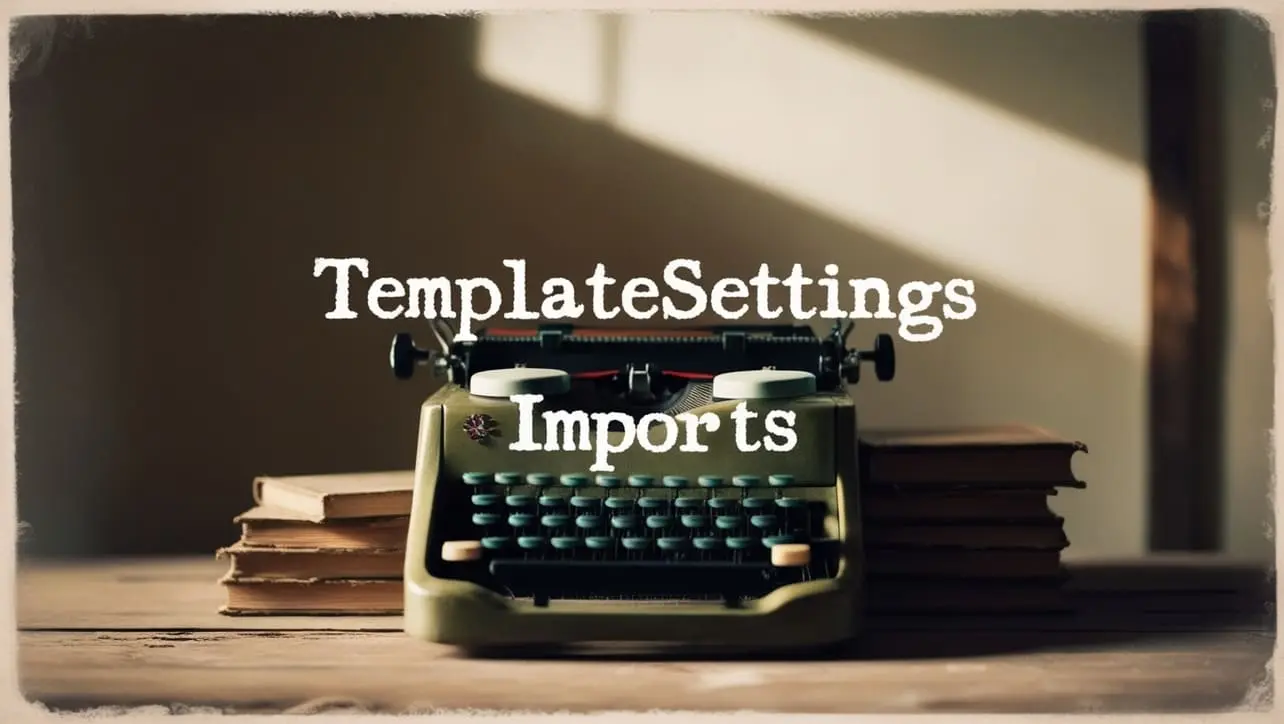
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.stubArray() Util Method

Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, simplifying code and improving its readability are common goals. Lodash, a popular utility library, offers various functions to achieve these objectives. One such function is _.stubArray()
, a versatile utility method that creates an empty array-like object.
This method is particularly useful for initializing placeholders or default values in functions, enhancing code clarity and maintainability.
🧠 Understanding _.stubArray() Method
The _.stubArray()
method in Lodash creates an empty array-like object. It allows developers to easily generate placeholder arrays or default values, eliminating the need for manual array creation.
💡 Syntax
The syntax for the _.stubArray()
method is straightforward:
_.stubArray()
📝 Example
Let's dive into a simple example to illustrate the usage of the _.stubArray()
method:
const _ = require('lodash');
const emptyArray = _.stubArray();
console.log(emptyArray);
// Output: []
In this example, _.stubArray()
creates an empty array-like object, which can be used as a placeholder or default value.
🏆 Best Practices
When working with the _.stubArray()
method, consider the following best practices:
Use as Default Function Parameters:
Utilize
_.stubArray()
as default parameters in functions to handle cases where arrays are expected but not provided. This ensures consistent behavior and prevents errors.example.jsCopiedfunction processArray(inputArray = _.stubArray()) { // Perform operations on inputArray } // Call function without providing an array processArray();
Placeholder for Array Arguments:
When defining functions that expect arrays as arguments, use
_.stubArray()
to initialize placeholder arrays. This simplifies the function definition and improves code readability.example.jsCopiedfunction mergeArrays(array1 = _.stubArray(), array2 = _.stubArray()) { return array1.concat(array2); } // Call function with only one array provided const result = mergeArrays([1, 2, 3]); console.log(result); // Output: [1, 2, 3]
Enhancing Function Clarity:
By using
_.stubArray()
in function definitions, you explicitly communicate the intention of expecting arrays as parameters. This enhances code clarity and makes the function's purpose more evident.example.jsCopiedfunction processData(dataArray = _.stubArray()) { // Process dataArray } // Call function with an array const data = [ /* data to be processed */ ]; processData(data);
📚 Use Cases
Default Values for Array Parameters:
_.stubArray()
can be used to provide default values for function parameters that expect arrays. This ensures that the function behaves predictably even when arrays are not provided.example.jsCopiedfunction processInput(inputArray = _.stubArray()) { // Process inputArray } // Call function without providing an array processInput();
Placeholder Arrays:
When defining functions that expect arrays as arguments,
_.stubArray()
can serve as a placeholder for empty arrays. This simplifies function definitions and improves code readability.example.jsCopiedfunction concatenateArrays(array1 = _.stubArray(), array2 = _.stubArray()) { return array1.concat(array2); } // Call function with only one array provided const result = concatenateArrays([1, 2, 3]); console.log(result); // Output: [1, 2, 3]
Default Return Values:
In scenarios where functions return arrays,
_.stubArray()
can be used to provide default return values. This ensures that the function always returns an array, even in edge cases.example.jsCopiedfunction fetchData() { // Fetch data from API or elsewhere const data = /* fetched data */ ; return data || _.stubArray(); } const result = fetchData(); console.log(result); // Output: [] if data is not available
🎉 Conclusion
The _.stubArray()
method in Lodash offers a convenient solution for creating empty array-like objects, serving as placeholders or default values in functions. By leveraging this utility method, developers can enhance code clarity, simplify function definitions, and ensure consistent behavior in their JavaScript applications.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.stubArray()
method in your Lodash projects.
👨💻 Join our Community:
Author
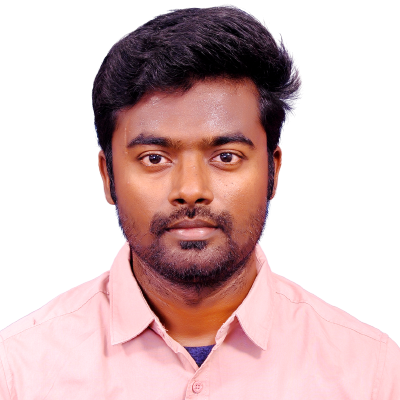
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
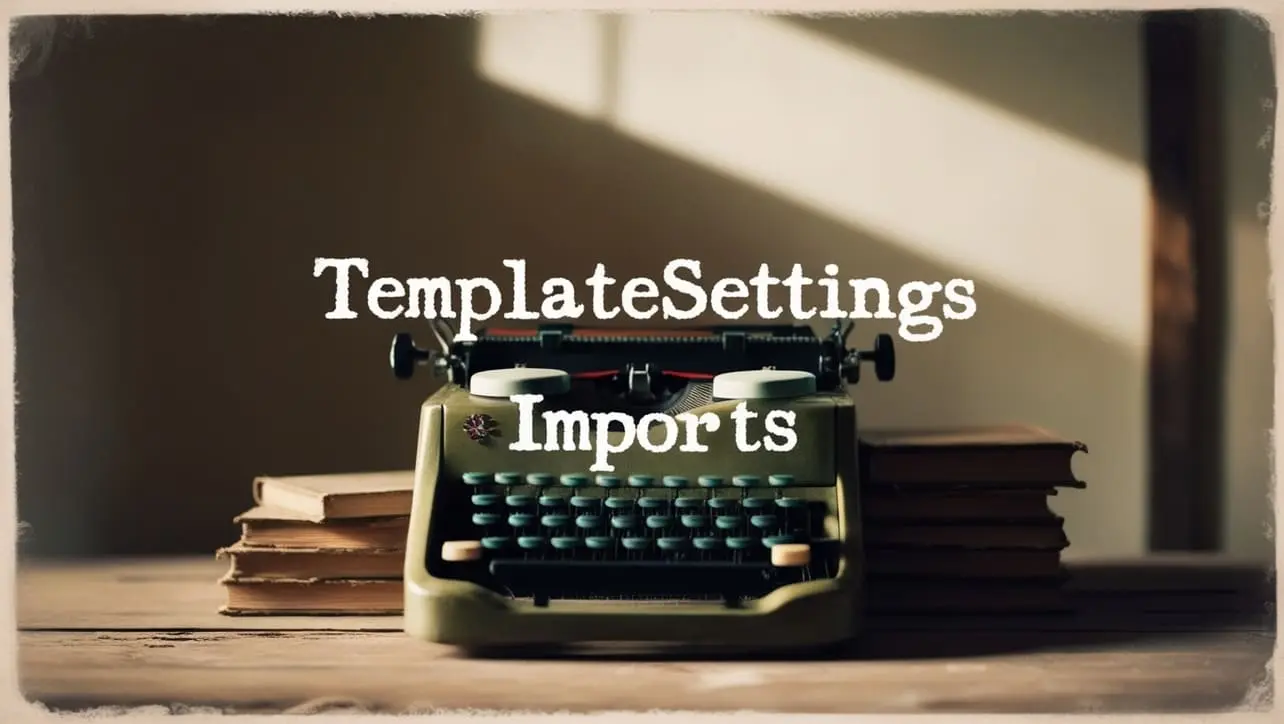
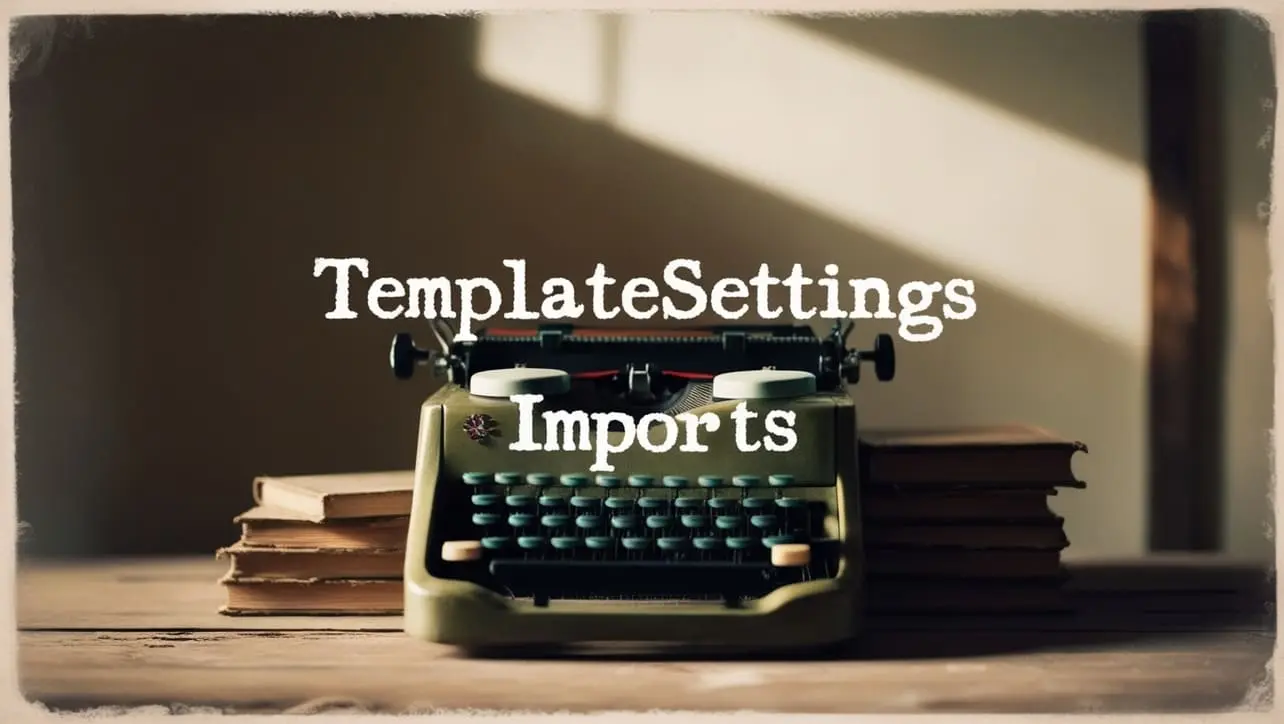
Lodash _.templateSettings.imports Property
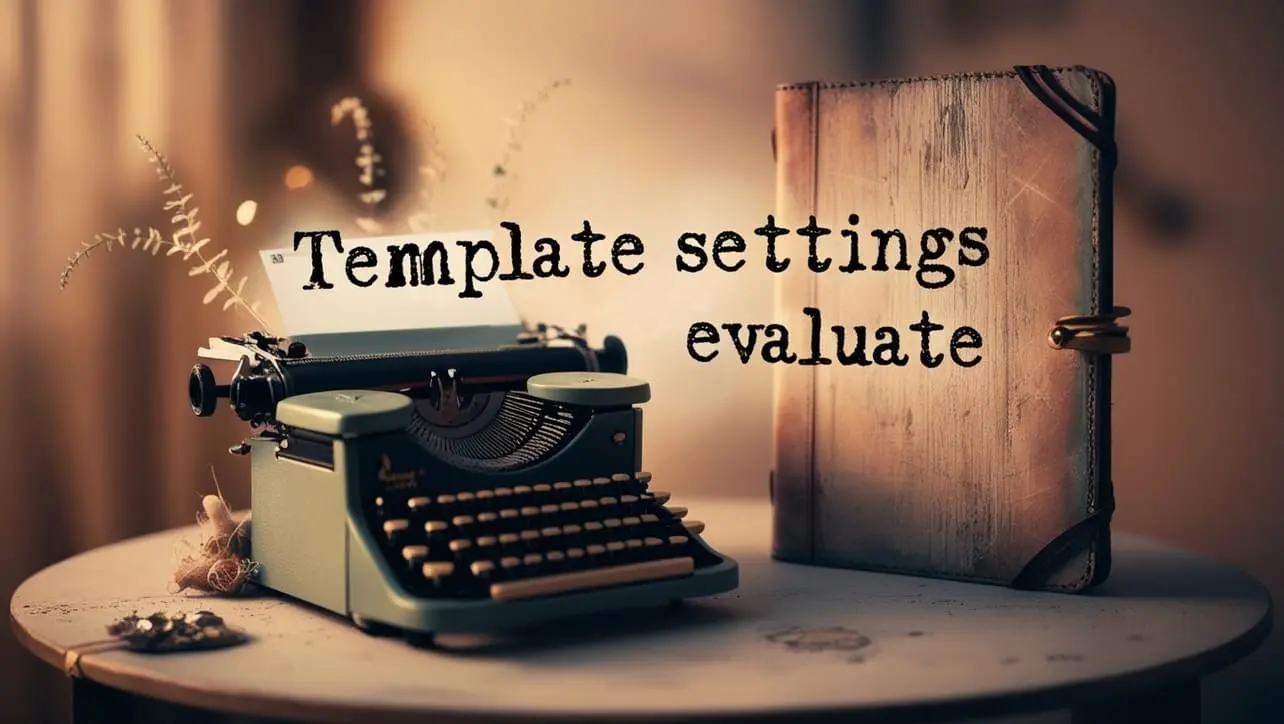
Lodash _.templateSettings.evaluate Property
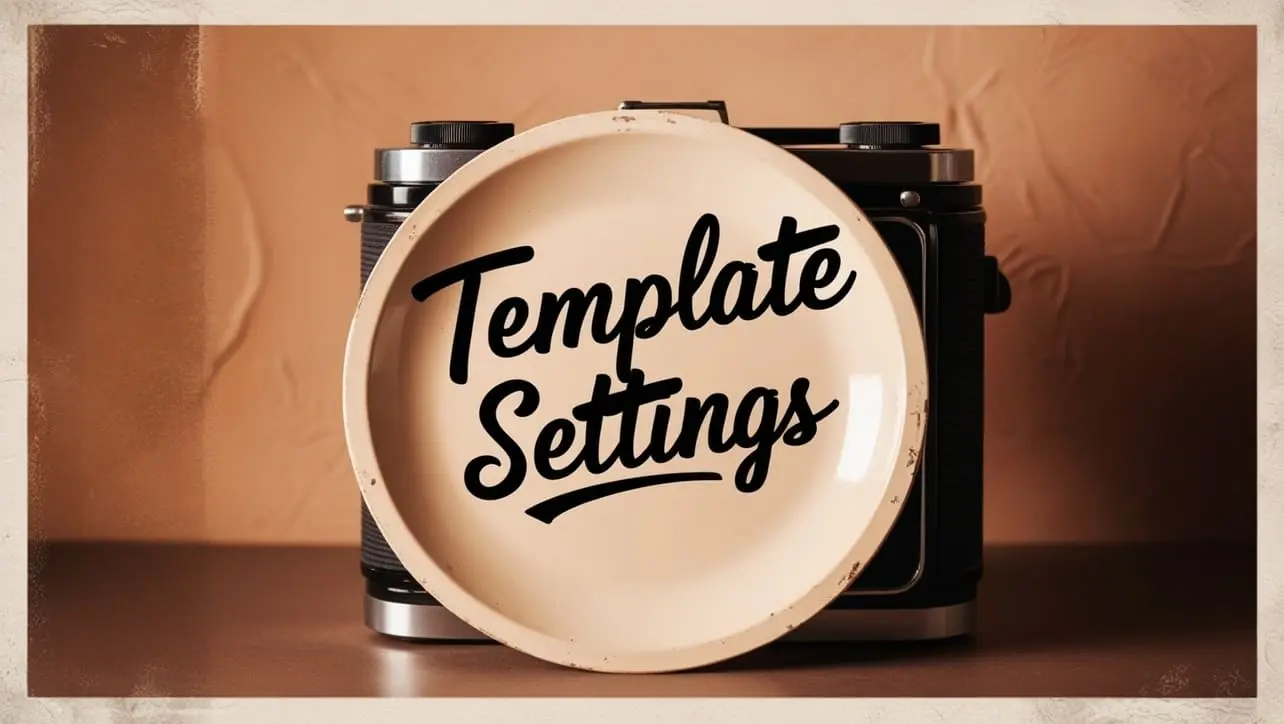
Lodash _.templateSettings Property
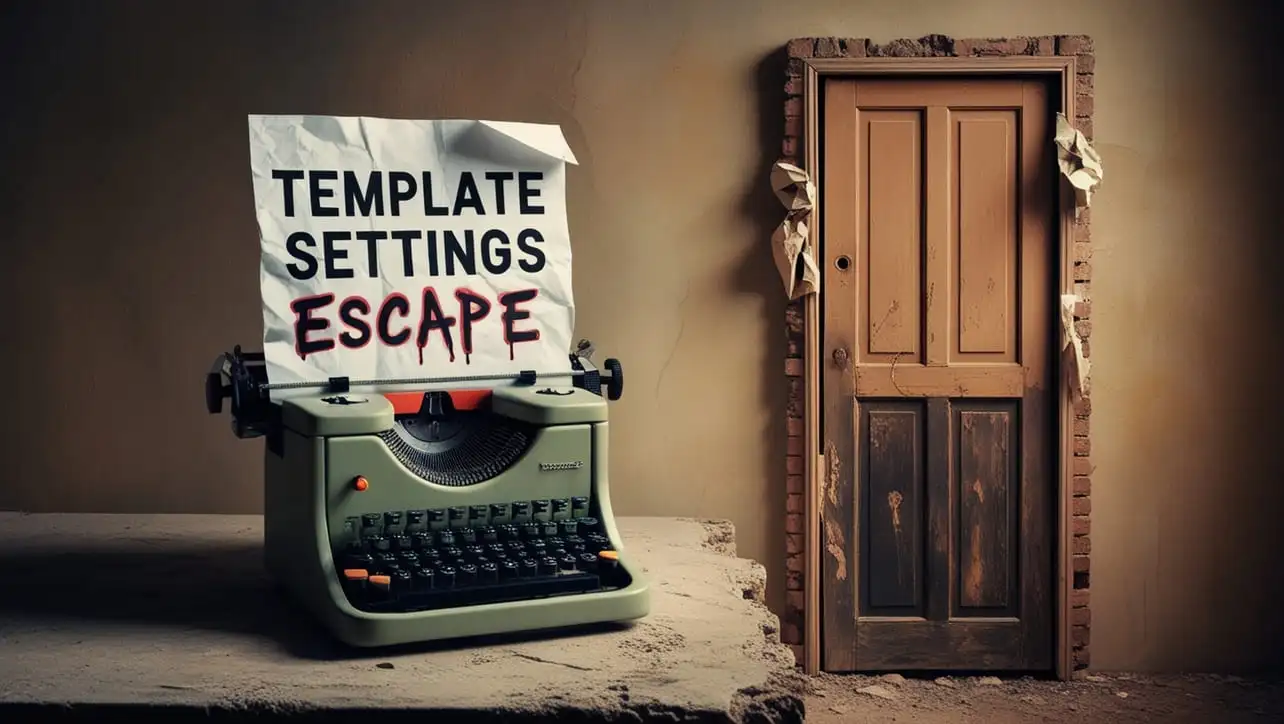
Lodash _.templateSettings.escape Property
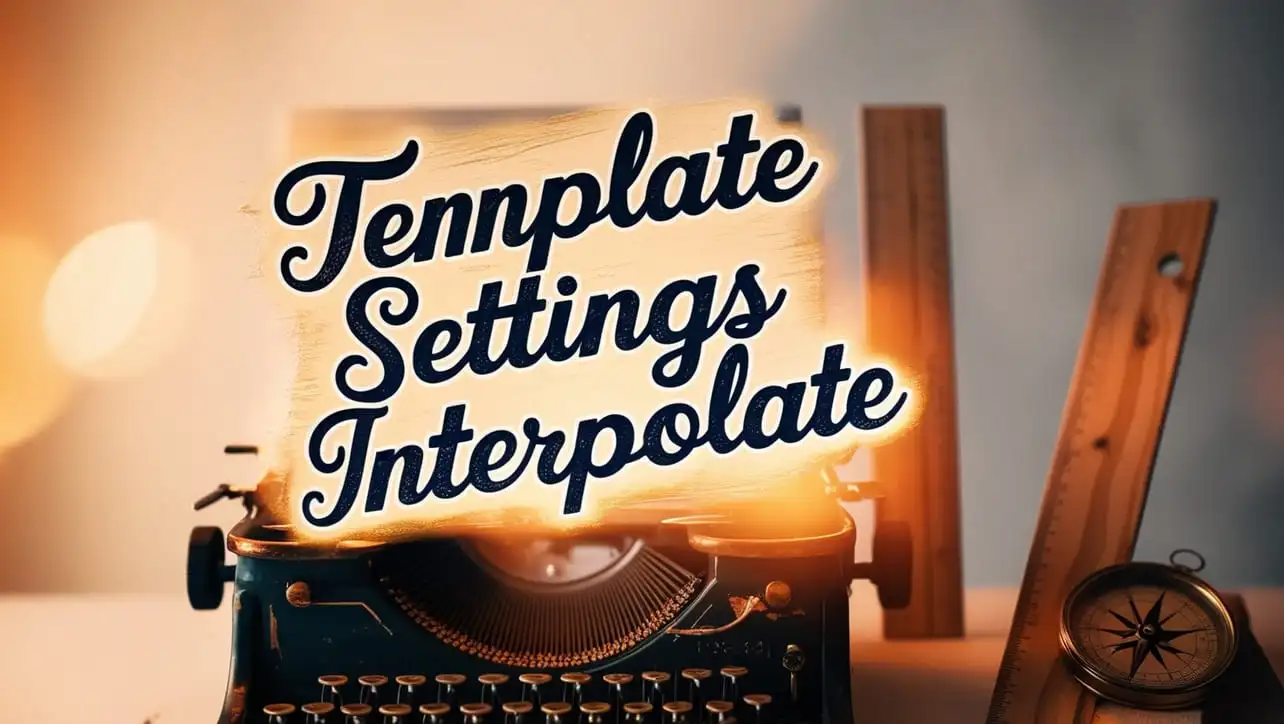
Lodash _.templateSettings.interpolate Property
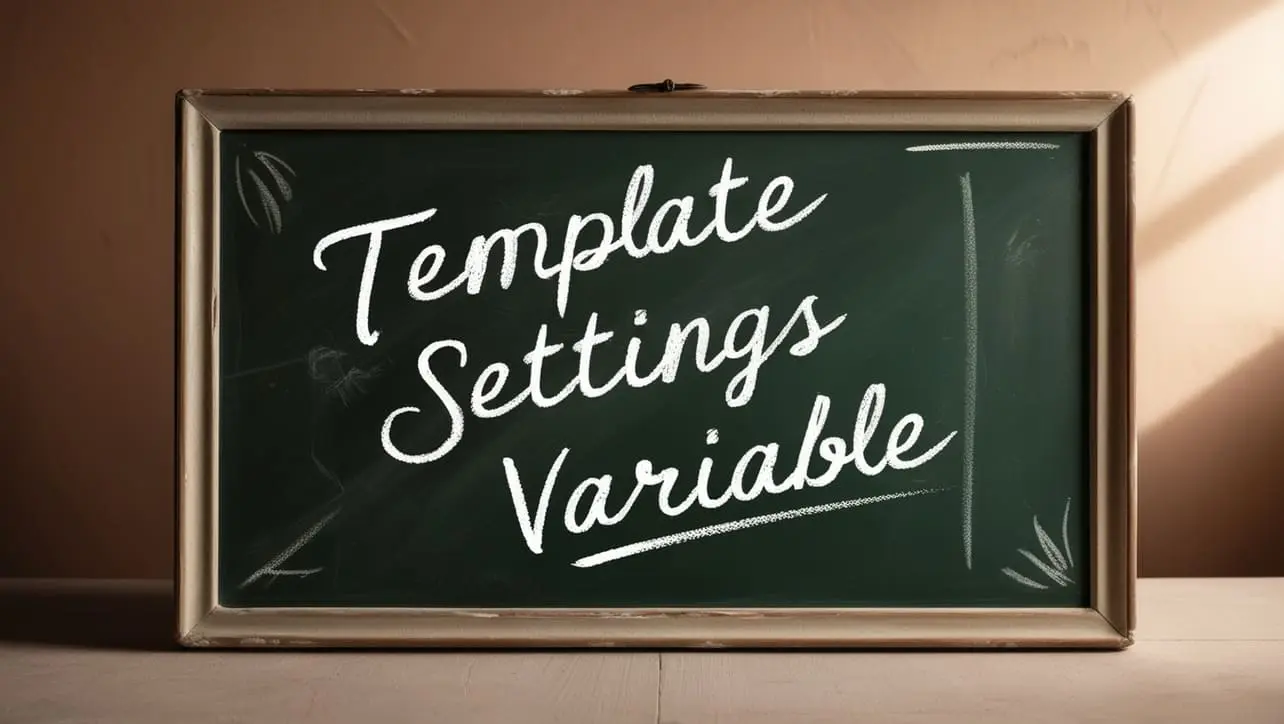
If you have any doubts regarding this article (Lodash _.stubArray() Util Method), please comment here. I will help you immediately.