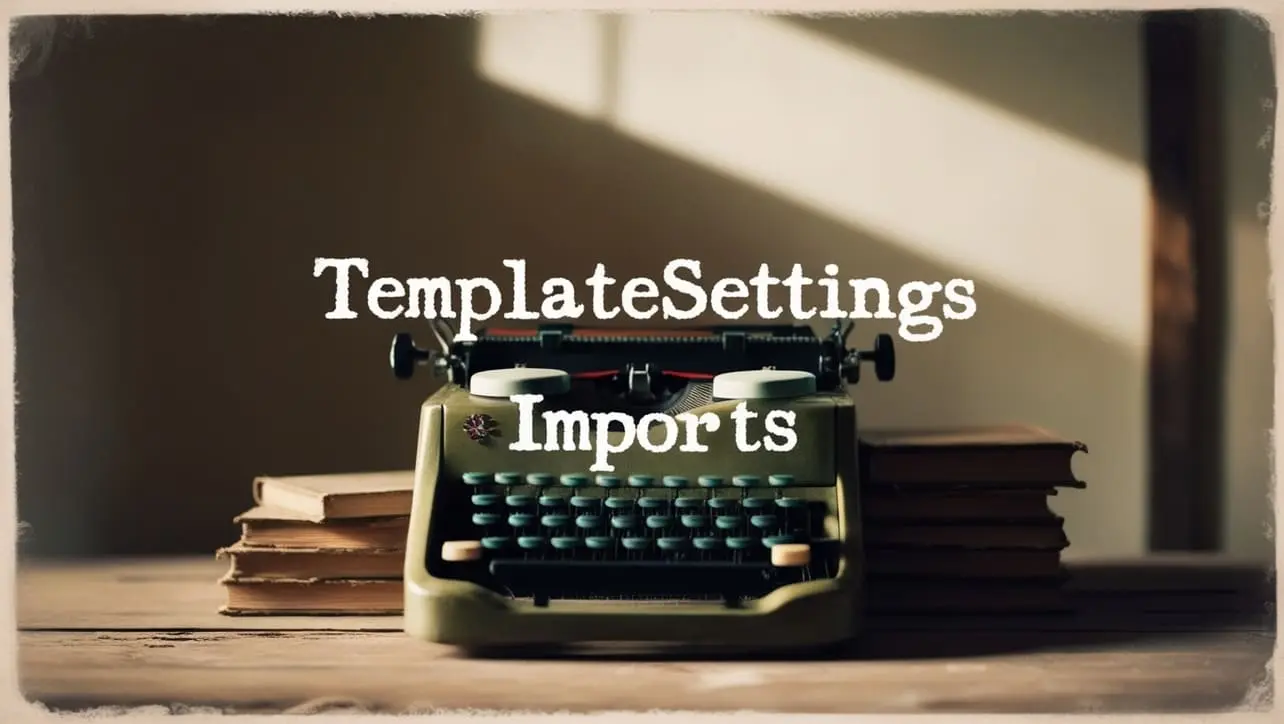
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.stubFalse() Util Method
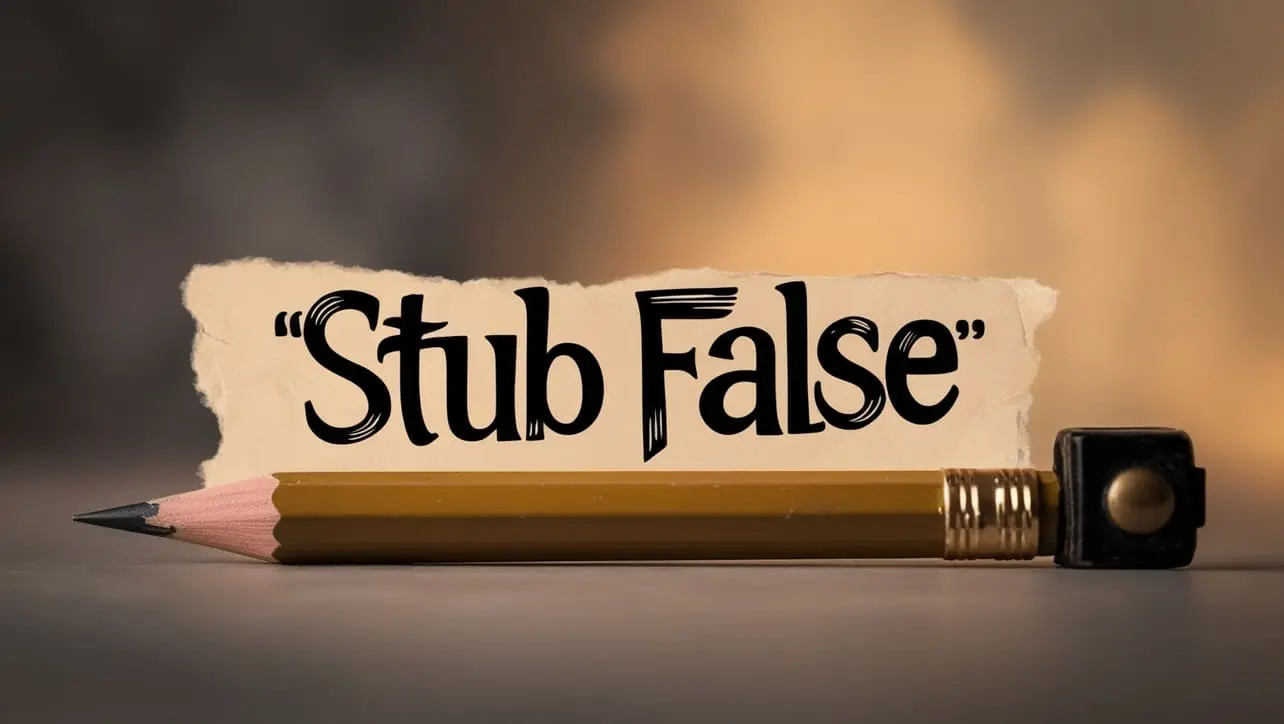
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, simplifying code and improving readability are paramount goals. Lodash, a widely used utility library, offers a plethora of functions to achieve these objectives. Among these functions is _.stubFalse()
, a versatile utility method that returns false regardless of the arguments passed to it.
This seemingly simple method can be incredibly useful in various scenarios, aiding developers in writing cleaner and more expressive code.
🧠 Understanding _.stubFalse() Method
The _.stubFalse()
utility method in Lodash is a straightforward function that always returns false. While this might seem trivial at first glance, it serves as a valuable tool for simplifying conditional logic, especially in scenarios where a falsy value is needed.
💡 Syntax
The syntax for the _.stubFalse()
method is straightforward:
_.stubFalse()
📝 Example
Let's dive into a simple example to illustrate the usage of the _.stubFalse()
method:
const _ = require('lodash');
const result = _.stubFalse();
console.log(result);
// Output: false
In this example, _.stubFalse()
is invoked without any arguments, and it returns false as expected.
🏆 Best Practices
When working with the _.stubFalse()
method, consider the following best practices:
Simplifying Conditional Statements:
Use
_.stubFalse()
to simplify conditional statements where a falsy value is required. This can lead to cleaner and more readable code.example.jsCopiedconst isLoggedIn = false; const isAdmin = true; if(isLoggedIn && isAdmin) { // Perform admin-specific actions } else { // Perform actions for non-admin users }
Placeholder for Function Arguments:
In cases where you need to pass a function that always returns false as an argument,
_.stubFalse()
can serve as a convenient placeholder.example.jsCopiedconst users = [ { name: 'Alice', isAdmin: true }, { name: 'Bob', isAdmin: _.stubFalse() }, { name: 'Charlie', isAdmin: false } ]; const nonAdminUsers = users.filter(user => !user.isAdmin); console.log(nonAdminUsers); // Output: [{ name: 'Charlie', isAdmin: false }]
Improving Code Readability:
By using
_.stubFalse()
in appropriate contexts, you can enhance the readability of your code by clearly conveying the intention to return false.example.jsCopiedconst shouldProcessData = _.stubFalse(); if(shouldProcessData()) { // Process data logic } else { console.log('Data processing is disabled.'); }
📚 Use Cases
Default Return Value:
When implementing functions that require a default return value of false,
_.stubFalse()
can serve as a concise and expressive solution.example.jsCopiedfunction validateInput(input) { if(!input) { return _.stubFalse(); } // Validation logic }
Mocking Functions:
During testing or development,
_.stubFalse()
can be used to mock functions that need to return false without executing any additional logic.example.jsCopiedconst validateUser = _.stubFalse(); // Use validateUser function in testing scenarios
Placeholder for Future Implementations:
In cases where a function or conditional logic is not yet implemented,
_.stubFalse()
can act as a temporary placeholder until the functionality is developed.example.jsCopiedconst isFeatureEnabled = _.stubFalse(); if(isFeatureEnabled()) { // Feature-specific logic } else { console.log('Feature is currently disabled.'); }
🎉 Conclusion
The _.stubFalse()
utility method in Lodash provides a straightforward yet powerful way to always return false. Whether simplifying conditional logic, providing default return values, or acting as a placeholder, this method offers versatility and clarity in code implementation.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.stubFalse()
method in your Lodash projects.
👨💻 Join our Community:
Author
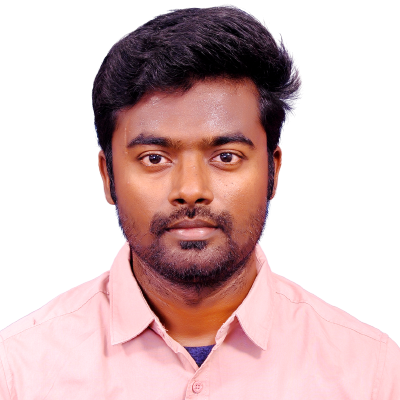
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
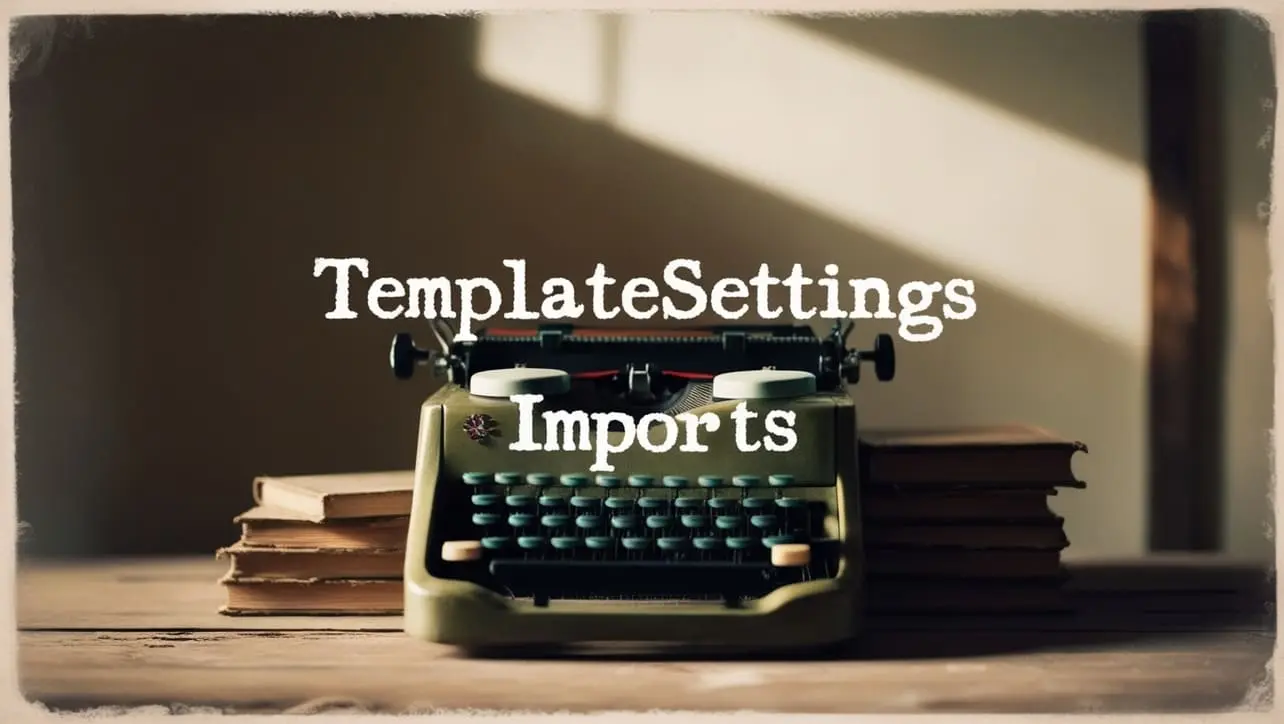
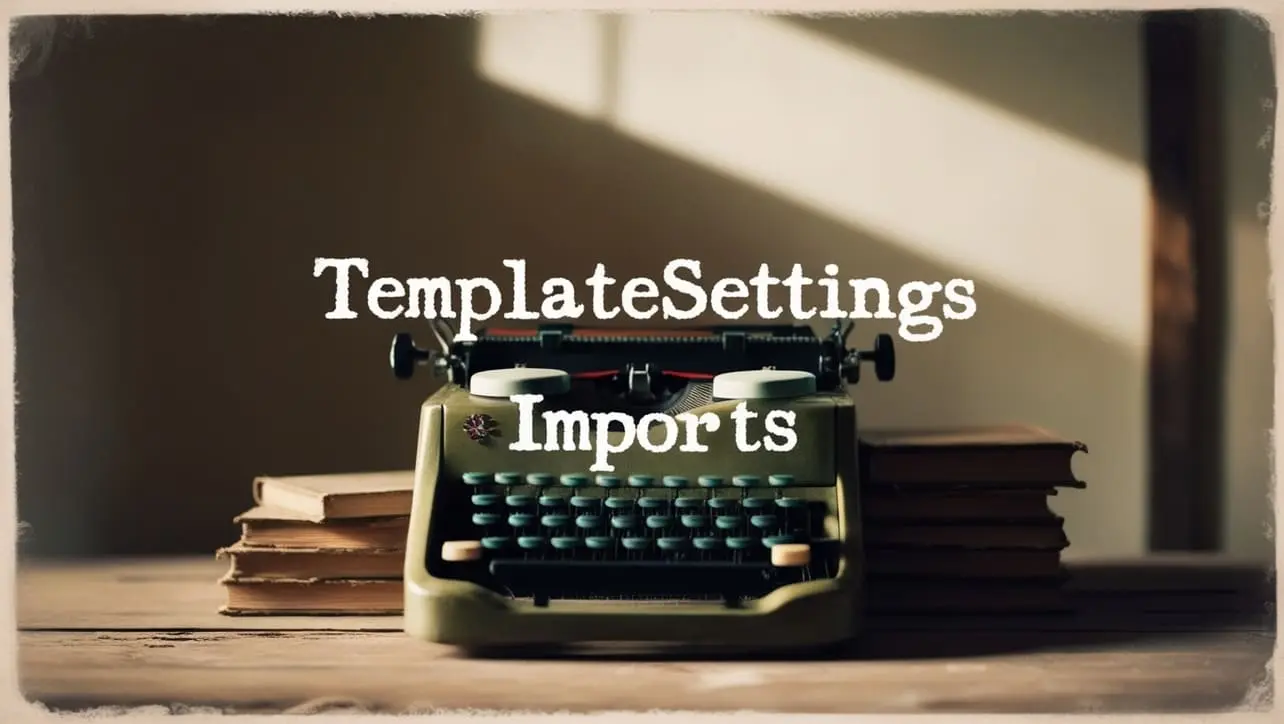
Lodash _.templateSettings.imports Property
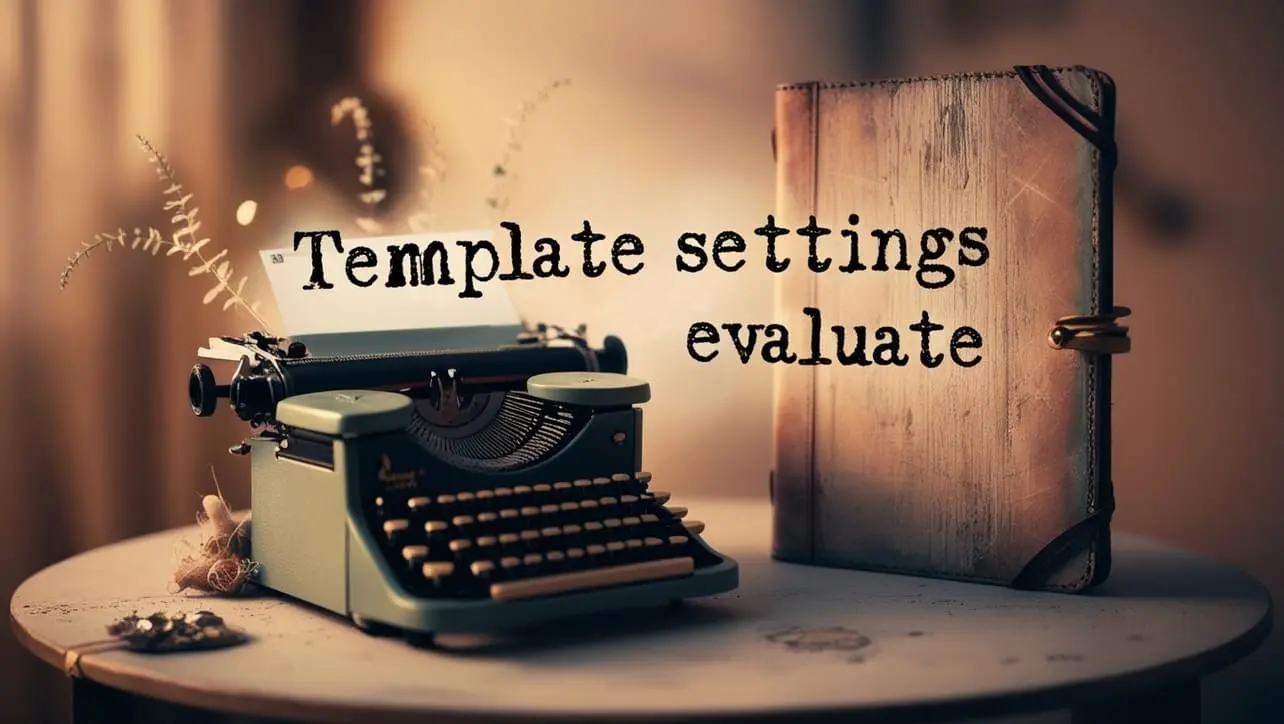
Lodash _.templateSettings.evaluate Property
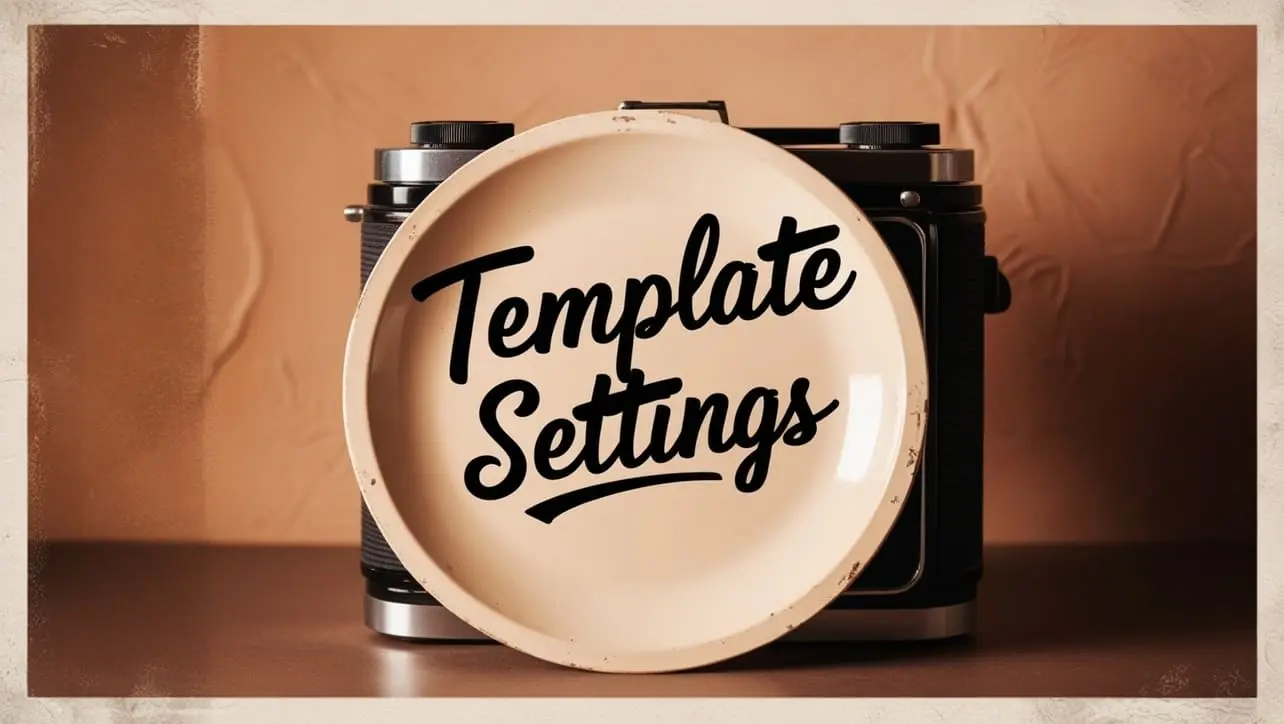
Lodash _.templateSettings Property
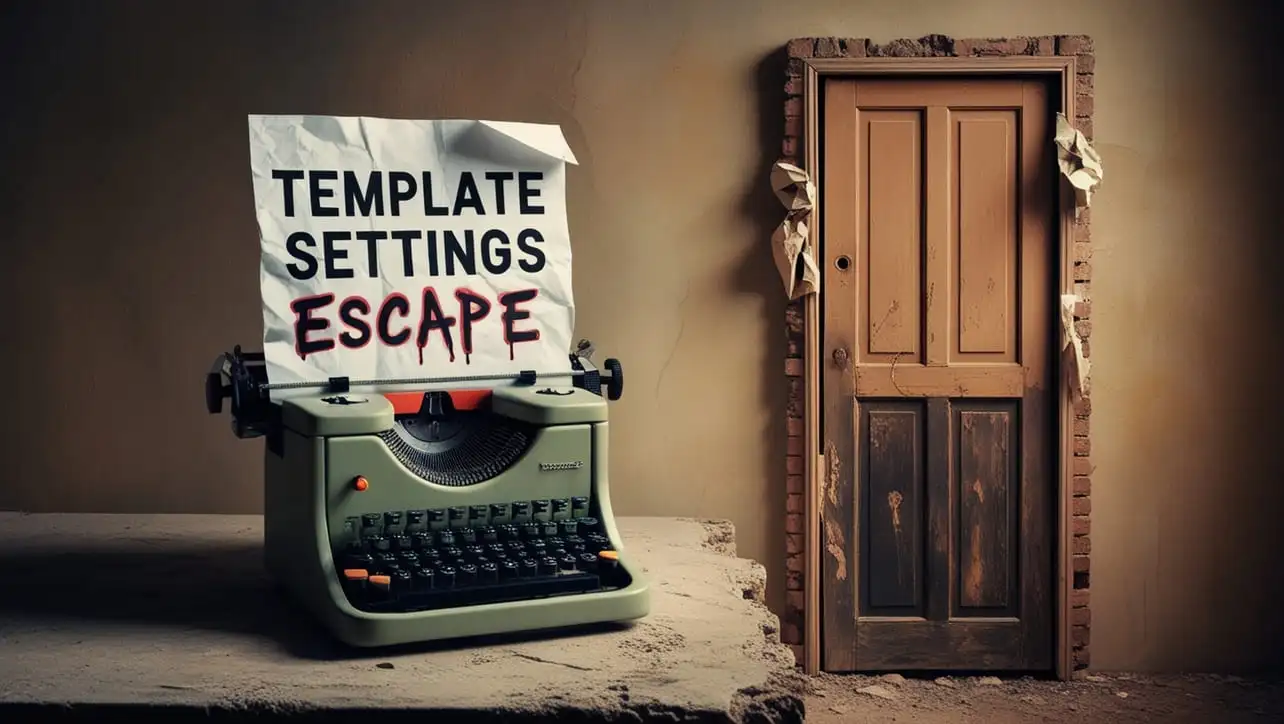
Lodash _.templateSettings.escape Property
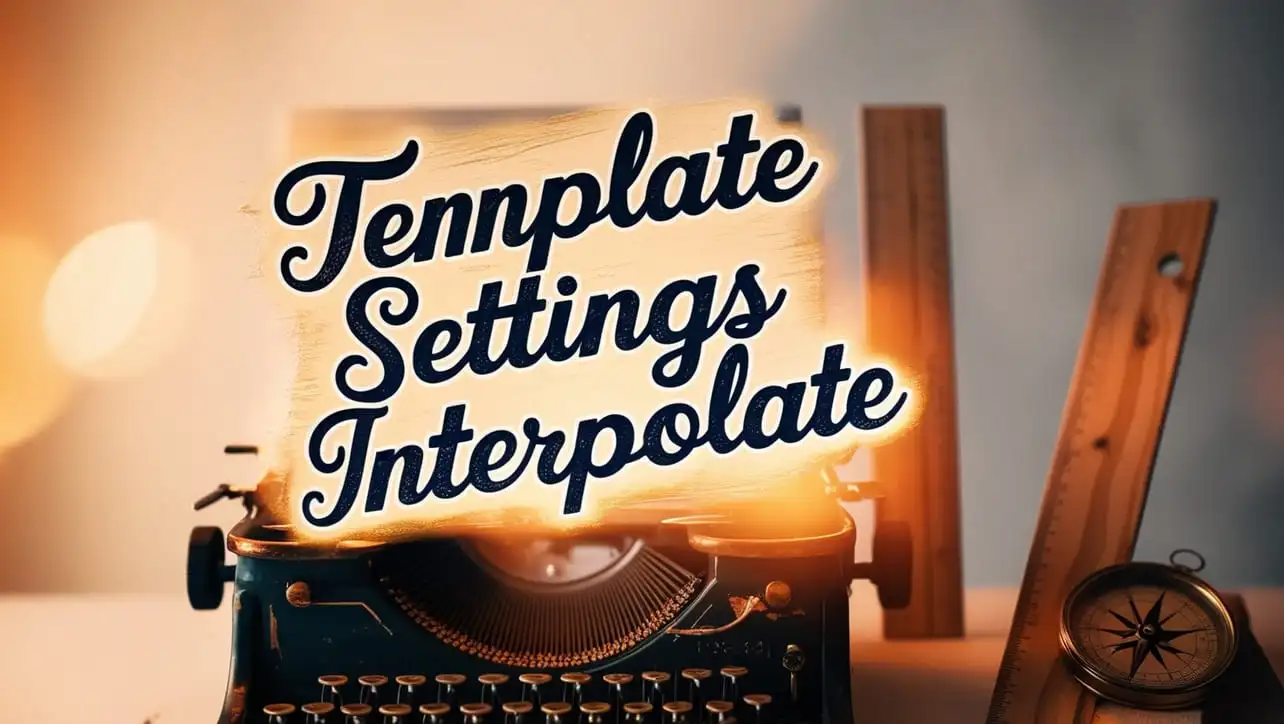
Lodash _.templateSettings.interpolate Property
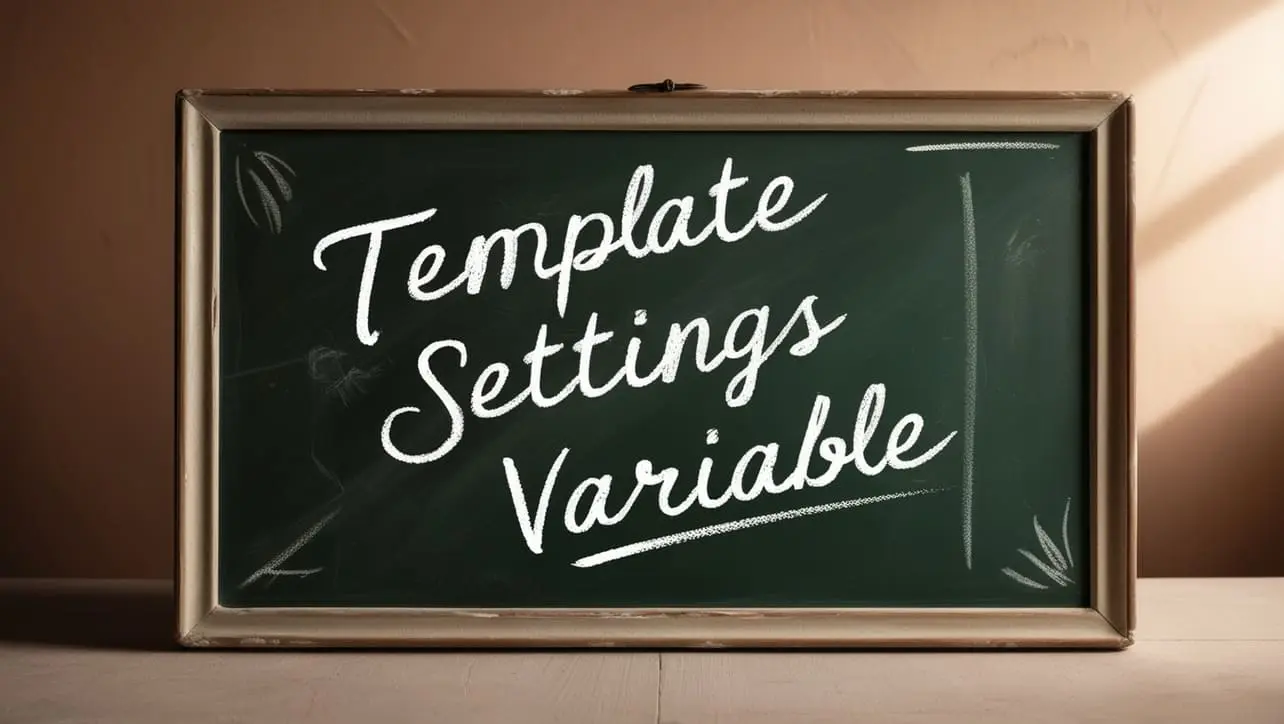
If you have any doubts regarding this article (Lodash _.stubFalse() Util Method), please comment here. I will help you immediately.