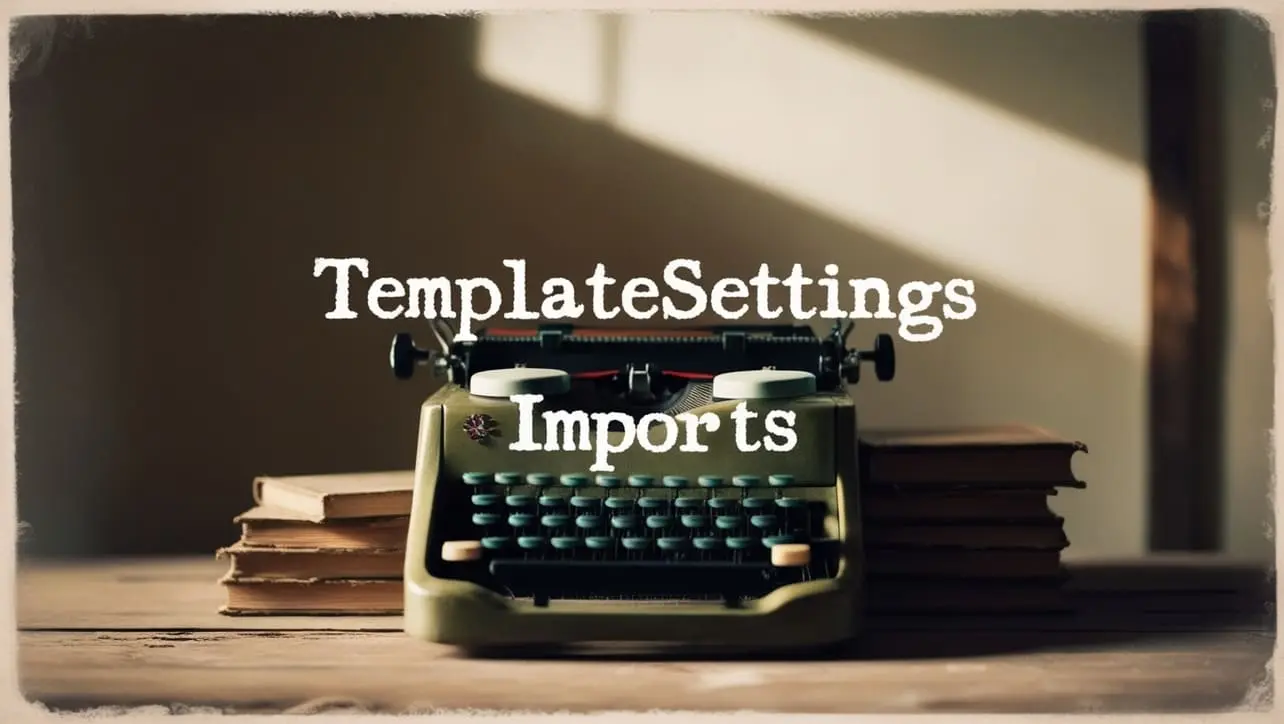
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.startCase() String Method
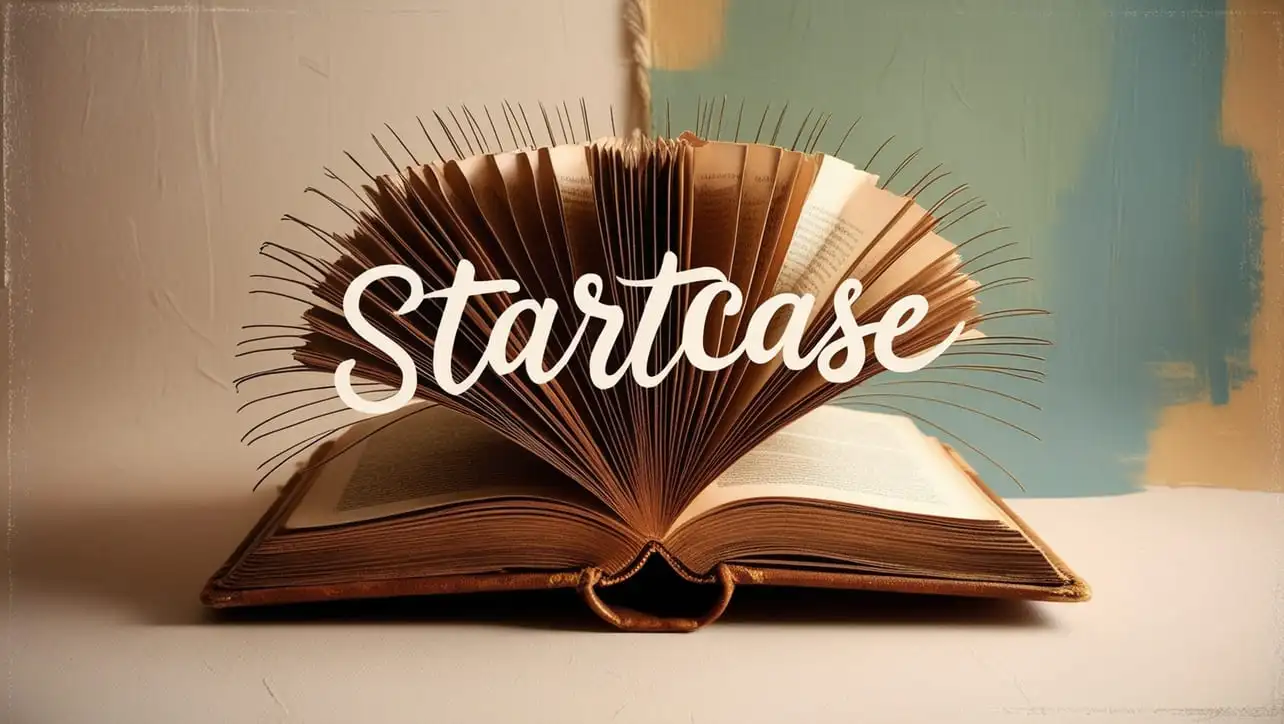
Photo Credit to CodeToFun
🙋 Introduction
String manipulation is a common task in JavaScript development, and Lodash provides a plethora of utility functions to simplify such operations. Among these functions is _.startCase()
, a method designed to convert a string into a new string with the first letter of each word capitalized.
This method enhances code readability and is particularly useful when formatting user input or generating user-friendly labels.
🧠 Understanding _.startCase() Method
The _.startCase()
method in Lodash transforms a string by capitalizing the first letter of each word and converting the rest of the characters to lowercase. This results in a string where each word is properly capitalized, making it suitable for display purposes.
💡 Syntax
The syntax for the _.startCase()
method is straightforward:
_.startCase([string=''])
- string: The string to transform (default is an empty string).
📝 Example
Let's dive into a simple example to illustrate the usage of the _.startCase()
method:
const _ = require('lodash');
const originalString = 'hello world';
const formattedString = _.startCase(originalString);
console.log(formattedString);
// Output: 'Hello World'
In this example, the originalString is transformed into formattedString using _.startCase()
, resulting in proper capitalization of each word.
🏆 Best Practices
When working with the _.startCase()
method, consider the following best practices:
Handling Special Characters:
Take into consideration how
_.startCase()
handles special characters, such as hyphens or underscores. These characters may affect the capitalization of adjacent words and should be handled appropriately.example.jsCopiedconst stringWithSpecialCharacters = 'first_name last-name'; const formattedString = _.startCase(stringWithSpecialCharacters); console.log(formattedString); // Output: 'First Name Last Name'
Preserving Acronyms:
Be mindful of preserving acronyms or abbreviations within the string.
_.startCase()
capitalizes the first letter of each word, which may inadvertently capitalize letters within acronyms if not handled correctly.example.jsCopiedconst stringWithAcronyms = 'united states of america (usa)'; const formattedString = _.startCase(stringWithAcronyms); console.log(formattedString); // Output: 'United States Of America (Usa)'
Error Handling:
Implement error handling for scenarios where the input string is empty or null. Depending on your application's requirements, you may choose to return a default value or provide feedback to the user.
example.jsCopiedconst emptyString = ''; const defaultString = _.startCase(emptyString || 'default'); console.log(defaultString); // Output: 'Default'
📚 Use Cases
Formatting User Input:
When collecting user input,
_.startCase()
can be used to format text fields to ensure consistency and improve readability in the user interface.example.jsCopiedconst userInput = 'john_doe@example.com'; const formattedInput = _.startCase(userInput); console.log(formattedInput); // Output: 'John Doe@Example Com'
Generating User-Friendly Labels:
In applications where labels or headings are dynamically generated,
_.startCase()
can be applied to ensure that the text is properly formatted for display.example.jsCopiedconst dynamicLabel = 'customer_firstname'; const formattedLabel = _.startCase(dynamicLabel); console.log(formattedLabel); // Output: 'Customer Firstname'
Displaying Data in UI Components:
When rendering data in UI components such as tables or cards,
_.startCase()
can enhance the presentation by capitalizing each word in the displayed text.example.jsCopiedconst dataField = 'product_description'; const formattedField = _.startCase(dataField); console.log(formattedField); // Output: 'Product Description'
🎉 Conclusion
The _.startCase()
method in Lodash offers a convenient solution for capitalizing the first letter of each word in a string, improving readability and enhancing the user experience. Whether you're formatting user input, generating labels, or displaying data in UI components, this method provides a versatile tool for string manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.startCase()
method in your Lodash projects.
👨💻 Join our Community:
Author
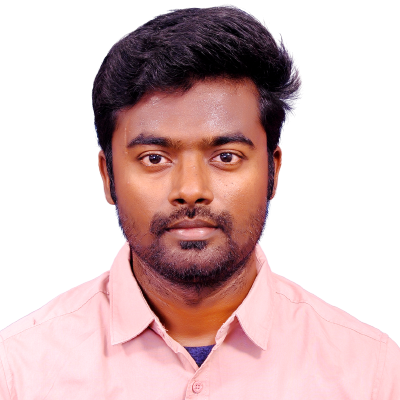
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
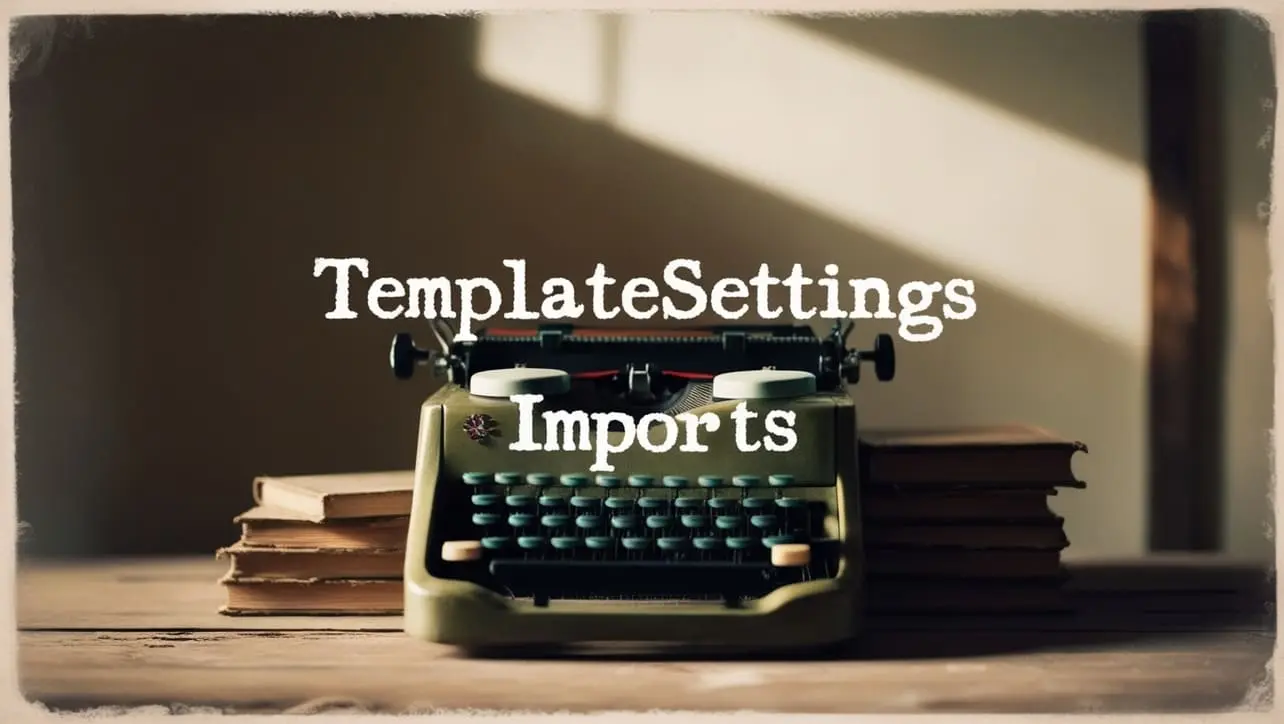
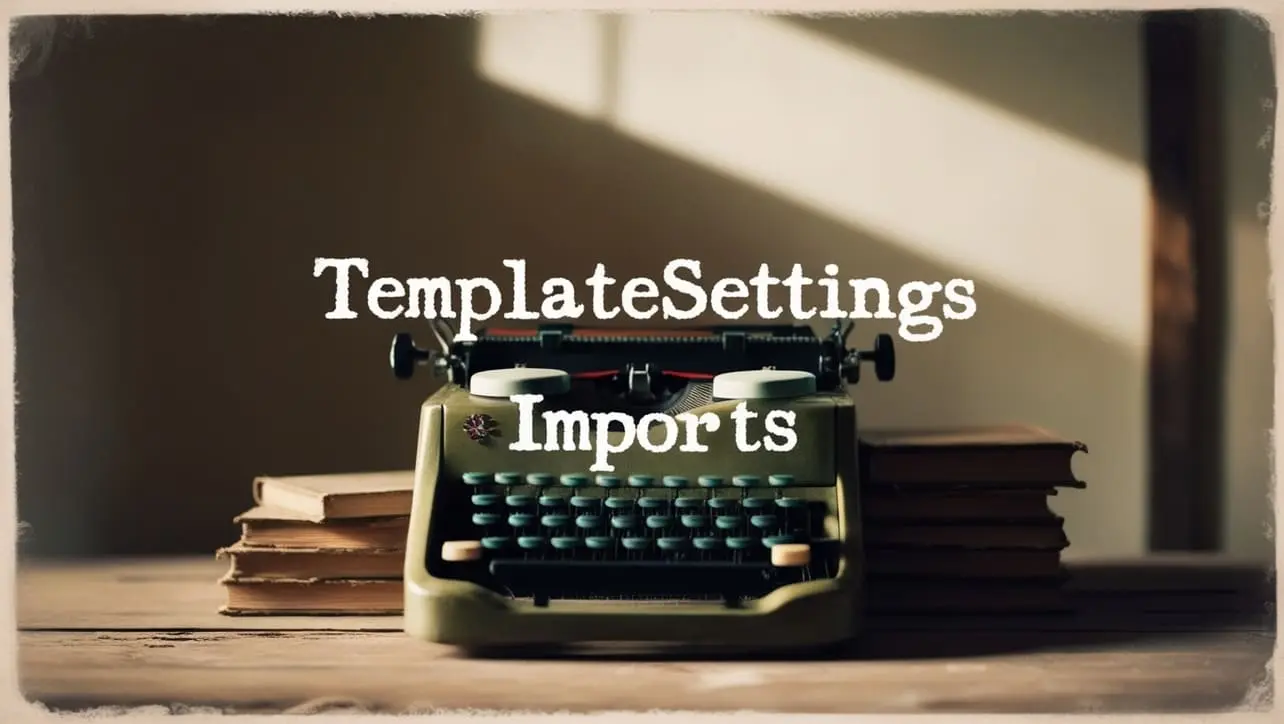
Lodash _.templateSettings.imports Property
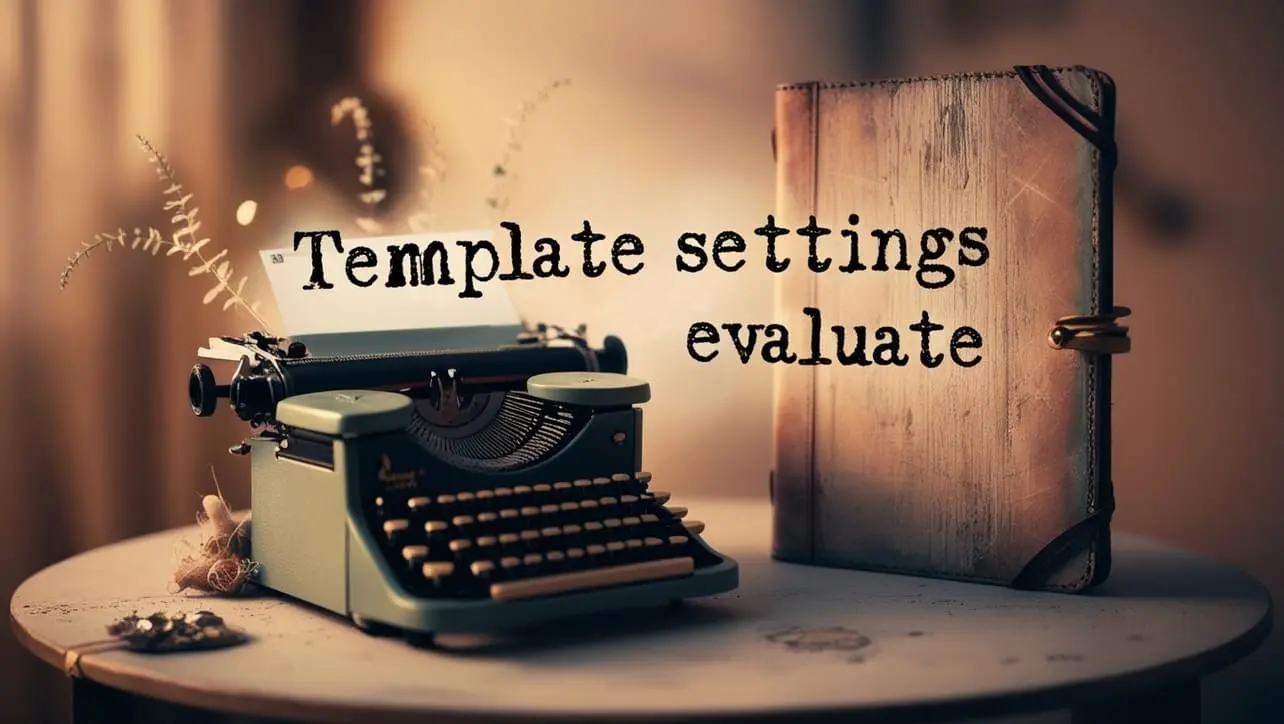
Lodash _.templateSettings.evaluate Property
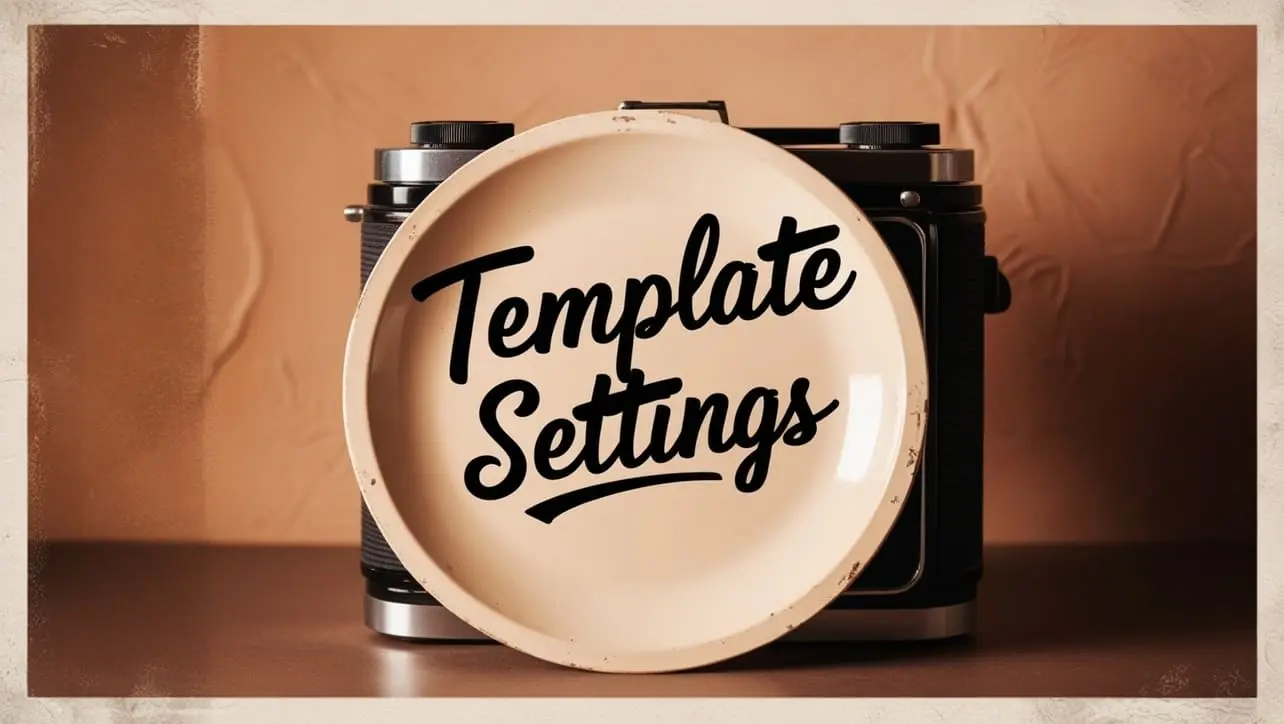
Lodash _.templateSettings Property
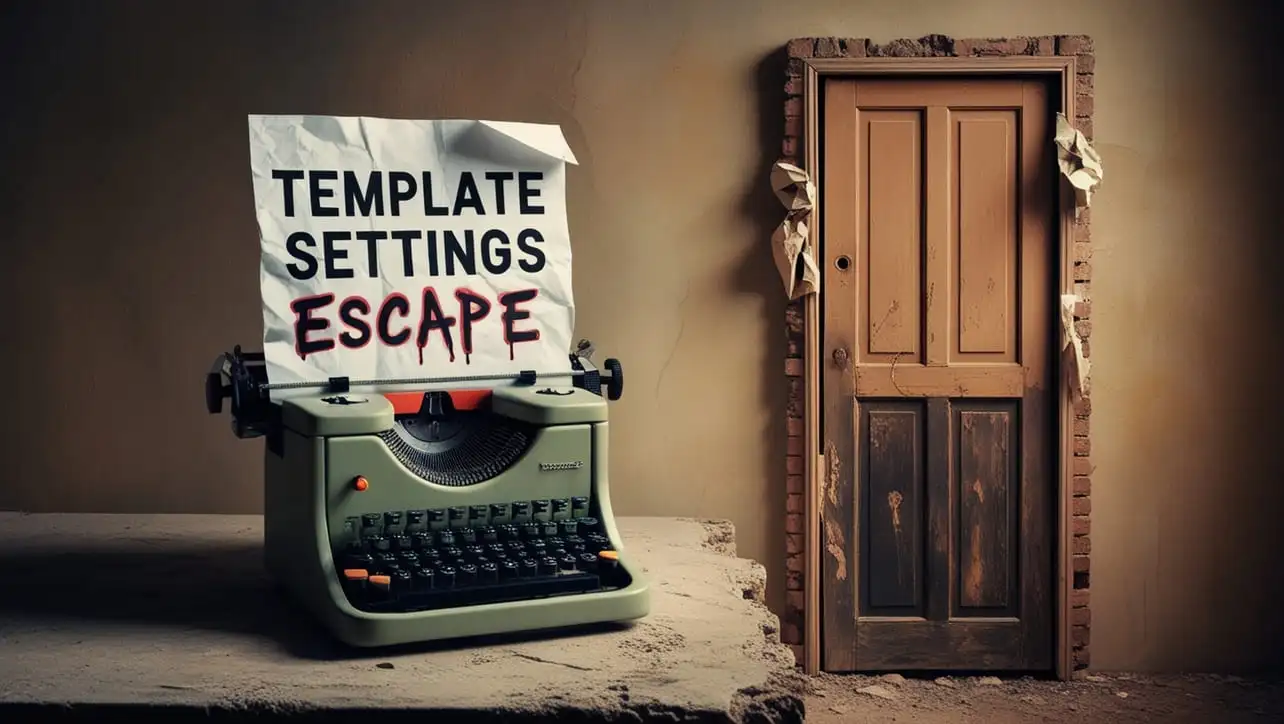
Lodash _.templateSettings.escape Property
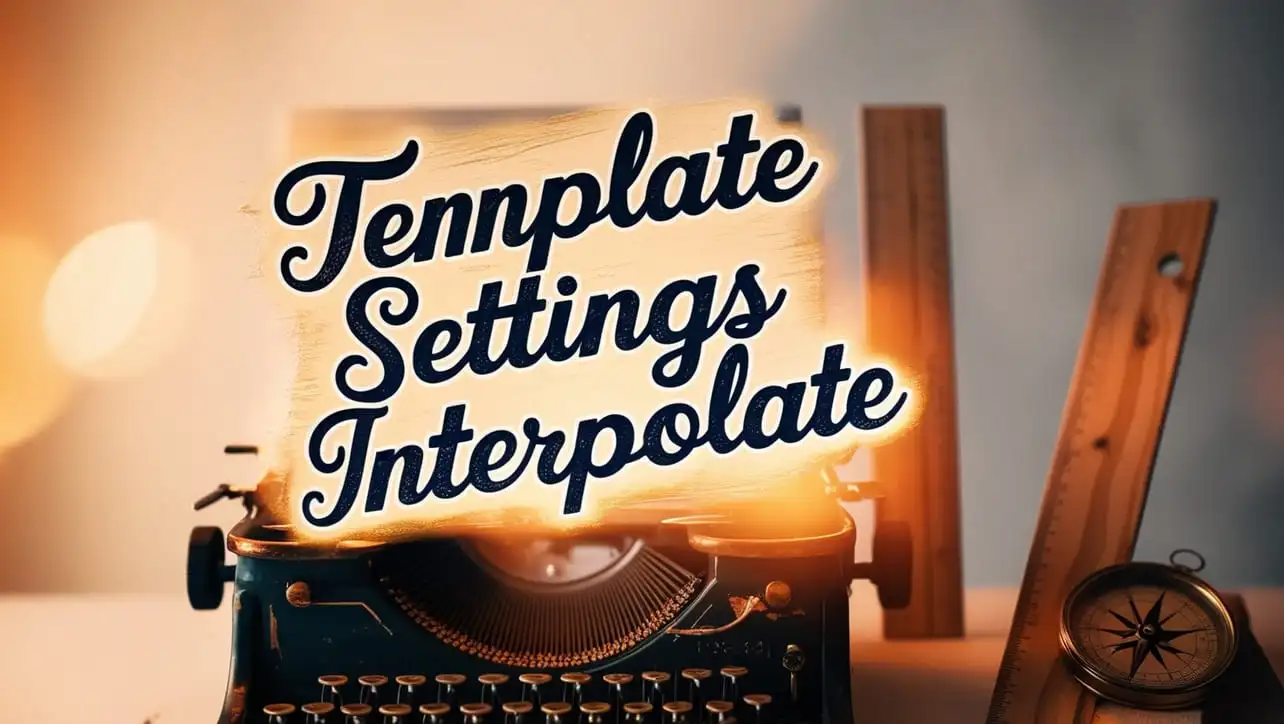
Lodash _.templateSettings.interpolate Property
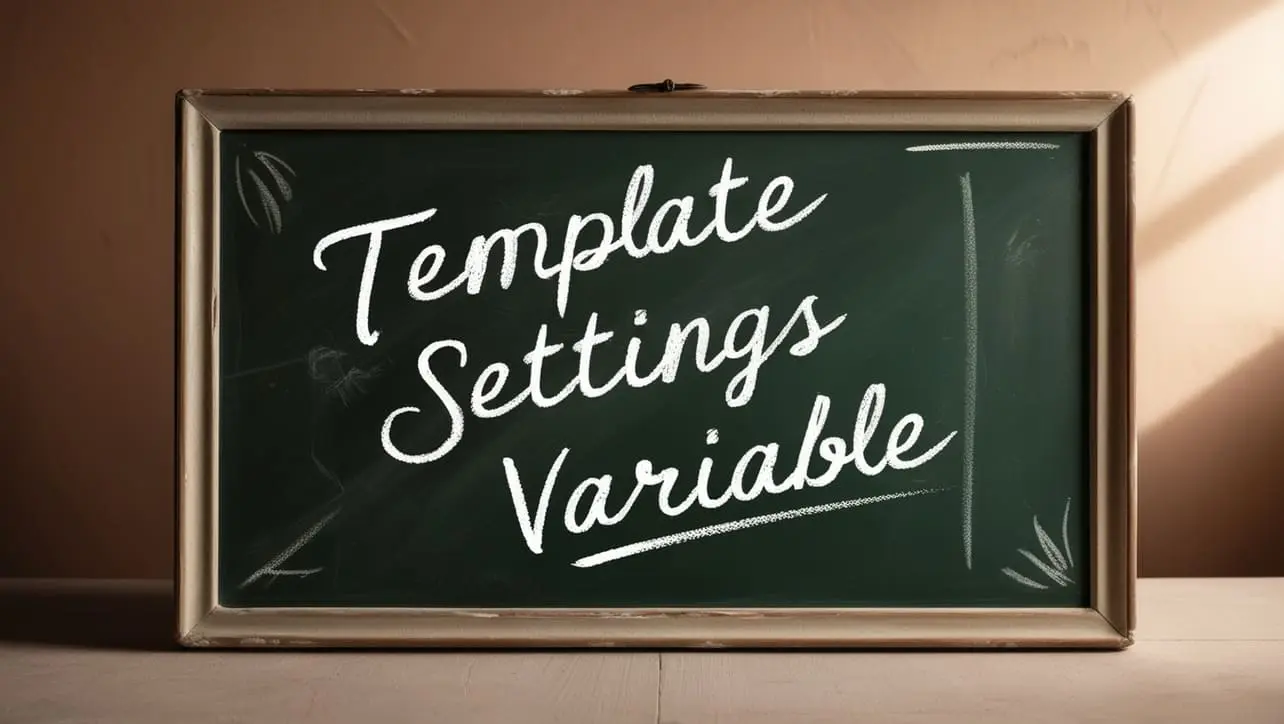
If you have any doubts regarding this article (Lodash _.startCase() String Method), please comment here. I will help you immediately.