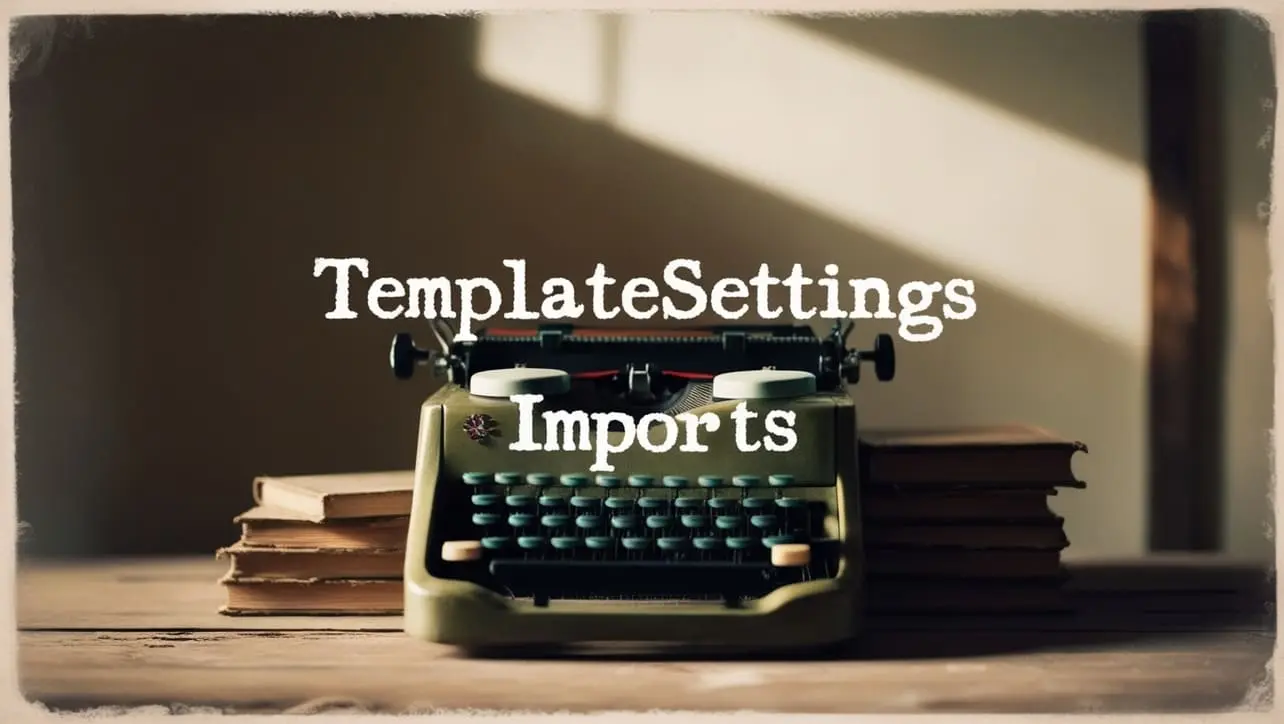
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.trimStart() String Method
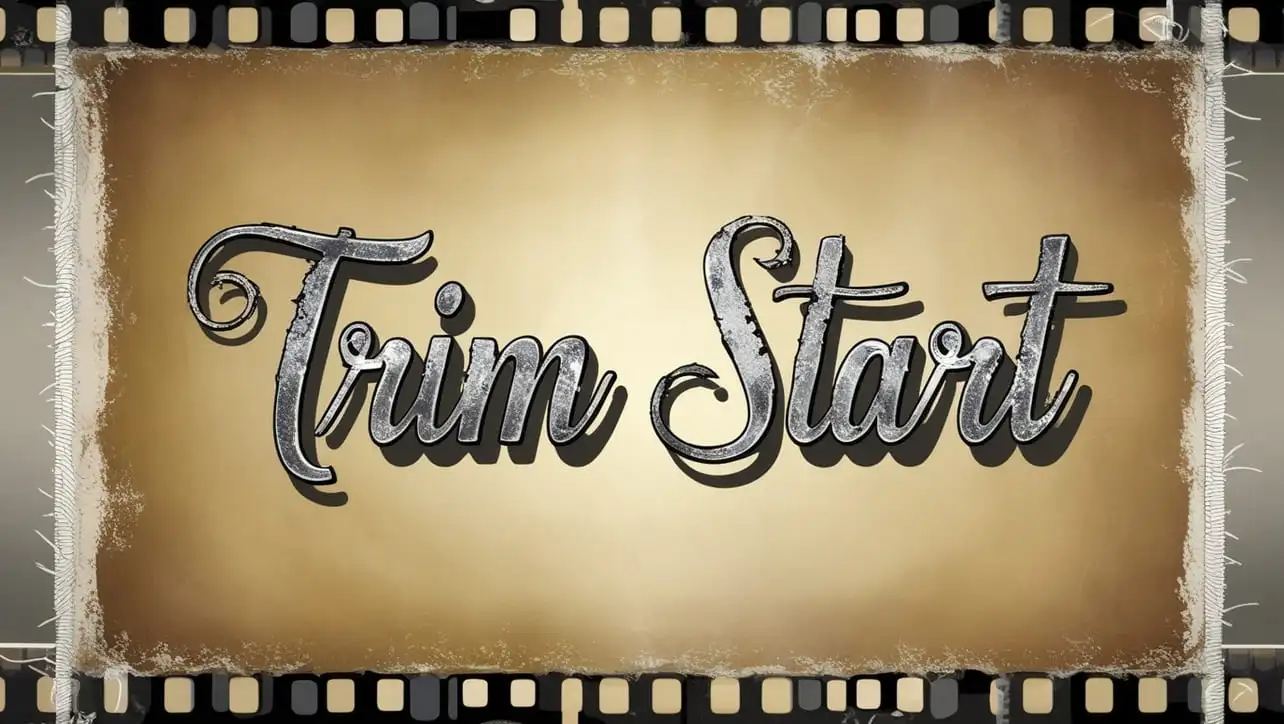
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, working with strings is a common task. Oftentimes, strings may contain leading whitespace characters, which can impact readability and data consistency. The Lodash library offers a comprehensive set of utility functions, including _.trimStart()
, designed to streamline string manipulation tasks.
This method removes leading whitespace characters from a string, ensuring cleaner and more predictable data processing.
🧠 Understanding _.trimStart() Method
The _.trimStart()
method in Lodash is specifically tailored to eliminate leading whitespace characters (spaces, tabs, etc.) from the beginning of a string. This simplifies string processing tasks and enhances data integrity by standardizing string representations.
💡 Syntax
The syntax for the _.trimStart()
method is straightforward:
_.trimStart(string)
- string: The input string to process.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.trimStart()
method:
const _ = require('lodash');
const stringWithWhitespace = ' Hello, World!';
const trimmedString = _.trimStart(stringWithWhitespace);
console.log(trimmedString);
// Output: 'Hello, World!'
In this example, the stringWithWhitespace is processed by _.trimStart()
, resulting in a new string with leading whitespace characters removed.
🏆 Best Practices
When working with the _.trimStart()
method, consider the following best practices:
Consistent Data Formatting:
Ensure consistent formatting of string data by applying
_.trimStart()
before performing further processing or comparison operations. This helps prevent discrepancies caused by leading whitespace characters.example.jsCopiedconst userInput = /* ...fetch user input... */; const formattedInput = _.trimStart(userInput); // Use formattedInput for further processing
Input Validation:
Prior to applying
_.trimStart()
, validate input strings to handle edge cases and ensure data integrity. This minimizes unexpected behavior and enhances the robustness of your code.example.jsCopiedconst userInput = /* ...fetch user input... */; if (typeof userInput === 'string') { const trimmedInput = _.trimStart(userInput); // Process trimmedInput } else { console.error('Invalid input type'); }
Custom Whitespace Characters:
Consider scenarios where custom whitespace characters need to be trimmed. Utilize regular expressions or custom functions in conjunction with
_.trimStart()
to accommodate specific requirements.example.jsCopiedconst stringWithCustomWhitespace = '---Hello, World!'; const trimmedString = _.trimStart(stringWithCustomWhitespace, '-'); console.log(trimmedString); // Output: 'Hello, World!'
📚 Use Cases
Form Input Processing:
When handling user input from forms or text fields,
_.trimStart()
can be used to sanitize input strings, removing leading whitespace characters before further processing.example.jsCopiedconst userInput = /* ...fetch user input from form... */; const trimmedInput = _.trimStart(userInput); // Process trimmedInput
Data Normalization:
In datasets containing strings with inconsistent leading whitespace,
_.trimStart()
facilitates data normalization by standardizing string representations, ensuring consistency across records.example.jsCopiedconst dataWithWhitespace = /* ...fetch data from database or API... */; const normalizedData = dataWithWhitespace.map(entry => _.trimStart(entry)); console.log(normalizedData);
File System Operations:
During file system operations, such as reading from or writing to files,
_.trimStart()
can be utilized to preprocess strings, removing leading whitespace characters from file contents.example.jsCopiedconst fs = require('fs'); const fileContents = fs.readFileSync('example.txt', 'utf-8'); const trimmedContents = _.trimStart(fileContents); // Process trimmedContents
🎉 Conclusion
The _.trimStart()
method in Lodash offers a convenient solution for removing leading whitespace characters from strings, improving data consistency and enhancing code readability. Whether you're sanitizing user input, normalizing data, or preprocessing file contents, _.trimStart()
provides a versatile tool for string manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.trimStart()
method in your Lodash projects.
👨💻 Join our Community:
Author
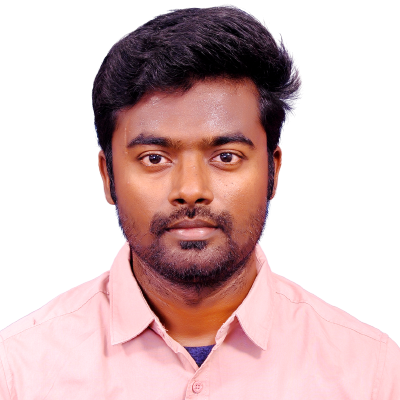
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
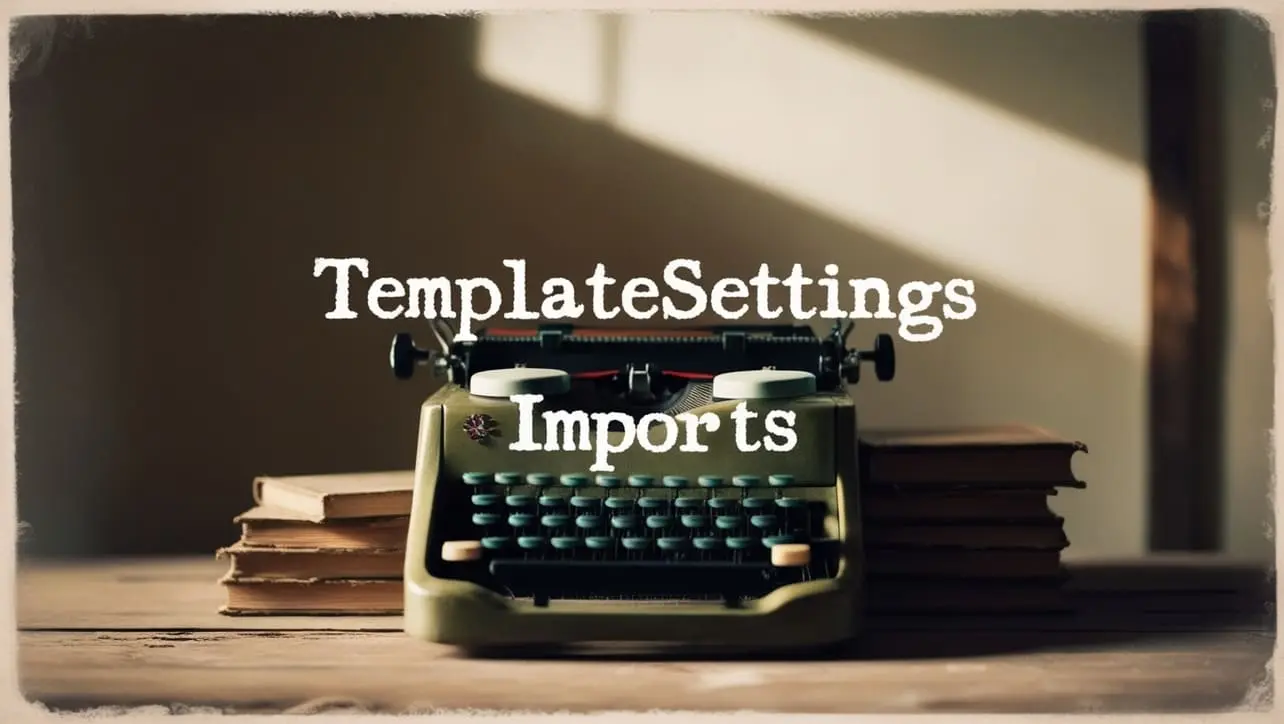
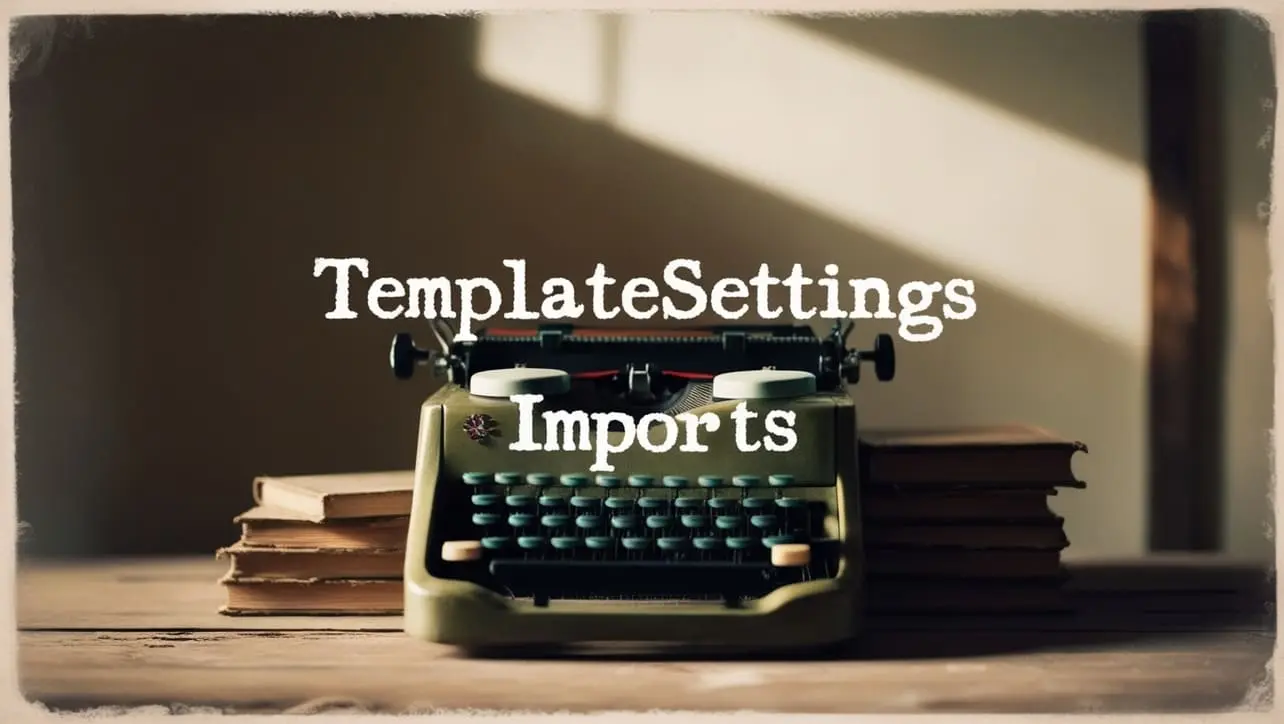
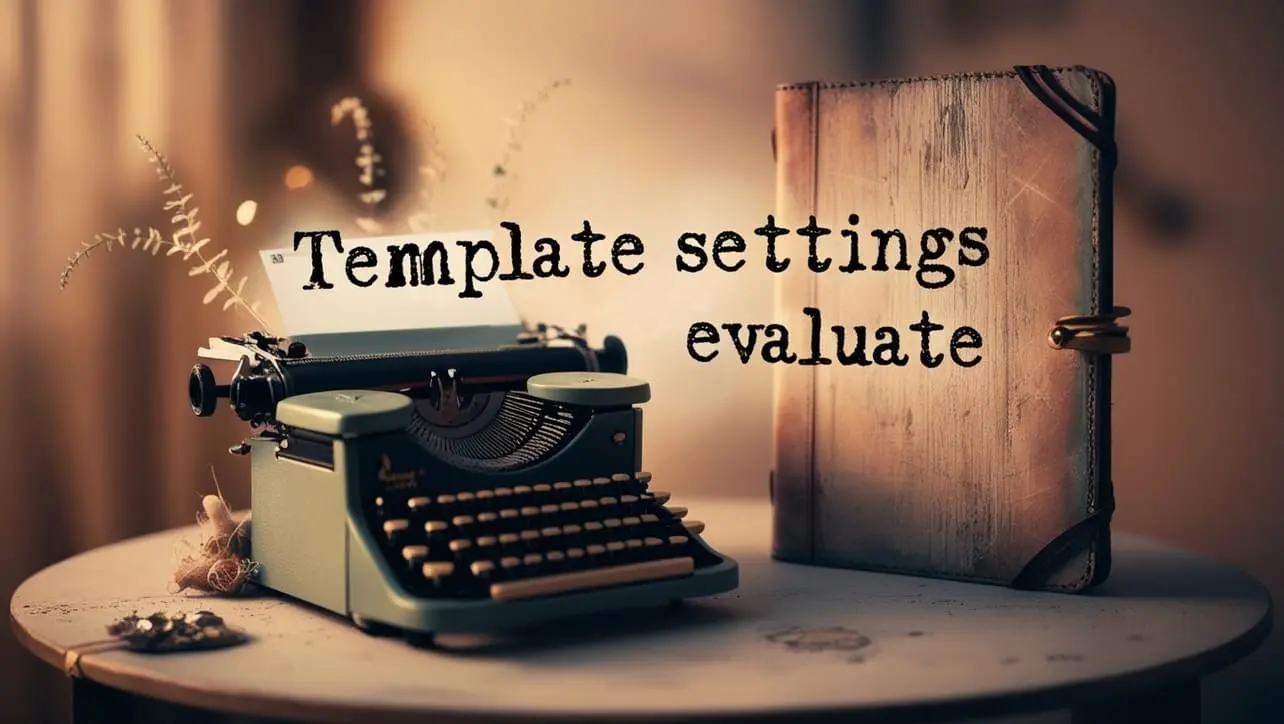
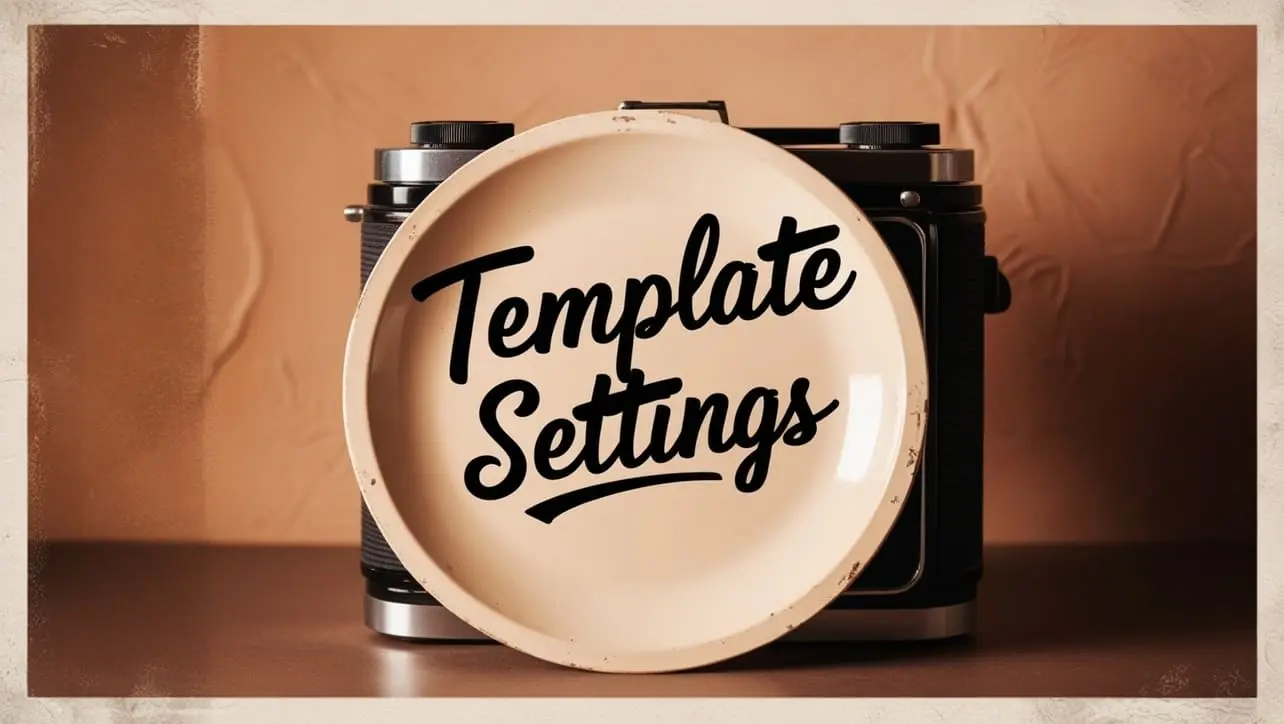
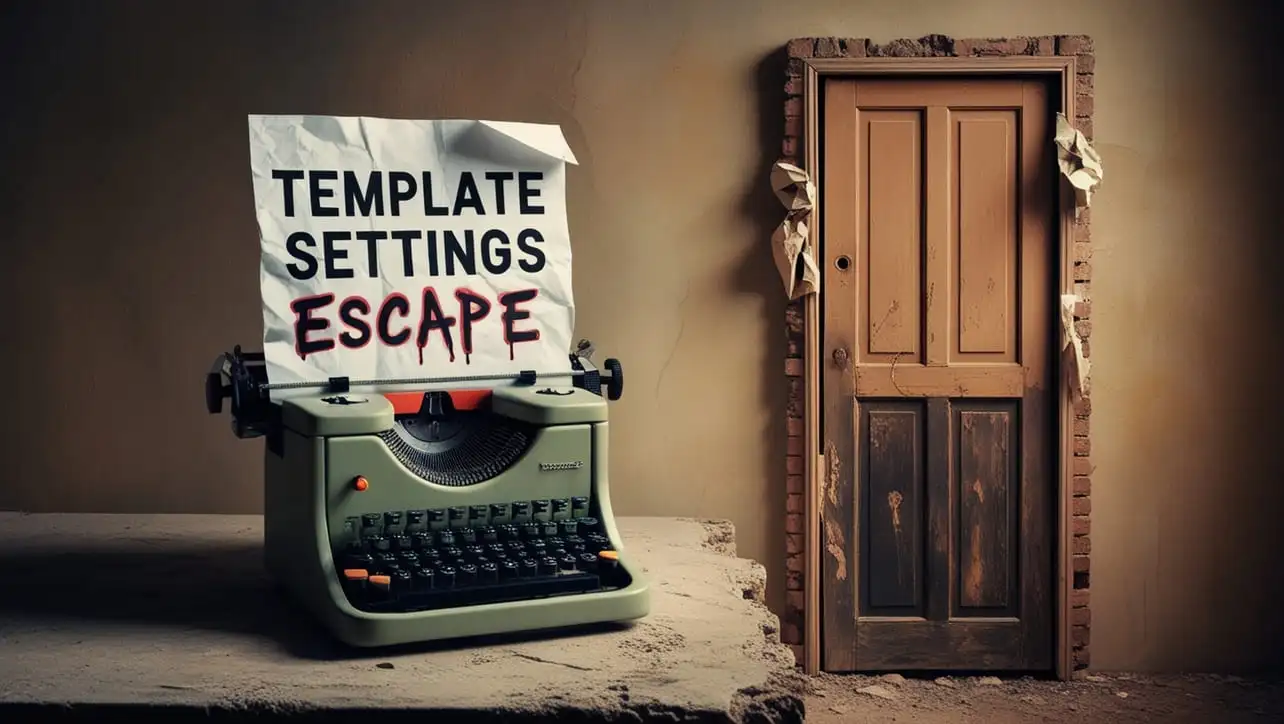
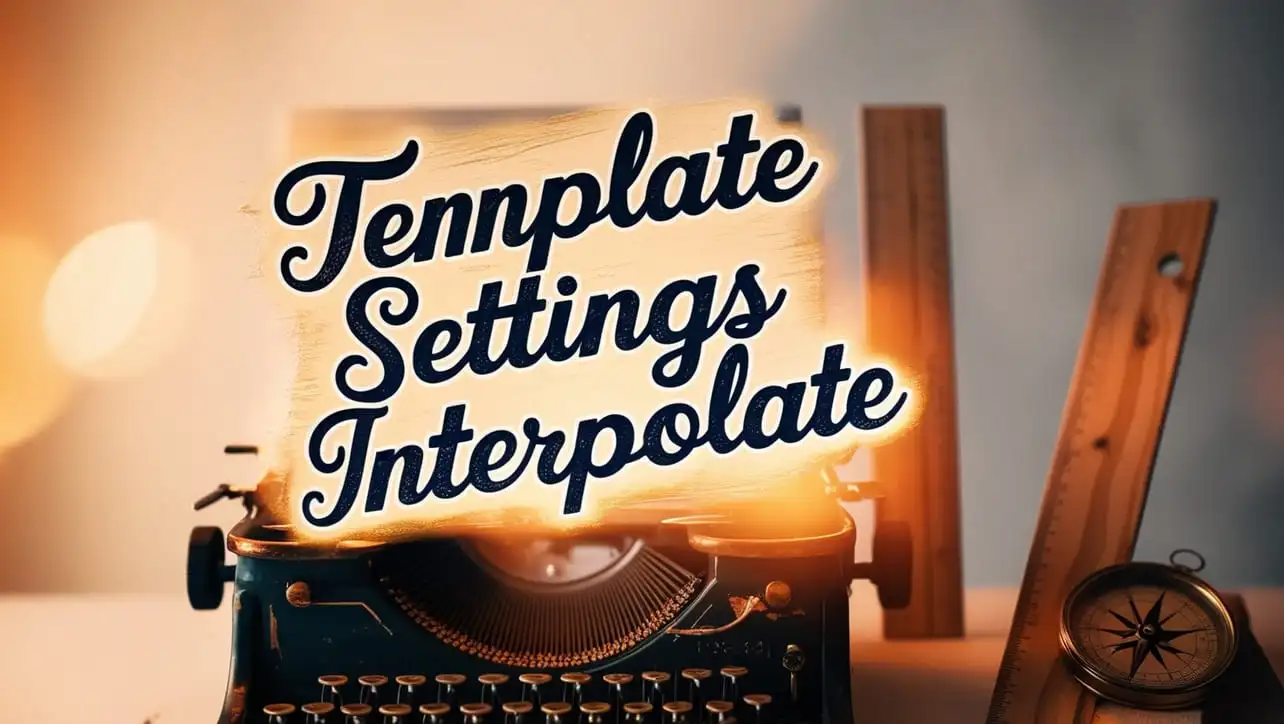
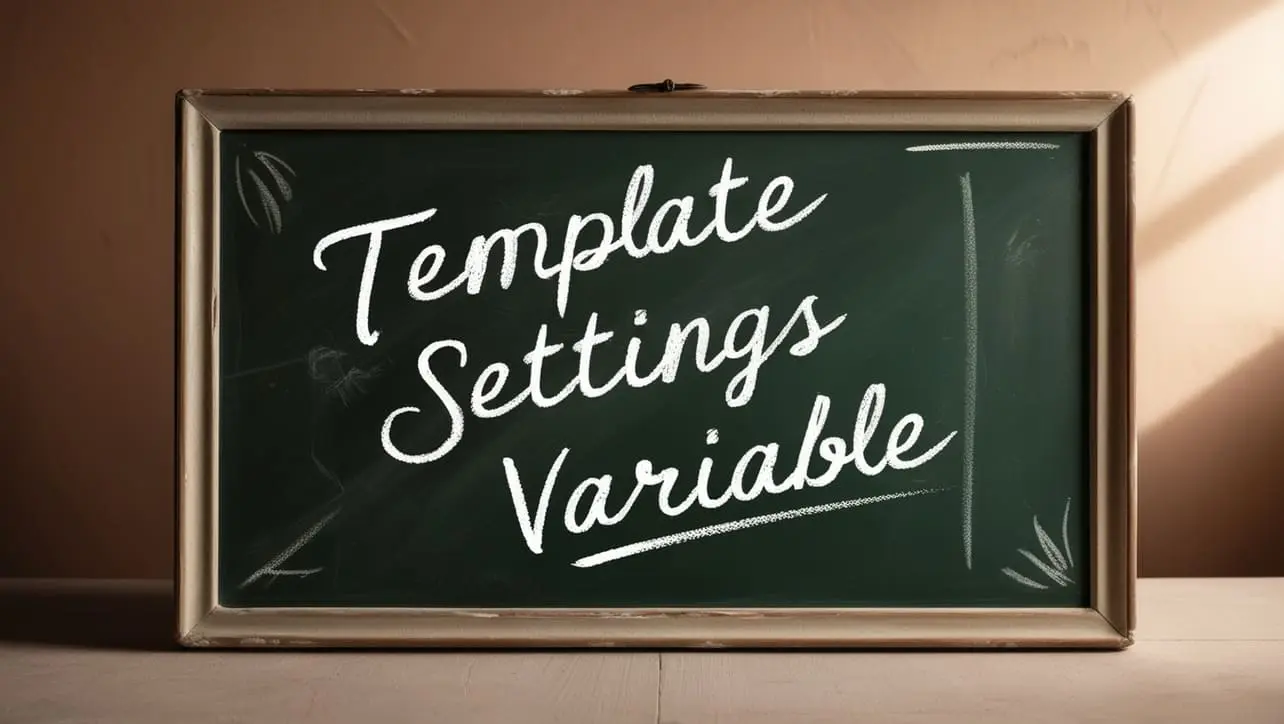
If you have any doubts regarding this article (Lodash _.trimStart() String Method), please comment here. I will help you immediately.