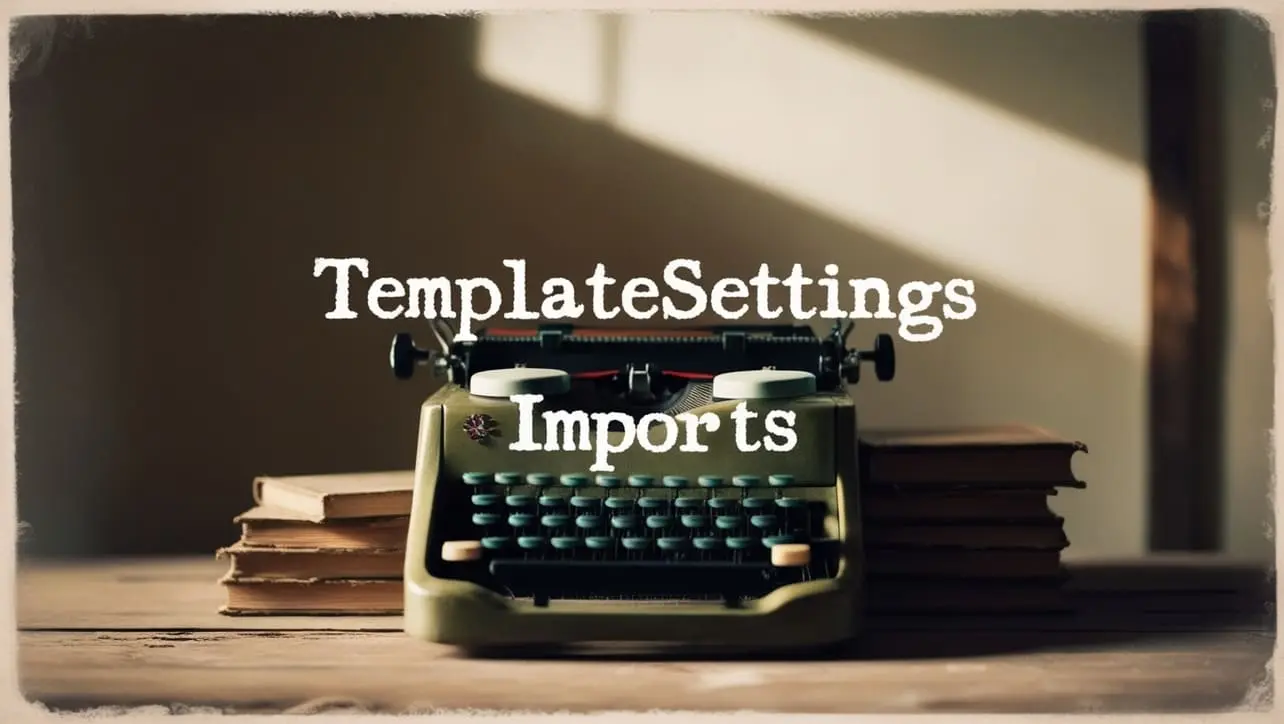
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.uniqueId() Util Method
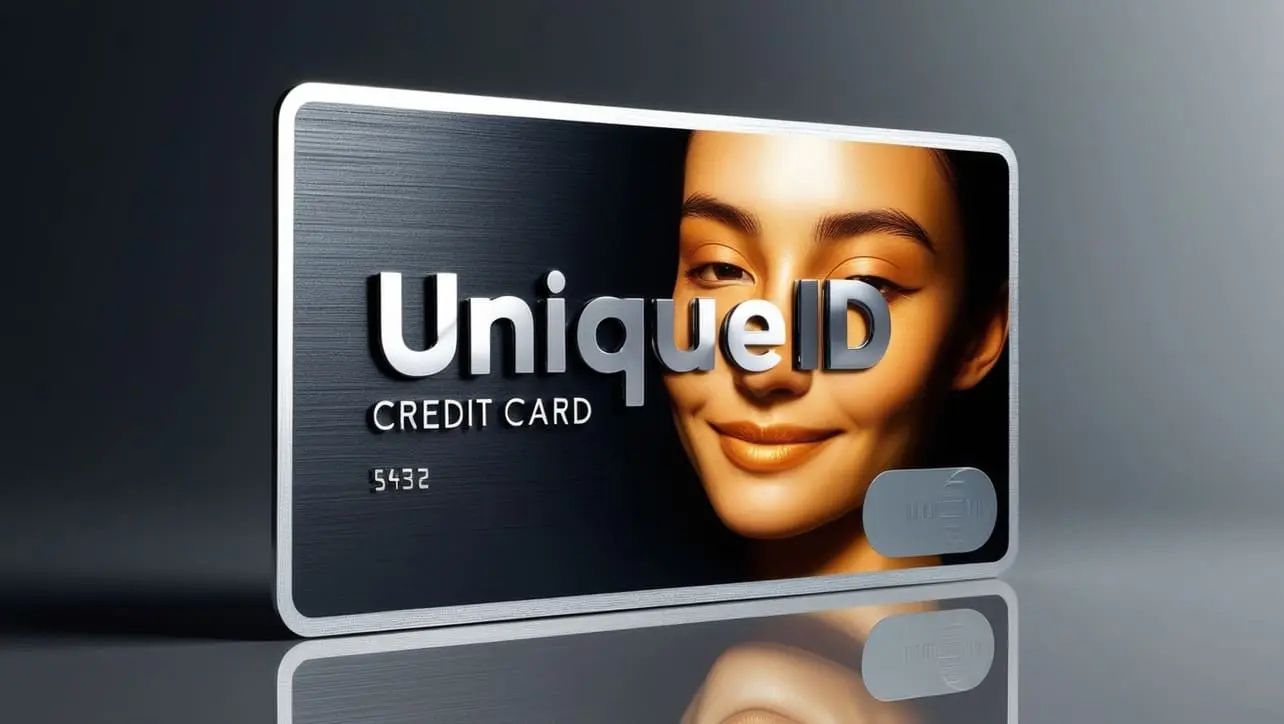
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, generating unique identifiers is a common requirement for various tasks, such as creating DOM elements dynamically, managing state, or ensuring data integrity. Lodash, a popular utility library, offers a convenient solution to this challenge with the _.uniqueId()
method.
This method generates unique IDs based on a specified prefix, making it a valuable tool for developers seeking to streamline their code and ensure uniqueness across their applications.
🧠 Understanding _.uniqueId() Method
The _.uniqueId()
method in Lodash generates a unique identifier, which is composed of a specified prefix followed by a unique numeric value. This enables developers to create consistent and distinguishable identifiers while minimizing the risk of collisions.
💡 Syntax
The syntax for the _.uniqueId()
method is straightforward:
_.uniqueId([prefix])
- prefix (Optional): The prefix to prepend to the unique identifier.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.uniqueId()
method:
const _ = require('lodash');
const uniqueID = _.uniqueId('user_');
console.log(uniqueID);
// Output: 'user_1'
In this example, the uniqueID variable holds the generated unique identifier with the specified prefix 'user_', followed by a unique numeric value.
🏆 Best Practices
When working with the _.uniqueId()
method, consider the following best practices:
Define Descriptive Prefixes:
When using
_.uniqueId()
, choose descriptive prefixes that reflect the purpose or context of the generated identifiers. This promotes code readability and clarity.example.jsCopiedconst elementID = _.uniqueId('element_'); const transactionID = _.uniqueId('transaction_'); console.log(elementID, transactionID);
Handle Concurrent Execution:
In scenarios involving concurrent execution, ensure thread safety by handling the generation of unique identifiers appropriately. Avoid race conditions and guarantee uniqueness across multiple instances or processes.
example.jsCopied// Simulate concurrent execution for(let i = 0; i < 5; i++) { setTimeout(() => { const uniqueID = _.uniqueId('task_'); console.log(uniqueID); }, Math.random() * 1000); }
Consider Performance:
Although
_.uniqueId()
provides a convenient way to generate unique identifiers, consider performance implications when used in performance-critical code paths or with large datasets. Evaluate alternatives or optimizations if necessary.example.jsCopiedconst start = Date.now(); for(let i = 0; i < 1000000; i++) { const uniqueID = _.uniqueId('item_'); } const end = Date.now(); console.log(`Time taken: ${end - start} ms`);
📚 Use Cases
DOM Element Creation:
_.uniqueId()
can be utilized when dynamically creating DOM elements to ensure each element has a unique identifier, facilitating manipulation and event handling.example.jsCopiedconst newDiv = document.createElement('div'); newDiv.id = _.uniqueId('div_'); document.body.appendChild(newDiv);
React Component Keys:
When rendering lists of components in React,
_.uniqueId()
can be employed to generate unique keys for each component, aiding in efficient rendering and reconciliation.example.jsCopiedconst items = ['apple', 'banana', 'cherry']; const list = items.map(item => ( <li key={_.uniqueId('item_')}>{item}</li> ));
Database Operations:
In database operations,
_.uniqueId()
can assist in generating unique identifiers for records or transactions, ensuring data integrity and avoiding conflicts.example.jsCopiedconst newRecord = { id: _.uniqueId('record_'), name: 'New Record', // other properties }; // Save new record to database
🎉 Conclusion
The _.uniqueId()
method in Lodash offers a convenient and reliable solution for generating unique identifiers in JavaScript applications. Whether you're creating DOM elements dynamically, managing state, or interacting with databases, this method provides a versatile tool for ensuring uniqueness and maintaining data integrity across your projects.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.uniqueId()
method in your Lodash projects.
👨💻 Join our Community:
Author
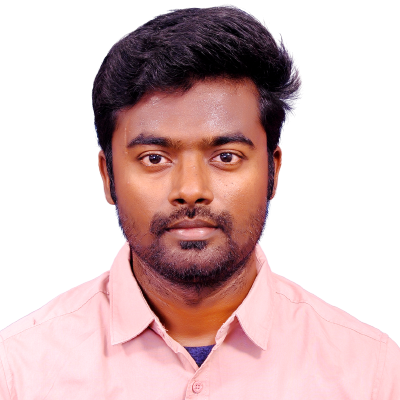
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
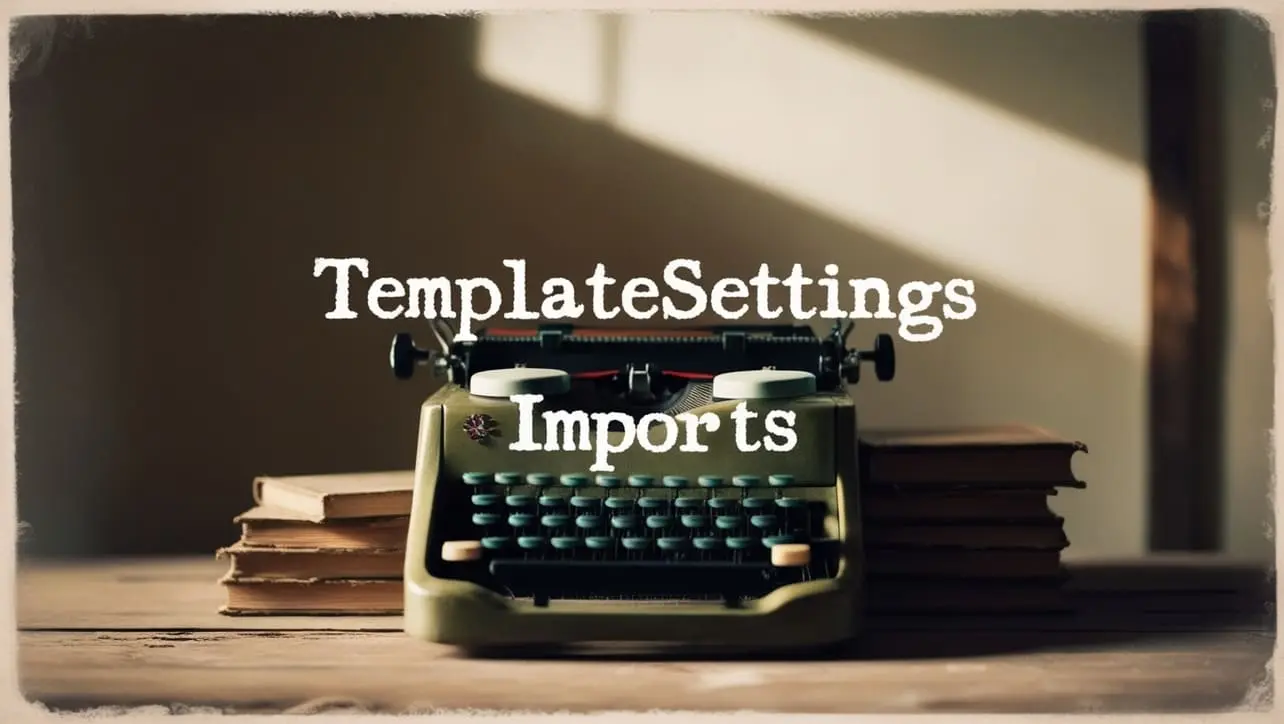
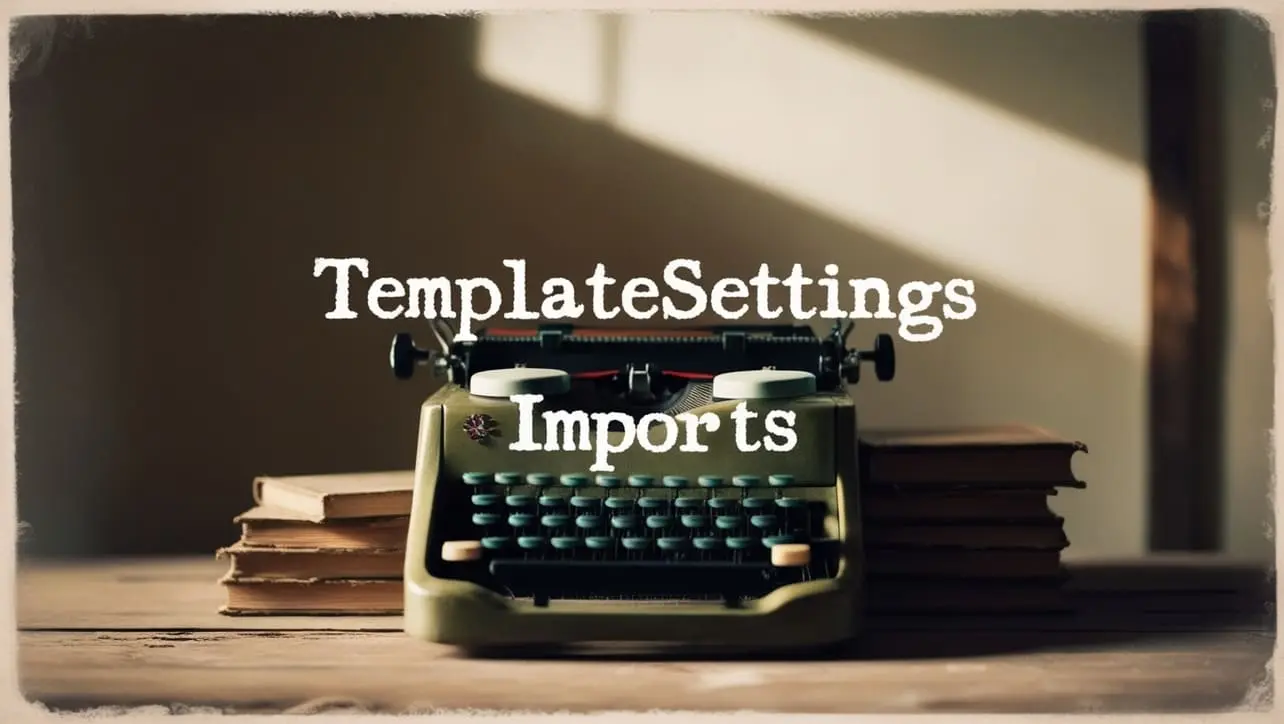
Lodash _.templateSettings.imports Property
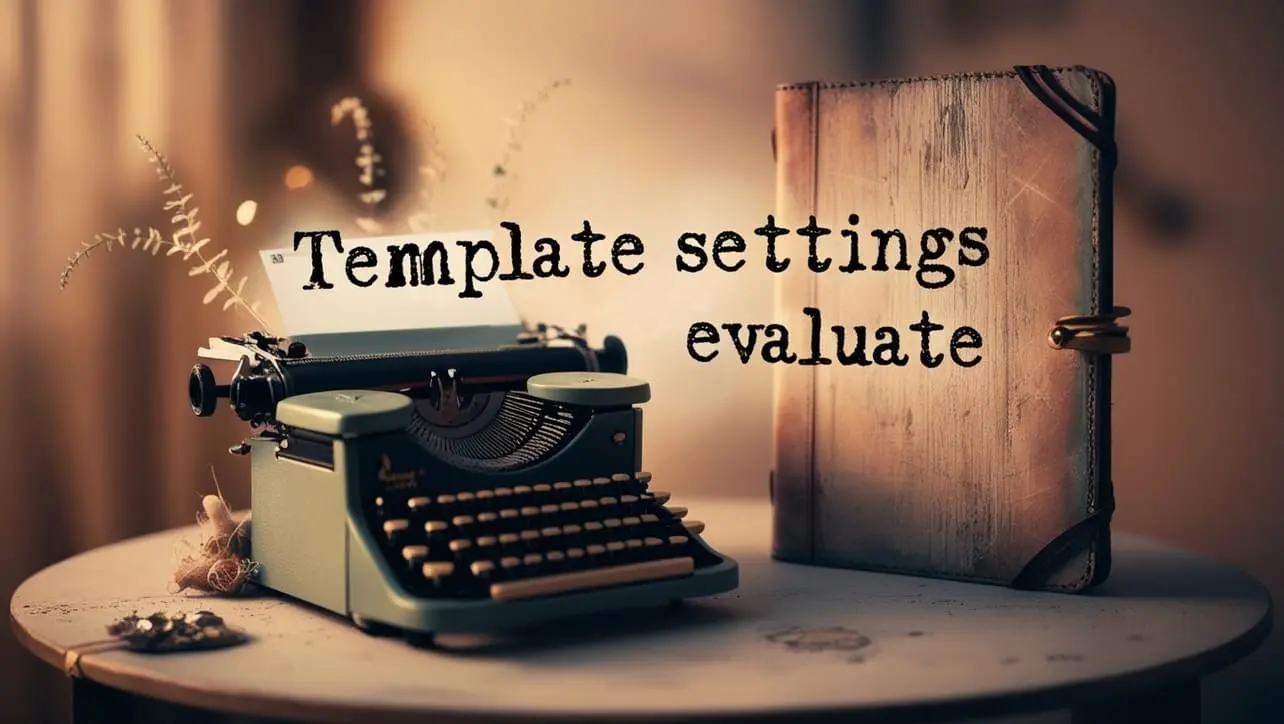
Lodash _.templateSettings.evaluate Property
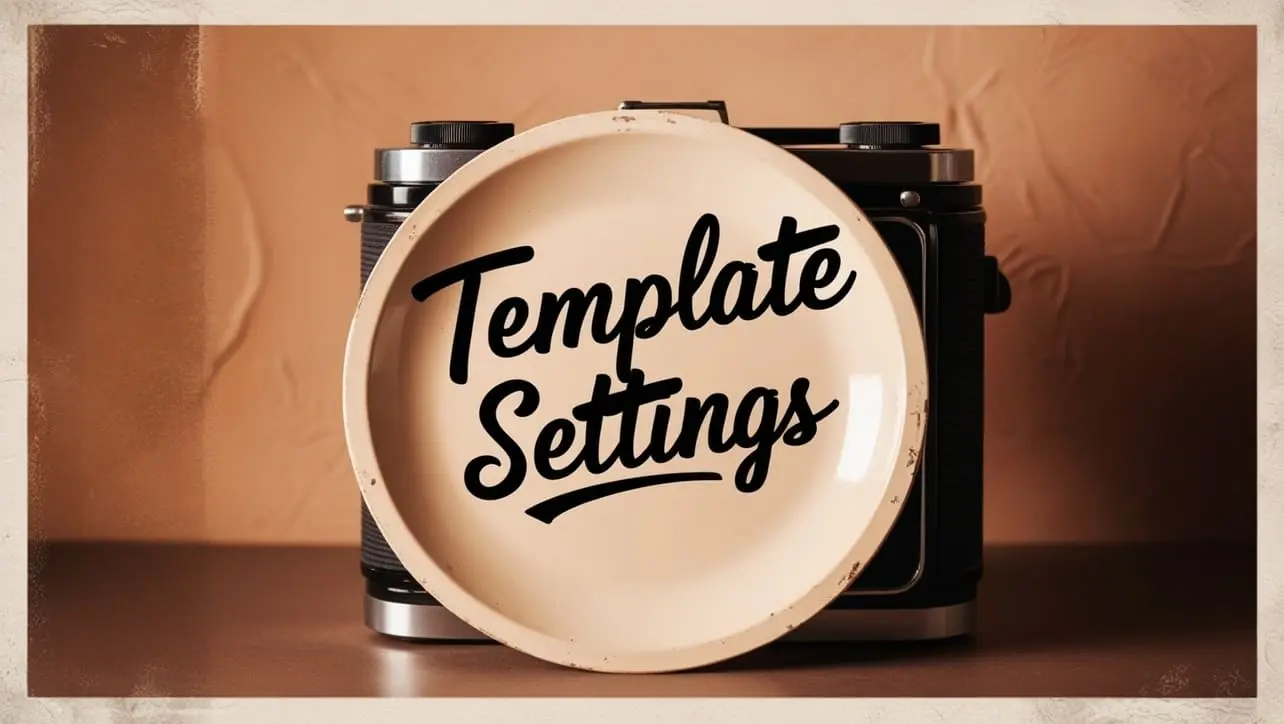
Lodash _.templateSettings Property
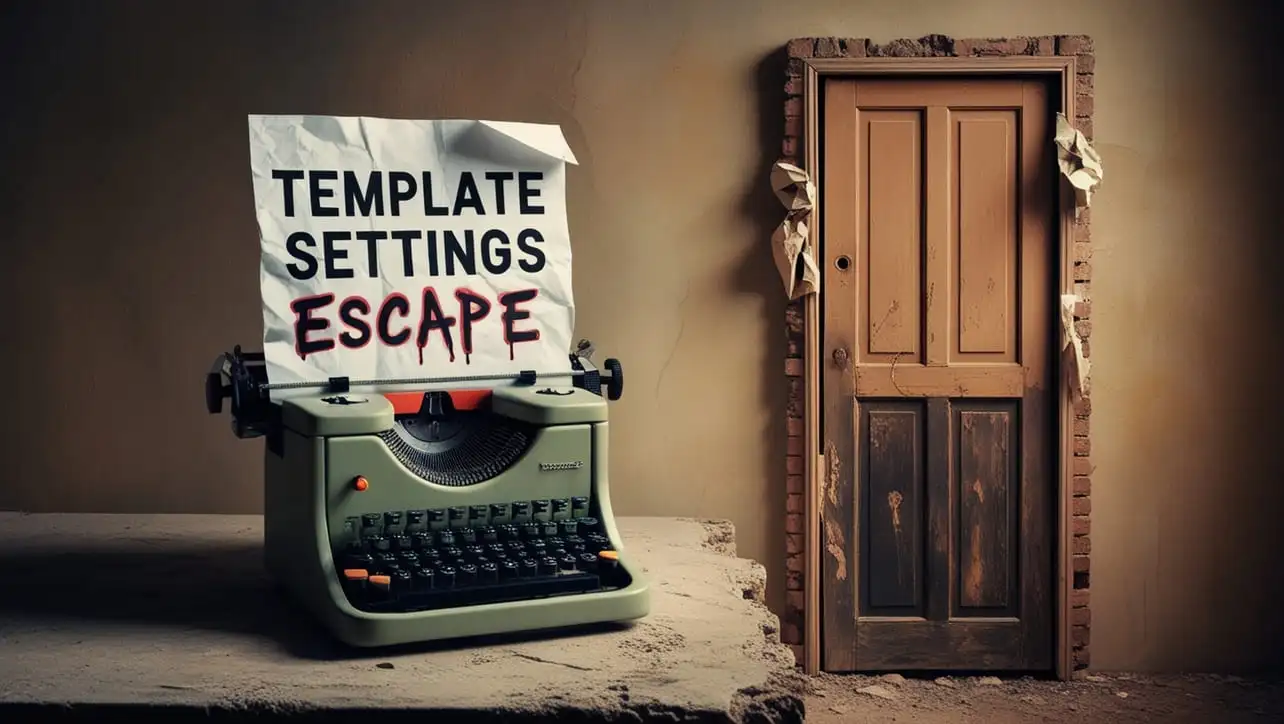
Lodash _.templateSettings.escape Property
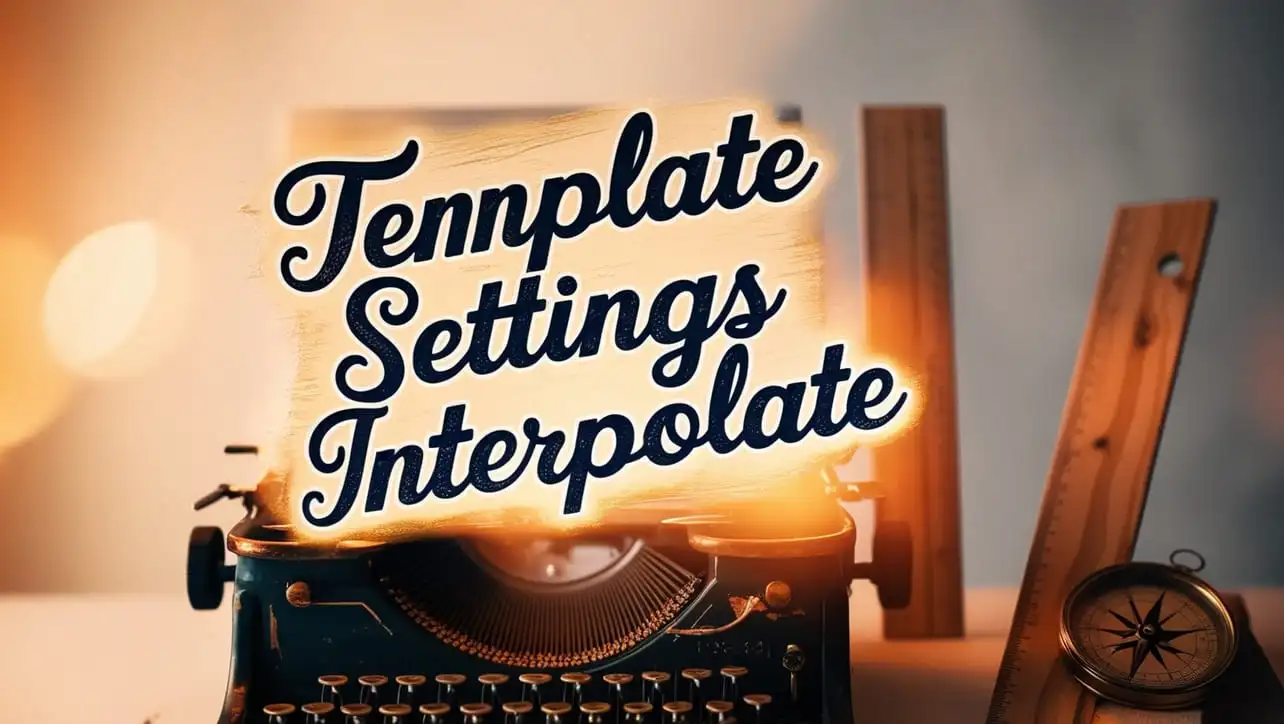
Lodash _.templateSettings.interpolate Property
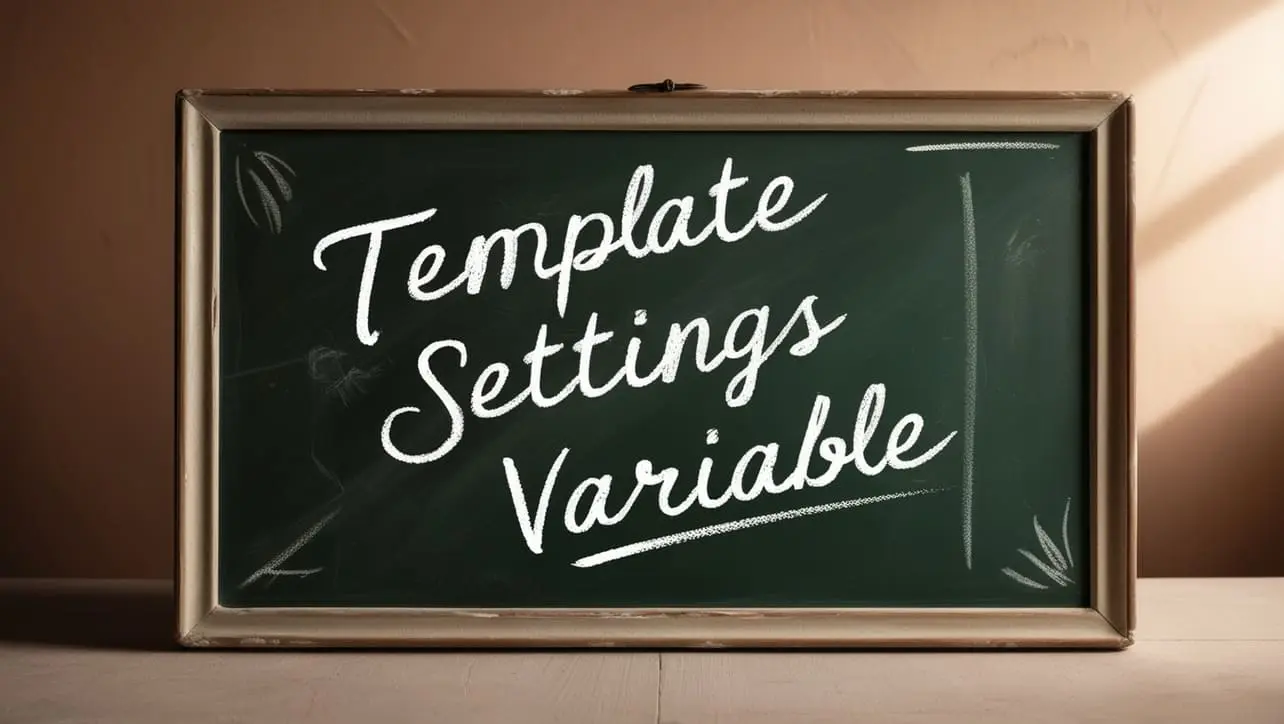
If you have any doubts regarding this article (Lodash _.uniqueId() Util Method), please comment here. I will help you immediately.