehensive set of utility functions that streamline JavaScript development. Among its arsenal of tools is the _.method()
method, a versatile utility that generates a function for invoking the specified method on a provided object.
This method empowers developers to dynamically access object methods, offering flexibility and efficiency in code execution.
🧠 Understanding _.method() Method
The _.method()
utility method in Lodash creates a function that invokes the specified method on a given object. This function generation enables dynamic invocation of object methods, facilitating cleaner and more concise code.
💡 Syntax
The syntax for the _.method()
method is straightforward:
_.method(path, [args])
- path: The path of the method to invoke.
- args (Optional): The arguments to invoke the method with.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.method()
method:
const _ = require('lodash');
const obj = {
greet: function(name) {
return `Hello, ${name}!`;
}
};
const greetMethod = _.method('greet');
const greeting = greetMethod(obj, 'John');
console.log(greeting);
// Output: Hello, John!
In this example, _.method()
generates a function greetMethod that invokes the greet method on the obj object with the provided argument John, resulting in the greeting message.
🏆 Best Practices
When working with the _.method()
method, consider the following best practices:
Object Method Invocation:
Utilize
_.method()
for dynamically invoking object methods, especially when the method name is determined at runtime. This promotes code reusability and flexibility in method invocation.example.jsCopiedconst obj = { add: function(a, b) { return a + b; }, subtract: function(a, b) { return a - b; } }; const operation = '+'; const method = operation === '+' ? 'add' : 'subtract'; const mathFunc = _.method(method); const result = mathFunc(obj, 10, 5); console.log(result); // Output: 15
Error Handling:
Ensure proper error handling when using
_.method()
to handle scenarios where the specified method does not exist on the object. Implement fallback mechanisms or validation checks to prevent runtime errors.example.jsCopiedconst obj = { // Only 'greet' method is defined greet: function(name) { return `Hello, ${name}!`; } }; const unknownMethod = _.method('unknownMethod'); const result = unknownMethod(obj); if(typeof result === 'function') { console.log('Method exists but was not invoked.'); } else { console.error('Method does not exist.'); }
📚 Use Cases
Dynamic Method Invocation:
_.method()
is ideal for scenarios where the method to invoke is determined dynamically at runtime. This enables flexible and dynamic code execution, especially in situations where method names vary based on external factors.example.jsCopiedconst user = { // Method name determined dynamically greet: function(name) { return `Hello, ${name}!`; } }; const methodName = /* ...determine method name dynamically... */ ; const dynamicMethod = _.method(methodName); const greeting = dynamicMethod(user, 'Alice'); console.log(greeting); // Output: Hello, Alice!
Simplifying Method Invocation:
By generating functions for method invocation,
_.method()
simplifies the process of accessing and invoking object methods, promoting cleaner and more readable code.example.jsCopiedconst calculator = { add: function(a, b) { return a + b; }, subtract: function(a, b) { return a - b; } }; const addFunc = _.method('add'); const result = addFunc(calculator, 10, 5); console.log(result); // Output: 15
🎉 Conclusion
The _.method()
utility method in Lodash provides a convenient solution for dynamically invoking object methods with ease. By generating functions for method invocation, this utility enhances code flexibility and promotes efficient JavaScript development.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.method()
method in your Lodash projects.
👨💻 Join our Community:
Author
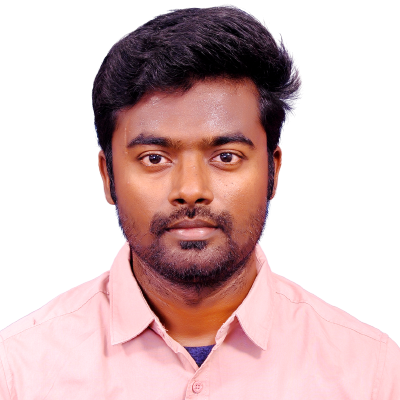
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
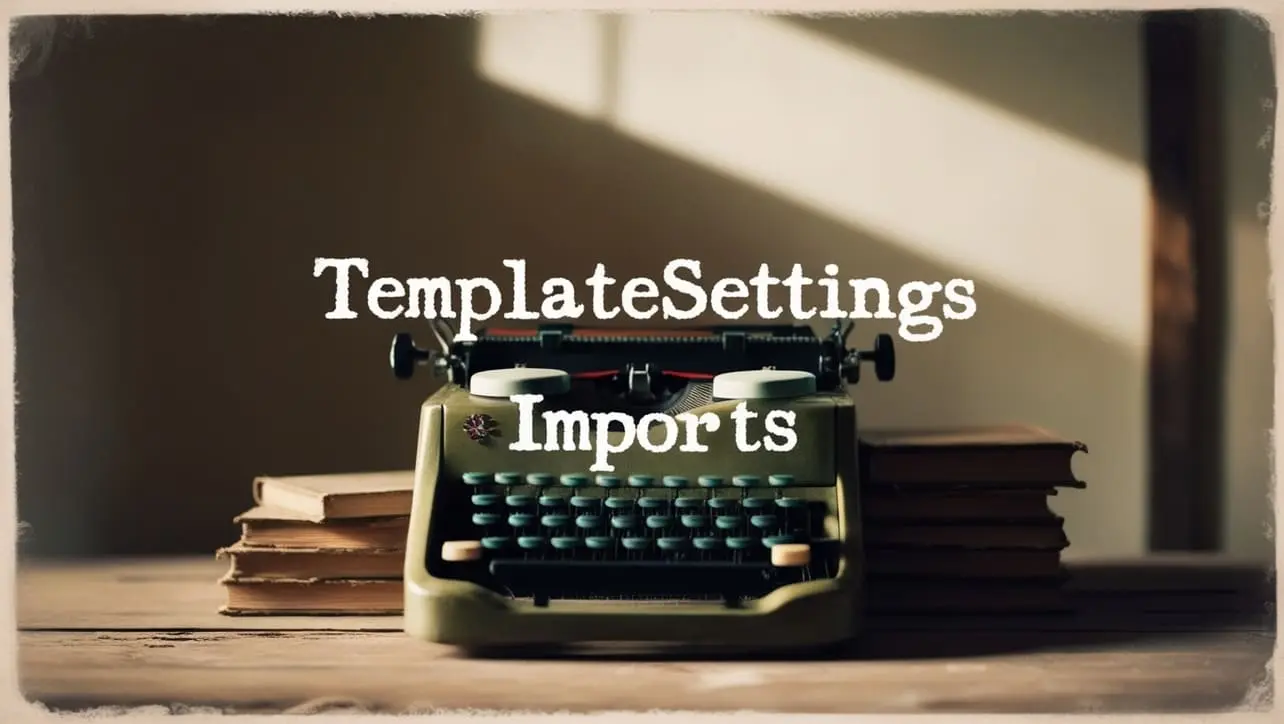
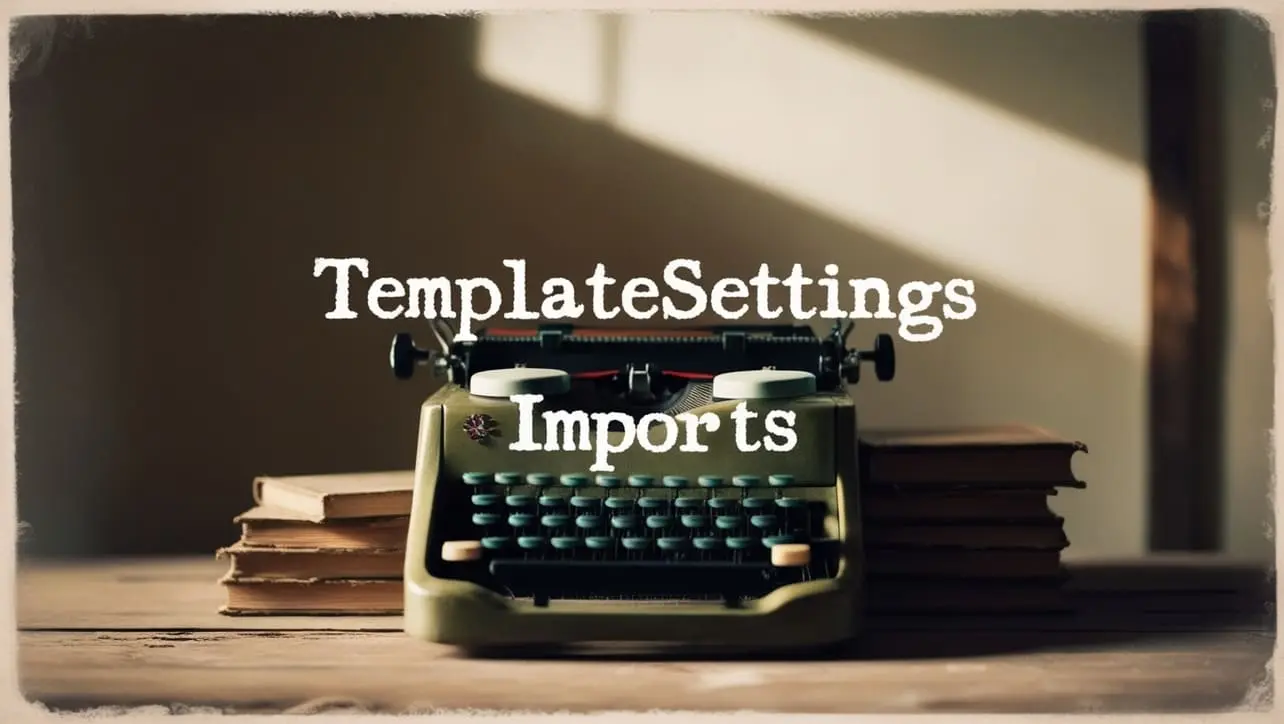
Lodash _.templateSettings.imports Property
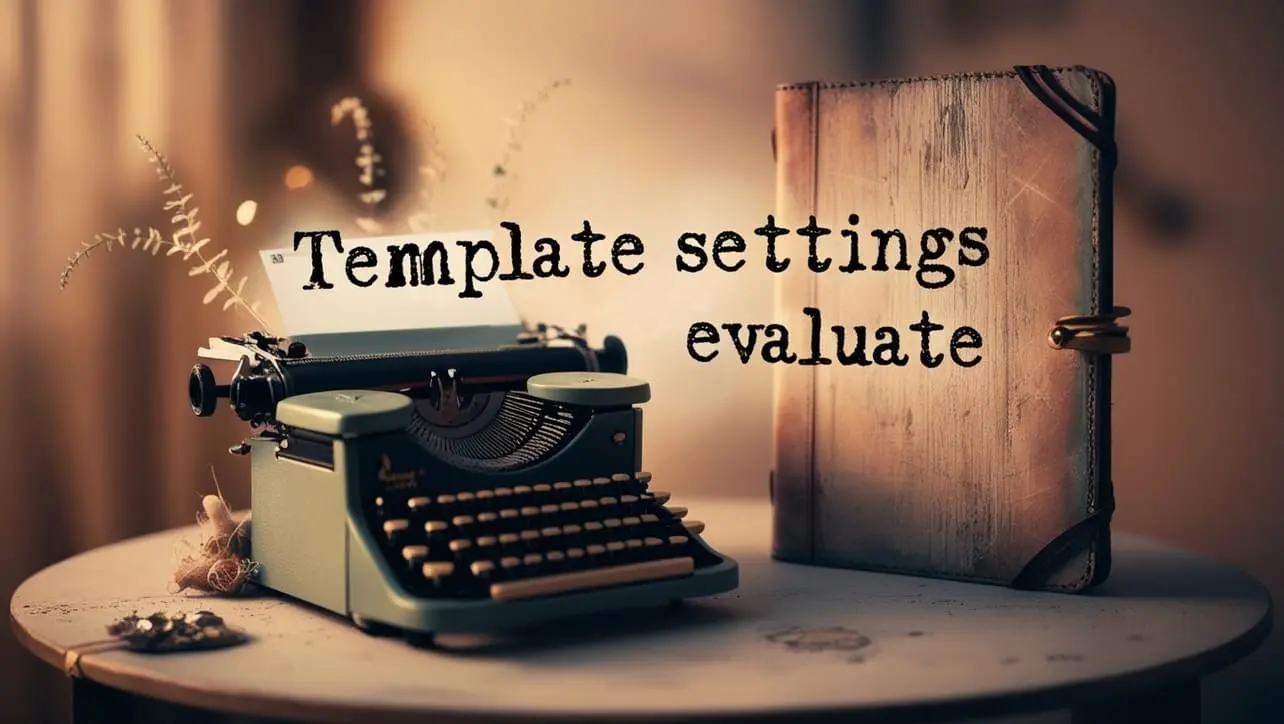
Lodash _.templateSettings.evaluate Property
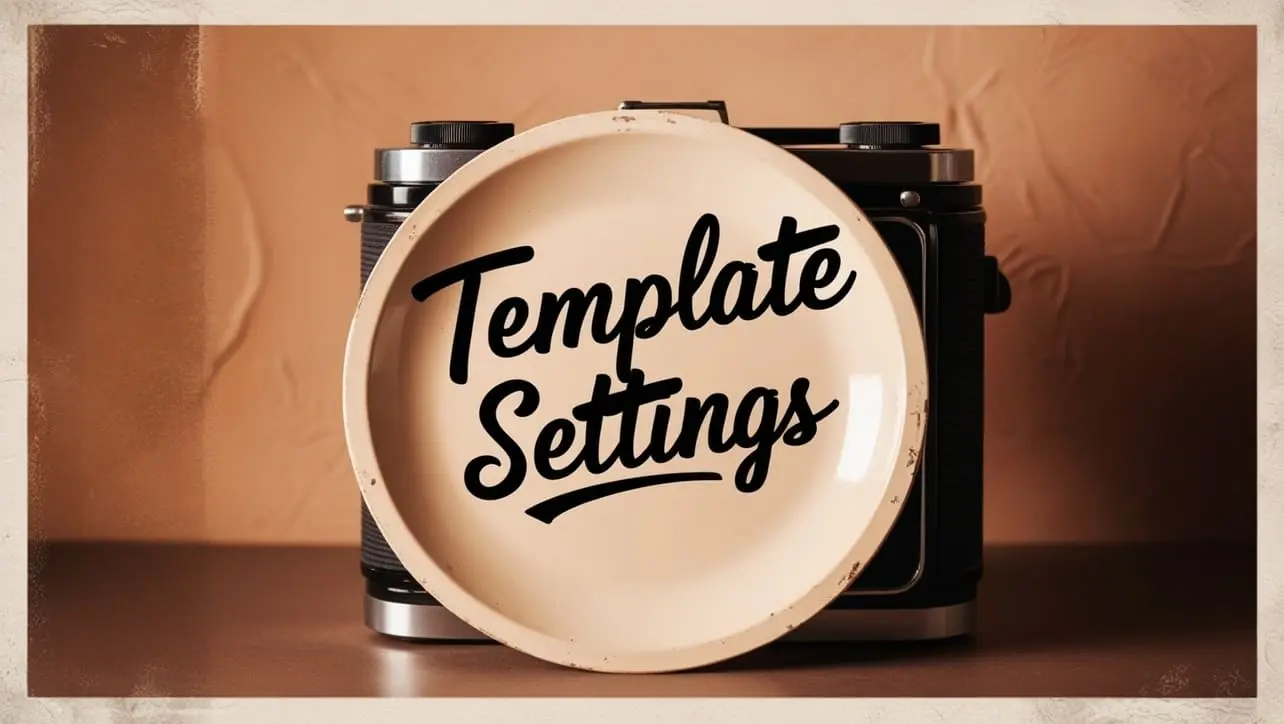
Lodash _.templateSettings Property
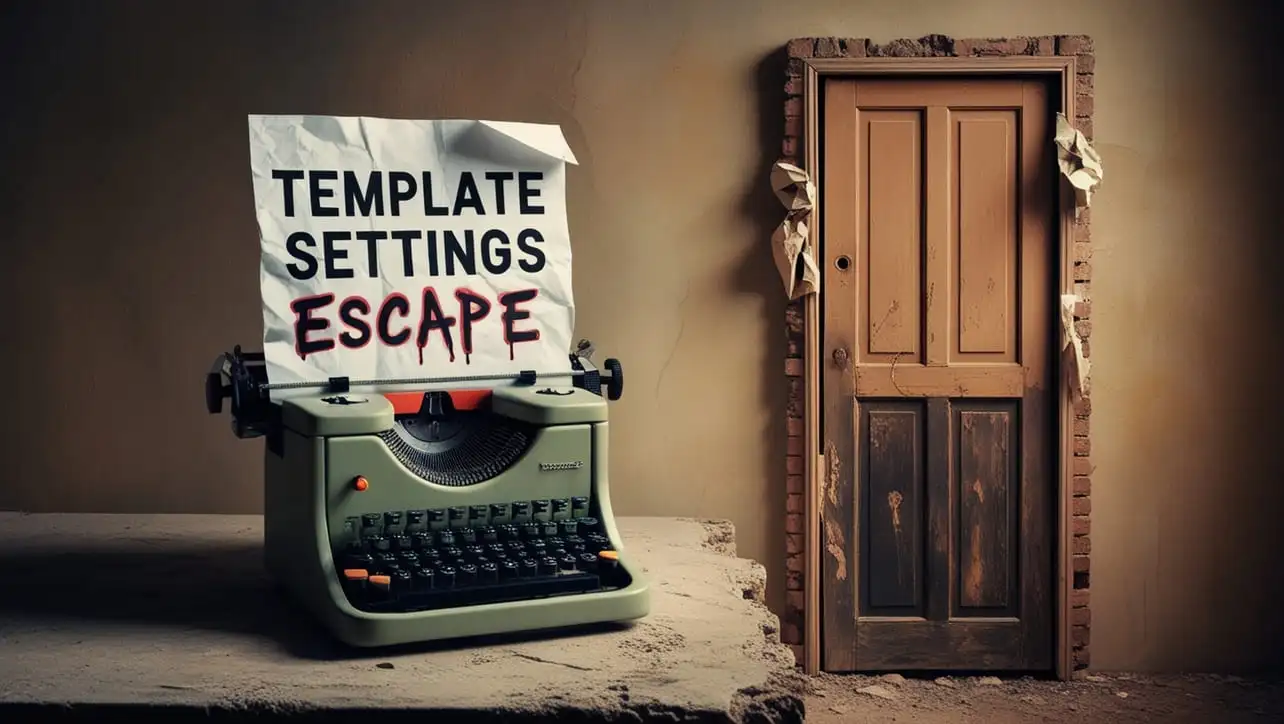
Lodash _.templateSettings.escape Property
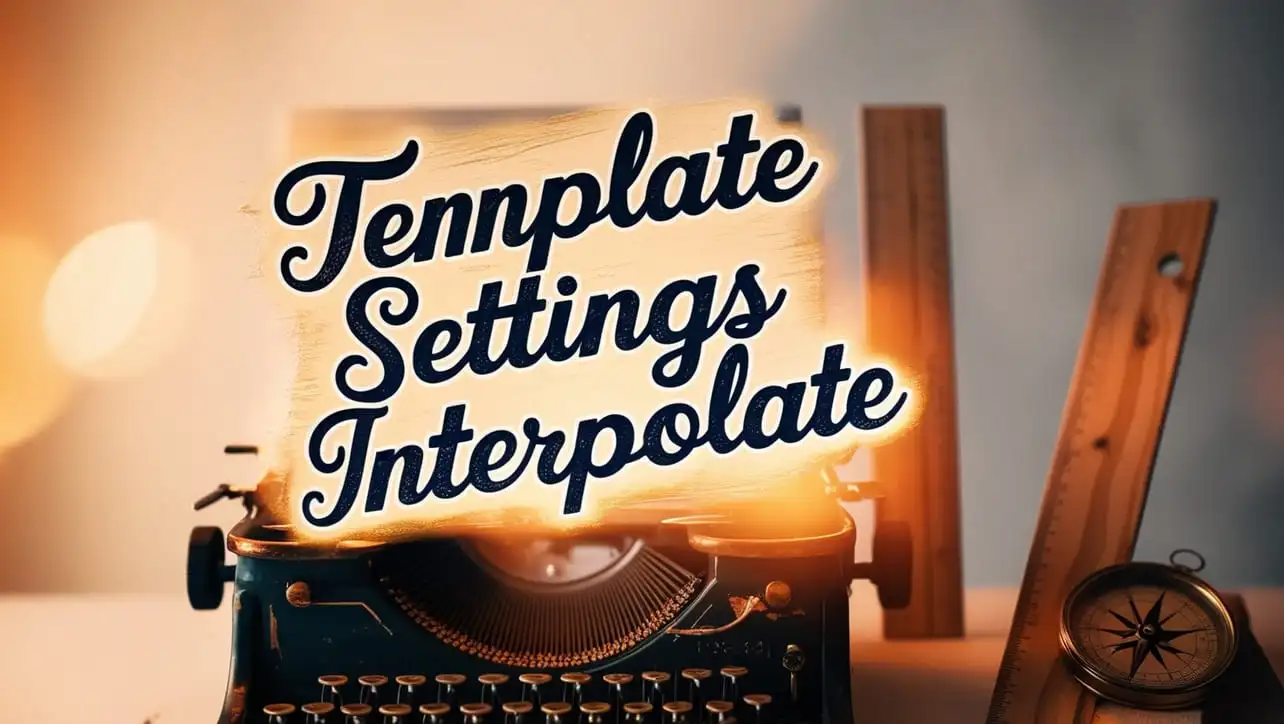
Lodash _.templateSettings.interpolate Property
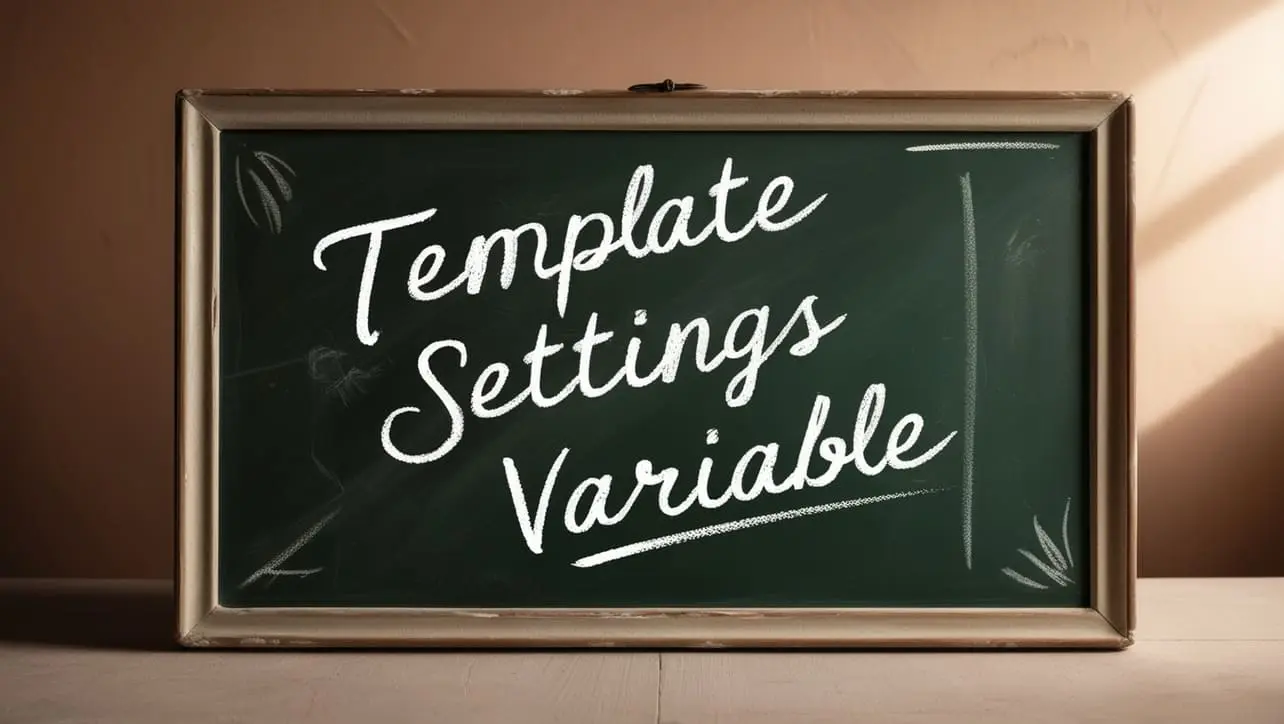
If you have any doubts regarding this article (Lodash _.method() Util Method), please comment here. I will help you immediately.