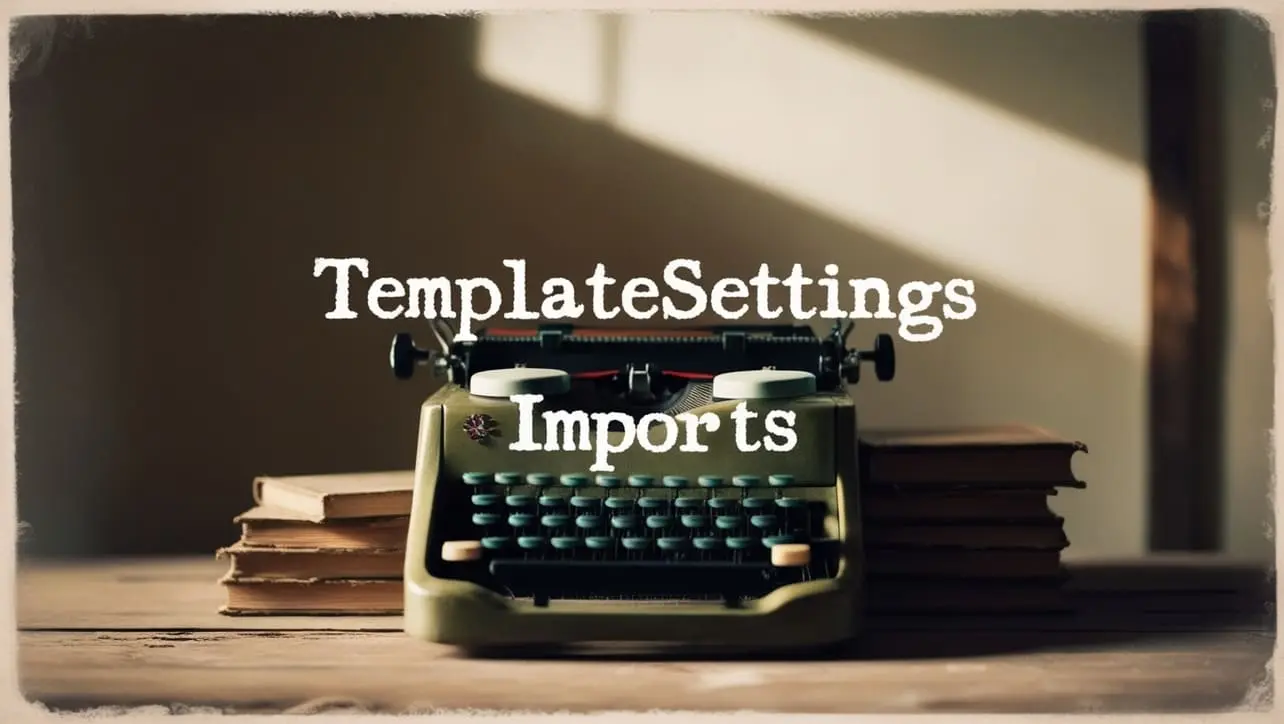
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- Lodash Util
- _.attempt
- _.bindAll
- _.cond
- _.conforms
- _.constant
- _.defaultTo
- _.flow
- _.flowRight
- _.identity
- _.iteratee
- _.matches
- _.matchesProperty
- _.method
- _.methodOf
- _.mixin
- _.noConflict
- _.noop
- _.nthArg
- _.over
- _.overEvery
- _.overSome
- _.property
- _.propertyOf
- _.range
- _.rangeRight
- _.runInContext
- _.stubArray
- _.stubFalse
- _.stubObject
- _.stubString
- _.stubTrue
- _.times
- _.toPath
- _.uniqueId
- Lodash Properties
- Lodash Methods
Lodash _.constant() Util Method
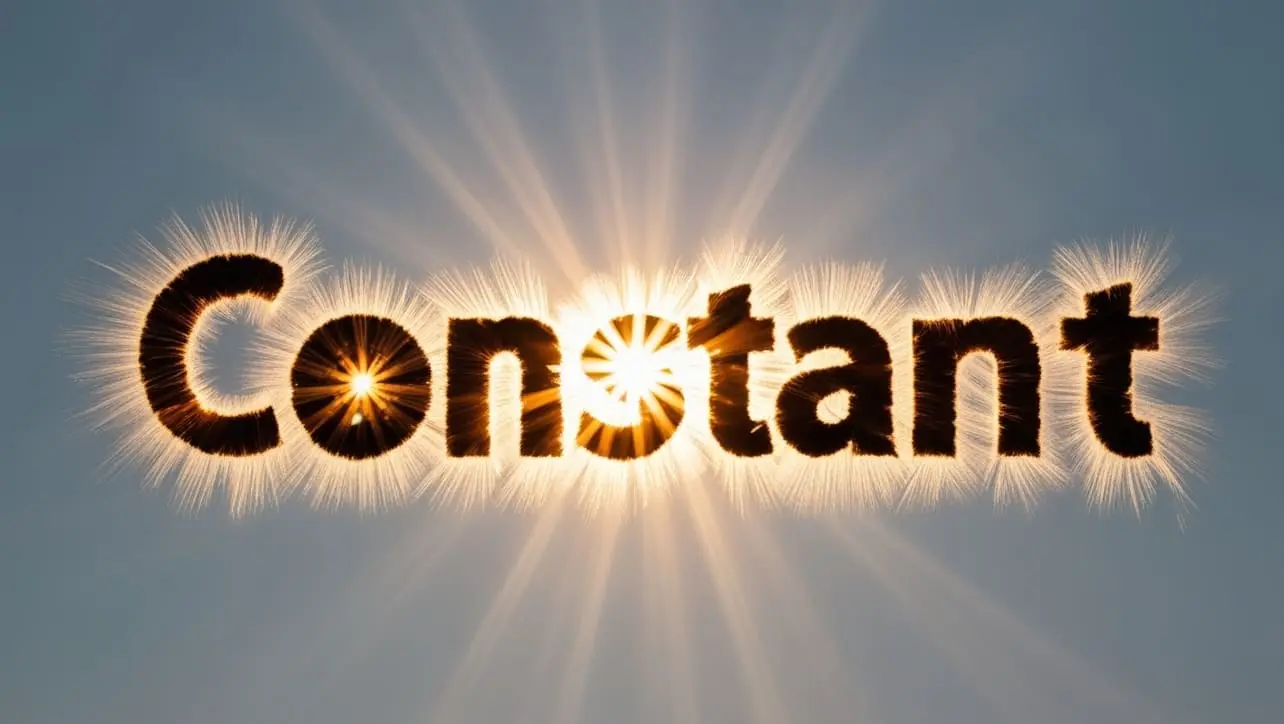
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, there are scenarios where you need to create functions that always return the same value regardless of their arguments. The Lodash utility library provides a convenient solution for this with the _.constant()
method.
This method creates a function that always returns the same value it was initialized with, simplifying code and enhancing readability.
🧠 Understanding _.constant() Method
The _.constant()
method in Lodash generates a function that returns the specified constant value. This function ignores any arguments passed to it and consistently returns the predetermined value.
💡 Syntax
The syntax for the _.constant()
method is straightforward:
_.constant(value)
- value: The constant value to be returned by the generated function.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.constant()
method:
const _ = require('lodash');
const always42 = _.constant(42);
console.log(always42()); // Output: 42
console.log(always42(100)); // Output: 42 (ignores arguments)
In this example, always42 is a function generated by _.constant(42), which always returns the value 42, regardless of any arguments passed to it.
🏆 Best Practices
When working with the _.constant()
method, consider the following best practices:
Creating Constant Functions:
Use
_.constant()
to create functions that consistently return a predetermined value. This is particularly useful for creating simple, reusable functions.example.jsCopiedconst alwaysHello = _.constant('Hello'); console.log(alwaysHello()); // Output: 'Hello' console.log(alwaysHello(123)); // Output: 'Hello' (ignores arguments)
Simplifying Conditional Logic:
Simplify conditional logic by replacing complex conditions with constant functions. This improves code readability and maintainability.
example.jsCopiedconst isAdult = _.constant(true); // Assume everyone is an adult for simplicity if(isAdult()) { console.log('Welcome to the club!'); } else { console.log('Sorry, adults only.'); }
Creating Default Values:
Use
_.constant()
to create default values for function parameters or object properties. This ensures consistency and reduces the likelihood of unexpected behavior.example.jsCopiedfunction greet(name = _.constant('Guest')) { console.log(`Hello, ${name()}!`); } greet(); // Output: 'Hello, Guest!' greet(_.constant('John')); // Output: 'Hello, John!'
📚 Use Cases
Default Values in Functions:
_.constant()
is handy for providing default values in functions, ensuring consistent behavior when no arguments are provided.example.jsCopiedfunction fetchData(callback = _.constant({})) { // Simulated asynchronous operation setTimeout(() => { const data = /* ...fetch data from server... */ ; callback(data); }, 1000); } fetchData(); // Returns an empty object if no callback is provided
Mocking Functions in Tests:
When writing tests,
_.constant()
can be used to create mock functions that consistently return specific values, simplifying test setup.example.jsCopiedconst mockApiCall = _.constant({ success: true, data: 'Mock data' }); // Test function that makes use of the mock API call function testFunction(callback = mockApiCall) { // Function logic that uses the callback } // Test cases console.log(testFunction()); // Output: { success: true, data: 'Mock data' }
Immutable Object Properties:
When defining immutable object properties,
_.constant()
ensures that the property always holds the same value.example.jsCopiedconst immutableObject = { PI: _.constant(3.14159), gravity: _.constant(9.81), }; console.log(immutableObject.PI()); // Output: 3.14159 console.log(immutableObject.gravity()); // Output: 9.81
🎉 Conclusion
The _.constant()
method in Lodash is a versatile tool for creating functions that consistently return a specified value. Whether you're simplifying conditional logic, providing default values, or creating immutable object properties, _.constant()
enhances code readability and maintainability in JavaScript development.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.constant()
method in your Lodash projects.
👨💻 Join our Community:
Author
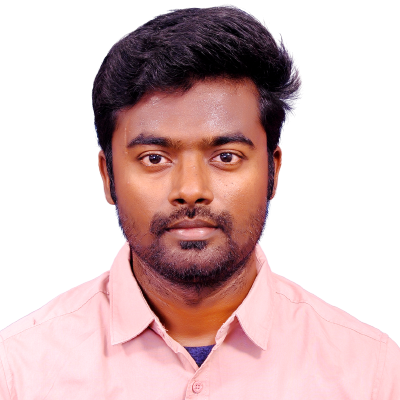
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
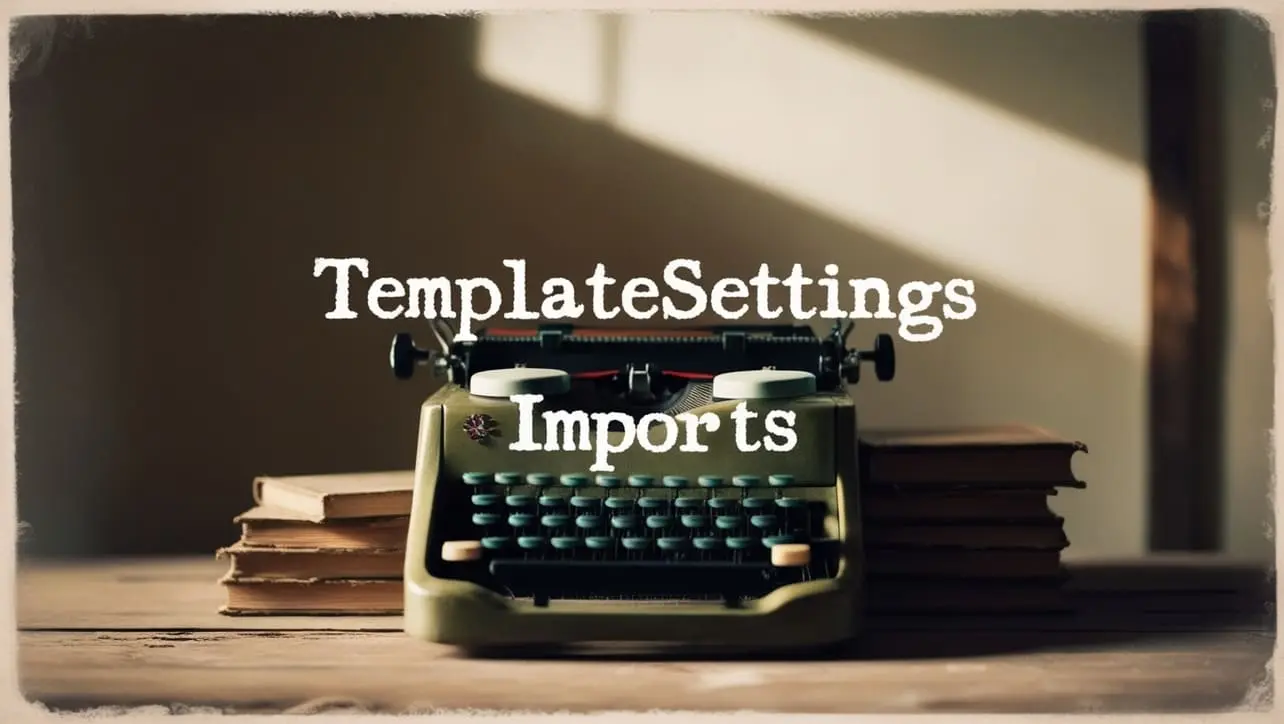
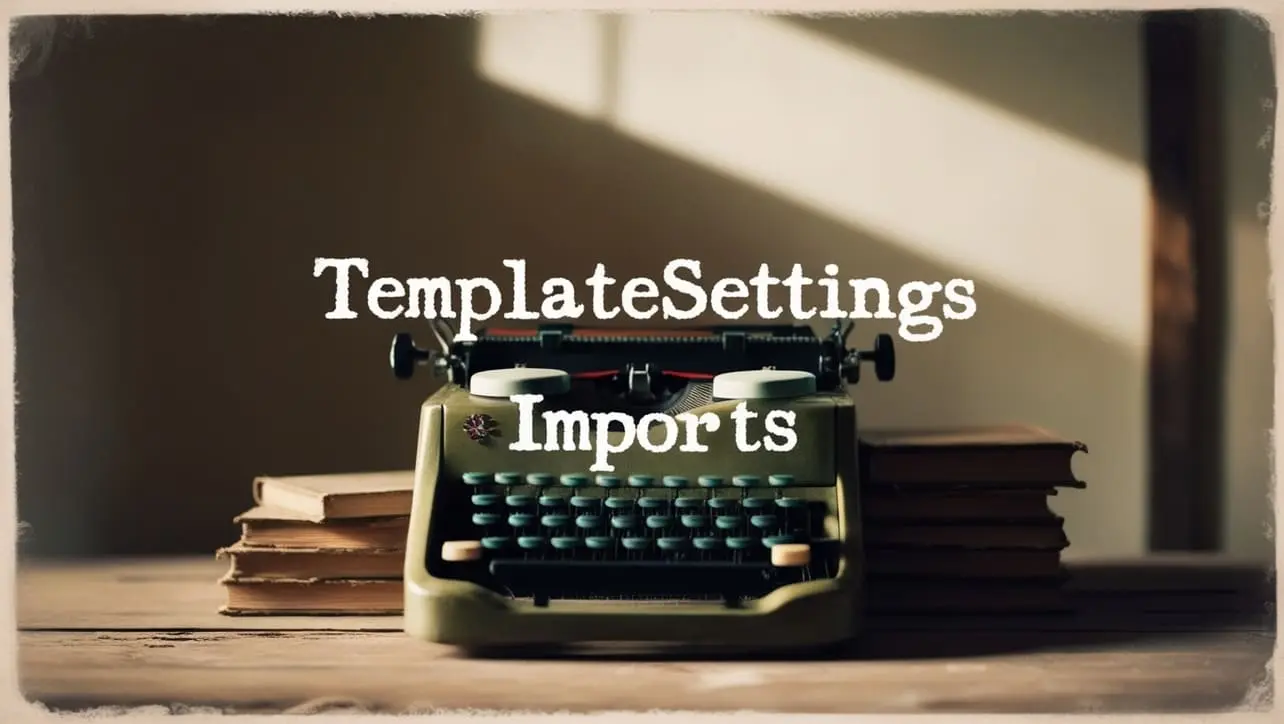
Lodash _.templateSettings.imports Property
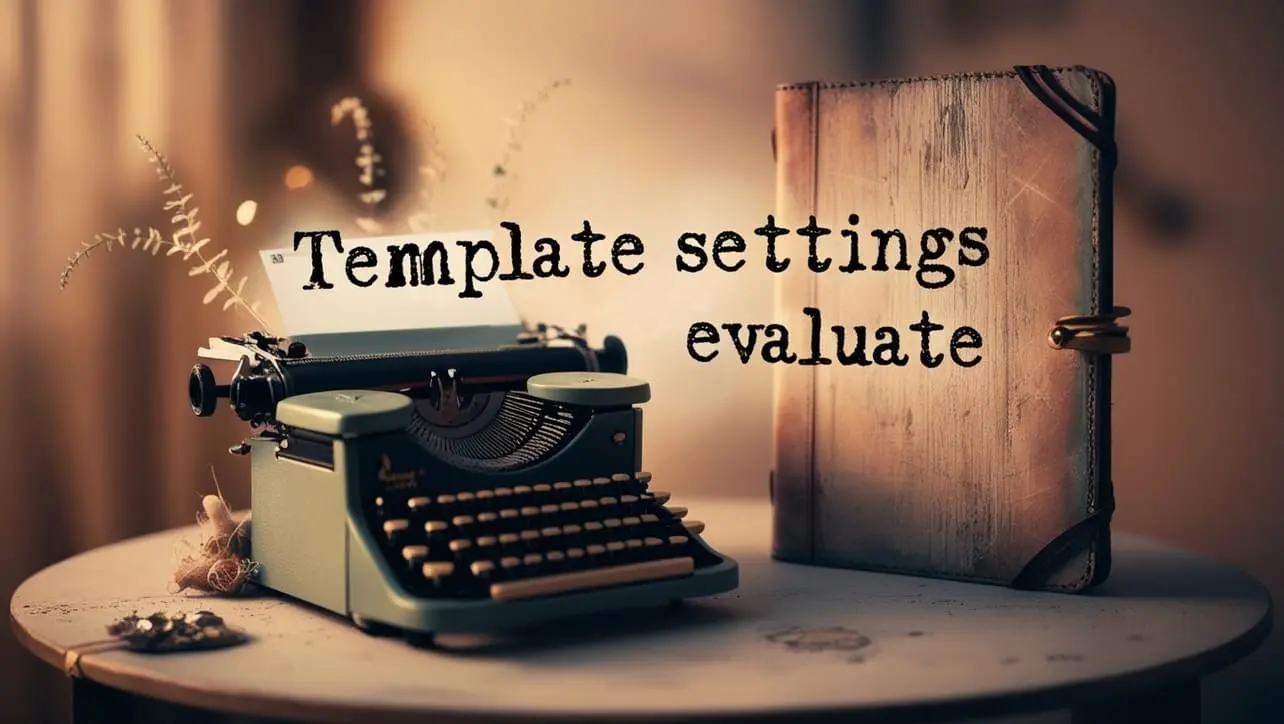
Lodash _.templateSettings.evaluate Property
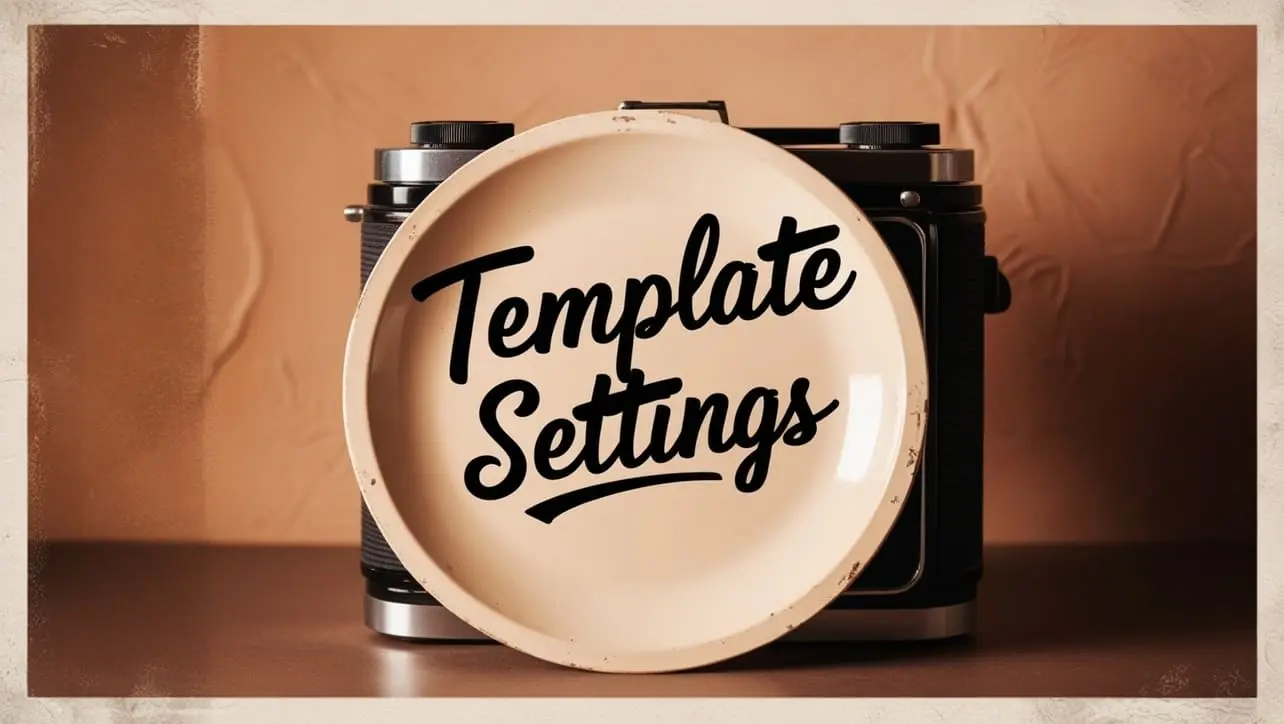
Lodash _.templateSettings Property
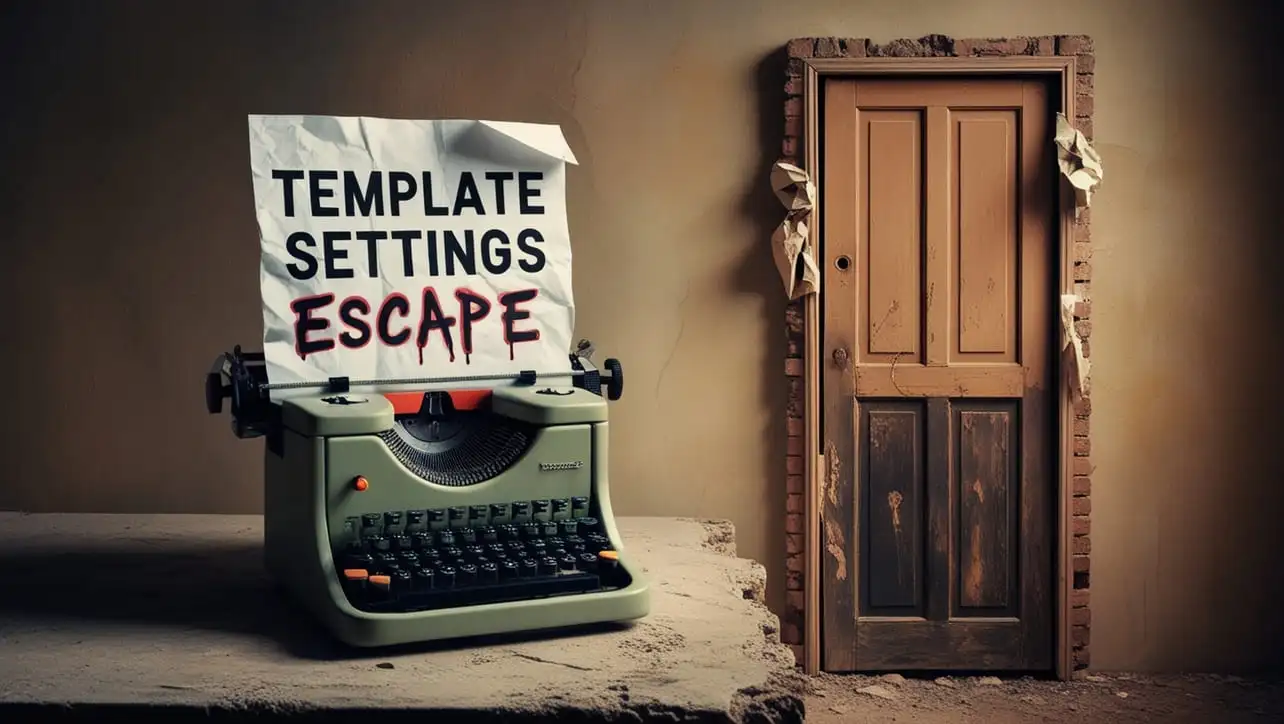
Lodash _.templateSettings.escape Property
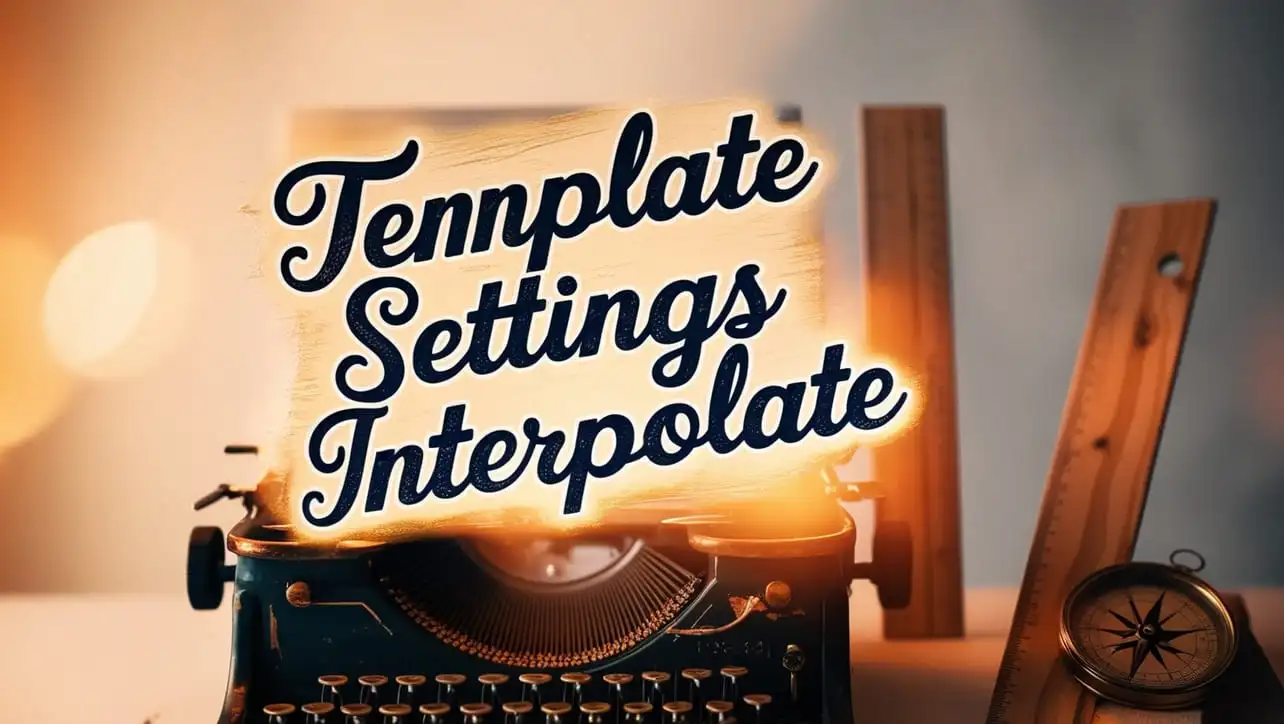
Lodash _.templateSettings.interpolate Property
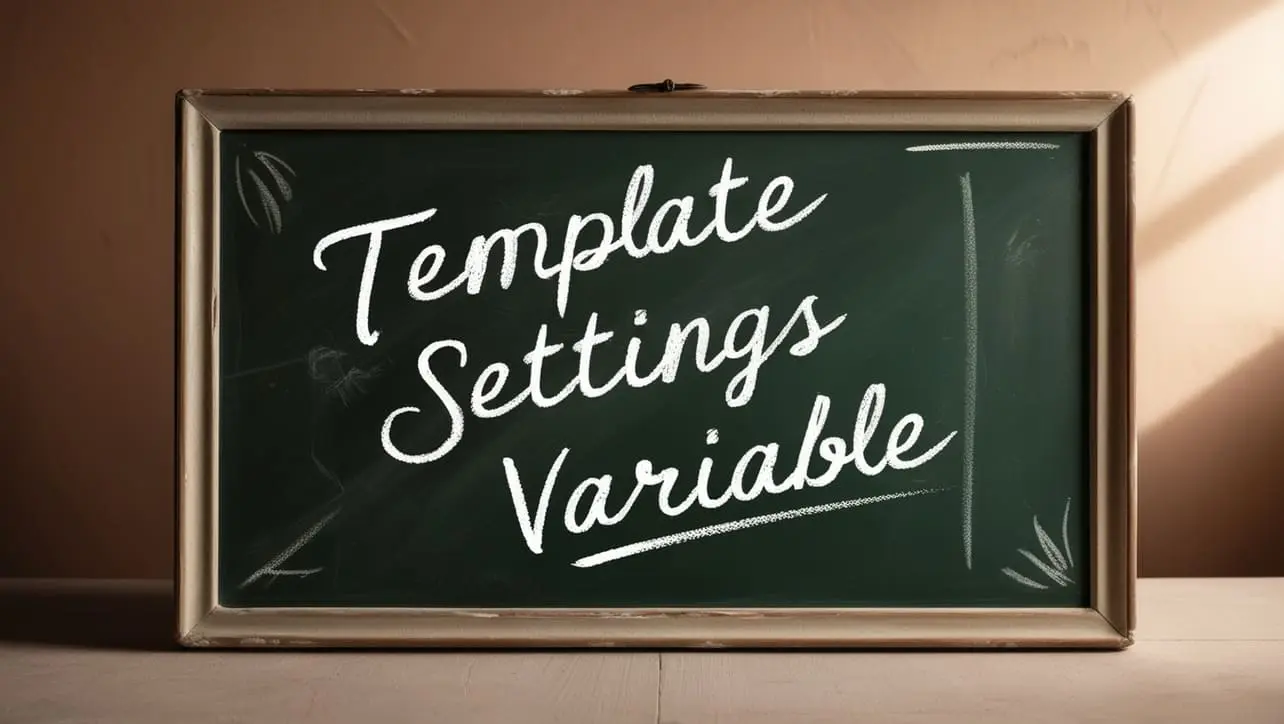
If you have any doubts regarding this article (Lodash _.constant() Util Method), please comment here. I will help you immediately.