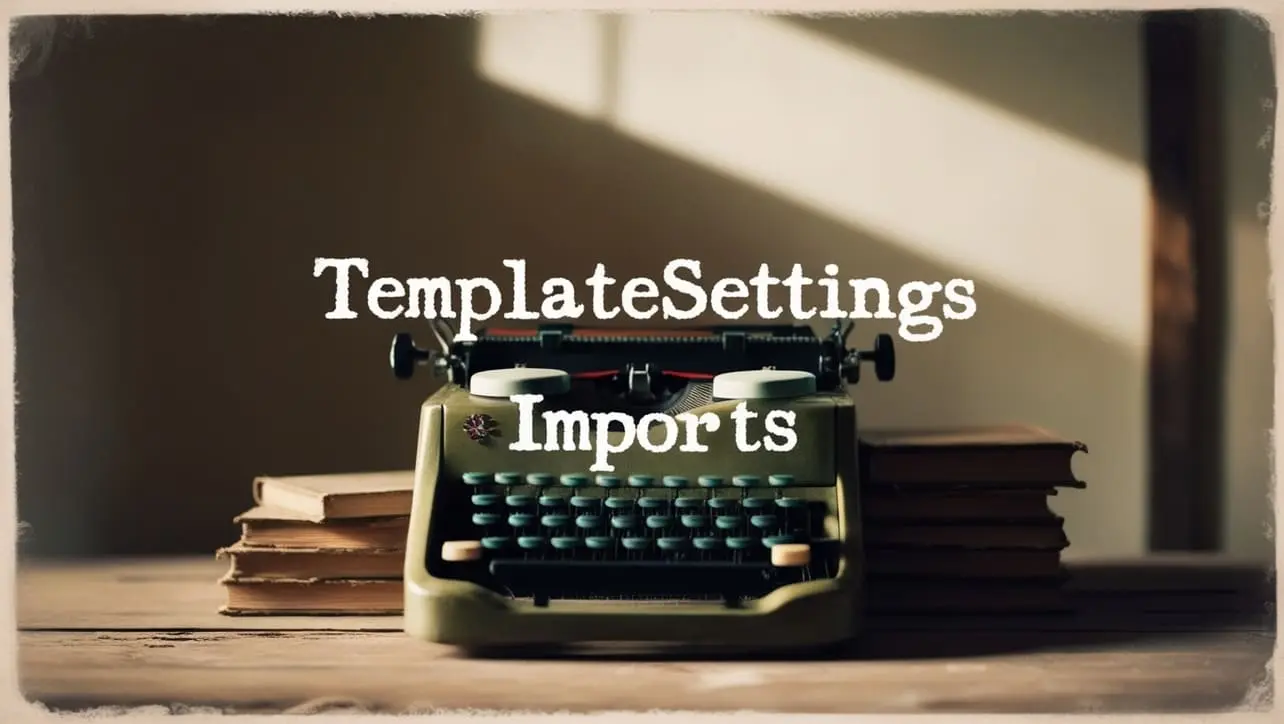
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.flowRight() Util Method
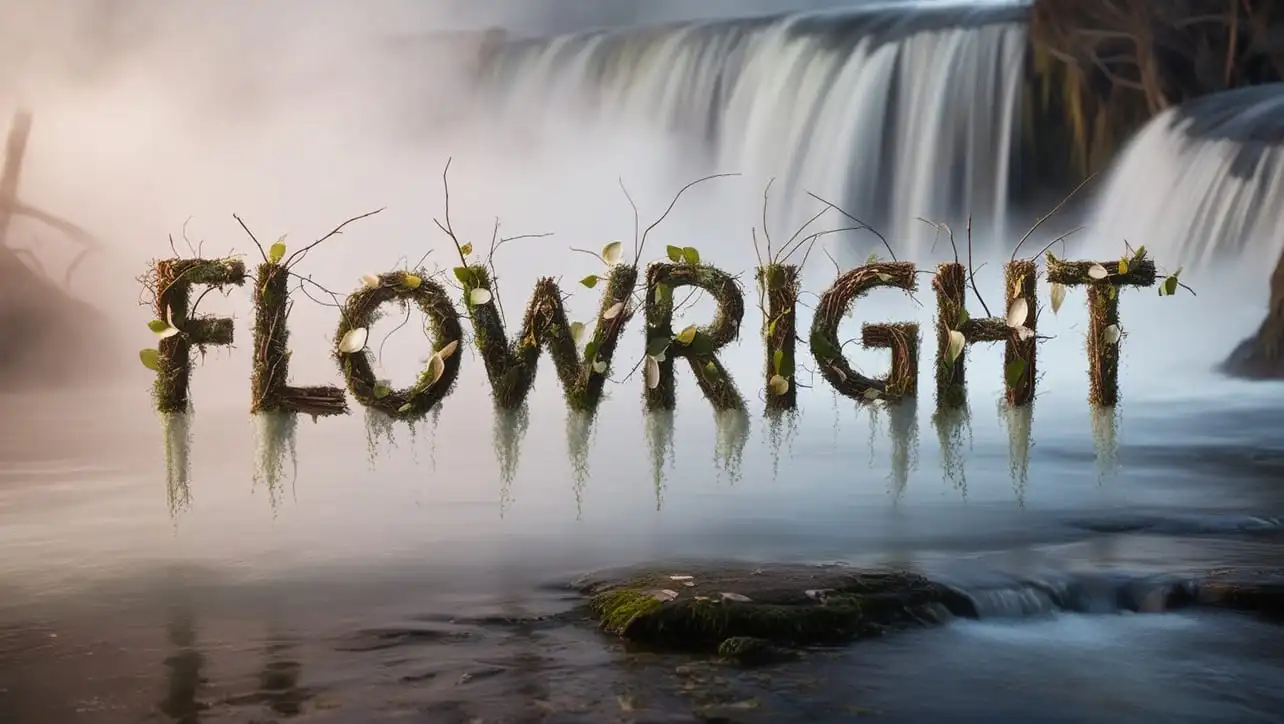
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, composing functions to perform complex operations is a common task. Lodash, a widely-used utility library, provides the _.flowRight()
method to streamline this process.
This method enables developers to create a new function by composing multiple functions from right to left, offering a convenient and intuitive way to organize and execute function chains.
🧠 Understanding _.flowRight() Method
The _.flowRight()
method in Lodash allows you to create a new function by composing multiple functions together, executing them from right to left. This enables developers to build function chains where the output of one function becomes the input of the next, simplifying complex operations and improving code readability.
💡 Syntax
The syntax for the _.flowRight()
method is straightforward:
_.flowRight([funcs])
- funcs: The functions to compose.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.flowRight()
method:
const _ = require('lodash');
const add = x => x + 5;
const multiply = x => x * 2;
const subtract = x => x - 10;
const combinedFunction = _.flowRight(add, multiply, subtract);
console.log(combinedFunction(10));
// Output: 15
In this example, three functions (add, multiply, and subtract) are composed using _.flowRight()
. The resulting combinedFunction performs the operations in the reverse order of their definition.
🏆 Best Practices
When working with the _.flowRight()
method, consider the following best practices:
Compose Pure Functions:
Ensure that the functions you compose with
_.flowRight()
are pure functions, meaning they have no side effects and always return the same output for a given input. This helps maintain code predictability and facilitates testing and debugging.example.jsCopiedconst pureFunction1 = /* ... */; const pureFunction2 = /* ... */; const pureFunction3 = /* ... */; const composedFunction = _.flowRight(pureFunction1, pureFunction2, pureFunction3);
Start with the End in Mind:
When composing functions with
_.flowRight()
, think about the desired output first and work backward to determine the sequence of operations. This approach helps clarify the logic and structure of your code.example.jsCopiedconst finalResult = _.flowRight( generateData, processData, analyzeData );
Break Down Complex Operations:
Break down complex operations into smaller, reusable functions and compose them with
_.flowRight()
. This promotes code modularity, reusability, and maintainability.example.jsCopiedconst transformData = _.flowRight( processUserData, filterActiveUsers, normalizeUserData );
📚 Use Cases
Data Transformation Pipeline:
_.flowRight()
is ideal for creating data transformation pipelines where data flows through a series of processing steps, each performed by a separate function.example.jsCopiedconst dataTransformationPipeline = _.flowRight( parseJSON, sanitizeData, validateData, processCSV ); const processedData = dataTransformationPipeline(rawData);
Middleware Composition:
In web development,
_.flowRight()
can be used to compose middleware functions in frameworks like Express.js, allowing for modular and flexible request processing.example.jsCopiedconst authenticateUser = /* ... */ ; const authorizeUser = /* ... */ ; const validateRequest = /* ... */ ; const processRequest = /* ... */ ; const requestHandler = _.flowRight( processRequest, validateRequest, authorizeUser, authenticateUser );
Functional Programming Paradigm:
_.flowRight()
aligns with the principles of functional programming, enabling developers to create composable and reusable functions, which are fundamental concepts in functional programming.example.jsCopiedconst compose = (f, g) => x => f(g(x)); const combinedFunction = compose(add, multiply); console.log(combinedFunction(10)); // Output: 25
🎉 Conclusion
The _.flowRight()
method in Lodash empowers JavaScript developers to compose functions seamlessly, facilitating the creation of modular, reusable, and readable code. Whether you're building data transformation pipelines, composing middleware, or embracing the functional programming paradigm, _.flowRight()
offers a versatile tool to streamline your development process.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.flowRight()
method in your Lodash projects.
👨💻 Join our Community:
Author
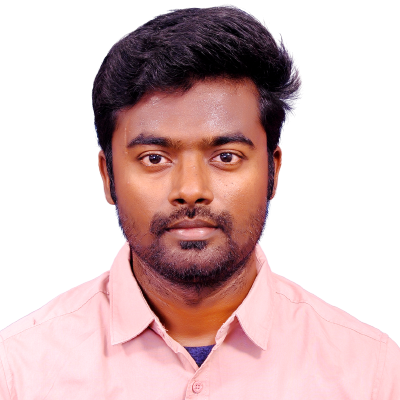
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
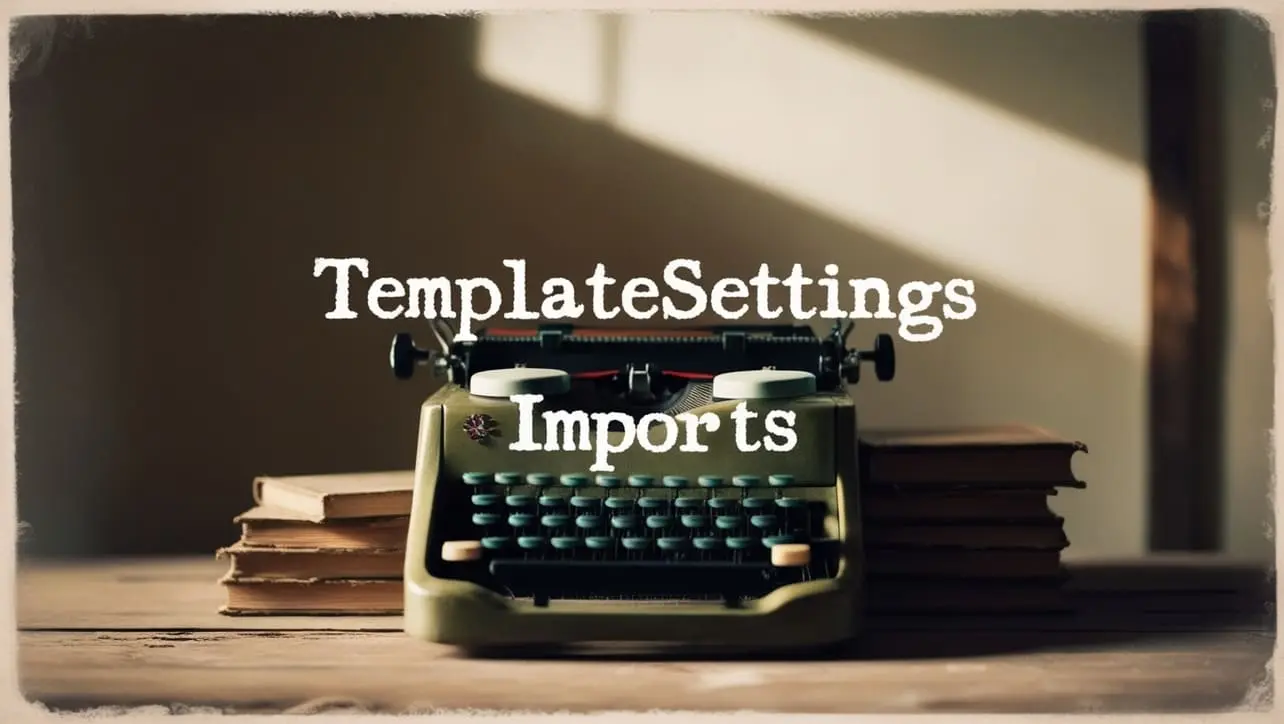
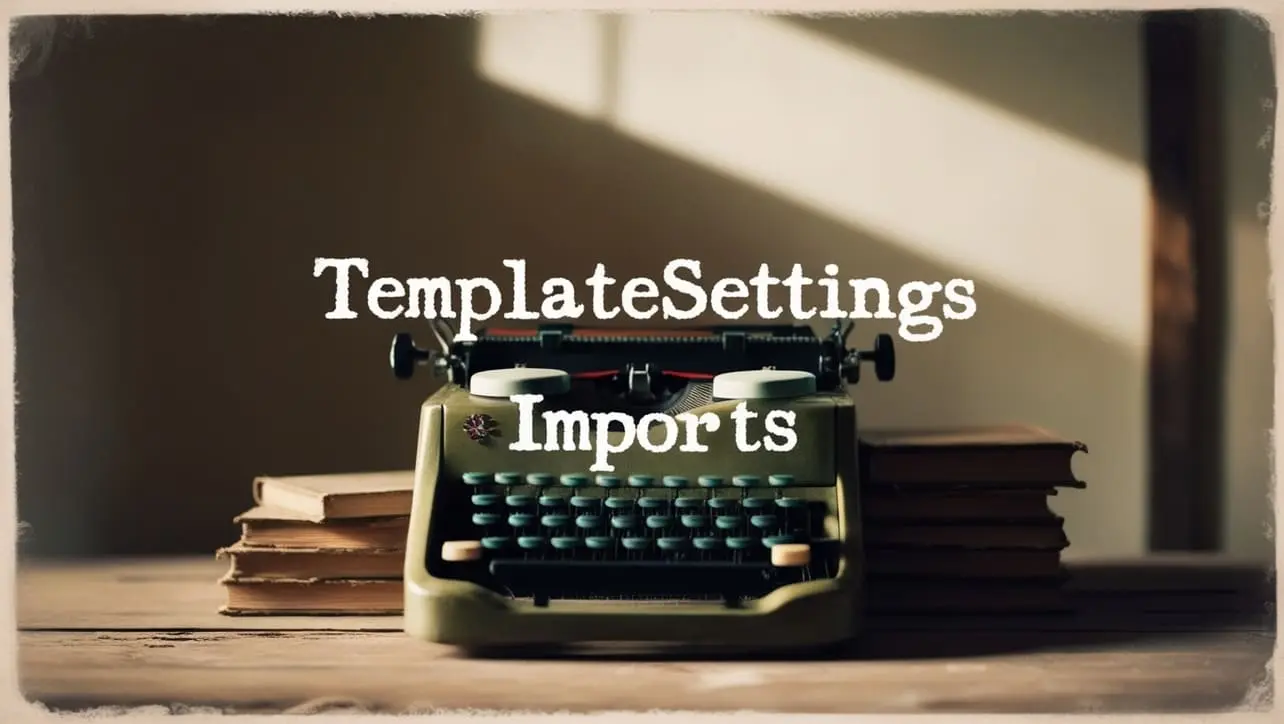
Lodash _.templateSettings.imports Property
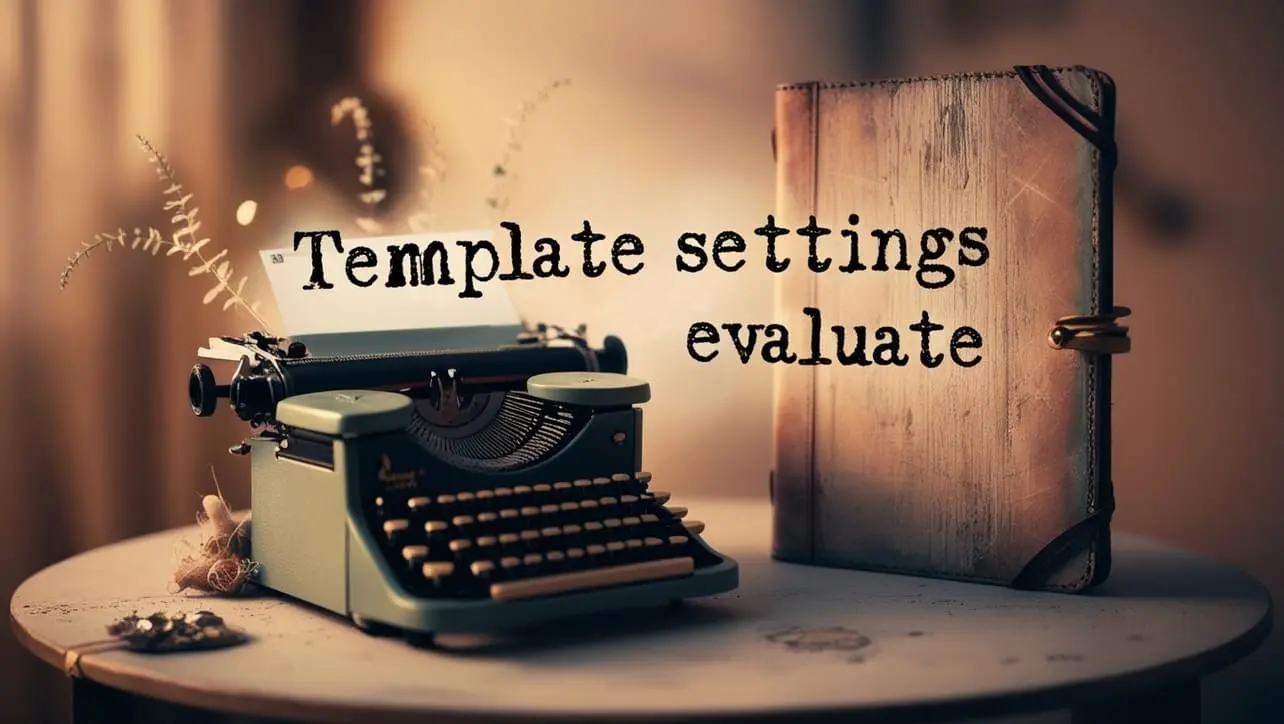
Lodash _.templateSettings.evaluate Property
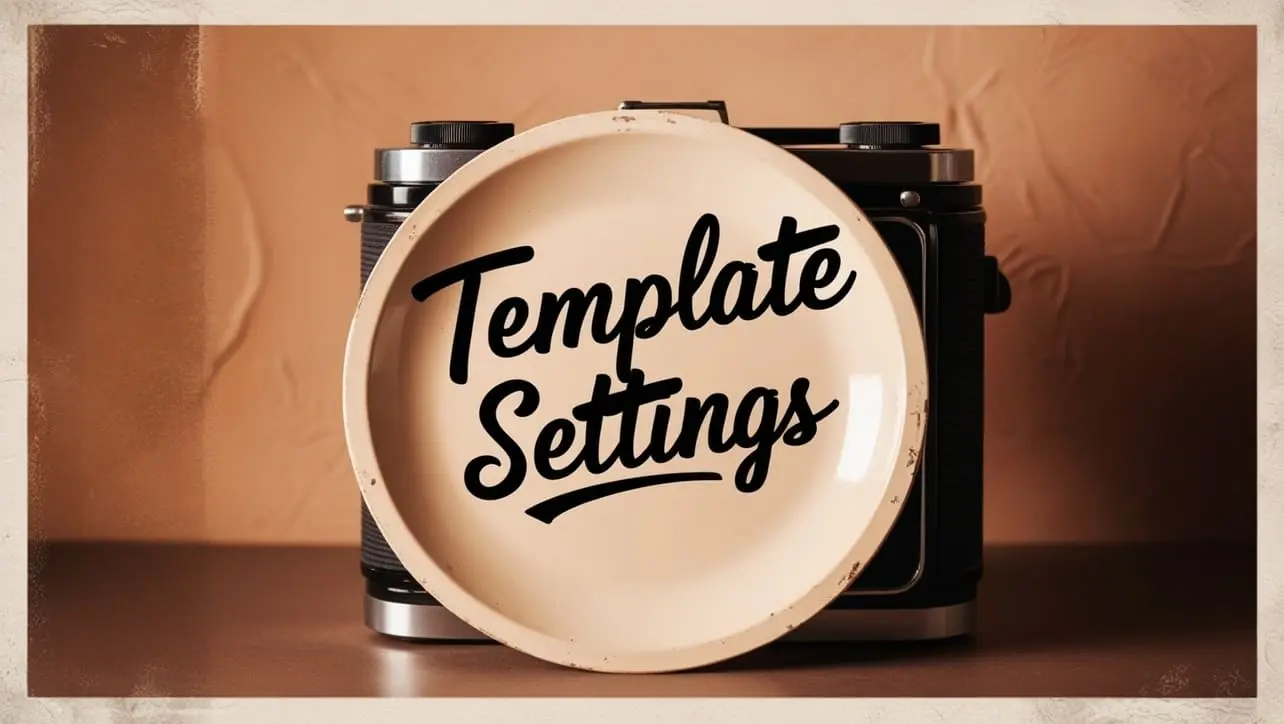
Lodash _.templateSettings Property
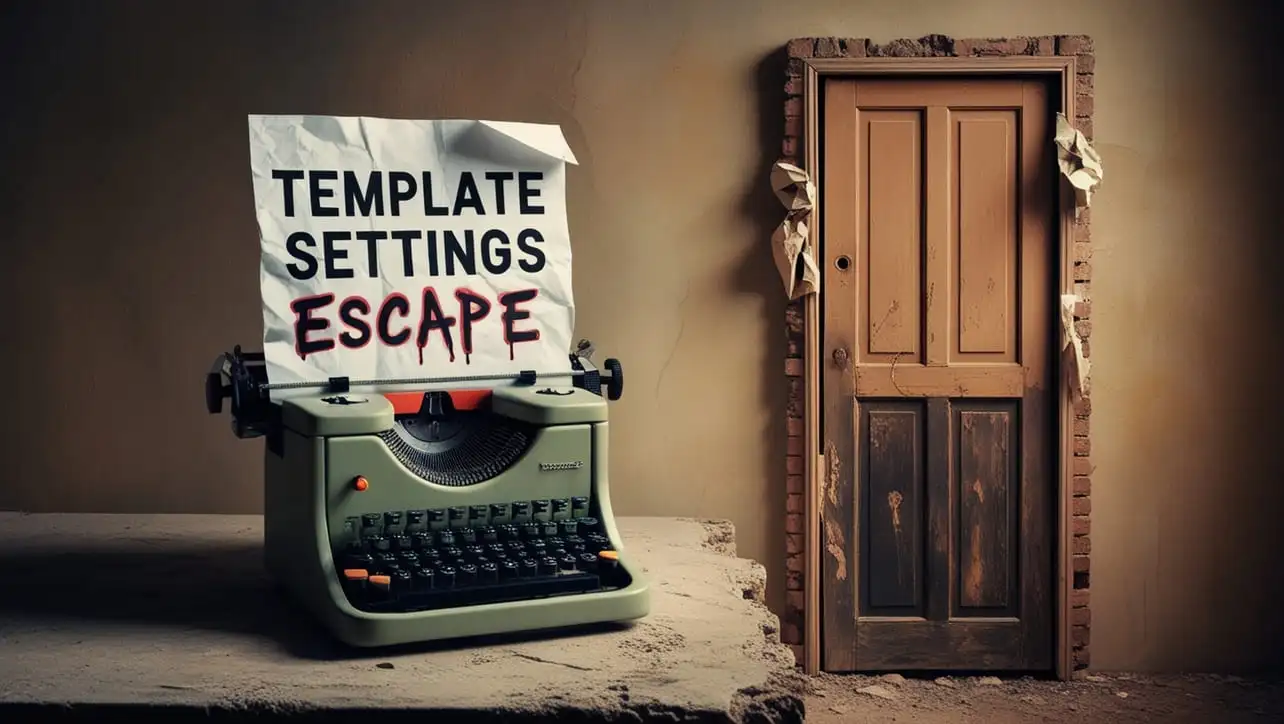
Lodash _.templateSettings.escape Property
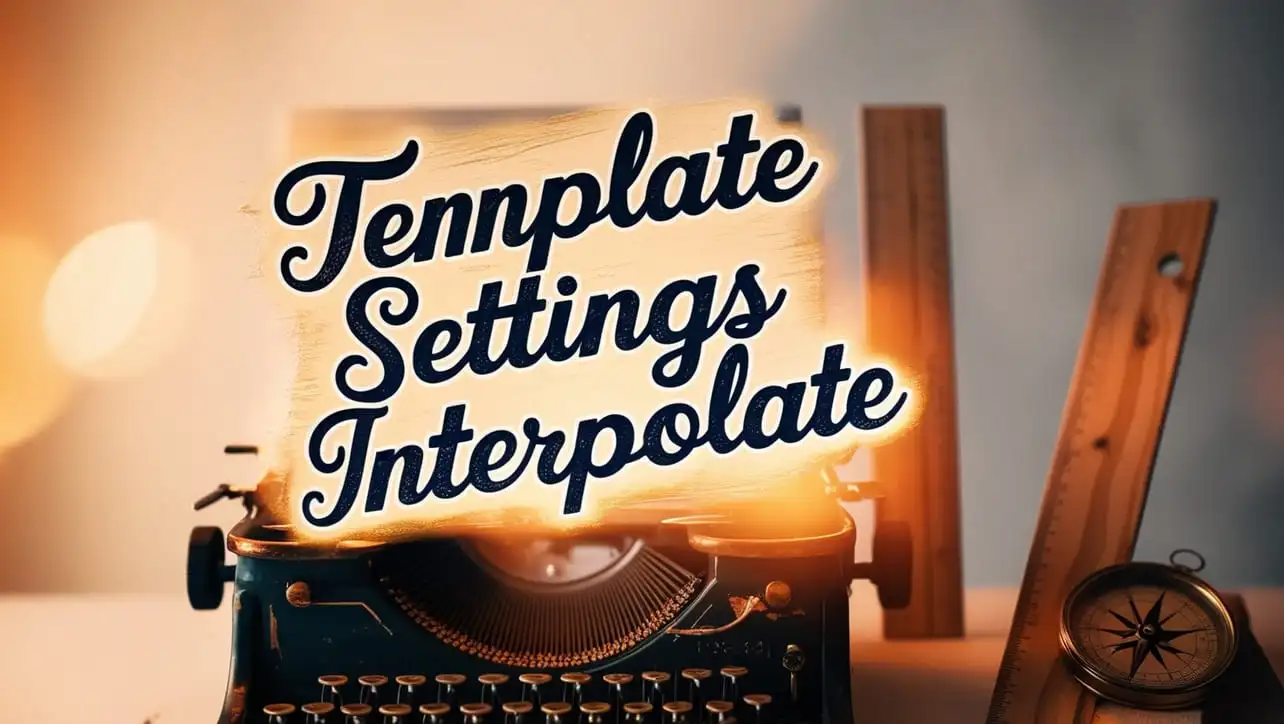
Lodash _.templateSettings.interpolate Property
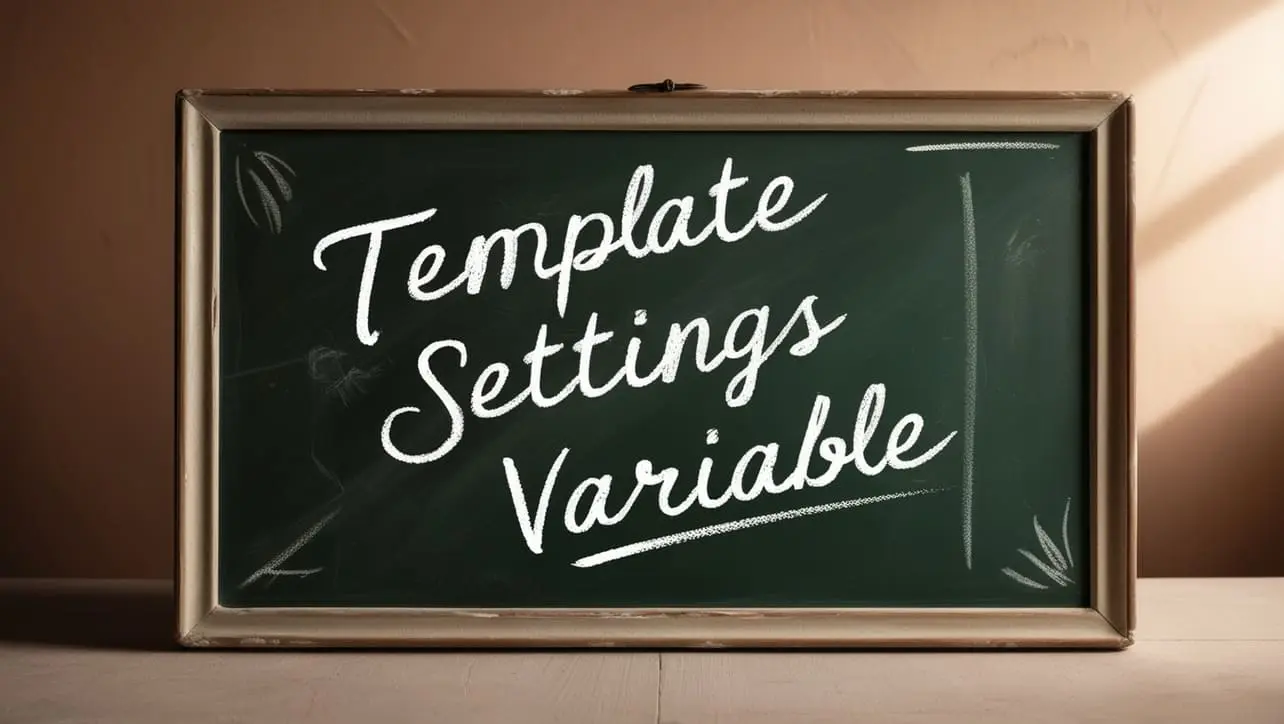
If you have any doubts regarding this article (Lodash _.flowRight() Util Method), please comment here. I will help you immediately.