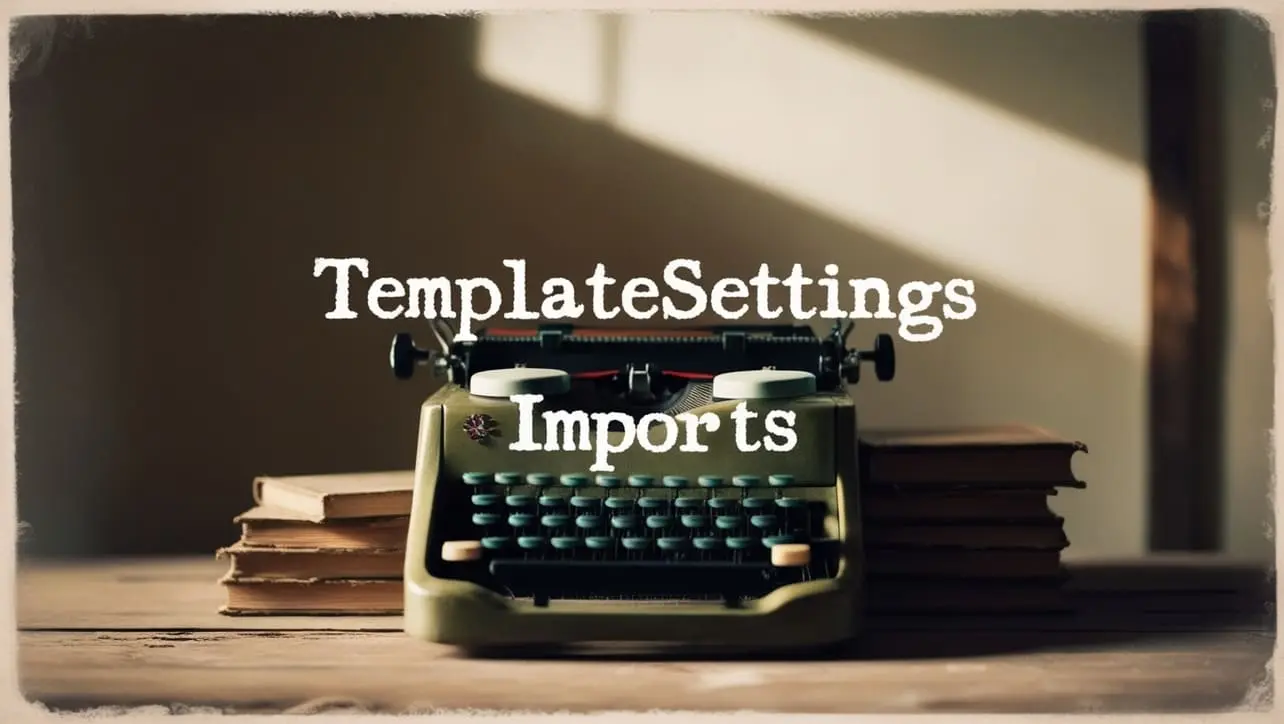
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.times() Util Method
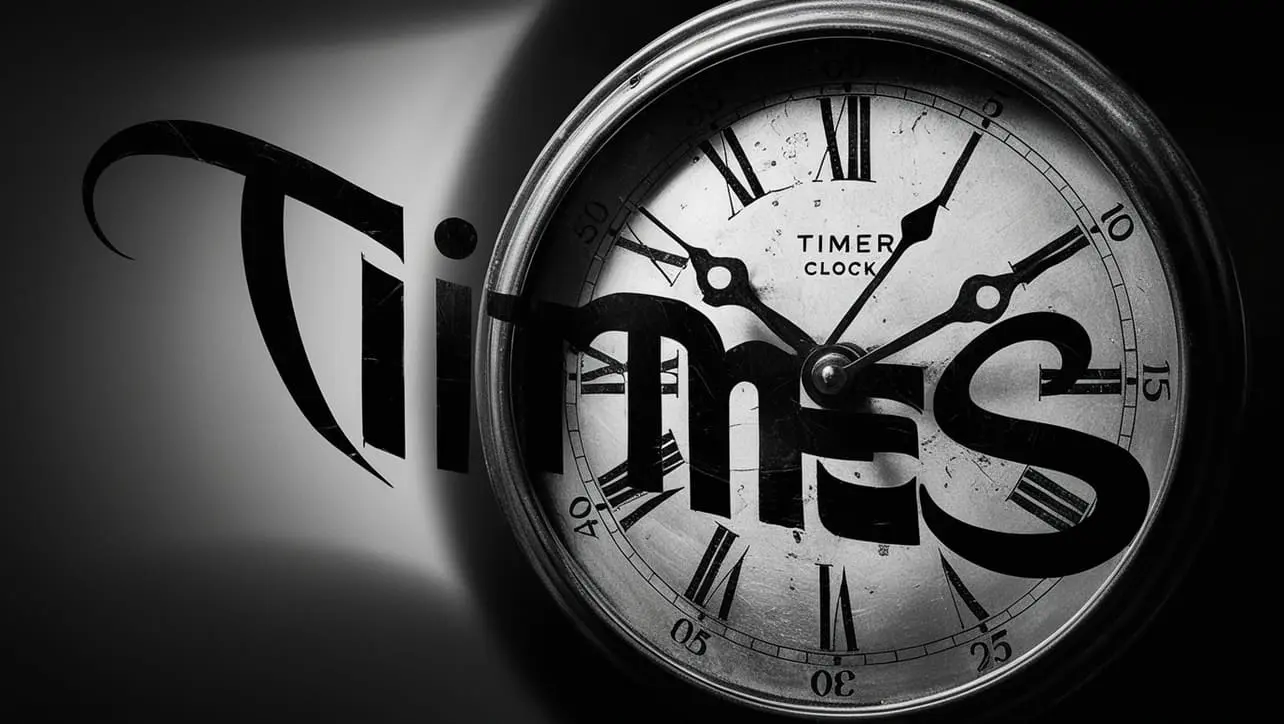
Photo Credit to CodeToFun
🙋 Introduction
In the world of JavaScript development, there are often scenarios where you need to execute a block of code repeatedly for a specified number of times. The Lodash utility library provides a convenient solution for this with the _.times()
method.
This method allows you to iterate a specified number of times and execute a function during each iteration, offering simplicity and flexibility in handling repetitive tasks.
🧠 Understanding _.times() Method
The _.times()
method in Lodash generates an array of values from 0 to n - 1, where n is the specified number of times to iterate. It then invokes the provided iteratee function for each value in the array, passing the current index as an argument.
💡 Syntax
The syntax for the _.times()
method is straightforward:
_.times(n, iteratee)
- n: The number of times to invoke the iteratee function.
- iteratee: The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.times()
method:
const _ = require('lodash');
_.times(3, index => {
console.log(`Iteration ${index + 1}`);
});
In this example, the _.times()
method is used to iterate 3 times, with the iteratee function logging each iteration to the console.
🏆 Best Practices
When working with the _.times()
method, consider the following best practices:
Handle Edge Cases:
Consider edge cases, such as when the number of times to iterate is 0 or negative. Implement appropriate error handling or default behaviors to address these scenarios.
example.jsCopiedconst numTimes = -1; if(numTimes <= 0) { console.error('Invalid number of times to iterate'); } else { _.times(numTimes, () => { // Execute code for each iteration }); }
Utilize Index Parameter:
Leverage the index parameter provided to the iteratee function to customize behavior based on the current iteration. This allows for dynamic processing and manipulation during each iteration.
example.jsCopied_.times(5, index => { console.log(`Processing item ${index}`); // Execute code based on the current index });
Encapsulate Complex Logic:
When dealing with complex logic within the iteratee function, consider encapsulating it into separate functions for improved readability and maintainability.
example.jsCopiedconst processItem = index => { // Complex processing logic console.log(`Processing item ${index}`); }; _.times(5, processItem);
📚 Use Cases
Generating Sequential IDs:
_.times()
can be used to generate sequential IDs for a specified number of items, facilitating data management and identification.example.jsCopiedconst items = []; _.times(10, index => { items.push({ id: index + 1, name: `Item ${index + 1}` }); }); console.log(items);
Batch Processing:
For tasks involving batch processing, such as making API requests in batches,
_.times()
can streamline the execution of repetitive tasks.example.jsCopiedconst batchSize = 10; _.times(3, batchIndex => { const startIndex = batchIndex * batchSize; const batchItems = /* ...fetch batch data based on startIndex and batchSize... */ ; // Process batch items console.log(`Processing batch ${batchIndex + 1}:`, batchItems); });
Dynamic Content Rendering:
In web development,
_.times()
can aid in dynamically rendering content based on a specified number of iterations, such as generating a list of items or rendering components.example.jsCopiedconst numItems = 5; _.times(numItems, index => { const listItem = document.createElement('li'); listItem.textContent = `Item ${index + 1}`; document.getElementById('list-container').appendChild(listItem); });
🎉 Conclusion
The _.times()
method in Lodash offers a convenient and efficient solution for executing code repeatedly for a specified number of times. Whether you're generating sequential IDs, processing data in batches, or dynamically rendering content, _.times()
provides a versatile tool for handling repetitive tasks in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.times()
method in your Lodash projects.
👨💻 Join our Community:
Author
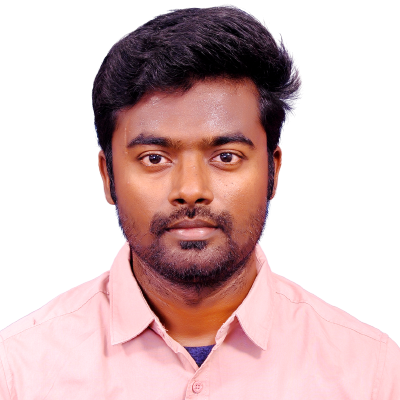
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
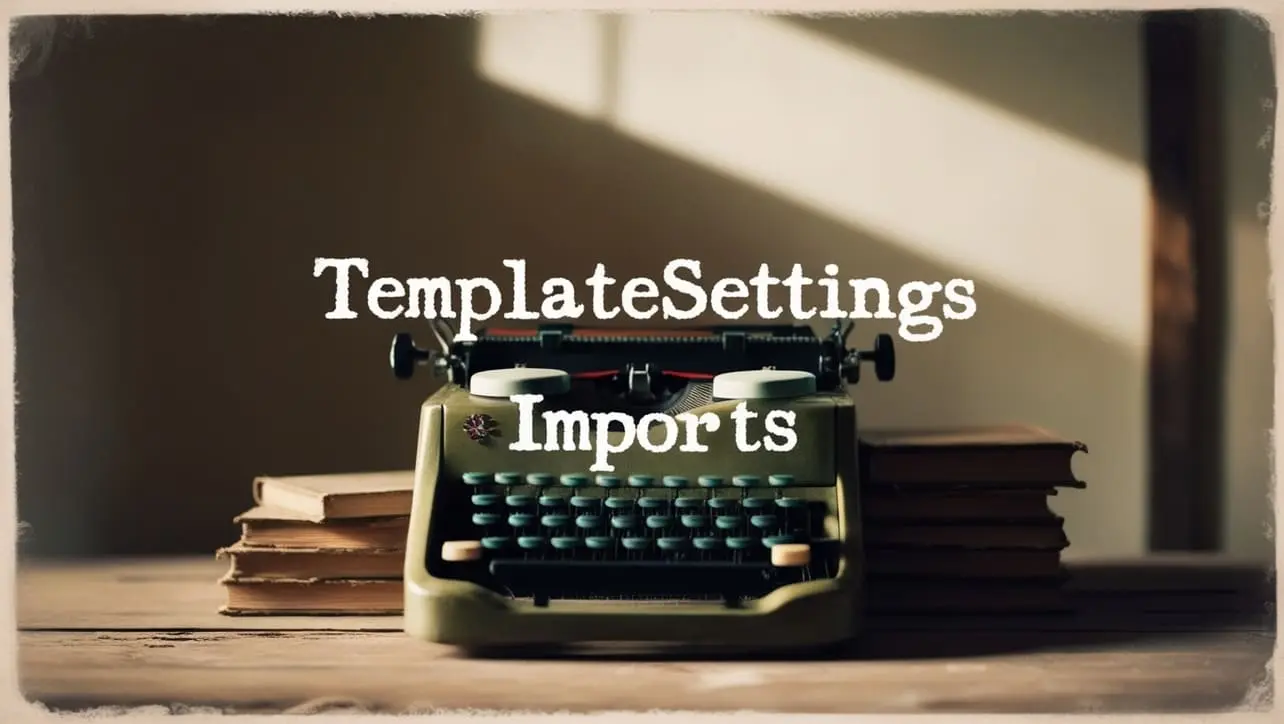
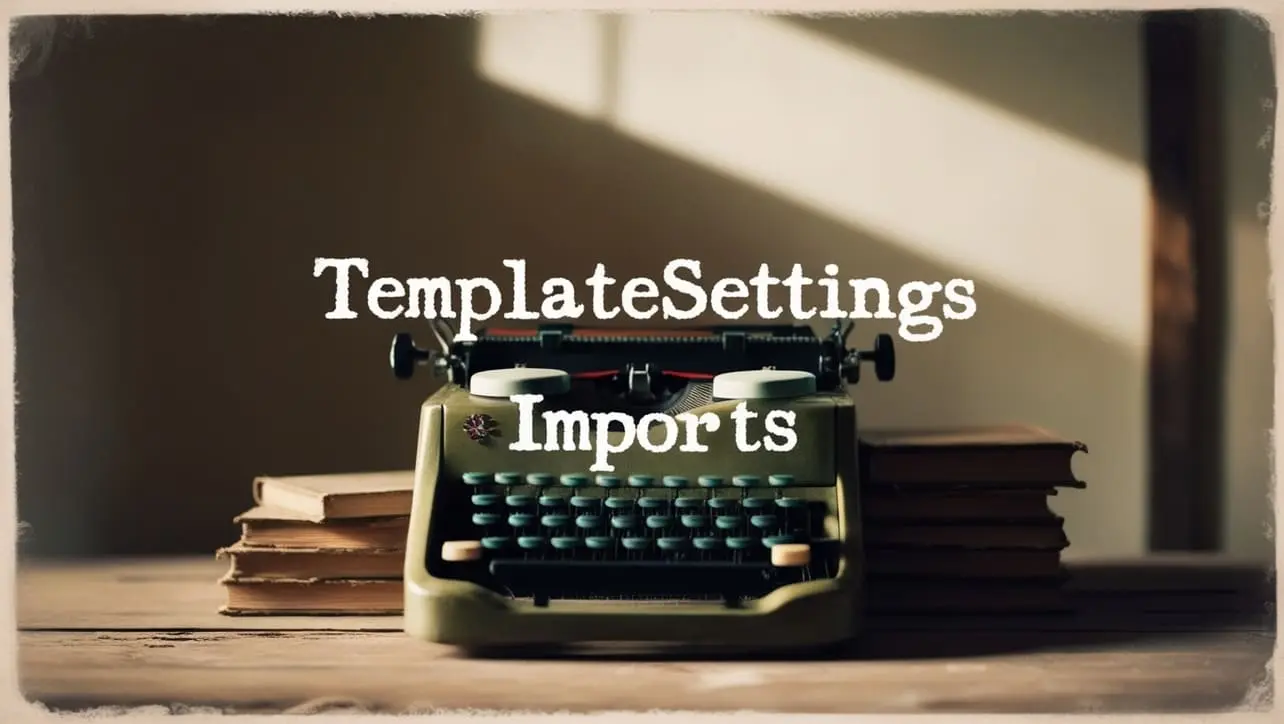
Lodash _.templateSettings.imports Property
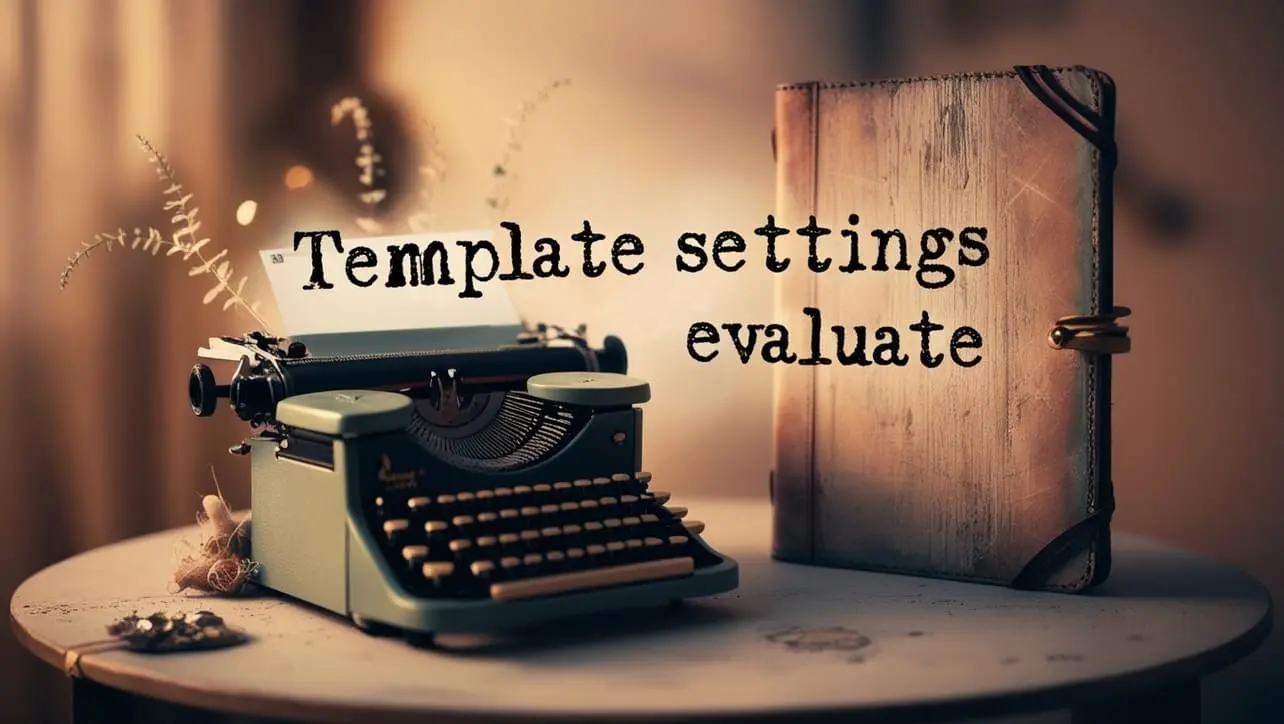
Lodash _.templateSettings.evaluate Property
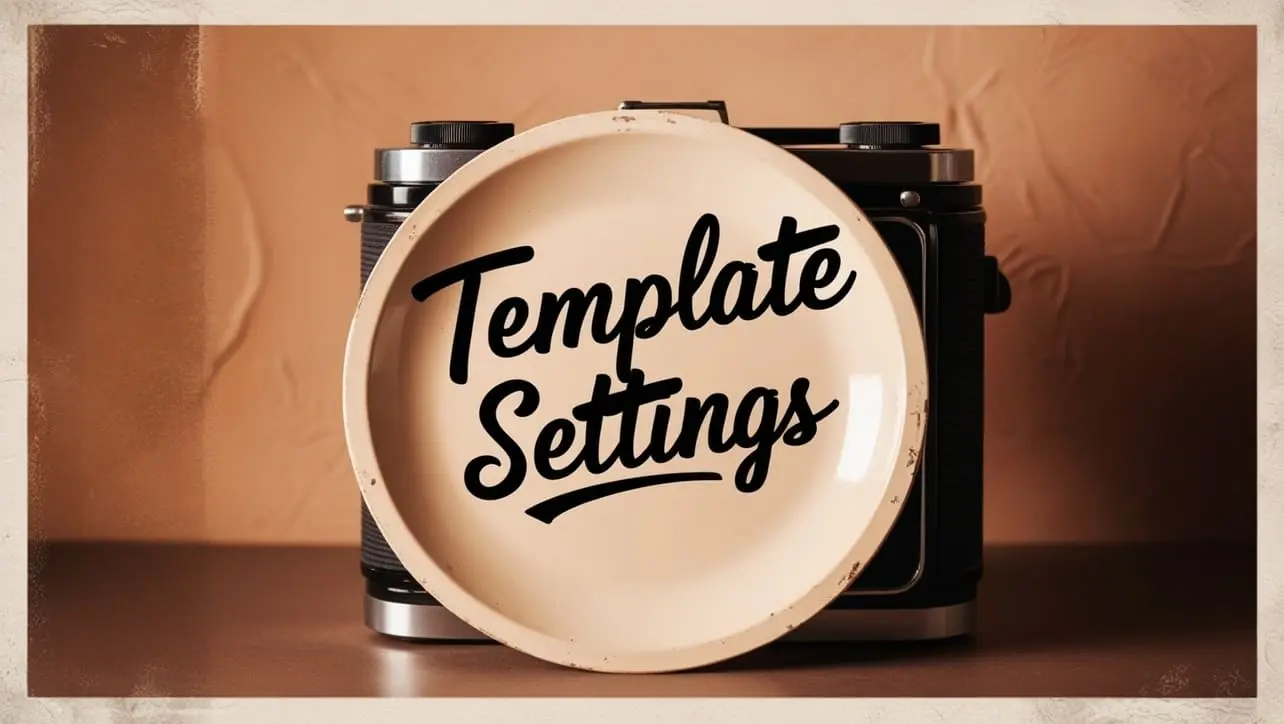
Lodash _.templateSettings Property
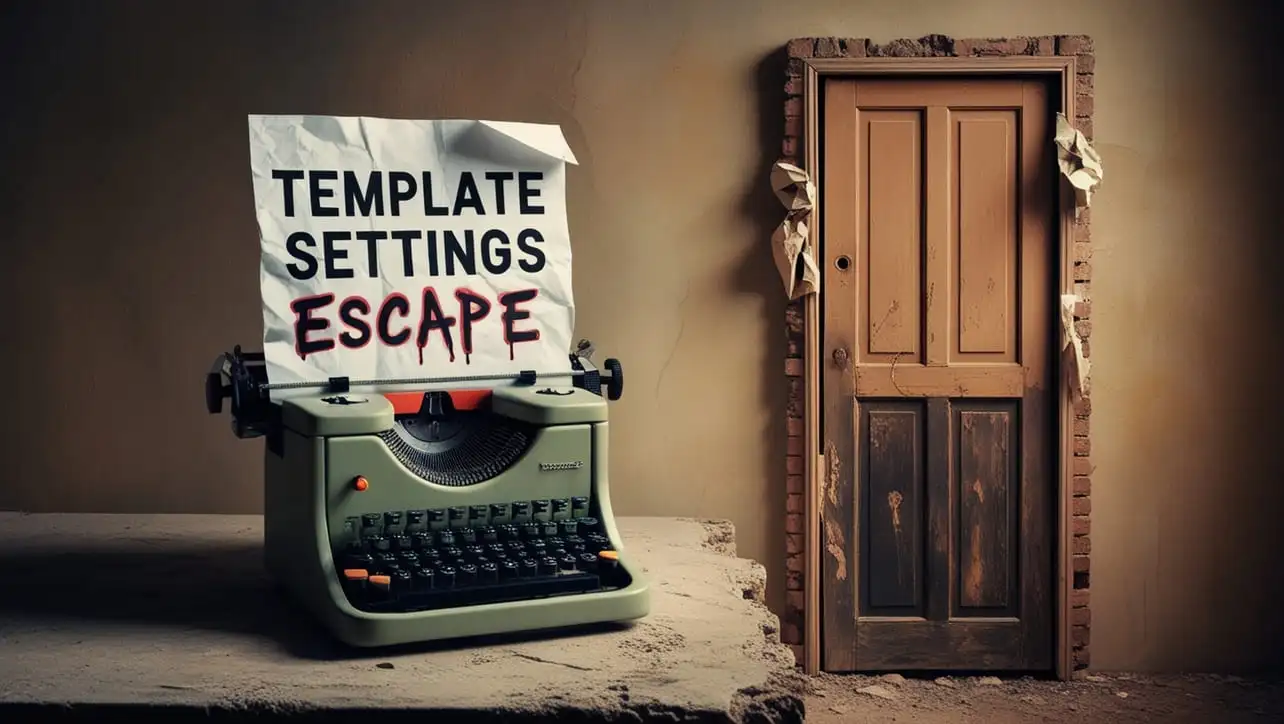
Lodash _.templateSettings.escape Property
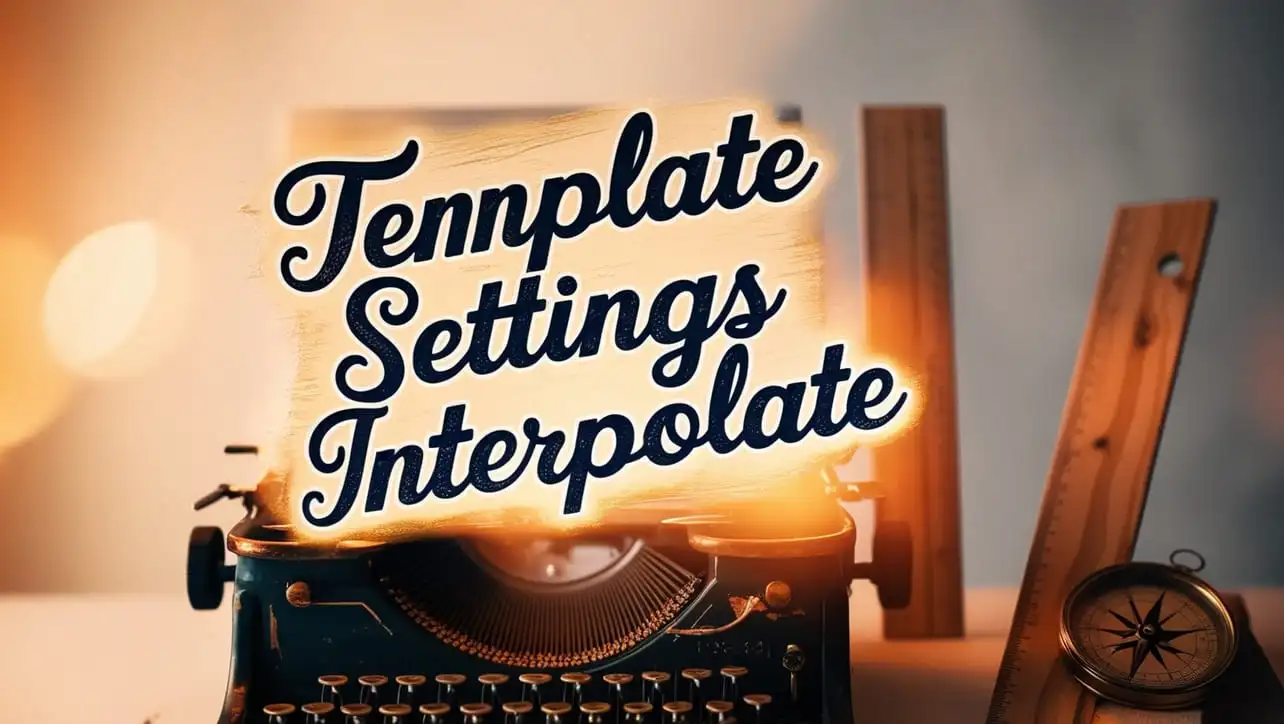
Lodash _.templateSettings.interpolate Property
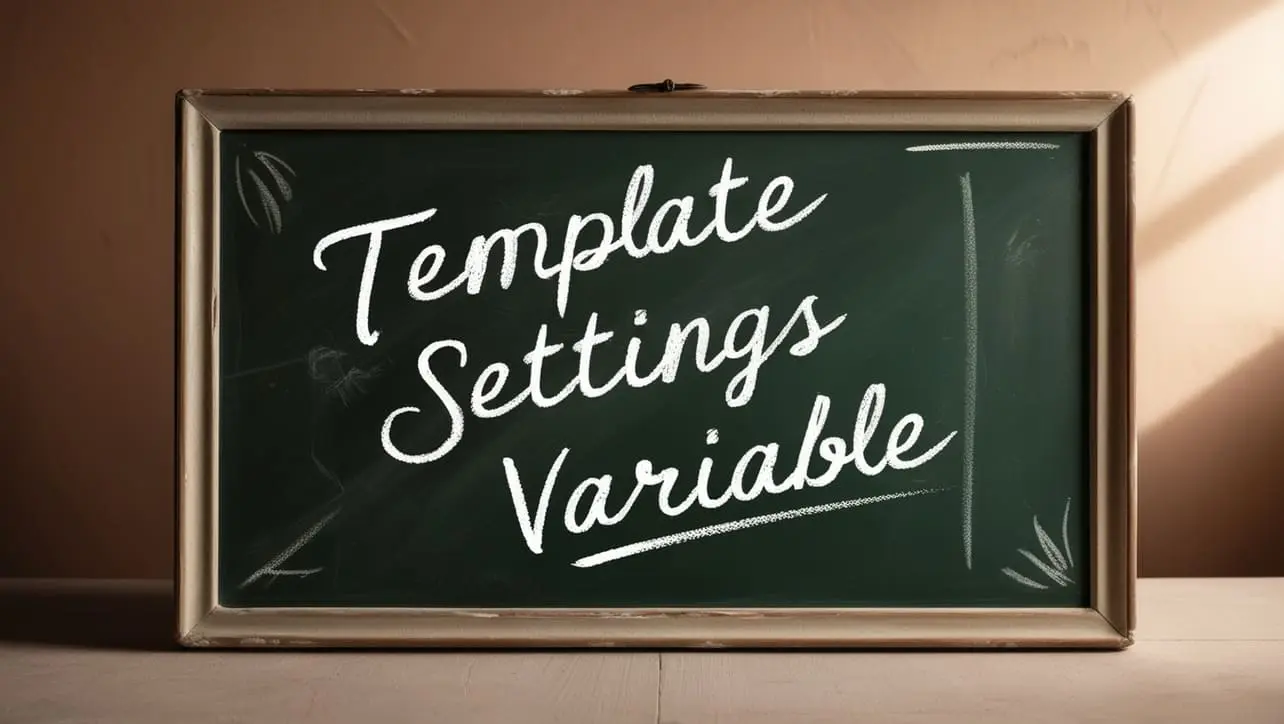
If you have any doubts regarding this article (Lodash _.times() Util Method), please comment here. I will help you immediately.