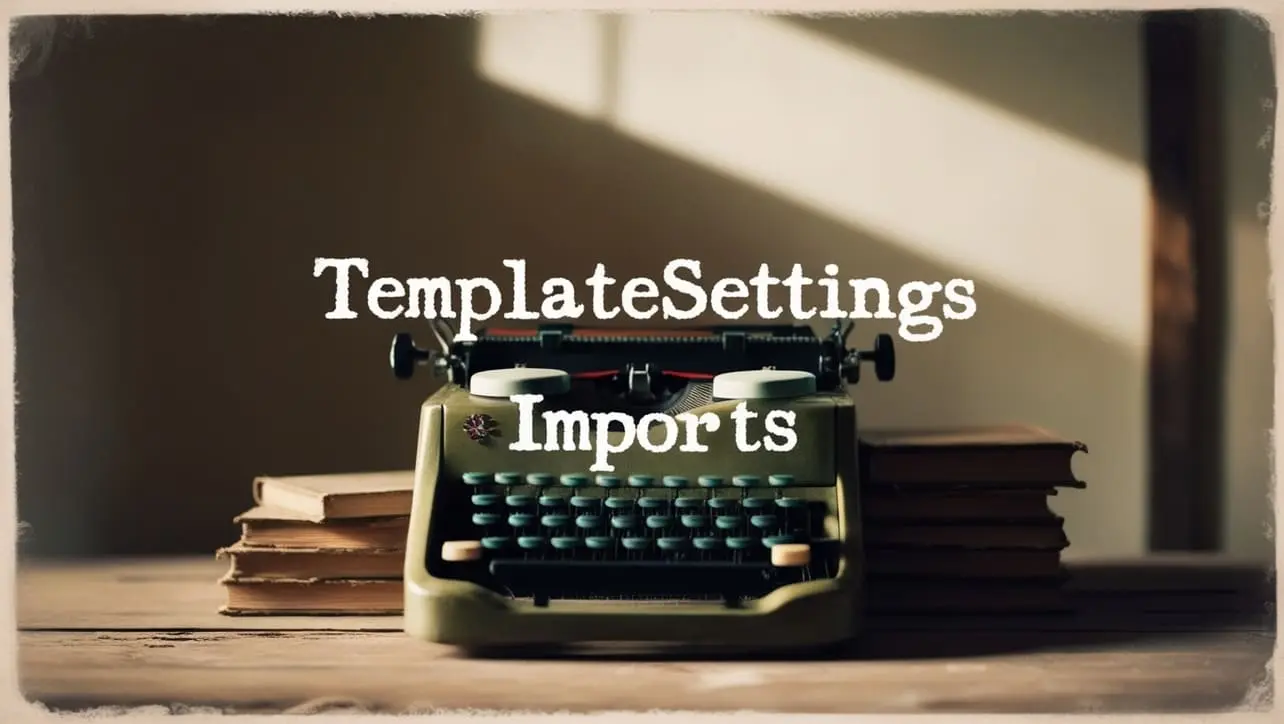
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.matchesProperty() Util Method
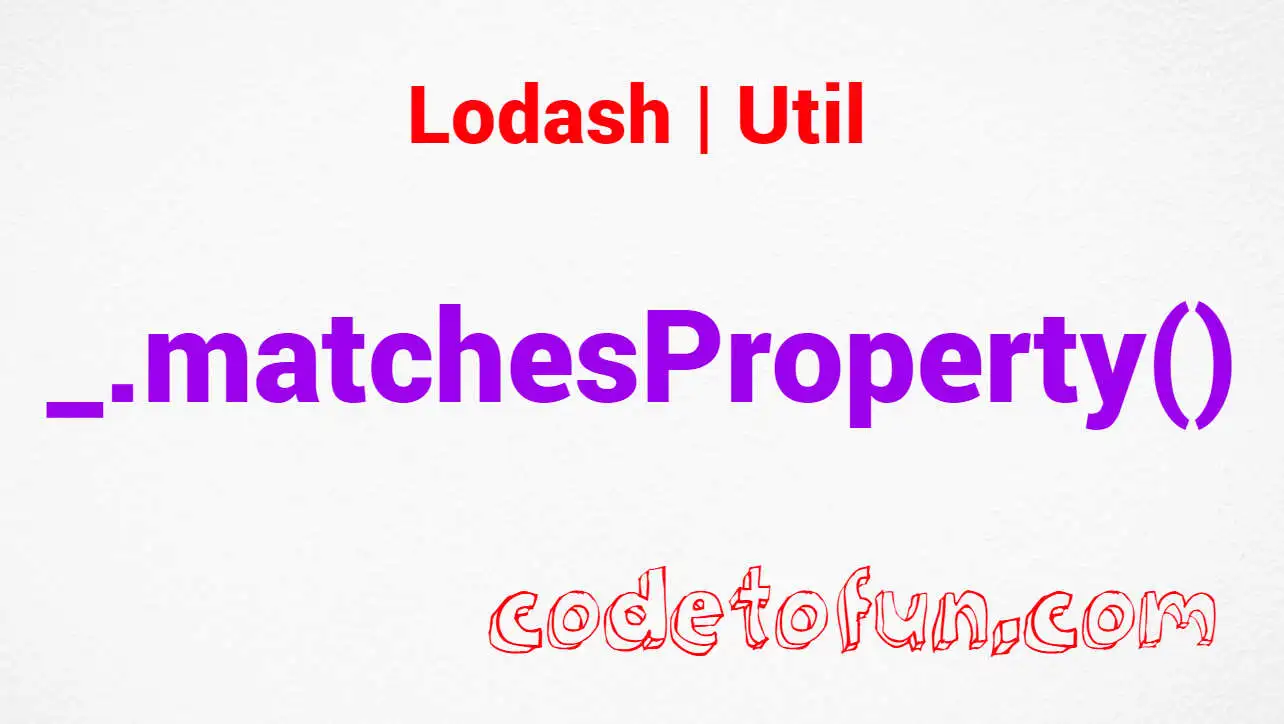
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, working with complex data structures often requires precise matching of property values. Lodash, a widely used utility library, offers a plethora of functions to simplify these tasks. Among these functions lies the _.matchesProperty()
method, a powerful tool for creating a predicate function that matches properties of objects.
This method enhances code clarity and conciseness, making it invaluable for developers dealing with data filtering and searching.
🧠 Understanding _.matchesProperty() Method
The _.matchesProperty()
method in Lodash creates a function that checks if an object has properties with specific values. It returns a predicate function that can be used with methods like _.filter() or _.find() to select objects that match the specified property values.
💡 Syntax
The syntax for the _.matchesProperty()
method is straightforward:
_.matchesProperty(path, value)
- path: The path of the property to match.
- value: The value to match.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.matchesProperty()
method:
const _ = require('lodash');
const users = [
{
id: 1,
name: 'John',
age: 30
},
{
id: 2,
name: 'Alice',
age: 25
},
{
id: 3,
name: 'Bob',
age: 35
}];
const filteredUsers = _.filter(users, _.matchesProperty('age', 30));
console.log(filteredUsers);
// Output: [{ id: 1, name: 'John', age: 30 }]
In this example, the _.matchesProperty()
method creates a predicate function that matches objects with the property age equal to 30, and then filters the users array based on this predicate.
🏆 Best Practices
When working with the _.matchesProperty()
method, consider the following best practices:
Understanding Property Paths:
Ensure the property path provided to
_.matchesProperty()
accurately reflects the structure of your data. Incorrect property paths will result in no matches being found.example.jsCopiedconst users = [ { user: { id: 1, name: 'John' } }, { user: { id: 2, name: 'Alice' } }, { user: { id: 3, name: 'Bob' } } ]; const filteredUsers = _.filter(users, _.matchesProperty('user.name', 'Alice')); console.log(filteredUsers); // Output: [{ user: { id: 2, name: 'Alice' } }]
Specifying Values:
Provide the value to match accurately, considering the data type and expected values. Mismatched values will lead to no matches being found.
example.jsCopiedconst users = [ { id: 1, name: 'John', status: 'active' }, { id: 2, name: 'Alice', status: 'inactive' }, { id: 3, name: 'Bob', status: 'active' } ]; const filteredUsers = _.filter(users, _.matchesProperty('status', 'active')); console.log(filteredUsers); // Output: [{ id: 1, name: 'John', status: 'active' }, { id: 3, name: 'Bob', status: 'active' }]
Combining with Other Methods:
Utilize
_.matchesProperty()
in conjunction with other Lodash methods like _.filter() or _.find() to perform sophisticated data filtering and searching operations.example.jsCopiedconst users = [ { id: 1, name: 'John', age: 30 }, { id: 2, name: 'Alice', age: 25 }, { id: 3, name: 'Bob', age: 35 } ]; const foundUser = _.find(users, _.matchesProperty('age', 25)); console.log(foundUser); // Output: { id: 2, name: 'Alice', age: 25 }
📚 Use Cases
Filtering Data:
_.matchesProperty()
is particularly useful for filtering data based on specific property values, allowing you to select objects that meet certain criteria.example.jsCopiedconst users = /* ...fetch users data from API or elsewhere... */; const activeUsers = _.filter(users, _.matchesProperty('status', 'active')); console.log(activeUsers);
Searching for Objects:
When searching for objects within an array based on property values,
_.matchesProperty()
enables precise matching, ensuring accurate search results.example.jsCopiedconst products = /* ...fetch products data from API or elsewhere... */; const foundProduct = _.find(products, _.matchesProperty('id', productId)); console.log(foundProduct);
Conditional Rendering:
In UI development,
_.matchesProperty()
can be used to conditionally render components based on specific data criteria, enhancing user experience.example.jsCopiedconst users = /* ...fetch users data from API or elsewhere... */; const isAdmin = _.some(users, _.matchesProperty('role', 'admin')); console.log(isAdmin ? 'Show Admin Panel' : 'Regular User View');
🎉 Conclusion
The _.matchesProperty()
method in Lodash provides a convenient way to create predicate functions for matching objects based on specific property values. Whether you're filtering data, searching for objects, or implementing conditional rendering, this method offers versatility and precision in data manipulation.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.matchesProperty()
method in your Lodash projects.
👨💻 Join our Community:
Author
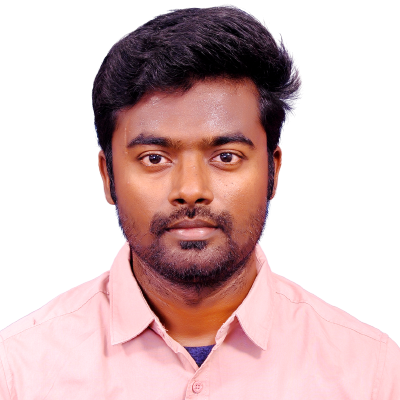
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
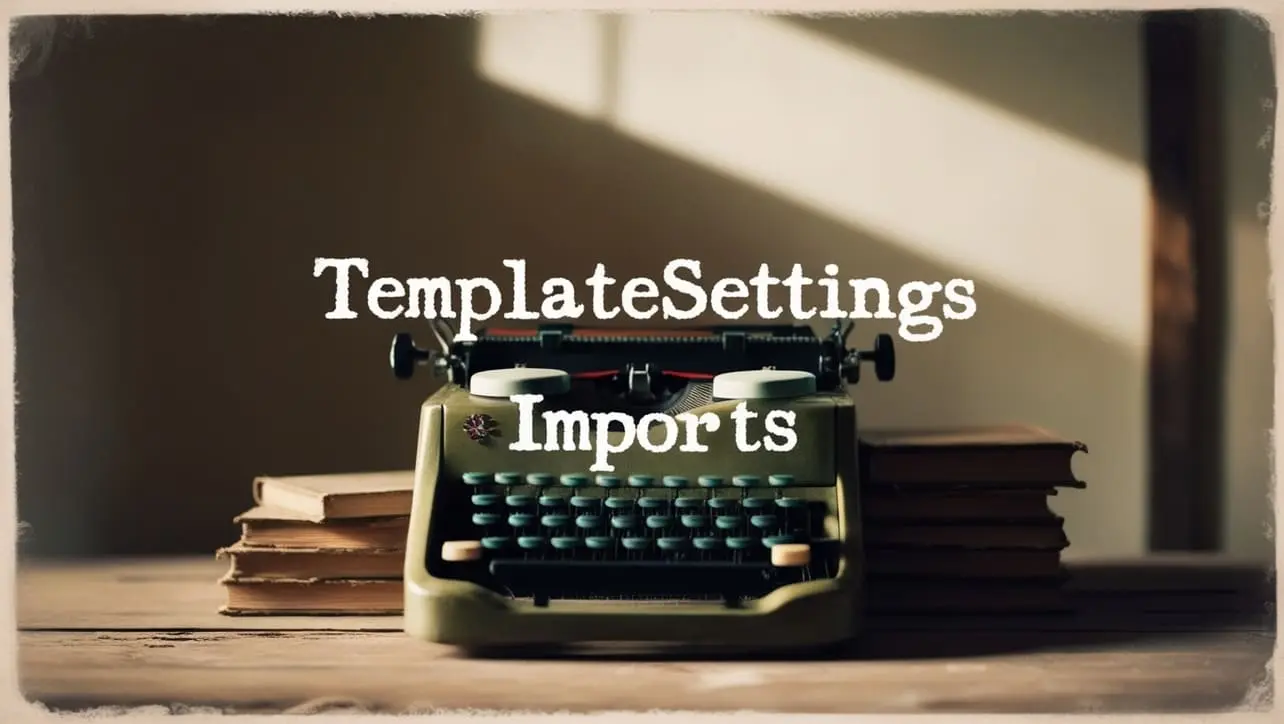
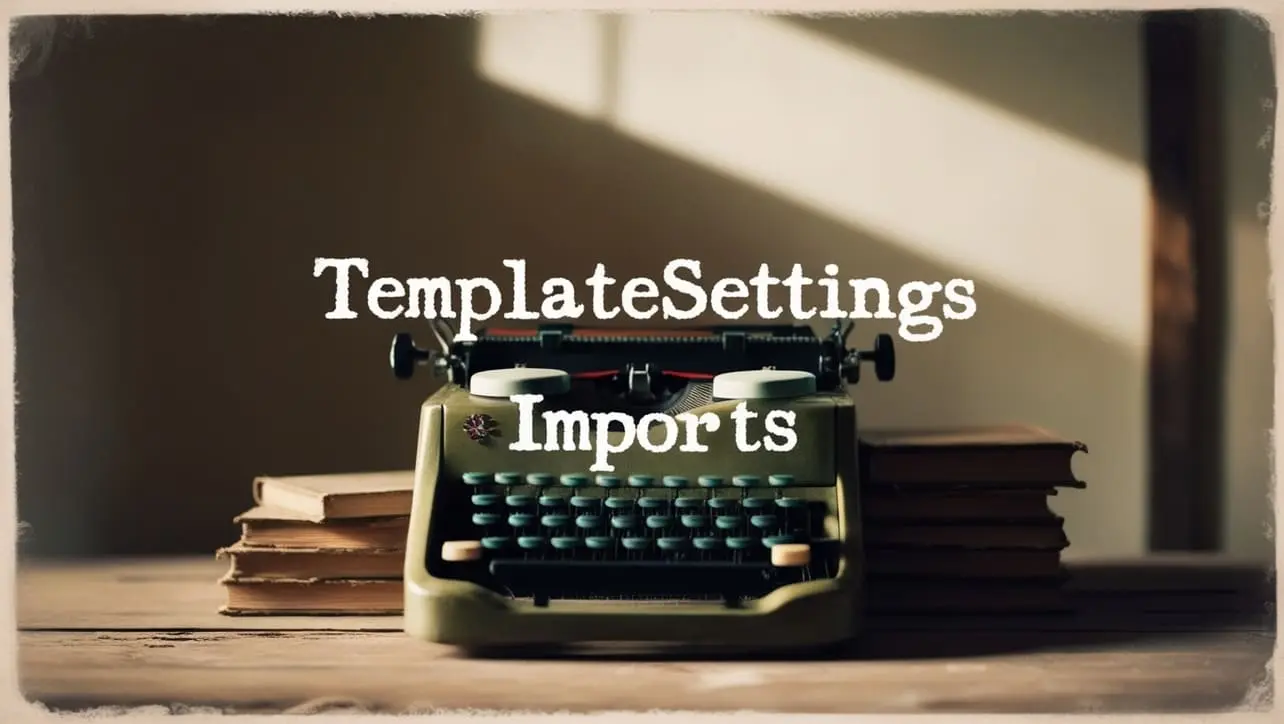
Lodash _.templateSettings.imports Property
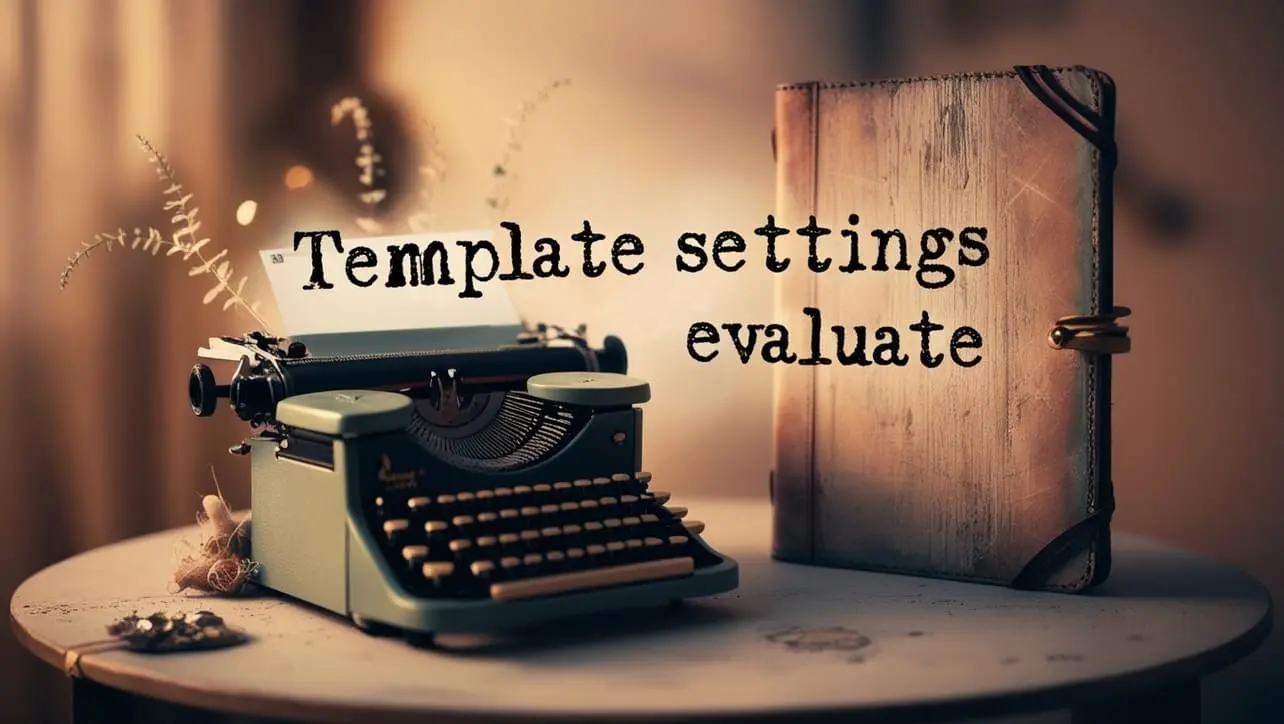
Lodash _.templateSettings.evaluate Property
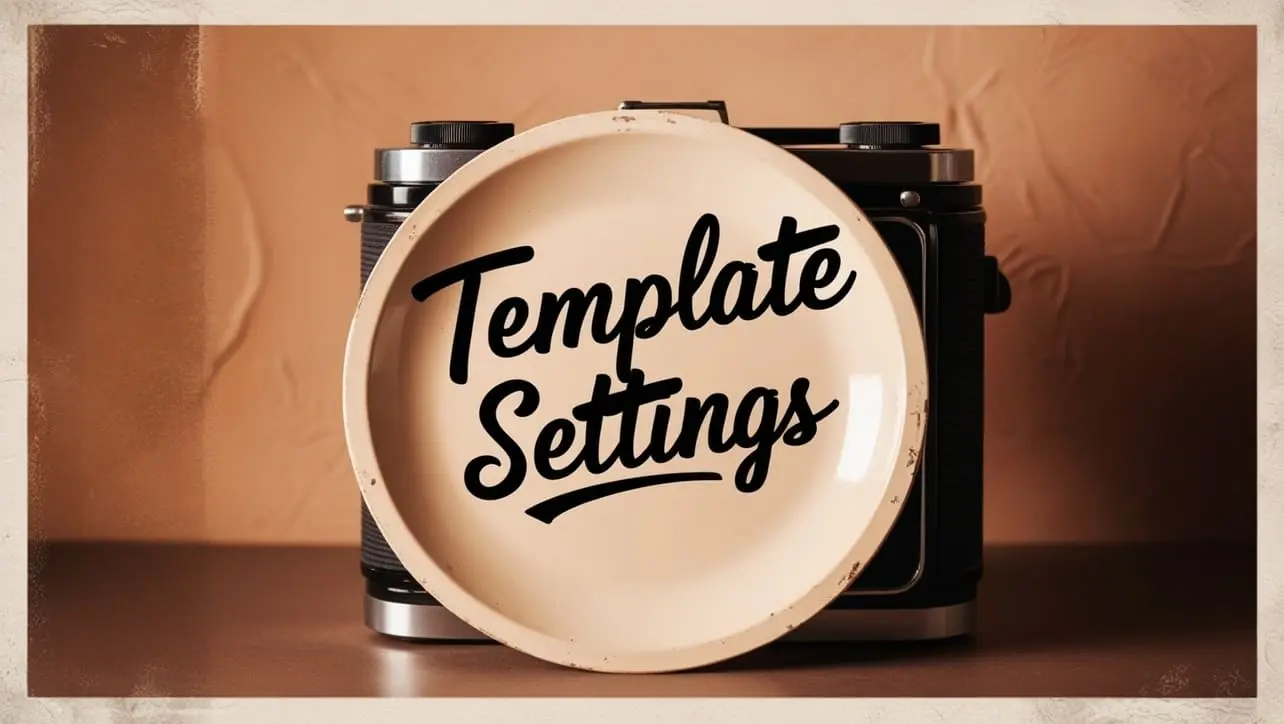
Lodash _.templateSettings Property
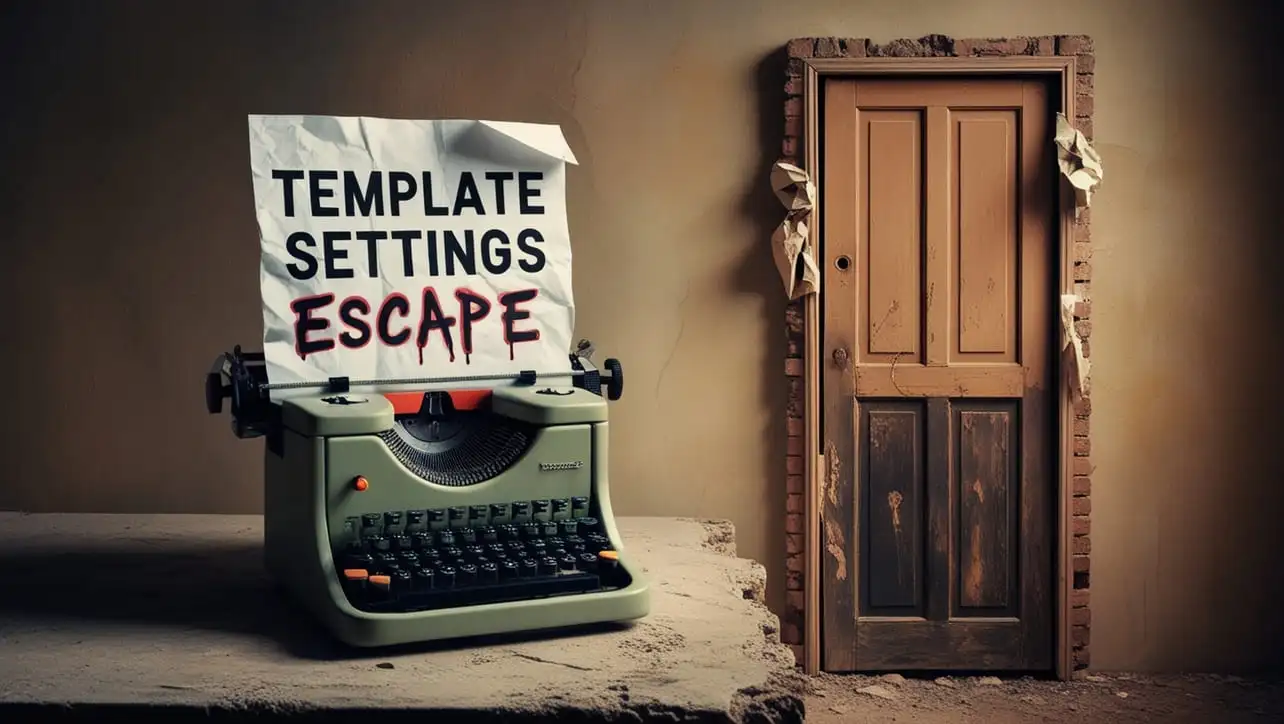
Lodash _.templateSettings.escape Property
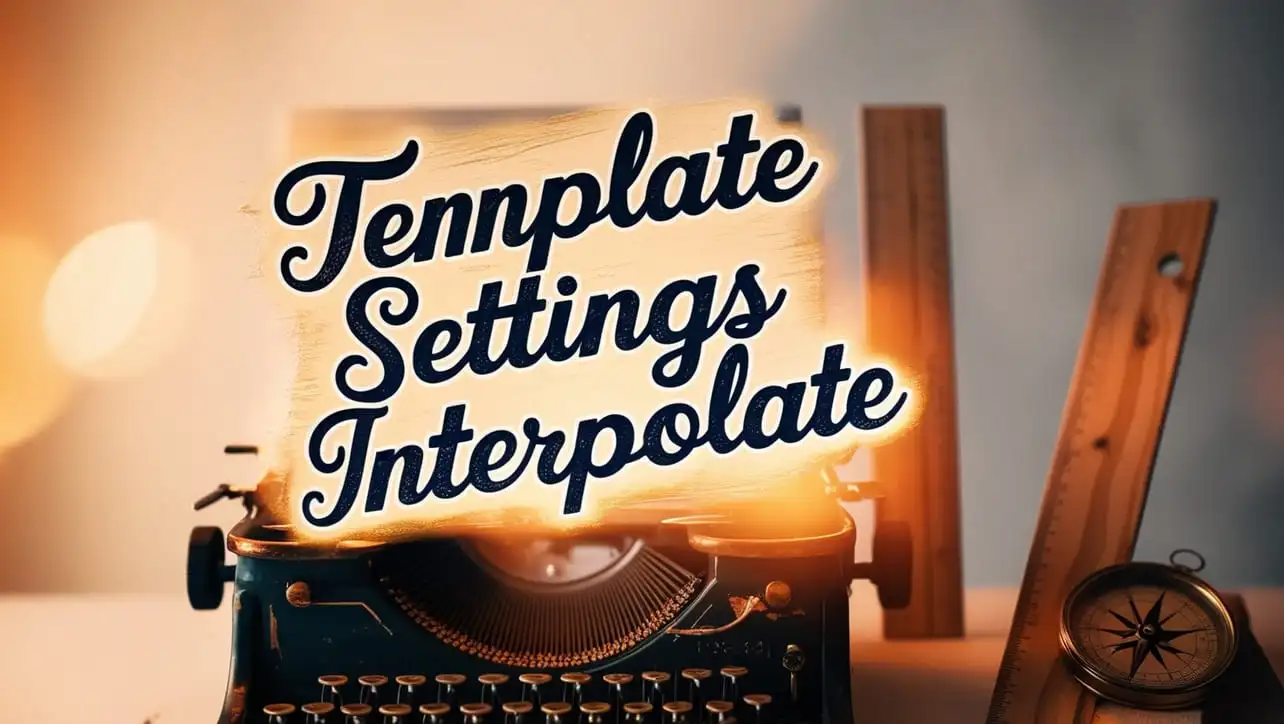
Lodash _.templateSettings.interpolate Property
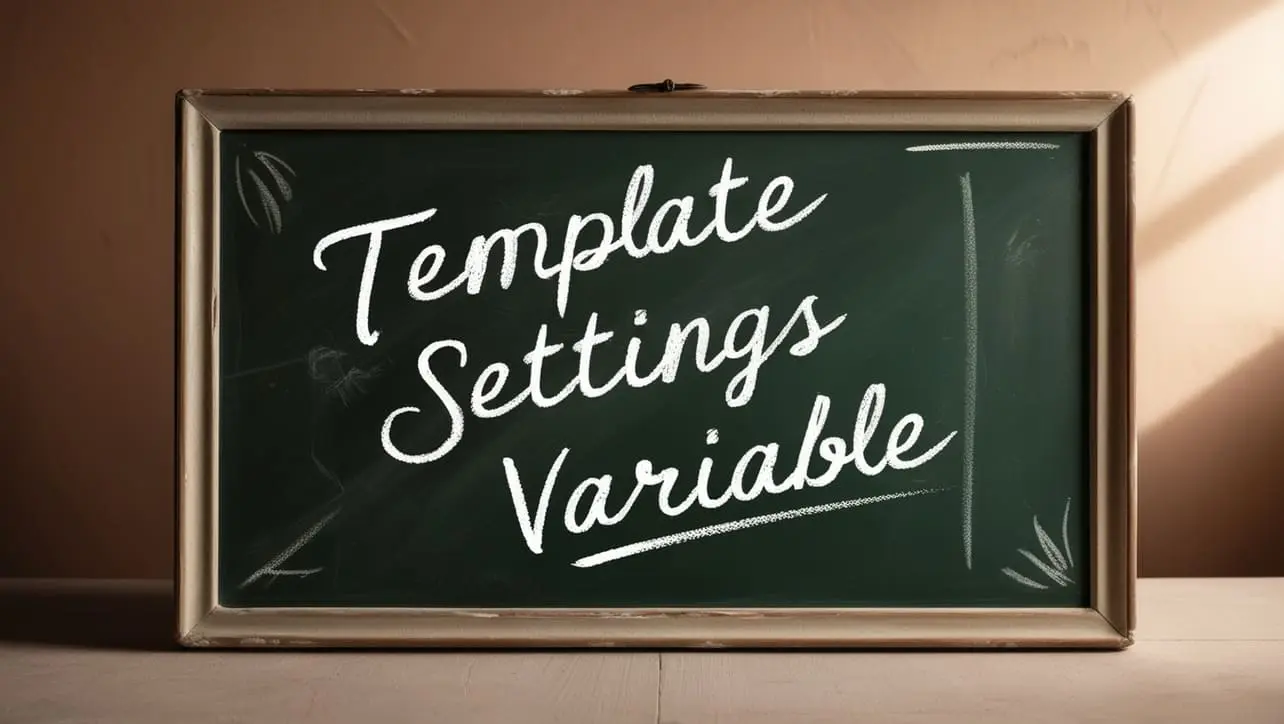
If you have any doubts regarding this article (Lodash _.matchesProperty() Util Method), please comment here. I will help you immediately.