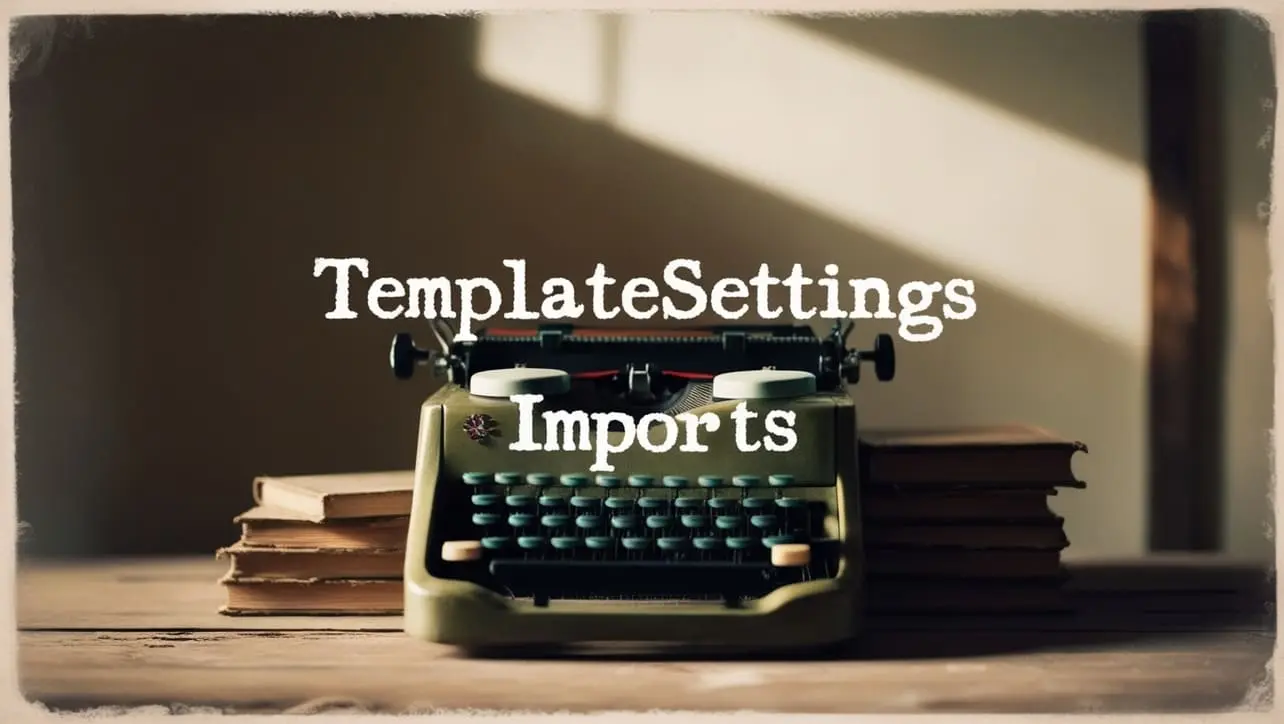
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.iteratee() Util Method
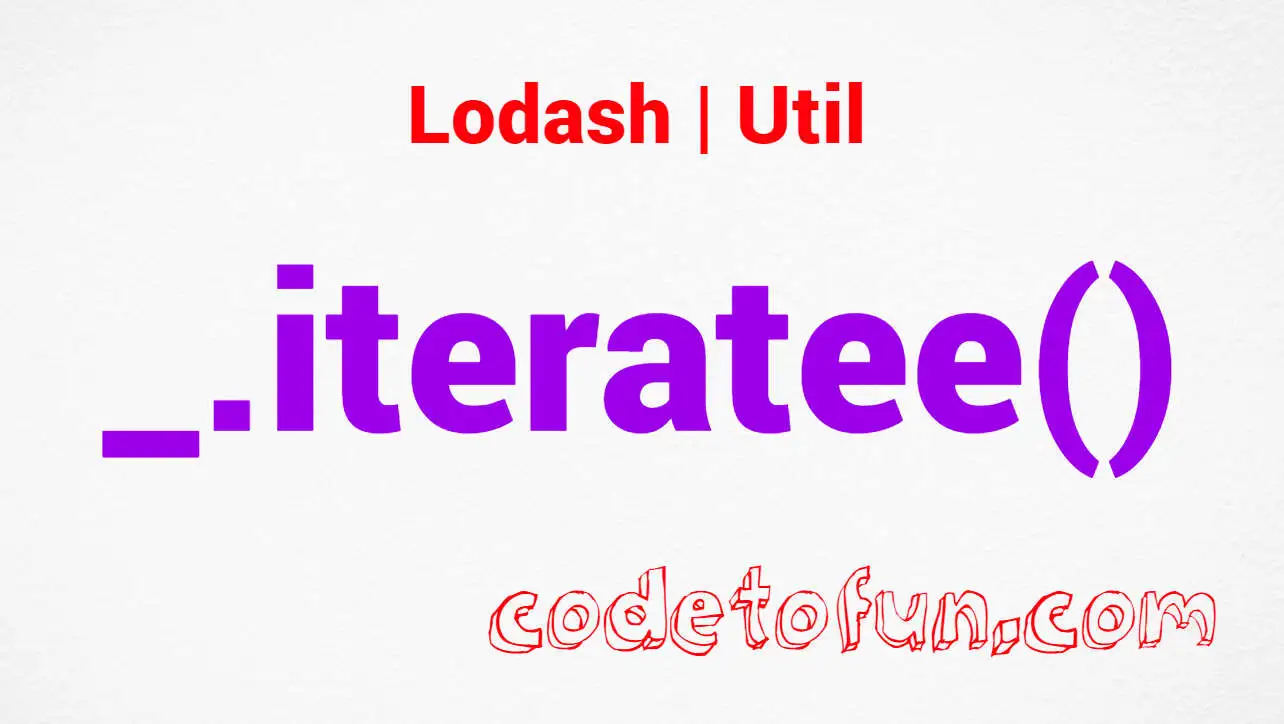
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, handling iteration logic often involves writing custom functions tailored to specific use cases. Lodash, a popular utility library, provides the _.iteratee()
method to simplify the creation of iteratee functions.
This method enhances code clarity and conciseness by allowing developers to express iteration logic more efficiently.
🧠 Understanding _.iteratee() Method
The _.iteratee()
method in Lodash transforms a value into an iteratee function. It accepts various types of input, including functions, property names, and predicates, and returns an iteratee function tailored to the provided input. This versatility enables developers to streamline iteration logic across different contexts.
💡 Syntax
The syntax for the _.iteratee()
method is straightforward:
_.iteratee(value)
- value: The value to transform into an iteratee function.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.iteratee()
method:
const _ = require('lodash');
const iterateeFunc = _.iteratee('age');
const people = [{ name: 'John', age: 30 }, { name: 'Jane', age: 25 }];
const ages = people.map(iterateeFunc);
console.log(ages);
// Output: [30, 25]
In this example, iterateeFunc is created using _.iteratee('age'), which generates an iteratee function that extracts the 'age' property from objects. This function is then applied to each object in the people array using Array.map().
🏆 Best Practices
When working with the _.iteratee()
method, consider the following best practices:
Simplify Property Access:
Use
_.iteratee()
to simplify property access when working with arrays of objects. This allows for cleaner and more expressive code, especially when dealing with complex data structures.example.jsCopiedconst cities = [{ name: 'New York', population: 8398748 }, { name: 'Los Angeles', population: 3990456 }]; const getPopulation = _.iteratee('population'); const populations = cities.map(getPopulation); console.log(populations); // Output: [8398748, 3990456]
Enhance Predicate Functions:
Transform predicate functions into iteratee functions using
_.iteratee()
, enabling seamless integration with Lodash methods that expect iteratee functions. This promotes code consistency and interoperability.example.jsCopiedconst numbers = [1, 2, 3, 4, 5]; const isEven = _.iteratee(num => num % 2 === 0); const evenNumbers = _.filter(numbers, isEven); console.log(evenNumbers); // Output: [2, 4]
Parameter Flexibility:
Take advantage of _.iteratee()'s parameter flexibility to adapt to different input types, including functions, property names, and predicates. This versatility empowers developers to handle diverse iteration requirements with ease.
example.jsCopiedconst data = [{ name: 'Alice', score: 85 }, { name: 'Bob', score: 90 }]; // Create iteratee functions based on various input types const getName = _.iteratee('name'); const passingScore = _.iteratee({ 'score': 90 }); const customFilter = _.iteratee(person => person.score > 80); const names = data.map(getName); const highScorers = _.filter(data, passingScore); const customFilteredData = _.filter(data, customFilter); console.log(names); console.log(highScorers); console.log(customFilteredData);
📚 Use Cases
Data Transformation:
Use
_.iteratee()
to transform data by extracting specific properties or applying custom logic to each element. This facilitates data manipulation and preparation for further processing.example.jsCopiedconst students = [{ name: 'Alice', grade: 'A' }, { name: 'Bob', grade: 'B' }]; const getGrade = _.iteratee('grade'); const grades = students.map(getGrade); console.log(grades); // Output: ['A', 'B']
Filtering Data:
Leverage
_.iteratee()
to create predicate functions for filtering data based on specific criteria. This simplifies the filtering process and enhances code readability.example.jsCopiedconst items = [{ name: 'Chair', price: 50 }, { name: 'Table', price: 100 }]; const isExpensive = _.iteratee(item => item.price > 75); const expensiveItems = _.filter(items, isExpensive); console.log(expensiveItems); // Output: [{ name: 'Table', price: 100 }]
Sorting Data:
Employ
_.iteratee()
to generate comparator functions for sorting data based on desired attributes or criteria. This facilitates flexible and customizable sorting operations.example.jsCopiedconst products = [{ name: 'Laptop', price: 1000 }, { name: 'Smartphone', price: 800 }]; const sortByPrice = _.sortBy(products, _.iteratee('price')); console.log(sortByPrice); // Output: [{ name: 'Smartphone', price: 800 }, { name: 'Laptop', price: 1000 }]
🎉 Conclusion
The _.iteratee()
method in Lodash provides a versatile solution for transforming values into iteratee functions, enhancing iteration logic in JavaScript. Whether you're simplifying property access, enhancing predicate functions, or adapting to diverse input types, _.iteratee()
offers a powerful tool for streamlining iteration workflows.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.iteratee()
method in your Lodash projects.
👨💻 Join our Community:
Author
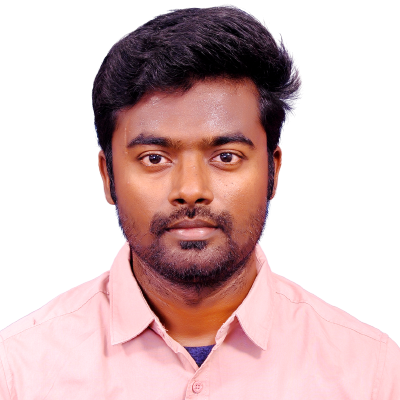
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
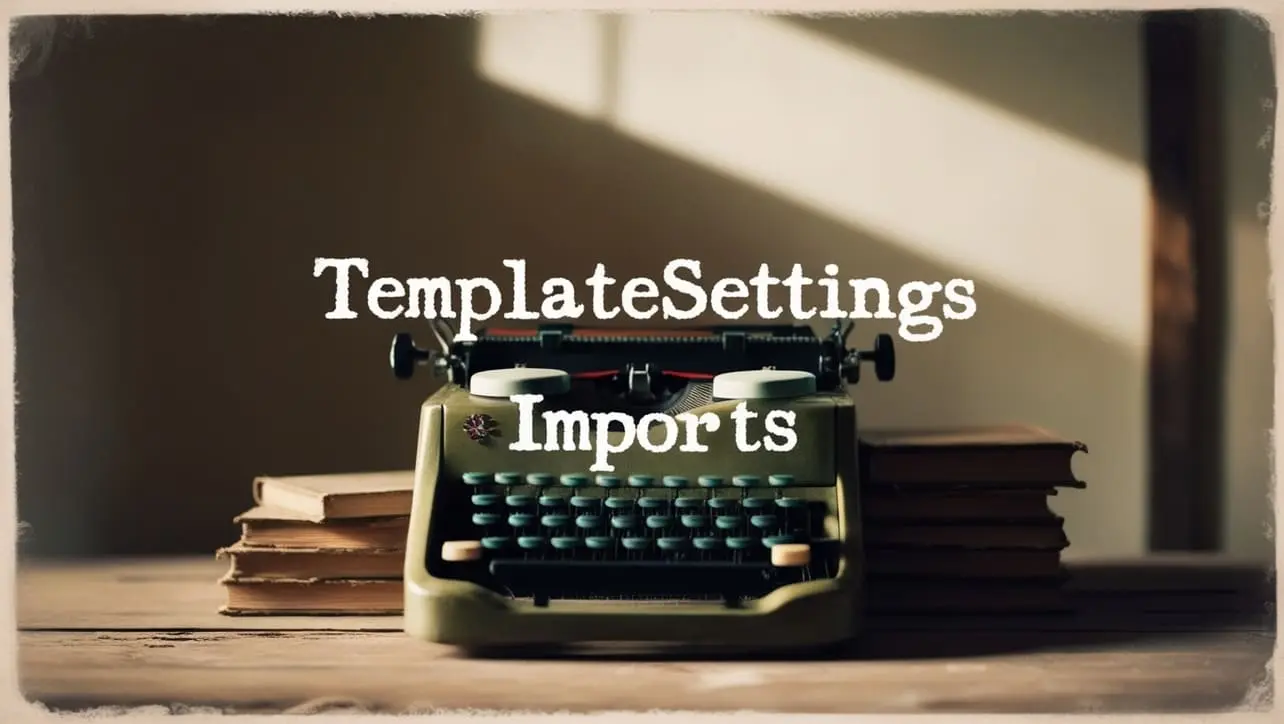
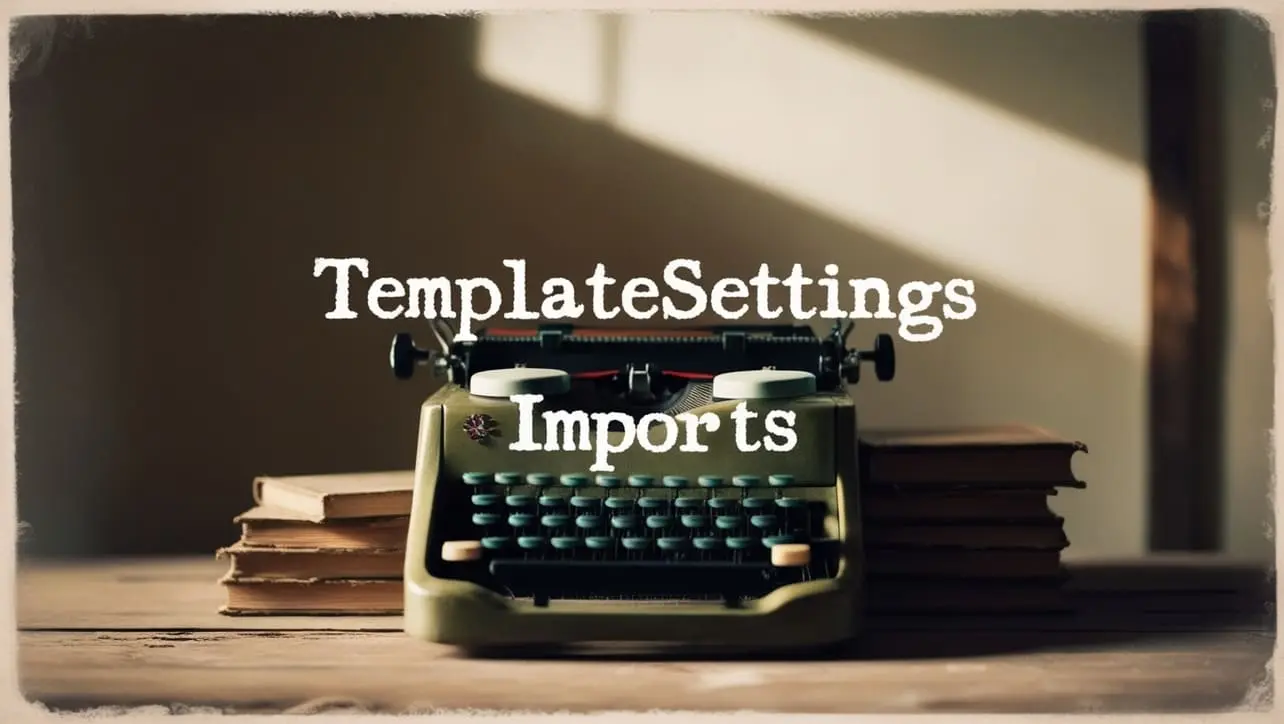
Lodash _.templateSettings.imports Property
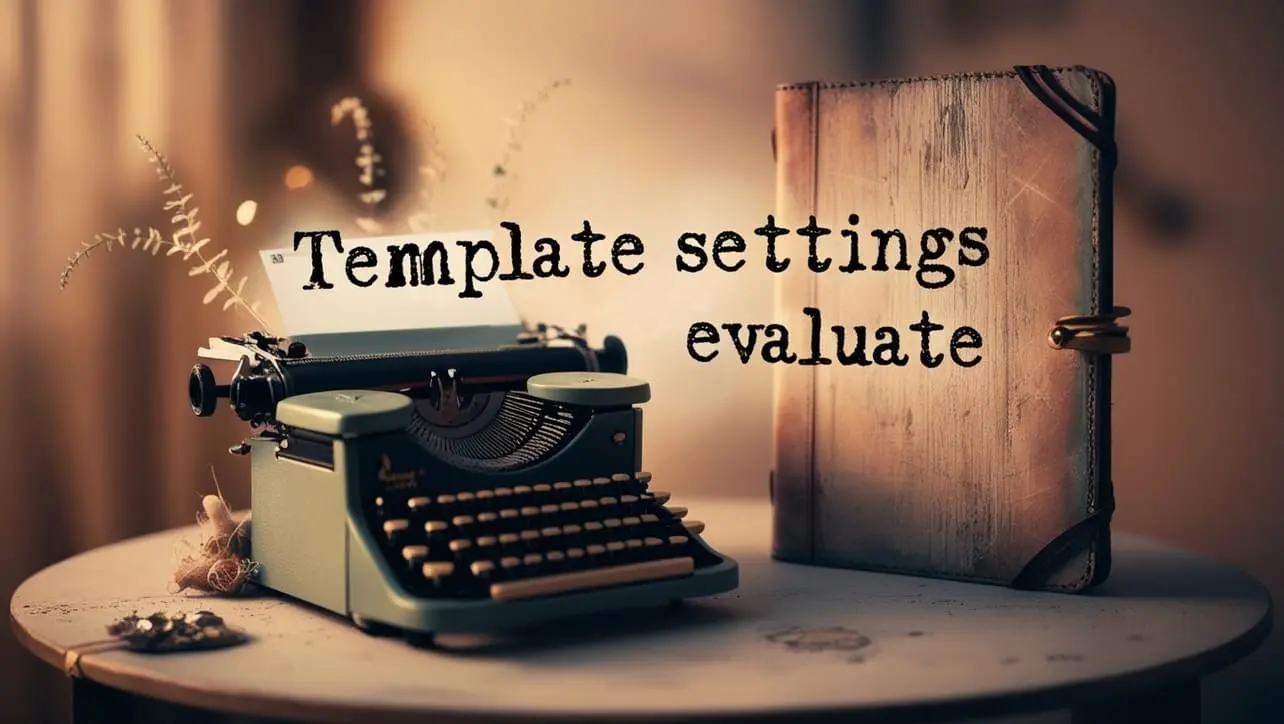
Lodash _.templateSettings.evaluate Property
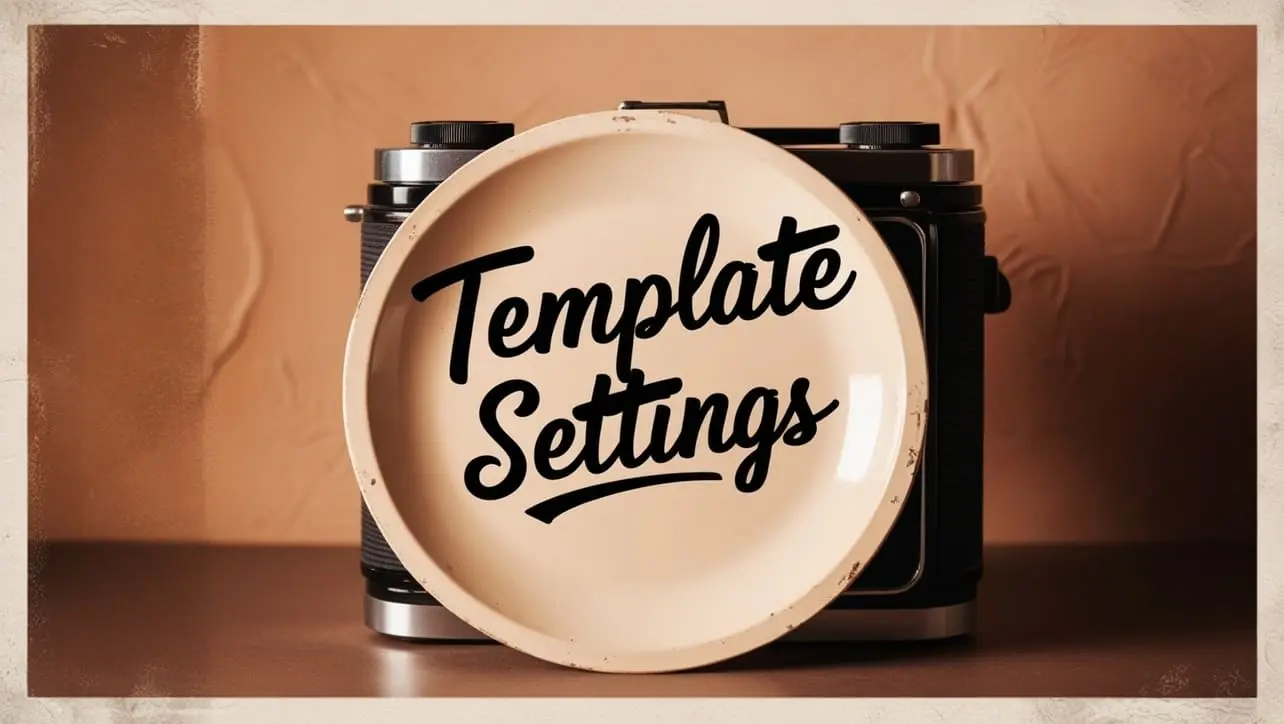
Lodash _.templateSettings Property
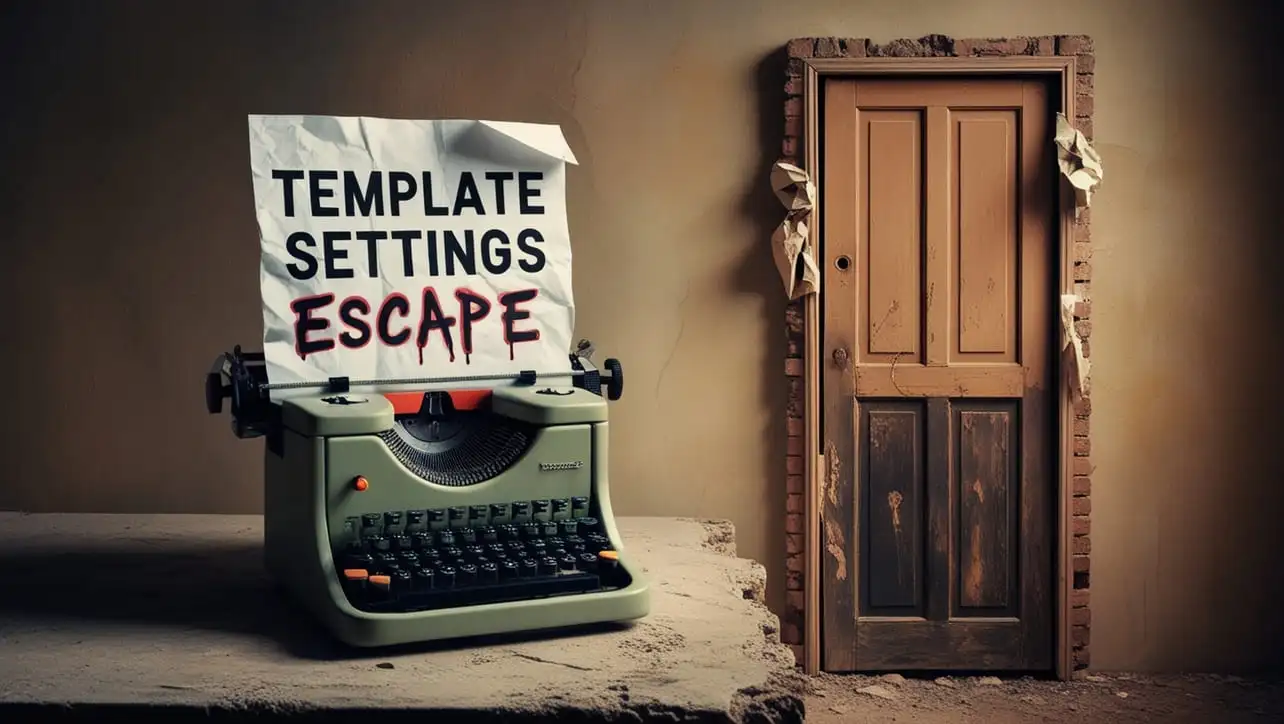
Lodash _.templateSettings.escape Property
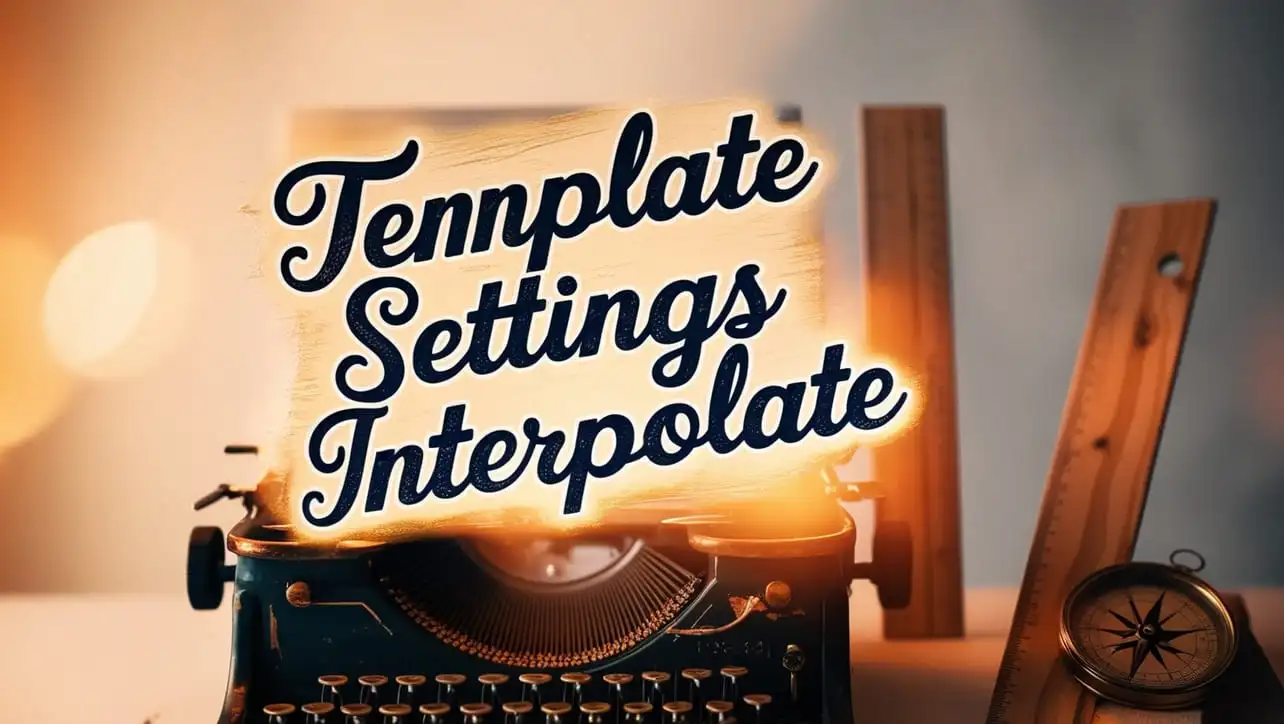
Lodash _.templateSettings.interpolate Property
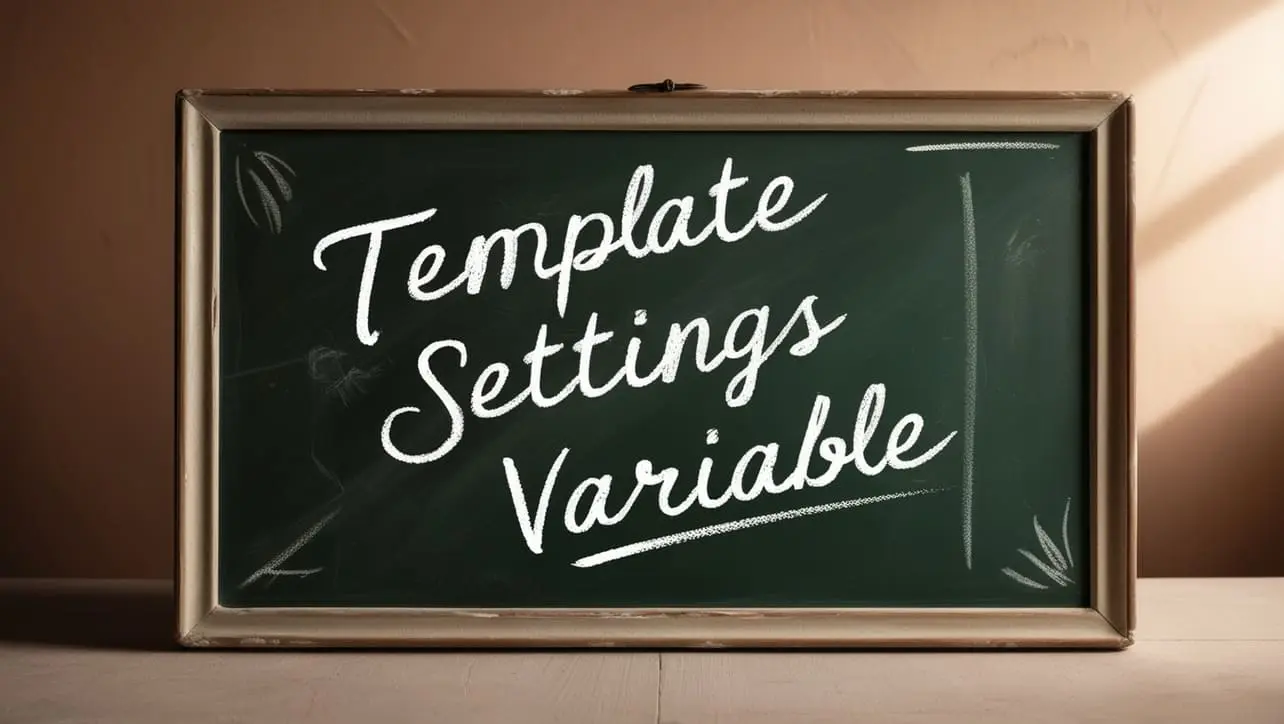
If you have any doubts regarding this article (Lodash _.iteratee() Util Method), please comment here. I will help you immediately.