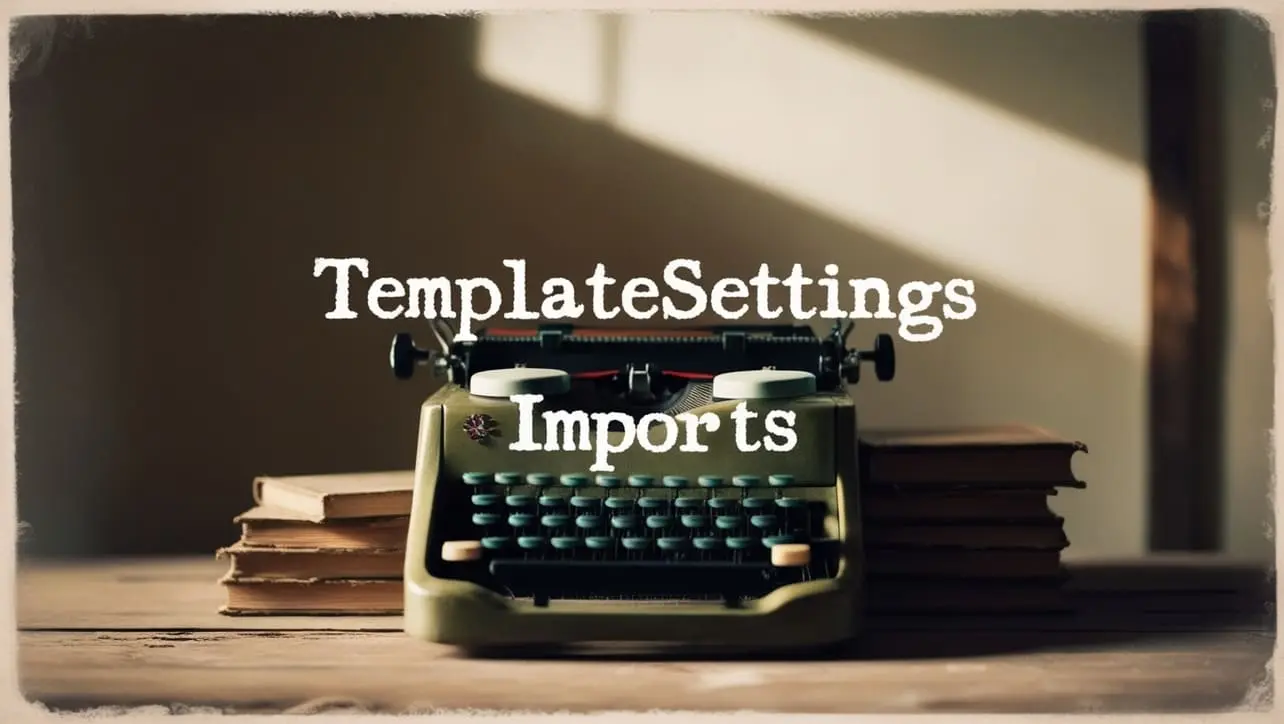
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.rangeRight() Util Method
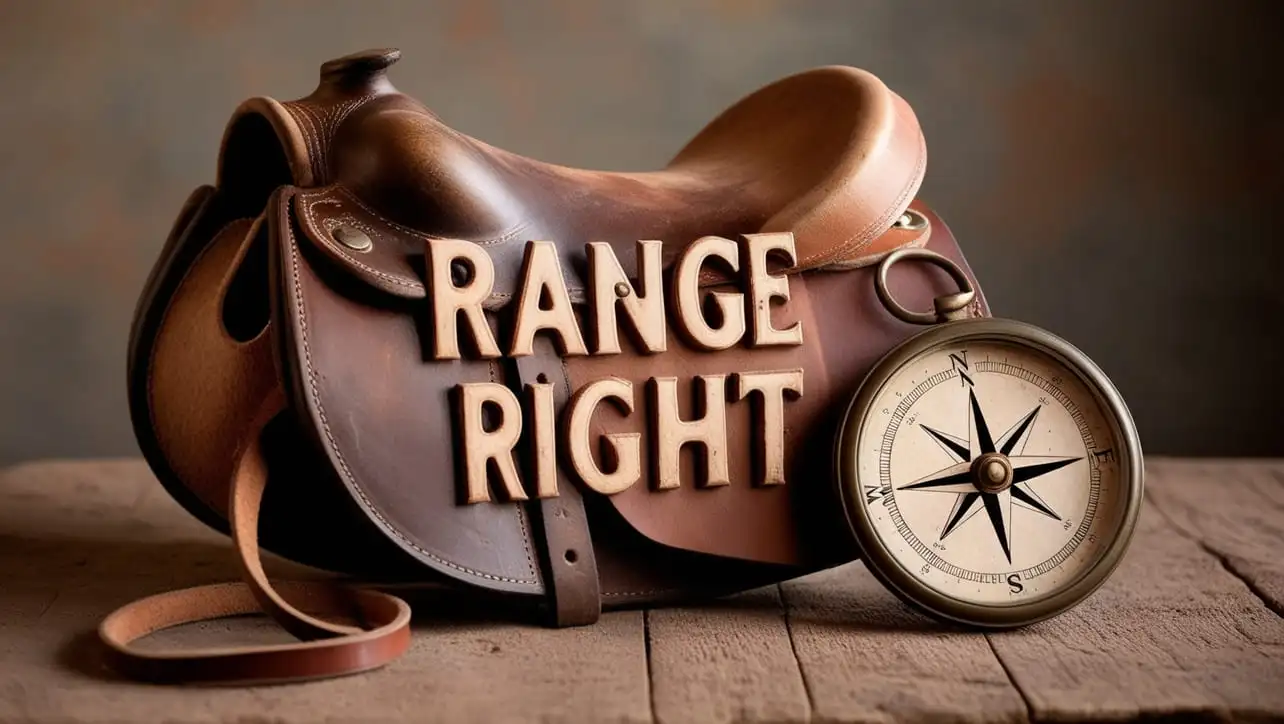
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, creating arrays with a sequence of numbers or values based on specified criteria is a common task. Lodash, a popular utility library, offers the _.rangeRight()
method, which simplifies the process of generating arrays with a sequence of values in a reverse order.
This method provides flexibility and convenience, enabling developers to create arrays tailored to their needs with ease.
🧠 Understanding _.rangeRight() Method
The _.rangeRight()
method in Lodash generates an array of numbers (or values) in a descending sequence, starting from a specified start value down to, but not including, a specified end value. It allows developers to define the step between consecutive values, providing fine-grained control over the generated sequence.
💡 Syntax
The syntax for the _.rangeRight()
method is straightforward:
_.rangeRight([start=0], end, [step=1])
- start: The start value of the sequence (default is 0).
- end: The end value of the sequence (not included).
- step: The step between consecutive values (default is 1).
📝 Example
Let's dive into a simple example to illustrate the usage of the _.rangeRight()
method:
const _ = require('lodash');
const sequence = _.rangeRight(1, 10, 2);
console.log(sequence);
// Output: [9, 7, 5, 3]
In this example, _.rangeRight()
generates a sequence starting from 9 (inclusive), down to 1 (exclusive), with a step of 2 between consecutive values.
🏆 Best Practices
When working with the _.rangeRight()
method, consider the following best practices:
Understanding Start and End Values:
Be mindful of the start and end values specified when using
_.rangeRight()
. The generated sequence includes the start value but excludes the end value, allowing for precise control over the range.example.jsCopiedconst sequence = _.rangeRight(1, 5); console.log(sequence); // Output: [4, 3, 2, 1]
Specifying Step Value:
Experiment with different step values to customize the spacing between consecutive values in the generated sequence. This enables flexibility in creating sequences tailored to specific requirements.
example.jsCopiedconst sequence = _.rangeRight(0, 10, 3); console.log(sequence); // Output: [9, 6, 3, 0]
Handling Edge Cases:
Consider edge cases, such as generating sequences with negative step values or specifying non-numeric parameters. Ensure that the input parameters are valid to avoid unexpected results.
example.jsCopiedconst sequence = _.rangeRight(10, 0, -1); console.log(sequence); // Output: [1, 2, 3, 4, 5, 6, 7, 8, 9]
📚 Use Cases
Generating Descending Sequences:
_.rangeRight()
is particularly useful for generating sequences of numbers or values in a descending order. This can be handy in various scenarios, such as creating countdown timers or iterating over arrays in reverse.example.jsCopiedconst countdownTimer = _.rangeRight(10, 0); console.log(countdownTimer); // Output: [9, 8, 7, 6, 5, 4, 3, 2, 1]
Iterating Over Arrays in Reverse:
When iterating over arrays in reverse,
_.rangeRight()
can provide the indices needed to access array elements in reverse order. This simplifies the process of iterating over arrays from the end to the beginning.example.jsCopiedconst array = ['a', 'b', 'c', 'd', 'e']; _.rangeRight(array.length).forEach(index => { console.log(array[index]); }); // Output: e, d, c, b, a
Creating Custom Sequences:
By adjusting the parameters of
_.rangeRight()
, developers can create custom sequences tailored to specific needs. Whether generating timestamps, iterating over numeric ranges, or creating unique sequences,_.rangeRight()
offers versatility in sequence generation.example.jsCopiedconst customSequence = _.rangeRight(100, 0, -10); console.log(customSequence); // Output: [10, 20, 30, 40, 50, 60, 70, 80, 90]
🎉 Conclusion
The _.rangeRight()
method in Lodash provides a convenient solution for generating arrays with sequences of numbers or values in a descending order. Whether creating countdown timers, iterating over arrays in reverse, or generating custom sequences, _.rangeRight()
offers versatility and ease of use in JavaScript development.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.rangeRight()
method in your Lodash projects.
👨💻 Join our Community:
Author
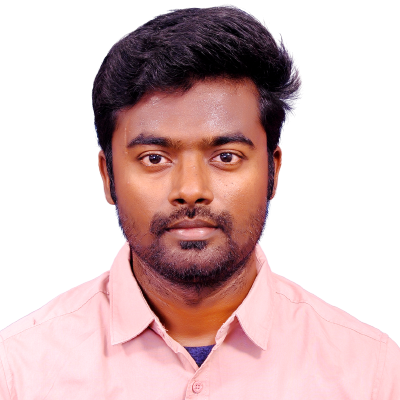
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
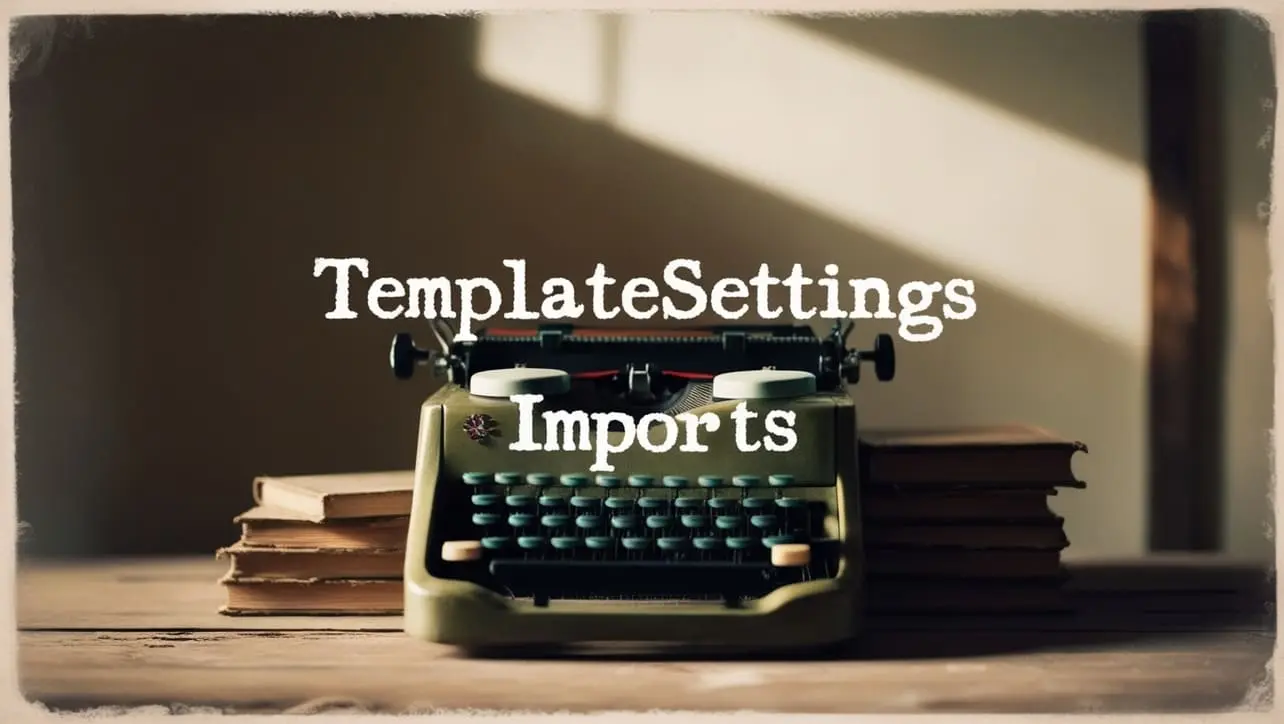
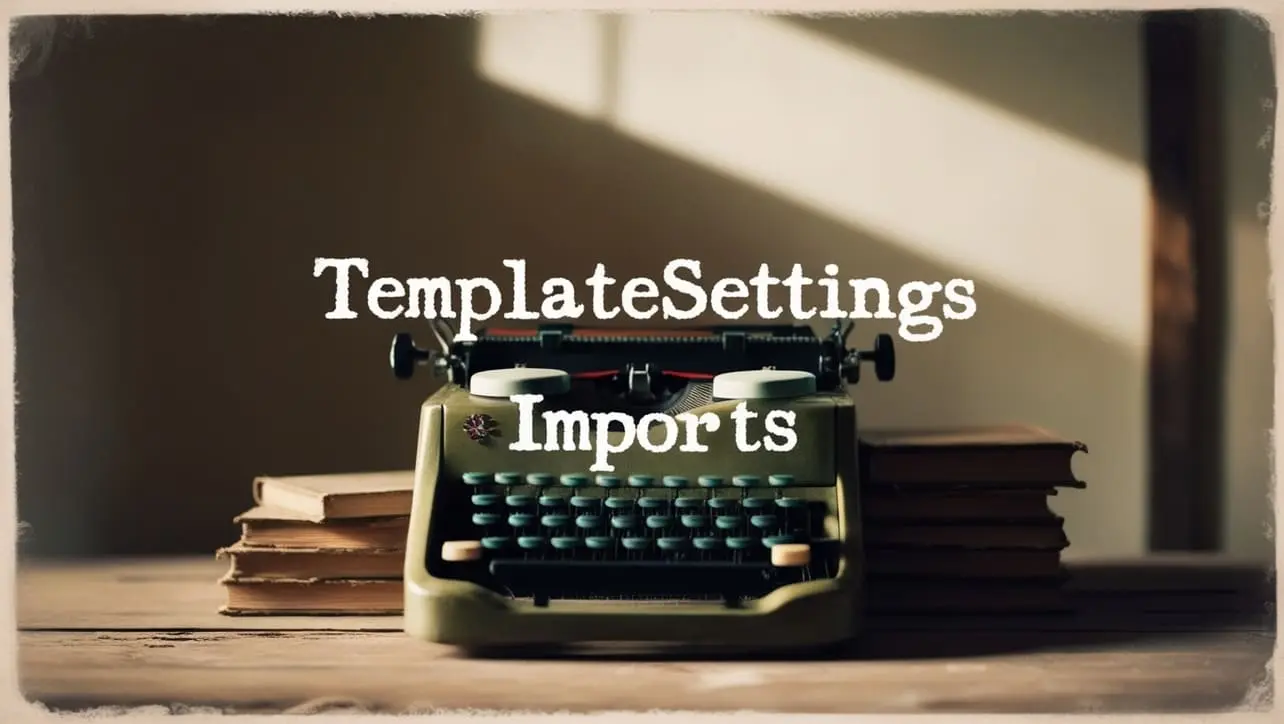
Lodash _.templateSettings.imports Property
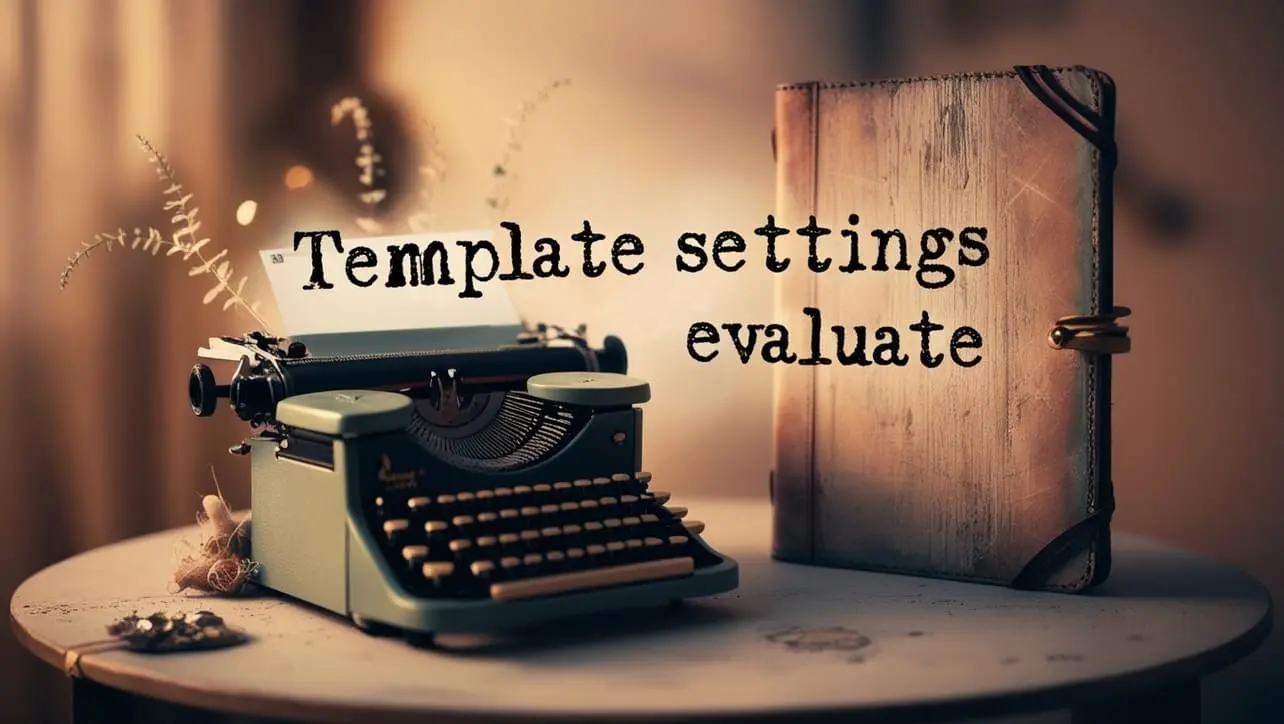
Lodash _.templateSettings.evaluate Property
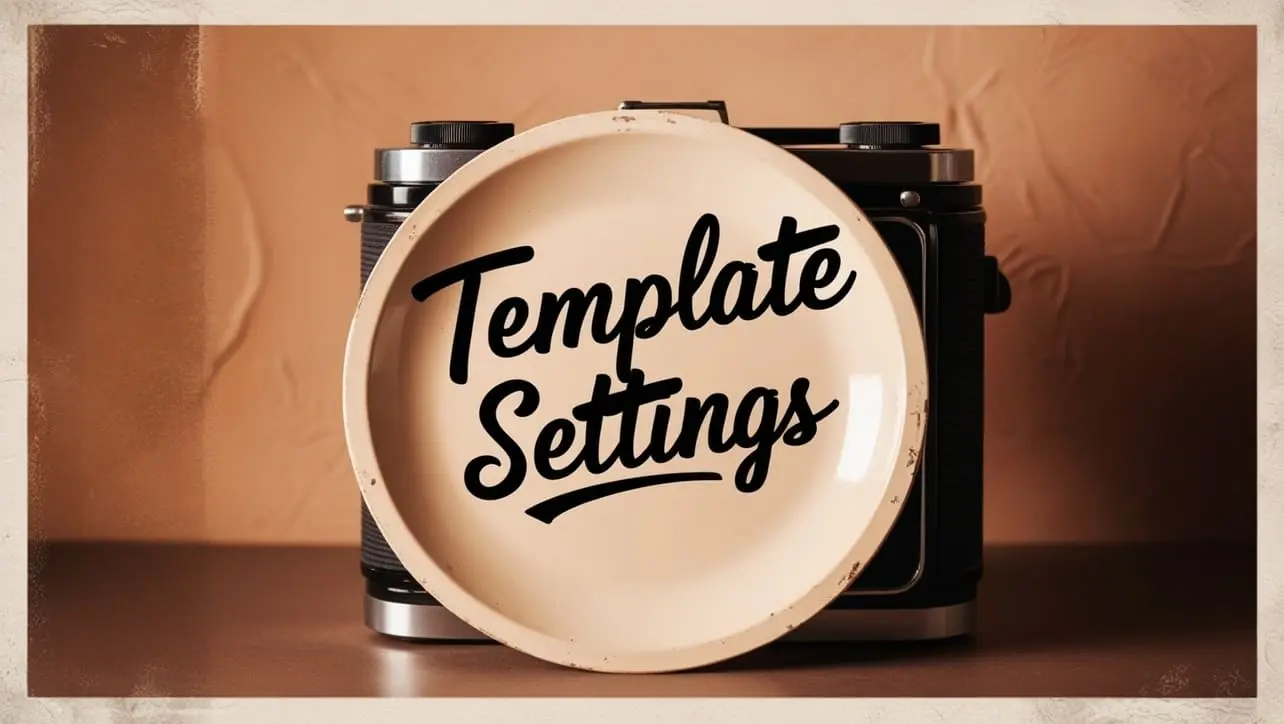
Lodash _.templateSettings Property
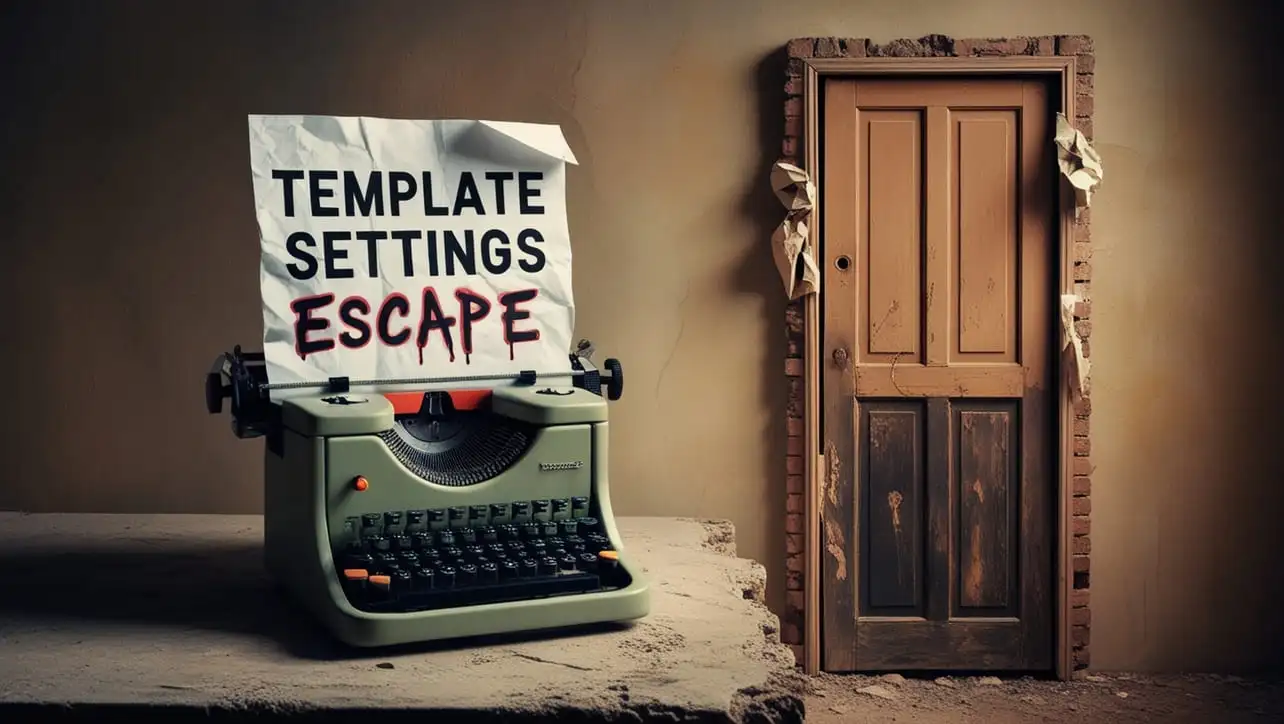
Lodash _.templateSettings.escape Property
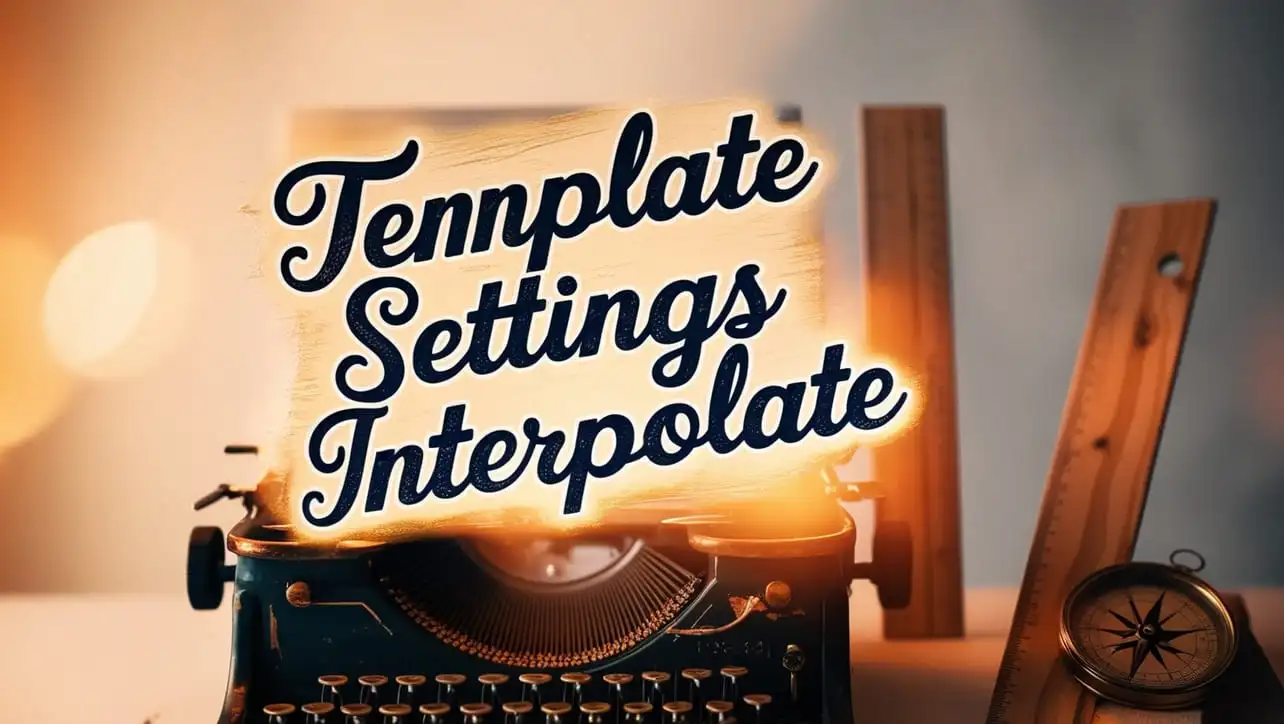
Lodash _.templateSettings.interpolate Property
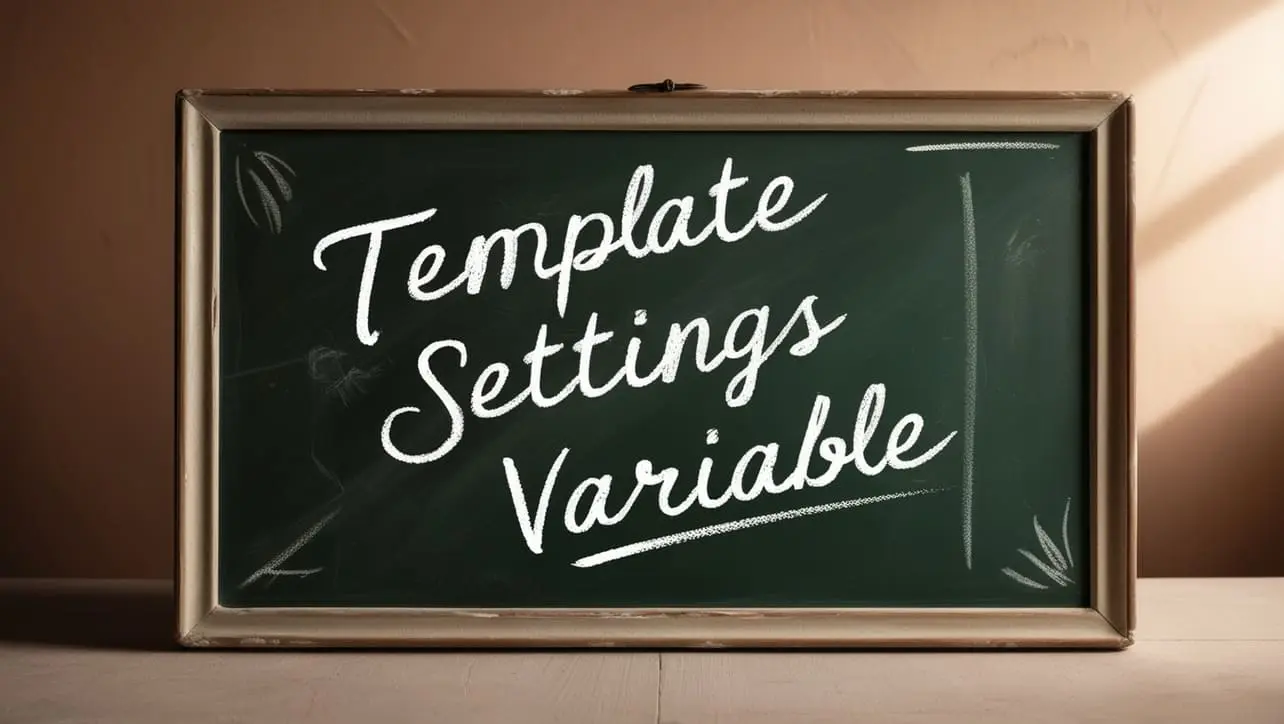
If you have any doubts regarding this article (Lodash _.rangeRight() Util Method), please comment here. I will help you immediately.