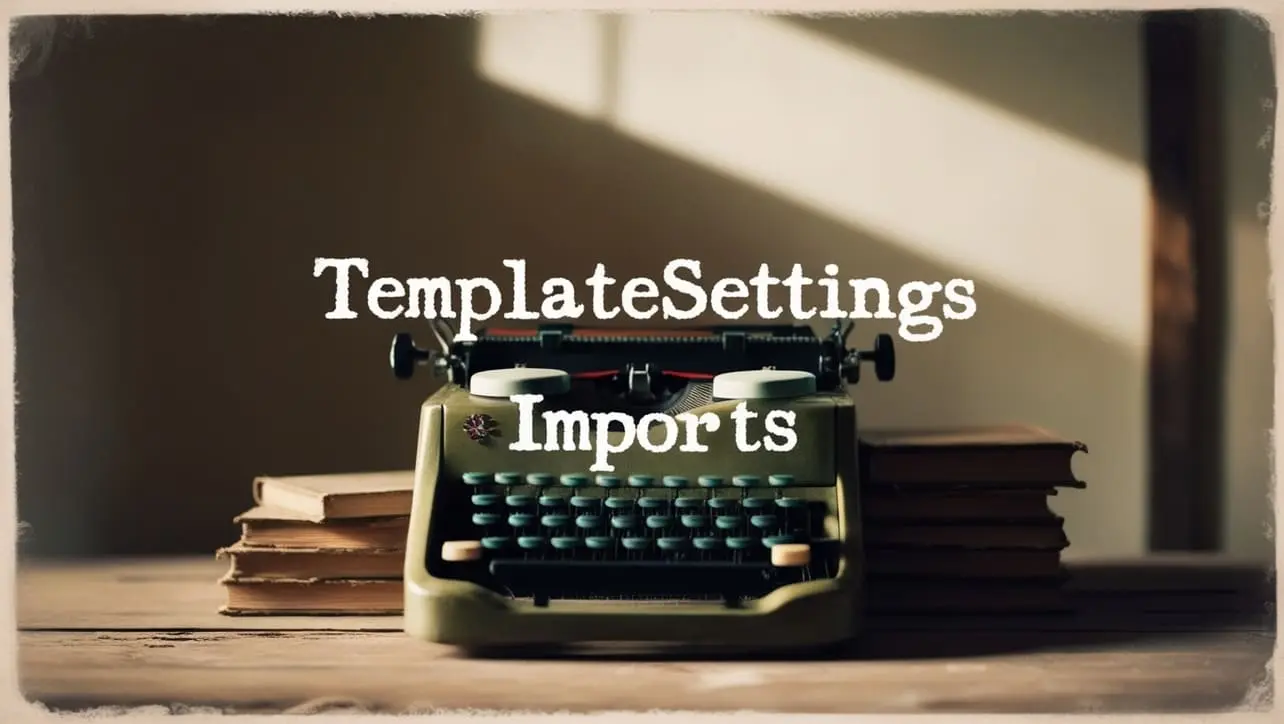
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.noConflict() Util Method
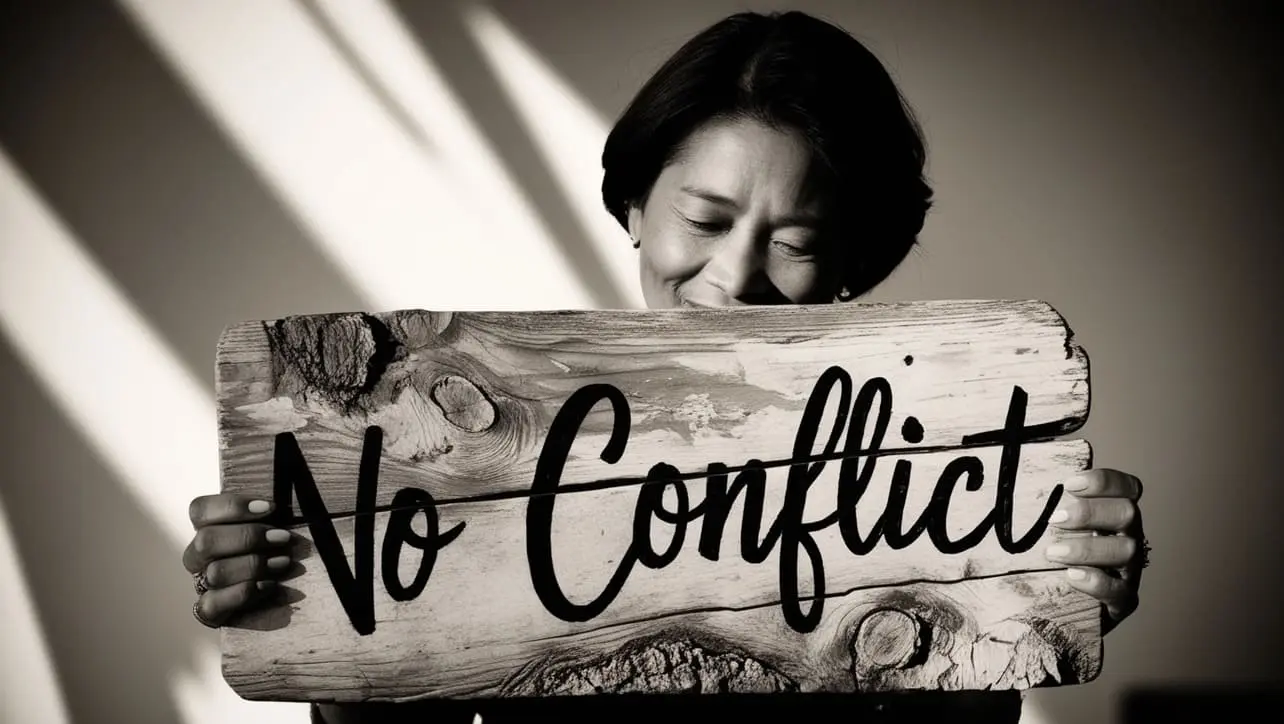
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, managing dependencies and avoiding conflicts between libraries are common challenges. Lodash, a popular utility library, offers a solution to this issue with its _.noConflict()
method. This method enables developers to restore the original global _ variable, ensuring compatibility with other libraries that may use the same identifier.
Understanding and utilizing _.noConflict()
is essential for maintaining a clean and conflict-free development environment.
🧠 Understanding _.noConflict() Method
The _.noConflict()
method in Lodash is designed to resolve conflicts that may arise due to the use of the _ variable by other libraries. By invoking _.noConflict()
, you can restore the original value of _ and assign Lodash to a different variable, mitigating conflicts and ensuring seamless integration with other libraries.
💡 Syntax
The syntax for the _.noConflict()
method is straightforward:
_.noConflict()
📝 Example
Let's dive into a simple example to illustrate the usage of the _.noConflict()
method:
// Assume Lodash is included before another library that uses the `_` variable
_.noConflict();
// Lodash is now assigned to the variable 'lodash'
const lodash = _.noConflict();
// Now, the global '_' variable is restored to its original value, allowing other libraries to use it
In this example, invoking _.noConflict() restores the global _ variable to its original state, ensuring compatibility with other libraries.
🏆 Best Practices
When working with the _.noConflict()
method, consider the following best practices:
Restore Original Variable Safely:
When invoking
_.noConflict()
, ensure that the original _ variable is properly restored and that Lodash functionality remains accessible through the assigned variable.example.jsCopiedconst lodash = _.noConflict(); // Verify that Lodash functionality is still accessible through the assigned variable lodash.map([1, 2, 3], n => n * 2);
Assign Lodash to Unique Variable:
To avoid potential conflicts with other libraries, assign Lodash to a unique variable name when using
_.noConflict()
. Choose a variable name that is unlikely to clash with identifiers used by other libraries.example.jsCopiedconst myCustomLodash = _.noConflict(); // Now, Lodash functionality is accessible through the 'myCustomLodash' variable myCustomLodash.forEach([1, 2, 3], console.log);
Document Usage:
Document the use of
_.noConflict()
in your codebase to ensure clarity and maintainability, especially when collaborating with other developers or integrating third-party libraries.example.jsCopied// Use _.noConflict() to avoid conflicts with other libraries const lodash = _.noConflict();
📚 Use Cases
Integration with Other Libraries:
_.noConflict()
is invaluable when integrating Lodash with other libraries that utilize the _ variable. By restoring the original value of _, you can prevent conflicts and ensure smooth interoperability.example.jsCopied// Include Lodash before another library that uses the '_' variable _.noConflict(); // Now, both Lodash and the other library can coexist peacefully
Legacy Code Compatibility:
When working with legacy codebases that use the _ variable for other purposes,
_.noConflict()
allows you to incorporate Lodash without disrupting existing functionality.example.jsCopied// Transition legacy code to use 'lodash' instead of '_' const lodash = _.noConflict(); // Update code to utilize 'lodash' for Lodash functionality
Third-Party Module Integration:
In scenarios where third-party modules or plugins rely on the _ variable,
_.noConflict()
ensures seamless integration by restoring the original global _ variable.example.jsCopied// Integrate Lodash with a third-party module that uses the '_' variable _.noConflict(); // Now, both Lodash and the third-party module can coexist without conflicts
🎉 Conclusion
The _.noConflict()
method in Lodash provides a straightforward solution for resolving conflicts with other libraries or legacy codebases that use the _ variable. By restoring the original global _ variable and assigning Lodash to a unique identifier, developers can ensure compatibility and maintain a clean and conflict-free development environment.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.noConflict()
method in your Lodash projects.
👨💻 Join our Community:
Author
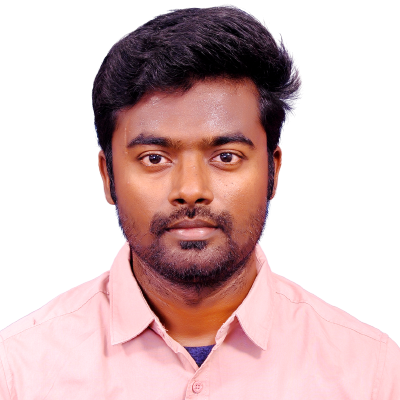
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
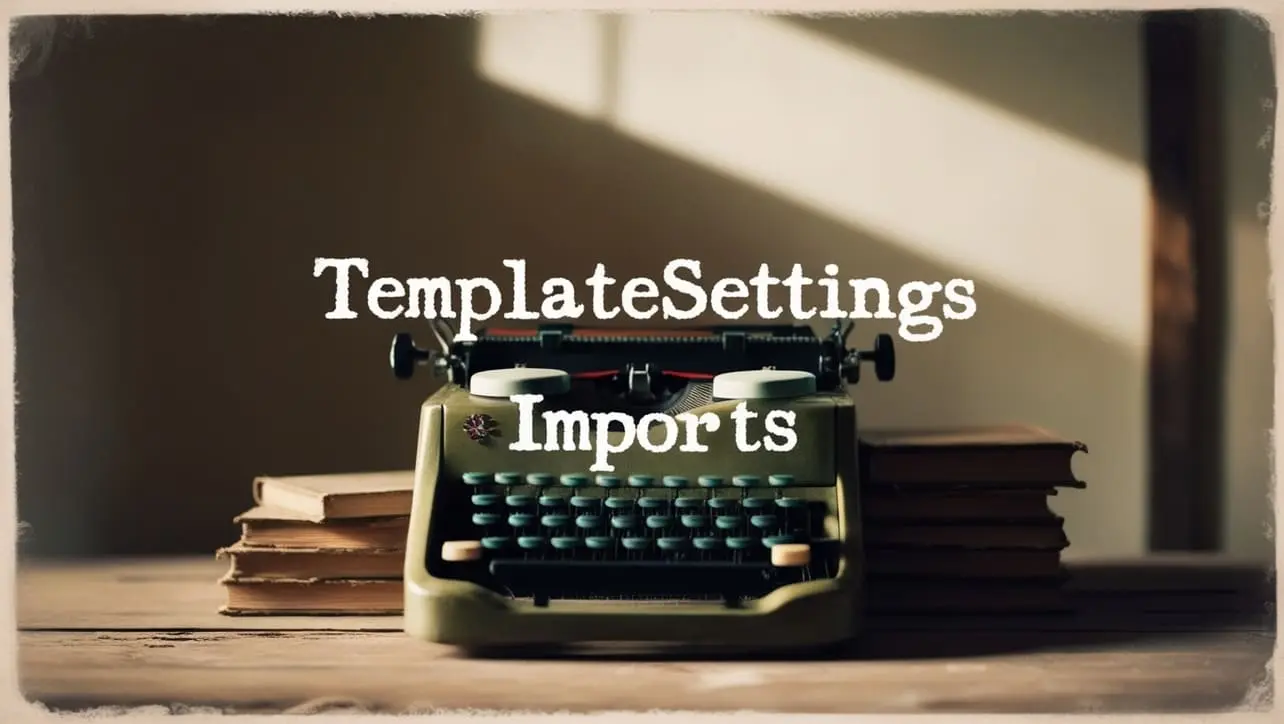
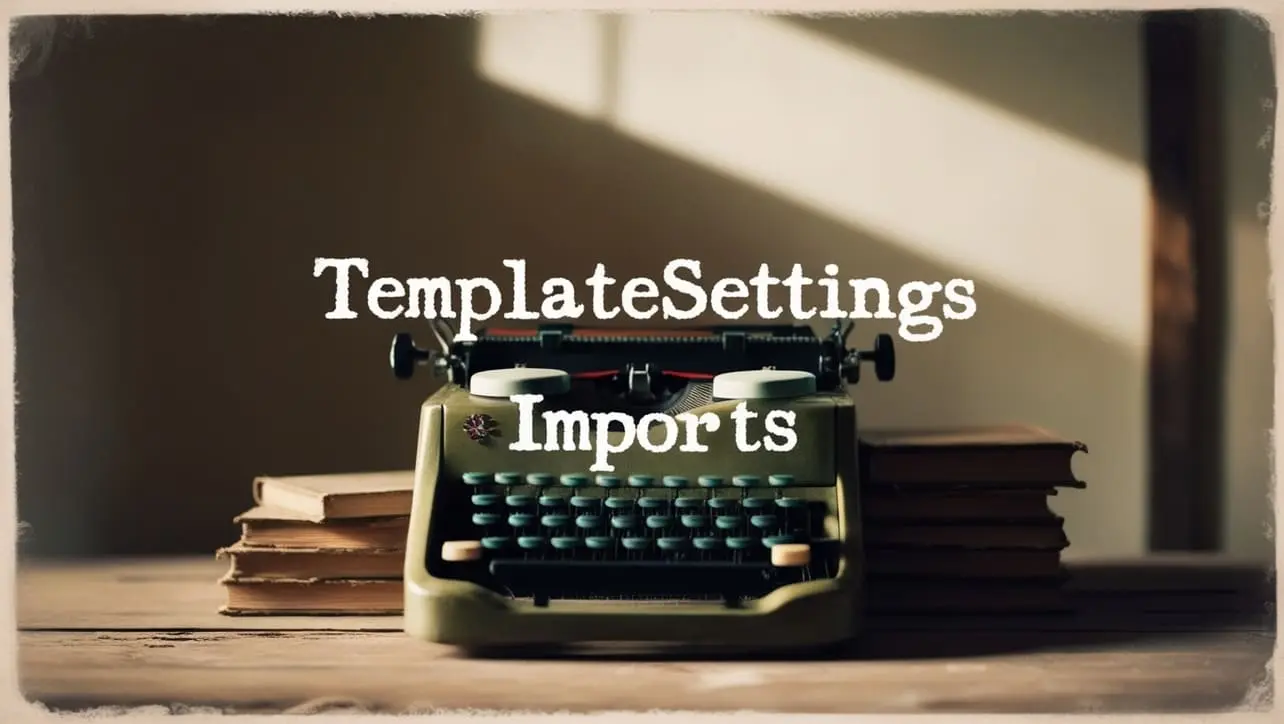
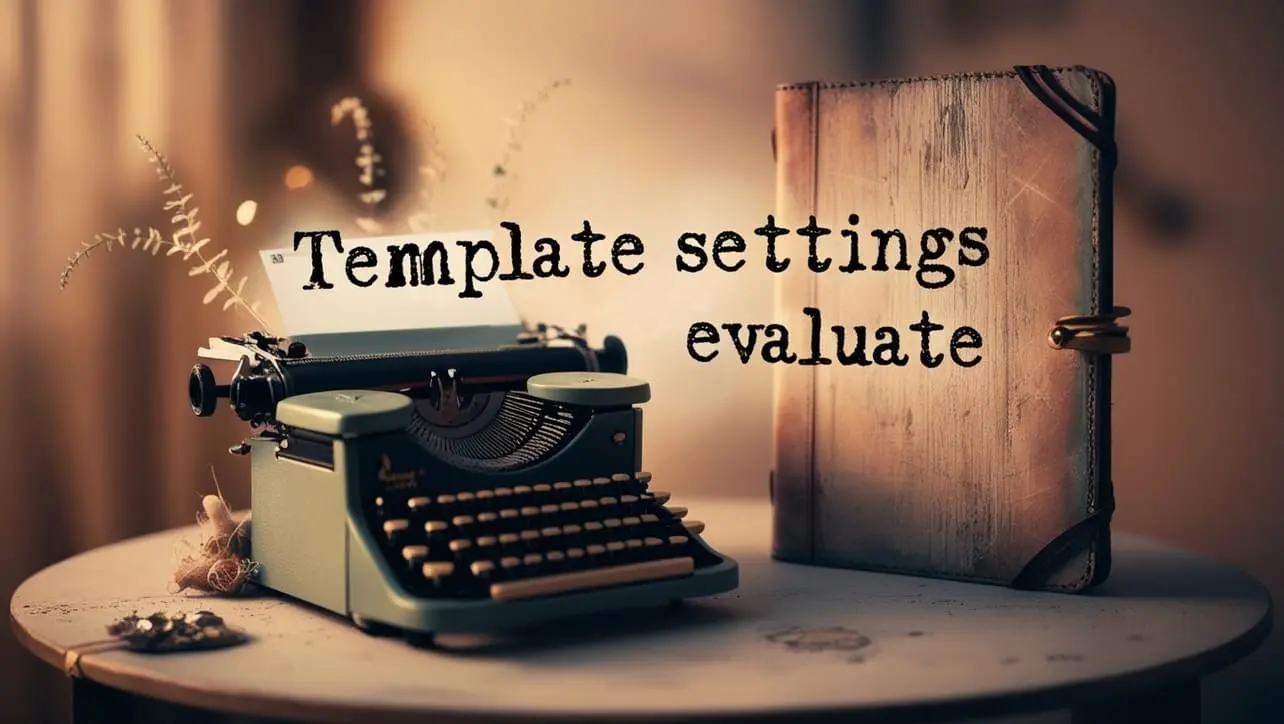
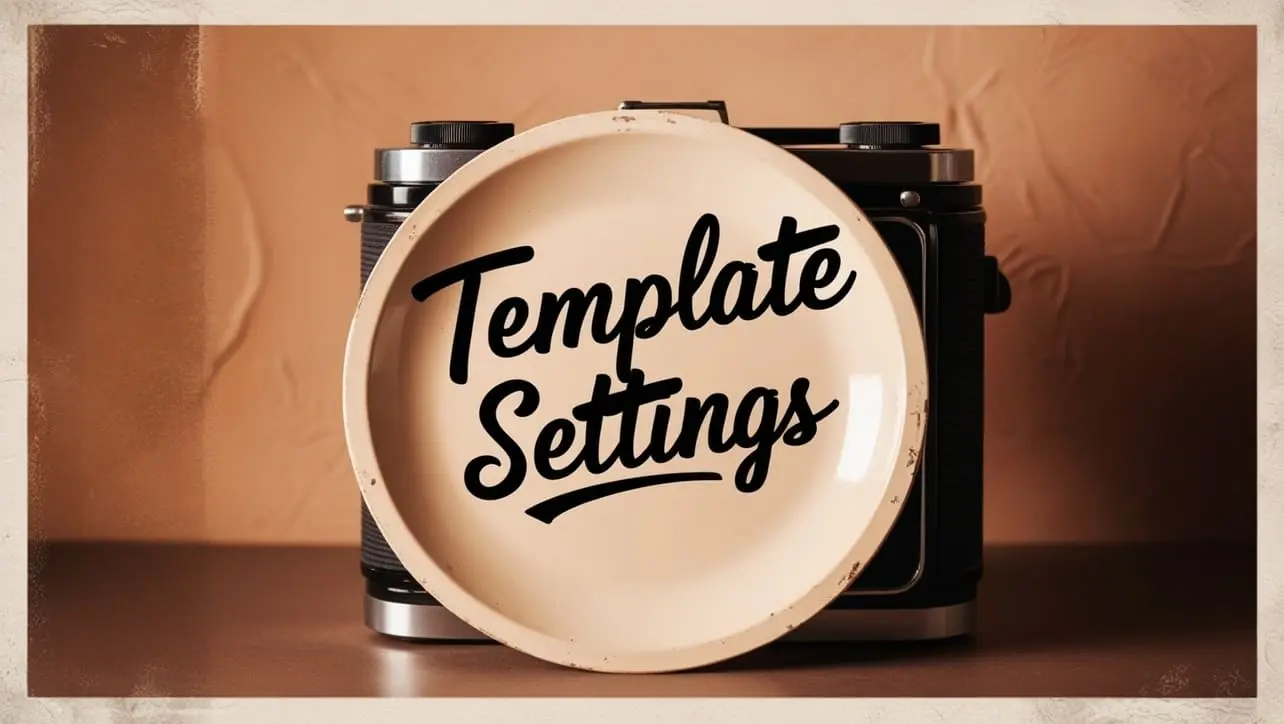
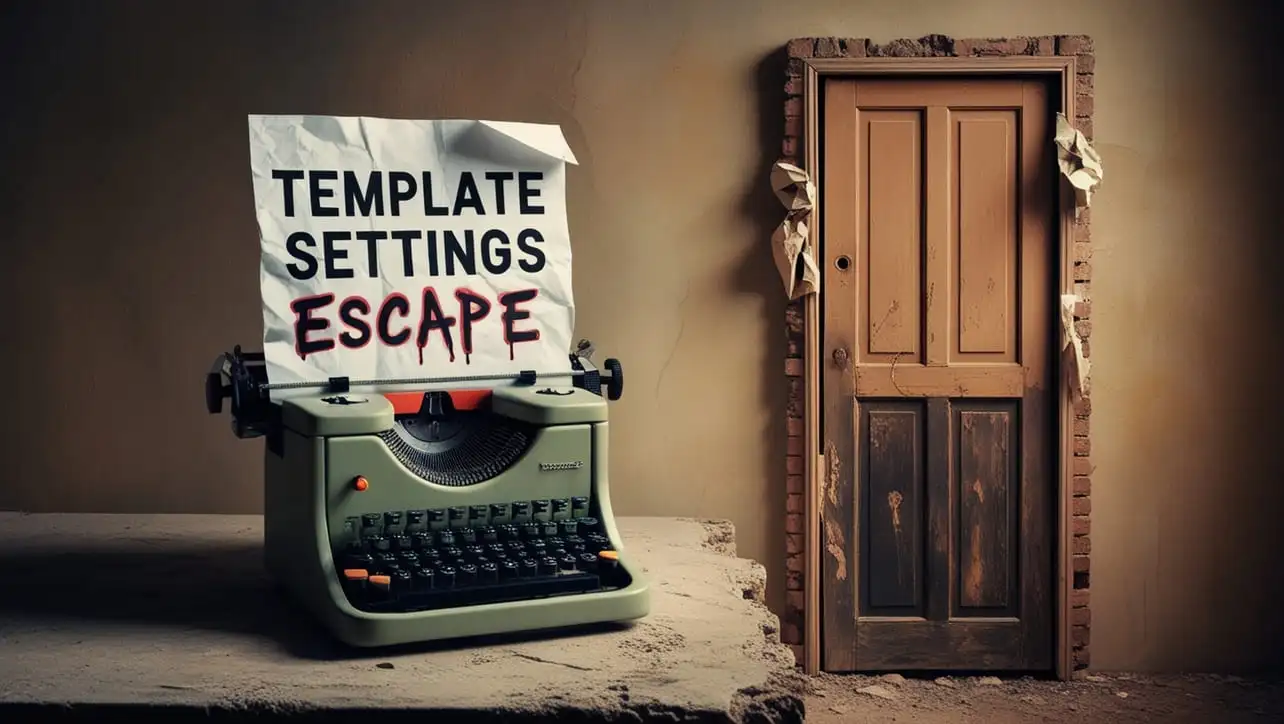
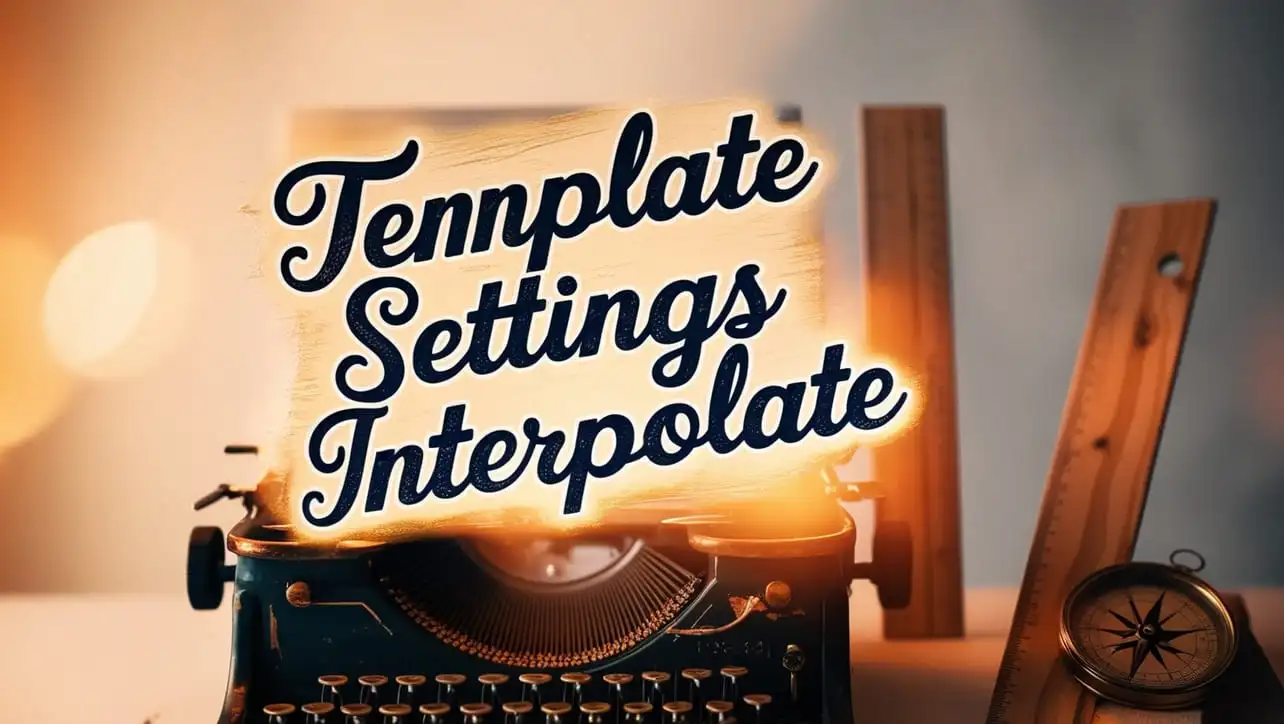
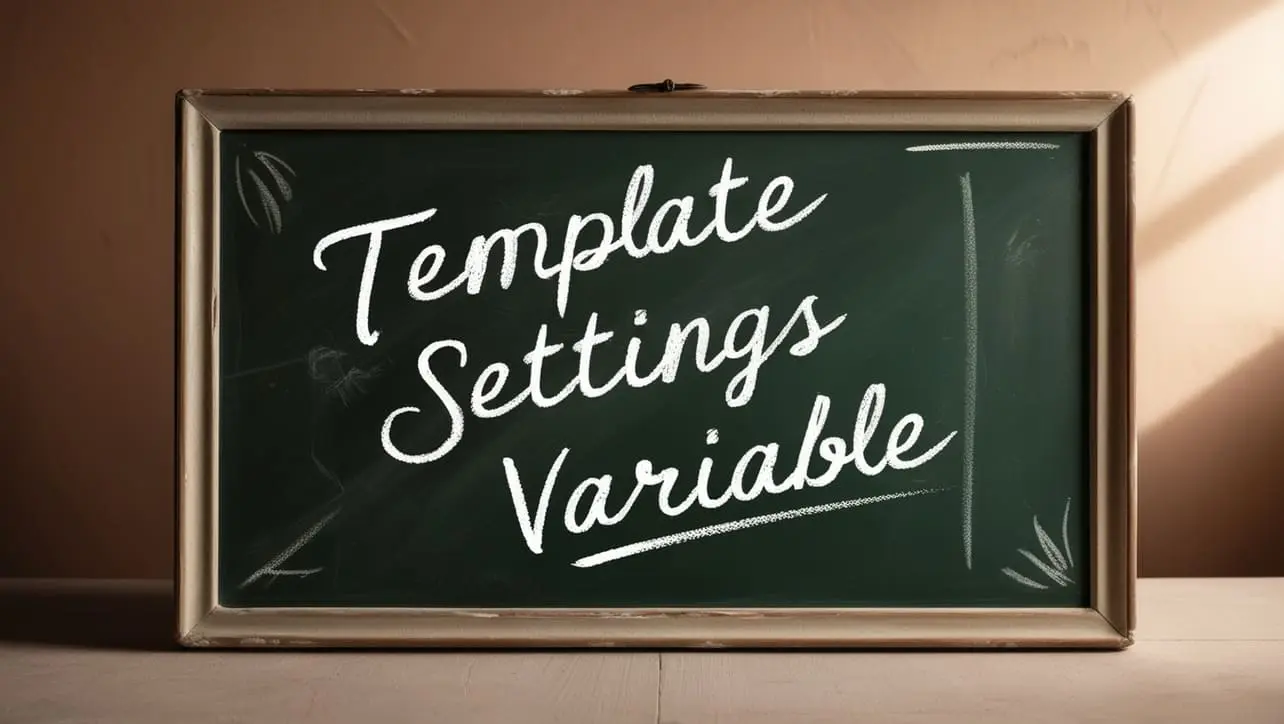
If you have any doubts regarding this article (Lodash _.noConflict() Util Method), please comment here. I will help you immediately.