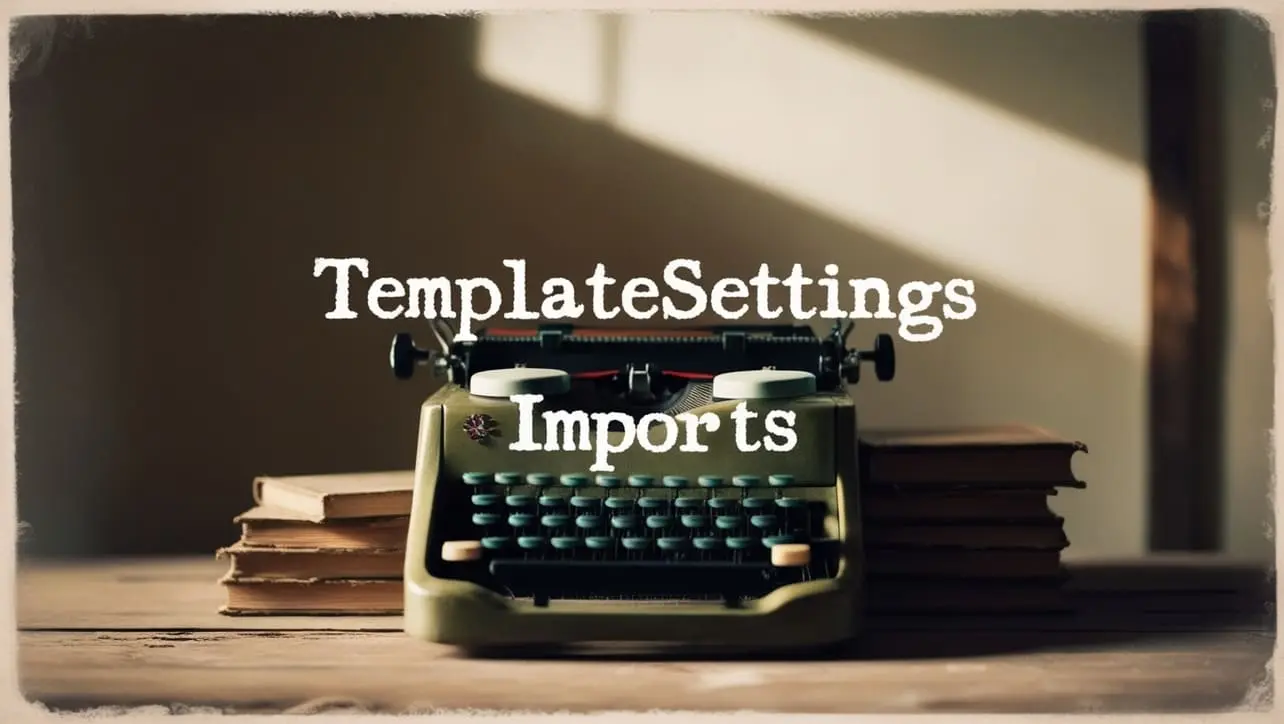
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.identity() Util Method
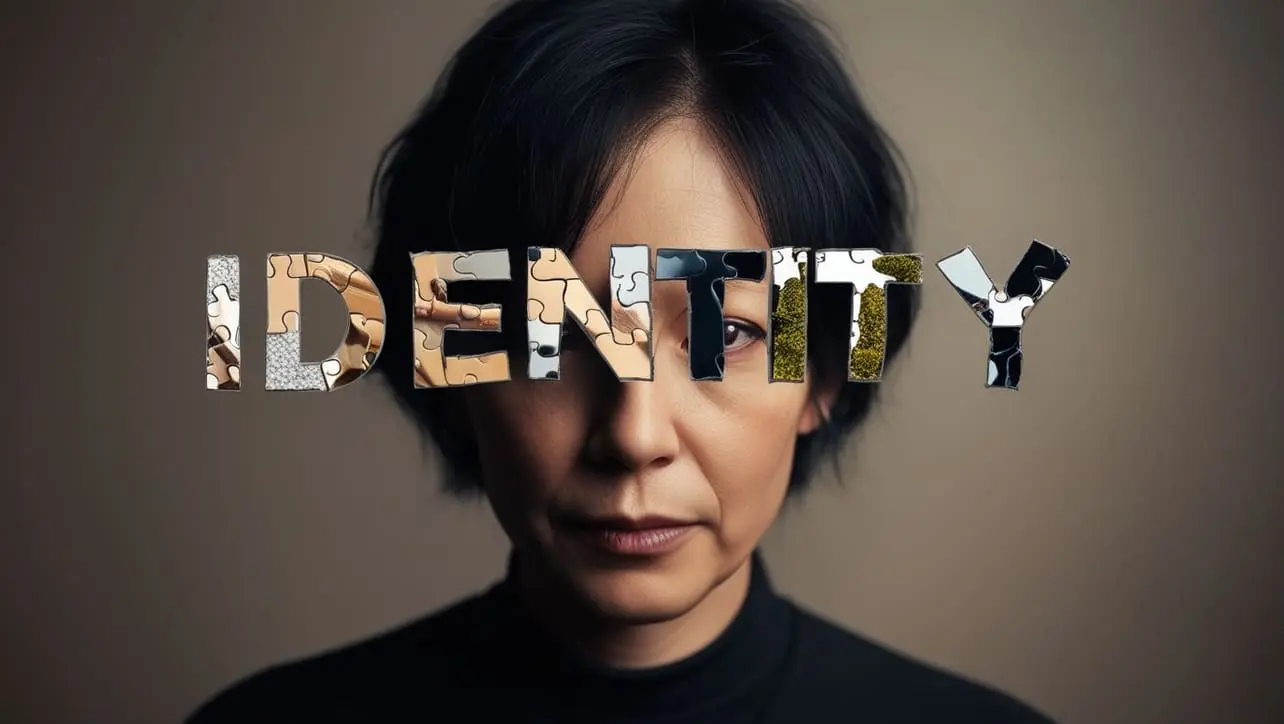
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, handling data transformation and manipulation is a common task. Lodash, a popular utility library, offers a plethora of functions to streamline these operations. Among them is the _.identity()
method, a versatile utility that simply returns the argument provided to it.
Though seemingly straightforward, this method plays a pivotal role in various programming scenarios, enhancing code readability and flexibility.
🧠 Understanding _.identity() Method
The _.identity()
method in Lodash is a simple yet powerful utility that returns the value it receives as an argument. While it may seem trivial at first glance, this function serves as a building block for more complex operations, enabling developers to write concise and expressive code.
💡 Syntax
The syntax for the _.identity()
method is straightforward:
_.identity(value)
- value: The value to return.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.identity()
method:
const _ = require('lodash');
const result = _.identity(42);
console.log(result);
// Output: 42
In this example, _.identity()
returns the argument 42 unchanged.
🏆 Best Practices
When working with the _.identity()
method, consider the following best practices:
No-op Transformation:
Use
_.identity()
when you need to perform a no-op transformation, i.e., when you want to retain the original value without modification.example.jsCopiedconst originalValue = /* ...some value... */; const transformedValue = _.identity(originalValue); console.log(transformedValue); // Output: originalValue
Placeholder for Callbacks:
Employ
_.identity()
as a placeholder for callbacks or mapping functions when you want to pass a function that does nothing.example.jsCopiedconst array = [1, 2, 3, 4]; // Apply a function to each element of the array (in this case, no-op transformation) const mappedArray = _.map(array, _.identity); console.log(mappedArray); // Output: [1, 2, 3, 4]
Code Readability:
Use
_.identity()
to enhance code readability by explicitly stating the intention to keep the value unchanged.example.jsCopiedconst originalValue = /* ...some value... */; const processedValue = someCondition ? _.identity(originalValue) : /* ...some other value... */; console.log(processedValue);
📚 Use Cases
Functional Programming:
In functional programming paradigms,
_.identity()
can be used to compose functions or as an argument to higher-order functions, enhancing code clarity and maintainability.example.jsCopiedconst data = /* ...some data... */ ; // Apply a series of transformations to the data const processedData = _.flow( _.filter(_.identity), // Filter out falsy values _.map(_.identity) // Map each element using identity function (no-op) )(data); console.log(processedData);
Default Values:
When setting default values for variables or function parameters,
_.identity()
can be used to indicate that no transformation is necessary.example.jsCopiedfunction processValue(value = _.identity) { return value; } console.log(processValue(42)); // Output: 42 console.log(processValue()); // Output: undefined
Conditional Operations:
In conditional operations where you need to choose between performing a transformation or keeping the value unchanged,
_.identity()
provides a concise and expressive solution.example.jsCopiedconst value = /* ...some value... */ ; const transformedValue = someCondition ? /* ...perform transformation... */ : _.identity(value); console.log(transformedValue);
🎉 Conclusion
The _.identity()
utility method in Lodash offers a simple yet invaluable tool for retaining the original value without modification. Whether you're writing functional code, setting default values, or performing conditional operations, _.identity()
serves as a reliable companion, enhancing code clarity and flexibility.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.identity()
method in your Lodash projects.
👨💻 Join our Community:
Author
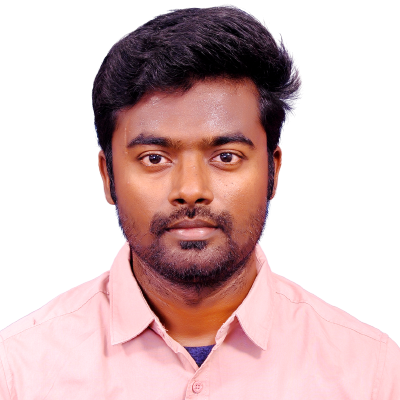
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
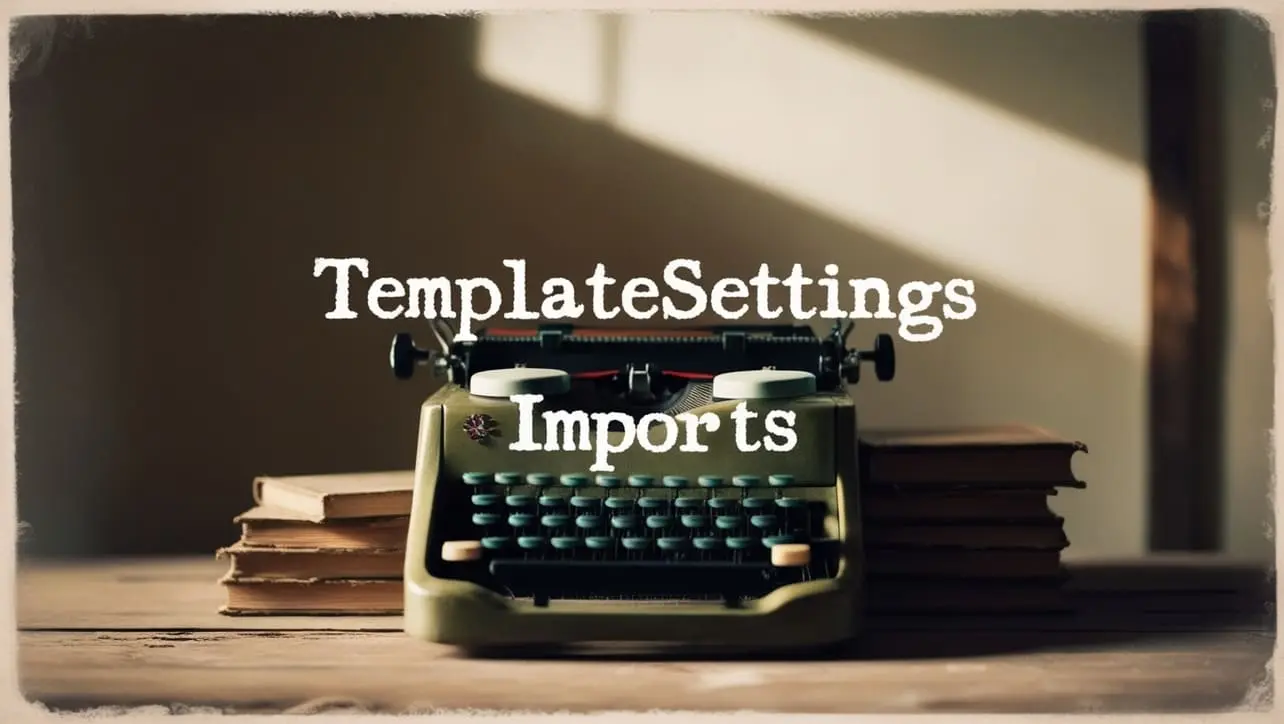
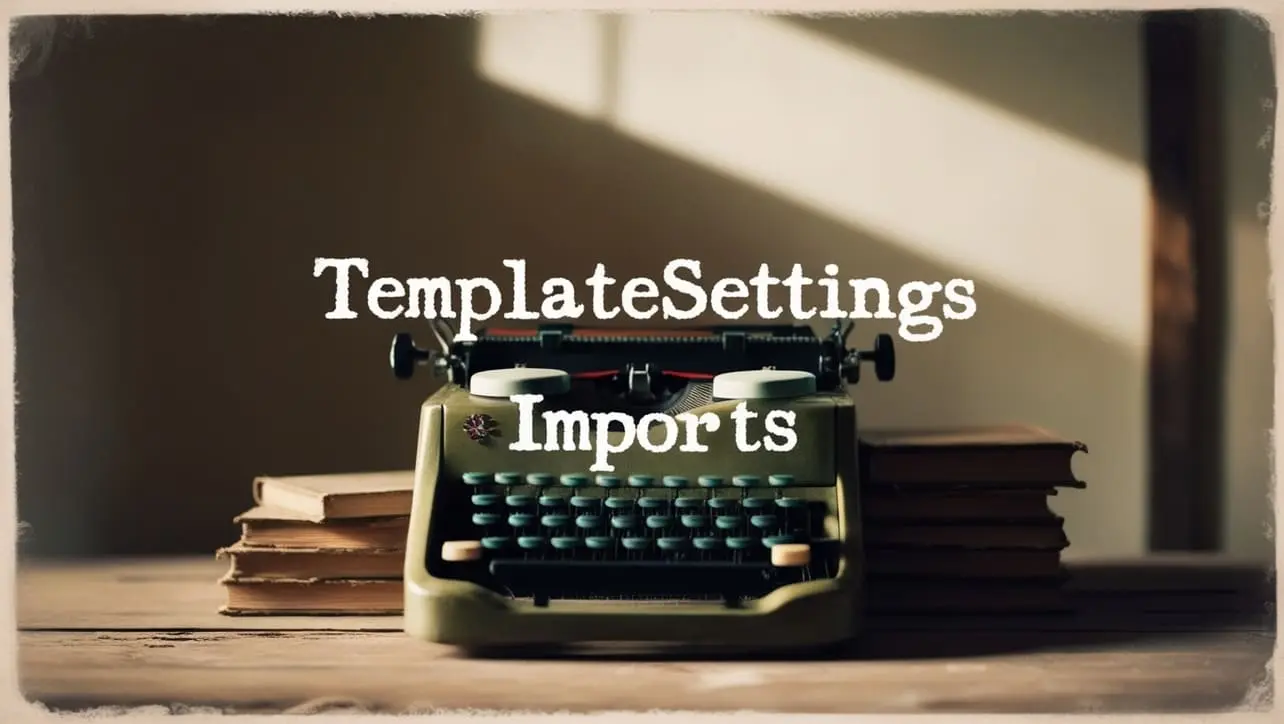
Lodash _.templateSettings.imports Property
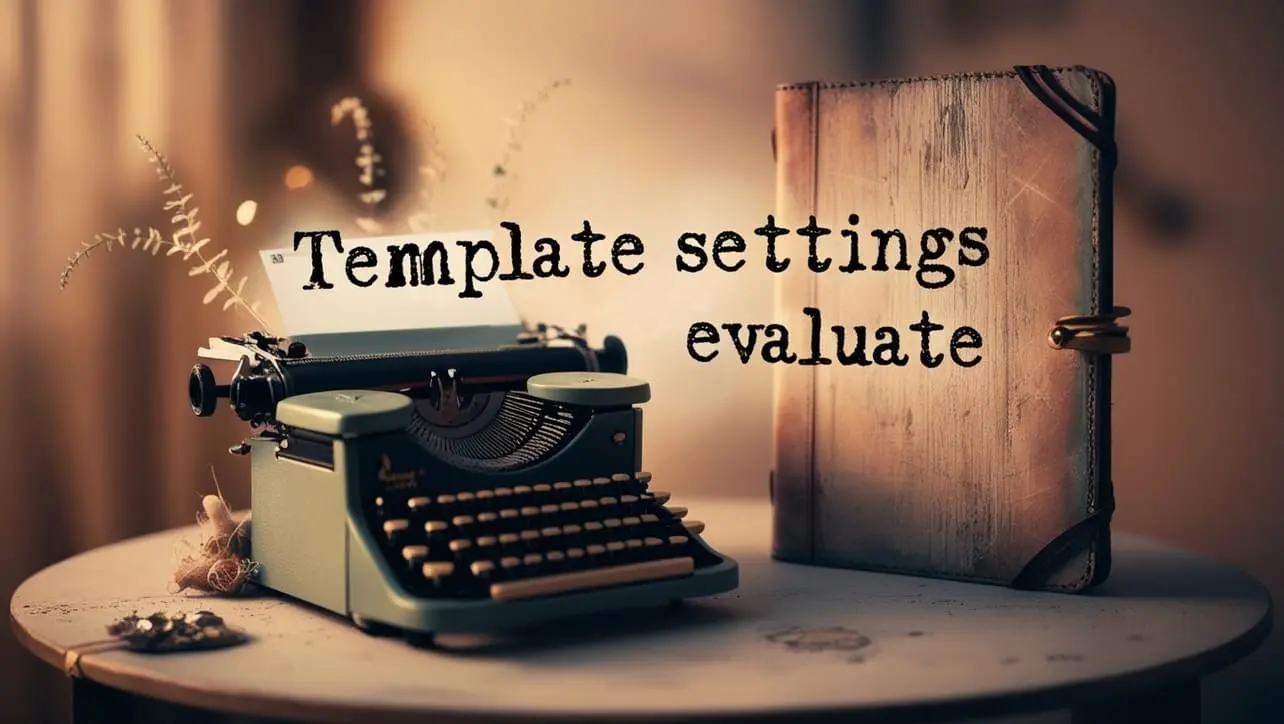
Lodash _.templateSettings.evaluate Property
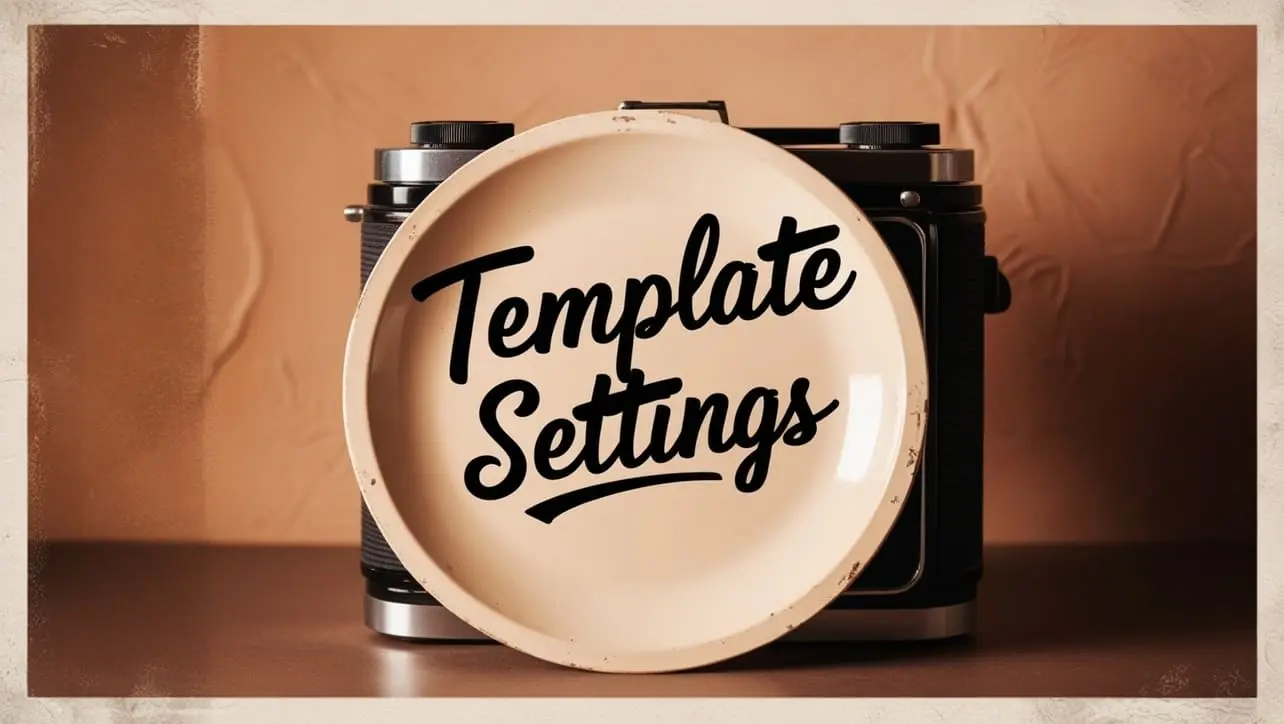
Lodash _.templateSettings Property
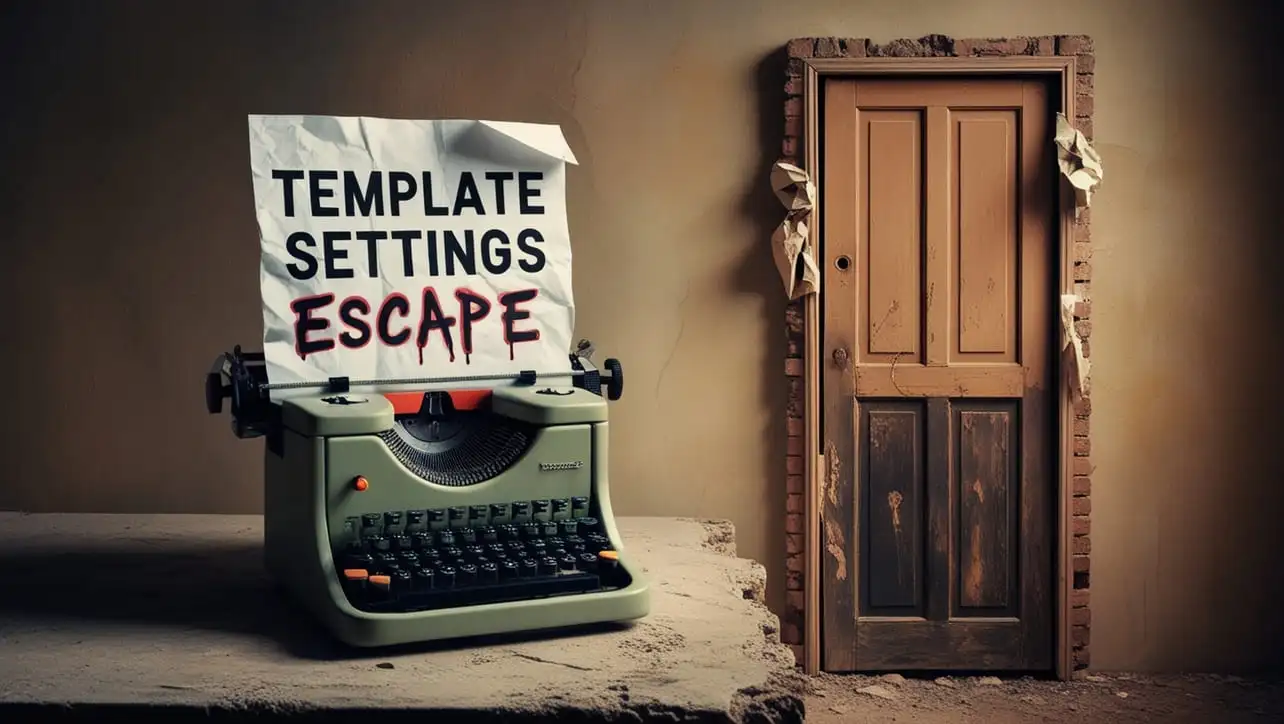
Lodash _.templateSettings.escape Property
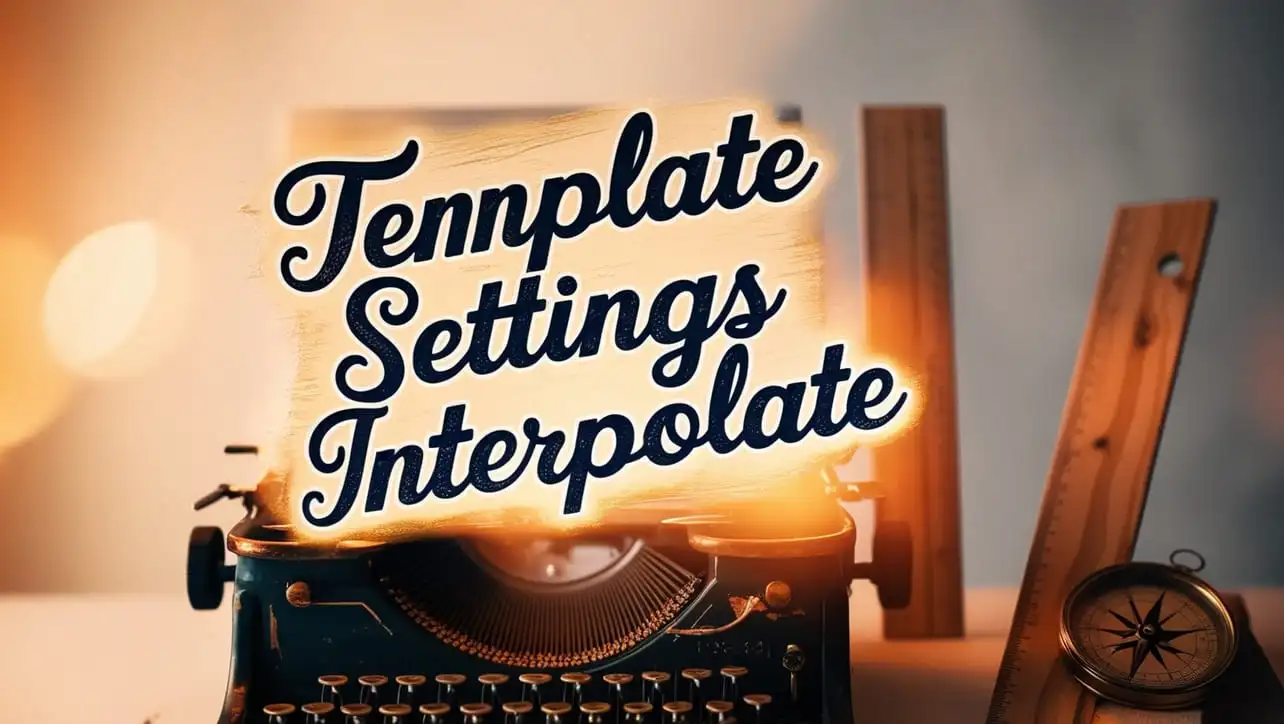
Lodash _.templateSettings.interpolate Property
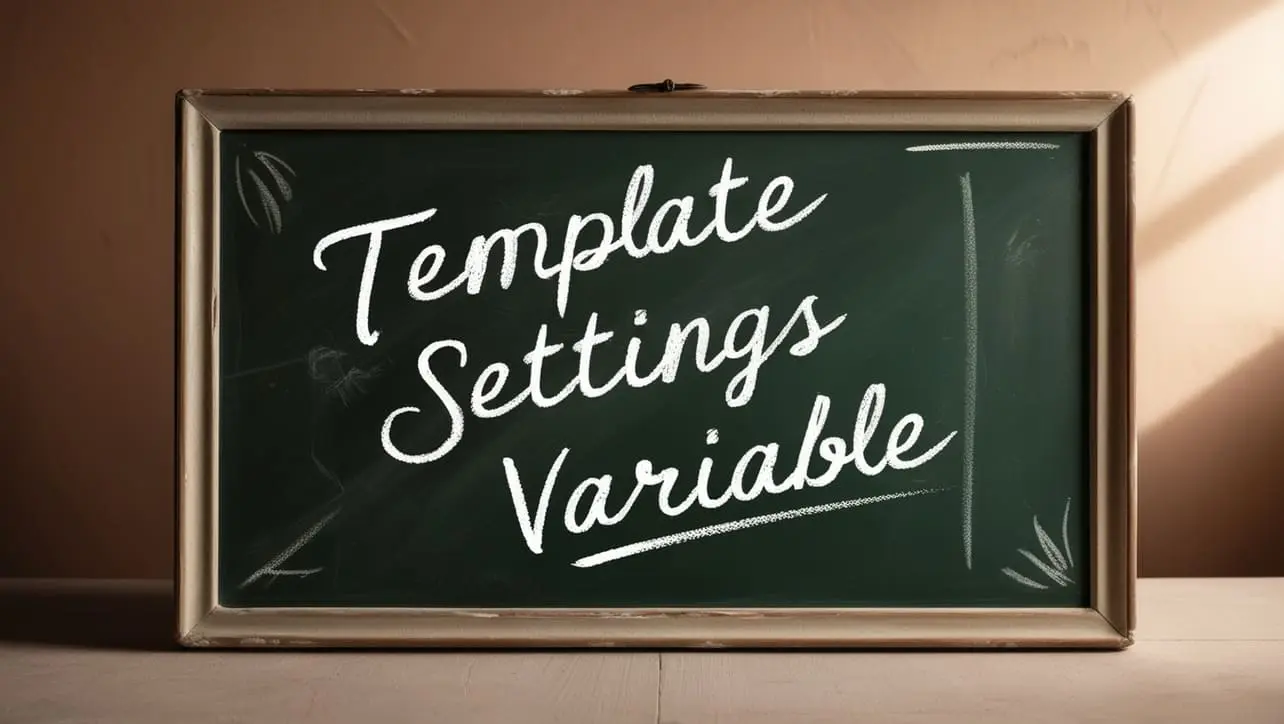
If you have any doubts regarding this article (Lodash _.identity() Util Method), please comment here. I will help you immediately.