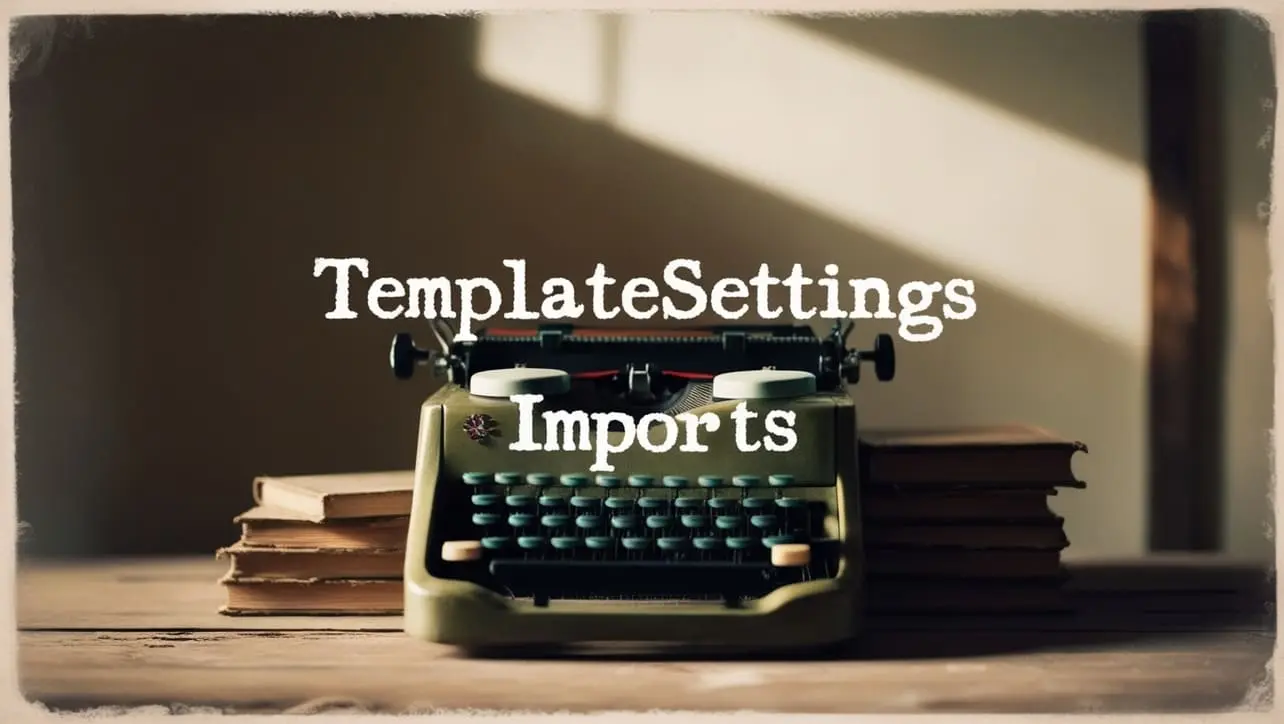
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.stubObject() Util Method
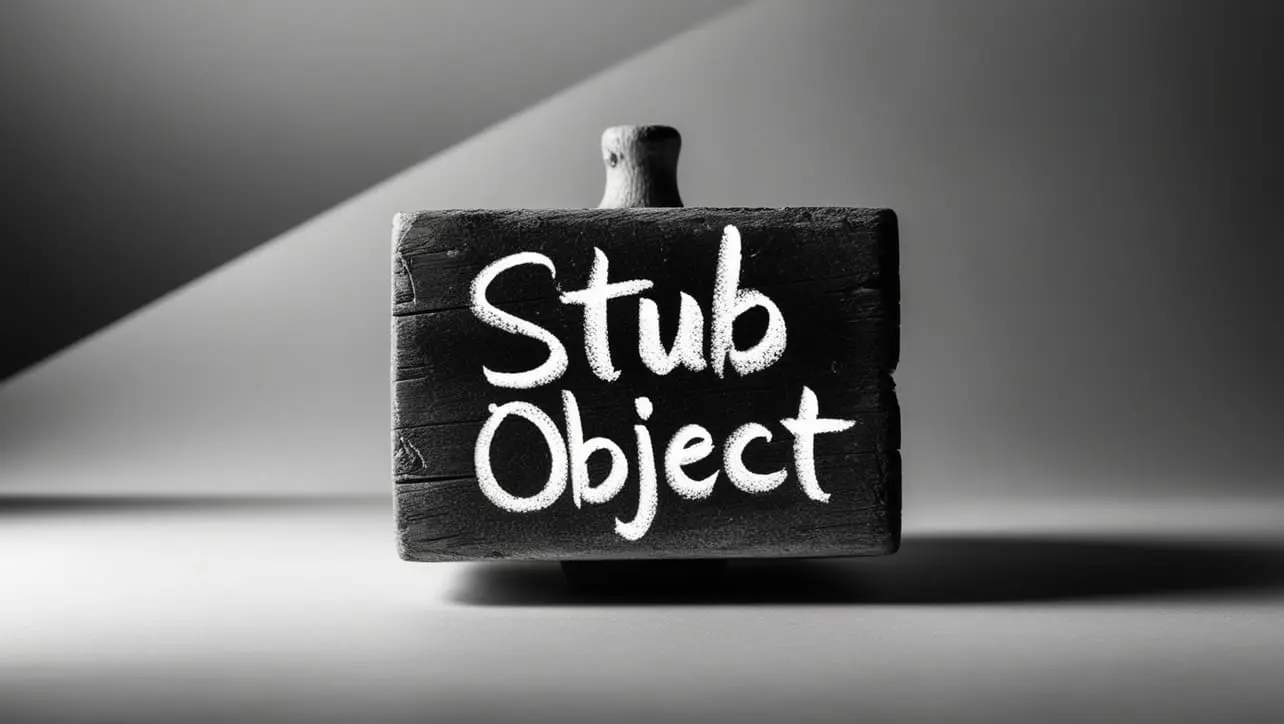
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, creating placeholder objects is a common requirement, especially when defining the structure of data or functions. Lodash, a popular utility library, offers a convenient solution to this need with its _.stubObject()
method.
This method generates a stub object with undefined properties, providing a foundation for further development or testing.
🧠 Understanding _.stubObject() Method
The _.stubObject()
method in Lodash is a utility function designed to create a stub object with undefined properties. This allows developers to define the structure of an object without specifying its values, providing flexibility and convenience in various programming scenarios.
💡 Syntax
The syntax for the _.stubObject()
method is straightforward:
_.stubObject()
📝 Example
Let's dive into a simple example to illustrate the usage of the _.stubObject()
method:
const _ = require('lodash');
const stub = _.stubObject();
console.log(stub);
// Output: {}
In this example, the stub object is created using _.stubObject()
, resulting in an empty object with undefined properties.
🏆 Best Practices
When working with the _.stubObject()
method, consider the following best practices:
Define Placeholder Objects:
Use
_.stubObject()
to define placeholder objects when the structure of data or functions needs to be established initially, with the intention of later filling in the details.example.jsCopiedconst userSchema = { id: _.uniqueId('user_'), name: 'John Doe', email: 'john@example.com', // Stub object for additional properties profile: _.stubObject(), }; console.log(userSchema);
Simplify Testing:
In unit testing scenarios, utilize
_.stubObject()
to create mock objects with placeholder properties, facilitating the setup of test cases and assertions.example.jsCopiedconst mockUserData = { id: 'user_123', name: 'Jane Doe', email: 'jane@example.com', // Stub object for additional properties profile: _.stubObject(), }; console.log(mockUserData);
📚 Use Cases
Data Structure Definition:
When defining the structure of complex data objects,
_.stubObject()
can be employed to create placeholders for properties that will be populated dynamically.example.jsCopiedconst dataStructure = { metadata: { // Stub object for metadata properties properties: _.stubObject(), // Stub object for nested metadata details: { // Stub object for details properties info: _.stubObject(), }, }, }; console.log(dataStructure);
Function Parameter Initialization:
In scenarios where functions expect objects as parameters,
_.stubObject()
can be used to initialize placeholder objects before populating them with relevant data.example.jsCopiedfunction processUser(userData) { const { id, name, email, profile } = userData; // Stub object for additional processing const additionalInfo = _.stubObject(); // Process user data... } const userData = { id: 'user_456', name: 'Alice Smith', email: 'alice@example.com', // Stub object for profile data profile: _.stubObject(), }; processUser(userData);
🎉 Conclusion
The _.stubObject()
method in Lodash offers a convenient way to create placeholder objects with undefined properties. Whether you're defining data structures, setting up mock objects for testing, or initializing function parameters, this utility function provides flexibility and simplicity in JavaScript development.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.stubObject()
method in your Lodash projects.
👨💻 Join our Community:
Author
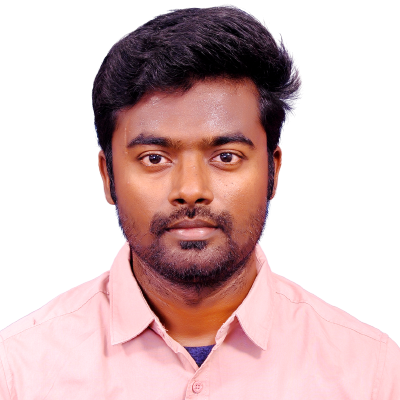
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
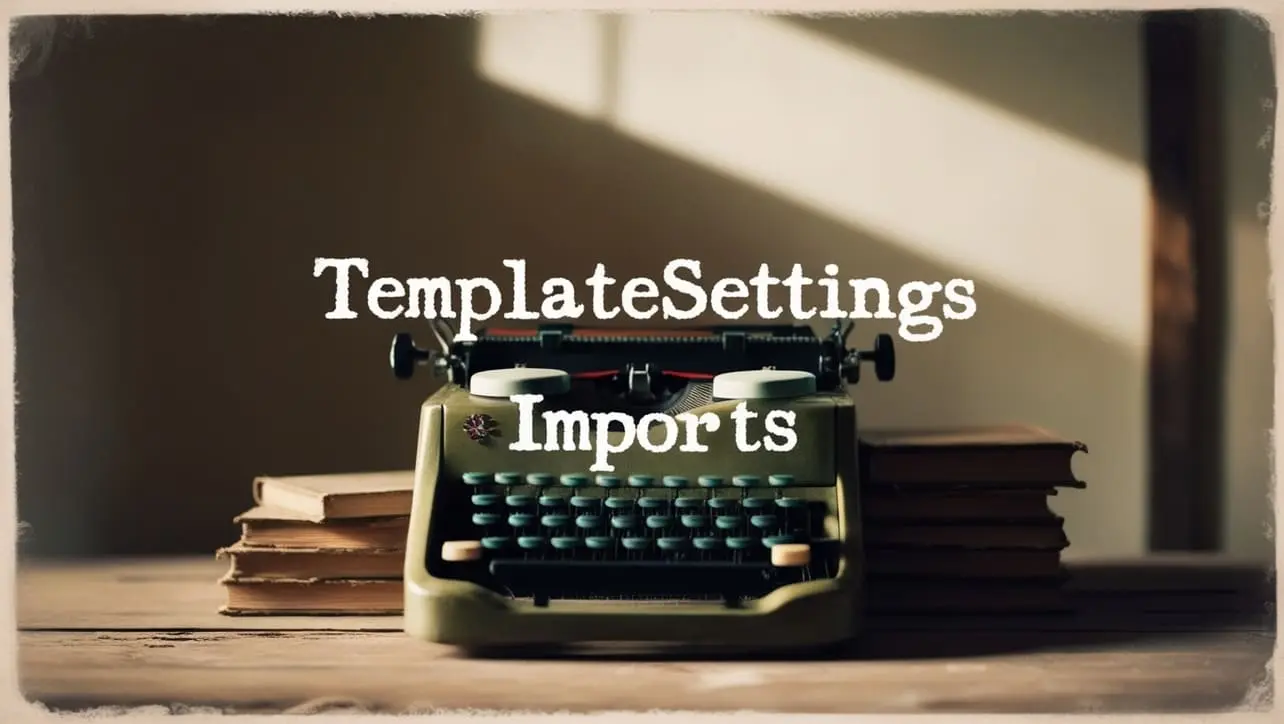
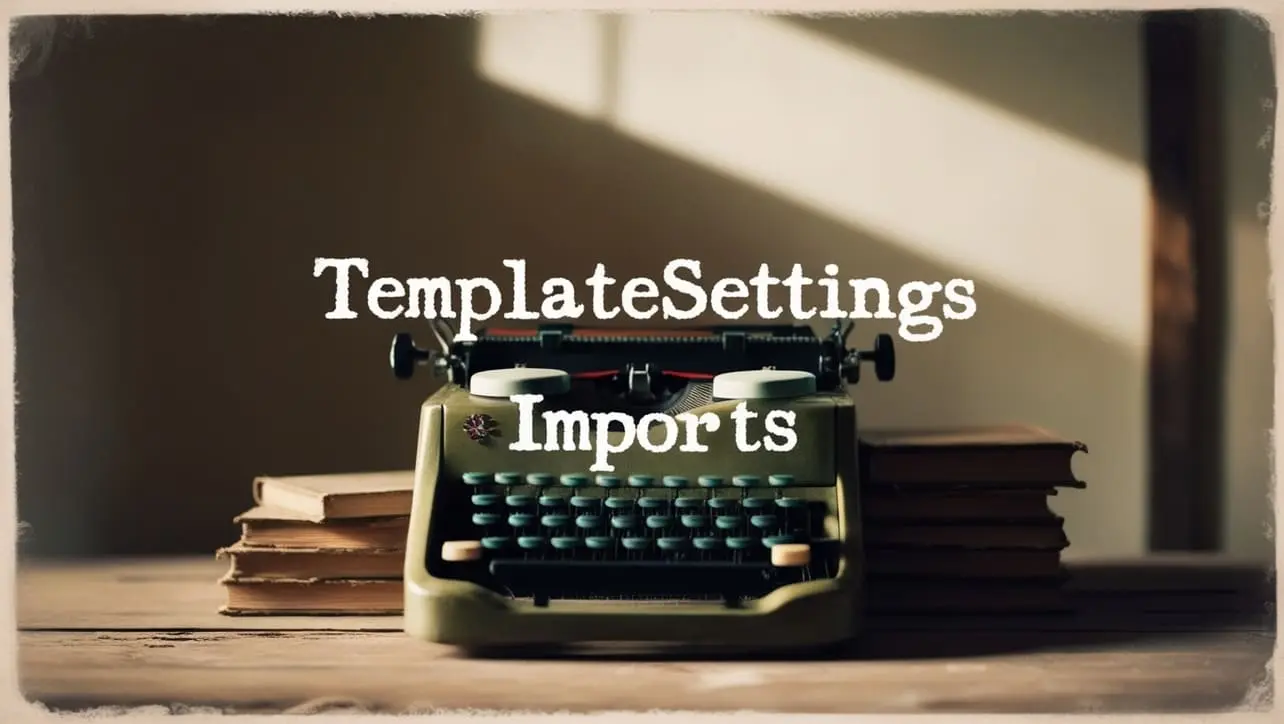
Lodash _.templateSettings.imports Property
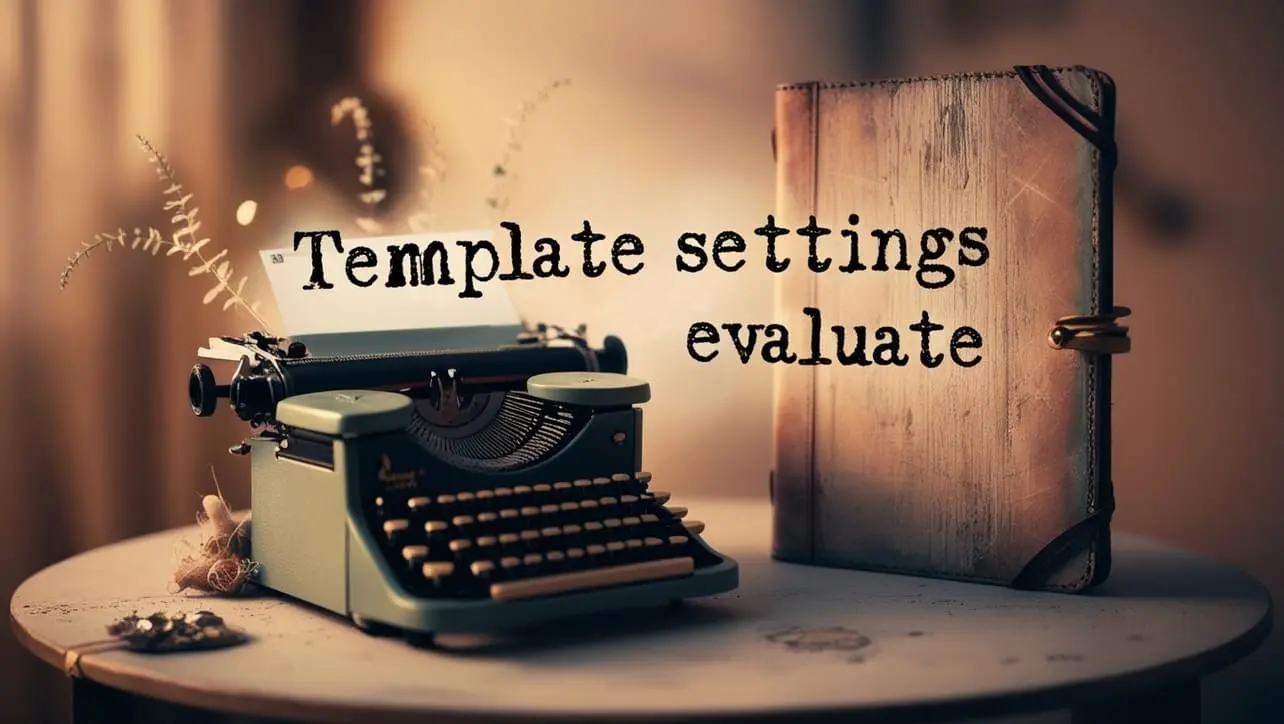
Lodash _.templateSettings.evaluate Property
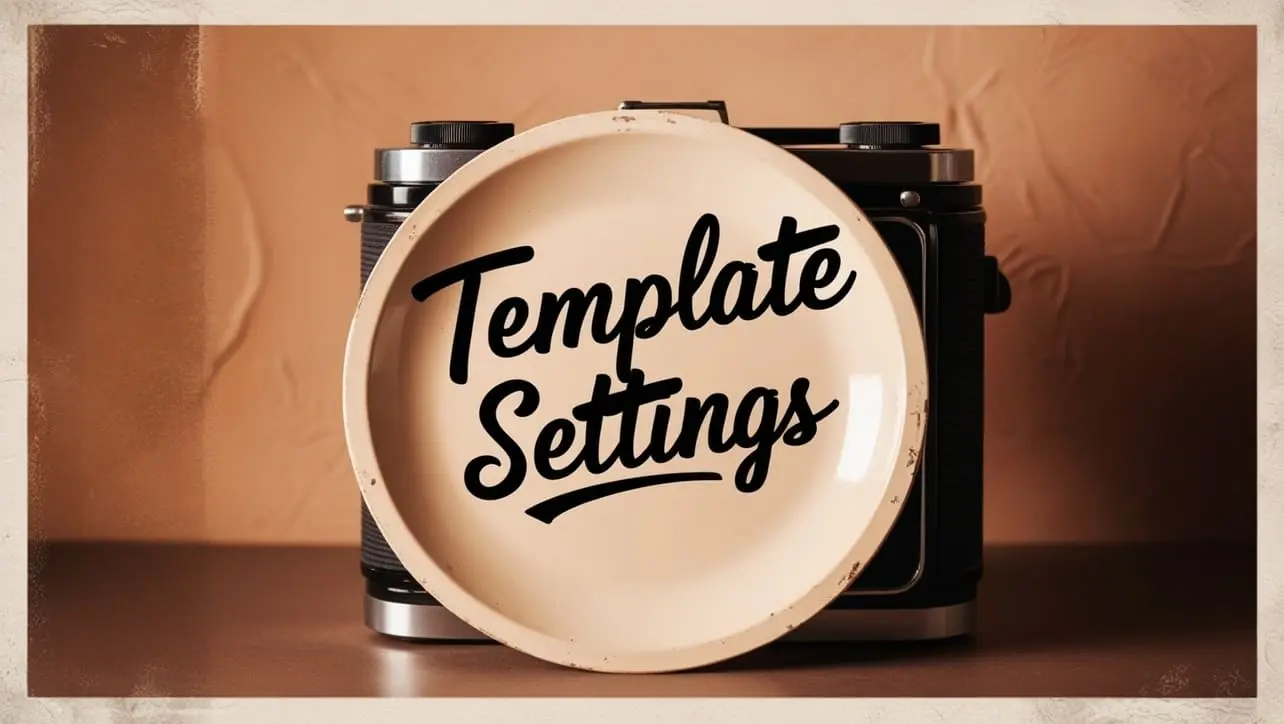
Lodash _.templateSettings Property
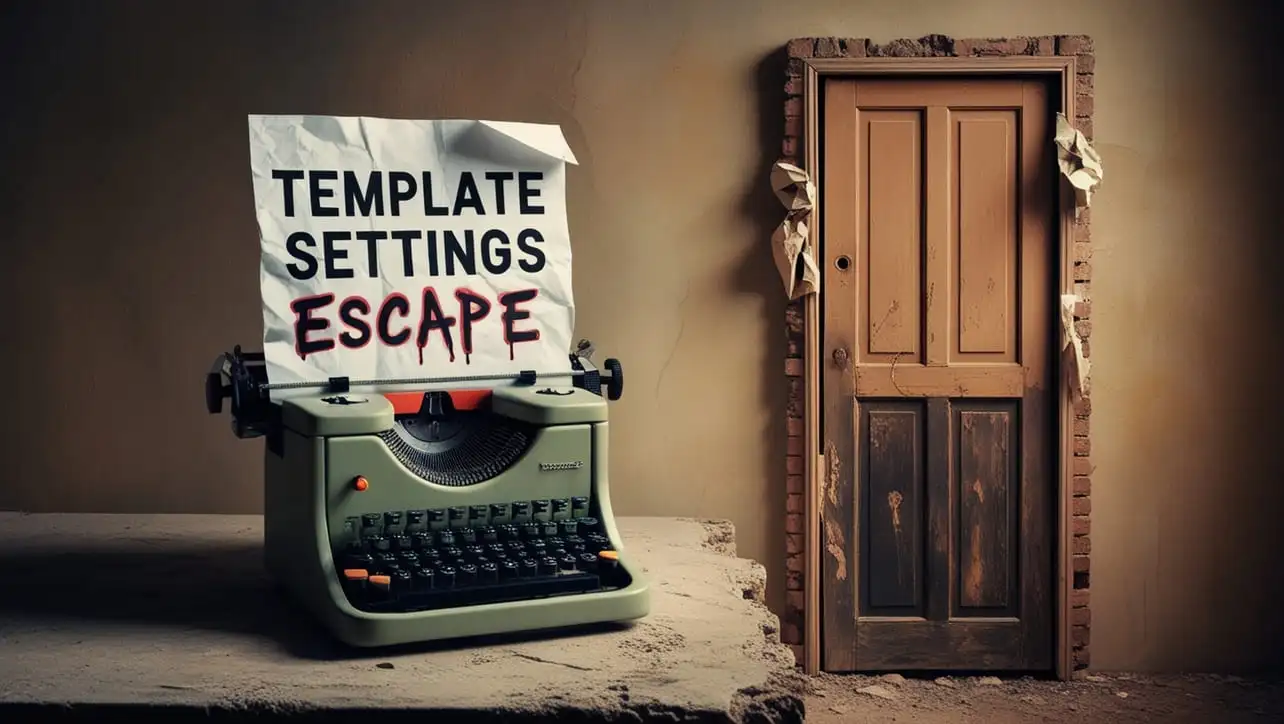
Lodash _.templateSettings.escape Property
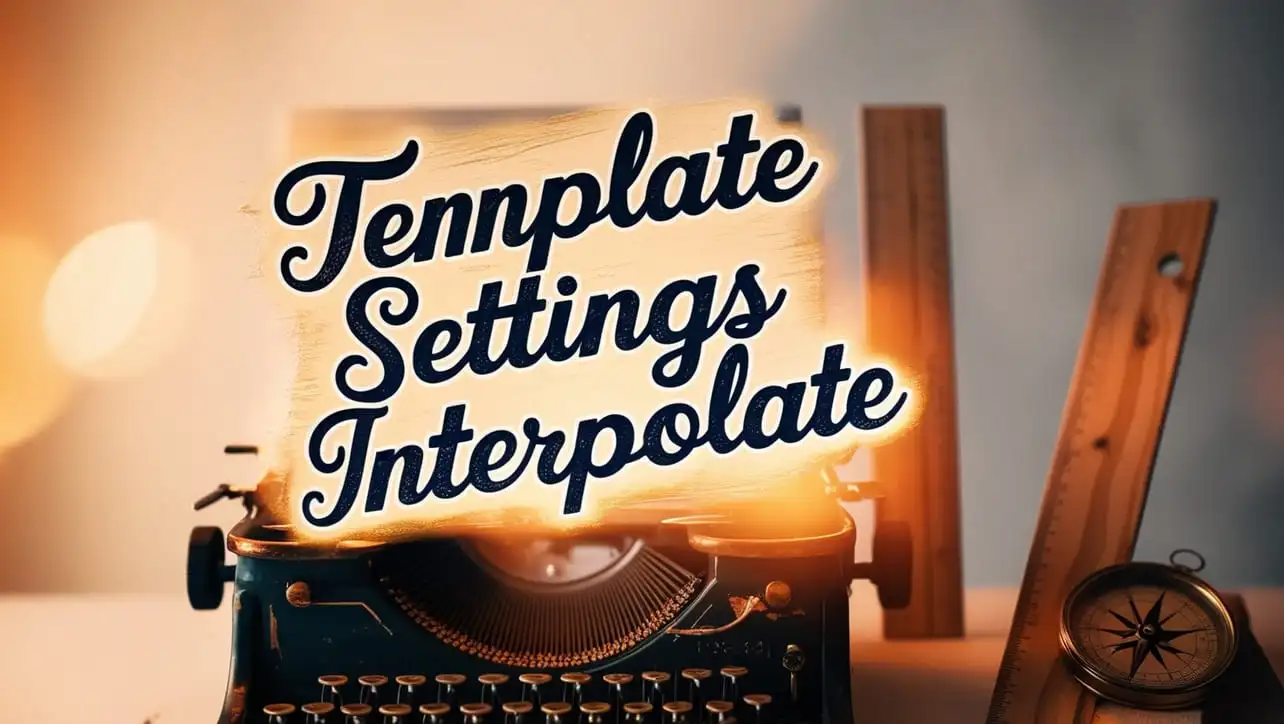
Lodash _.templateSettings.interpolate Property
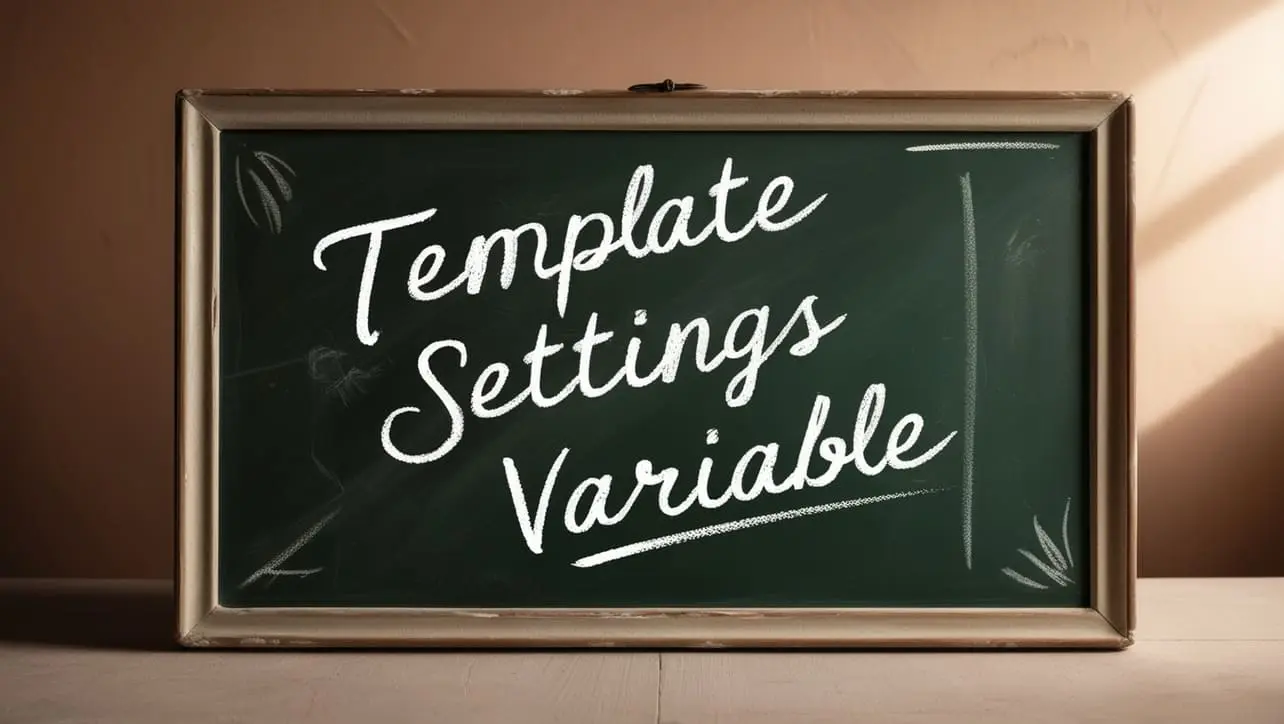
If you have any doubts regarding this article (Lodash _.stubObject() Util Method), please comment here. I will help you immediately.