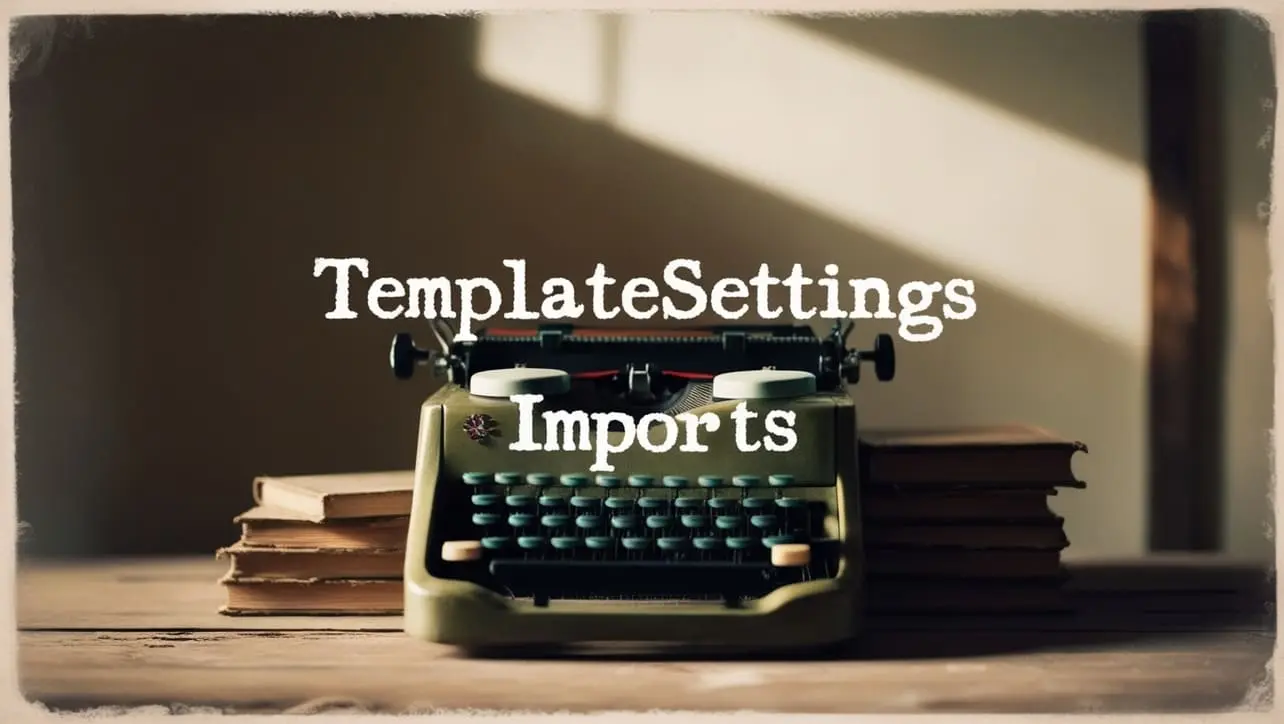
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.stubString() Util Method
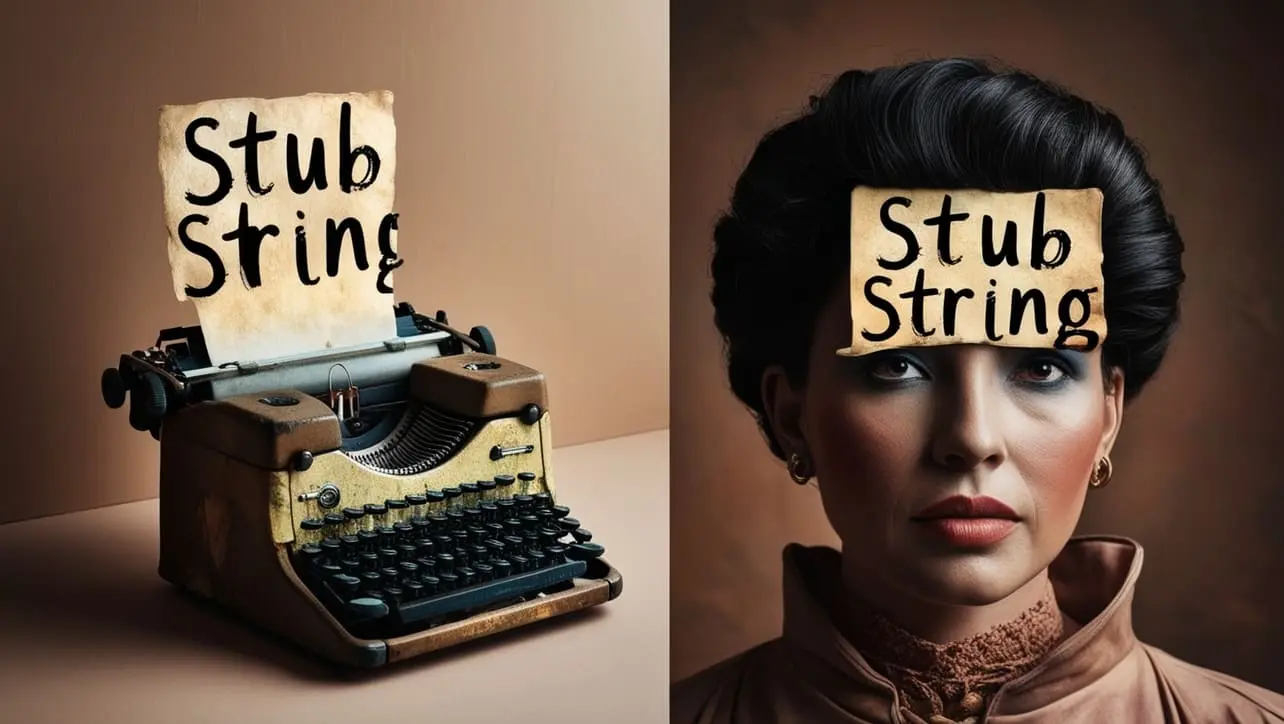
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, handling edge cases and providing default values is a common task. Lodash, a popular utility library, offers a range of methods to simplify such operations. Among these methods is _.stubString()
, a handy utility for creating placeholder string functions.
This method allows developers to quickly generate default string values or placeholders, enhancing code clarity and maintainability.
🧠 Understanding _.stubString() Method
The _.stubString()
method in Lodash returns an empty string. While this might seem trivial at first glance, it serves as a useful placeholder or default value in various scenarios, reducing the need for repetitive string initialization in code.
💡 Syntax
The syntax for the _.stubString()
method is straightforward:
_.stubString()
📝 Example
Let's dive into a simple example to illustrate the usage of the _.stubString()
method:
const _ = require('lodash');
const defaultName = _.stubString();
console.log(defaultName);
// Output: ''
In this example, _.stubString()
returns an empty string, which is assigned to the variable defaultName.
🏆 Best Practices
When working with the _.stubString()
method, consider the following best practices:
Default Values:
Use
_.stubString()
to provide default values or placeholders in function parameters or return statements. This enhances code readability and reduces the verbosity of default value assignments.example.jsCopiedfunction greet(name = _.stubString()) { return `Hello, ${name}!`; } console.log(greet()); // Output: Hello, !
Placeholder Functions:
Create placeholder functions using
_.stubString()
to stub out functionality during development or testing. This allows you to focus on implementing core features without worrying about specific details at early stages.example.jsCopiedconst fetchDataFromAPI = _.stubString(); // Implement actual API fetching logic later
Simplifying Conditions:
Simplify conditional logic by using
_.stubString()
as a default value when handling optional string parameters. This streamlines code and reduces the need for explicit null or undefined checks.example.jsCopiedfunction logMessage(message = _.stubString()) { console.log(`Message: ${message}`); } logMessage(); // Output: Message: logMessage('Hello, world!'); // Output: Message: Hello, world!
📚 Use Cases
Default Values:
When defining functions with optional string parameters,
_.stubString()
can be used to provide default values, ensuring consistent behavior.example.jsCopiedfunction generateMessage(name = _.stubString()) { return `Hello, ${name}!`; } console.log(generateMessage()); // Output: Hello, ! console.log(generateMessage('John')); // Output: Hello, John!
Placeholder Functions:
During the initial stages of development,
_.stubString()
can be utilized to create placeholder functions, allowing developers to focus on high-level architecture without diving into implementation details.example.jsCopiedconst fetchDataFromAPI = _.stubString(); // Implement actual API fetching logic later
Simplifying Conditions:
By using
_.stubString()
as default values for optional string parameters, conditional logic can be simplified, resulting in cleaner and more concise code.example.jsCopiedfunction logMessage(message = _.stubString()) { console.log(`Message: ${message}`); } logMessage(); // Output: Message: logMessage('Hello, world!'); // Output: Message: Hello, world!
🎉 Conclusion
The _.stubString()
method in Lodash offers a simple yet powerful utility for generating default string values and placeholders in JavaScript. Whether you're providing default values for function parameters, creating placeholder functions, or simplifying conditional logic, _.stubString()
serves as a versatile tool to enhance code readability and maintainability.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.stubString()
method in your Lodash projects.
👨💻 Join our Community:
Author
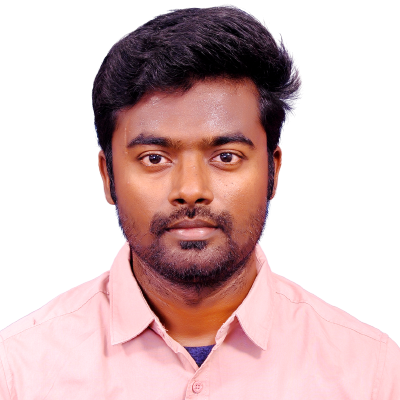
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
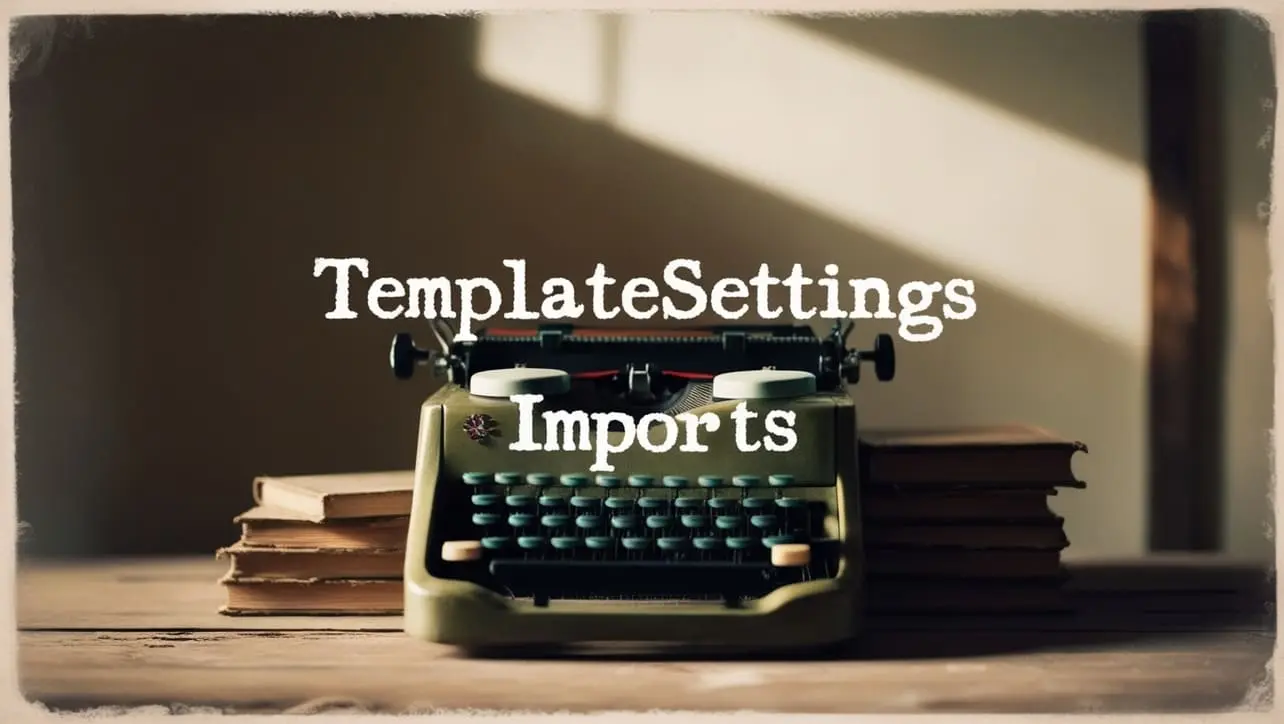
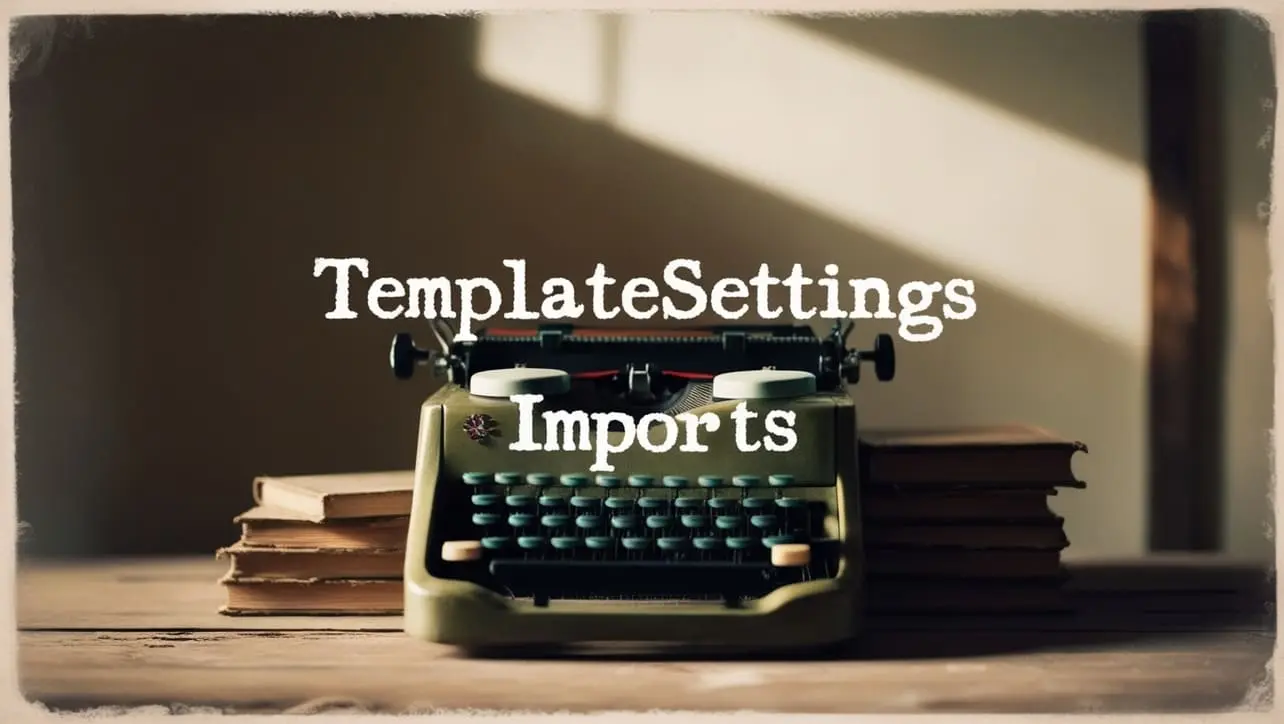
Lodash _.templateSettings.imports Property
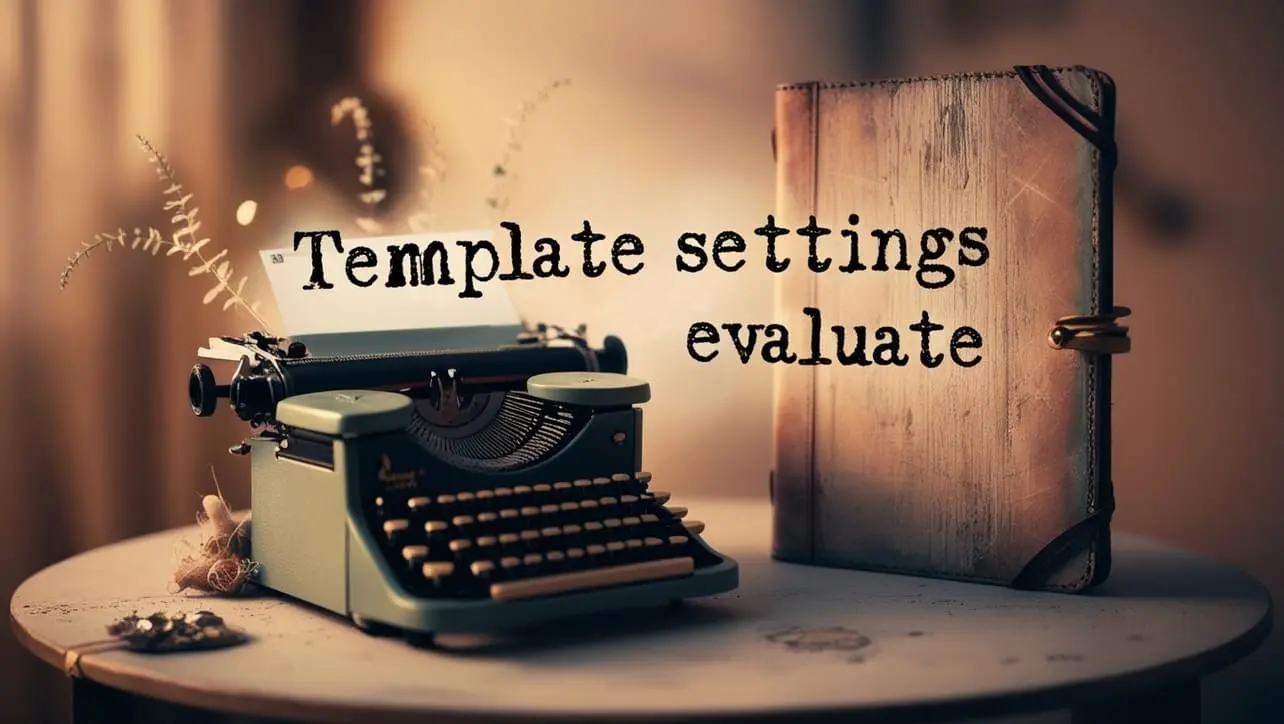
Lodash _.templateSettings.evaluate Property
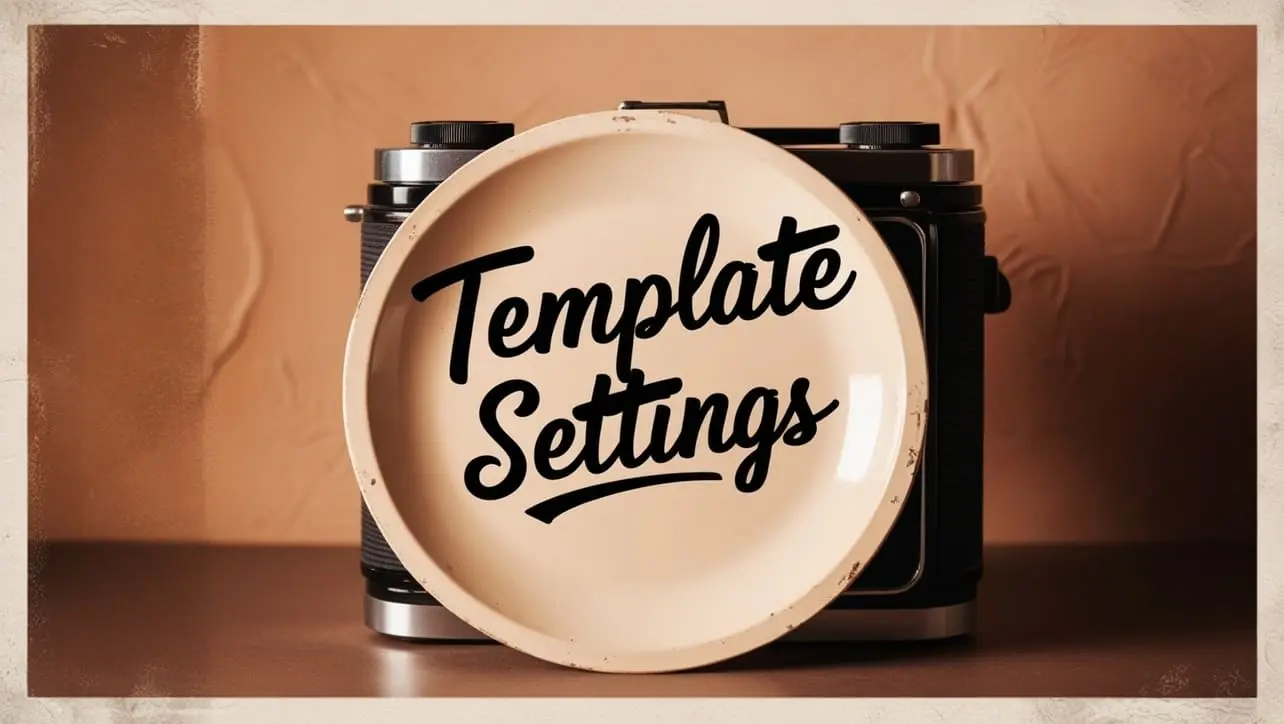
Lodash _.templateSettings Property
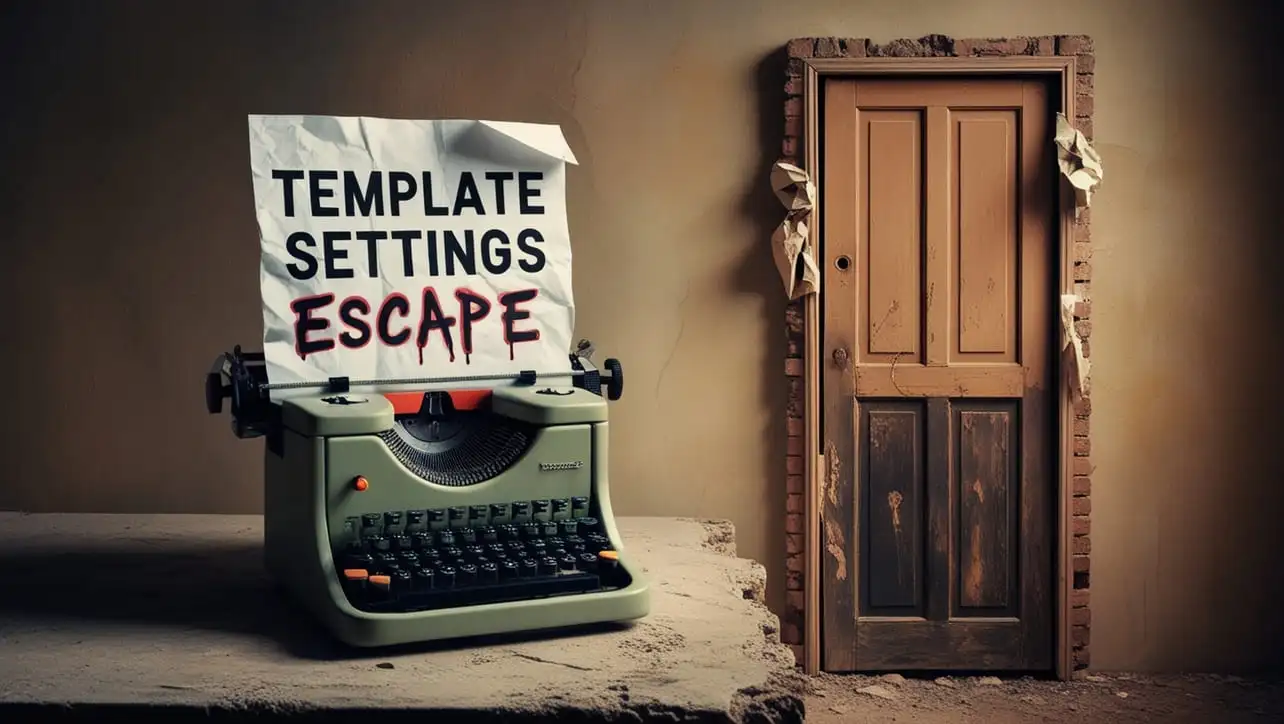
Lodash _.templateSettings.escape Property
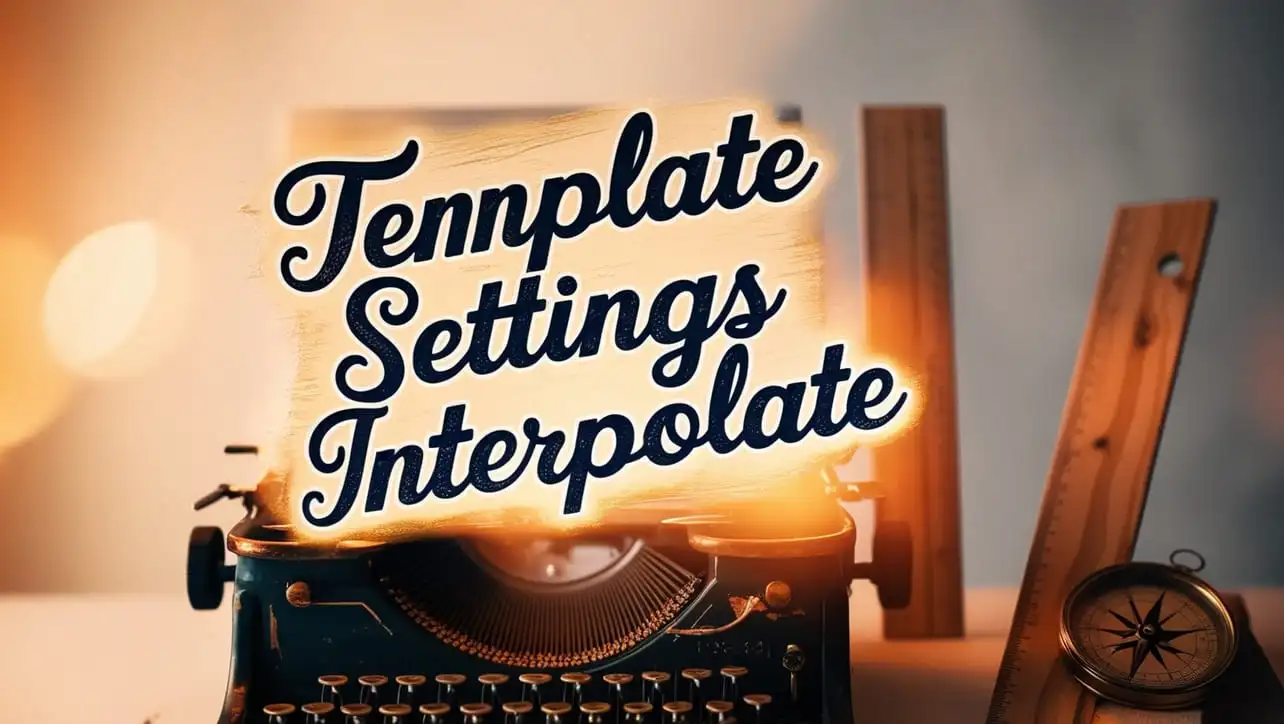
Lodash _.templateSettings.interpolate Property
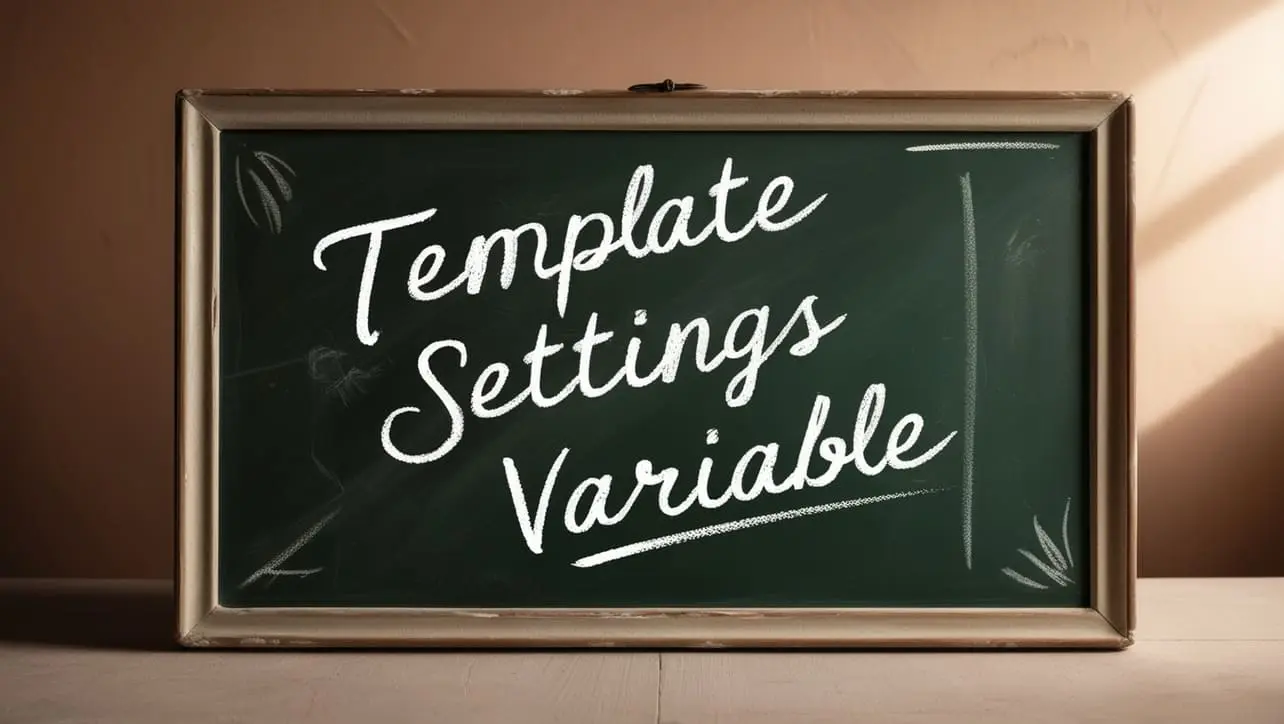
If you have any doubts regarding this article (Lodash _.stubString() Util Method), please comment here. I will help you immediately.