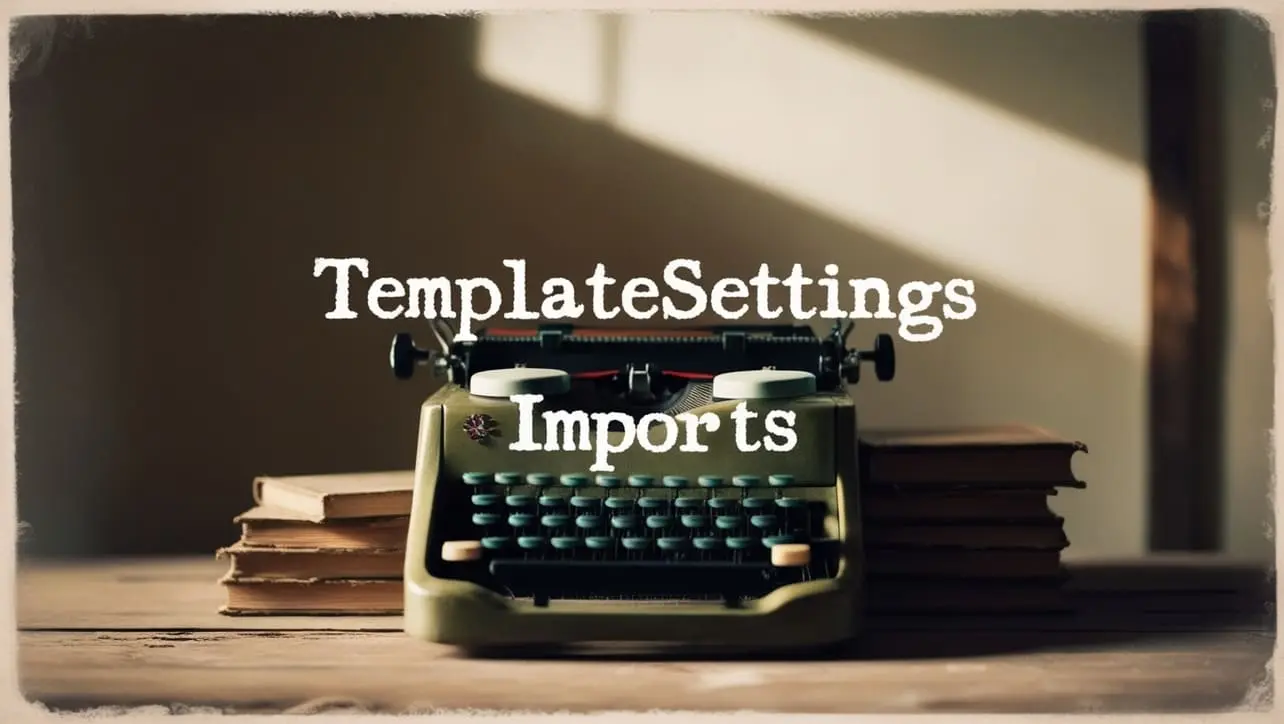
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.toUpper() String Method
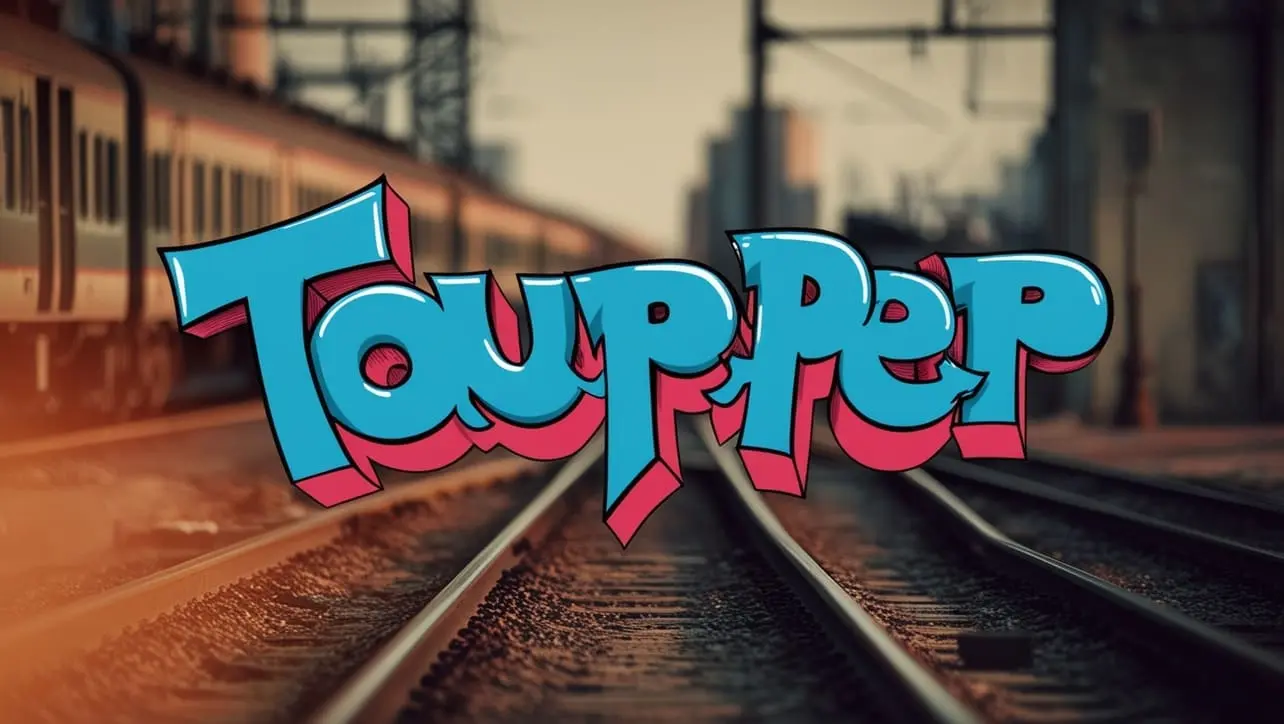
Photo Credit to CodeToFun
π Introduction
In JavaScript, string manipulation is a common task in web development. Lodash, a widely-used utility library, offers various functions to simplify string operations. Among these functions is _.toUpper()
, a method designed to convert a string to uppercase.
This method provides a convenient way to ensure consistent casing in strings, enhancing readability and facilitating data processing.
π§ Understanding _.toUpper() Method
The _.toUpper()
method in Lodash converts all characters in a string to uppercase. This simple yet powerful functionality can be applied to a wide range of use cases, from formatting user input to standardizing data for comparison.
π‘ Syntax
The syntax for the _.toUpper()
method is straightforward:
_.toUpper([string=''])
- string: The string to convert to uppercase.
π Example
Let's dive into a simple example to illustrate the usage of the _.toUpper()
method:
const _ = require('lodash');
const lowercaseString = 'hello, world!';
const uppercaseString = _.toUpper(lowercaseString);
console.log(uppercaseString);
// Output: 'HELLO, WORLD!'
In this example, the lowercaseString is converted to uppercase using _.toUpper()
, resulting in HELLO, WORLD!.
π Best Practices
When working with the _.toUpper()
method, consider the following best practices:
Input Validation:
Before applying
_.toUpper()
, ensure that the input is a valid string. This helps prevent unexpected behavior and improves error handling.example.jsCopiedconst input = /* ...user input or data... */; if (typeof input === 'string') { const uppercaseInput = _.toUpper(input); console.log(uppercaseInput); } else { console.error('Invalid input: not a string'); }
Unicode Support:
Be aware of Unicode characters in the input string, as
_.toUpper()
may not handle certain characters as expected. Test with a variety of inputs to ensure compatibility with different character sets.example.jsCopiedconst unicodeString = 'γγγ«γ‘γ―γδΈηοΌ'; const uppercaseUnicodeString = _.toUpper(unicodeString); console.log(uppercaseUnicodeString); // Output: 'γγγ«γ‘γ―γδΈηοΌ' (no change)
Performance Considerations:
While
_.toUpper()
provides convenience, be mindful of performance implications when processing large volumes of data. Evaluate whether alternative approaches or optimizations are necessary for optimal performance.example.jsCopiedconst largeString = /* ...large string data... */; console.time('toUpper'); const uppercaseLargeString = _.toUpper(largeString); console.timeEnd('toUpper'); console.log(uppercaseLargeString);
π Use Cases
User Input Formatting:
_.toUpper()
can be used to format user input, ensuring consistency in data processing and display.example.jsCopiedconst userInput = /* ...user input... */; const formattedInput = _.toUpper(userInput); console.log(formattedInput);
Data Normalization:
When dealing with data from different sources,
_.toUpper()
can normalize string casing, facilitating accurate comparison and analysis.example.jsCopiedconst dataFromVariousSources = /* ...fetch data from different sources... */; const normalizedData = dataFromVariousSources.map(item => _.toUpper(item)); console.log(normalizedData);
Output Rendering:
In user interfaces or reports,
_.toUpper()
can be utilized to ensure consistent and visually appealing text rendering.example.jsCopiedconst textToDisplay = /* ...retrieve text data... */; const formattedText = _.toUpper(textToDisplay); console.log(formattedText);
π Conclusion
The _.toUpper()
method in Lodash offers a convenient solution for converting strings to uppercase, enhancing consistency and readability in JavaScript applications. Whether you're formatting user input, normalizing data, or rendering output, this method provides a versatile tool for string manipulation.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.toUpper()
method in your Lodash projects.
π¨βπ» Join our Community:
Author
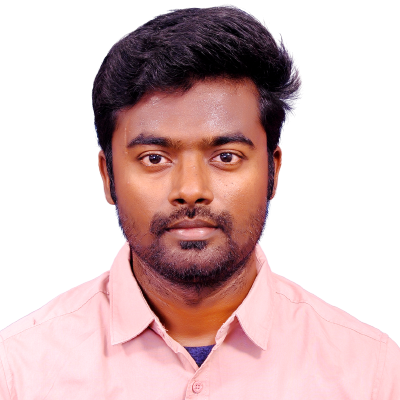
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
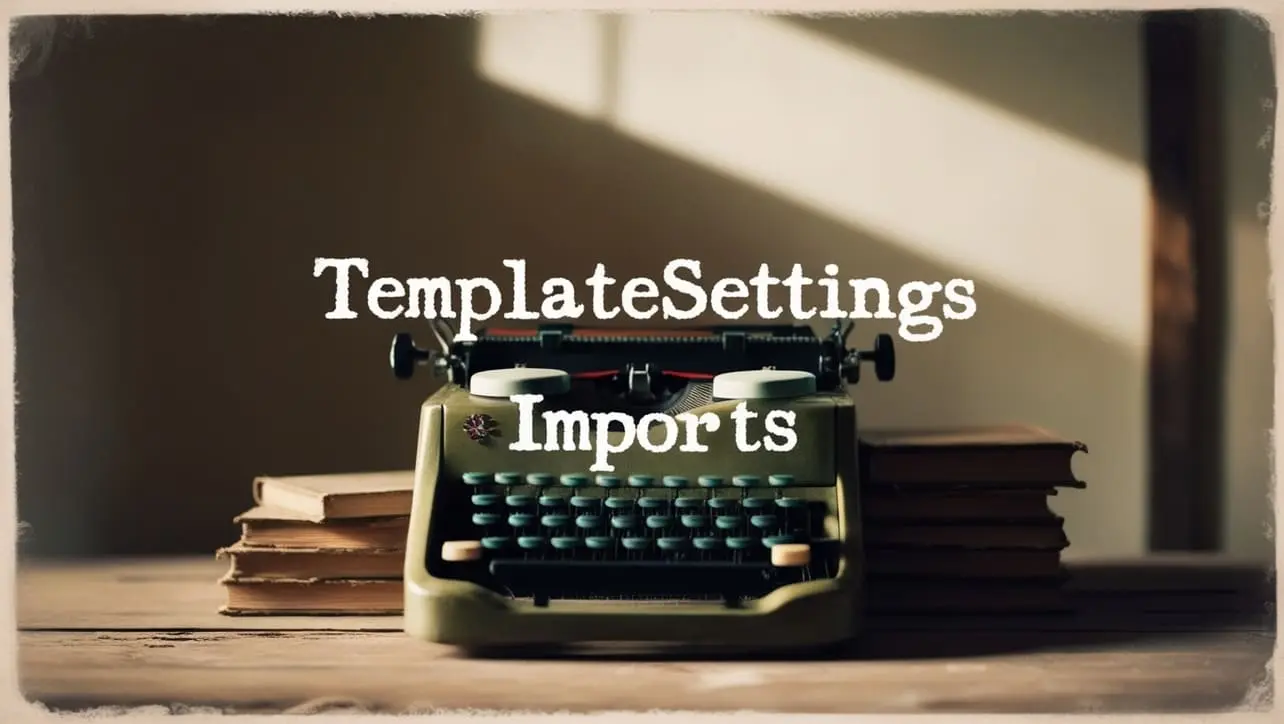
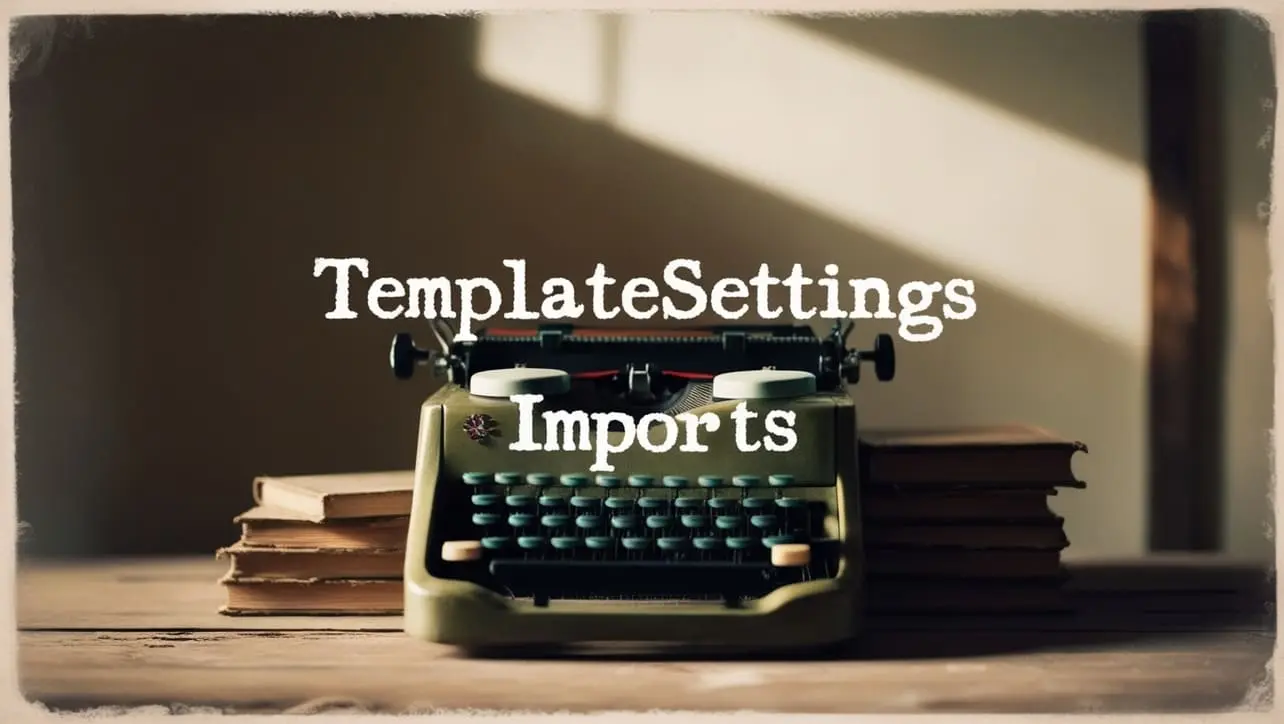
Lodash _.templateSettings.imports Property
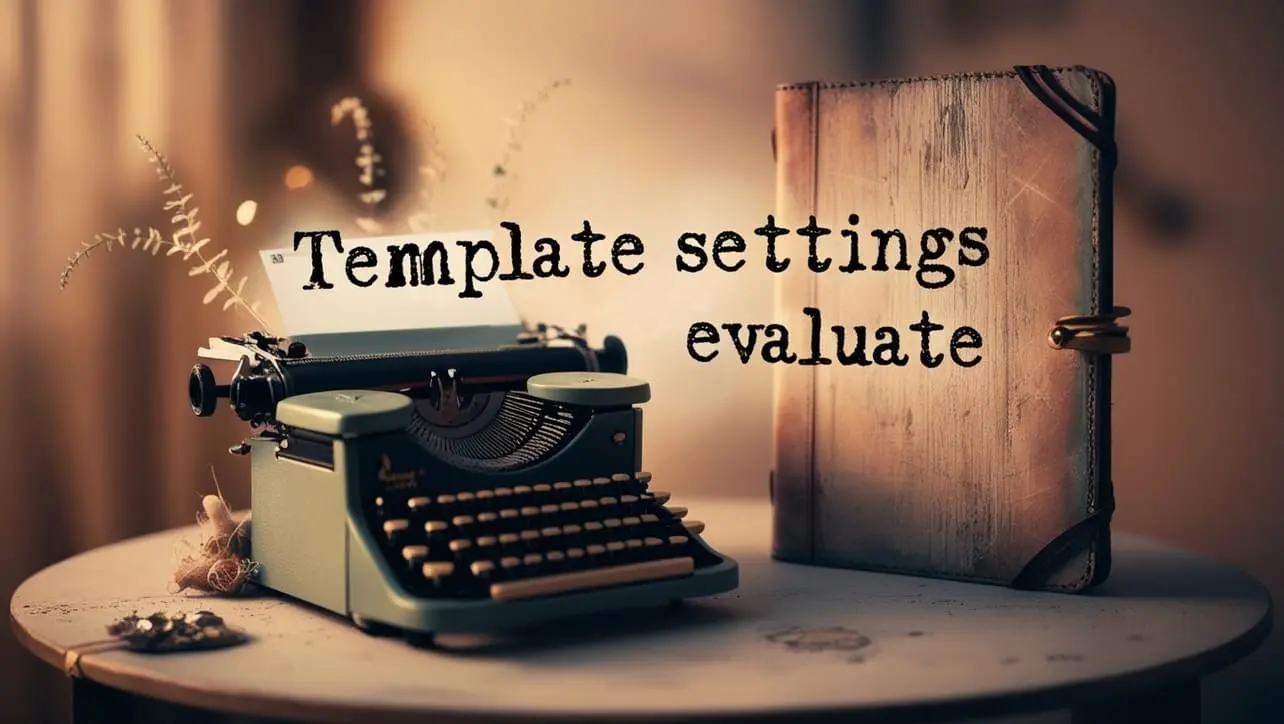
Lodash _.templateSettings.evaluate Property
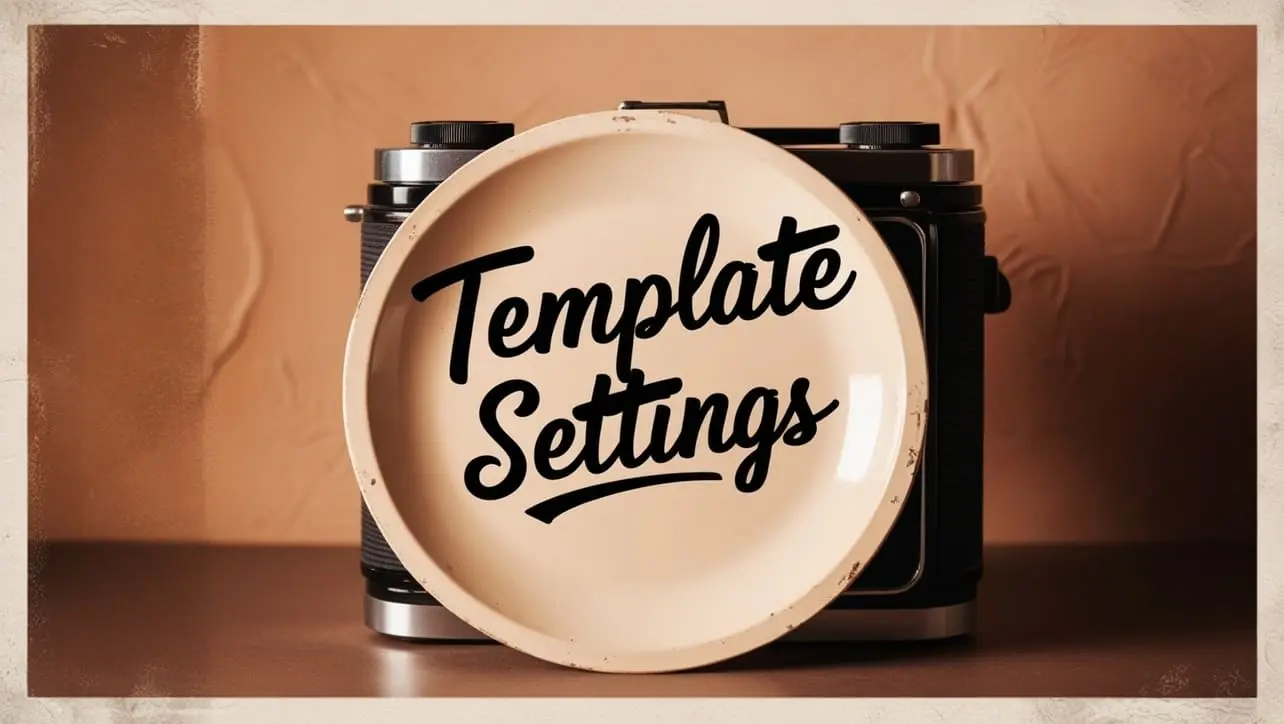
Lodash _.templateSettings Property
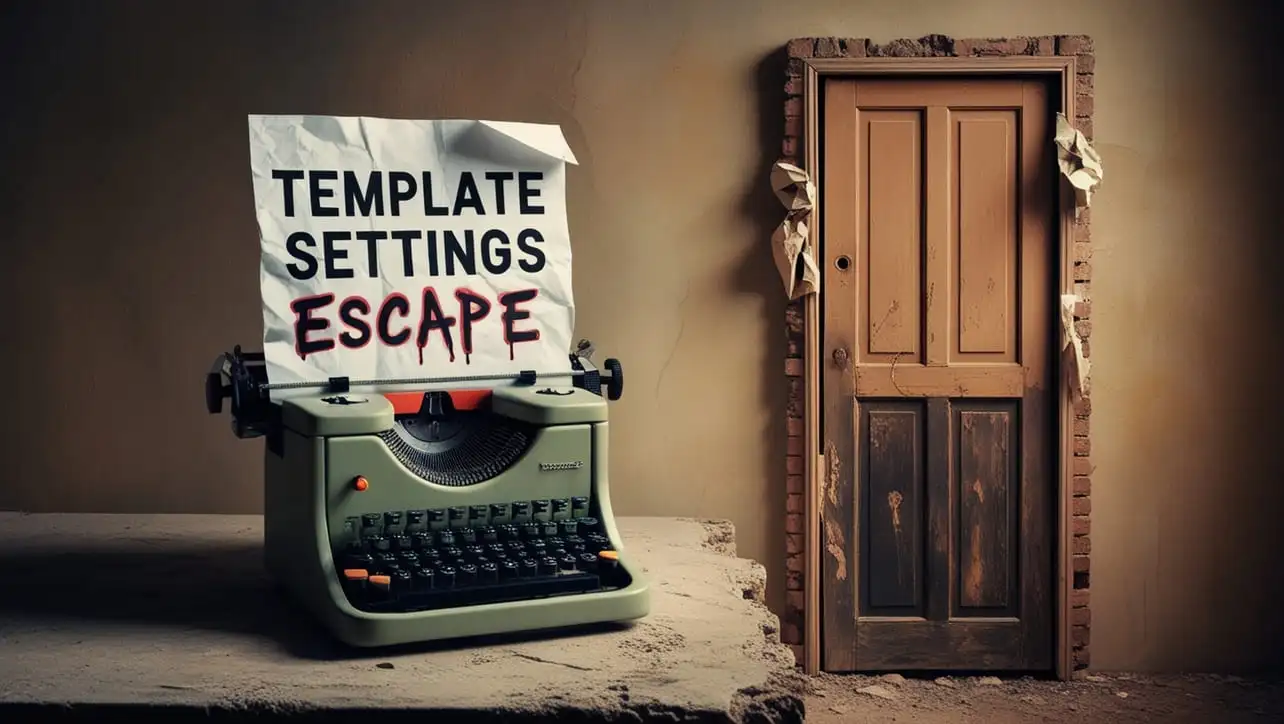
Lodash _.templateSettings.escape Property
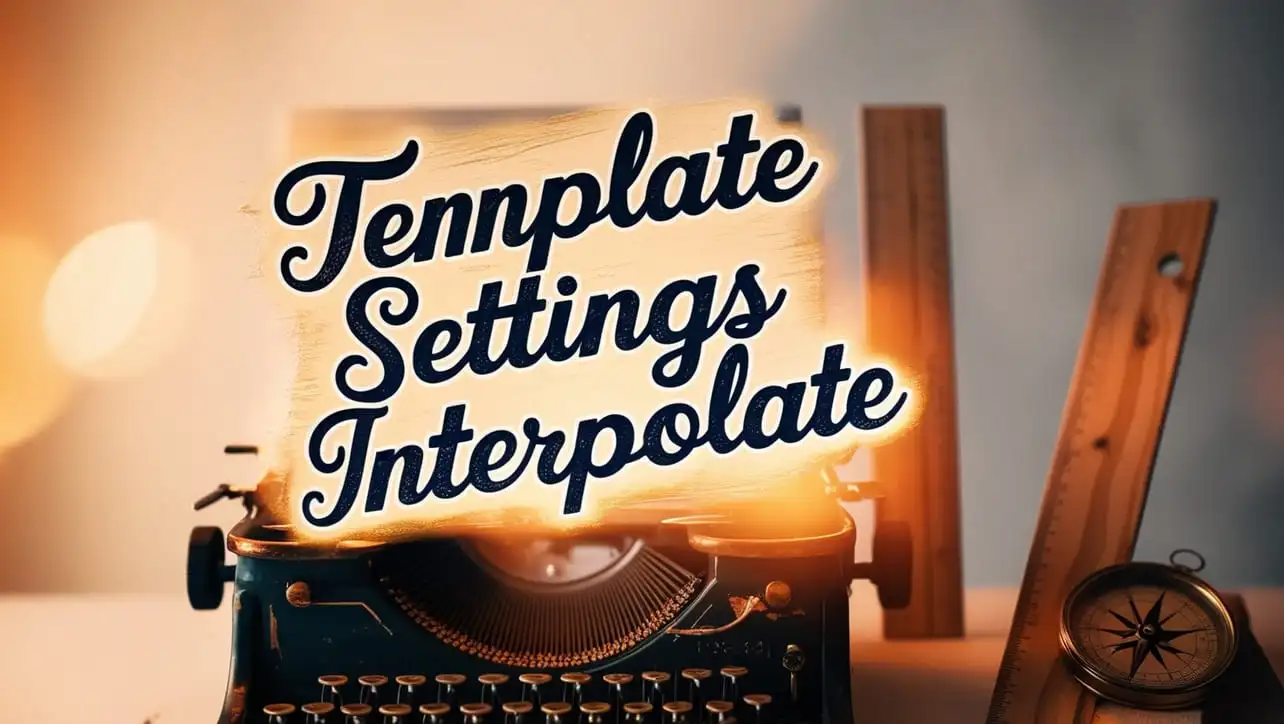
Lodash _.templateSettings.interpolate Property
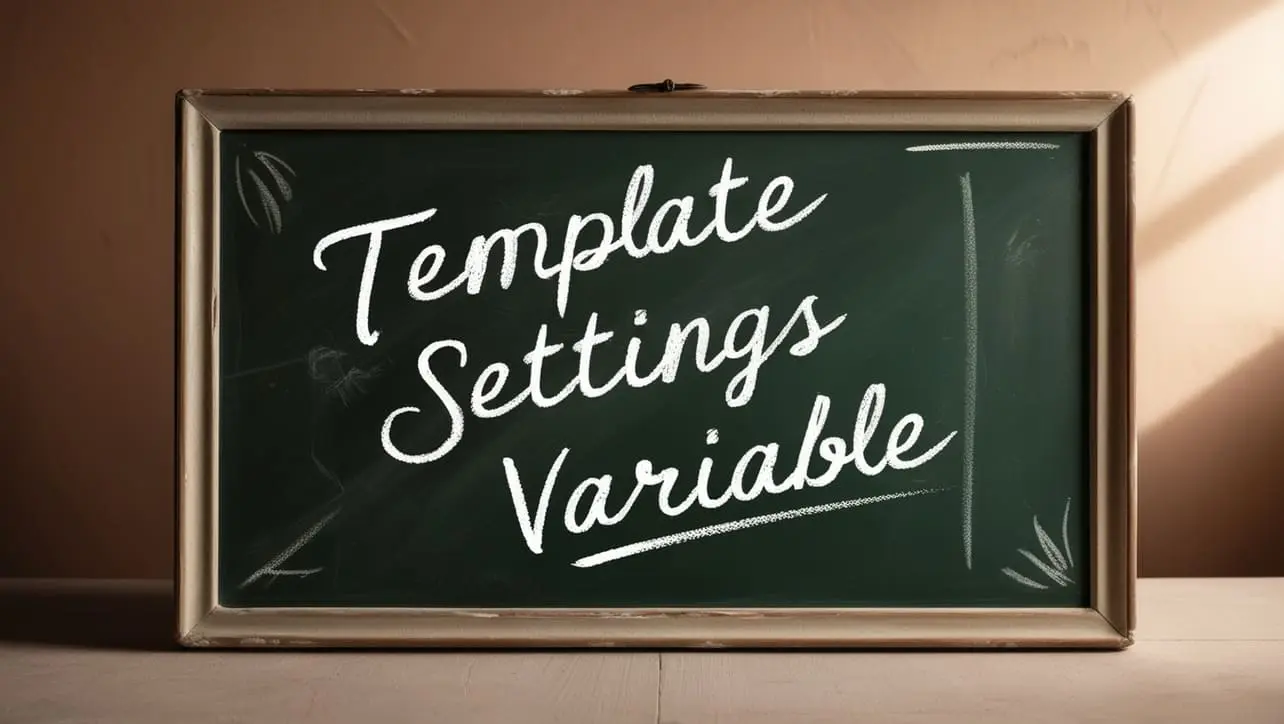
If you have any doubts regarding this article (Lodash _.toUpper() String Method), please comment here. I will help you immediately.