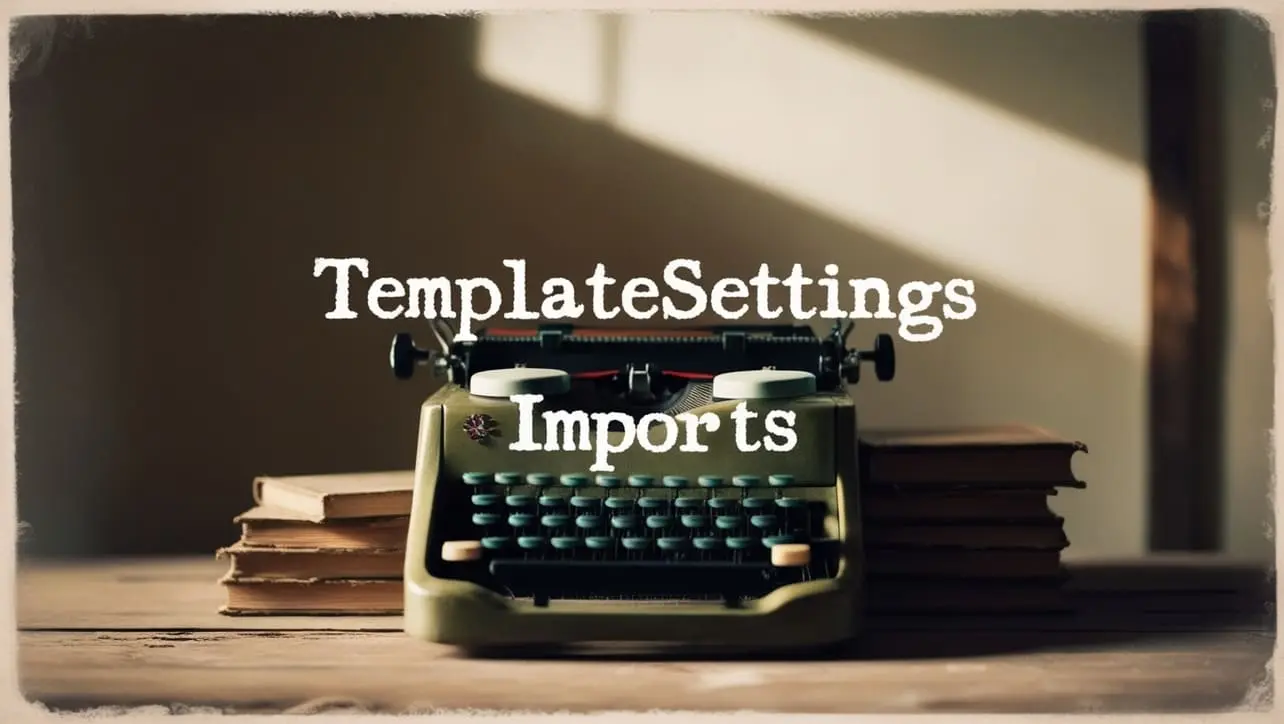
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.toLower() String Method
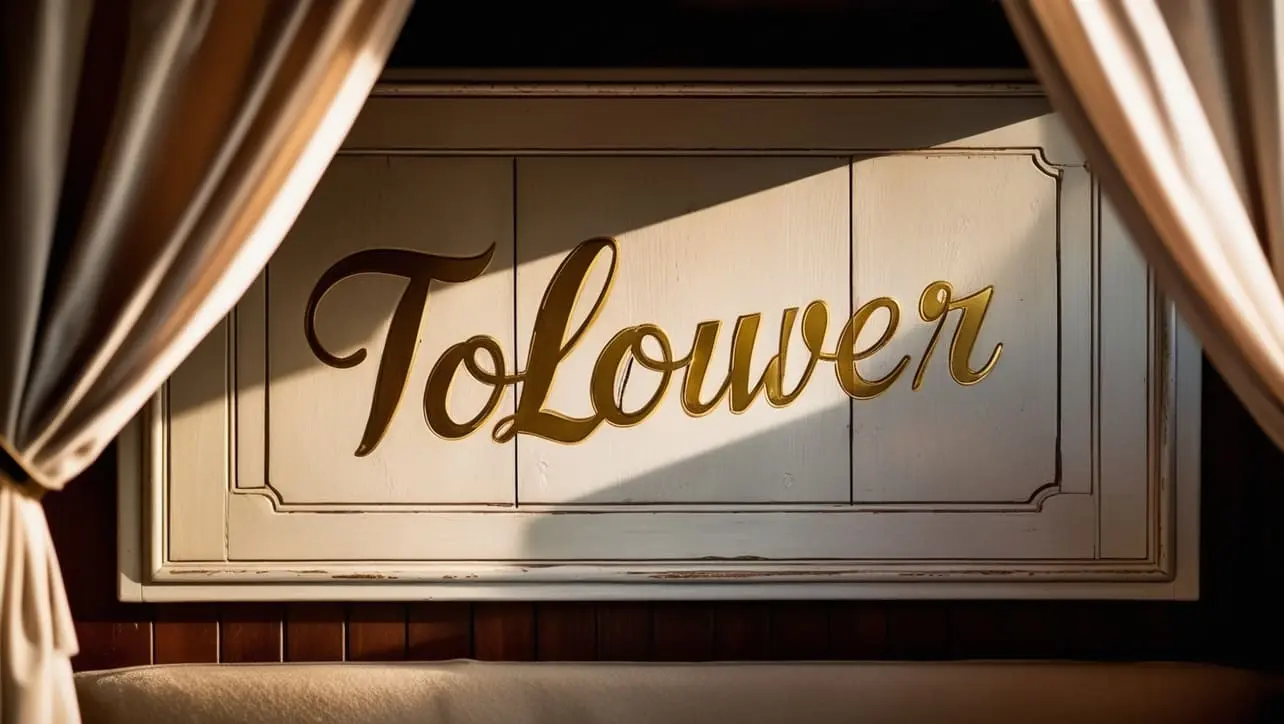
Photo Credit to CodeToFun
π Introduction
String manipulation is a common task in JavaScript development, and having efficient tools at your disposal can greatly streamline your workflow. Enter Lodash, a utility library renowned for its comprehensive set of functions. Among these functions lies the _.toLower()
method, a simple yet powerful tool for converting strings to lowercase.
This method enhances code readability and consistency, making it a valuable asset for developers working with text data.
π§ Understanding _.toLower() Method
The _.toLower()
method in Lodash is straightforward: it converts all characters in a string to lowercase. While this functionality may seem basic, it provides a convenient and reliable solution for ensuring uniformity in string casing across your applications.
π‘ Syntax
The syntax for the _.toLower()
method is straightforward:
_.toLower(string)
- string: The string to convert to lowercase.
π Example
Let's dive into a simple example to illustrate the usage of the _.toLower()
method:
const _ = require('lodash');
const originalString = 'Hello World';
const lowercasedString = _.toLower(originalString);
console.log(lowercasedString);
// Output: 'hello world'
In this example, the originalString is converted to lowercase using _.toLower()
, resulting in hello world.
π Best Practices
When working with the _.toLower()
method, consider the following best practices:
Consistent Casing:
Maintaining consistent casing throughout your application is essential for readability and code consistency. Use
_.toLower()
to ensure uniformity in string casing, especially when dealing with user input or external data sources.example.jsCopiedconst userInput = /* ...get user input... */; const formattedInput = _.toLower(userInput); console.log(formattedInput);
Case-Insensitive Comparisons:
When performing string comparisons, converting strings to lowercase using
_.toLower()
can simplify the process by making the comparison case-insensitive. This helps avoid issues stemming from differences in casing.example.jsCopiedconst inputString = /* ...get input string... */; const targetString = 'example'; if (_.toLower(inputString) === _.toLower(targetString)) { console.log('Strings match (case-insensitive)'); } else { console.log('Strings do not match'); }
Internationalization Considerations:
Keep in mind that
_.toLower()
operates based on Unicode characters, ensuring compatibility with various languages and character sets. This makes it suitable for internationalization scenarios where consistent casing is crucial.example.jsCopiedconst unicodeString = 'Δ°stanbul'; const lowercasedUnicodeString = _.toLower(unicodeString); console.log(lowercasedUnicodeString); // Output: 'iΜstanbul'
π Use Cases
Normalizing Input:
When processing user input or data from external sources, normalizing strings to lowercase using
_.toLower()
can help standardize the data format, simplifying subsequent operations and comparisons.example.jsCopiedconst userInput = /* ...get user input... */; const normalizedInput = _.toLower(userInput); console.log(normalizedInput);
String Matching:
For tasks involving string matching or searching, converting strings to lowercase ensures that case differences do not affect the outcome of the operation. This promotes robust and reliable string handling in your applications.
example.jsCopiedconst searchString = /* ...get search string... */ ; const targetString = /* ...get target string... */ ; if(_.toLower(targetString).includes(_.toLower(searchString))) { console.log('Search string found in target string'); } else { console.log('Search string not found'); }
Data Transformation:
In data transformation pipelines or text processing workflows,
_.toLower()
can be applied to standardize the casing of strings, facilitating seamless data integration and analysis.example.jsCopiedconst dataToProcess = /* ...retrieve data to process... */ ; const processedData = dataToProcess.map(item => ({ ...item, name: _.toLower(item.name), // Convert name to lowercase })); console.log(processedData);
π Conclusion
The _.toLower()
method in Lodash offers a convenient and reliable solution for converting strings to lowercase, promoting consistency and readability in your JavaScript applications. Whether you're normalizing user input, performing case-insensitive comparisons, or standardizing string casing, _.toLower()
provides a versatile tool for string manipulation tasks.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.toLower()
method in your Lodash projects.
π¨βπ» Join our Community:
Author
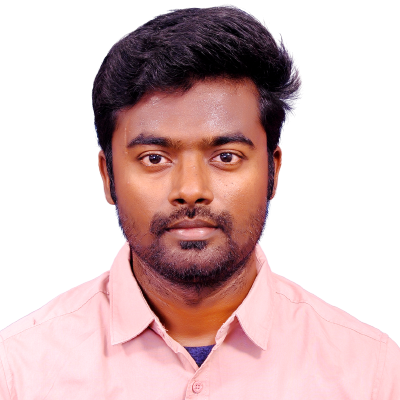
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
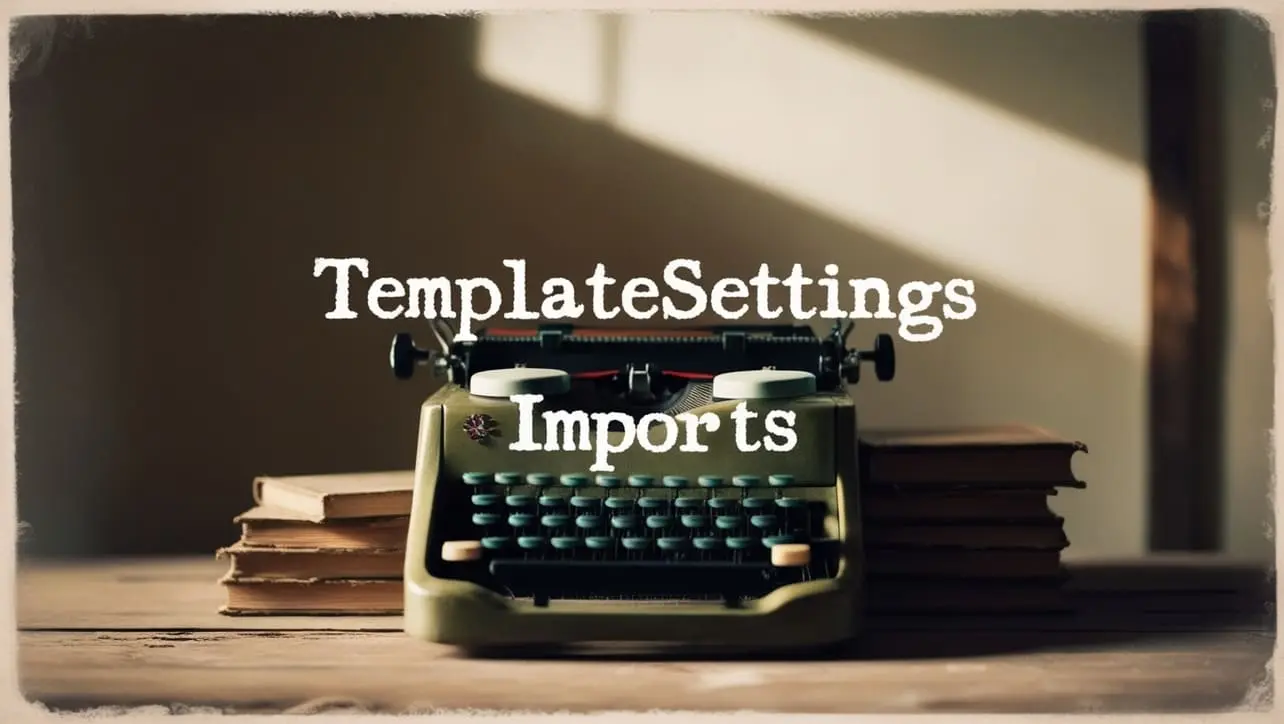
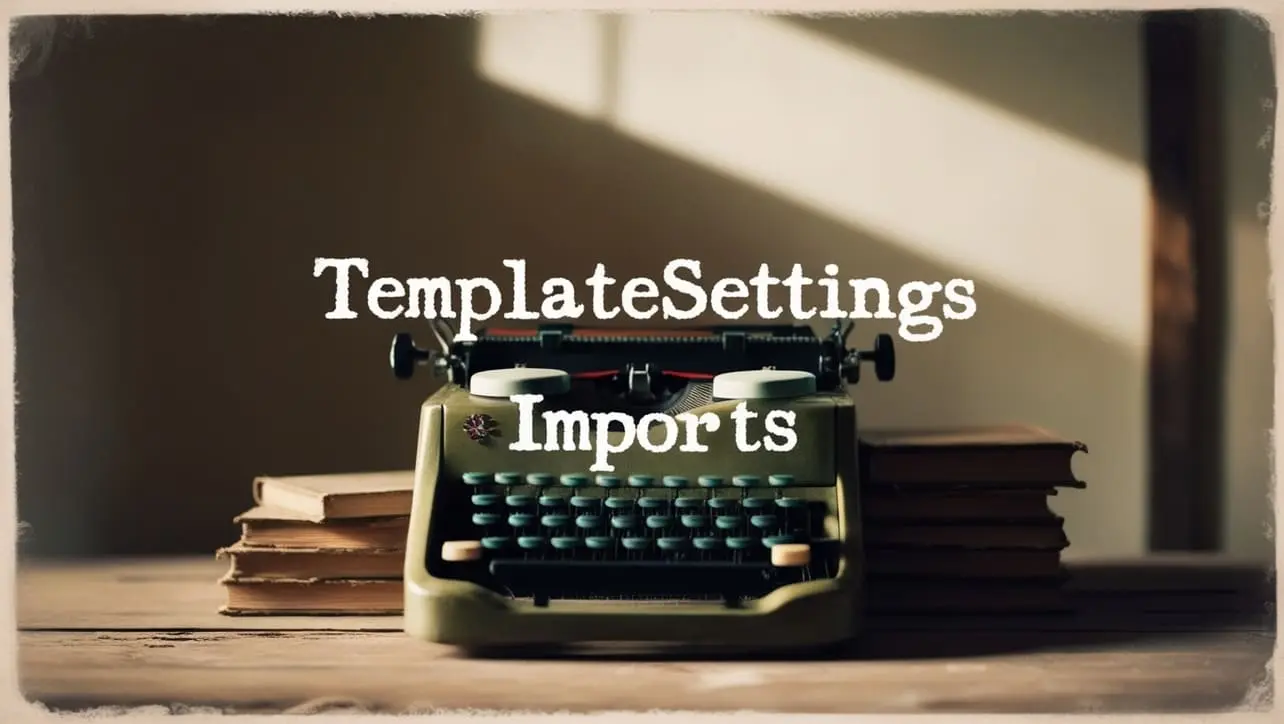
Lodash _.templateSettings.imports Property
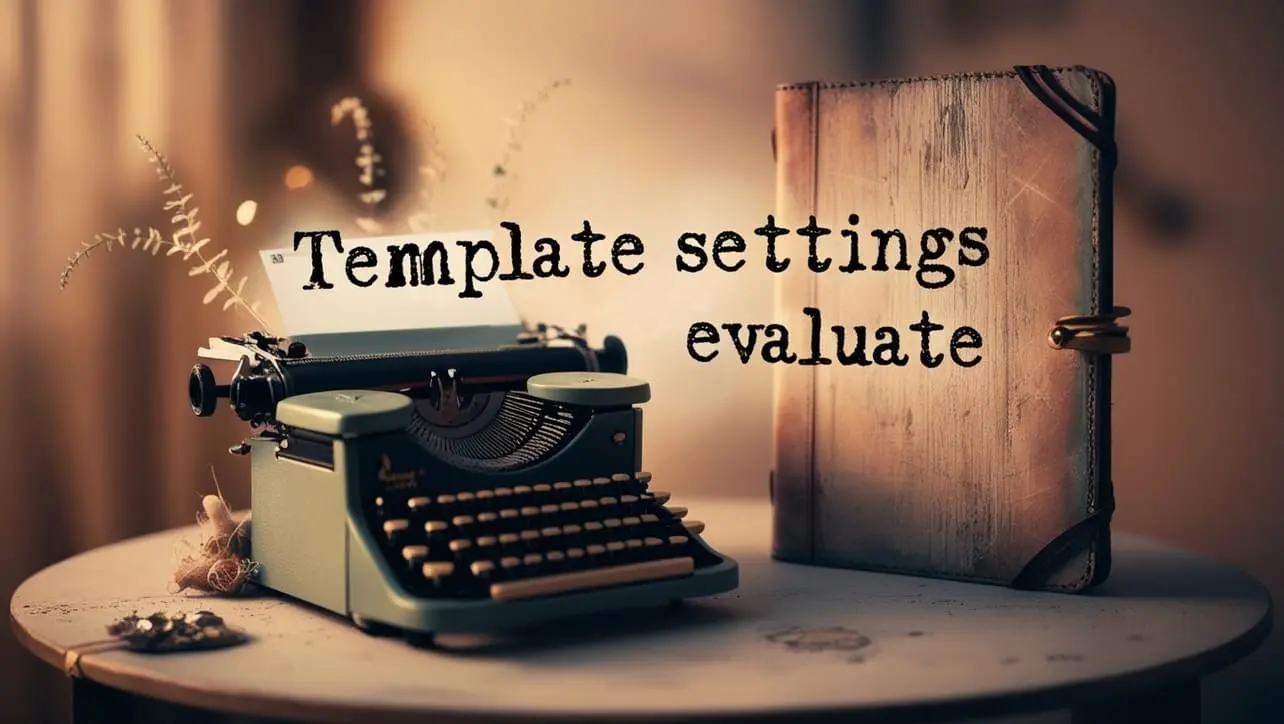
Lodash _.templateSettings.evaluate Property
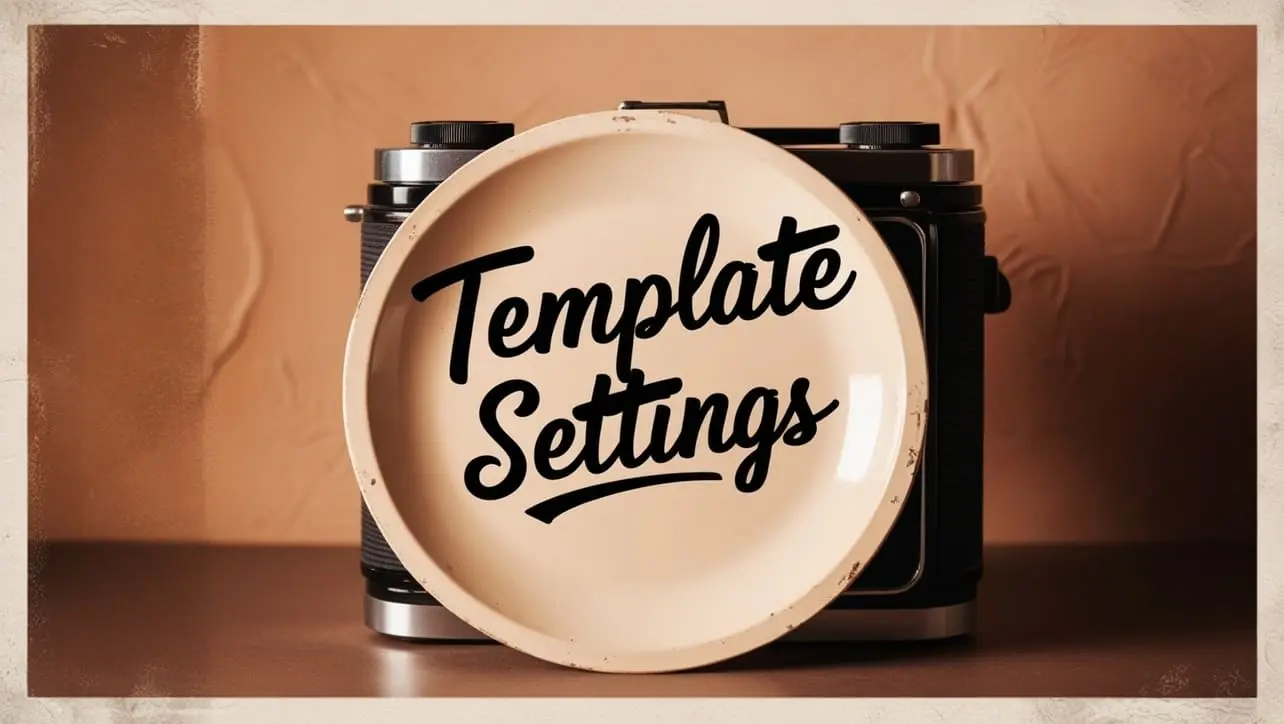
Lodash _.templateSettings Property
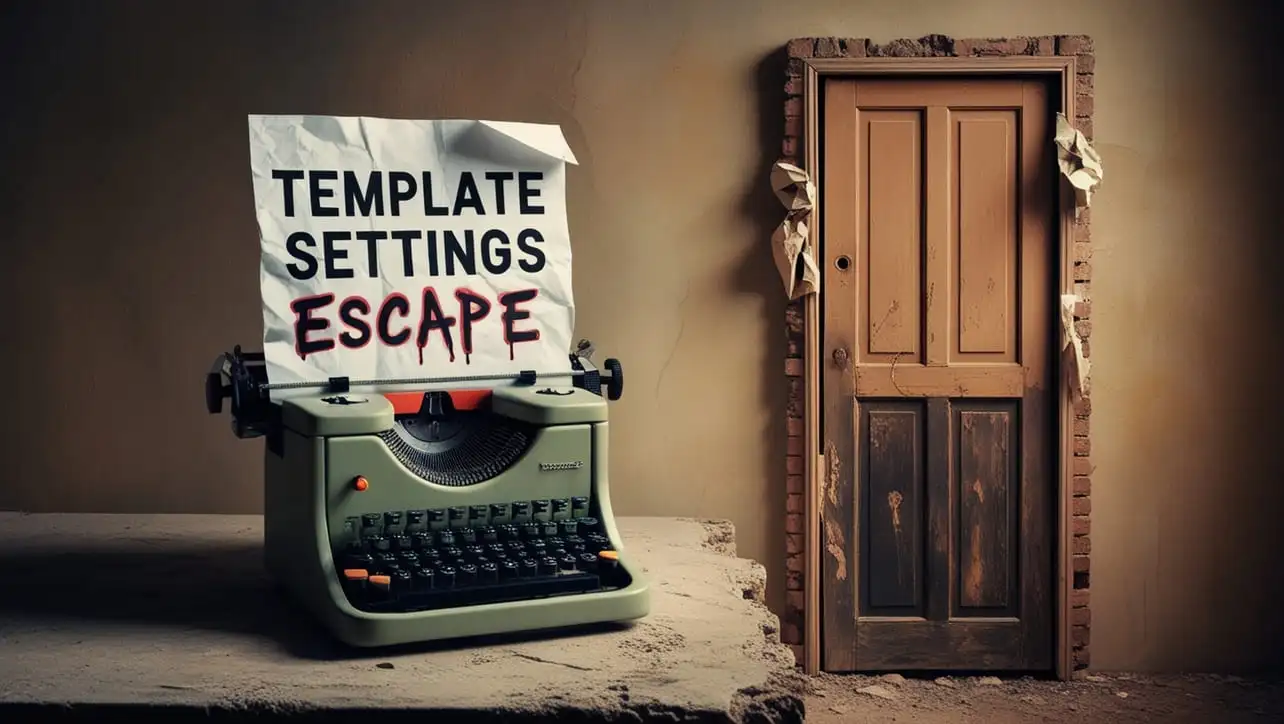
Lodash _.templateSettings.escape Property
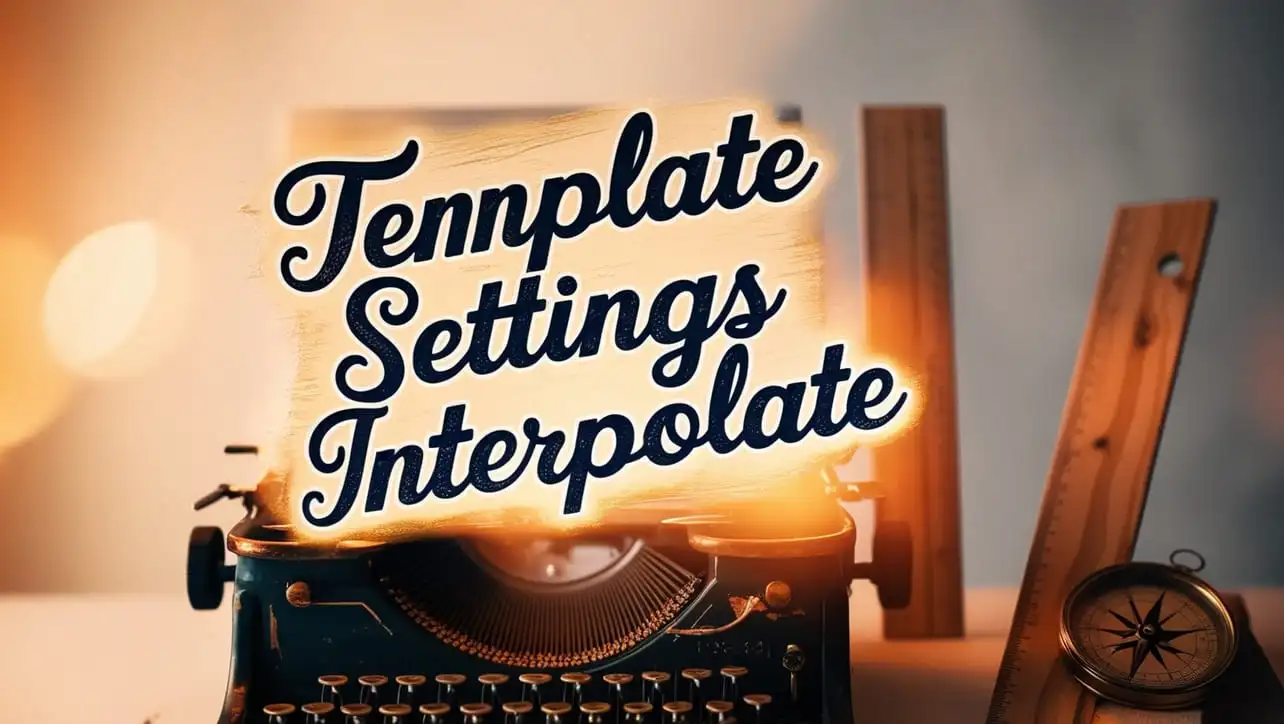
Lodash _.templateSettings.interpolate Property
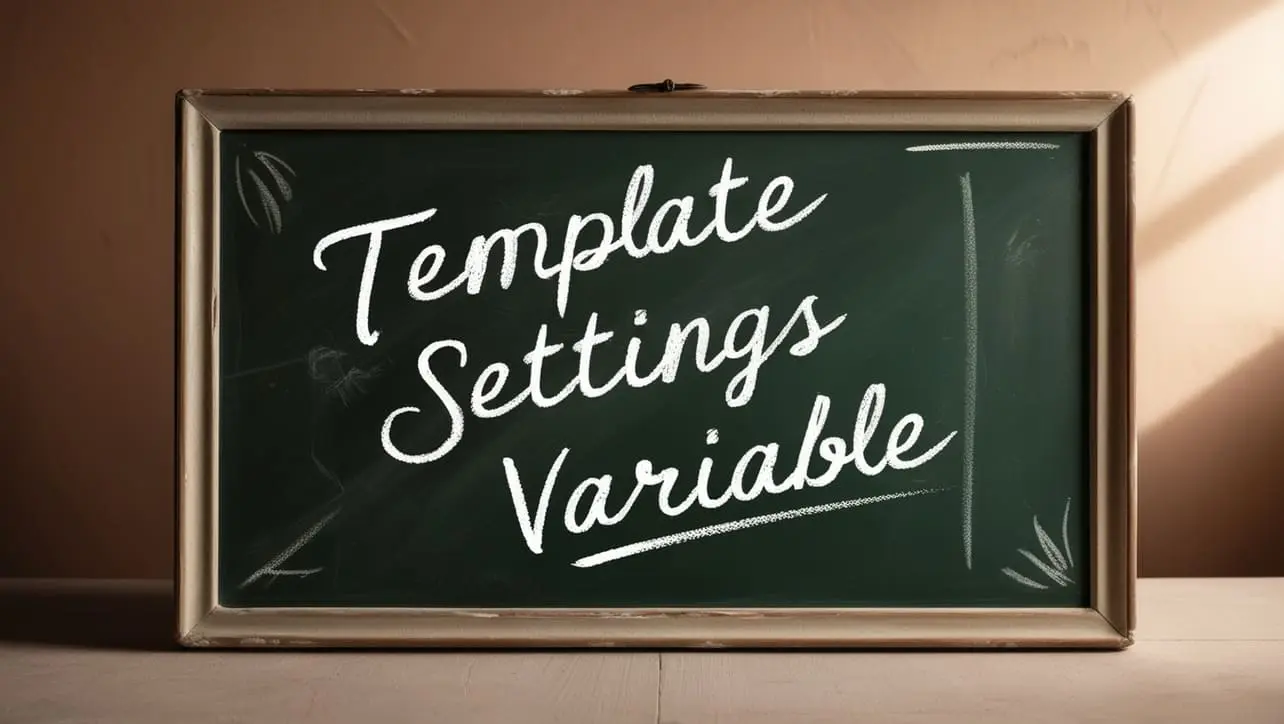
If you have any doubts regarding this article (Lodash _.toLower() String Method), please comment here. I will help you immediately.