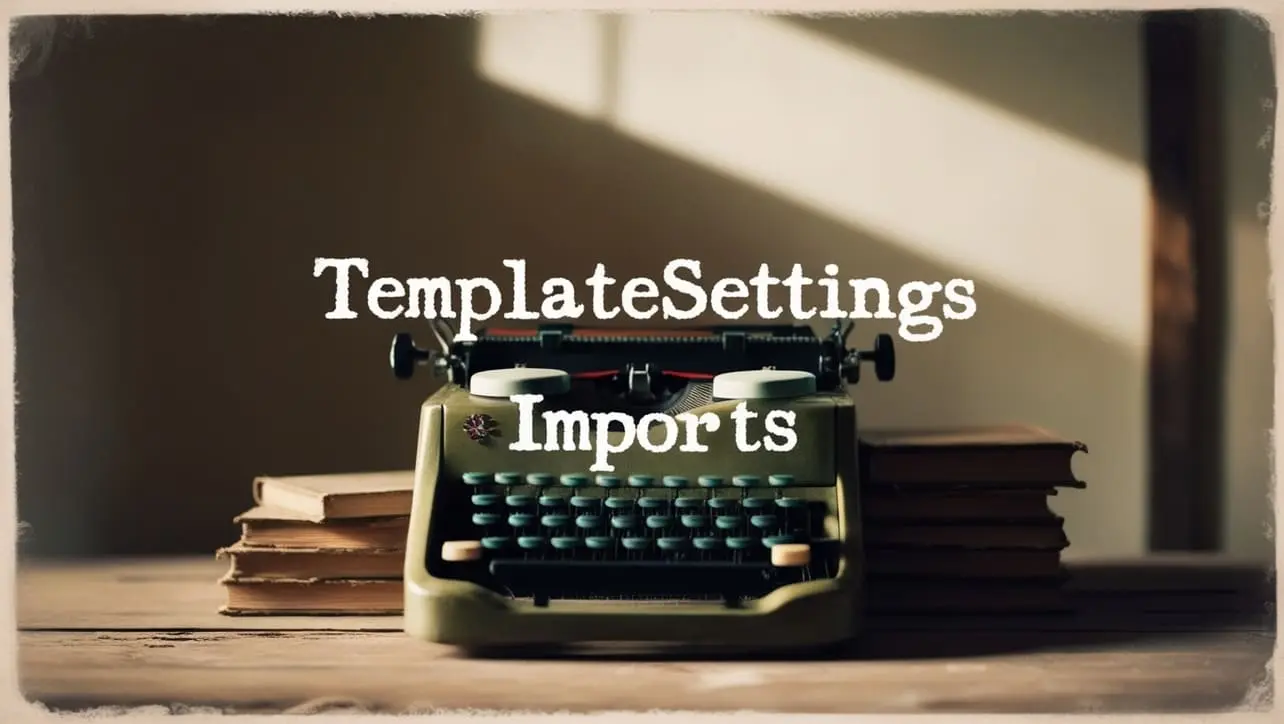
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.noop() Util Method
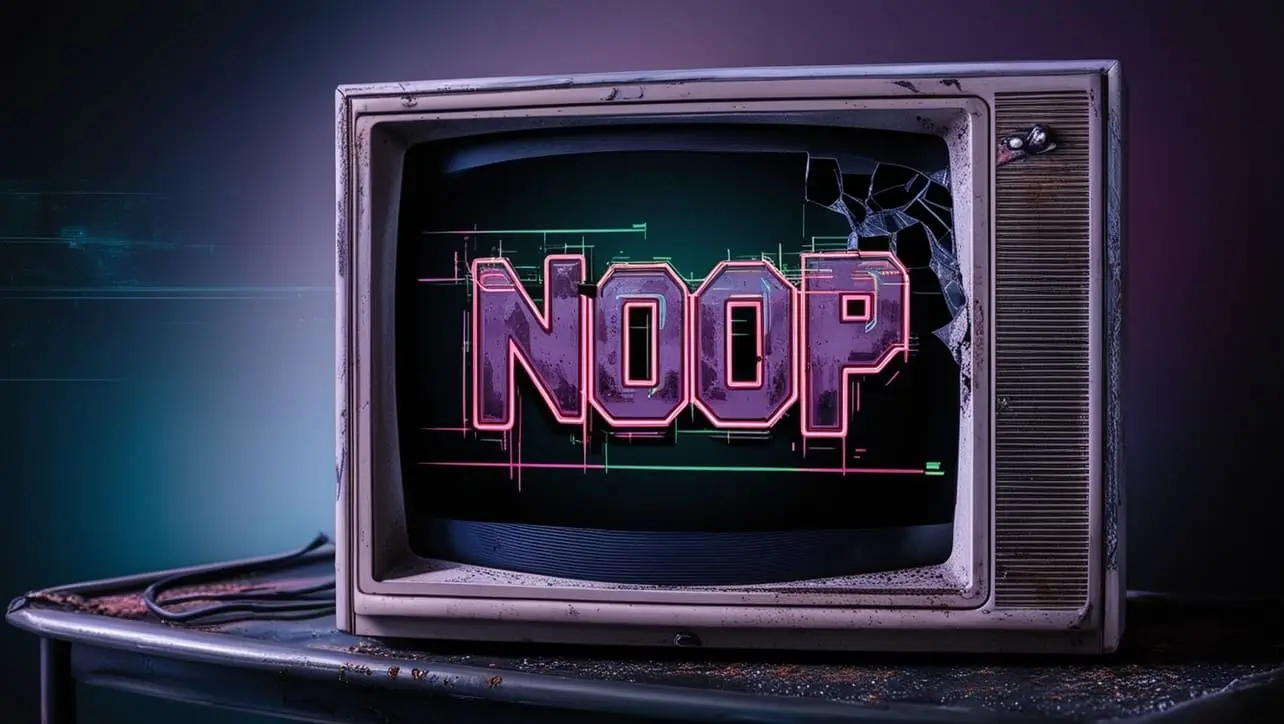
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript development, simplicity and efficiency are key. Lodash, a popular utility library, offers a myriad of functions to streamline common programming tasks. Among these functions is _.noop()
, a utility method designed to simplify function creation by returning a no-operation function.
While seemingly trivial, _.noop()
plays a crucial role in various programming scenarios, enhancing code readability and maintainability.
🧠 Understanding _.noop() Method
The _.noop()
method in Lodash returns a function that does nothing. It acts as a placeholder or stub, allowing developers to create functions with predefined behavior without explicitly defining them. This can be particularly useful in situations where a function is required as an argument but its implementation is unnecessary.
💡 Syntax
The syntax for the _.noop()
method is straightforward:
_.noop()
📝 Example
Let's dive into a simple example to illustrate the usage of the _.noop()
method:
const _ = require('lodash');
// Define a function that takes a callback and invokes it
function process(callback) {
// Perform some processing
console.log('Processing...');
// Invoke the callback (if provided)
callback();
}
// Call the process function with _.noop() as a callback
process(_.noop());
In this example, _.noop()
is passed as a callback to the process function. As _.noop()
returns a no-operation function, the callback is essentially a placeholder that does nothing.
🏆 Best Practices
When working with the _.noop()
method, consider the following best practices:
Placeholder Functions:
Use
_.noop()
as a placeholder when defining functions that require callbacks but don't necessarily need them to perform any operation.example.jsCopiedfunction fetchDataFromAPI(callback = _.noop()) { // Fetch data from API // Invoke callback with data (if provided) callback(data); }
Callback Default Values:
Leverage
_.noop()
to provide default values for callback parameters, simplifying function definitions and reducing boilerplate code.example.jsCopiedfunction process(callback = _.noop()) { // Perform processing // Invoke callback callback(); }
Conditional Execution:
Use
_.noop()
in conditional statements to provide a default action when a condition is not met.example.jsCopiedfunction performAction(action = _.noop()) { if(condition) { action(); } else { // Perform default action } }
📚 Use Cases
Optional Callbacks:
_.noop()
can be used to define optional callbacks in functions where callback execution is not mandatory.example.jsCopiedfunction fetchDataFromServer(callback = _.noop()) { // Fetch data from server // Invoke callback with data (if provided) callback(data); }
Event Handlers:
In event-driven programming,
_.noop()
can serve as a placeholder for event handlers that are not yet defined.example.jsCopiedelement.addEventListener('click', _.noop());
Default Actions:
When defining functions with conditional behavior,
_.noop()
can provide a default action in case no specific action is required.example.jsCopiedfunction performTask(task = _.noop()) { if(condition) { // Perform specific task } else { task(); // Perform default task (if no specific task is provided) } }
🎉 Conclusion
The _.noop()
method in Lodash offers a simple yet powerful solution for defining placeholder functions. Whether you're creating optional callbacks, event handlers, or defining default actions, _.noop()
simplifies function creation and enhances code readability.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.noop()
method in your Lodash projects.
👨💻 Join our Community:
Author
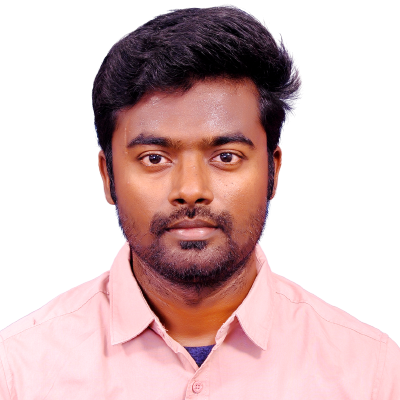
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
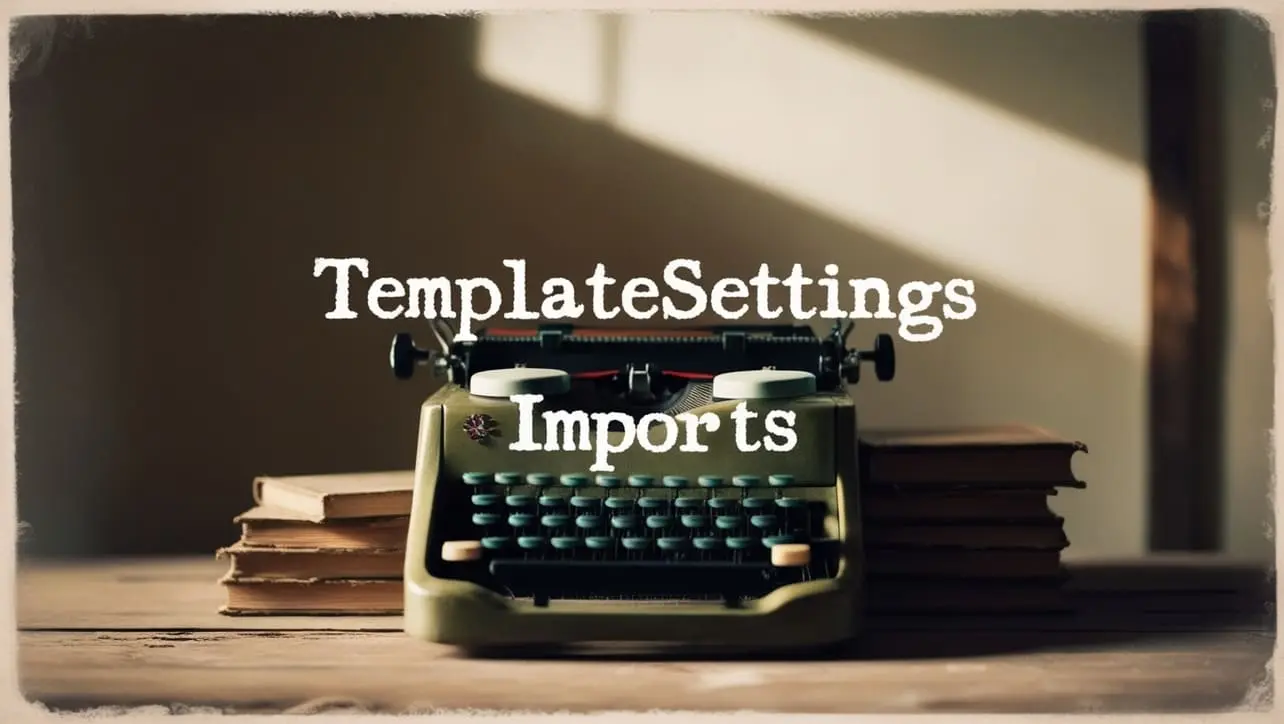
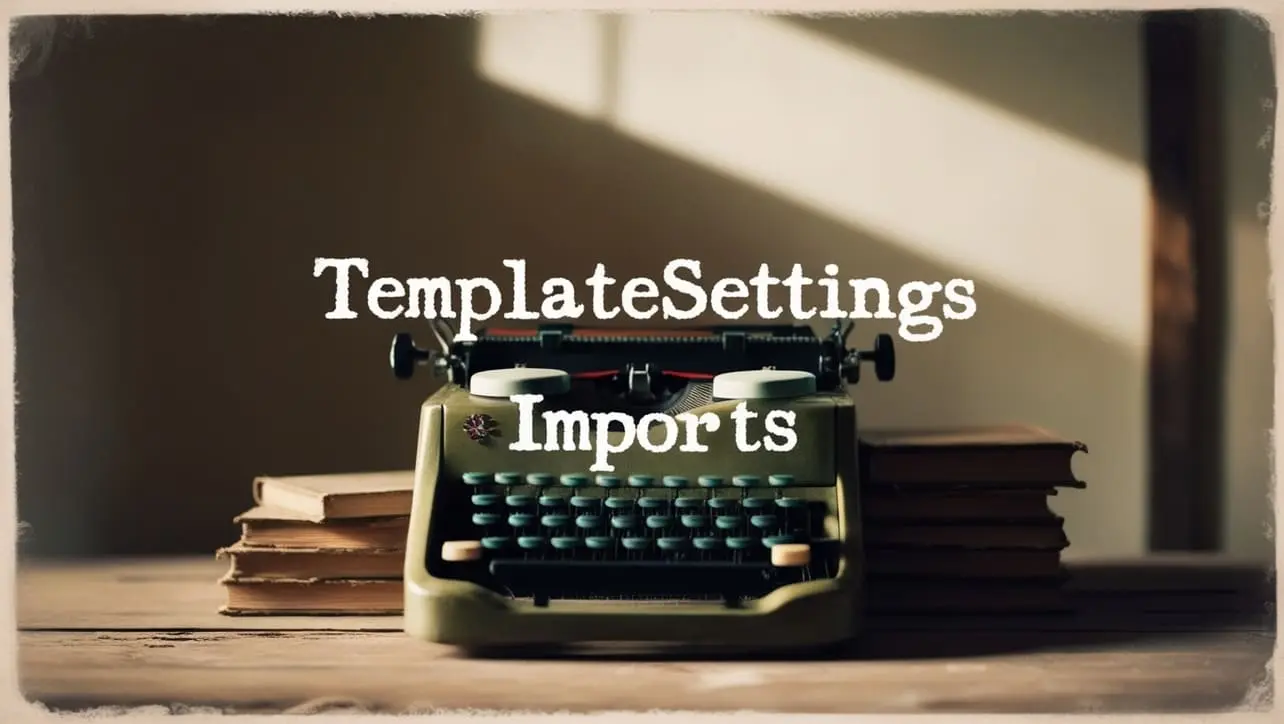
Lodash _.templateSettings.imports Property
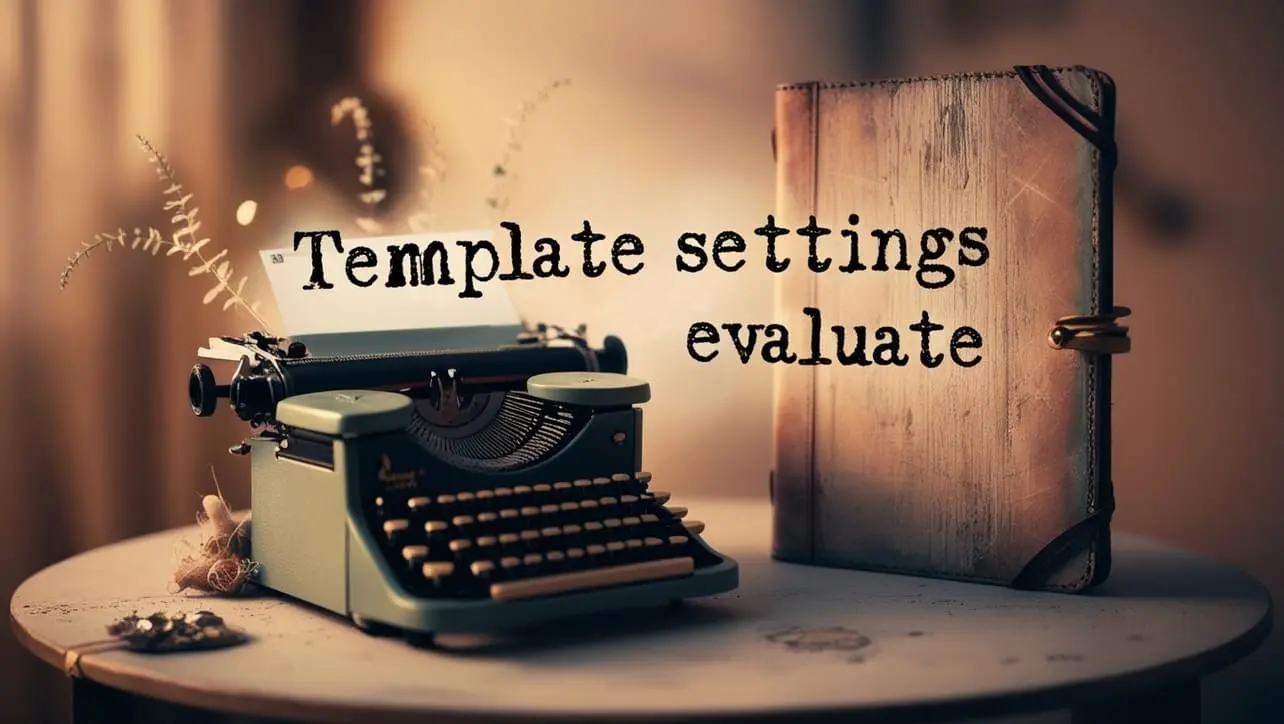
Lodash _.templateSettings.evaluate Property
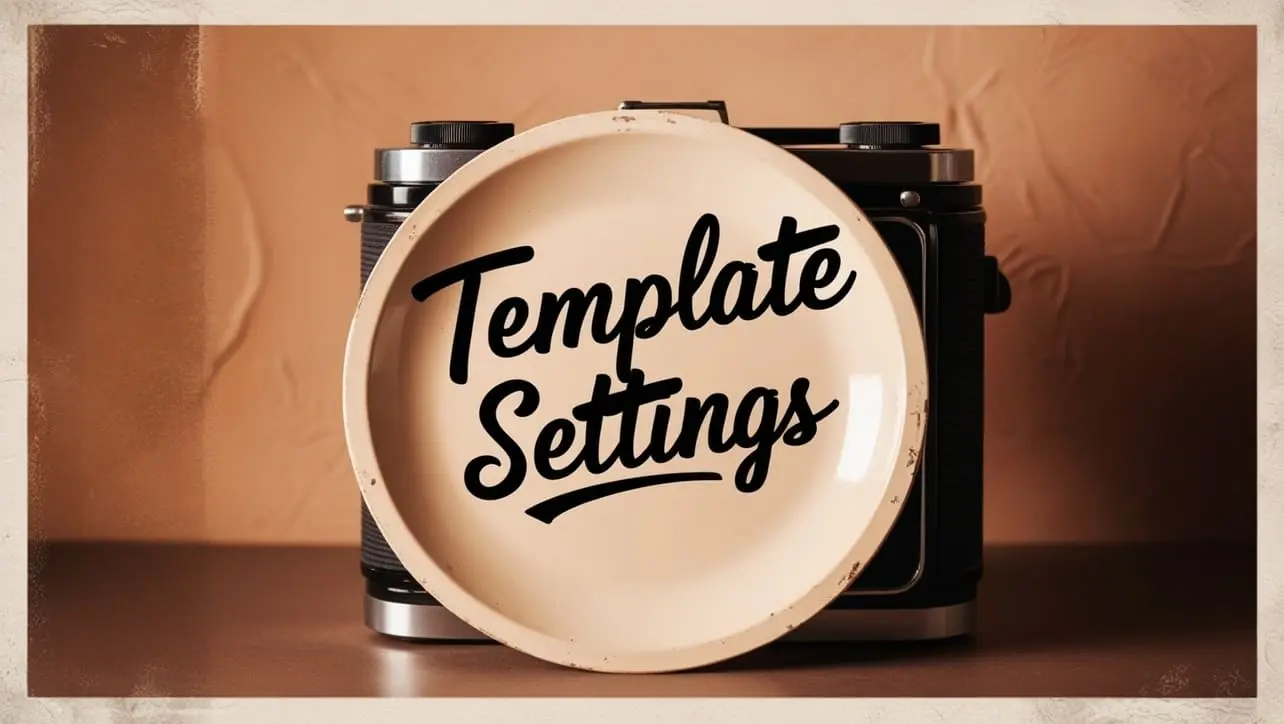
Lodash _.templateSettings Property
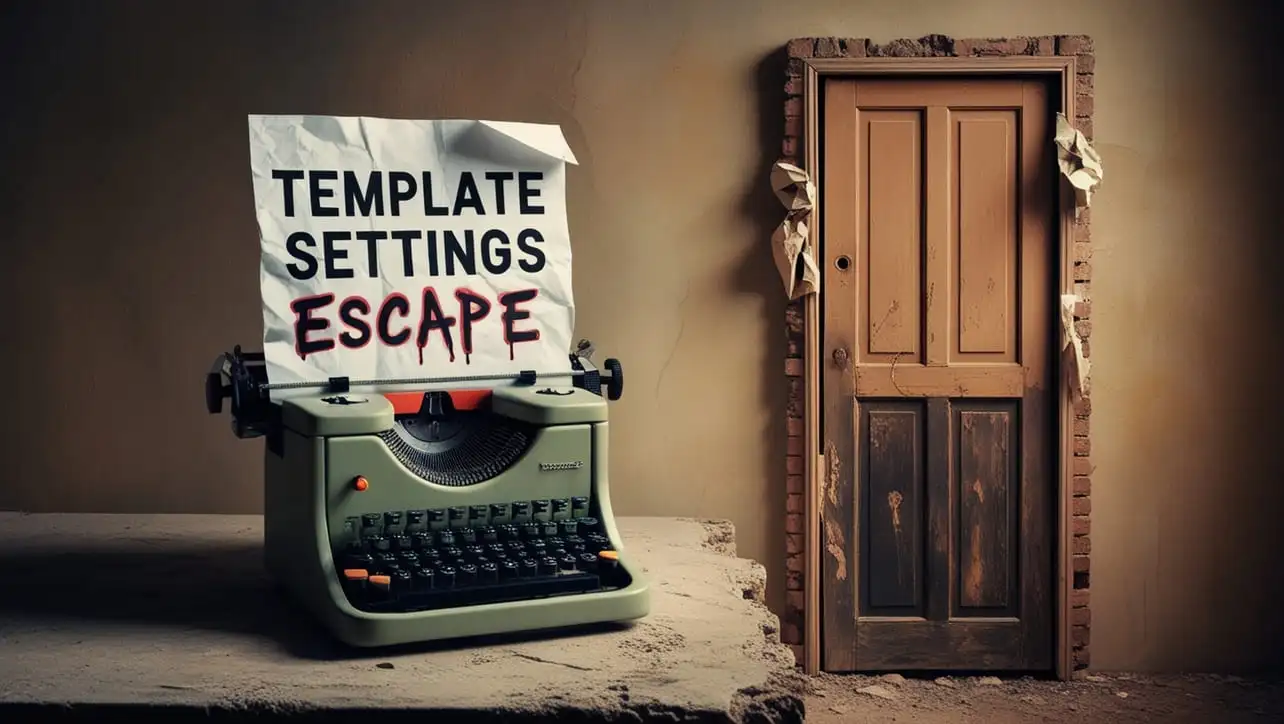
Lodash _.templateSettings.escape Property
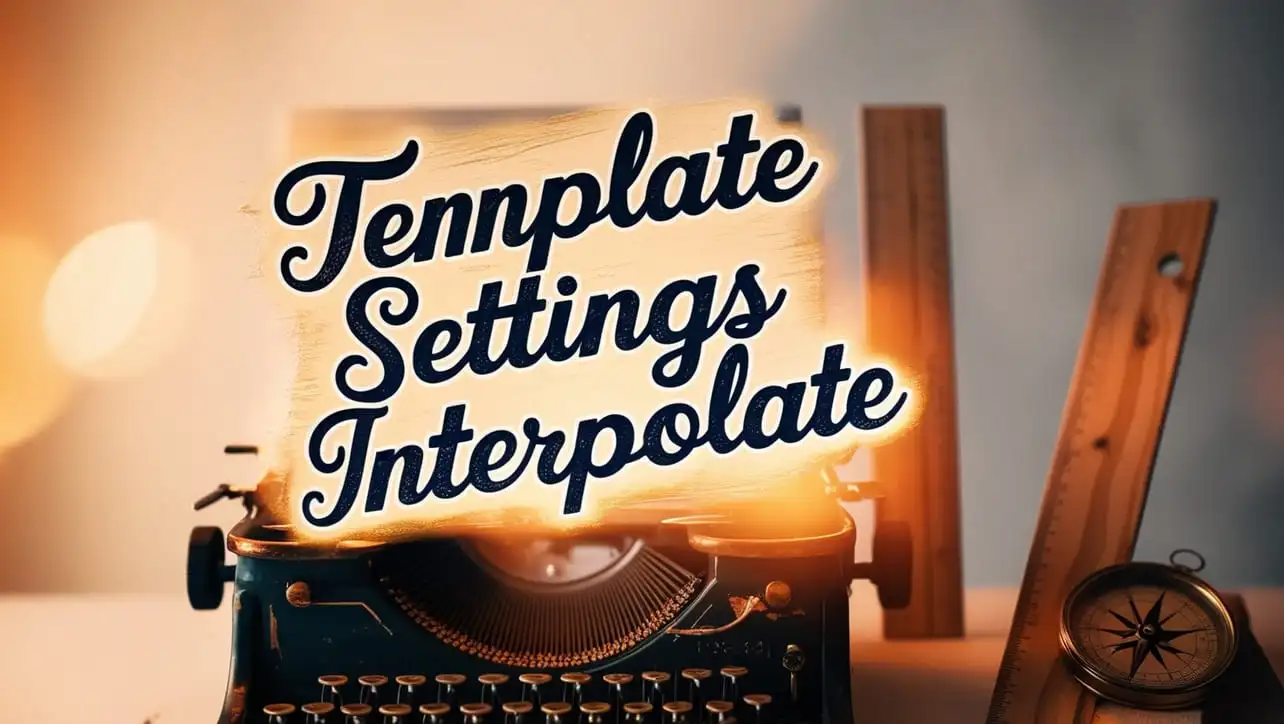
Lodash _.templateSettings.interpolate Property
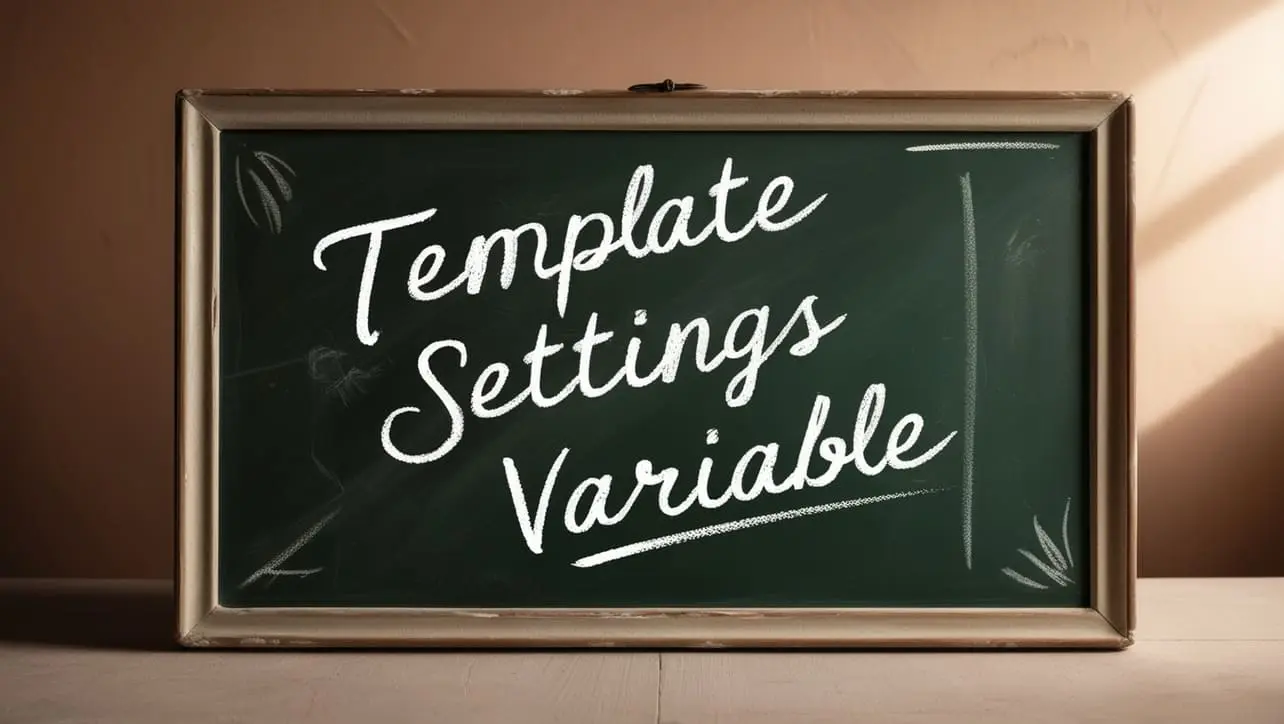
If you have any doubts regarding this article (Lodash _.noop() Util Method), please comment here. I will help you immediately.