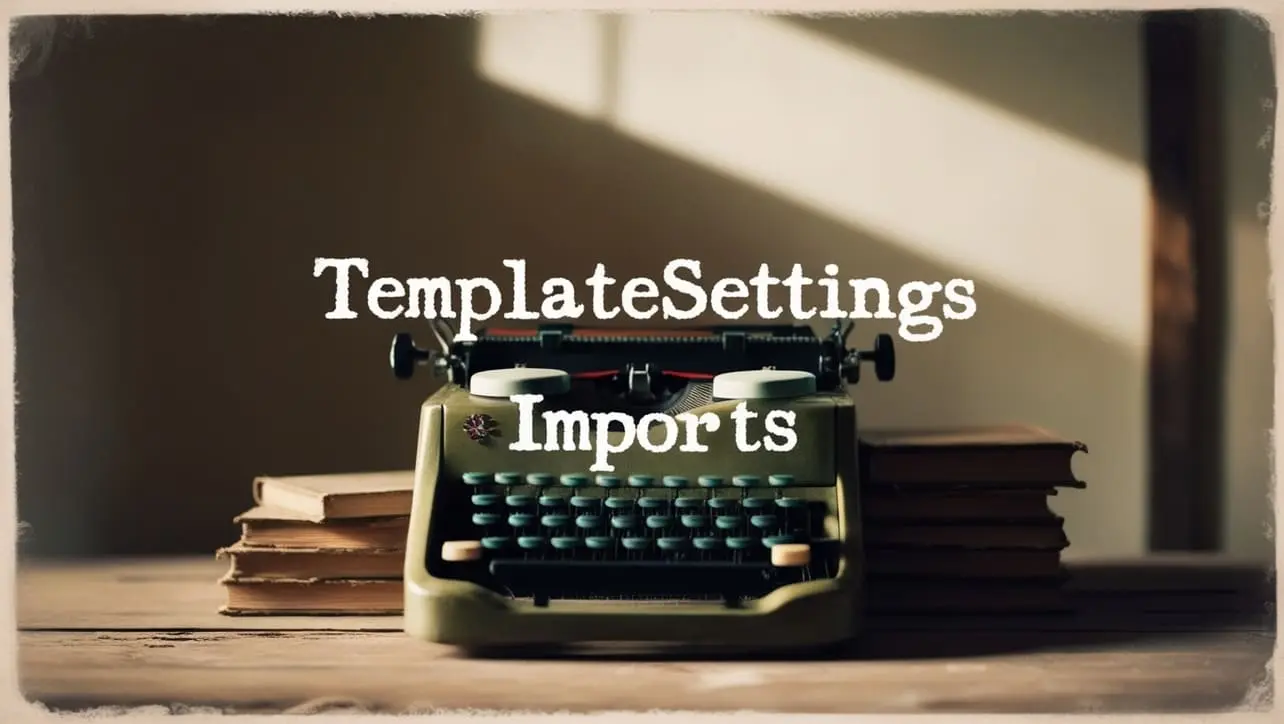
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.matches() Util Method
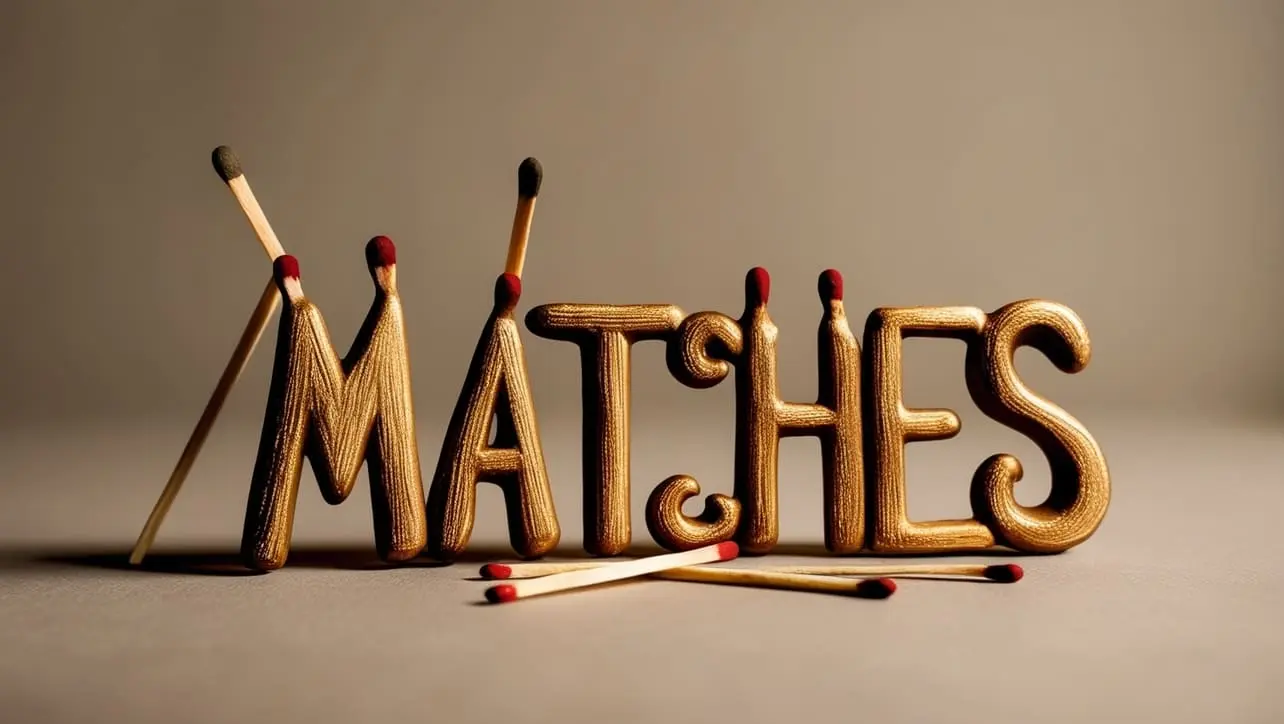
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, working with complex data structures often requires precise matching of object properties. Lodash, a popular utility library, provides a range of functions to simplify such tasks.
Among these functions is _.matches()
, a versatile utility method for creating a predicate function that performs partial deep comparison.
🧠 Understanding _.matches() Method
The _.matches()
method in Lodash creates a predicate function that checks if the provided object properties match those of a given source object. This allows for flexible and customizable matching criteria, making it suitable for various use cases such as filtering or finding objects in collections.
💡 Syntax
The syntax for the _.matches()
method is straightforward:
_.matches(source)
- source: The object to match against.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.matches()
method:
const _ = require('lodash');
const users = [
{ id: 1, name: 'John', age: 30 },
{ id: 2, name: 'Jane', age: 25 },
{ id: 3, name: 'Alice', age: 35 }
];
const ageFilter = _.filter(users, _.matches({ age: 30 }));
console.log(ageFilter);
// Output: [{ id: 1, name: 'John', age: 30 }]
In this example, _.matches()
is used to create a predicate function that filters users based on their age.
🏆 Best Practices
When working with the _.matches()
method, consider the following best practices:
Precise Property Matching:
Ensure that the properties provided in the source object exactly match the properties of the objects being compared. Inexact property names or values may lead to unexpected results.
example.jsCopiedconst data = [ { id: 1, name: 'John', age: 30 }, { id: 2, name: 'Jane', age: 25 }, ]; const matcher = _.matches({ id: 1, name: 'John' }); const result = _.find(data, matcher); console.log(result); // Output: { id: 1, name: 'John', age: 30 }
Combining with Other Lodash Functions:
Utilize
_.matches()
in conjunction with other Lodash functions, such as _.filter() or _.find(), to perform advanced data manipulation tasks efficiently.example.jsCopiedconst filteredData = _.filter(users, _.matches({ age: 30, name: 'John' })); console.log(filteredData); // Output: [{ id: 1, name: 'John', age: 30 }]
Handling Nested Objects:
_.matches()
supports deep comparison of nested objects. Ensure that the source object reflects the structure of the objects being compared to accurately match nested properties.example.jsCopiedconst nestedData = [ { id: 1, user: { name: 'John', age: 30 } }, { id: 2, user: { name: 'Jane', age: 25 } } ]; const nestedMatcher = _.matches({ user: { name: 'John' } }); const nestedResult = _.find(nestedData, nestedMatcher); console.log(nestedResult); // Output: { id: 1, user: { name: 'John', age: 30 } }
📚 Use Cases
Filtering Arrays of Objects:
_.matches()
is commonly used to filter arrays of objects based on specific criteria, simplifying the process of data manipulation and selection.example.jsCopiedconst filteredUsers = _.filter(users, _.matches({ age: 30 })); console.log(filteredUsers); // Output: [{ id: 1, name: 'John', age: 30 }]
Finding Objects in Collections:
The method can be utilized to find objects within collections that match a given set of properties, providing a convenient way to search for specific data.
example.jsCopiedconst foundUser = _.find(users, _.matches({ name: 'Jane' })); console.log(foundUser); // Output: { id: 2, name: 'Jane', age: 25 }
Conditional Logic:
_.matches()
can be used within conditional statements to perform actions based on whether an object meets certain criteria.example.jsCopiedconst user = { name: 'John', age: 30 }; const isAdmin = _.matches({ role: 'admin' })(user); if (isAdmin) { console.log('User is an admin'); } else { console.log('User is not an admin'); }
🎉 Conclusion
The _.matches()
method in Lodash provides a powerful and flexible solution for partial deep comparison of objects in JavaScript. By creating predicate functions based on specific criteria, developers can efficiently filter, find, and manipulate data structures with ease.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.matches()
method in your Lodash projects.
👨💻 Join our Community:
Author
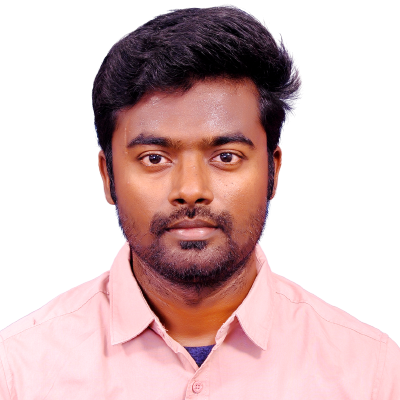
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
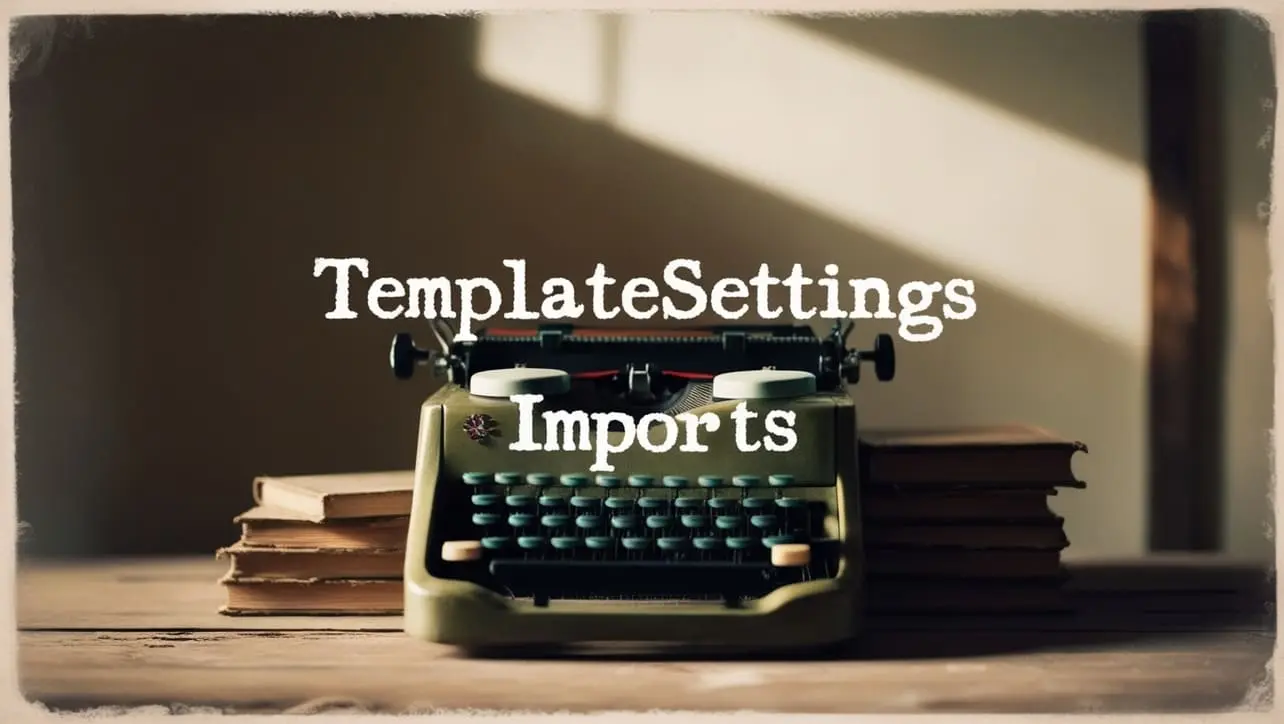
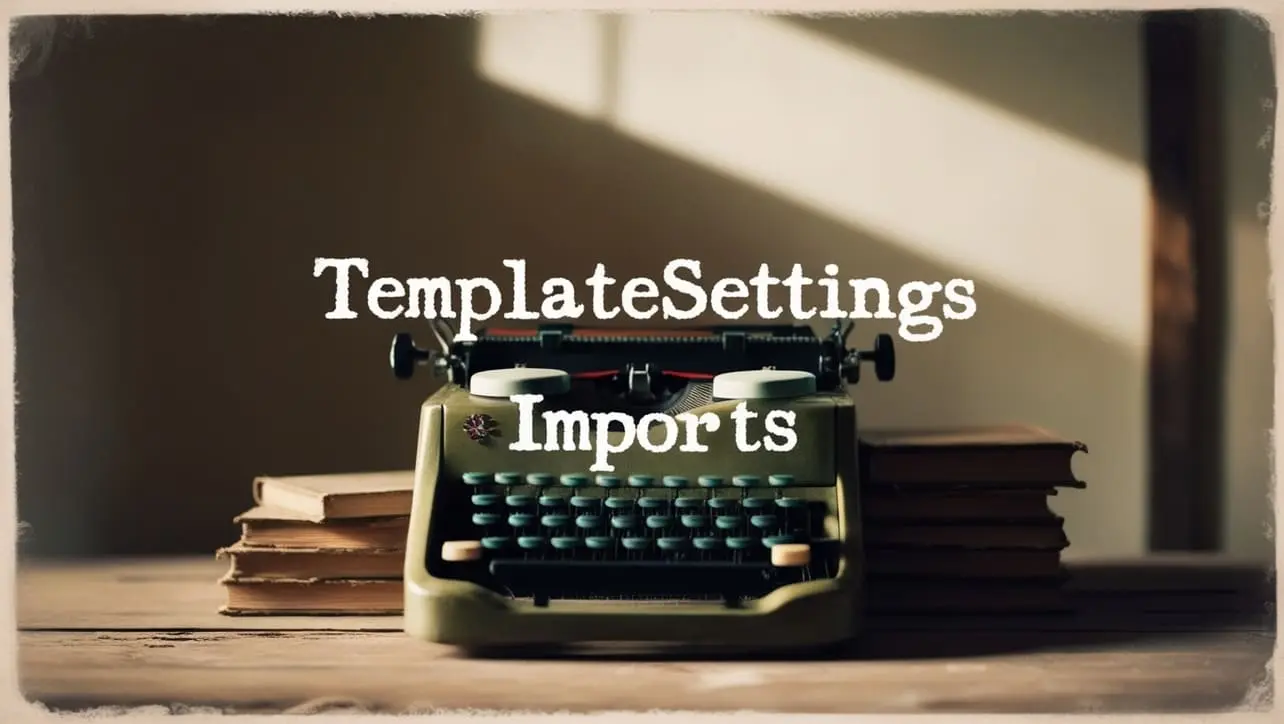
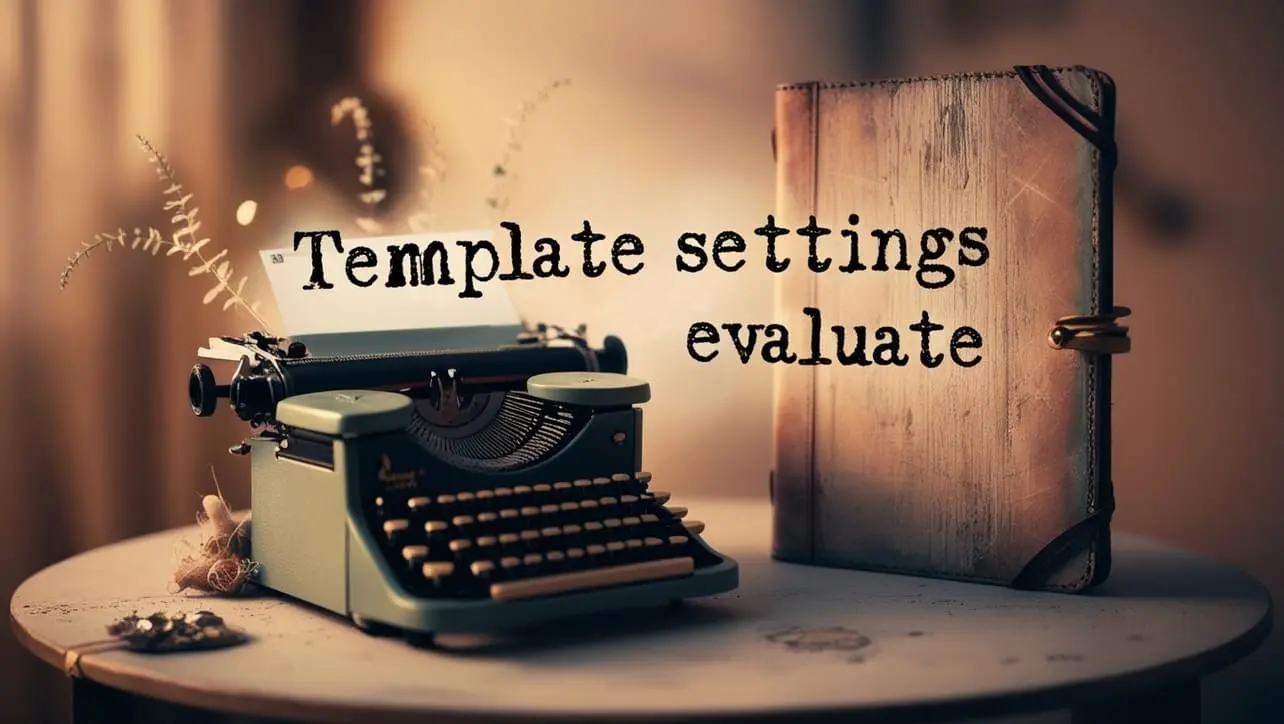
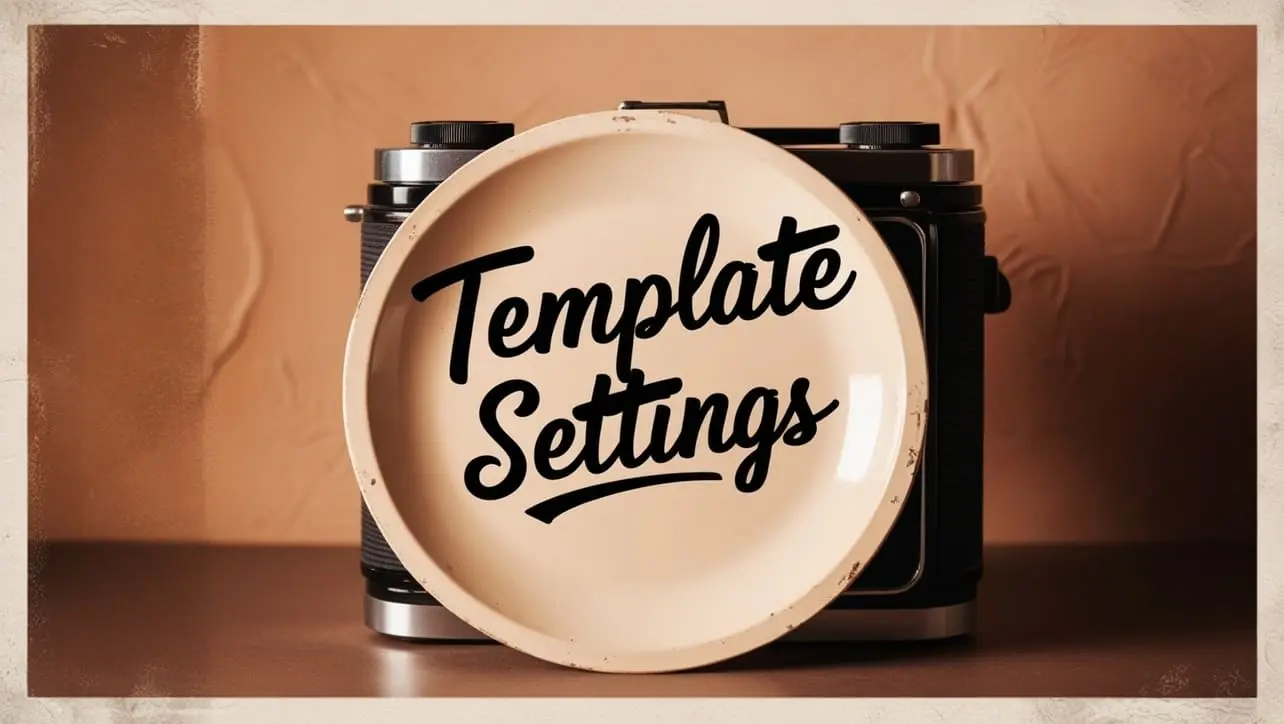
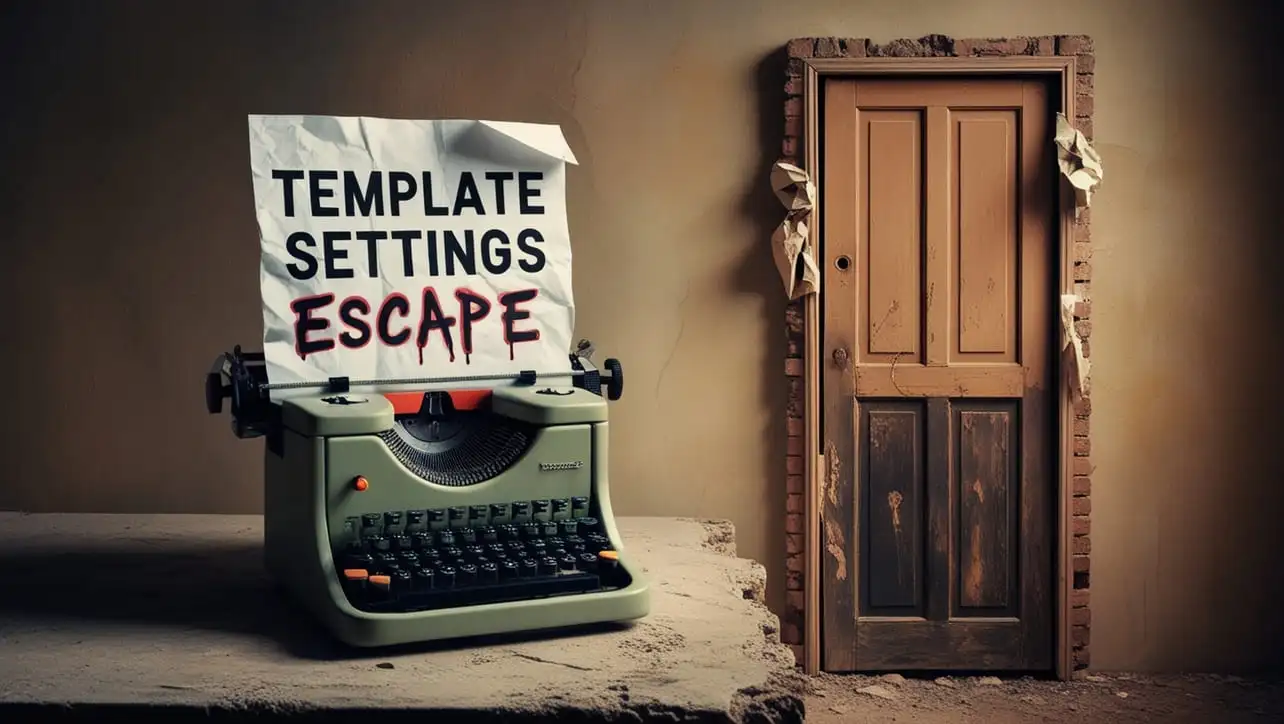
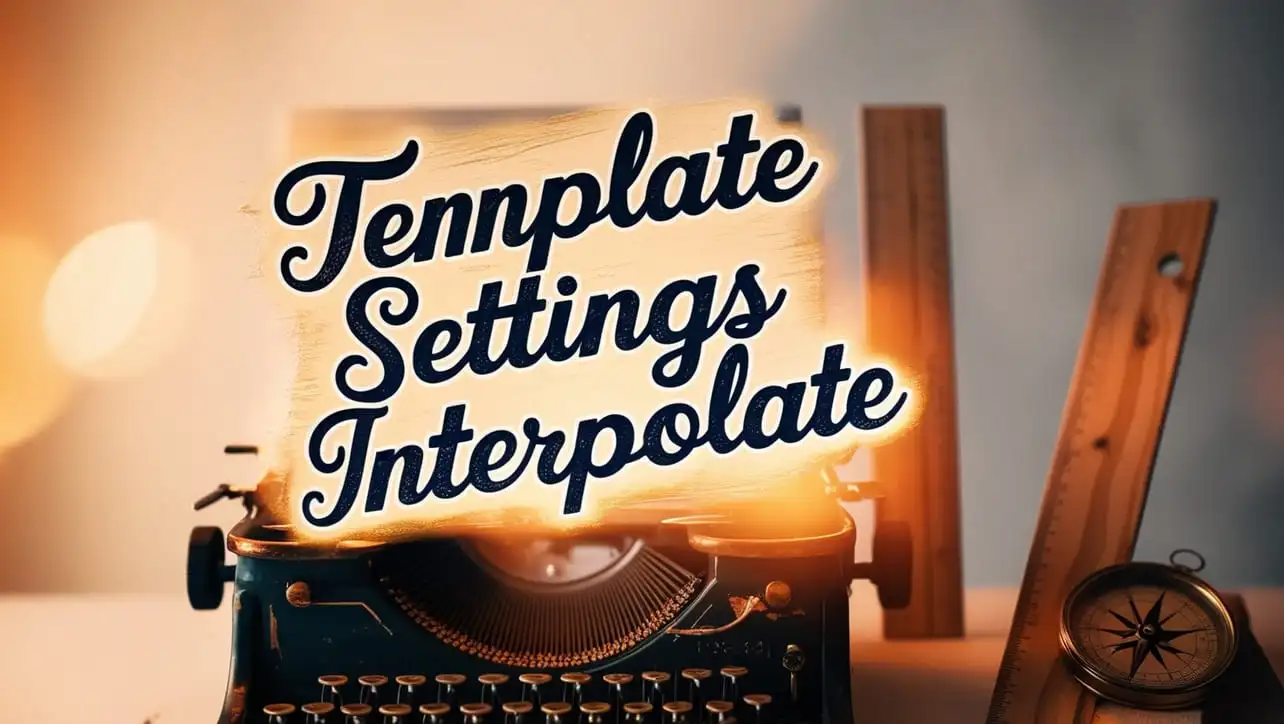
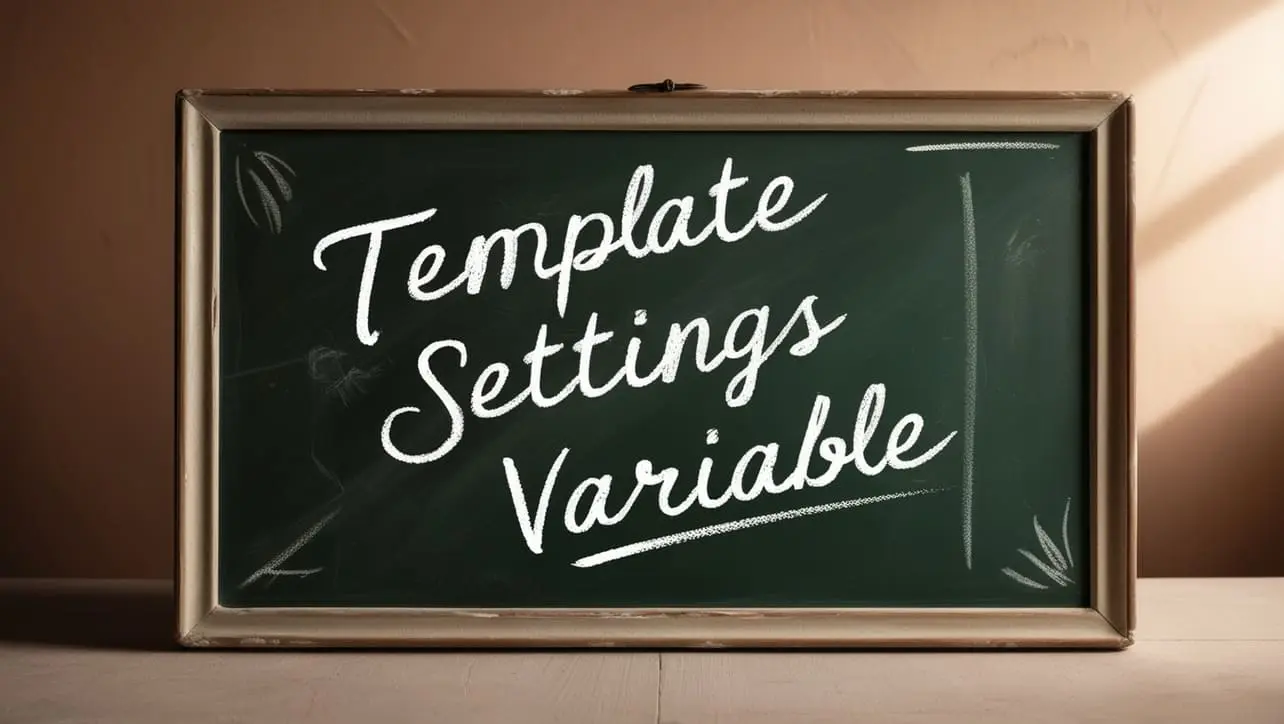
If you have any doubts regarding this article (Lodash _.matches() Util Method), please comment here. I will help you immediately.