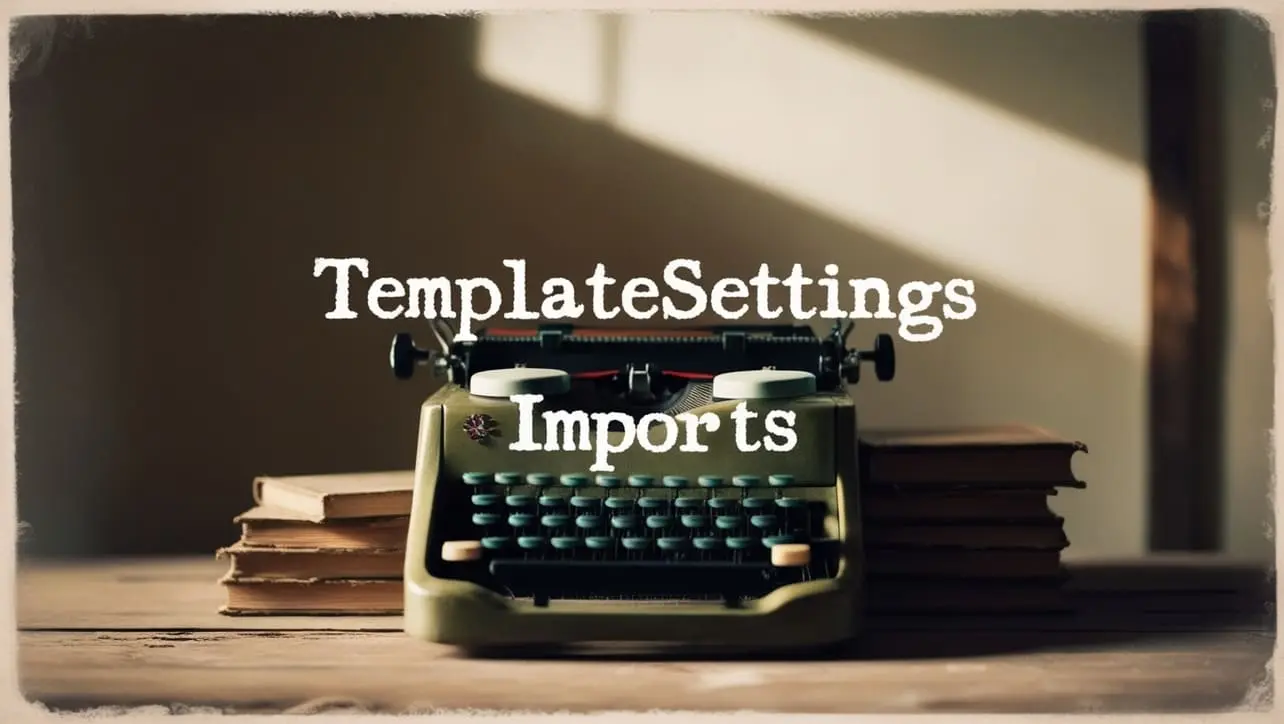
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.conforms() Util Method
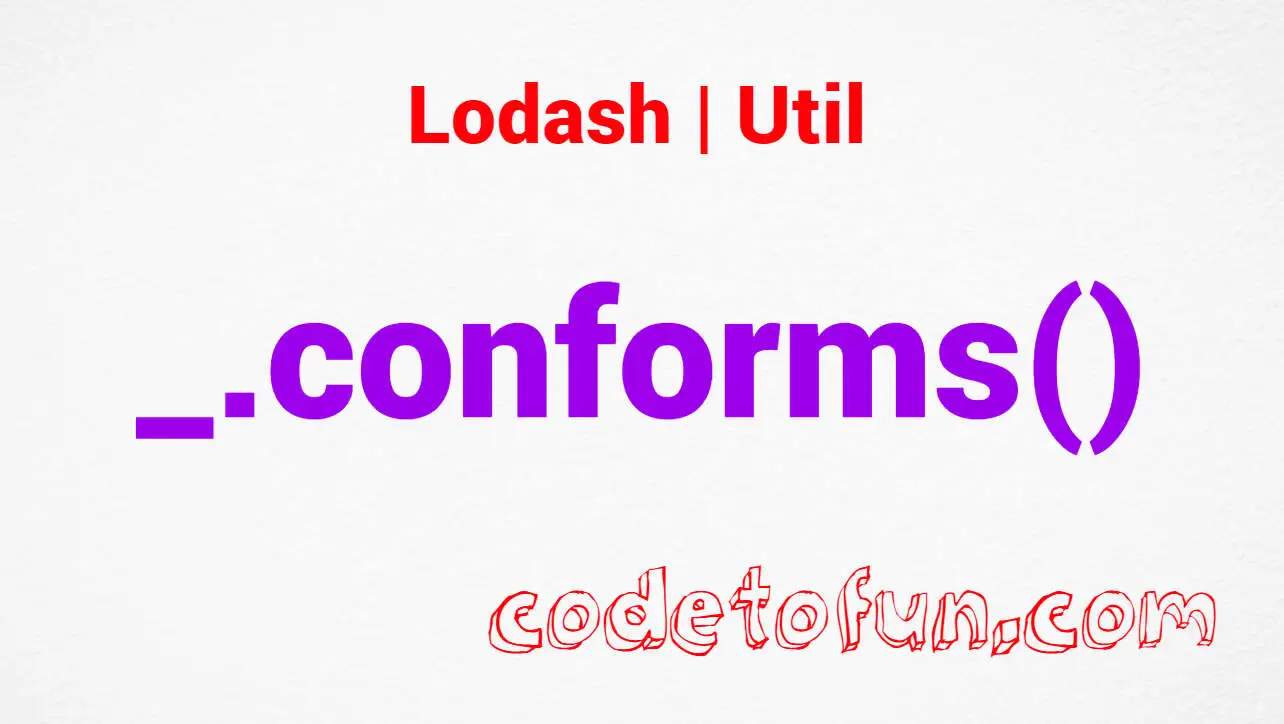
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, working with data often involves validating objects against specific criteria. Lodash, a popular utility library, offers the _.conforms()
method, which enables developers to create custom validation functions for objects.
This method provides a flexible and concise way to ensure that objects conform to desired shapes or conditions, enhancing code readability and maintainability.
🧠 Understanding _.conforms() Method
The _.conforms()
method in Lodash creates a predicate function that checks if an object conforms to a provided template object. This template object defines the shape and properties that the target object must match for validation to succeed. _.conforms()
returns a new function that evaluates objects against the specified criteria, making it a powerful tool for data validation and filtering.
💡 Syntax
The syntax for the _.conforms()
method is straightforward:
_.conforms(source)
- source: The template object defining the validation criteria.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.conforms()
method:
const _ = require('lodash');
// Define a template object for validation
const template = {
name: value => typeof value === 'string',
age: value => typeof value === 'number' && value >= 18
};
// Create a predicate function using _.conforms()
const validatePerson = _.conforms(template);
// Test objects against the validation criteria
console.log(validatePerson({
name: 'John',
age: 25
}));
// Output: true
console.log(validatePerson({
name: 'Alice',
age: '30'
}));
// Output: false
In this example, validatePerson is a predicate function created with _.conforms()
, which checks if objects meet the specified validation criteria defined in the template object.
🏆 Best Practices
When working with the _.conforms()
method, consider the following best practices:
Define Clear Validation Criteria:
Clearly define the validation criteria in the template object passed to
_.conforms()
. This ensures that the predicate function accurately evaluates objects against the desired conditions.example.jsCopiedconst template = { name: value => typeof value === 'string' && value.length > 0, email: value => typeof value === 'string' && /^[^\s@]+@[^\s@]+\.[^\s@]+$/.test(value) };
Handle Complex Validation Logic:
Leverage the flexibility of
_.conforms()
to handle complex validation logic by defining custom validation functions for each property in the template object.example.jsCopiedconst template = { username: value => typeof value === 'string' && value.length >= 5 && value.length <= 20, password: value => typeof value === 'string' && value.length >= 8, confirmPassword: (value, object) => value === object.password };
Reusability and Modularity:
Encapsulate validation logic into reusable predicate functions created with
_.conforms()
, promoting modularity and code reusability across different parts of your application.example.jsCopiedconst validateUserCredentials = _.conforms({ username: value => typeof value === 'string' && value.length >= 5 && value.length <= 20, password: value => typeof value === 'string' && value.length >= 8 }); const validateUserProfile = _.conforms({ name: value => typeof value === 'string' && value.length > 0, email: value => typeof value === 'string' && /^[^\s@]+@[^\s@]+\.[^\s@]+$/.test(value) });
📚 Use Cases
Form Validation:
Use
_.conforms()
to validate form inputs against predefined criteria, ensuring that user-submitted data meets specific requirements before submission.example.jsCopiedconst validateLoginForm = _.conforms({ username: value => typeof value === 'string' && value.length >= 5 && value.length <= 20, password: value => typeof value === 'string' && value.length >= 8 }); const formData = /* ...extract form data... */; if (validateLoginForm(formData)) { // Proceed with form submission } else { // Display error messages to the user }
API Request Validation:
Validate request payloads sent to APIs to ensure that they adhere to expected formats and data types, preventing malformed requests from causing errors in the backend.
example.jsCopiedconst validateRequestBody = _.conforms({ title: value => typeof value === 'string' && value.length > 0, content: value => typeof value === 'string' && value.length > 0 }); app.post('/createPost', (req, res) => { if (validateRequestBody(req.body)) { // Process the request } else { res.status(400).send('Invalid request body'); } });
Data Filtering:
Filter arrays of objects based on specific criteria using
_.conforms()
to select only the objects that match the desired properties or conditions.example.jsCopiedconst users = [ { name: 'John', age: 25, role: 'admin' }, { name: 'Alice', age: 30, role: 'user' }, { name: 'Bob', age: 20, role: 'user' } ]; const filterCriteria = { age: value => value >= 25, role: 'user' }; const filteredUsers = users.filter(_.conforms(filterCriteria)); console.log(filteredUsers); // Output: [{ name: 'Alice', age: 30, role: 'user' }]
🎉 Conclusion
The _.conforms()
method in Lodash offers a convenient and versatile solution for object validation and filtering in JavaScript applications. By defining clear validation criteria and leveraging the flexibility of predicate functions, developers can ensure data integrity and streamline data processing workflows.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.conforms()
method in your Lodash projects.
👨💻 Join our Community:
Author
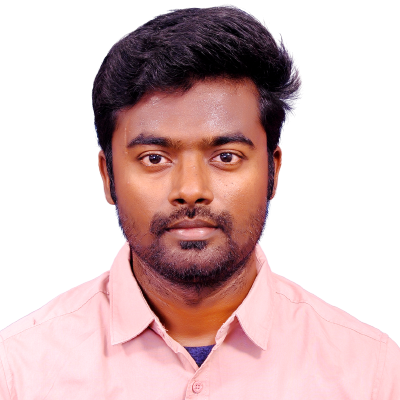
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
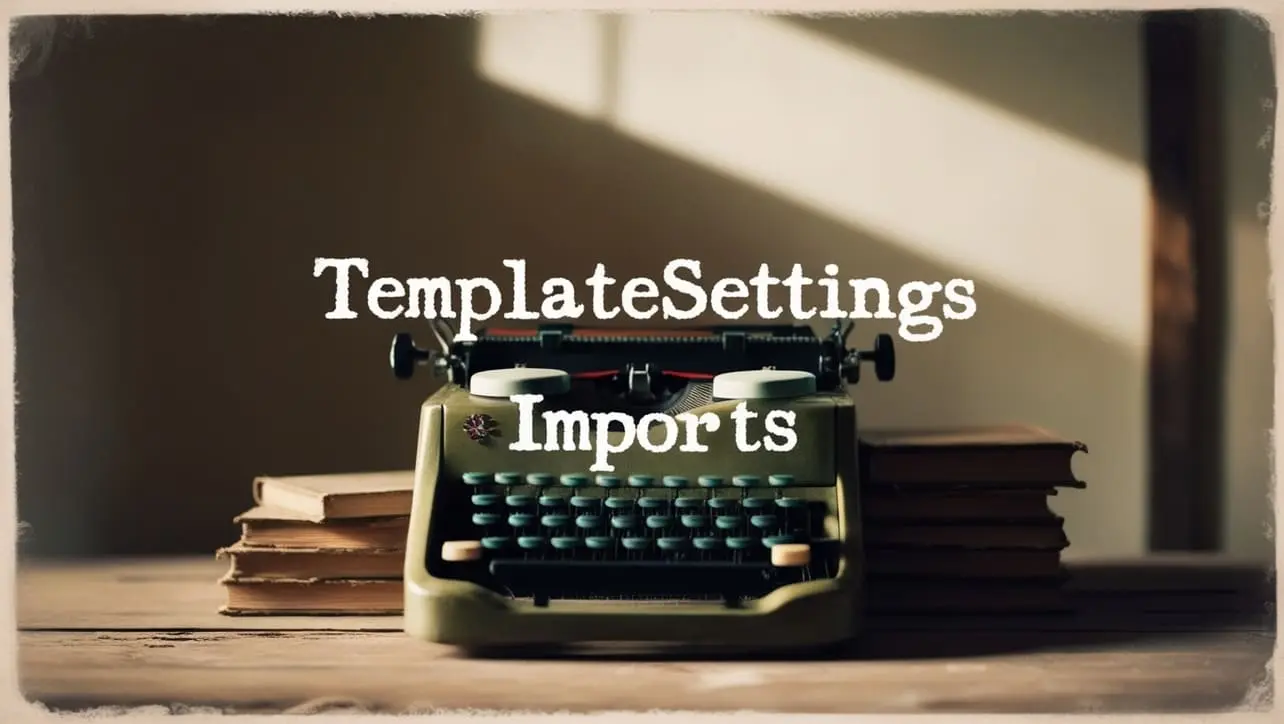
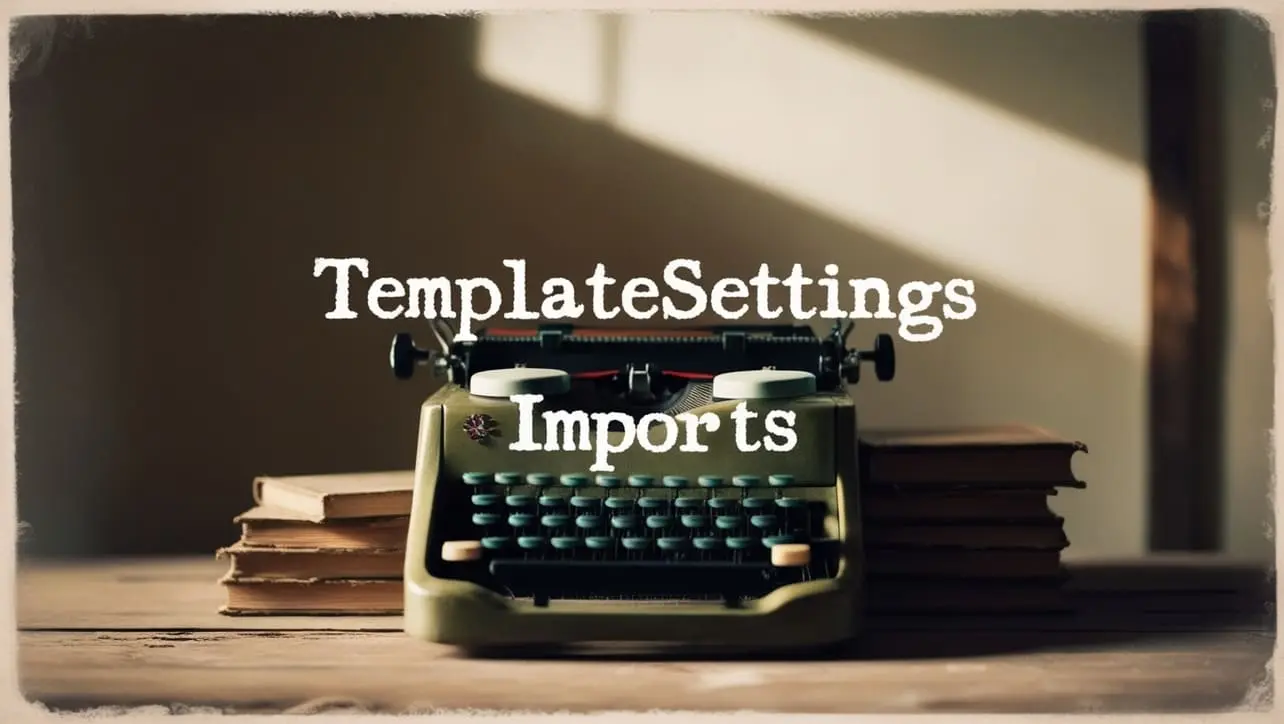
Lodash _.templateSettings.imports Property
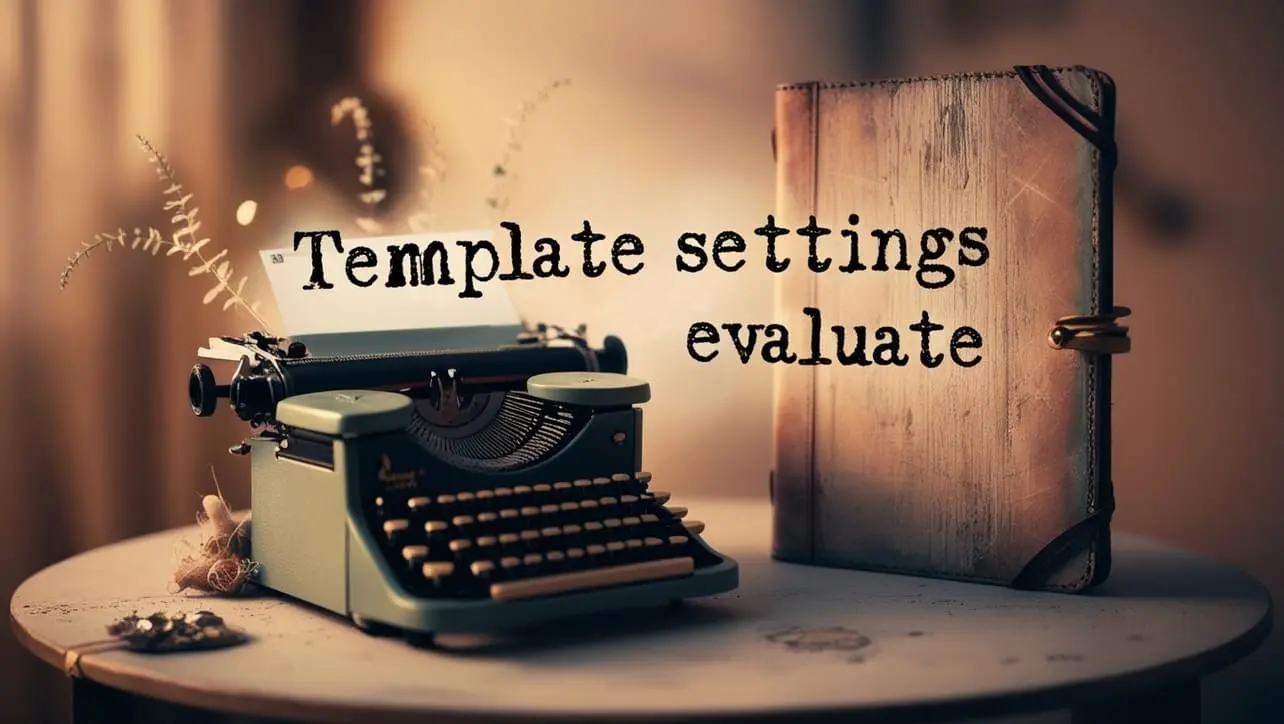
Lodash _.templateSettings.evaluate Property
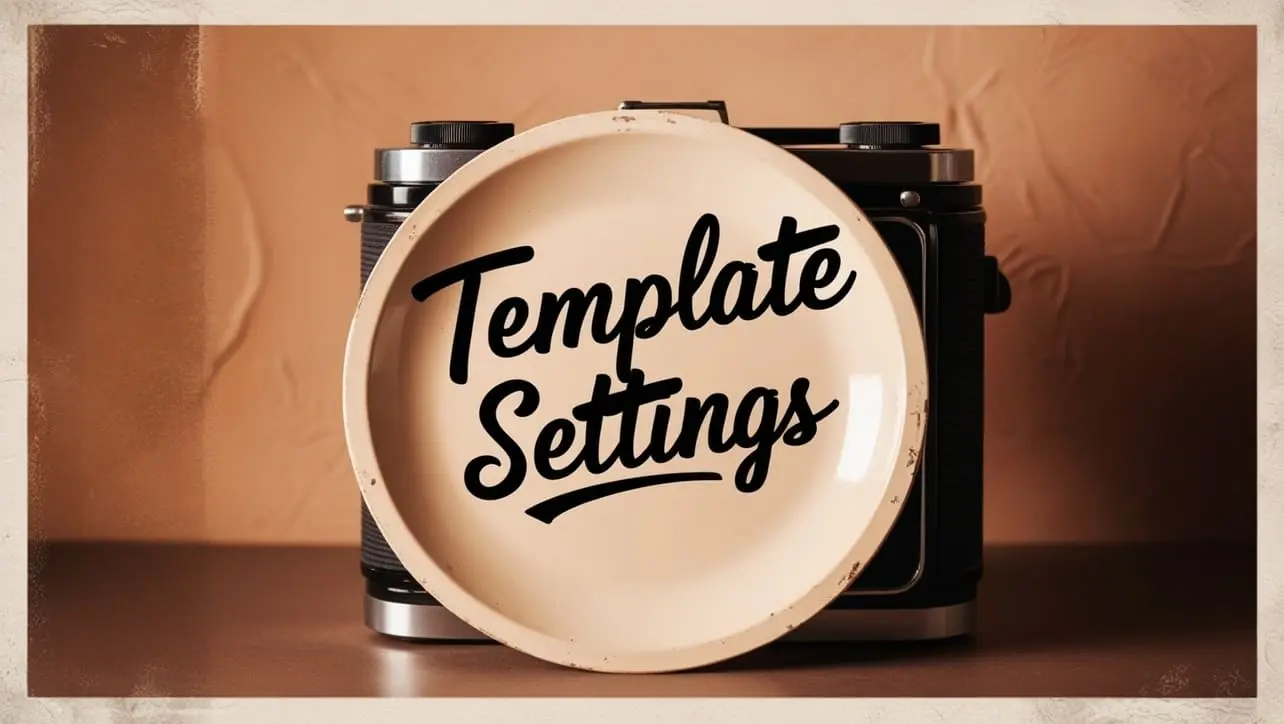
Lodash _.templateSettings Property
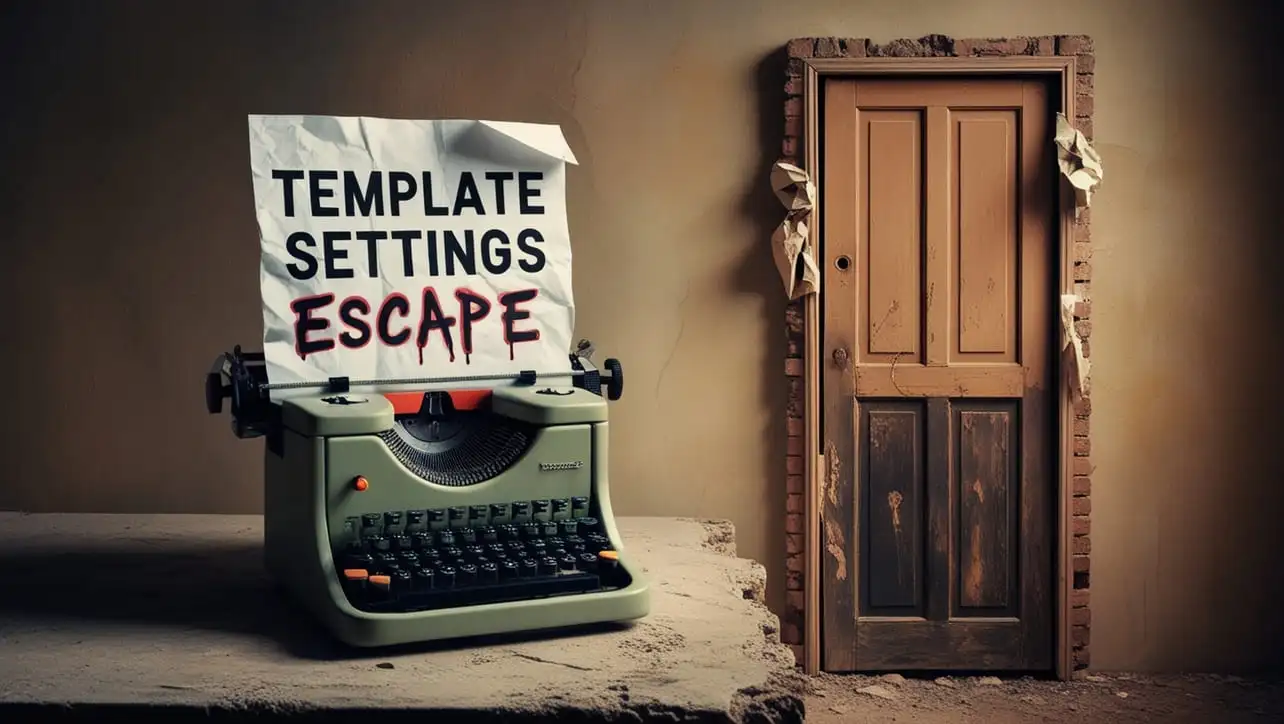
Lodash _.templateSettings.escape Property
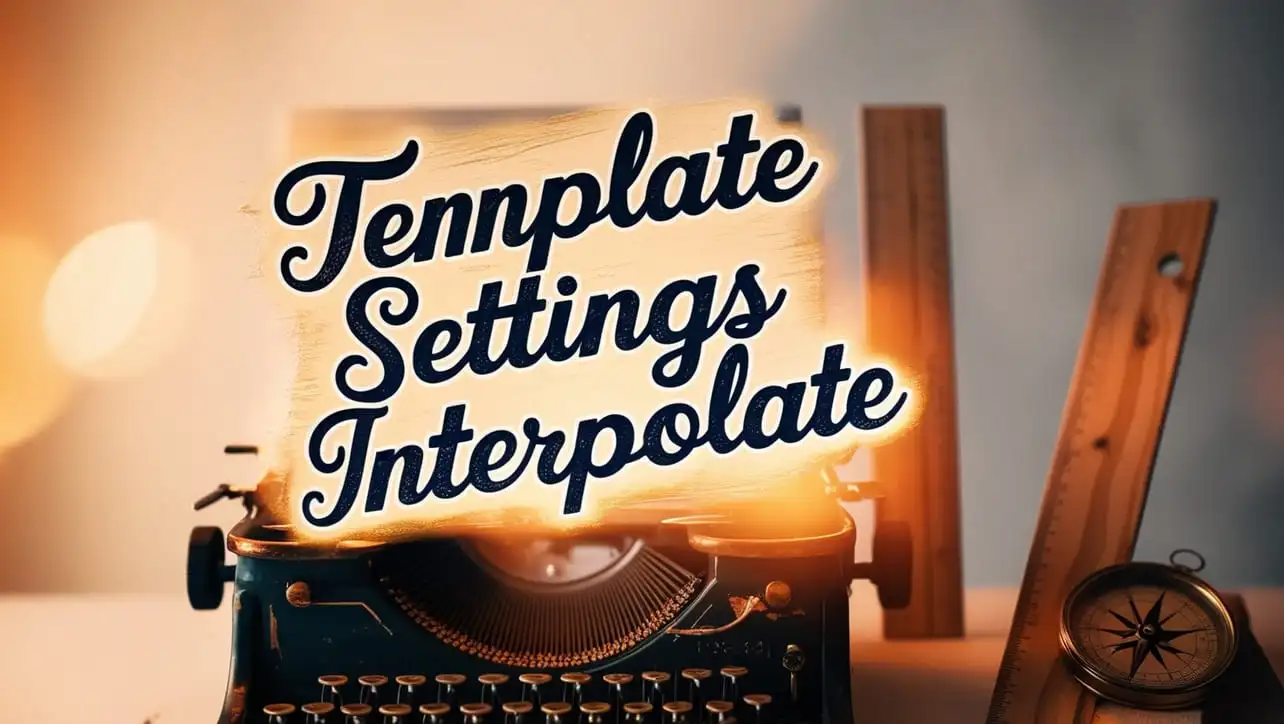
Lodash _.templateSettings.interpolate Property
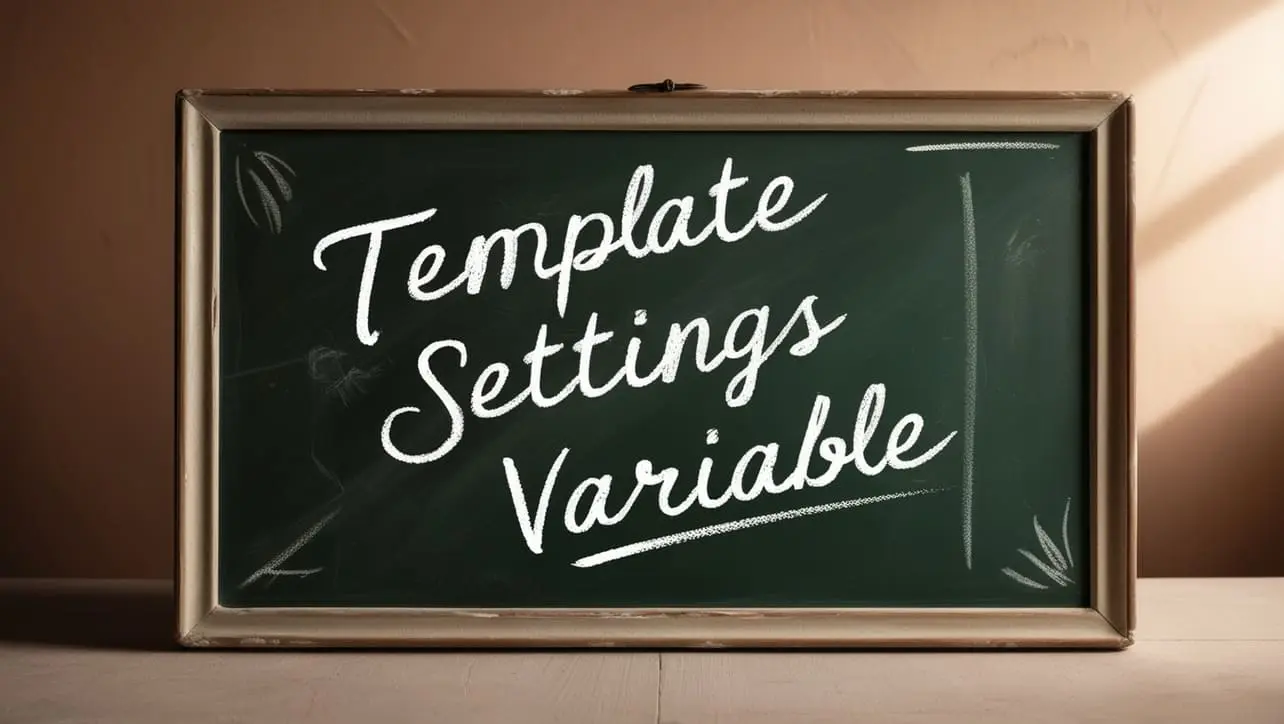
If you have any doubts regarding this article (Lodash _.conforms() Util Method), please comment here. I will help you immediately.