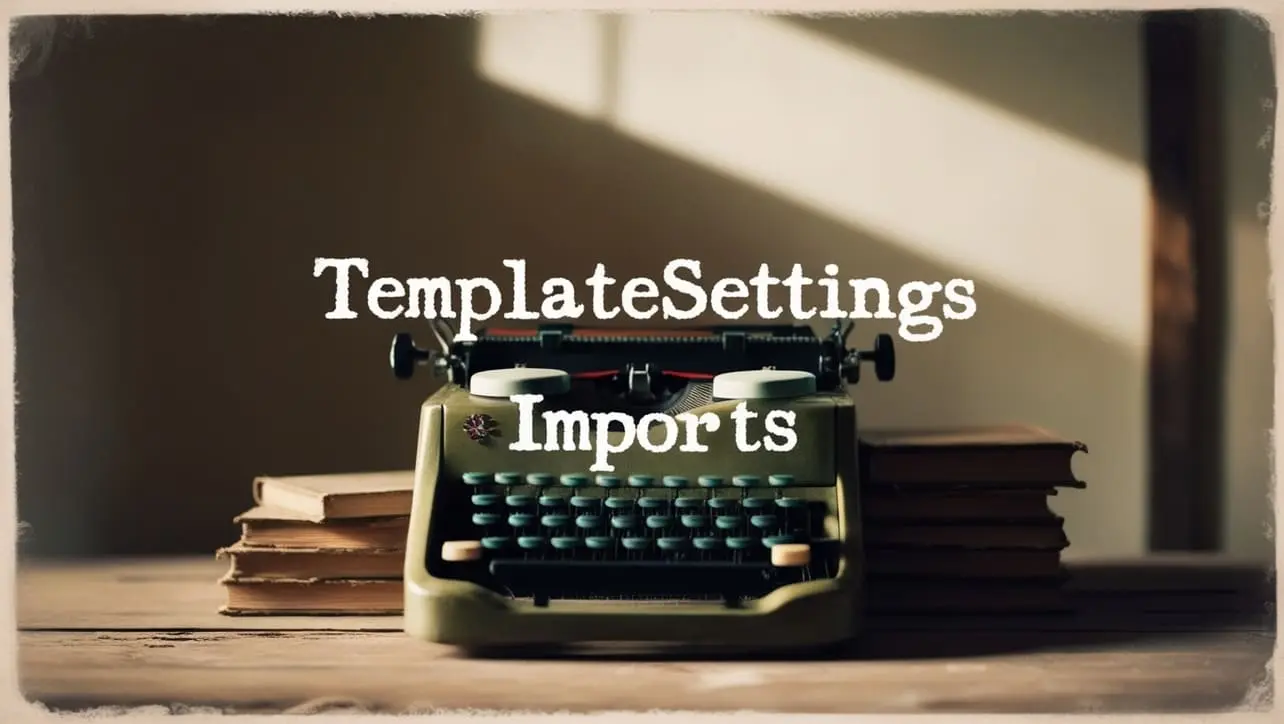
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.lowerFirst() String Method
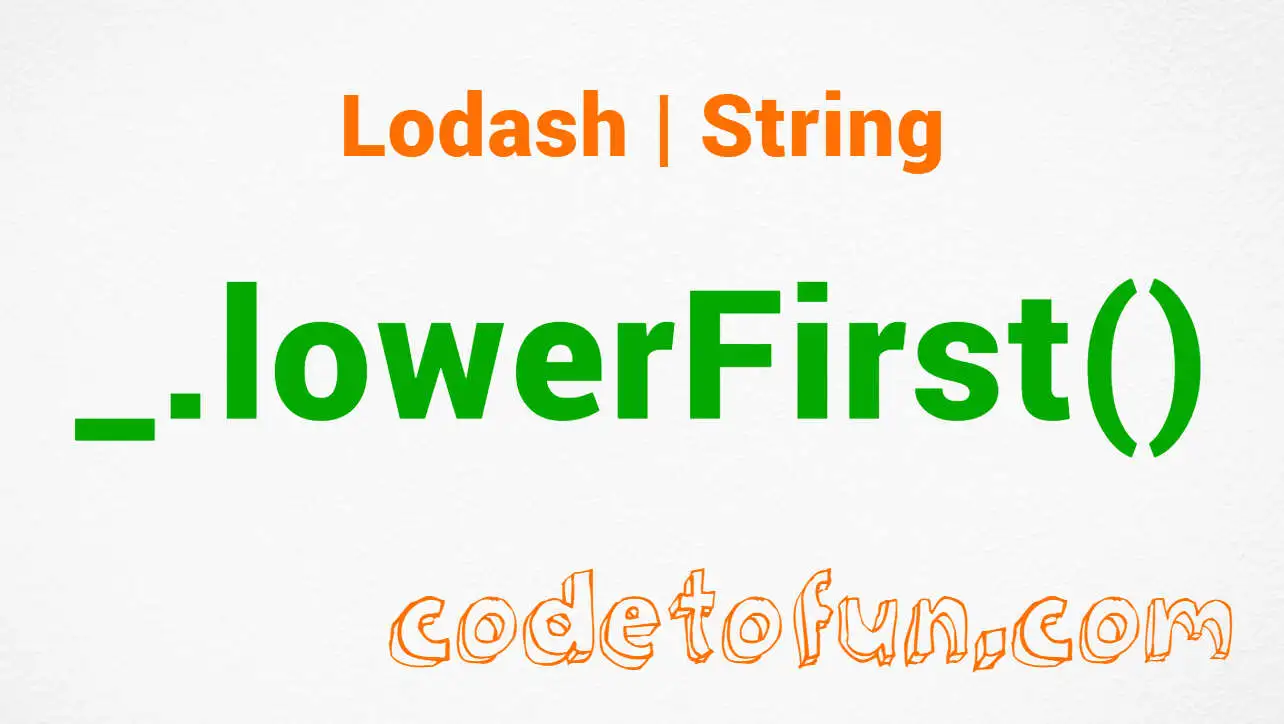
Photo Credit to CodeToFun
🙋 Introduction
String manipulation is a common task in JavaScript development, and having tools to streamline this process can greatly improve code readability and efficiency. The Lodash library provides a wide range of utility functions, including the _.lowerFirst()
method, which allows developers to convert the first character of a string to lowercase.
This method is particularly useful when working with strings where consistent casing is important.
🧠 Understanding _.lowerFirst() Method
The _.lowerFirst()
method in Lodash takes a string as input and converts the first character to lowercase, returning the modified string. This simple yet powerful functionality can be employed in various scenarios, such as formatting text or ensuring consistent capitalization.
💡 Syntax
The syntax for the _.lowerFirst()
method is straightforward:
_.lowerFirst(string)
- string: The string to process.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.lowerFirst()
method:
const _ = require('lodash');
const originalString = 'HelloWorld';
const lowerFirstString = _.lowerFirst(originalString);
console.log(lowerFirstString);
// Output: 'helloWorld'
In this example, the originalString is processed by _.lowerFirst()
, resulting in a new string where the first character is converted to lowercase.
🏆 Best Practices
When working with the _.lowerFirst()
method, consider the following best practices:
Consistent Casing:
Ensure consistent casing throughout your application by utilizing
_.lowerFirst()
where necessary. This helps maintain readability and consistency in user interfaces and data representations.example.jsCopiedconst inputString = 'UserName'; const formattedString = _.lowerFirst(inputString); console.log(formattedString); // Output: 'userName'
Handling User Input:
When dealing with user input,
_.lowerFirst()
can be used to standardize the formatting of strings, ensuring that the first character is always lowercase regardless of user input.example.jsCopiedconst userInput = 'jOhN'; const standardizedInput = _.lowerFirst(userInput); console.log(standardizedInput); // Output: 'jOhN' -> 'jOhN' (first character remains lowercase)
String Concatenation:
In scenarios where string concatenation is involved,
_.lowerFirst()
can be employed to ensure proper casing of concatenated strings, resulting in a consistent and aesthetically pleasing output.example.jsCopiedconst firstName = 'john'; const lastName = 'doe'; const fullName = _.lowerFirst(firstName) + ' ' + _.lowerFirst(lastName); console.log(fullName); // Output: 'john doe'
📚 Use Cases
Formatting Text:
_.lowerFirst()
is ideal for formatting text displayed in user interfaces, ensuring that headings, labels, and other text elements begin with a lowercase character for a polished appearance.example.jsCopiedconst heading = 'ContactUs'; const formattedHeading = _.lowerFirst(heading); console.log(formattedHeading); // Output: 'contactUs'
Data Transformation:
When transforming data,
_.lowerFirst()
can be applied to ensure consistency in data representation, making it easier to work with and understand.example.jsCopiedconst data = ['FirstName', 'LastName', 'EmailAddress']; const transformedData = data.map(_.lowerFirst); console.log(transformedData); // Output: ['firstName', 'lastName', 'emailAddress']
API Integration:
When integrating with external APIs that expect specific casing conventions,
_.lowerFirst()
can be used to format data according to those conventions, ensuring seamless communication.example.jsCopiedconst requestData = { UserName: 'john_doe', EmailAddress: 'john@example.com', }; const formattedRequestData = _.mapKeys(requestData, (_, key) => _.lowerFirst(key)); console.log(formattedRequestData); // Output: { userName: 'john_doe', emailAddress: 'john@example.com' }
🎉 Conclusion
The _.lowerFirst()
method in Lodash provides a convenient solution for converting the first character of a string to lowercase. Whether you're formatting text, transforming data, or integrating with external systems, this method offers a versatile tool for consistent string manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.lowerFirst()
method in your Lodash projects.
👨💻 Join our Community:
Author
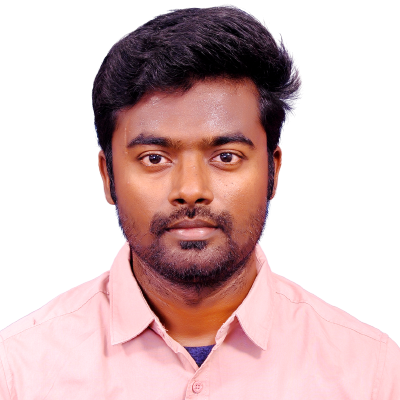
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
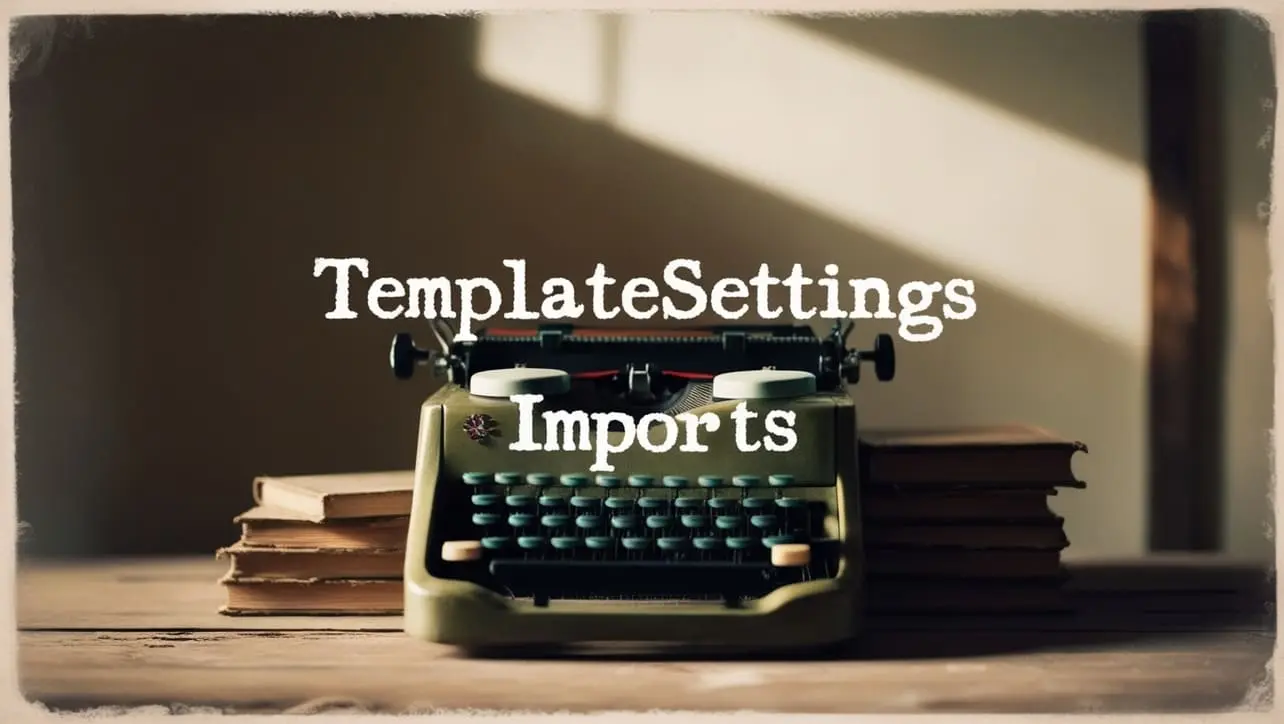
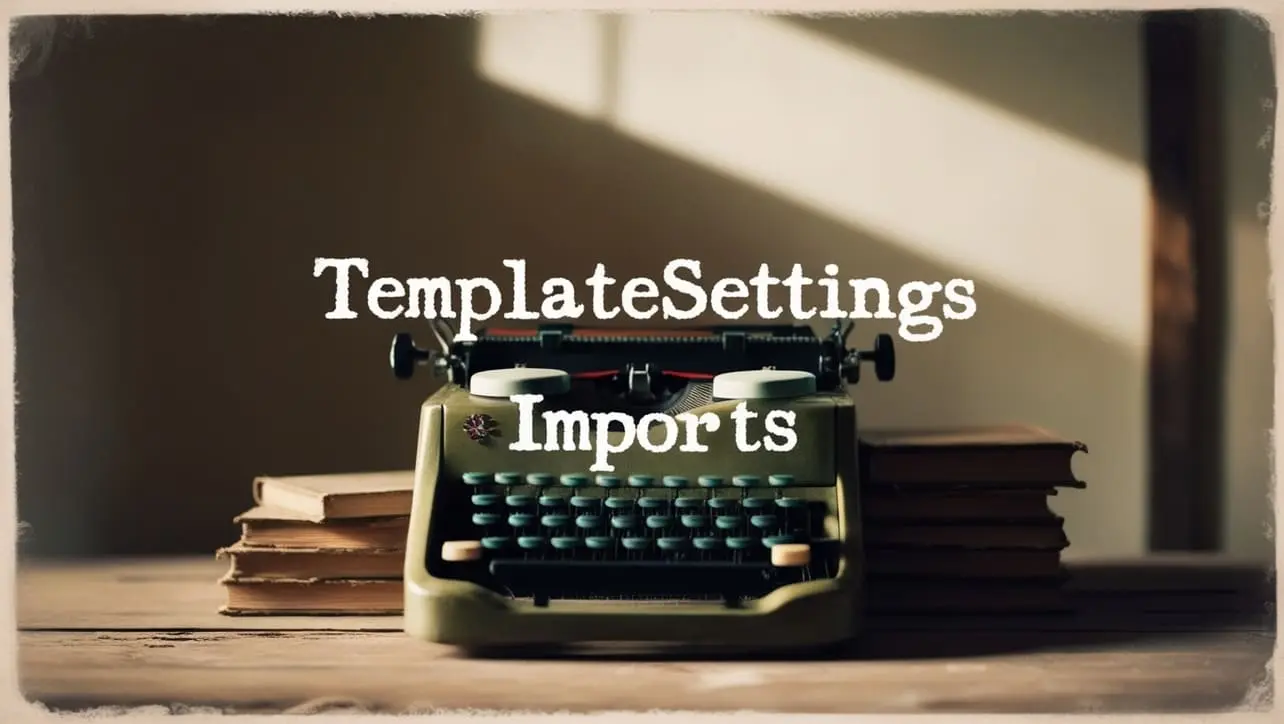
Lodash _.templateSettings.imports Property
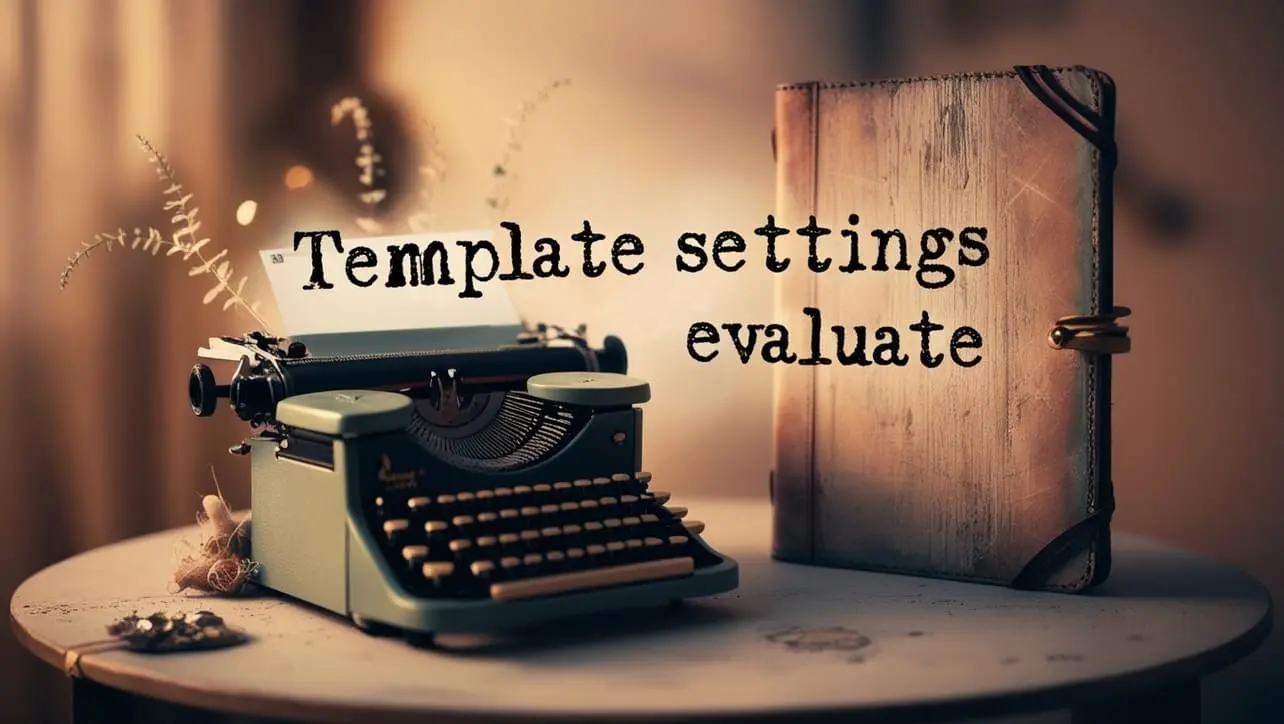
Lodash _.templateSettings.evaluate Property
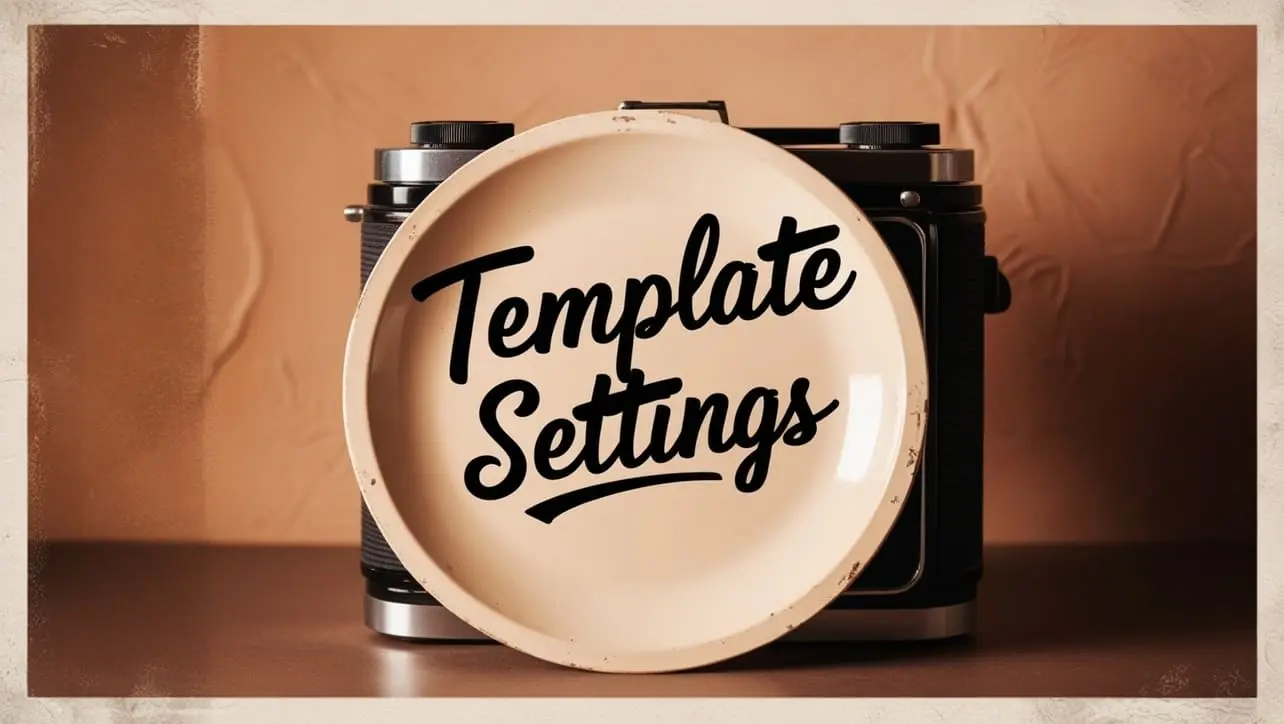
Lodash _.templateSettings Property
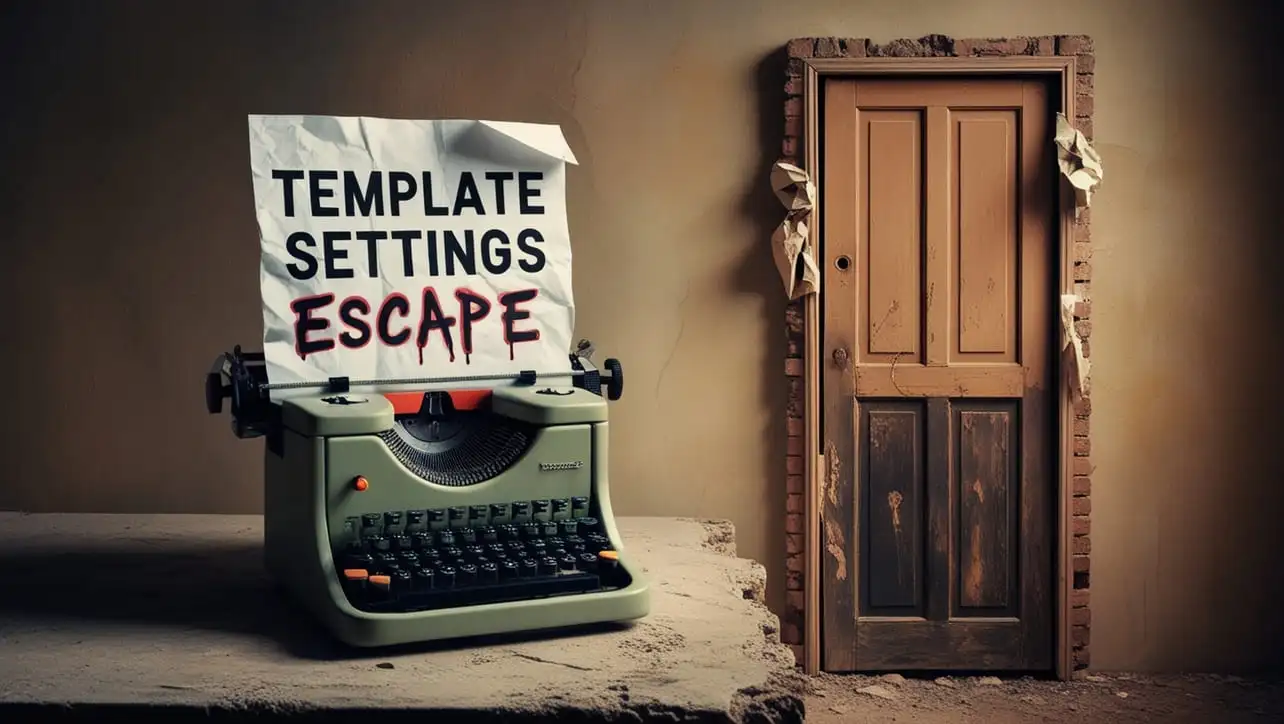
Lodash _.templateSettings.escape Property
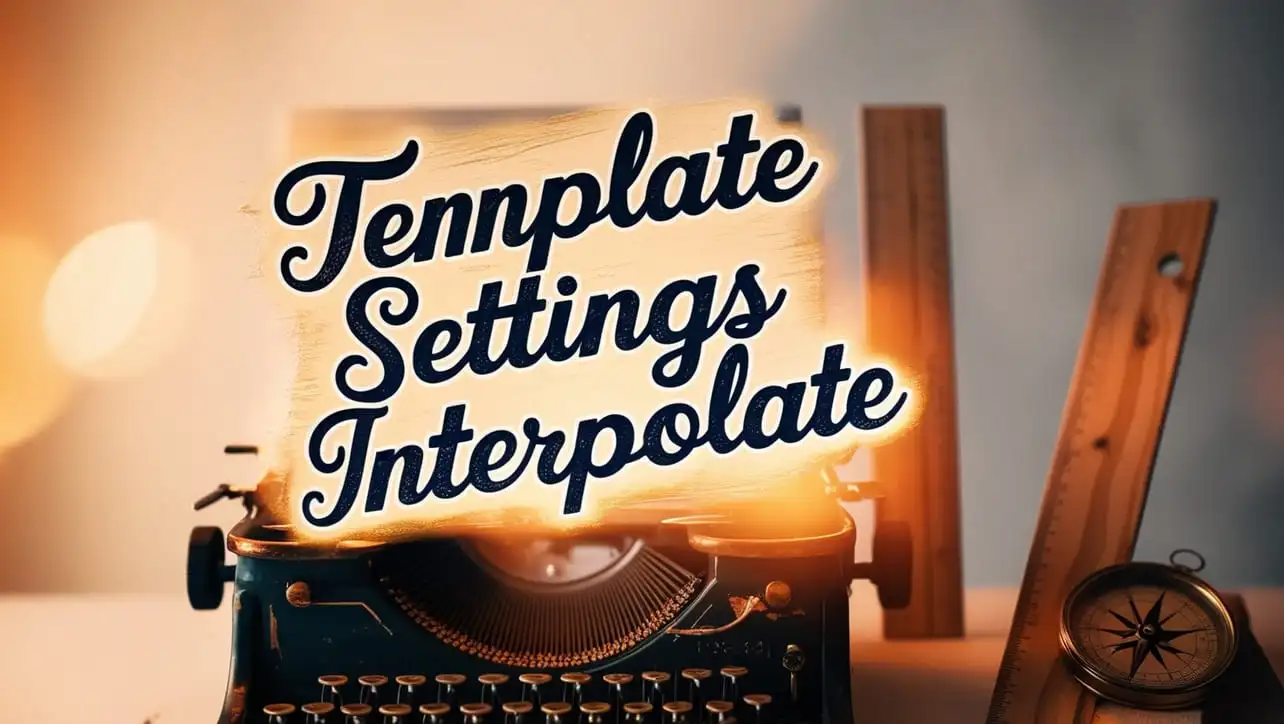
Lodash _.templateSettings.interpolate Property
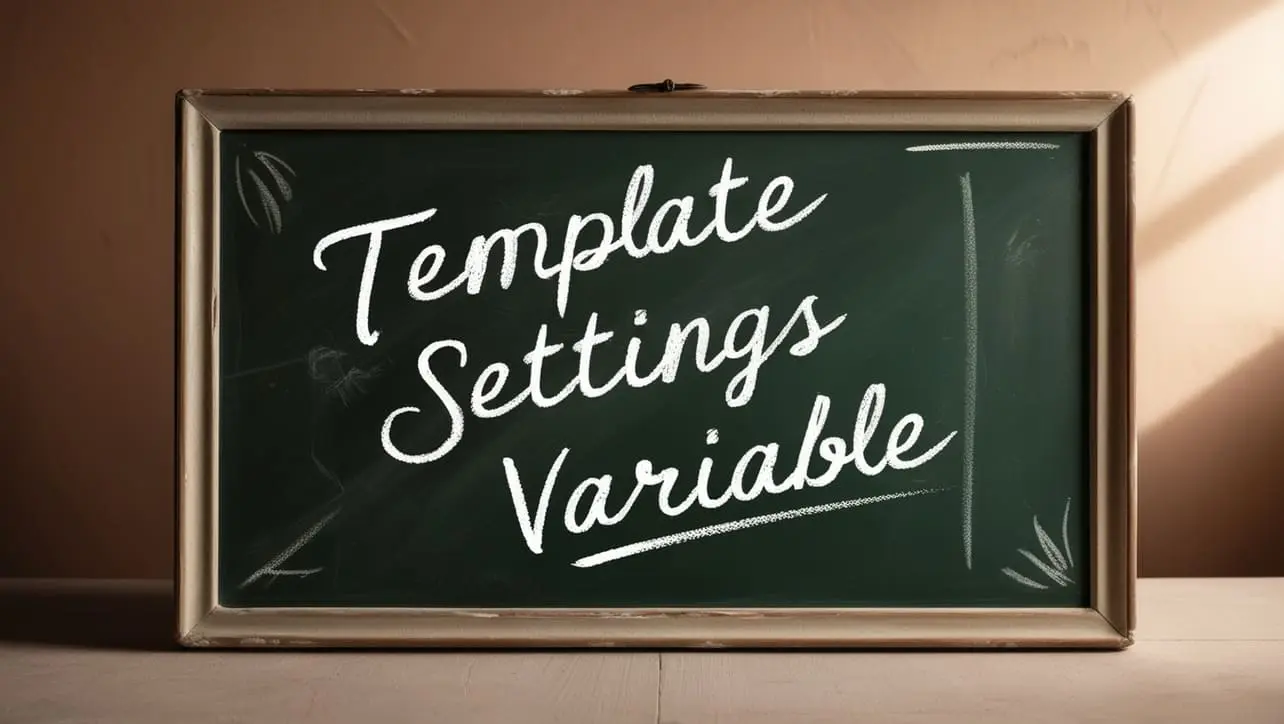
If you have any doubts regarding this article (Lodash _.lowerFirst() String Method), please comment here. I will help you immediately.