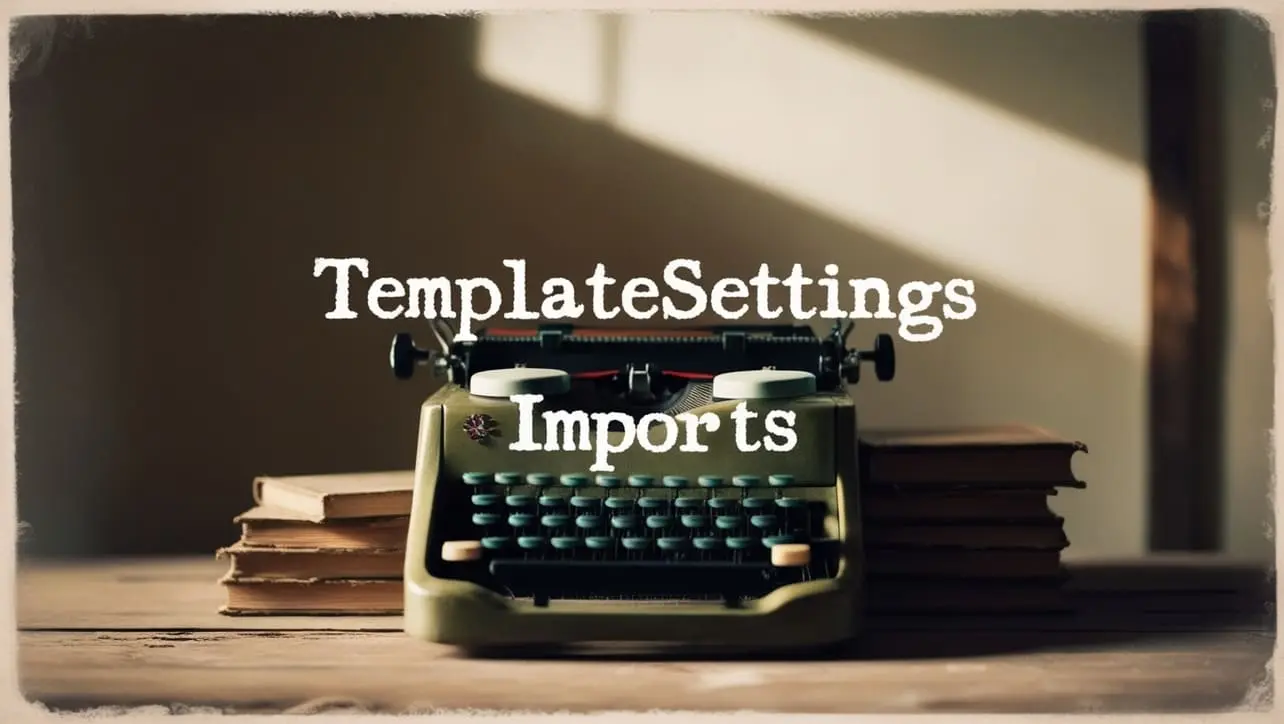
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.stubTrue() Util Method
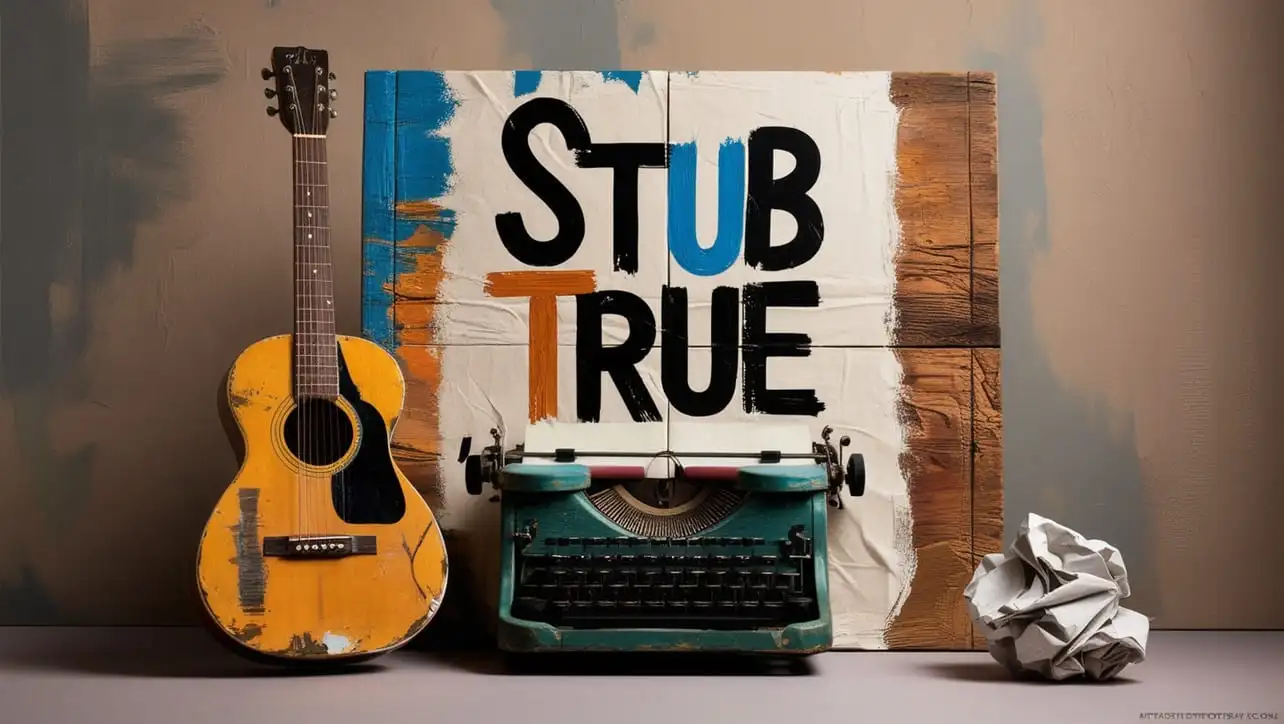
Photo Credit to CodeToFun
🙋 Introduction
In the domain of JavaScript programming, simplifying common tasks often requires clever utility functions. Lodash, a popular JavaScript utility library, provides a plethora of such functions to streamline development. Among these is the _.stubTrue()
method, a simple yet powerful utility that always returns true.
While seemingly basic, this function proves invaluable in scenarios where a true boolean value is required as a placeholder or for logical operations.
🧠 Understanding _.stubTrue() Method
The _.stubTrue()
method in Lodash is a utility function that returns true regardless of the arguments passed to it. This straightforward behavior makes it useful for scenarios where a constant true value is needed, such as in callback functions, predicate functions, or as a placeholder value.
💡 Syntax
The syntax for the _.stubTrue()
method is straightforward:
_.stubTrue()
📝 Example
Let's dive into a simple example to illustrate the usage of the _.stubTrue()
method:
const _ = require('lodash');
const alwaysTrue = _.stubTrue();
console.log(alwaysTrue); // Output: true
In this example, alwaysTrue is assigned the result of _.stubTrue()
, which is always true.
🏆 Best Practices
When working with the _.stubTrue()
method, consider the following best practices:
Placeholder Values:
Use
_.stubTrue()
as a placeholder value when defining default values or callbacks that should always return true.example.jsCopiedconst callback = _.stubTrue(); // Use as a placeholder callback const result = _.filter(array, callback);
Predicate Functions:
In situations where a predicate function is required but the condition is irrelevant,
_.stubTrue()
can serve as a concise and clear placeholder.example.jsCopiedconst isValid = _.filter(data, _.stubTrue);
Logical Operations:
When composing logical operations and a constant true value is needed,
_.stubTrue()
provides a clean and efficient solution.example.jsCopiedconst alwaysTrue = _.stubTrue(); const result = alwaysTrue && someOtherCondition;
📚 Use Cases
Default Callbacks:
In scenarios where a callback function is required but its logic is inconsequential,
_.stubTrue()
can serve as a default callback to ensure that operations proceed without unnecessary complexity.example.jsCopiedconst data = [/* array of objects */]; const filteredData = _.filter(data, _.stubTrue);
Mock Functions:
During testing or development,
_.stubTrue()
can be used to create placeholder functions that always return true, simplifying the testing process.example.jsCopiedconst mockCallback = _.stubTrue(); const result = someFunctionThatRequiresCallback(mockCallback);
Simplifying Conditional Logic:
When writing conditional logic and a constant true value is needed,
_.stubTrue()
eliminates the need for additional condition checks, resulting in cleaner and more concise code.example.jsCopiedif (_.stubTrue()) { // Code execution always enters this block }
🎉 Conclusion
The _.stubTrue()
method in Lodash may seem simple at first glance, but its utility shines in scenarios requiring a constant true value. Whether as a placeholder, default callback, or simplification of conditional logic, _.stubTrue()
offers a convenient solution to common programming challenges.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.stubTrue()
method in your Lodash projects.
👨💻 Join our Community:
Author
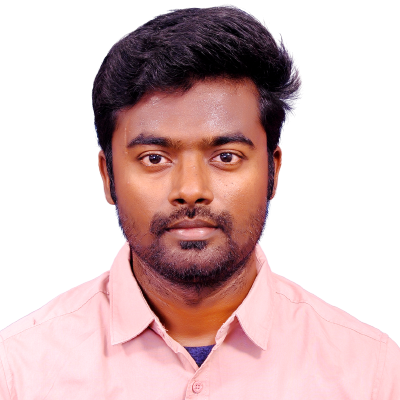
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
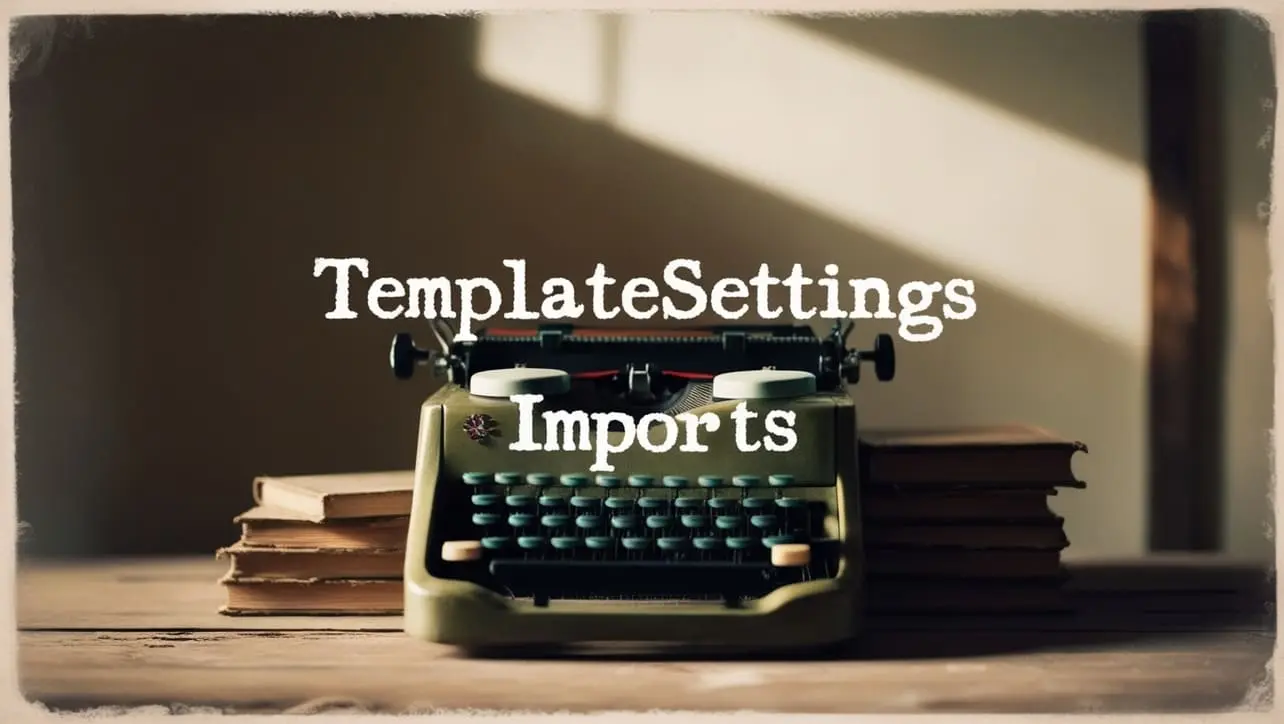
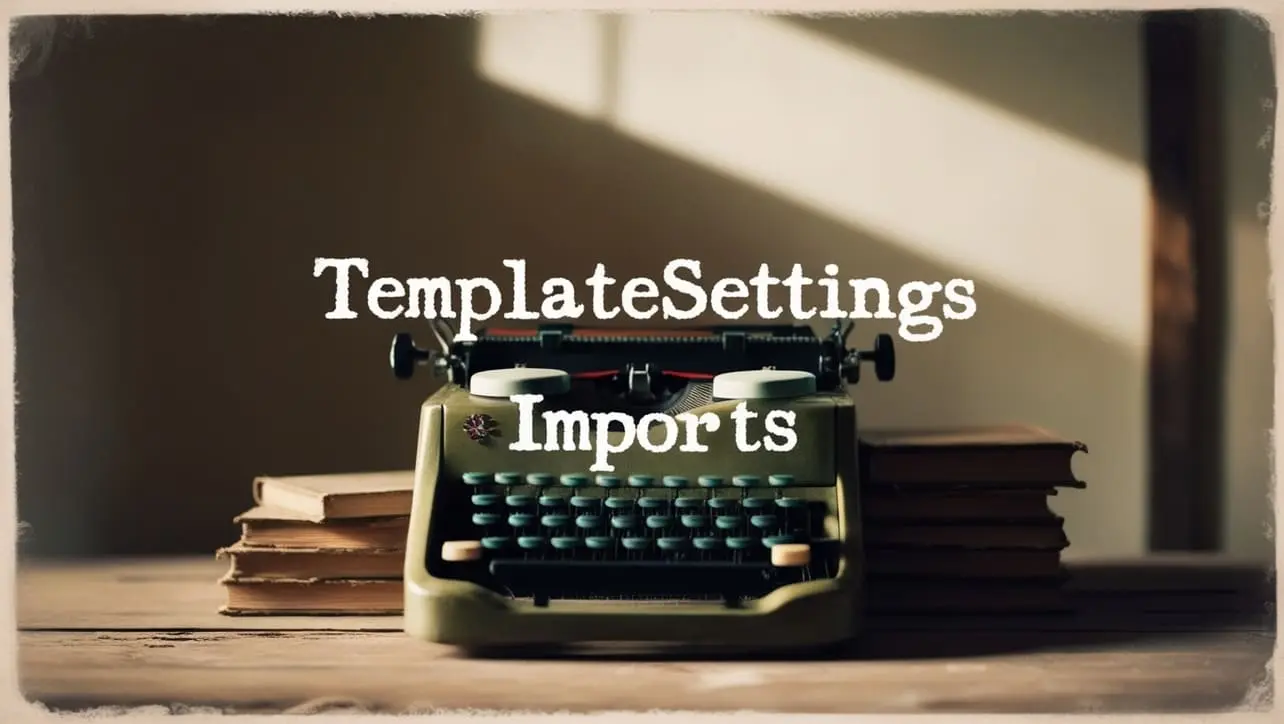
Lodash _.templateSettings.imports Property
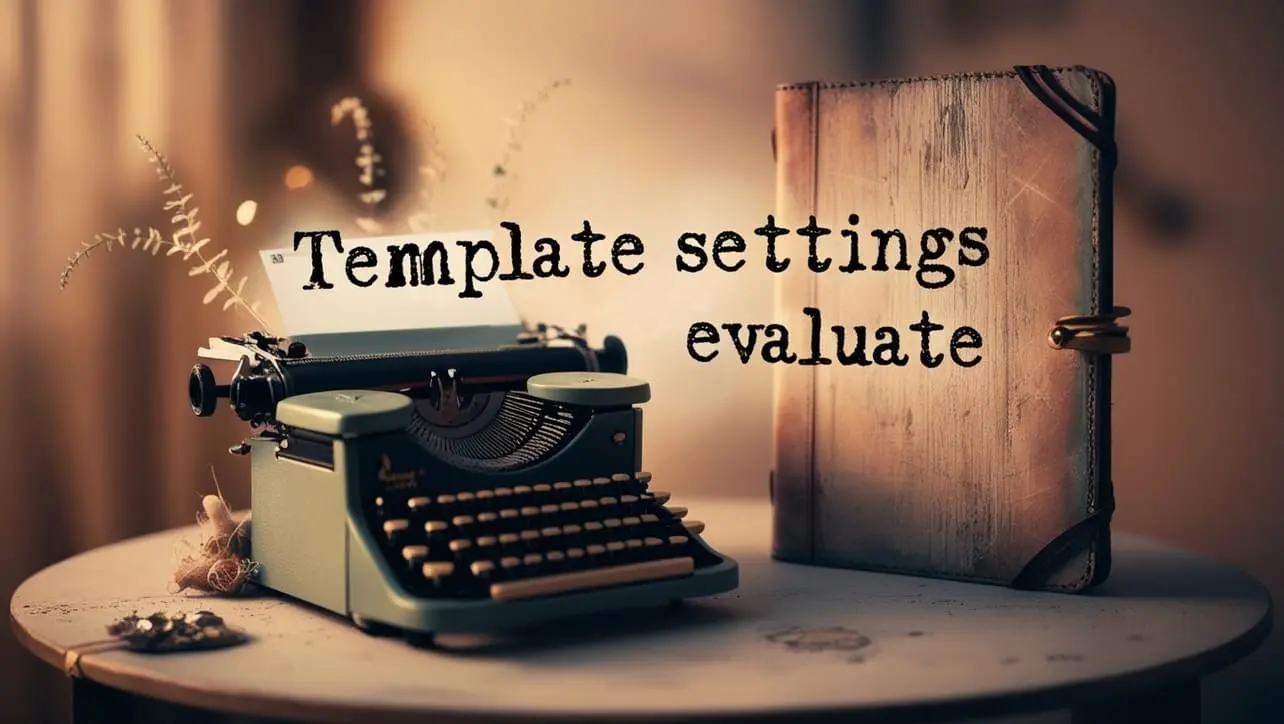
Lodash _.templateSettings.evaluate Property
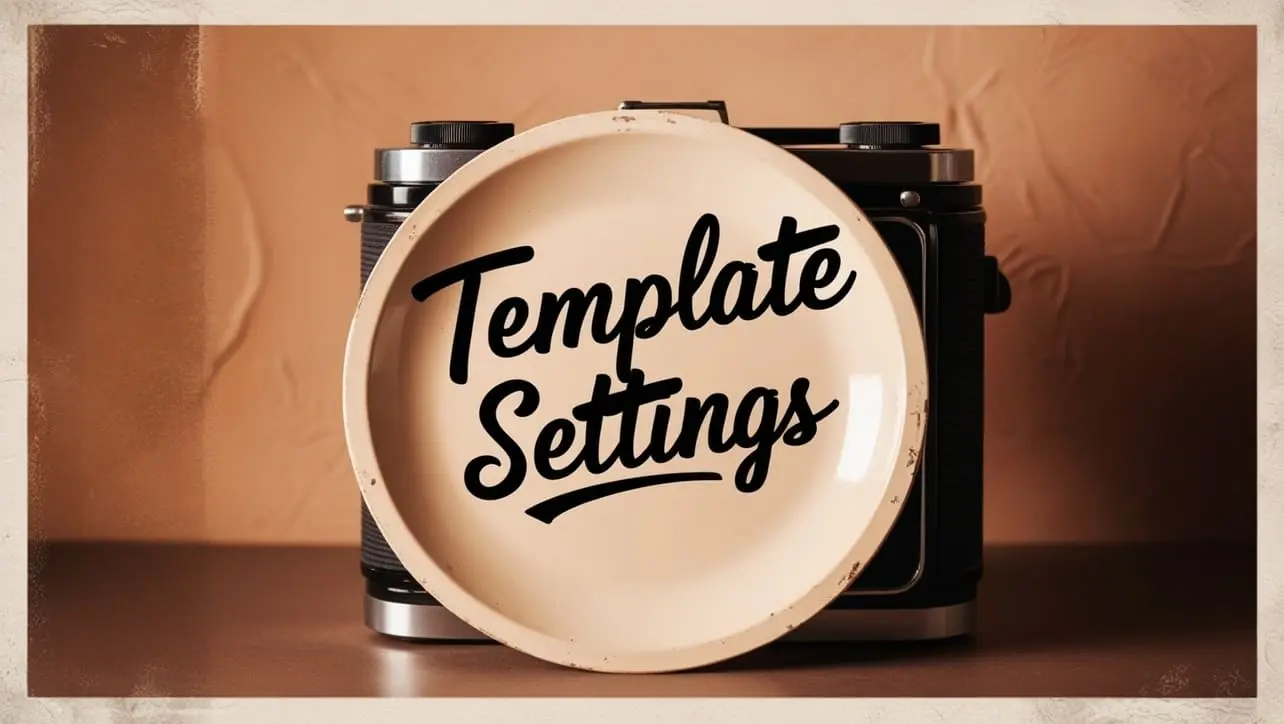
Lodash _.templateSettings Property
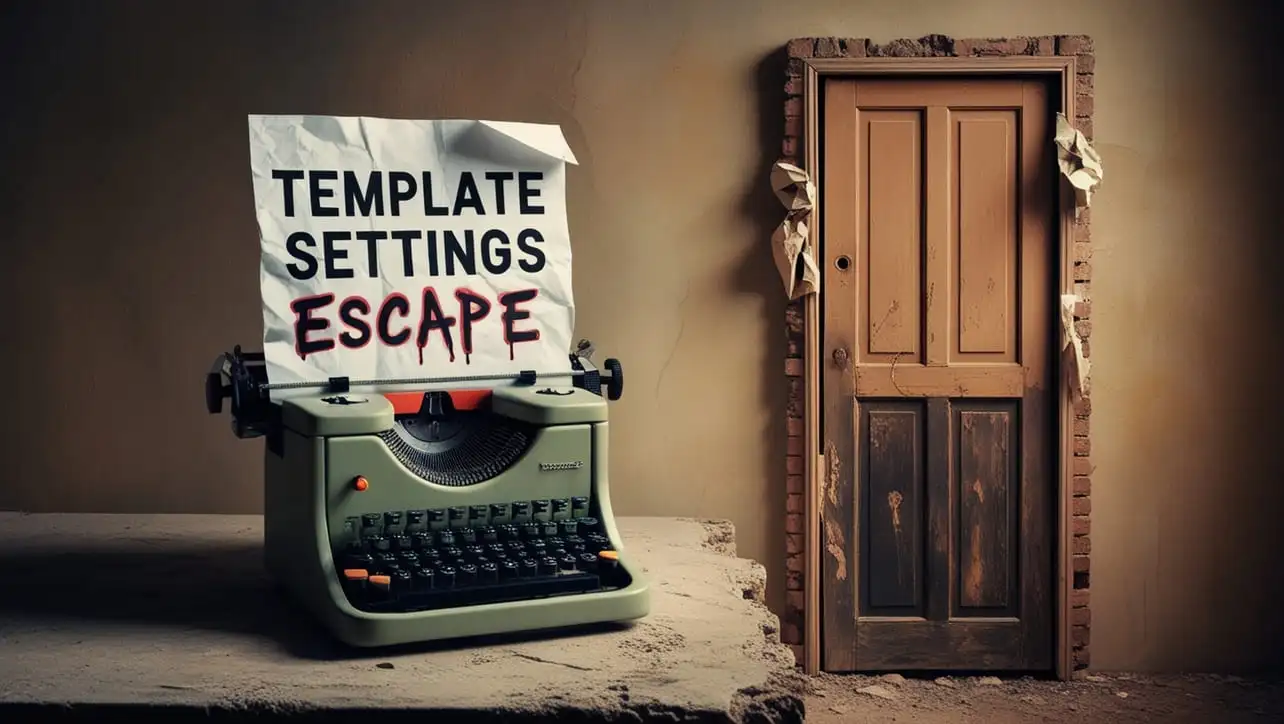
Lodash _.templateSettings.escape Property
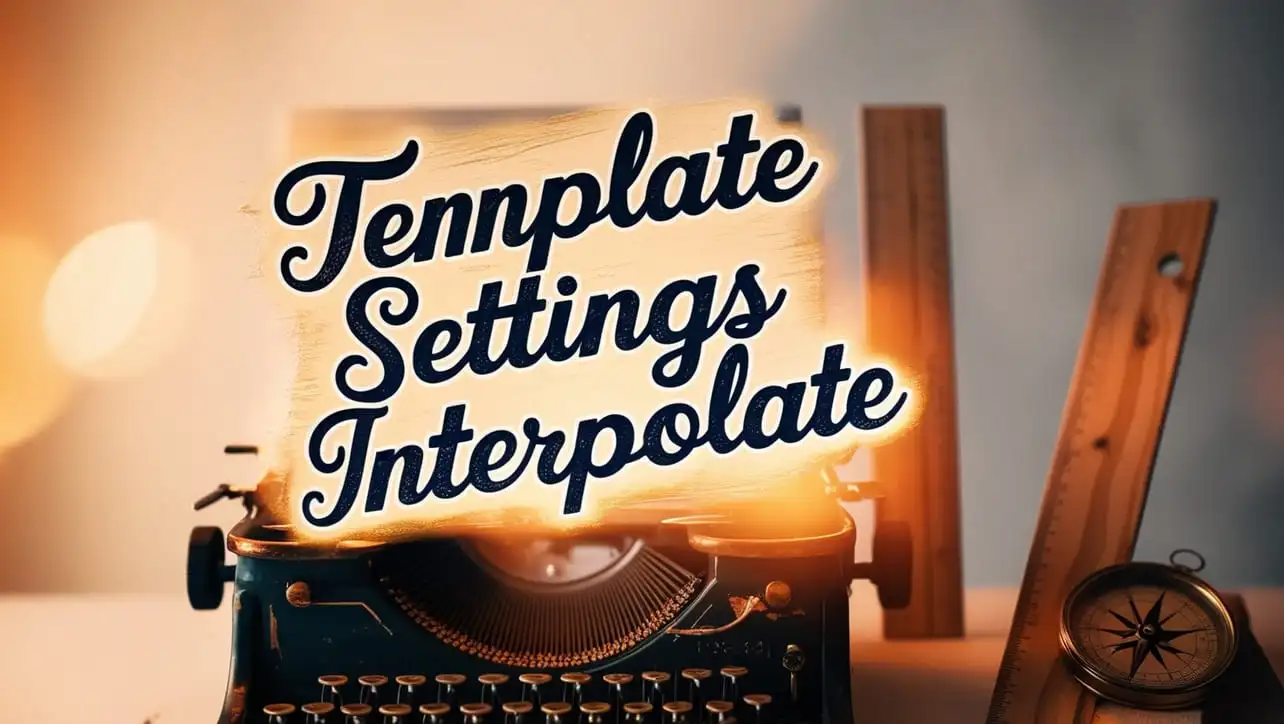
Lodash _.templateSettings.interpolate Property
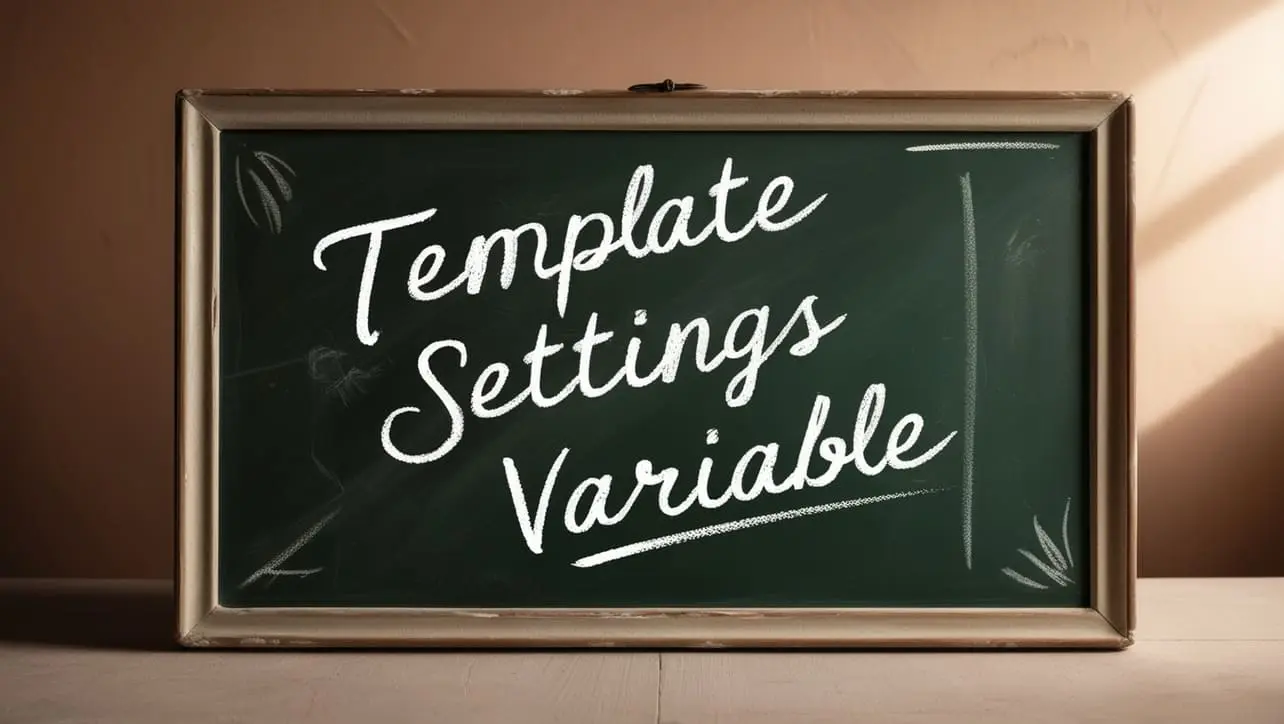
If you have any doubts regarding this article (Lodash _.stubTrue() Util Method), please comment here. I will help you immediately.