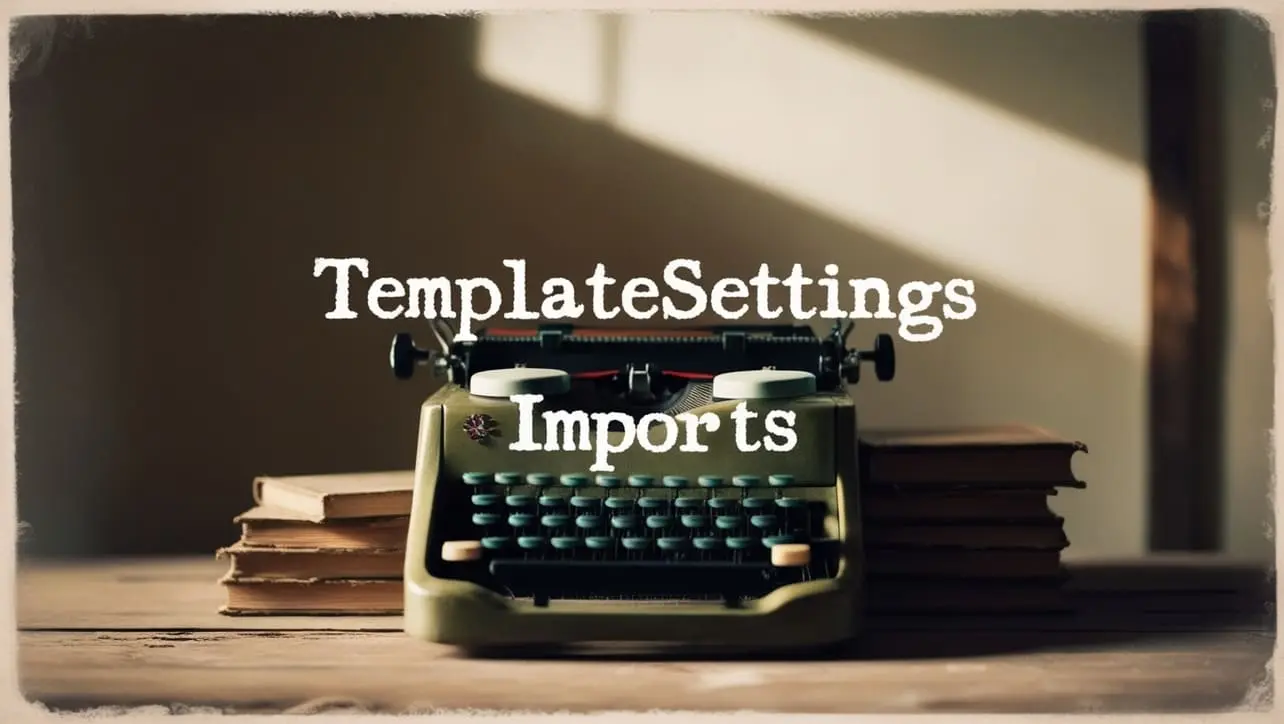
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.flow() Util Method
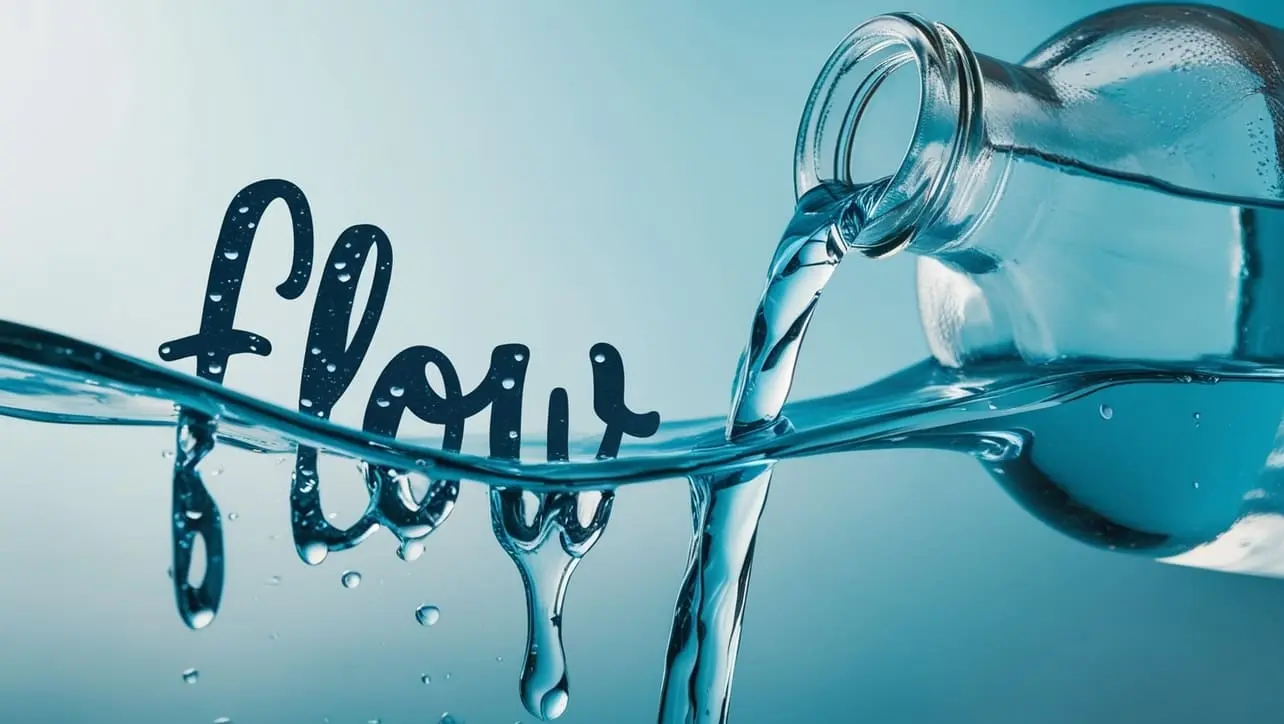
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, composing functions to perform complex operations in a concise and readable manner is often a challenge. Enter Lodash, a comprehensive utility library that offers a plethora of functions to streamline code organization and enhance developer productivity.
Among its arsenal of tools lies the _.flow()
method, a powerful utility for creating function pipelines and composing multiple functions into a single, cohesive unit.
🧠 Understanding _.flow() Method
The _.flow()
method in Lodash facilitates the creation of function pipelines by sequentially executing a series of functions, with each function passing its output to the next function in the pipeline. This enables developers to break down complex operations into smaller, composable functions, resulting in more modular and maintainable code.
💡 Syntax
The syntax for the _.flow()
method is straightforward:
_.flow([funcs])
- funcs: An array of functions to compose.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.flow()
method:
const _ = require('lodash');
// Define individual functions
const double = x => x * 2;
const square = x => x * x;
const subtractTen = x => x - 10;
// Compose a function pipeline using _.flow()
const pipeline = _.flow([double, square, subtractTen]);
// Apply the pipeline to a value
const result = pipeline(5);
console.log(result);
// Output: ((5 * 2) * 2) - 10 = 10
In this example, a function pipeline is created using _.flow()
to compose the functions double, square, and subtractTen. The resulting pipeline applies each function sequentially to its input value, producing the final result.
🏆 Best Practices
When working with the _.flow()
method, consider the following best practices:
Modular Function Definitions:
Define individual functions with single responsibilities to promote code reusability and maintainability. This allows you to compose functions seamlessly using
_.flow()
.example.jsCopiedconst add = (a, b) => a + b; const subtract = (a, b) => a - b; const multiply = (a, b) => a * b; const operationPipeline = _.flow([add, subtract, multiply]); const result = operationPipeline(10, 5); console.log(result);
Function Composition:
Compose functions in a logical order to ensure the desired operation sequence in the function pipeline. Consider the input/output requirements of each function to determine the optimal composition.
example.jsCopiedconst processString = str => str.trim(); const toLowerCase = str => str.toLowerCase(); const removeSpaces = str => str.replace(/\s/g, ''); const stringPipeline = _.flow([processString, toLowerCase, removeSpaces]); const inputString = ' Hello, World! '; const processedString = stringPipeline(inputString); console.log(processedString);
Error Handling:
Implement error handling within individual functions to handle unexpected scenarios gracefully. Consider incorporating error-checking mechanisms to ensure robustness in the function pipeline.
example.jsCopiedconst divide = (a, b) => { if(b === 0) { throw new Error('Division by zero'); } return a / b; }; const safeDivide = (a, b) => { try { return divide(a, b); } catch (error) { console.error(error.message); return NaN; } }; const calculationPipeline = _.flow([add, safeDivide, subtract]); const result = calculationPipeline(10, 0); console.log(result);
📚 Use Cases
Data Transformation:
Use
_.flow()
to create function pipelines for transforming data through a series of processing steps. This enables you to apply a sequence of operations to input data efficiently.example.jsCopiedconst parseJSON = json => JSON.parse(json); const filterValues = data => data.filter(value => value > 0); const calculateAverage = data => data.reduce((acc, curr) => acc + curr, 0) / data.length; const dataPipeline = _.flow([parseJSON, filterValues, calculateAverage]); const jsonData = '[1, 2, 3, -4, 5]'; const average = dataPipeline(jsonData); console.log(average);
Functional Programming:
Incorporate
_.flow()
into functional programming paradigms to compose pure functions and avoid mutable state. This promotes code clarity and facilitates reasoning about program behavior.example.jsCopiedconst pureFunctions = [ x => x + 1, x => x * 2, x => x - 3 ]; const composedFunction = _.flow(pureFunctions); const result = composedFunction(5); console.log(result);
Middleware Composition:
Utilize
_.flow()
to compose middleware functions in web development frameworks like Express.js. This allows you to define middleware chains for handling HTTP requests sequentially.example.jsCopiedconst express = require('express'); const _ = require('lodash'); const app = express(); const middleware1 = (req, res, next) => { // Middleware logic next(); }; const middleware2 = (req, res, next) => { // Middleware logic next(); }; const middleware3 = (req, res, next) => { // Middleware logic next(); }; app.get('/', _.flow([middleware1, middleware2, middleware3]), (req, res) => { // Route handler logic }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
🎉 Conclusion
The _.flow()
method in Lodash empowers developers to create function pipelines and compose multiple functions into cohesive units. By leveraging function composition, error handling, and modular design principles, _.flow()
enhances code readability, maintainability, and performance. Whether used for data transformation, functional programming, or middleware composition, _.flow()
serves as a valuable tool in the JavaScript developer's toolkit.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.flow()
method in your Lodash projects.
👨💻 Join our Community:
Author
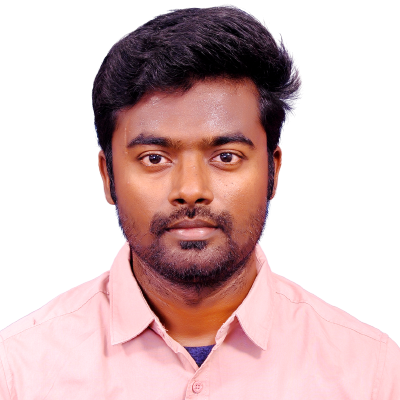
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
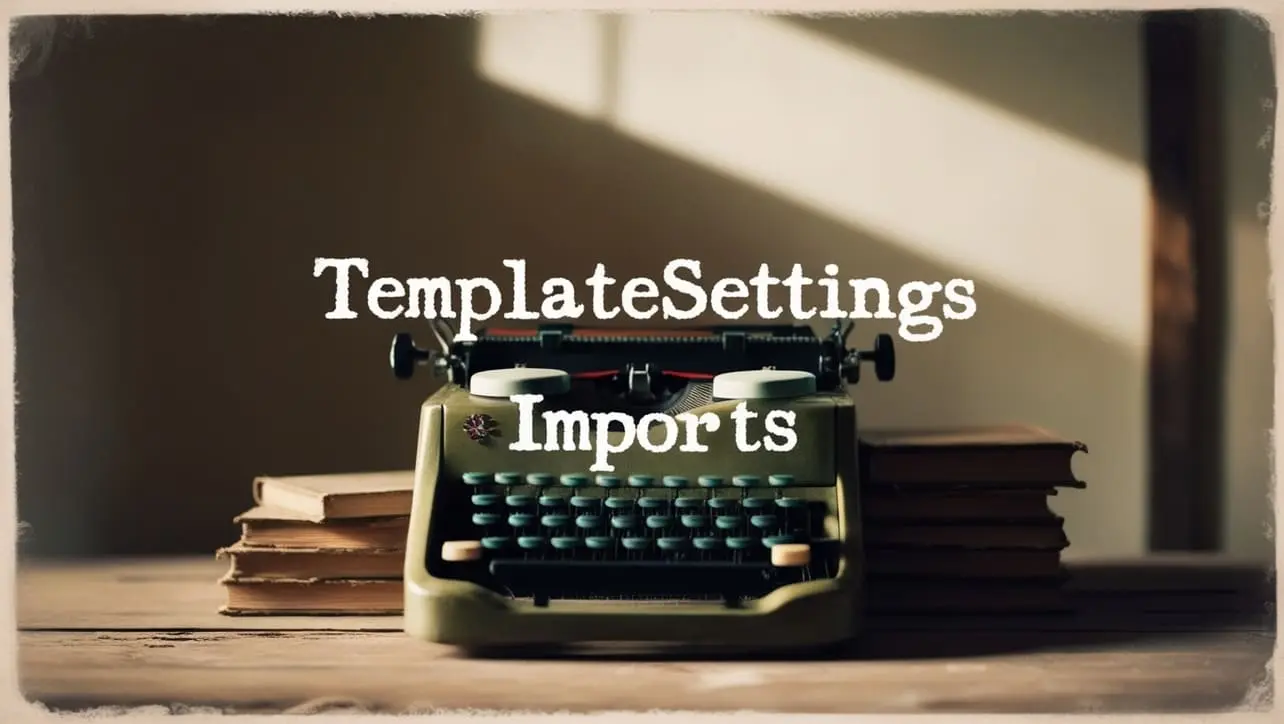
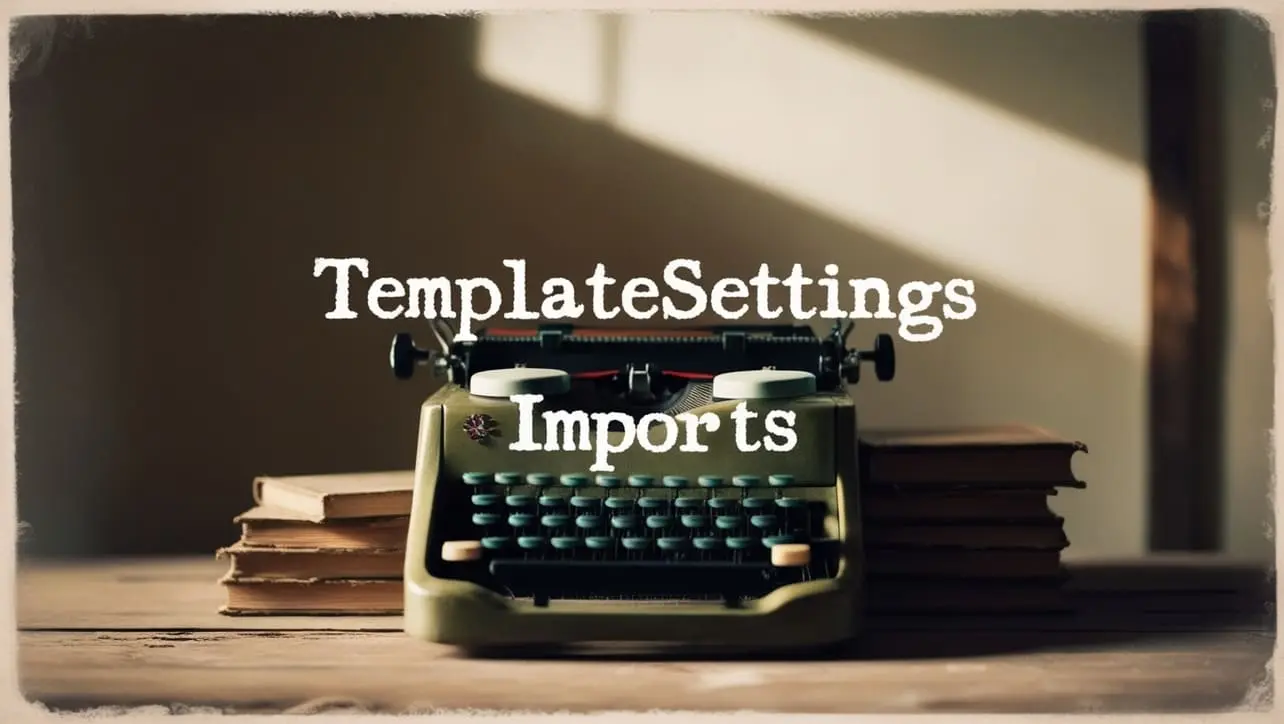
Lodash _.templateSettings.imports Property
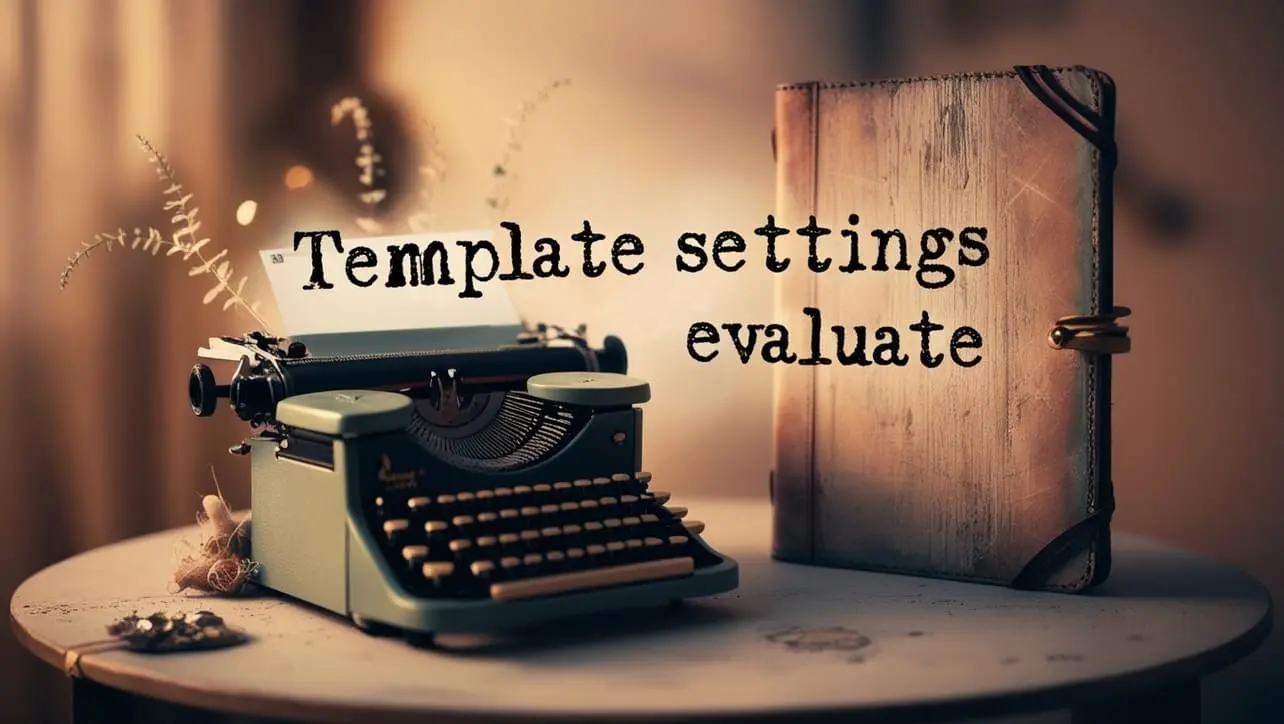
Lodash _.templateSettings.evaluate Property
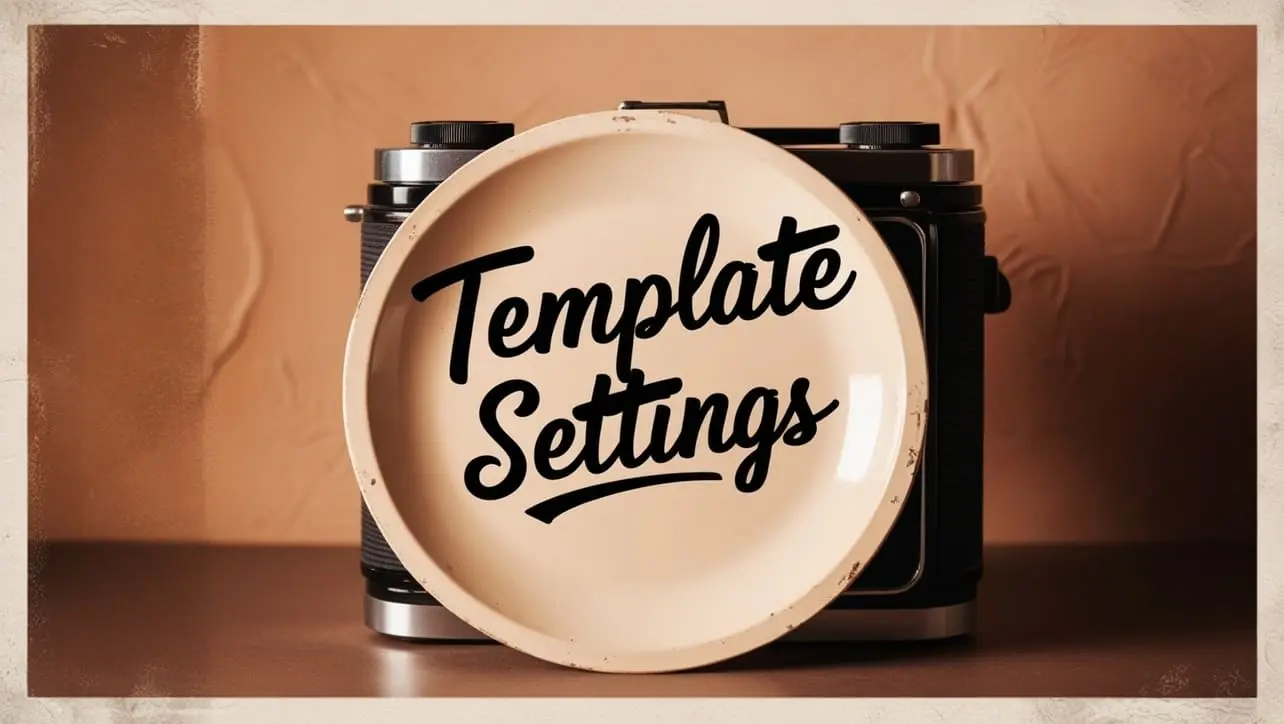
Lodash _.templateSettings Property
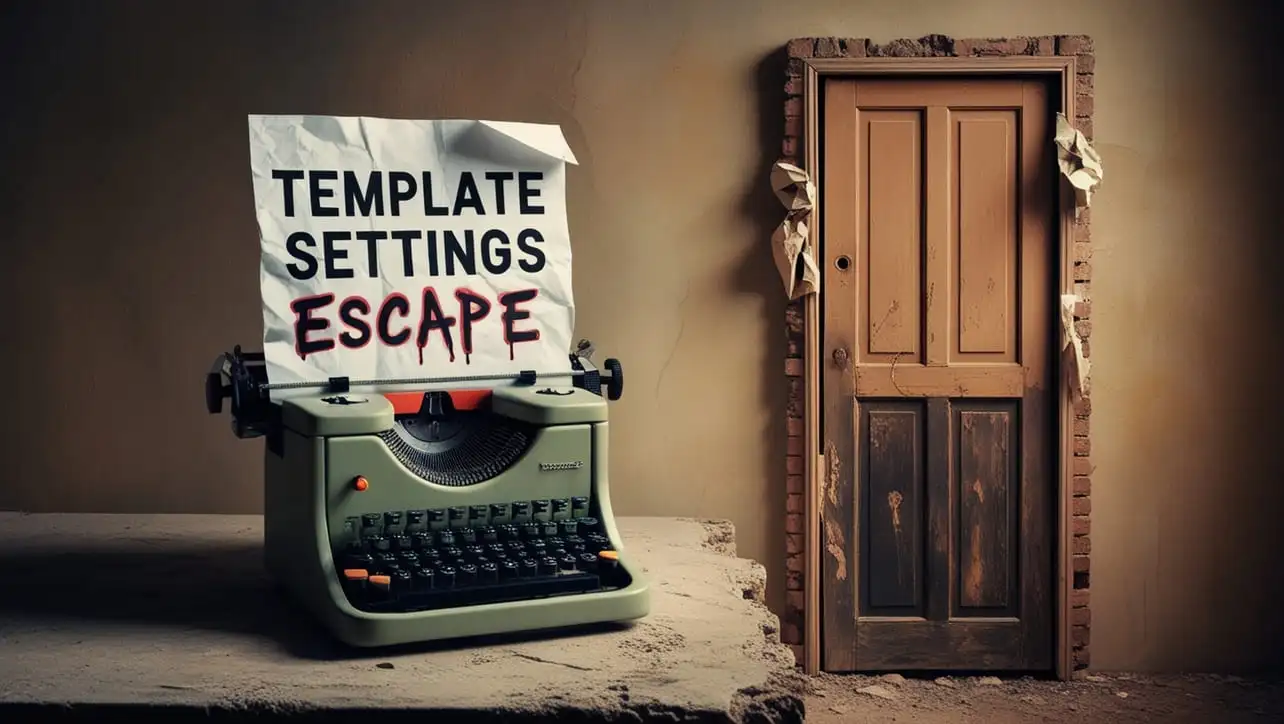
Lodash _.templateSettings.escape Property
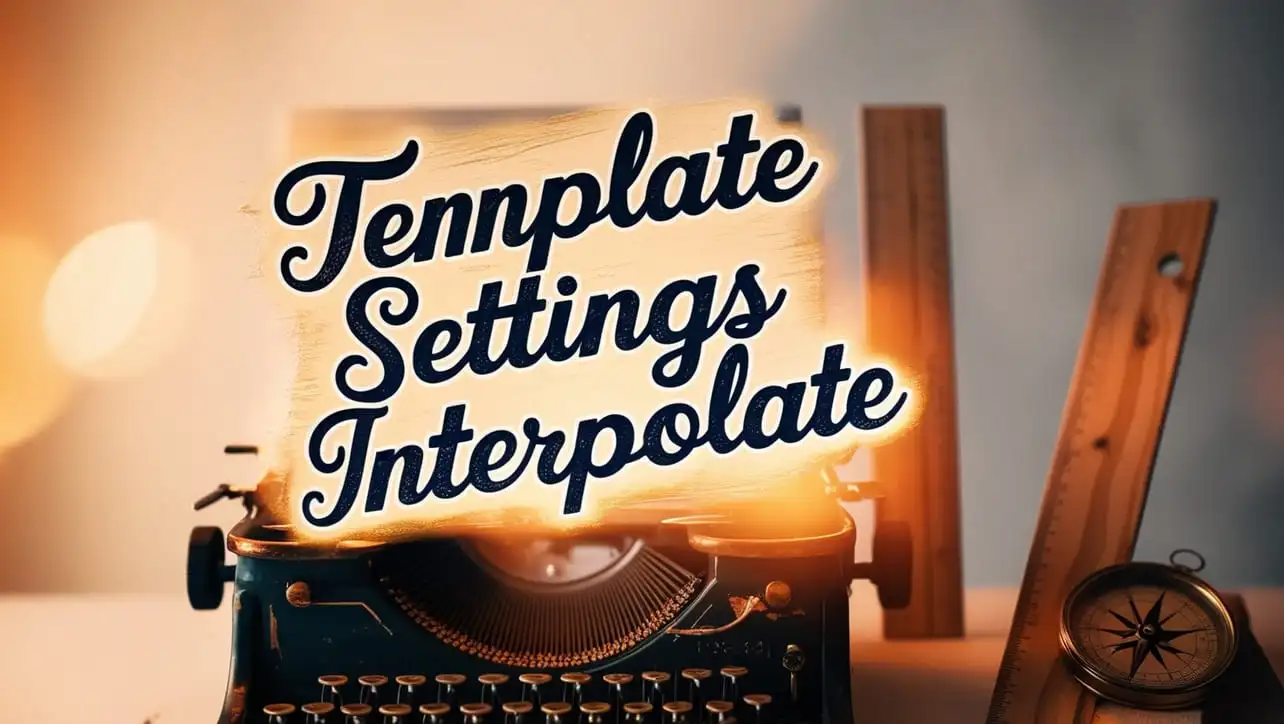
Lodash _.templateSettings.interpolate Property
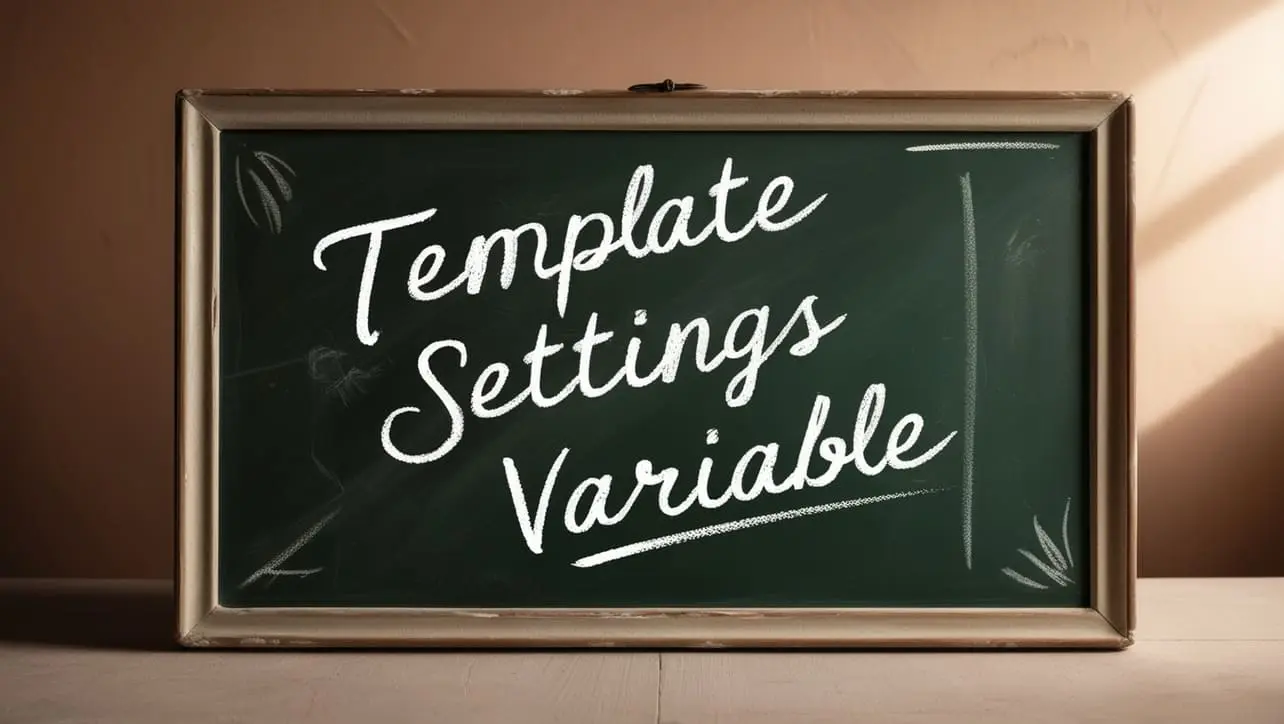
If you have any doubts regarding this article (Lodash _.flow() Util Method), please comment here. I will help you immediately.