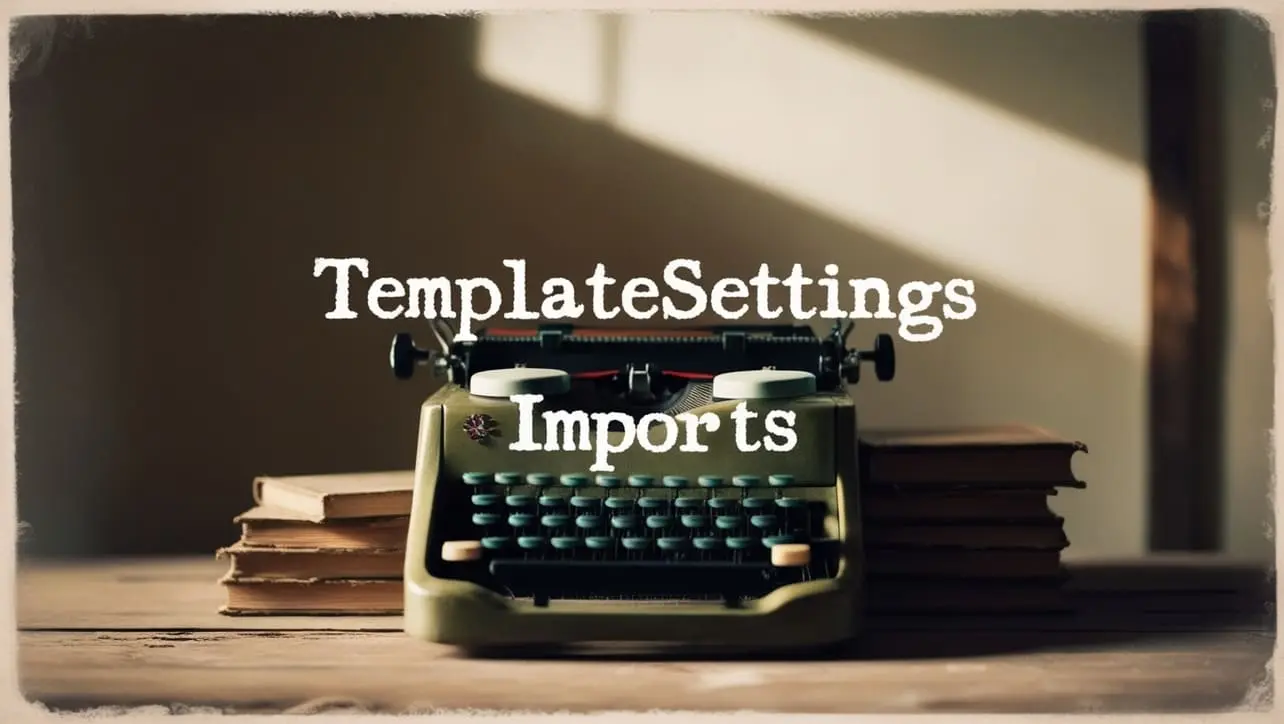
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.overSome() Util Method
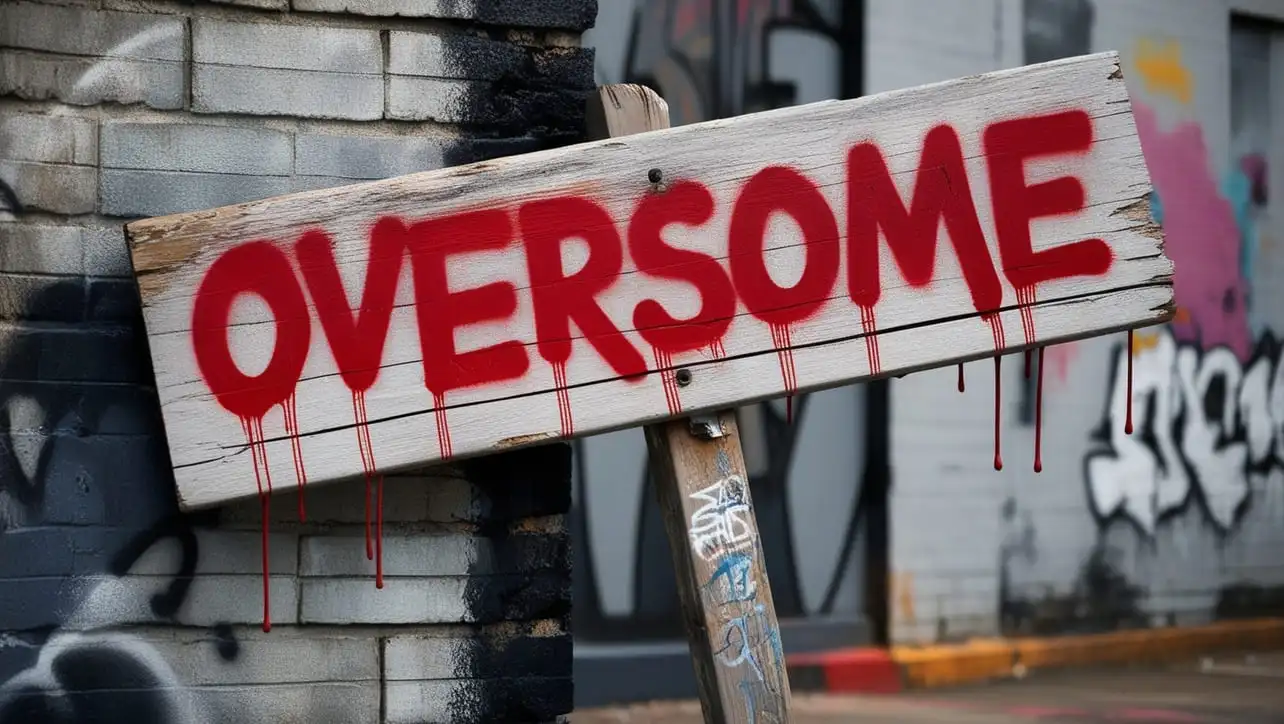
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, handling conditional logic often involves evaluating multiple conditions. Lodash, a popular utility library, provides developers with various functions to streamline such tasks. Among these functions lies the _.overSome()
method, a versatile utility designed to simplify the process of determining if at least one of multiple predicates is truthy.
This method enhances code readability and offers a concise solution to complex conditional scenarios.
🧠 Understanding _.overSome() Method
The _.overSome()
method in Lodash allows developers to create a composite predicate function that combines multiple individual predicates. It returns a new function that evaluates whether at least one of the provided predicates returns a truthy value when applied to the given arguments.
💡 Syntax
The syntax for the _.overSome()
method is straightforward:
_.overSome([predicates])
- predicates: An array of functions to be evaluated.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.overSome()
method:
const _ = require('lodash');
const isEvenOrGreaterThanTen = _.overSome(
num => num % 2 === 0,
num => num > 10
);
console.log(isEvenOrGreaterThanTen(8)); // Output: true
console.log(isEvenOrGreaterThanTen(15)); // Output: true
console.log(isEvenOrGreaterThanTen(3)); // Output: false
In this example, the isEvenOrGreaterThanTen function is created using _.overSome()
to check if a number is either even or greater than ten.
🏆 Best Practices
When working with the _.overSome()
method, consider the following best practices:
Understanding Composite Predicates:
Understand that
_.overSome()
creates a composite predicate function that evaluates multiple predicates and returns true if at least one of them is truthy.example.jsCopiedconst isEvenOrGreaterThanTen = _.overSome( num => num % 2 === 0, num => num > 10 ); console.log(isEvenOrGreaterThanTen(8)); // Output: true console.log(isEvenOrGreaterThanTen(15)); // Output: true console.log(isEvenOrGreaterThanTen(3)); // Output: false
Handling Complex Conditions:
Use
_.overSome()
to handle complex conditional scenarios where multiple conditions need to be evaluated simultaneously.example.jsCopiedconst isValidInput = _.overSome( input => typeof input === 'string' && input.length > 0, input => Array.isArray(input) && input.length > 0, input => input !== null && typeof input === 'object' && Object.keys(input).length > 0 ); console.log(isValidInput('hello')); // Output: true console.log(isValidInput([1, 2, 3])); // Output: true console.log(isValidInput({ a: 1, b: 2 })); // Output: true
Enhancing Readability:
Utilize
_.overSome()
to improve code readability by consolidating multiple conditional checks into a single composite predicate function.example.jsCopiedconst isAuthorized = _.overSome( user => user.role === 'admin', user => user.role === 'editor' ); if(isAuthorized(currentUser)) { // Perform authorized actions } else { // Handle unauthorized access }
📚 Use Cases
Access Control:
_.overSome()
can be used to implement access control logic, where authorization is granted if at least one of the specified conditions is met.example.jsCopiedconst isAuthorized = _.overSome( user => user.role === 'admin', user => user.role === 'editor' ); if(isAuthorized(currentUser)) { // Perform authorized actions } else { // Handle unauthorized access }
Data Filtering:
In scenarios where data filtering based on multiple conditions is required,
_.overSome()
offers a concise solution to determine if any of the conditions are satisfied.example.jsCopiedconst data = /* ...fetch data from API or elsewhere... */ ; const filterConditions = [ item => item.status === 'active', item => item.quantity > 0 ]; const filteredData = data.filter(_.overSome(filterConditions)); console.log(filteredData);
Validation:
Use
_.overSome()
for input validation, where validation is considered successful if at least one of the validation rules is met.example.jsCopiedconst isValidInput = _.overSome( input => typeof input === 'string' && input.length > 0, input => Array.isArray(input) && input.length > 0, input => input !== null && typeof input === 'object' && Object.keys(input).length > 0 ); if(isValidInput(userInput)) { // Process valid input } else { // Handle invalid input }
🎉 Conclusion
The _.overSome()
method in Lodash provides a powerful solution for evaluating multiple predicates and determining if at least one of them is truthy. Whether you're implementing access control, data filtering, or input validation, this utility method offers a concise and efficient approach to handling complex conditional scenarios in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.overSome()
method in your Lodash projects.
👨💻 Join our Community:
Author
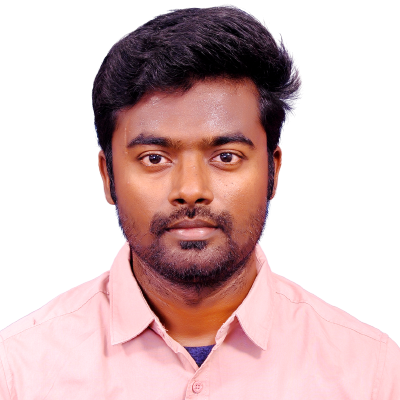
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
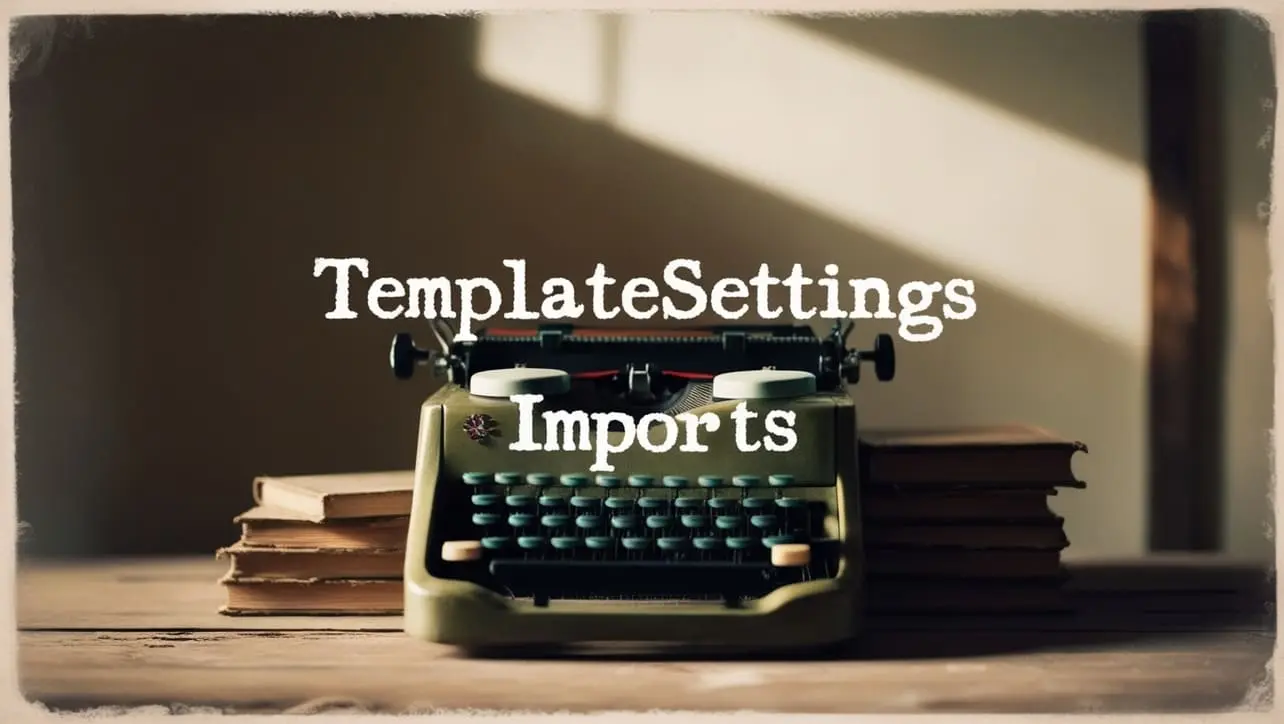
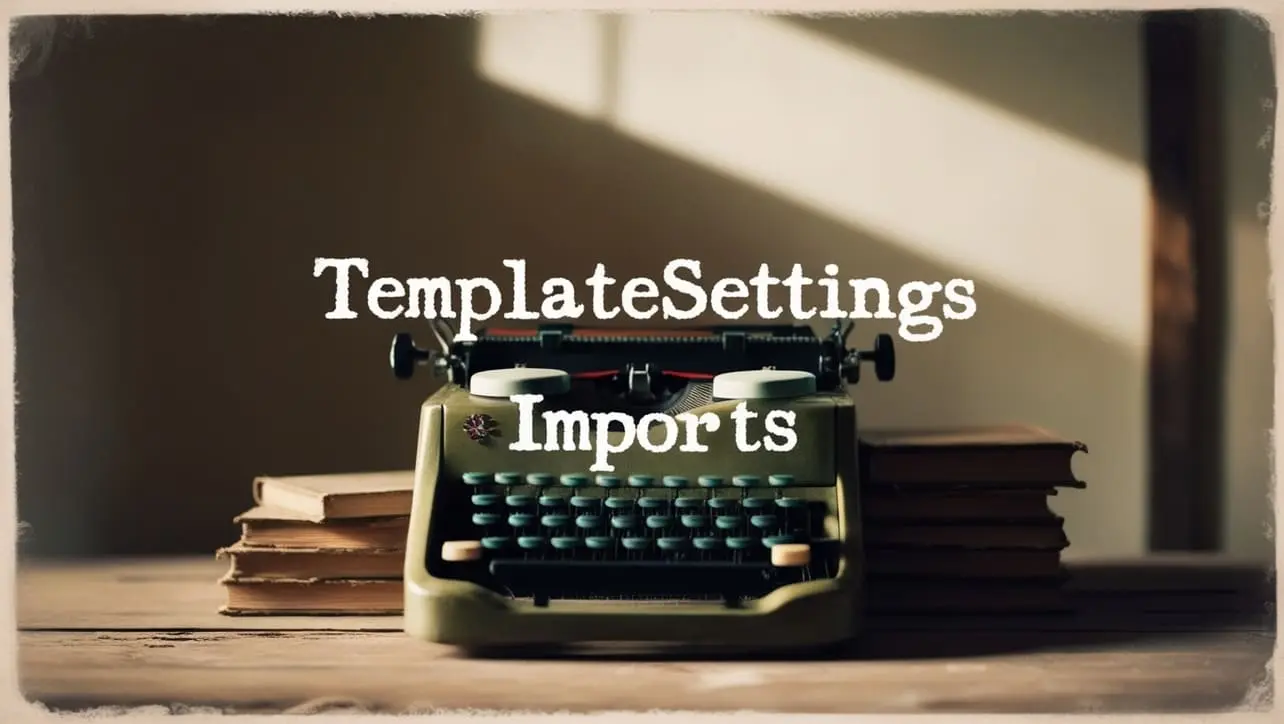
Lodash _.templateSettings.imports Property
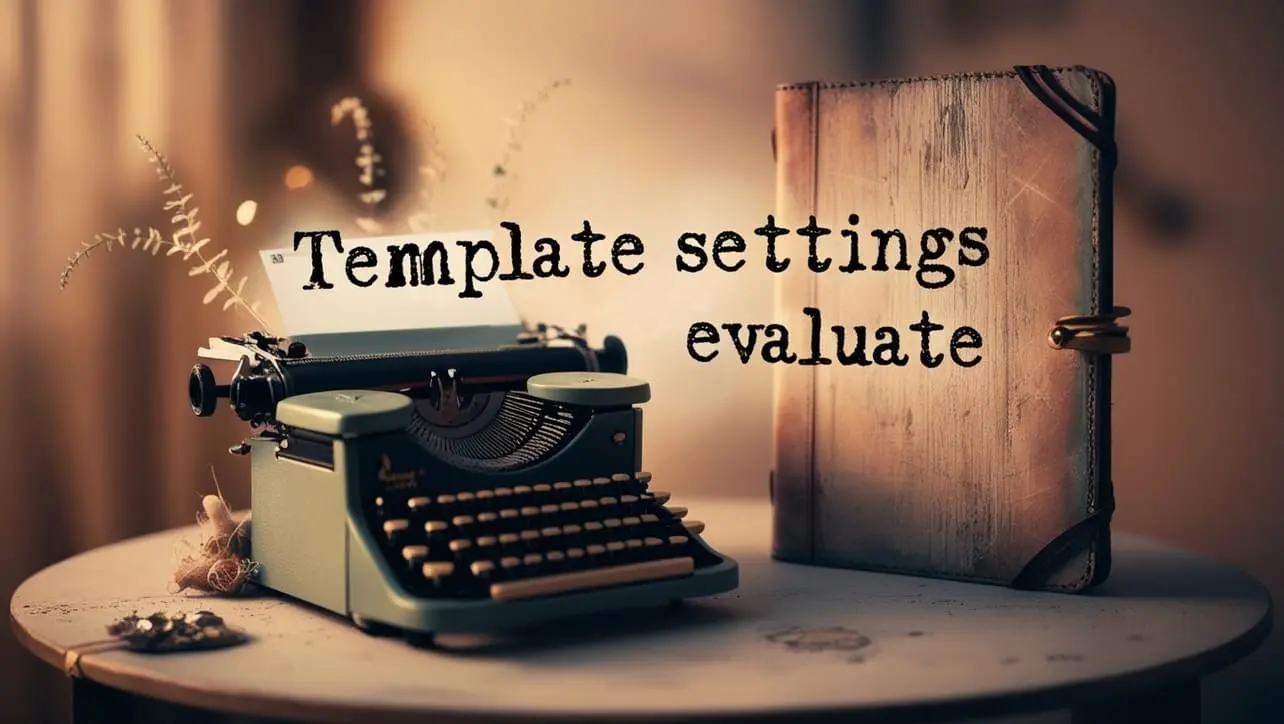
Lodash _.templateSettings.evaluate Property
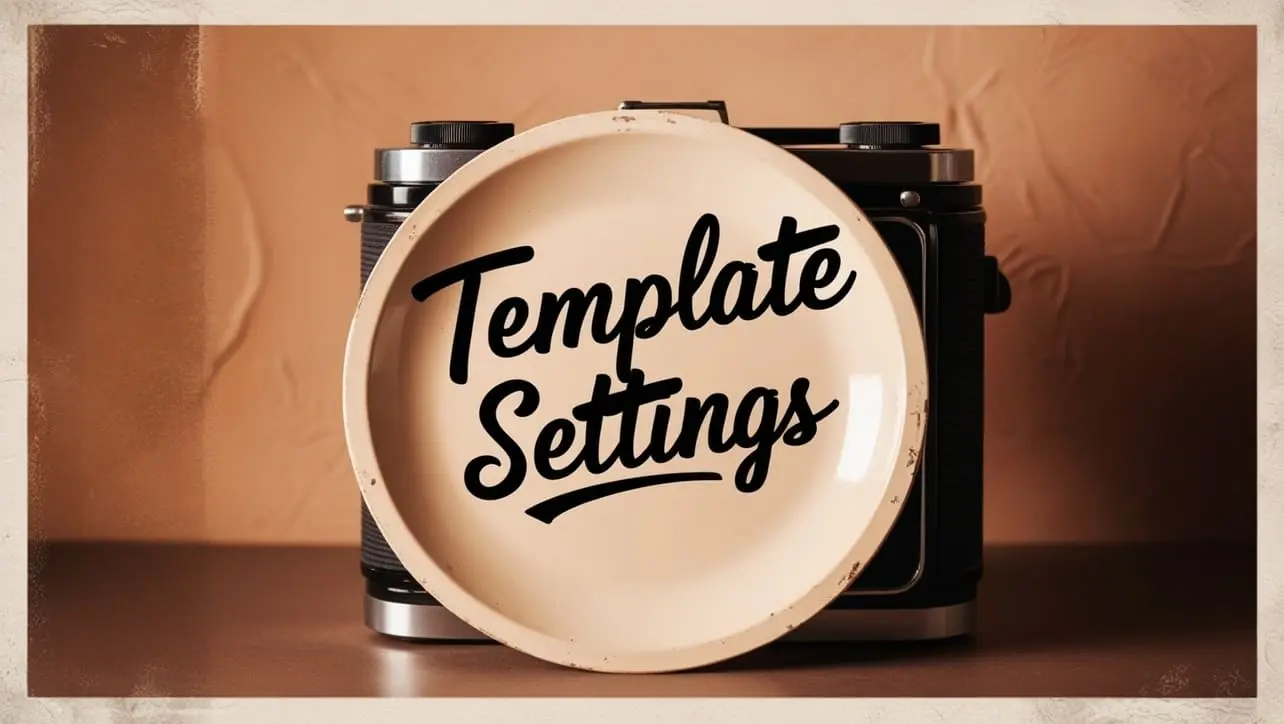
Lodash _.templateSettings Property
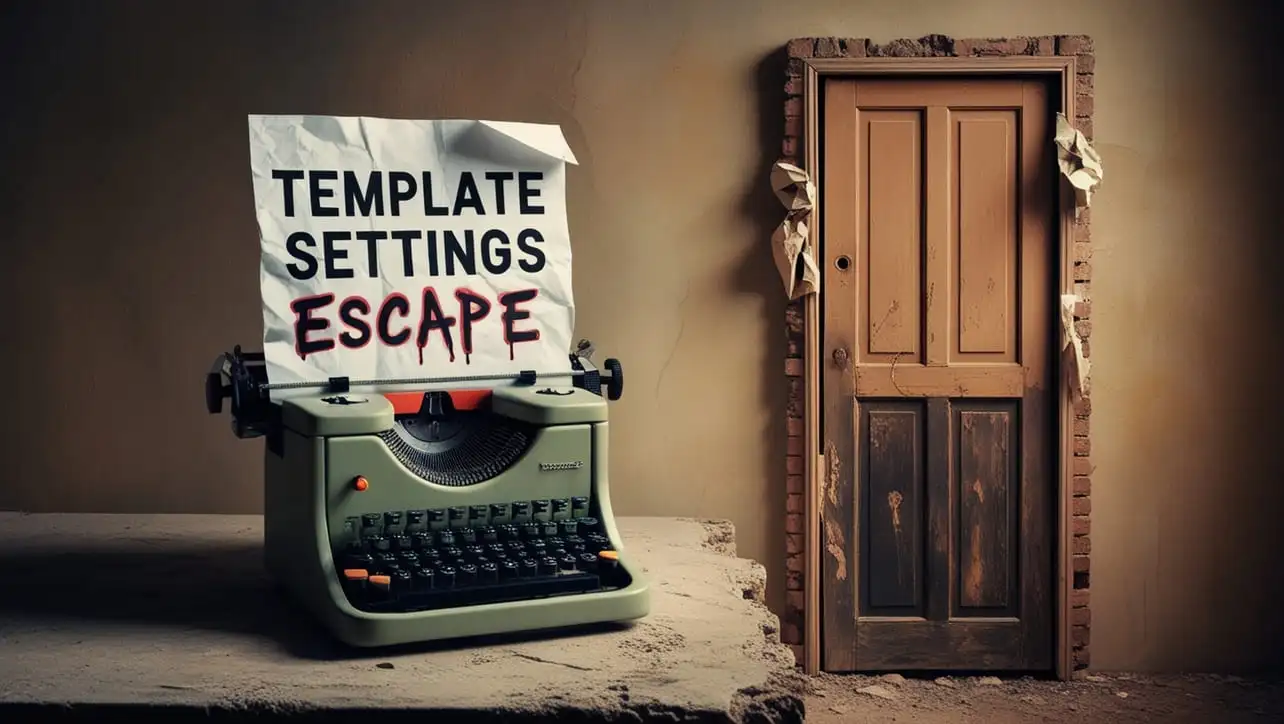
Lodash _.templateSettings.escape Property
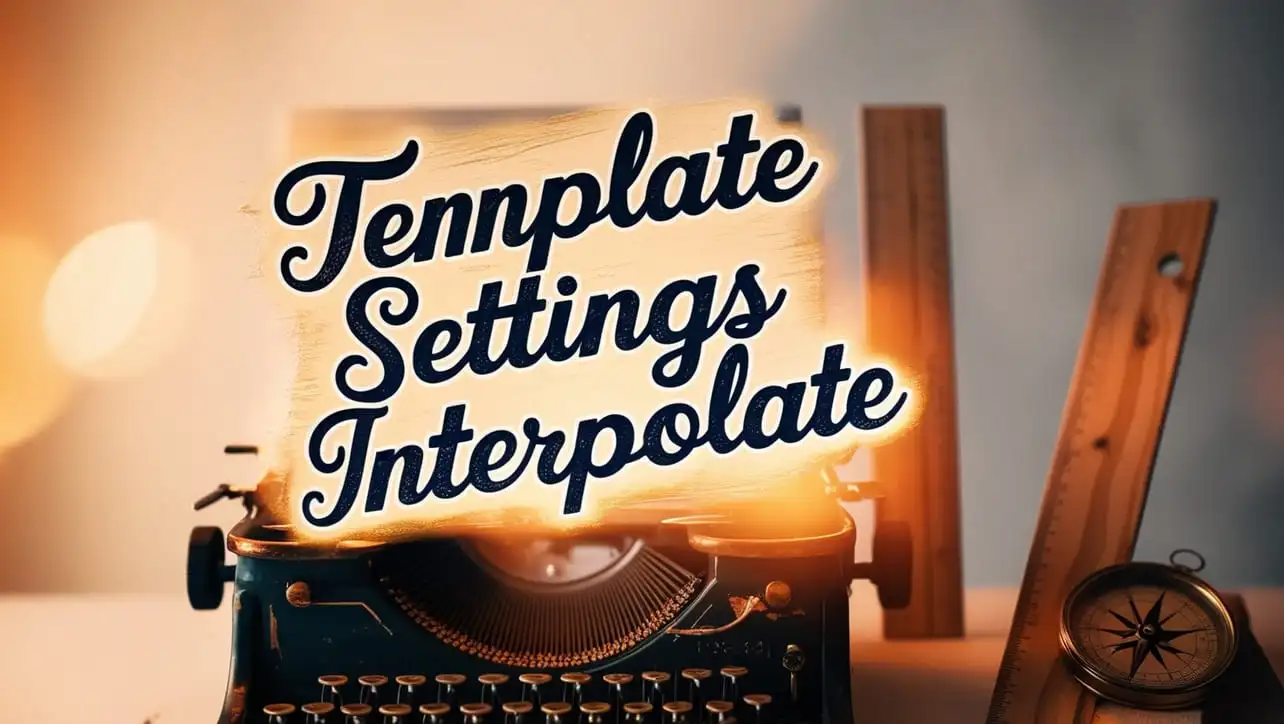
Lodash _.templateSettings.interpolate Property
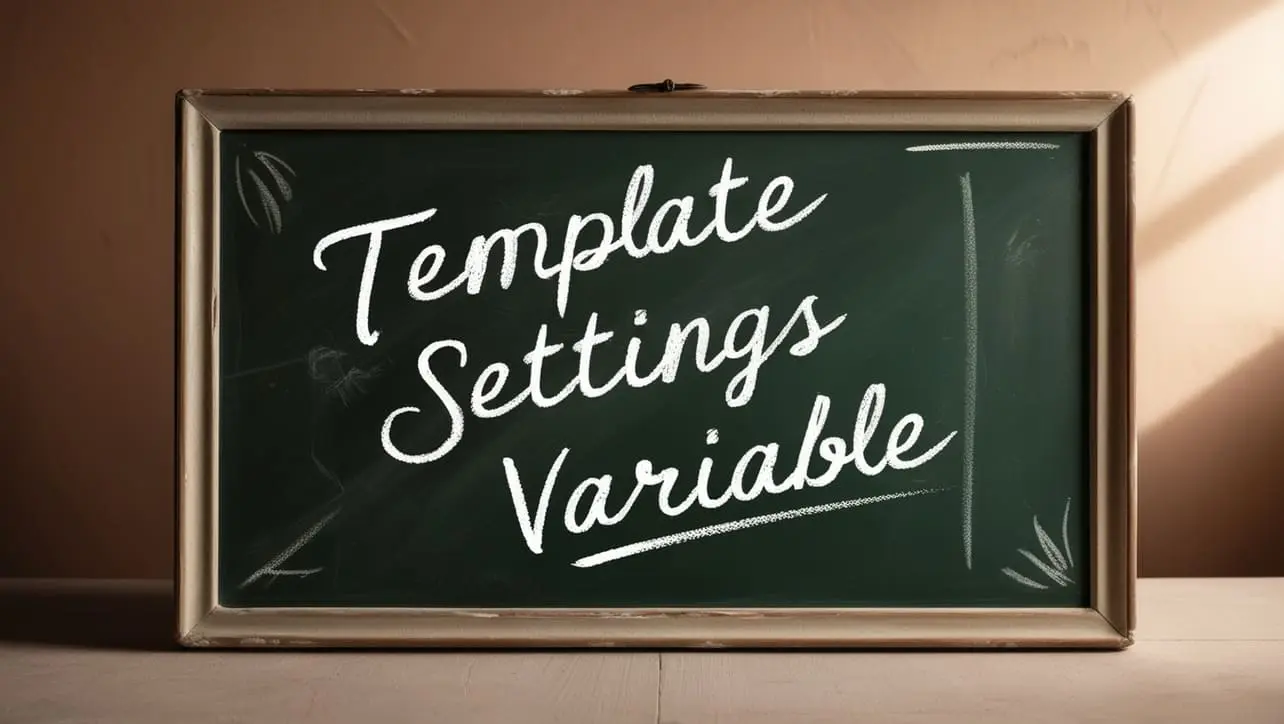
If you have any doubts regarding this article (Lodash _.overSome() Util Method), please comment here. I will help you immediately.