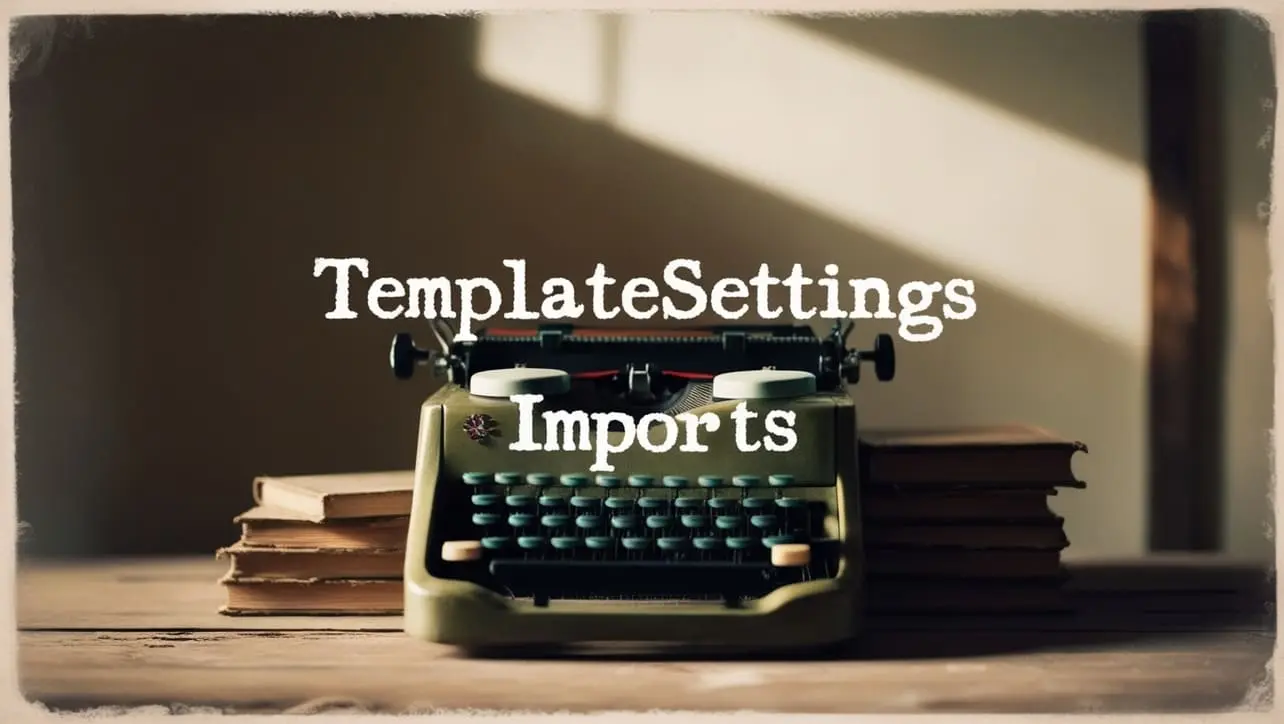
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.bindAll() Util Method
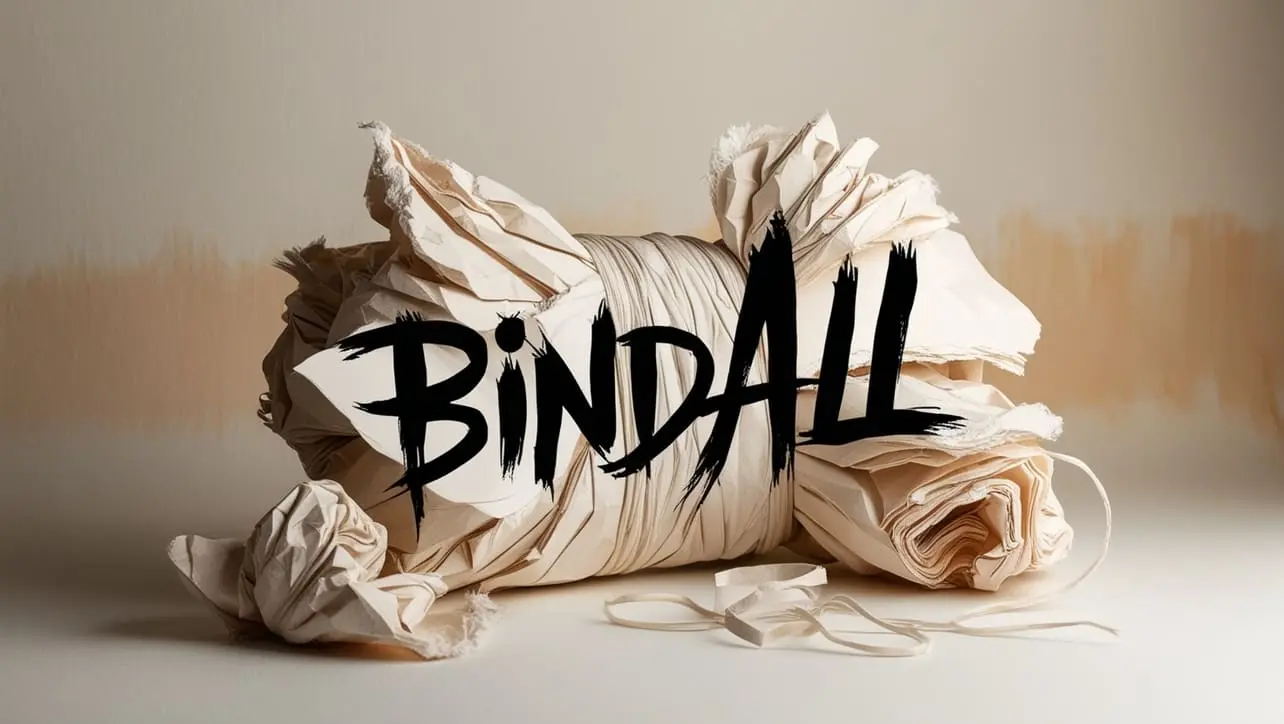
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, managing the context (or this value) of functions can sometimes be challenging, especially in object-oriented programming. Lodash, a popular utility library, offers a solution to this problem with its _.bindAll()
method. This method allows developers to bind specified methods of an object to the object itself, ensuring that the this value remains consistent within those methods.
This capability enhances code readability and maintainability, making _.bindAll()
a valuable tool for JavaScript developers.
🧠 Understanding _.bindAll() Method
The _.bindAll()
method in Lodash is used to bind specified methods of an object to the object itself. By doing so, it ensures that when these methods are invoked, the this value within them refers to the object to which they belong. This prevents unexpected behavior and enables cleaner and more predictable code.
💡 Syntax
The syntax for the _.bindAll()
method is straightforward:
_.bindAll(object, methodNames)
- object: The object containing the methods to bind.
- methodNames: The names of the methods to bind.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.bindAll()
method:
const _ = require('lodash');
const obj = {
name: 'John',
greet() {
console.log(`Hello, ${this.name}!`);
}
};
_.bindAll(obj, 'greet');
const boundGreet = obj.greet;
boundGreet(); // Output: Hello, John!
In this example, the greet method of the obj object is bound using _.bindAll()
. Consequently, even when the greet method is assigned to boundGreet and invoked independently, the this value still references obj, resulting in the expected output.
🏆 Best Practices
When working with the _.bindAll()
method, consider the following best practices:
Specify Methods Carefully:
Be selective when specifying methods to bind with
_.bindAll()
. Binding unnecessary methods may lead to performance overhead and unnecessary complexity in the codebase.example.jsCopied_.bindAll(obj, ['method1', 'method2']);
Consider Object Composition:
In cases where an object contains methods from multiple sources (such as mixins or inheritance), exercise caution when using
_.bindAll()
. Consider object composition patterns to manage method bindings more effectively.example.jsCopiedconst obj = Object.assign({}, mixin1, mixin2); _.bindAll(obj, 'methodFromMixin1', 'methodFromMixin2');
Performance Considerations:
Avoid overusing
_.bindAll()
in performance-critical code paths. While_.bindAll()
provides convenience, excessive use may impact performance, especially in applications with high-frequency method invocations.example.jsCopiedconst obj = { method1() { /* ... */ }, method2() { /* ... */ }, // More methods... }; _.bindAll(obj);
📚 Use Cases
Event Handlers:
When defining event handlers within an object,
_.bindAll()
ensures that the handlers retain the correct context, preventing potential bugs related to the this value.example.jsCopiedconst obj = { init() { document.addEventListener('click', this.handleClick); }, handleClick(event) { console.log(`Clicked at (${event.clientX}, ${event.clientY})`); } }; _.bindAll(obj, 'handleClick'); obj.init();
React Component Methods:
In React components,
_.bindAll()
can be used to bind event handlers and lifecycle methods, ensuring that they always have access to the component instance.example.jsCopiedclass MyComponent extends React.Component { constructor(props) { super(props); _.bindAll(this, 'handleClick'); } handleClick() { console.log('Button clicked!'); } render() { return <button onClick={this.handleClick}>Click me</button>; } }
Functional Programming:
In functional programming paradigms,
_.bindAll()
can be employed to bind utility methods or helpers, enhancing code organization and readability.example.jsCopiedconst mathUtils = { double(n) { return n * 2; }, triple(n) { return n * 3; } }; _.bindAll(mathUtils); console.log(mathUtils.double(3)); // Output: 6
🎉 Conclusion
The _.bindAll()
method in Lodash provides a convenient mechanism for binding object methods to their containing object. By ensuring consistent context within bound methods, it helps prevent common bugs related to the this value in JavaScript. Whether you're building complex applications or following functional programming principles, _.bindAll()
serves as a valuable tool for maintaining code integrity and readability.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.bindAll()
method in your Lodash projects.
👨💻 Join our Community:
Author
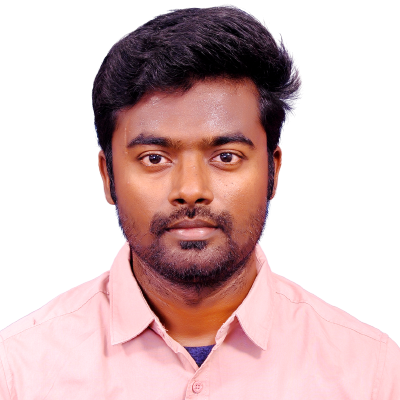
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
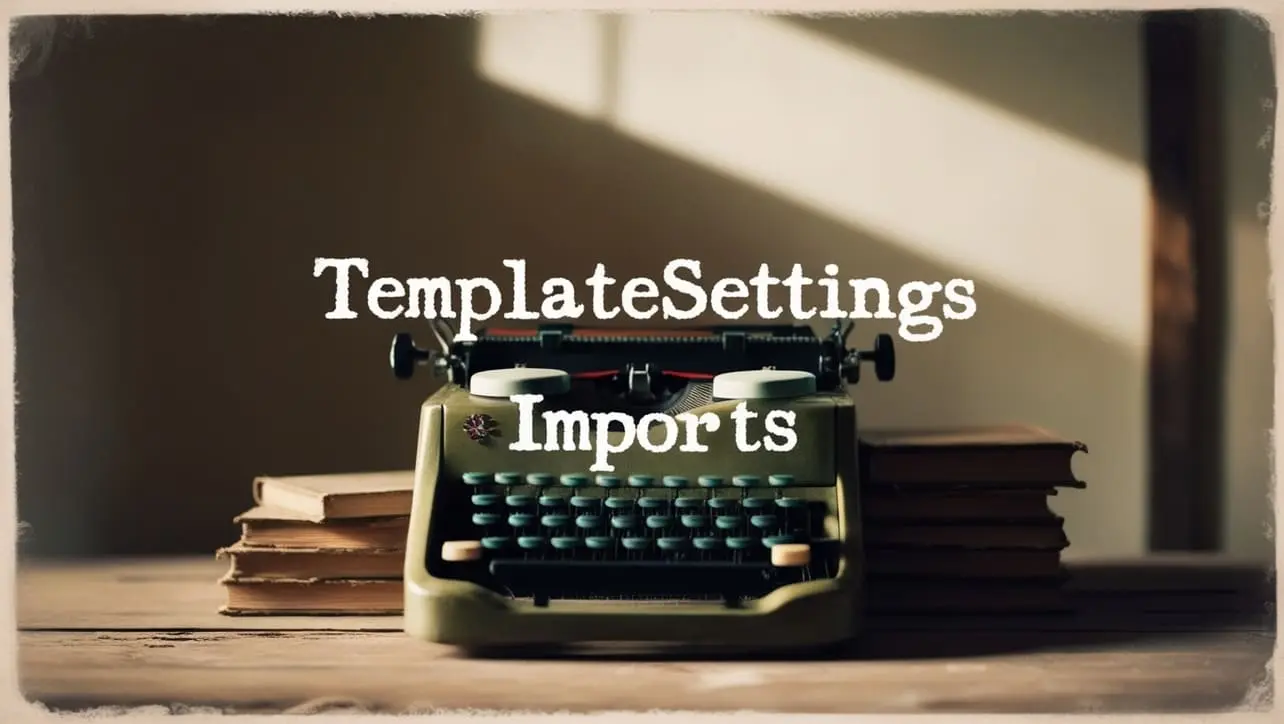
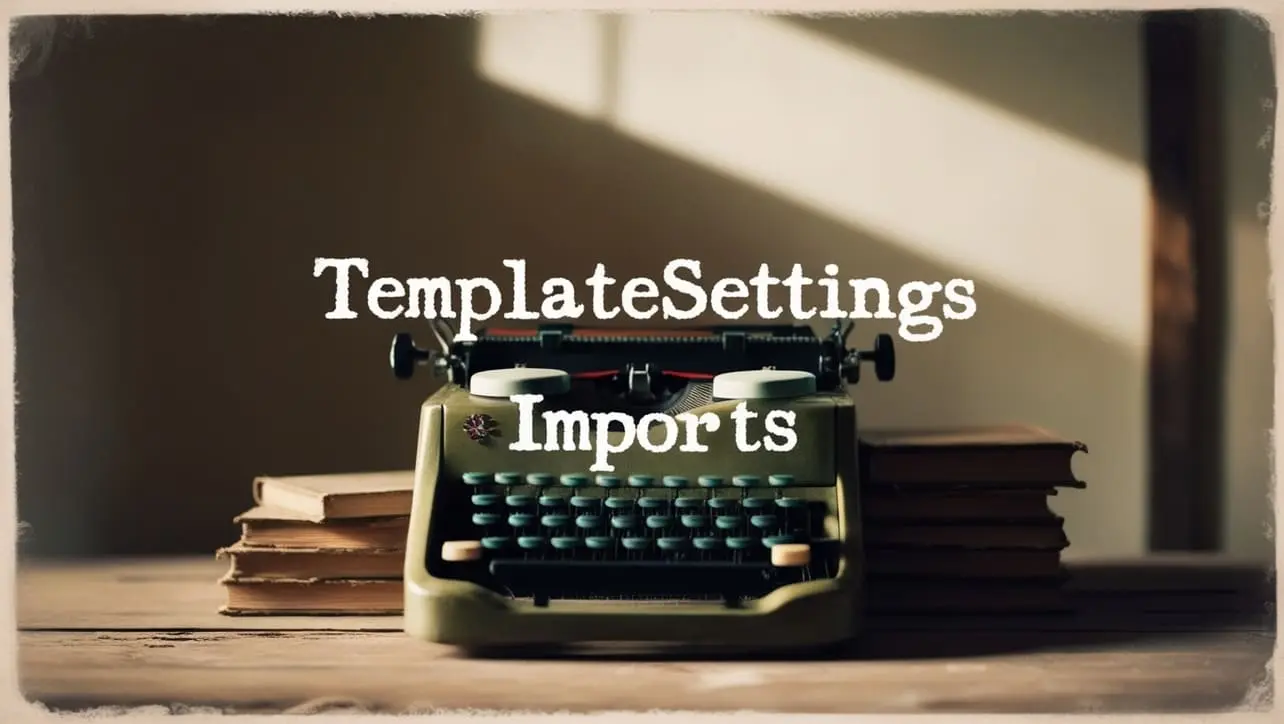
Lodash _.templateSettings.imports Property
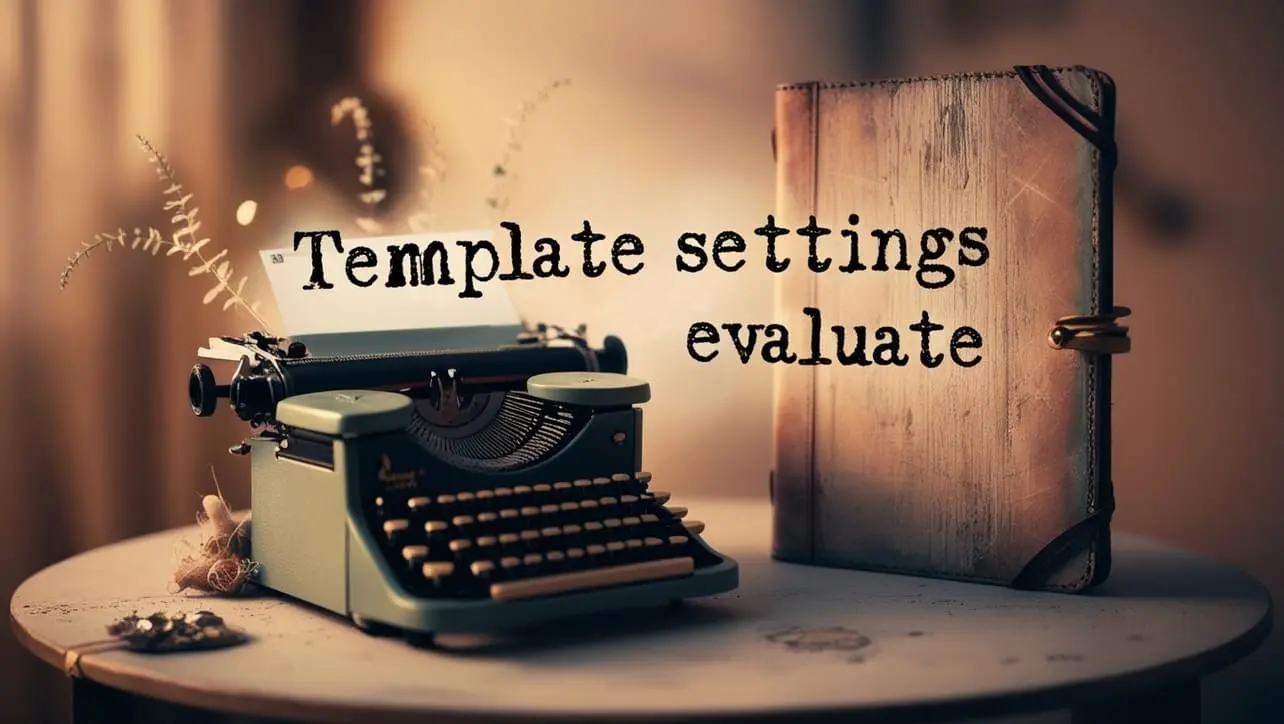
Lodash _.templateSettings.evaluate Property
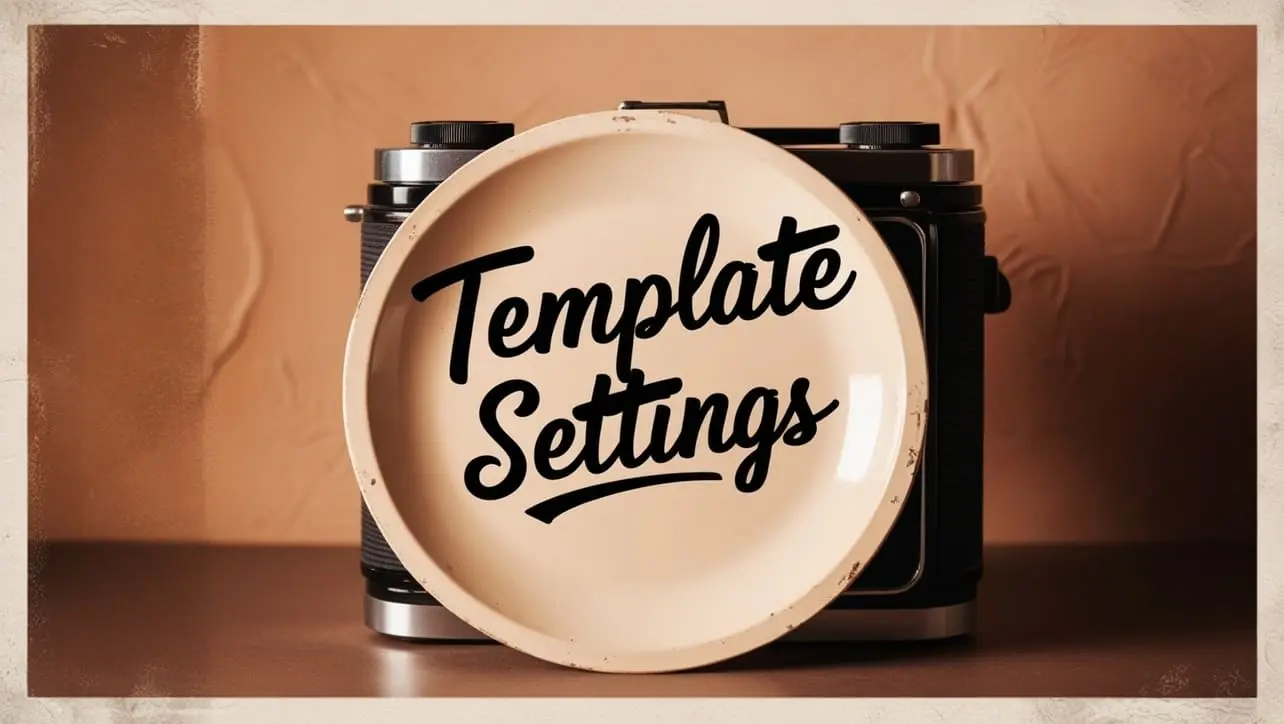
Lodash _.templateSettings Property
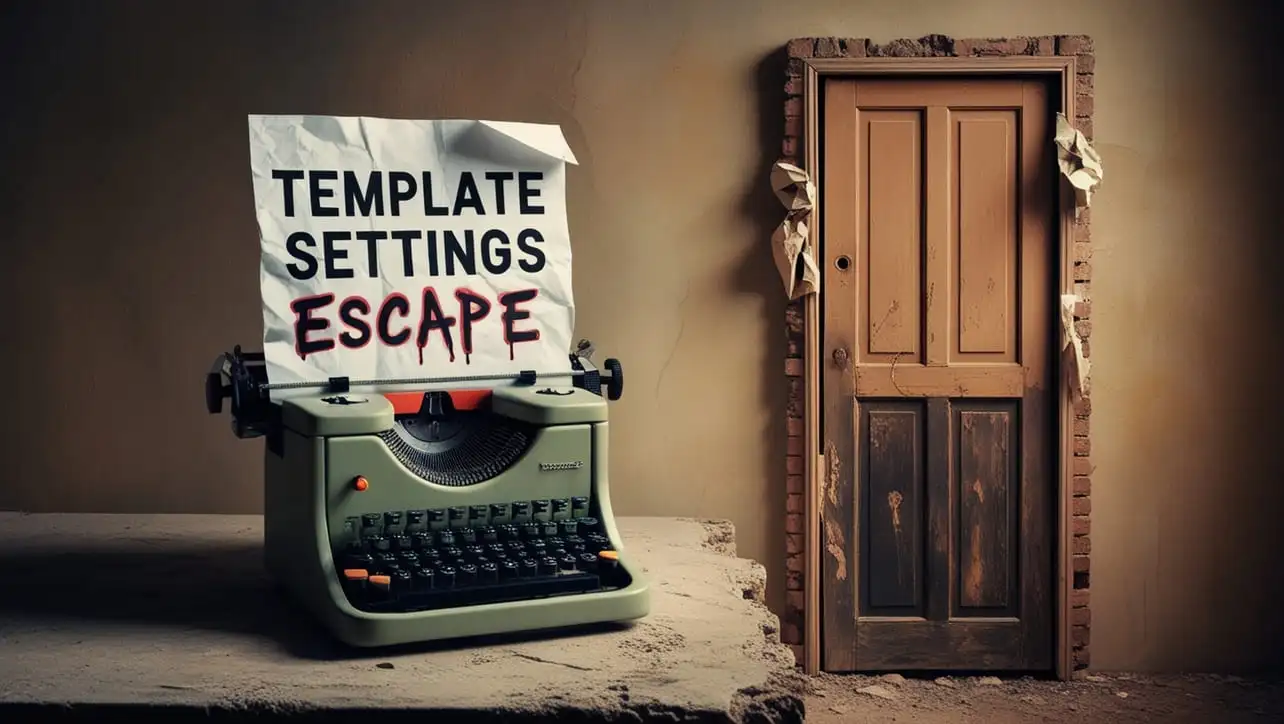
Lodash _.templateSettings.escape Property
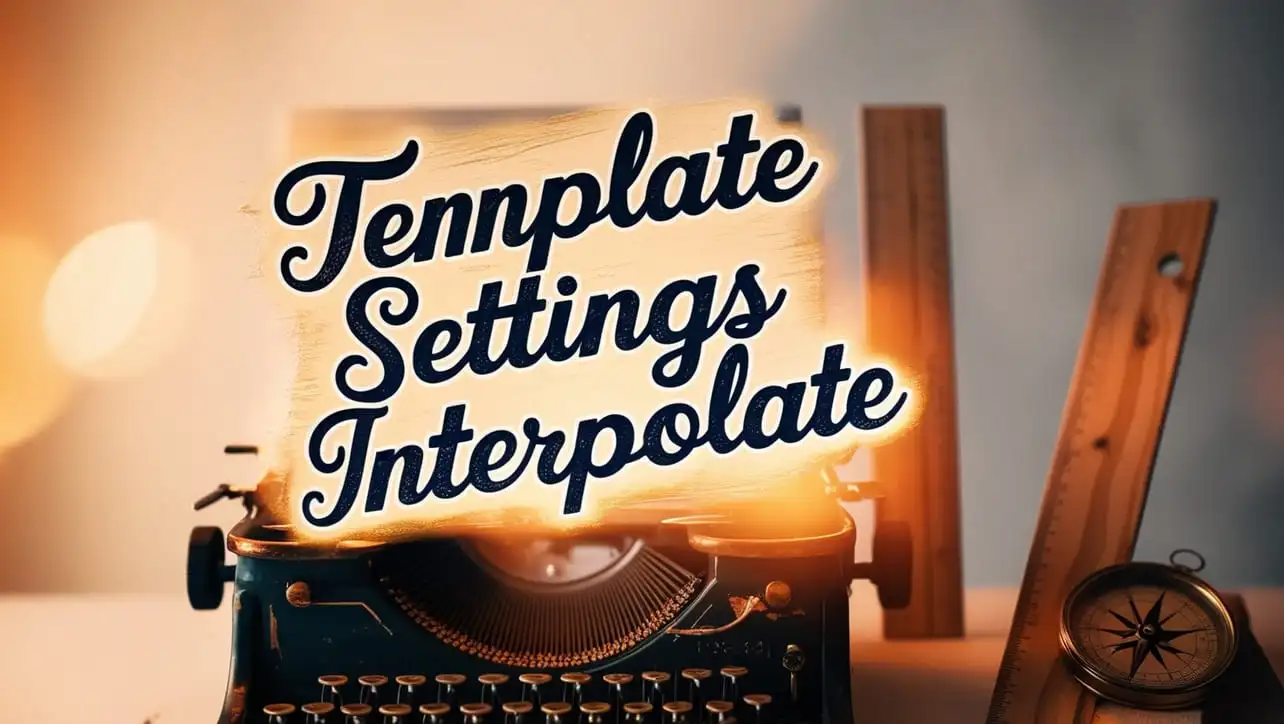
Lodash _.templateSettings.interpolate Property
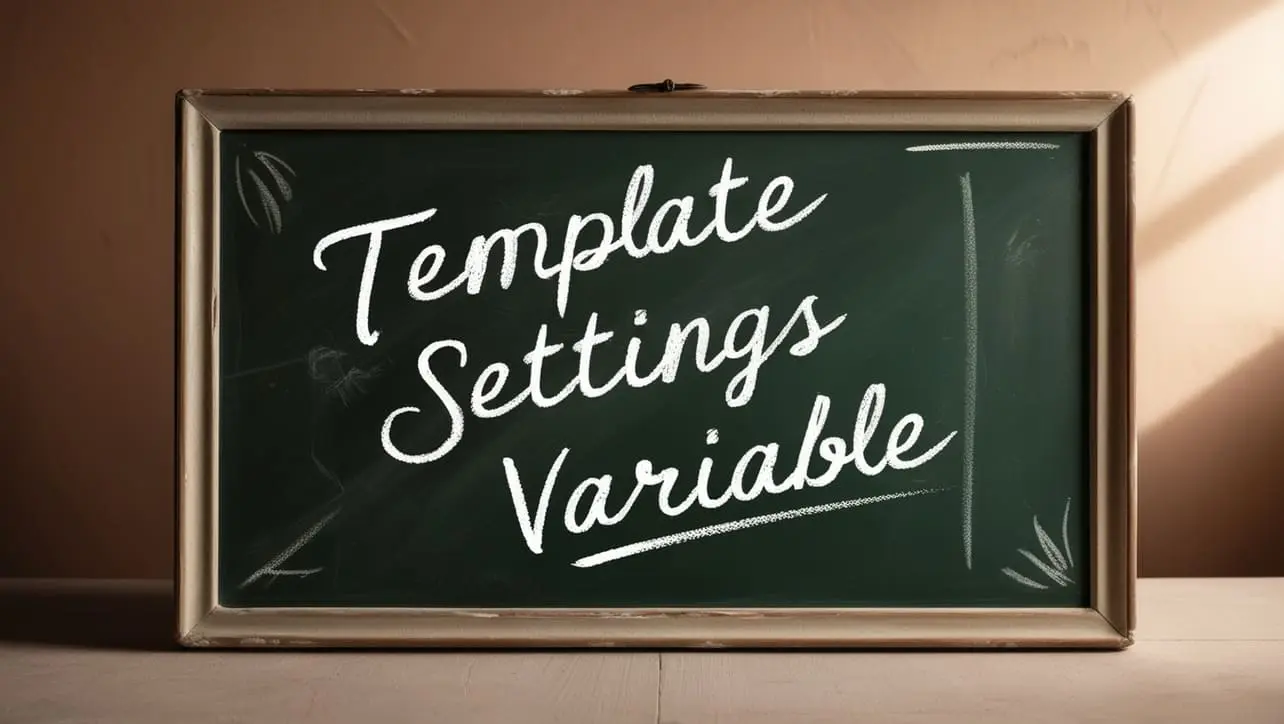
If you have any doubts regarding this article (Lodash _.bindAll() Util Method), please comment here. I will help you immediately.