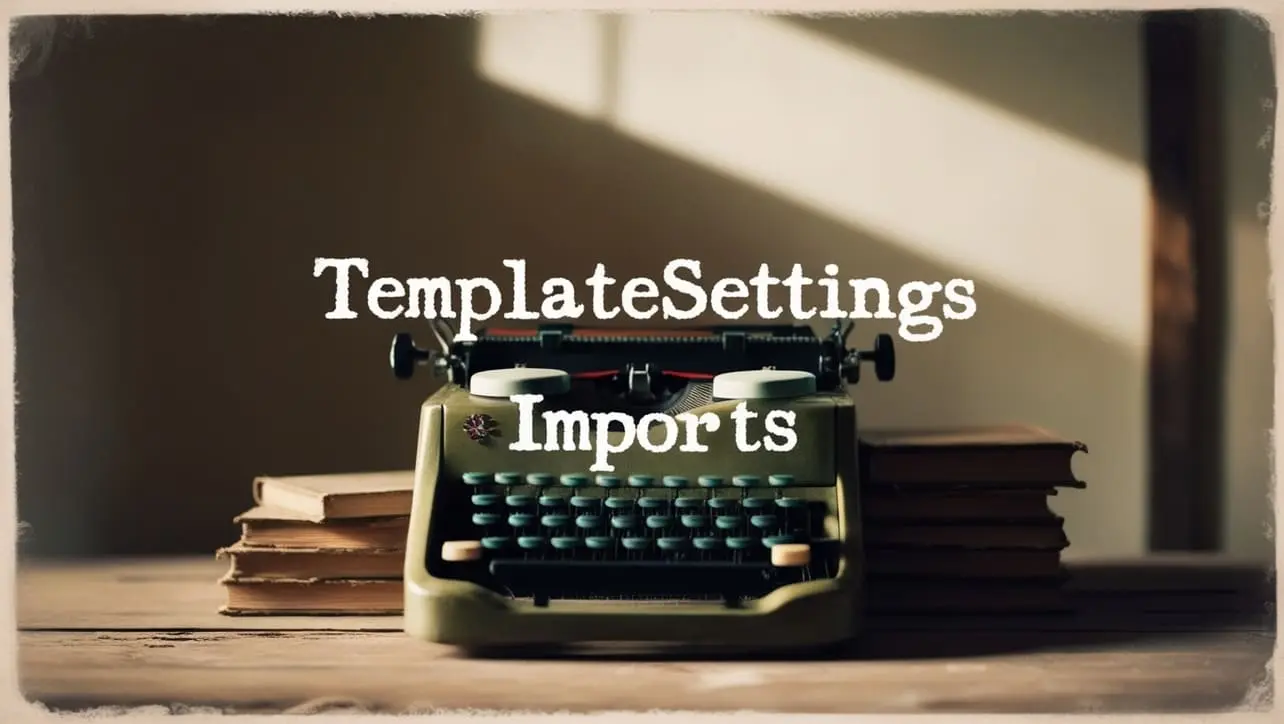
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.replace() String Method
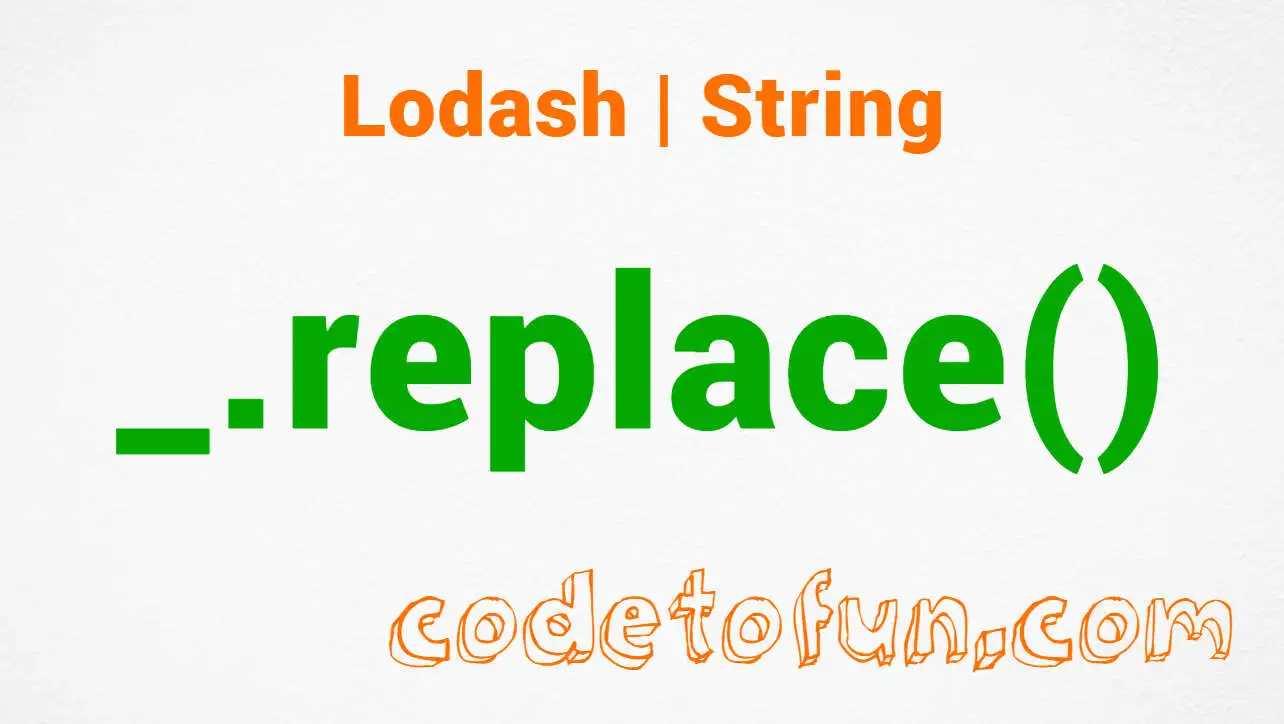
Photo Credit to CodeToFun
🙋 Introduction
Manipulating strings is a common task in JavaScript programming, and the Lodash library provides numerous utility functions to streamline these operations. Among them is the _.replace()
method, which allows developers to easily replace occurrences of a specified pattern within a string.
This method offers flexibility and convenience, making it a valuable tool for string manipulation tasks.
🧠 Understanding _.replace() Method
The _.replace()
method in Lodash is used to search for a specified pattern within a string and replace all occurrences with a replacement string or function. This provides a straightforward approach to string modification, enabling developers to perform complex replacements with ease.
💡 Syntax
The syntax for the _.replace()
method is straightforward:
_.replace(string, pattern, replacement)
- string: The string to modify.
- pattern: The pattern to search for.
- replacement: The replacement string or function.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.replace()
method:
const _ = require('lodash');
const originalString = 'Hello world, hello universe!';
const replacedString = _.replace(originalString, 'hello', 'hi');
console.log(replacedString);
// Output: 'Hello world, hi universe!'
In this example, the originalString is modified using _.replace()
to replace all occurrences of 'hello' with 'hi'.
🏆 Best Practices
When working with the _.replace()
method, consider the following best practices:
Handling Case Sensitivity:
Be mindful of case sensitivity when using
_.replace()
. By default, it performs case-sensitive replacements. Use appropriate flags or options to control case sensitivity based on your requirements.example.jsCopiedconst originalString = 'Hello world, hello universe!'; const replacedString = _.replace(originalString, /hello/i, 'hi'); // Perform case-insensitive replacement console.log(replacedString);
Regular Expressions:
Utilize regular expressions for more complex pattern matching and replacements. Regular expressions provide powerful capabilities for string manipulation, allowing for versatile and precise replacements.
example.jsCopiedconst originalString = 'The quick brown fox jumps over the lazy dog.'; const replacedString = _.replace(originalString, /\b\w{5}\b/g, '*****'); // Replace 5-letter words with asterisks console.log(replacedString);
Dynamic Replacements:
Take advantage of dynamic replacements using functions. Instead of providing a static replacement string, you can use a function to dynamically generate replacement values based on matched patterns.
example.jsCopiedconst originalString = 'Hello John, hello Jane, hello Tom!'; const replacedString = _.replace(originalString, /hello/g, match => match.toUpperCase()); console.log(replacedString);
📚 Use Cases
Text Formatting:
_.replace()
is commonly used for text formatting tasks, such as replacing placeholders with dynamic values or standardizing text formats.example.jsCopiedconst template = 'Hello, {name}! Welcome to {place}.'; const data = { name: 'Alice', place: 'Wonderland' }; const formattedString = _.replace(template, /{([^}]+)}/g, (match, key) => data[key]); console.log(formattedString);
Data Sanitization:
In scenarios where data sanitization is necessary,
_.replace()
can be employed to remove or replace sensitive information, such as personally identifiable information (PII) or special characters.example.jsCopiedconst sensitiveData = 'User: John Doe, SSN: 123-45-6789'; const sanitizedData = _.replace(sensitiveData, /\b\d{3}-\d{2}-\d{4}\b/g, '***-**-****'); console.log(sanitizedData);
URL Rewriting:
For tasks involving URL manipulation,
_.replace()
can be utilized to rewrite URLs or replace specific path segments.example.jsCopiedconst originalUrl = 'https://example.com/users/12345'; const rewrittenUrl = _.replace(originalUrl, /\/users\/\d+/, '/profile'); console.log(rewrittenUrl);
🎉 Conclusion
The _.replace()
method in Lodash provides a convenient and powerful solution for string replacement tasks in JavaScript. Whether you're performing simple text substitutions or complex pattern matching, this method offers the flexibility and versatility needed for effective string manipulation.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.replace()
method in your Lodash projects.
👨💻 Join our Community:
Author
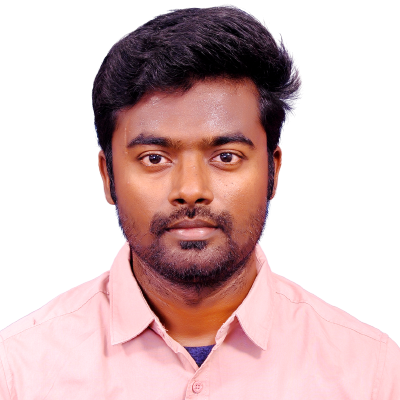
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
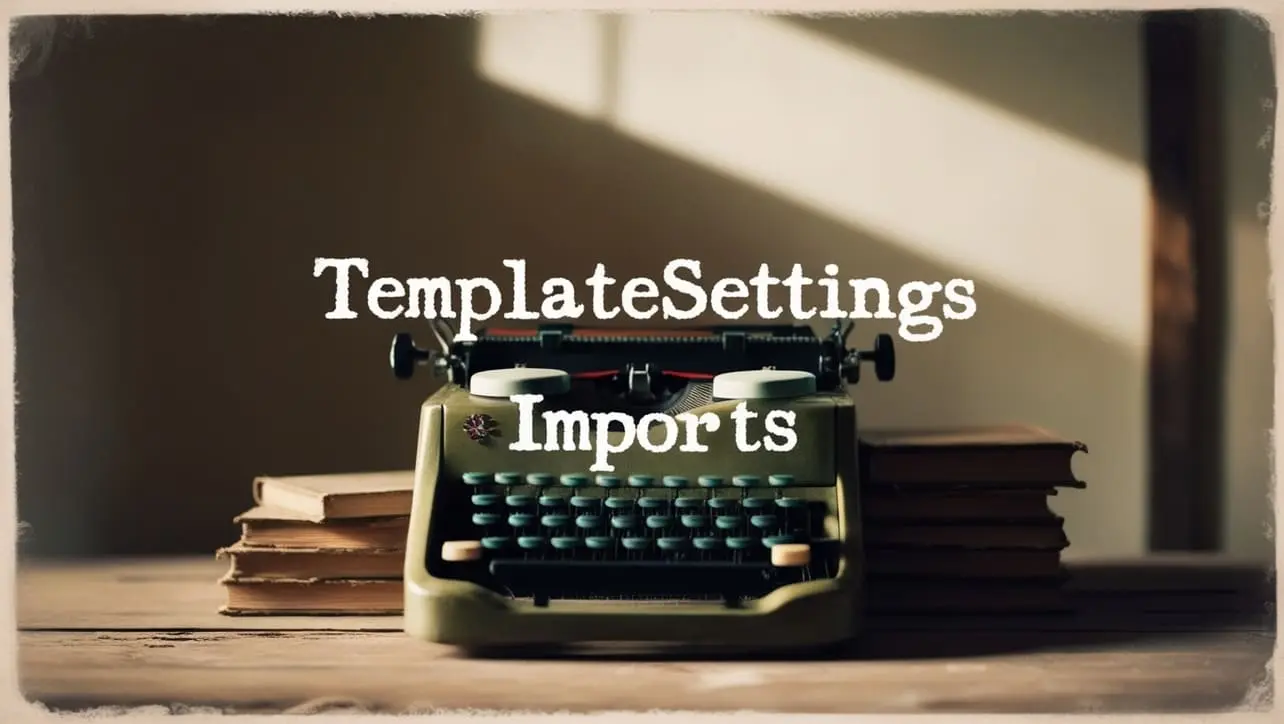
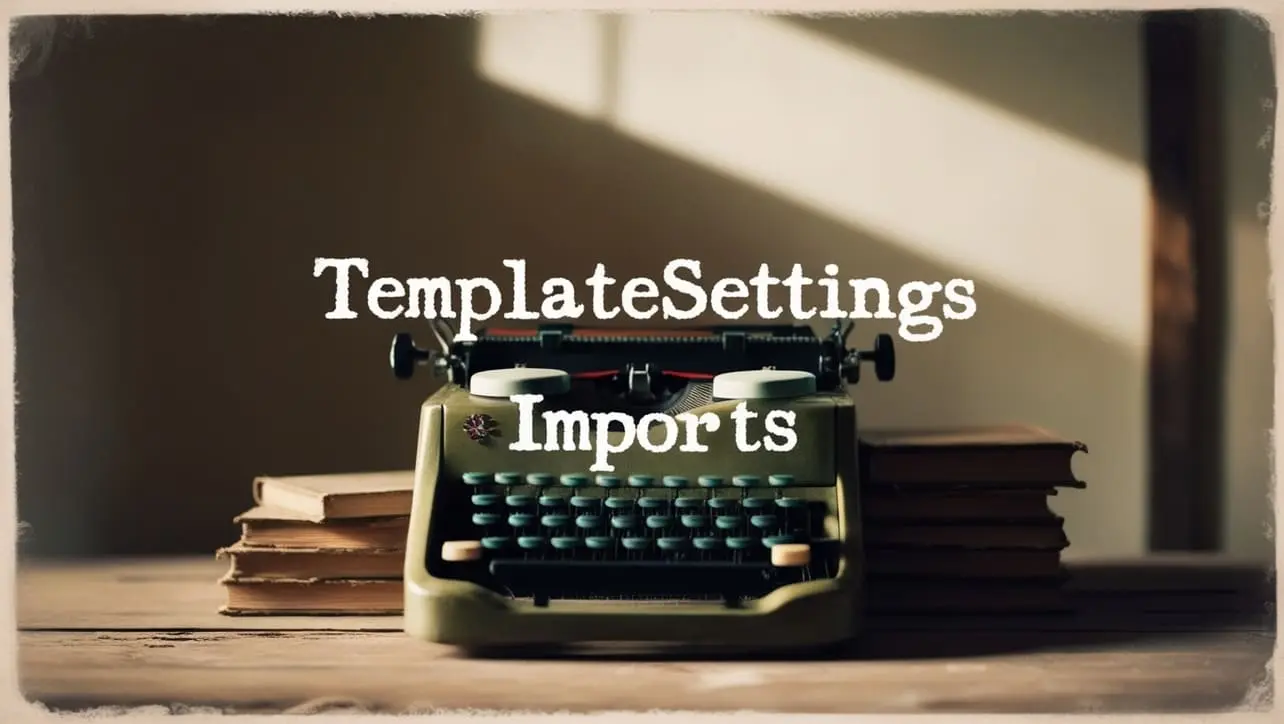
Lodash _.templateSettings.imports Property
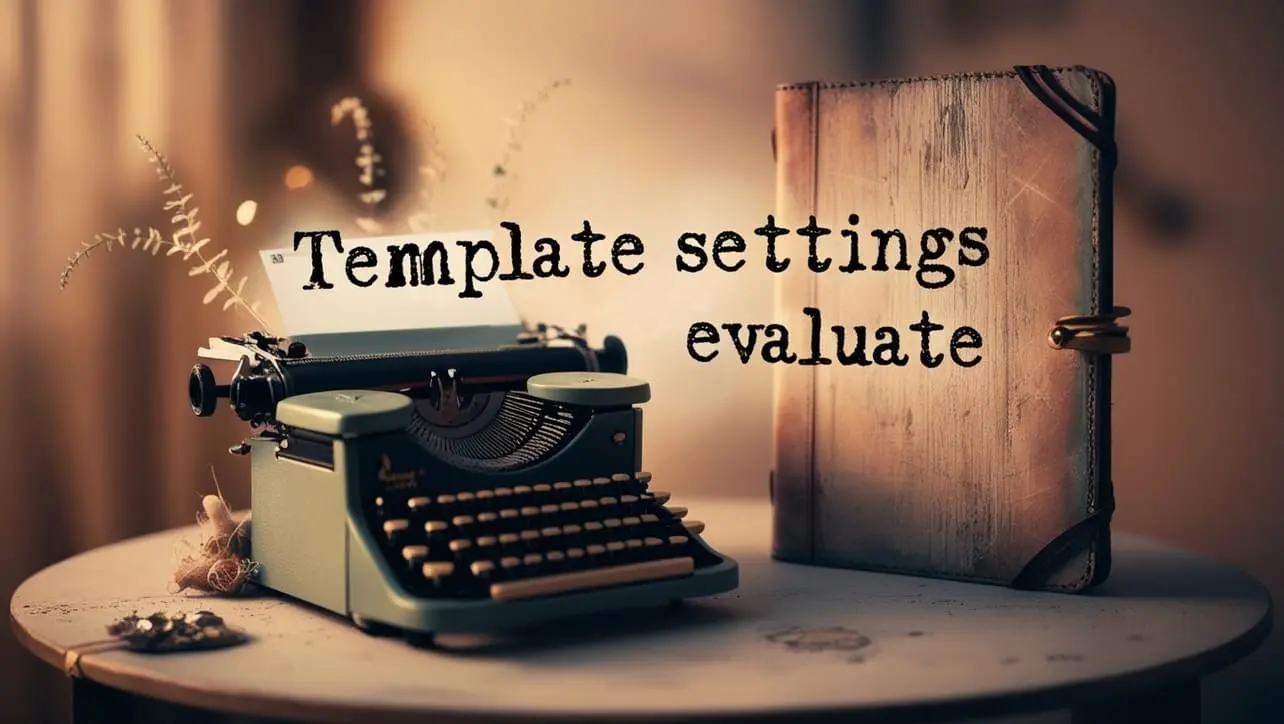
Lodash _.templateSettings.evaluate Property
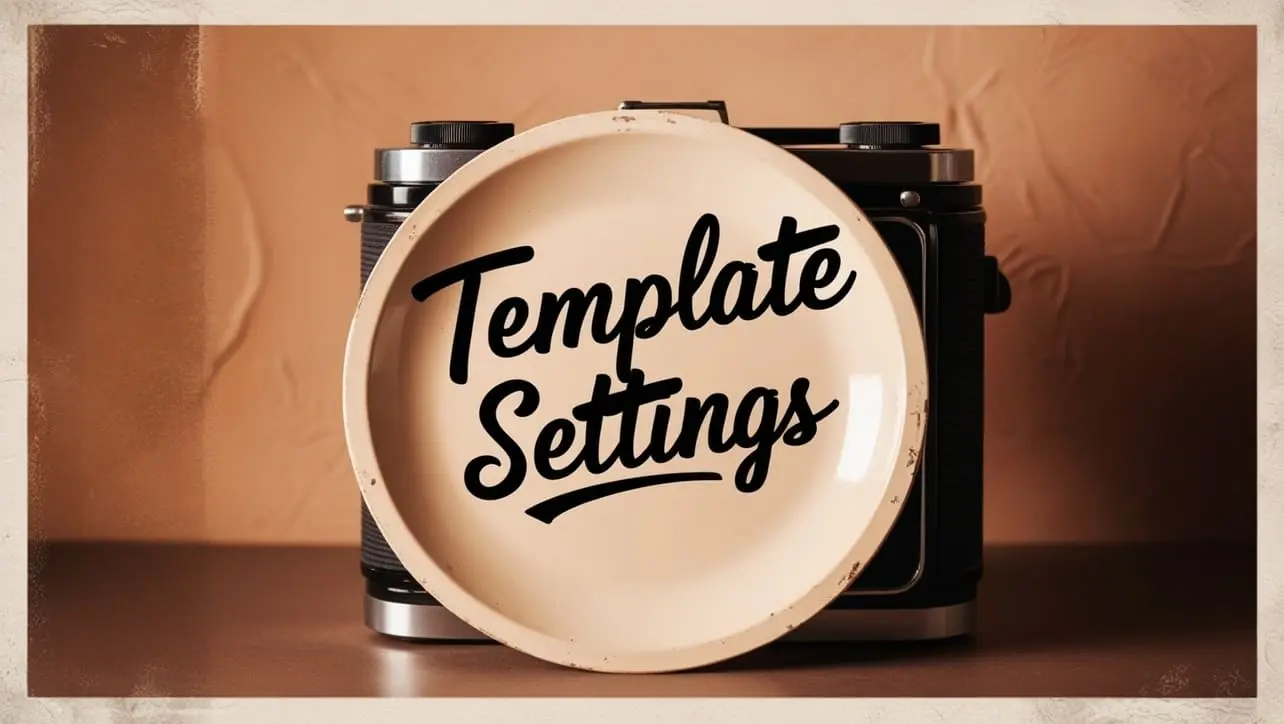
Lodash _.templateSettings Property
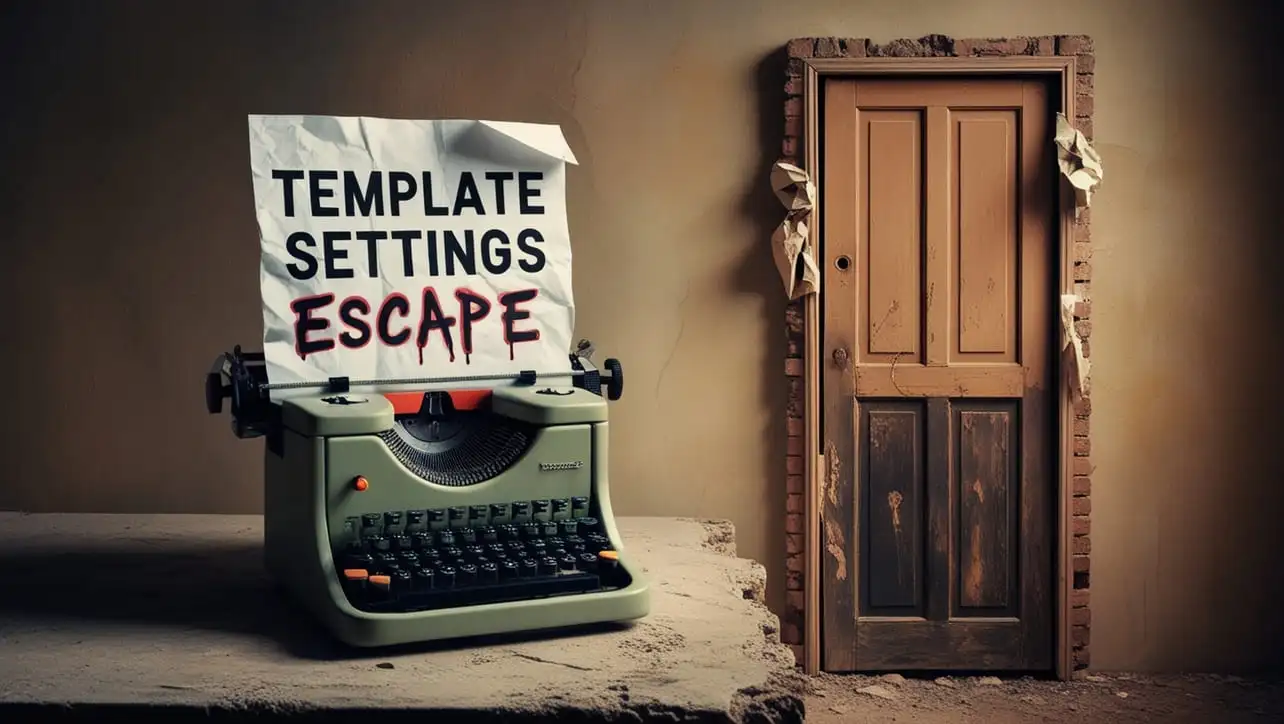
Lodash _.templateSettings.escape Property
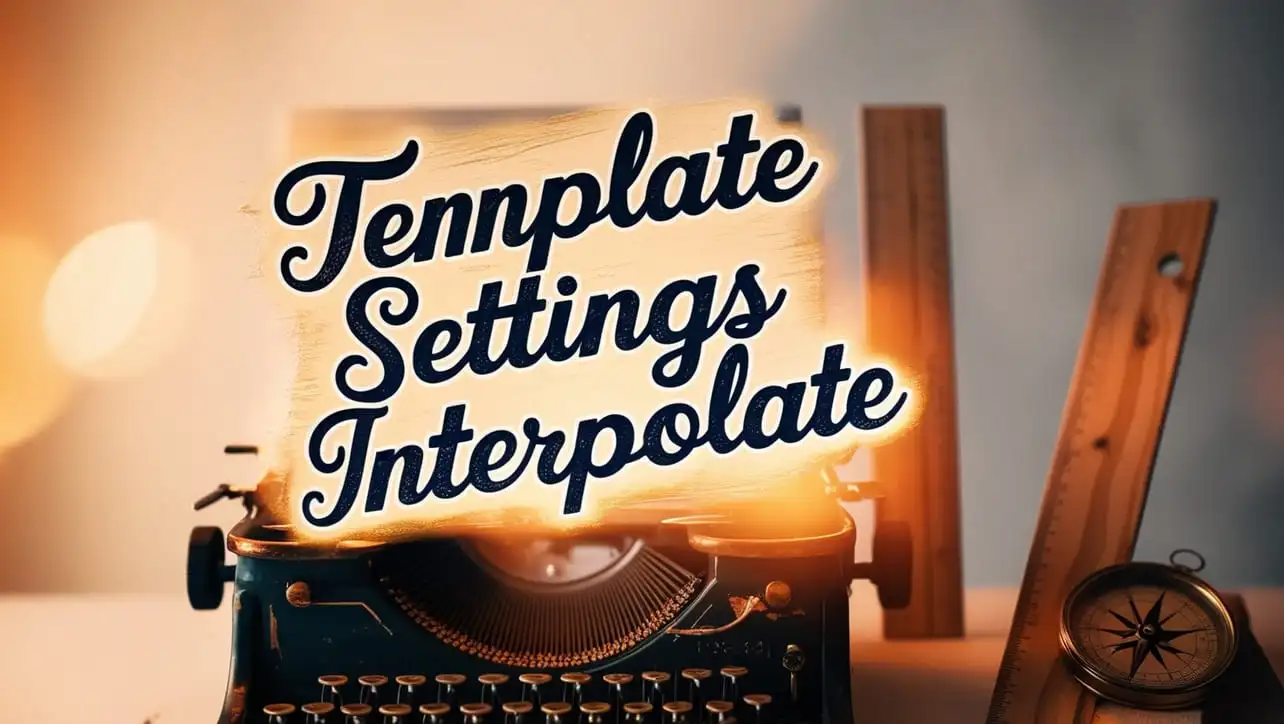
Lodash _.templateSettings.interpolate Property
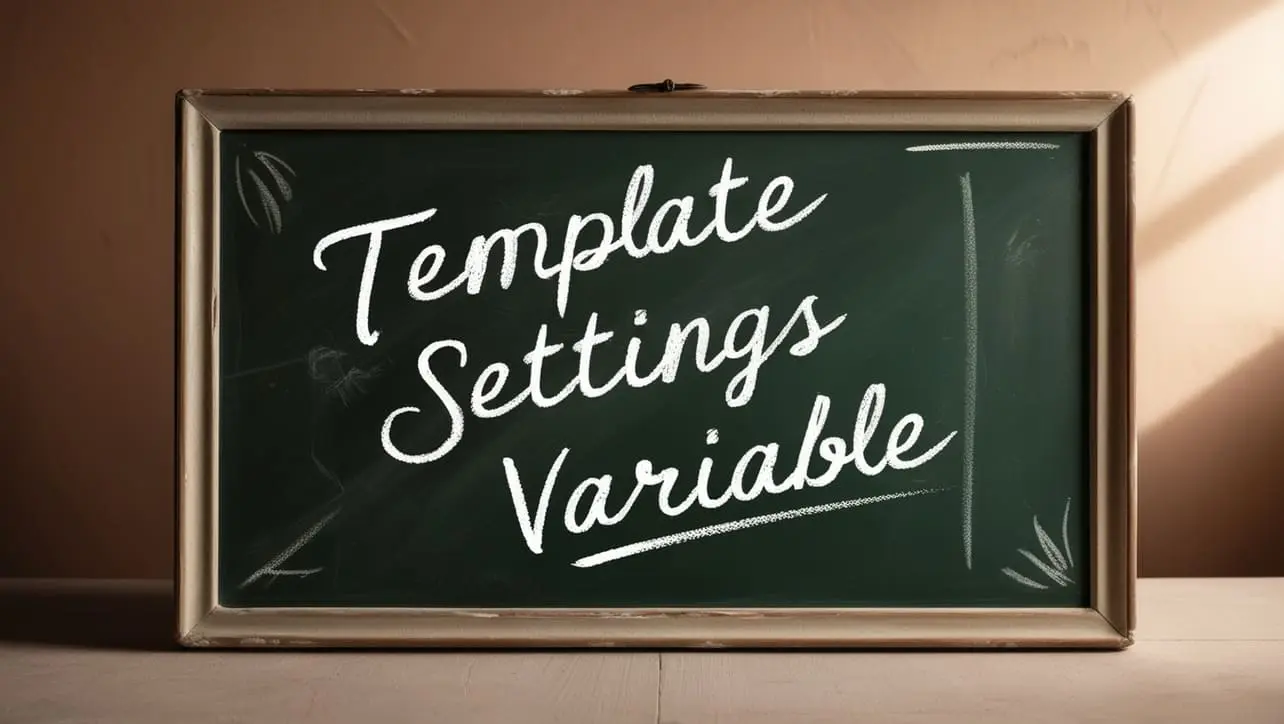
If you have any doubts regarding this article (Lodash _.replace() String Method), please comment here. I will help you immediately.