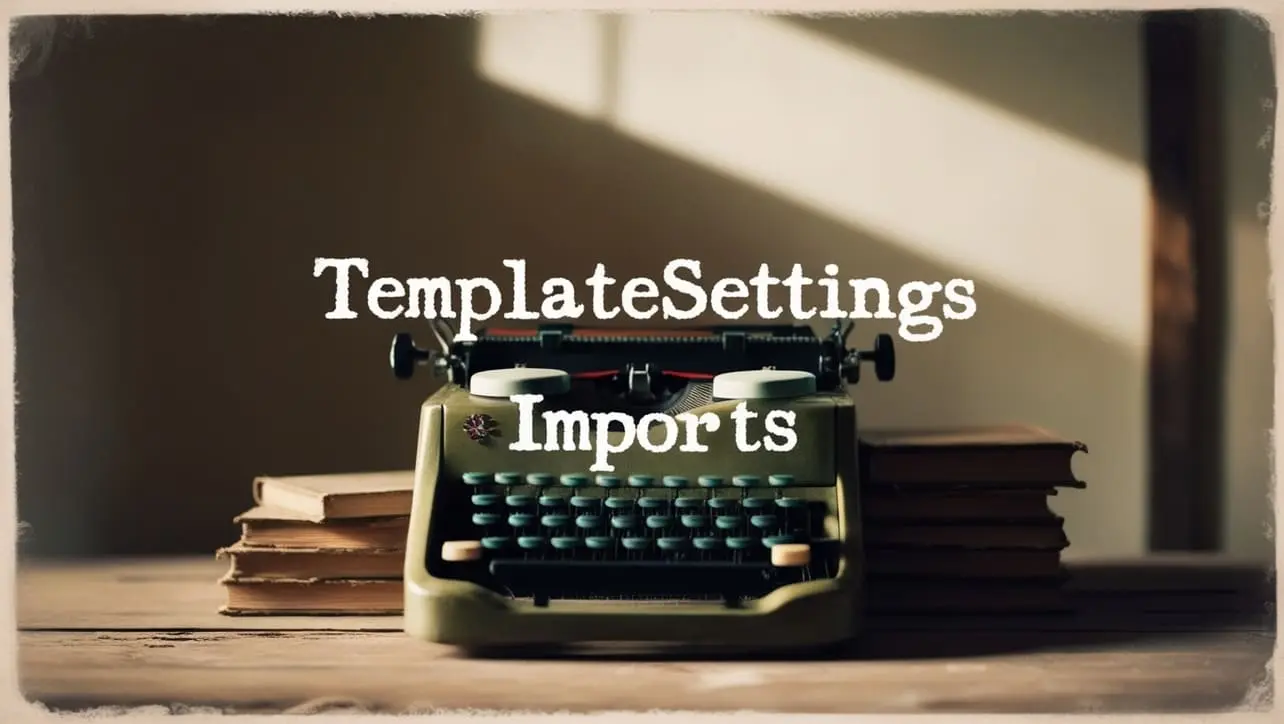
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.trimEnd() String Method
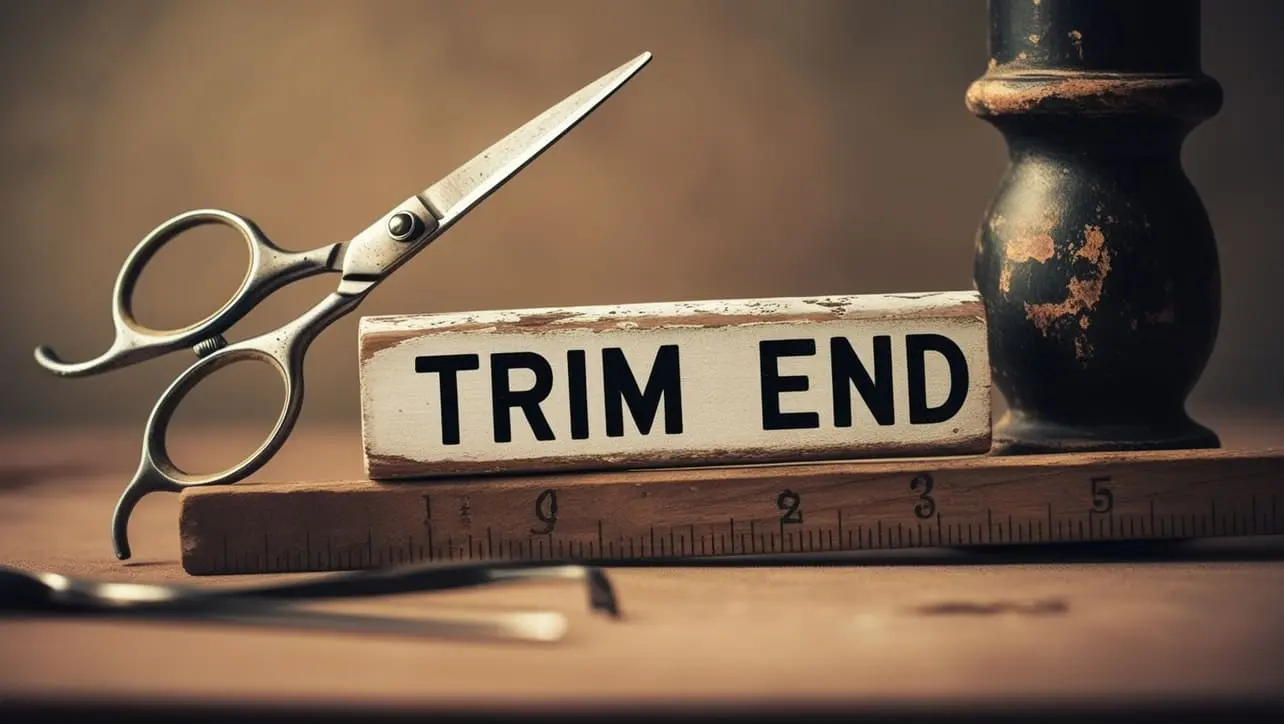
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, managing strings is a common task, and sometimes, it's necessary to remove trailing whitespace from them. The Lodash library offers a variety of utility functions, including _.trimEnd()
, which provides a convenient way to trim whitespace from the end of a string.
This method is particularly useful for data normalization and improving user input validation.
🧠 Understanding _.trimEnd() Method
The _.trimEnd()
method in Lodash is designed to remove trailing whitespace from a string. It ensures that strings are clean and consistent, preventing unintended errors caused by whitespace characters at the end.
💡 Syntax
The syntax for the _.trimEnd()
method is straightforward:
_.trimEnd(string)
- string: The string to trim.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.trimEnd()
method:
const _ = require('lodash');
const stringWithWhitespace = ' Hello, World! ';
const trimmedString = _.trimEnd(stringWithWhitespace);
console.log(trimmedString);
// Output: ' Hello, World!'
In this example, the stringWithWhitespace is processed by _.trimEnd()
, resulting in a new string with trailing whitespace removed.
🏆 Best Practices
When working with the _.trimEnd()
method, consider the following best practices:
Consistent Data Input:
Ensure consistent data input by trimming whitespace from user-provided strings. This helps maintain data integrity and consistency across your application.
example.jsCopiedconst userInput = /* ...retrieve user input... */; const trimmedInput = _.trimEnd(userInput); console.log(trimmedInput);
Data Normalization:
Use
_.trimEnd()
for data normalization, ensuring that strings adhere to a standardized format. This is particularly useful when working with user-generated content or external data sources.example.jsCopiedconst messyData = /* ...retrieve data... */; const normalizedData = messyData.map(_.trimEnd); console.log(normalizedData);
String Validation:
Incorporate
_.trimEnd()
into string validation routines to ensure that trailing whitespace does not interfere with data processing or comparisons.example.jsCopiedconst validateString = (str) => { const trimmedStr = _.trimEnd(str); // Perform validation logic on trimmed string }; validateString(' validString ');
📚 Use Cases
Form Input Processing:
When handling form input, use
_.trimEnd()
to sanitize user-entered strings, ensuring that trailing whitespace does not affect data integrity or validation.example.jsCopiedconst formInput = /* ...retrieve form input... */; const sanitizedInput = _.trimEnd(formInput); console.log(sanitizedInput);
Database Query Results:
When retrieving data from a database, use
_.trimEnd()
to clean up string fields, ensuring consistency and eliminating trailing whitespace.example.jsCopiedconst databaseResults = /* ...fetch data from database... */; const cleanedResults = databaseResults.map(result => _.trimEnd(result)); console.log(cleanedResults);
Text Processing:
In text processing tasks, such as parsing log files or analyzing textual data,
_.trimEnd()
can be used to prepare strings for further analysis or manipulation.example.jsCopiedconst logEntry = /* ...retrieve log entry... */; const trimmedLogEntry = _.trimEnd(logEntry); console.log(trimmedLogEntry);
🎉 Conclusion
The _.trimEnd()
method in Lodash offers a convenient solution for removing trailing whitespace from strings. Whether you're working with user input, database queries, or text processing tasks, this method provides a reliable way to ensure data consistency and integrity.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.trimEnd()
method in your Lodash projects.
👨💻 Join our Community:
Author
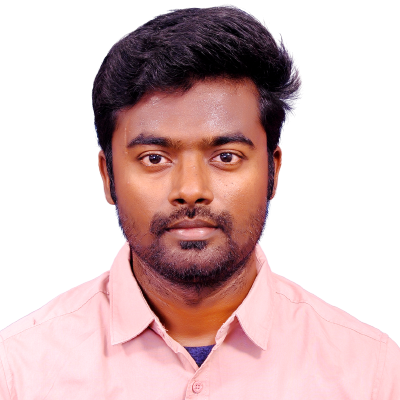
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
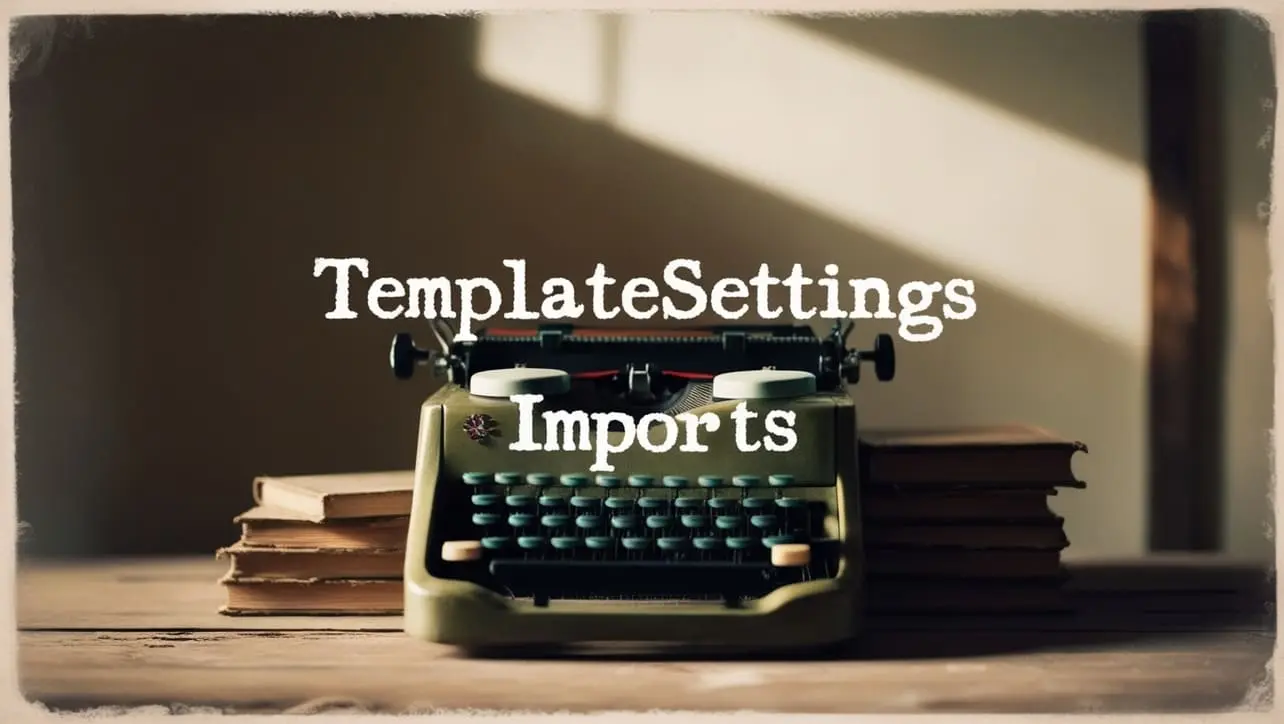
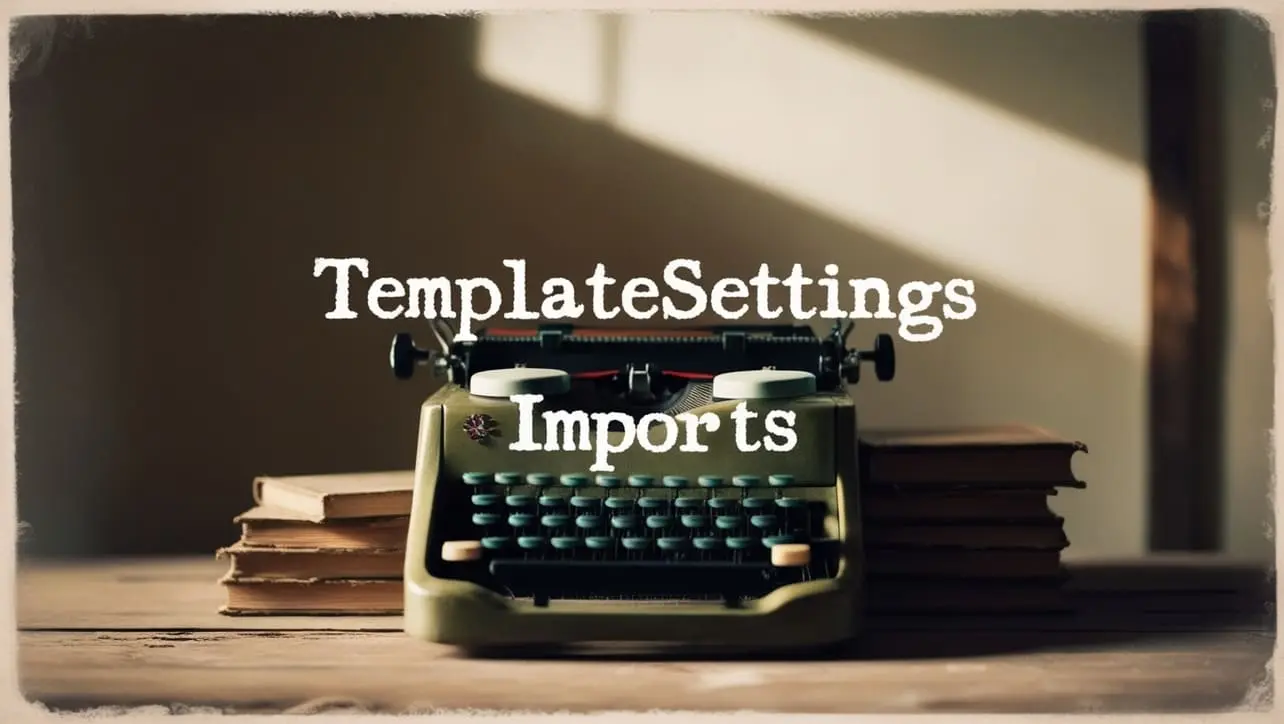
Lodash _.templateSettings.imports Property
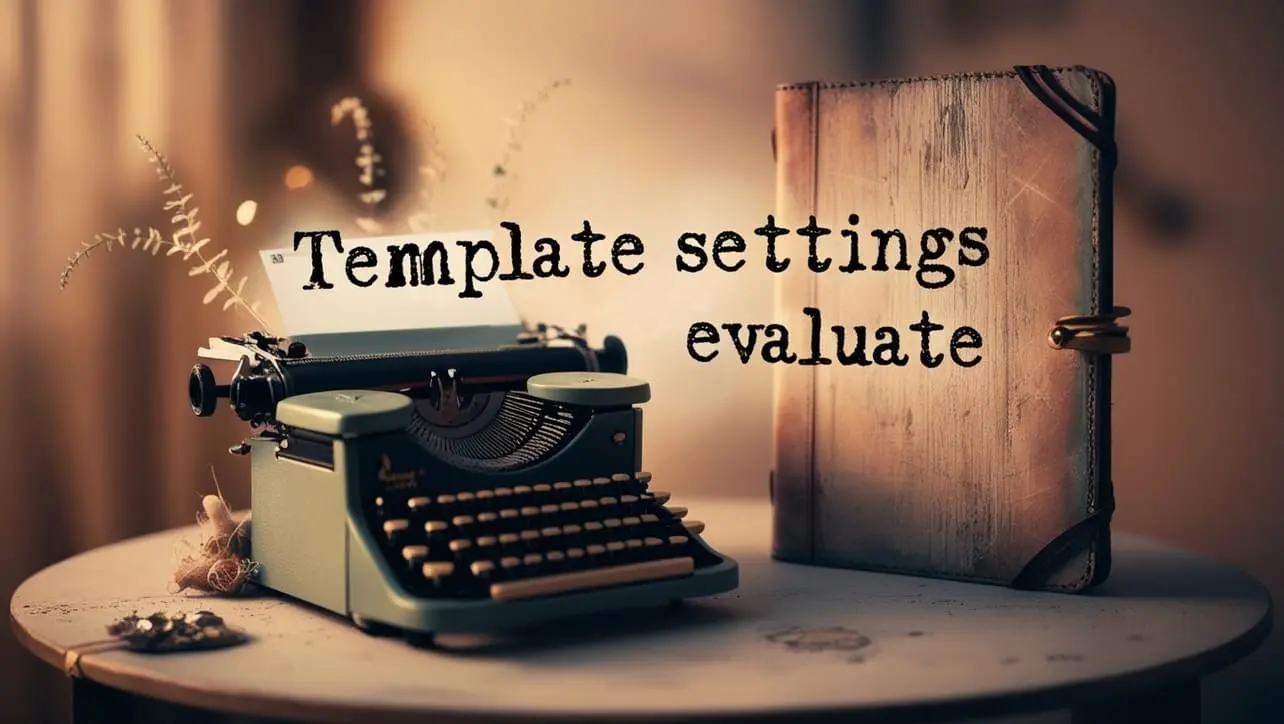
Lodash _.templateSettings.evaluate Property
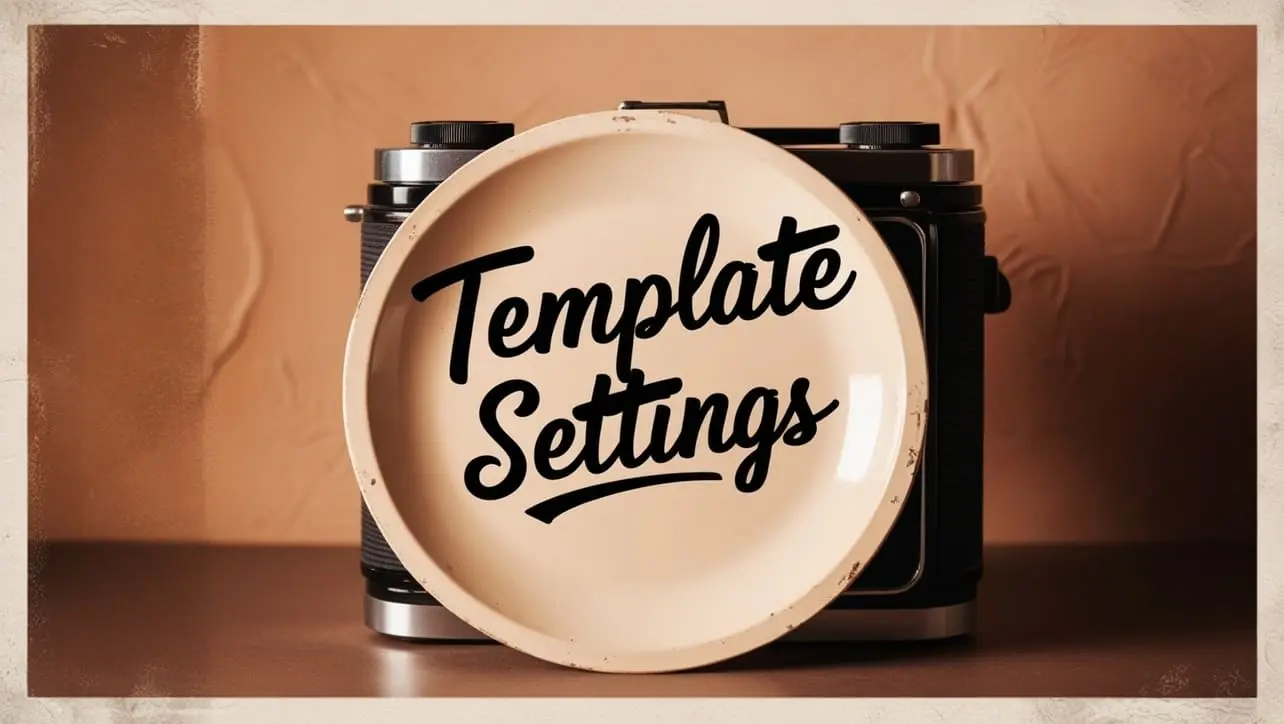
Lodash _.templateSettings Property
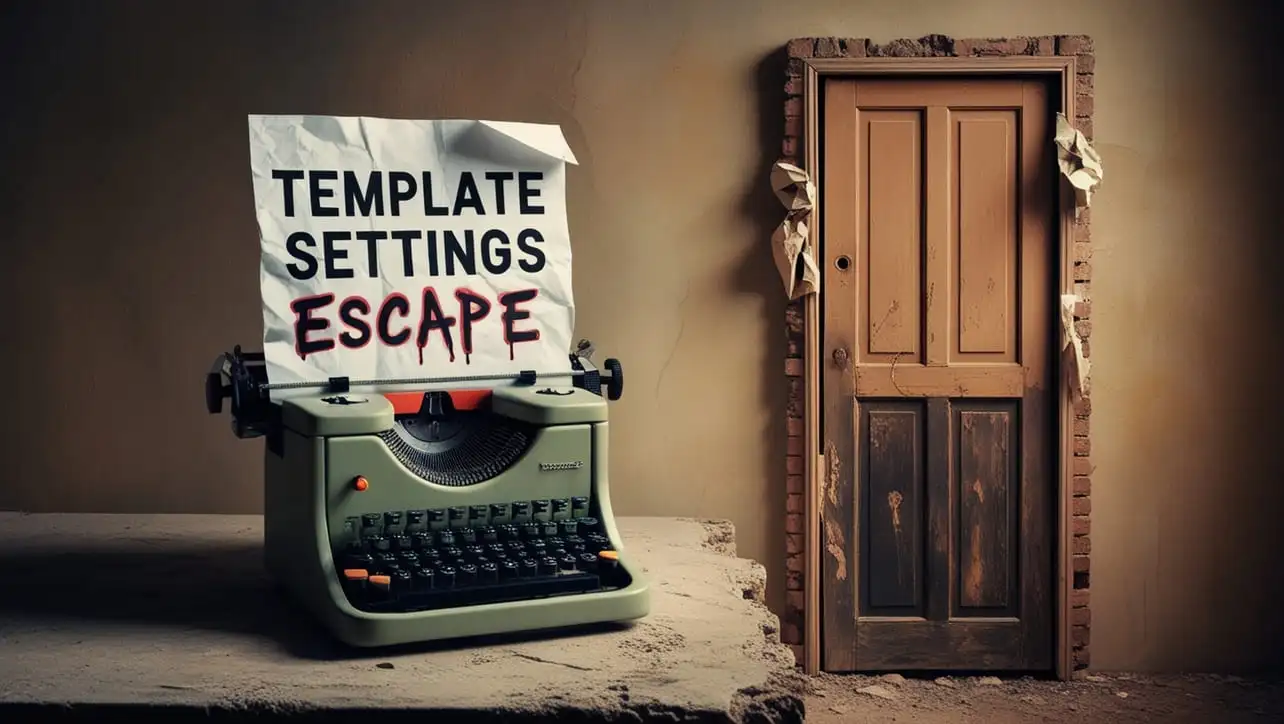
Lodash _.templateSettings.escape Property
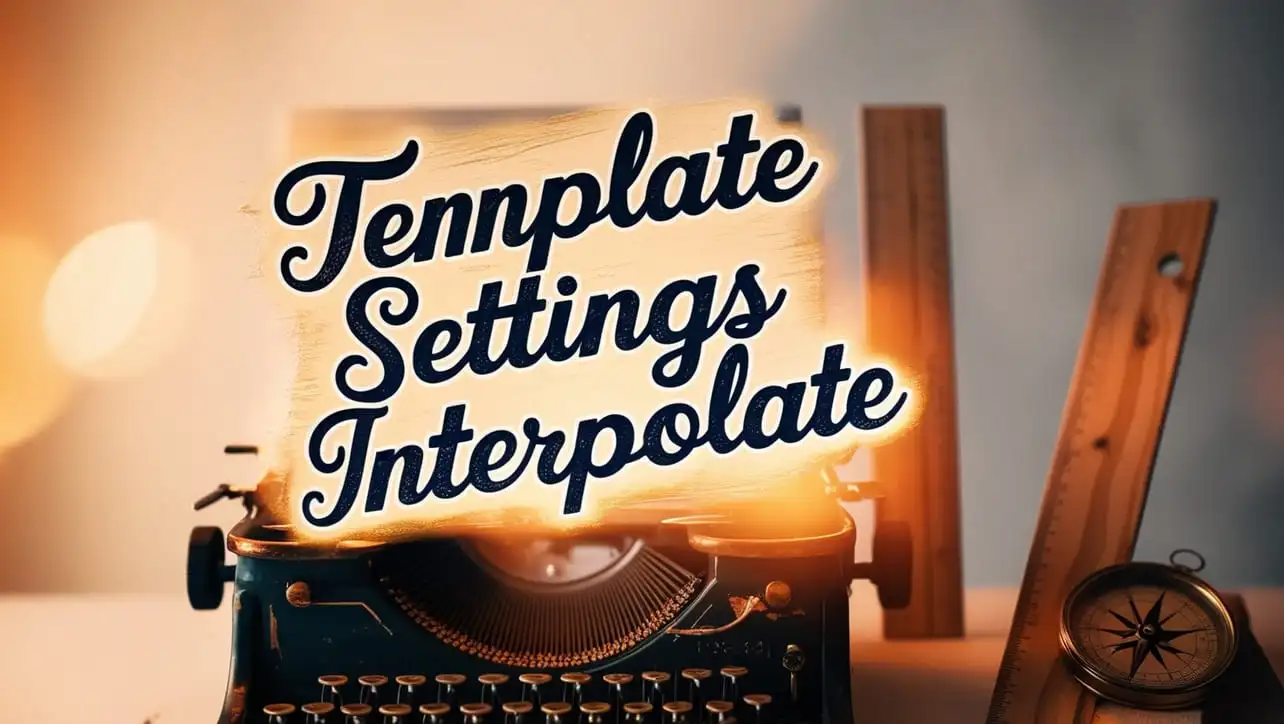
Lodash _.templateSettings.interpolate Property
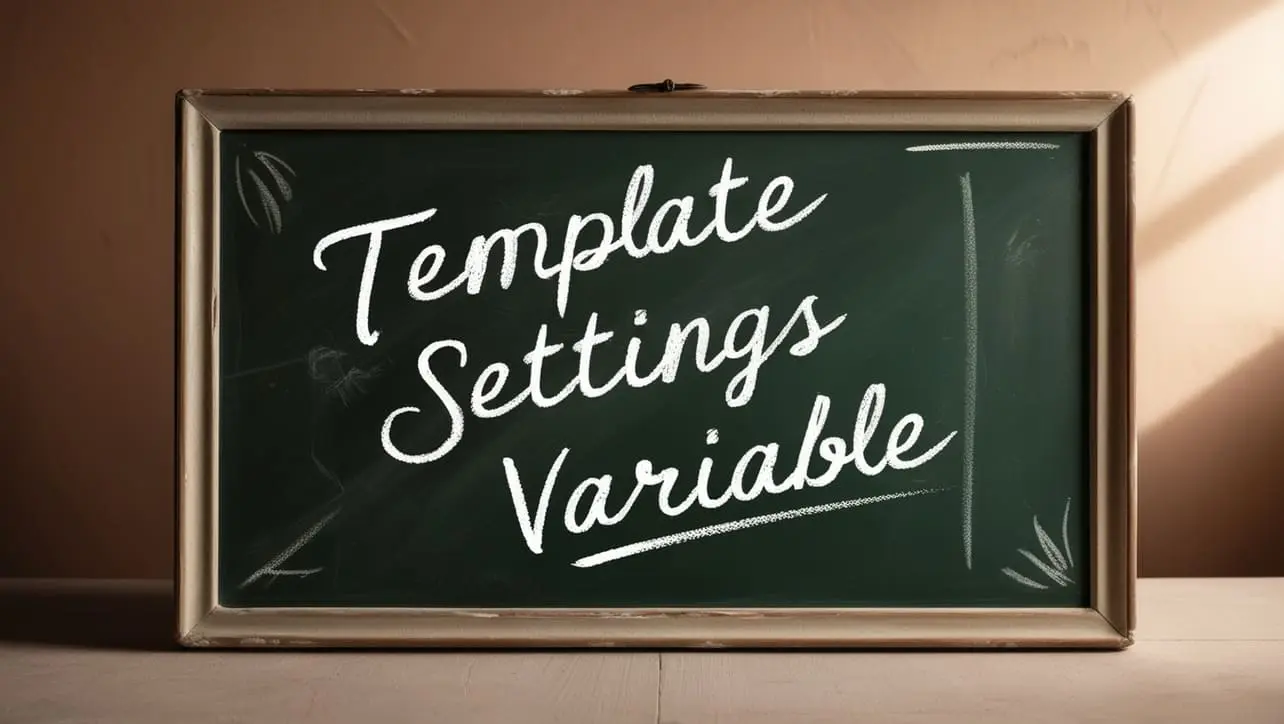
If you have any doubts regarding this article (Lodash _.trimEnd() String Method), please comment here. I will help you immediately.