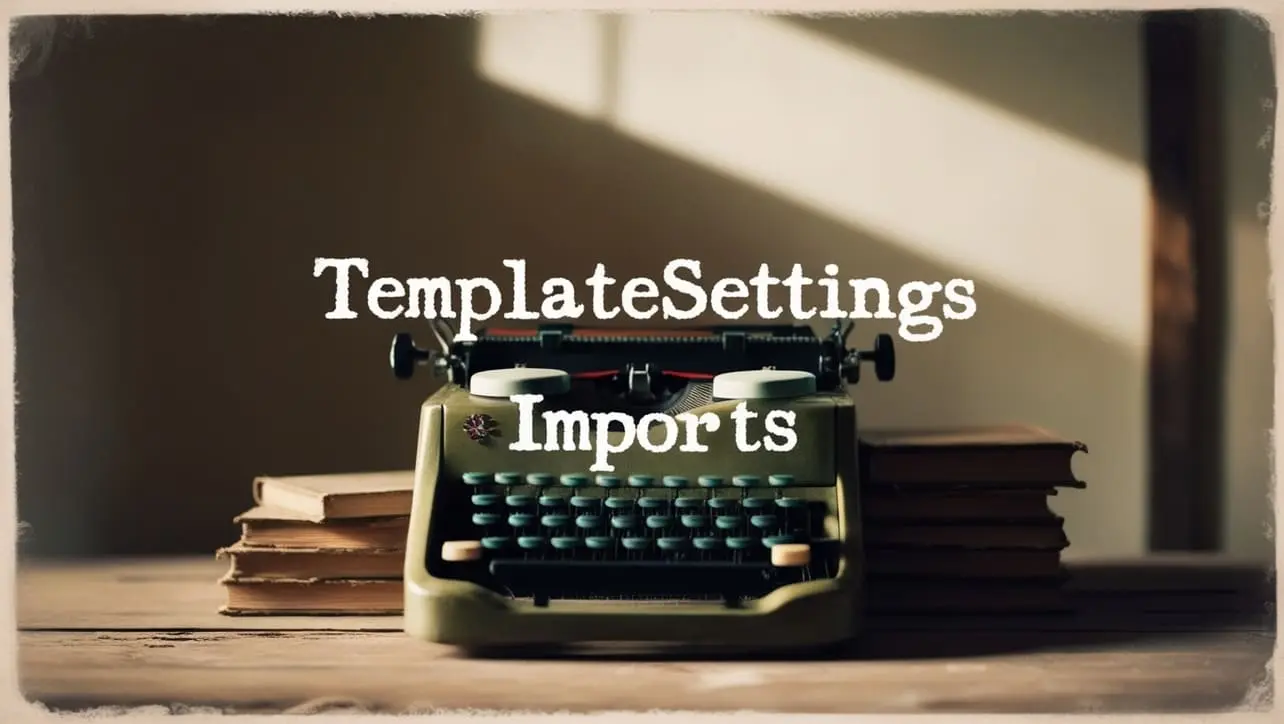
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.snakeCase() String Method
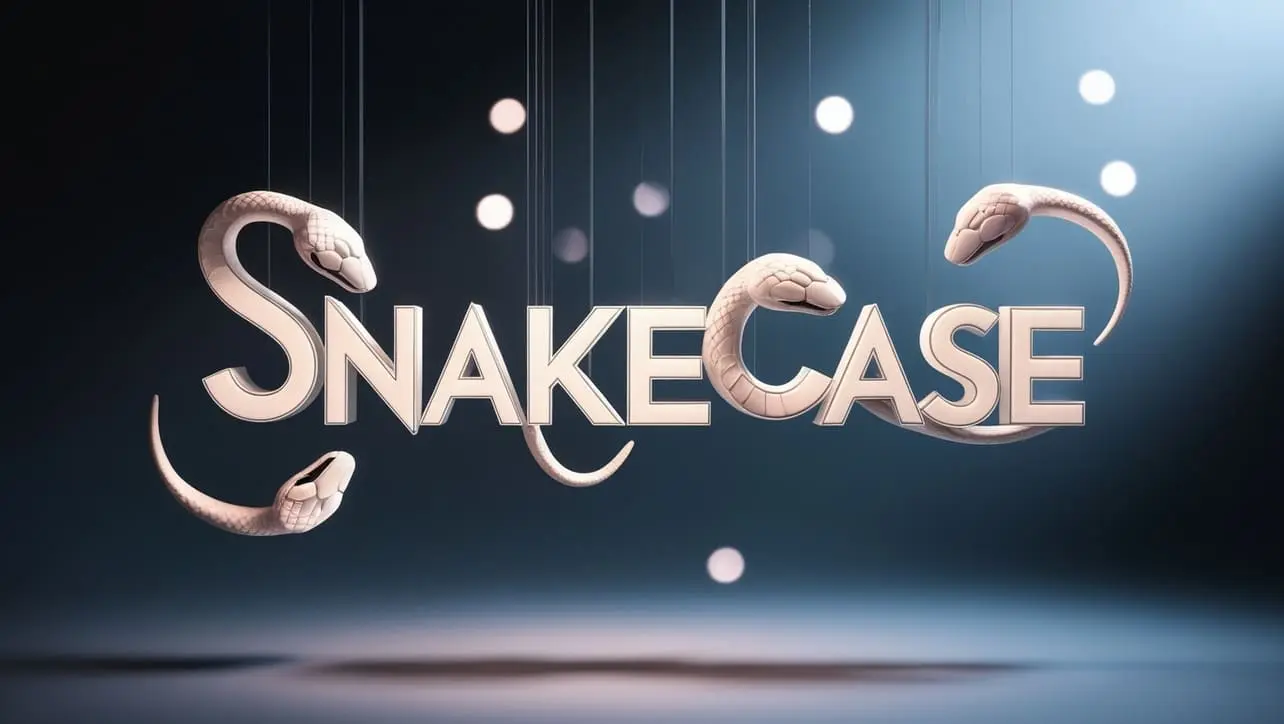
Photo Credit to CodeToFun
🙋 Introduction
String manipulation is a common task in JavaScript development, and the Lodash library provides a wealth of utility functions to streamline this process. Among these functions is _.snakeCase()
, which offers a convenient way to convert strings into snake_case format.
This method is invaluable for developers working with APIs, database fields, or any scenario where consistent formatting is essential.
🧠 Understanding _.snakeCase() Method
The _.snakeCase()
method in Lodash transforms a string into snake_case format, where words are joined by underscores and all letters are lowercase. This format is commonly used for variables, filenames, and URLs, enhancing readability and interoperability across systems.
💡 Syntax
The syntax for the _.snakeCase()
method is straightforward:
_.snakeCase(string)
- string: The string to convert to snake_case format.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.snakeCase()
method:
const _ = require('lodash');
const camelCaseString = 'helloWorldExample';
const snakeCaseString = _.snakeCase(camelCaseString);
console.log(snakeCaseString);
// Output: hello_world_example
In this example, the camelCaseString is converted to snake_case using _.snakeCase()
, resulting in a new string with words separated by underscores and all lowercase letters.
🏆 Best Practices
When working with the _.snakeCase()
method, consider the following best practices:
Consistent Naming Conventions:
Adopt consistent naming conventions across your codebase to improve readability and maintainability. Utilize
_.snakeCase()
to ensure uniformity in variable names, function names, and other identifiers.example.jsCopiedconst variableName = 'someVariableName'; const functionName = 'someFunctionName';
Handle Special Characters:
Be mindful of special characters and whitespace in input strings.
_.snakeCase()
automatically handles spaces and punctuation, ensuring that the resulting string conforms to snake_case format.example.jsCopiedconst stringWithSpecialCharacters = 'Hello, World! How are you?'; const snakeCaseString = _.snakeCase(stringWithSpecialCharacters); console.log(snakeCaseString); // Output: hello_world_how_are_you
Customize Separator:
Explore the possibility of customizing the separator used in snake_case formatting. While underscores are commonly used, you can specify a different separator to suit your requirements using
_.snakeCase()
.example.jsCopiedconst stringWithSpaces = 'hello world example'; const customSeparatorString = _.snakeCase(stringWithSpaces).replace(/_/g, '-'); console.log(customSeparatorString); // Output: hello-world-example
📚 Use Cases
API Endpoint Formatting:
When working with APIs,
_.snakeCase()
can be utilized to format endpoint paths, ensuring consistency and adherence to naming conventions.example.jsCopiedconst endpointName = 'getUserDetails'; const formattedEndpoint = _.snakeCase(endpointName); console.log(`/api/${formattedEndpoint}`); // Output: /api/get_user_details
Database Field Names:
In database interactions,
_.snakeCase()
facilitates the transformation of field names to match database conventions, simplifying queries and maintenance.example.jsCopiedconst fieldName = 'firstName'; const formattedField = _.snakeCase(fieldName); console.log(`SELECT ${formattedField} FROM users`); // Output: SELECT first_name FROM users
File Naming:
When generating filenames dynamically,
_.snakeCase()
ensures that filenames are standardized and easily recognizable.example.jsCopiedconst fileName = 'MyDocumentTitle'; const formattedFileName = _.snakeCase(fileName); console.log(`${formattedFileName}.pdf`); // Output: my_document_title.pdf
🎉 Conclusion
The _.snakeCase()
method in Lodash provides a straightforward solution for converting strings to snake_case format, facilitating consistent naming conventions and enhancing code readability. Whether you're working with variables, API endpoints, or file names, this method offers a convenient way to maintain uniformity and clarity in your JavaScript projects.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.snakeCase()
method in your Lodash projects.
👨💻 Join our Community:
Author
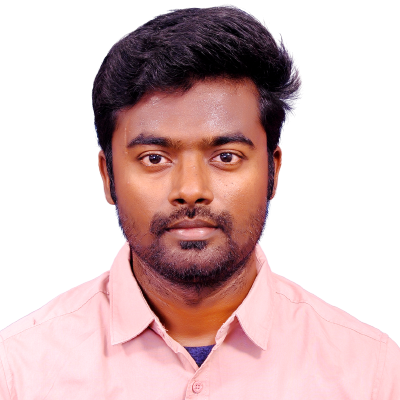
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
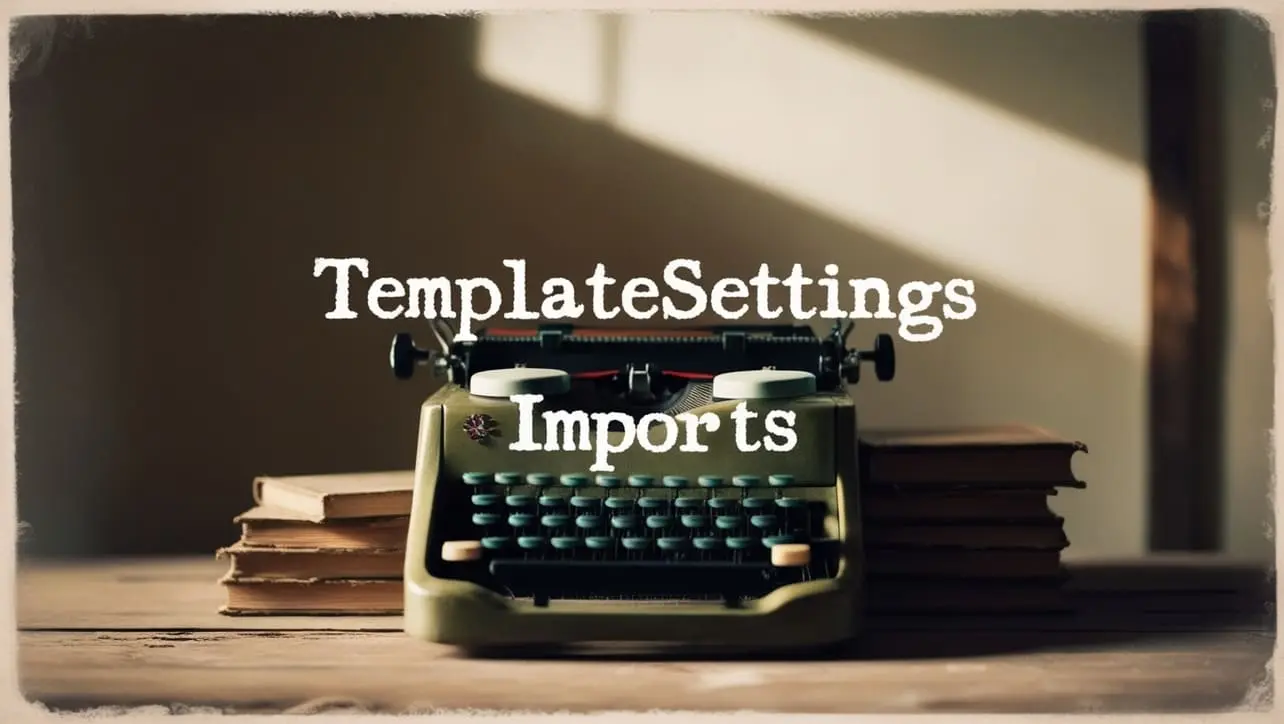
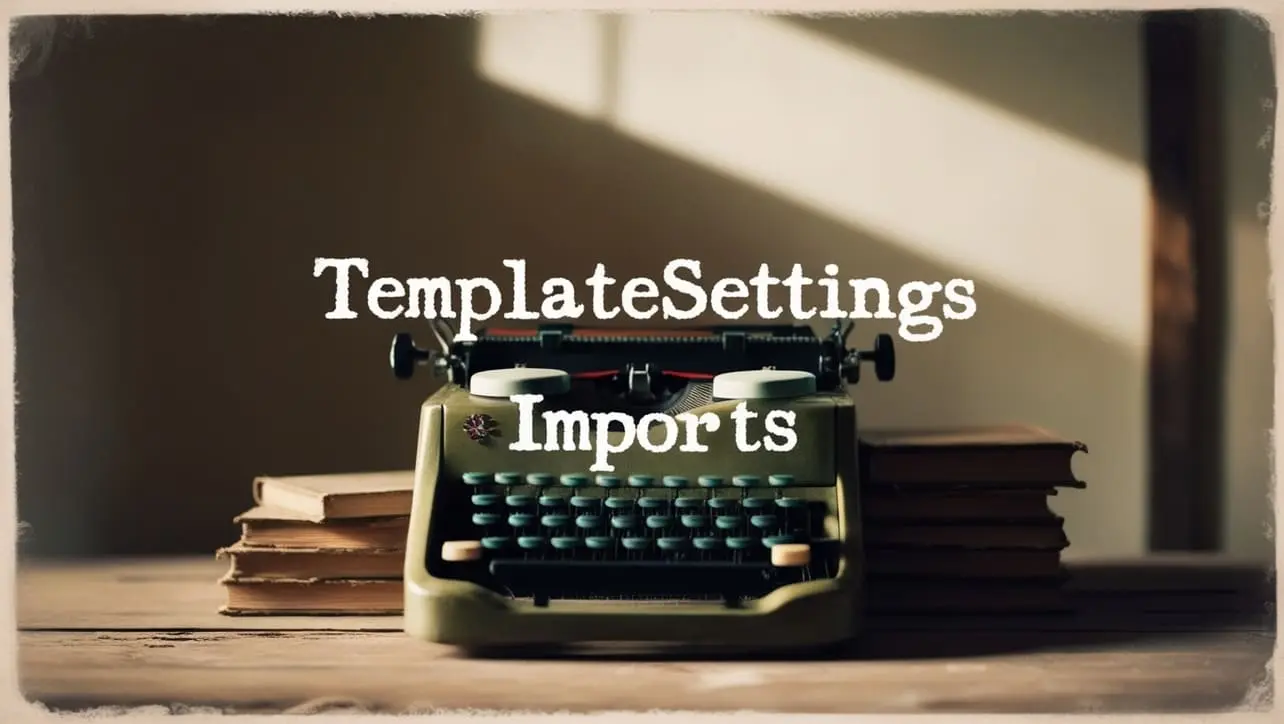
Lodash _.templateSettings.imports Property
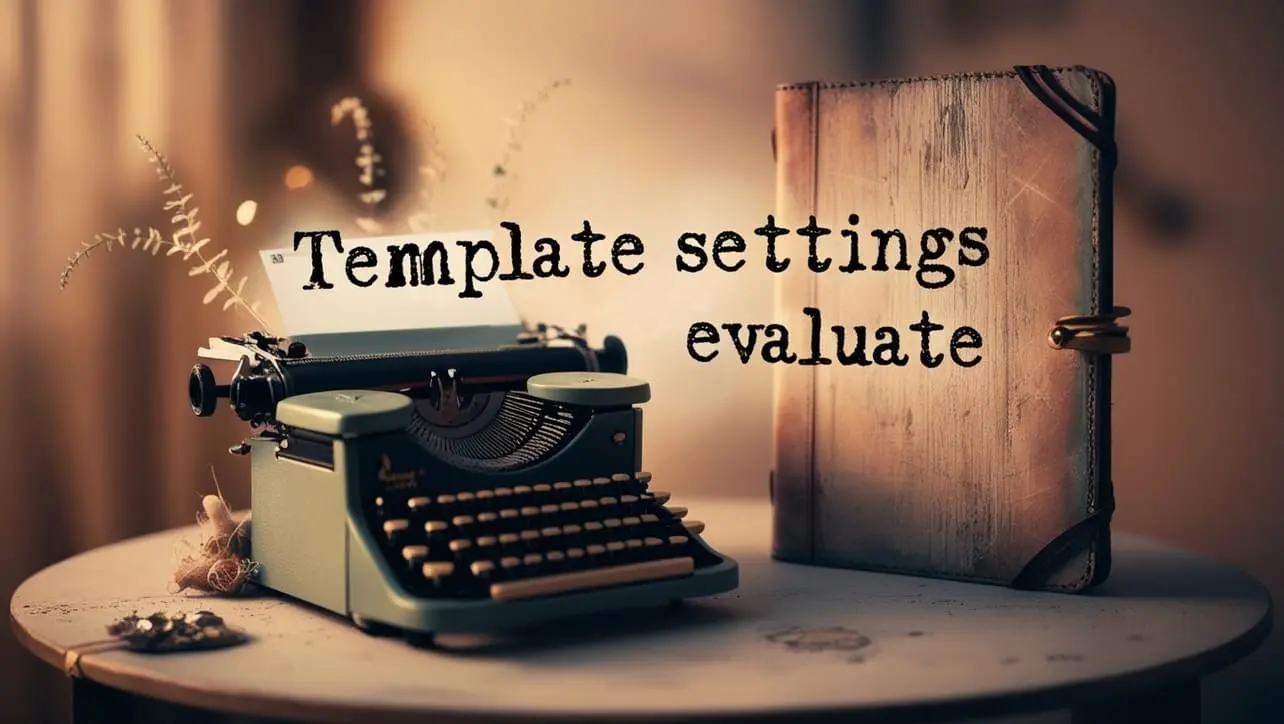
Lodash _.templateSettings.evaluate Property
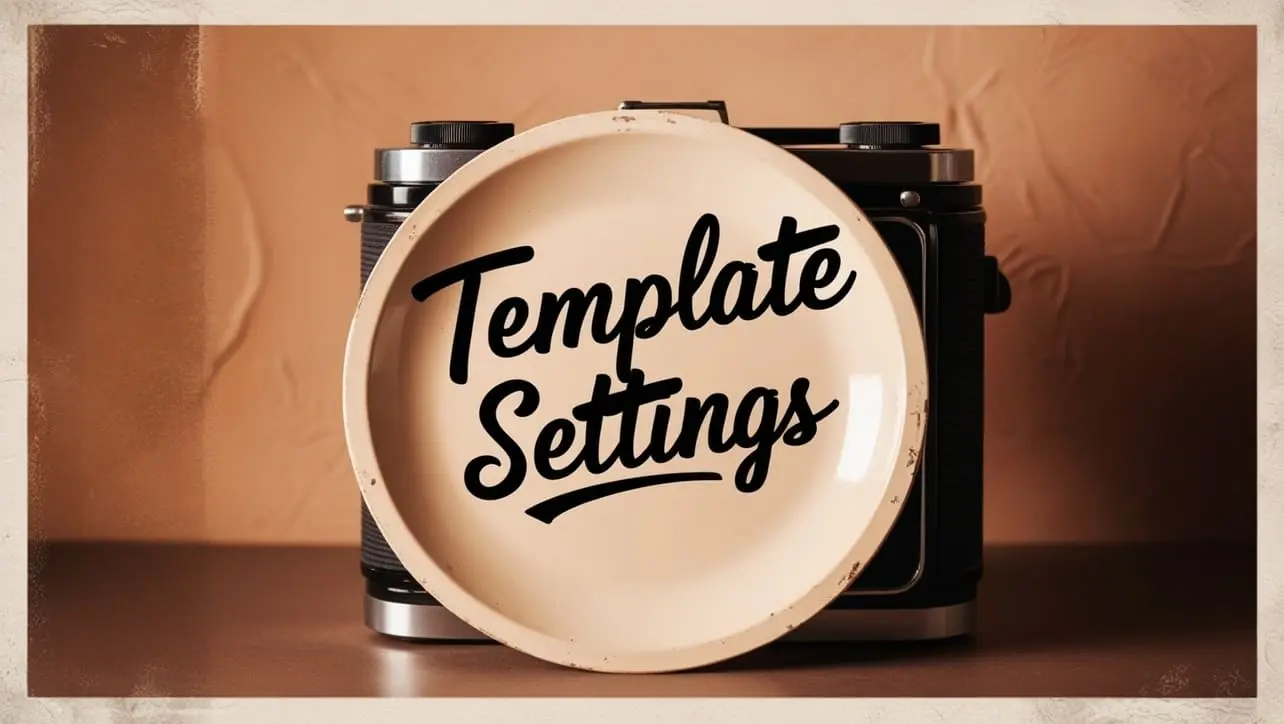
Lodash _.templateSettings Property
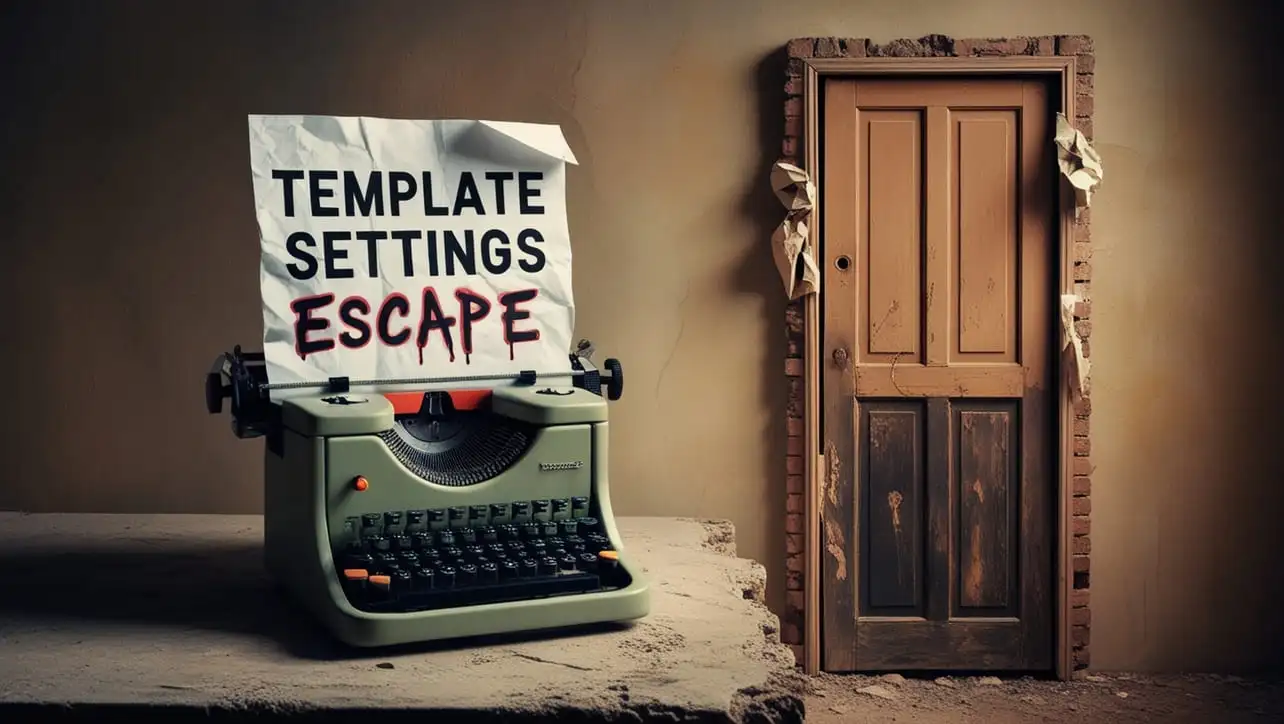
Lodash _.templateSettings.escape Property
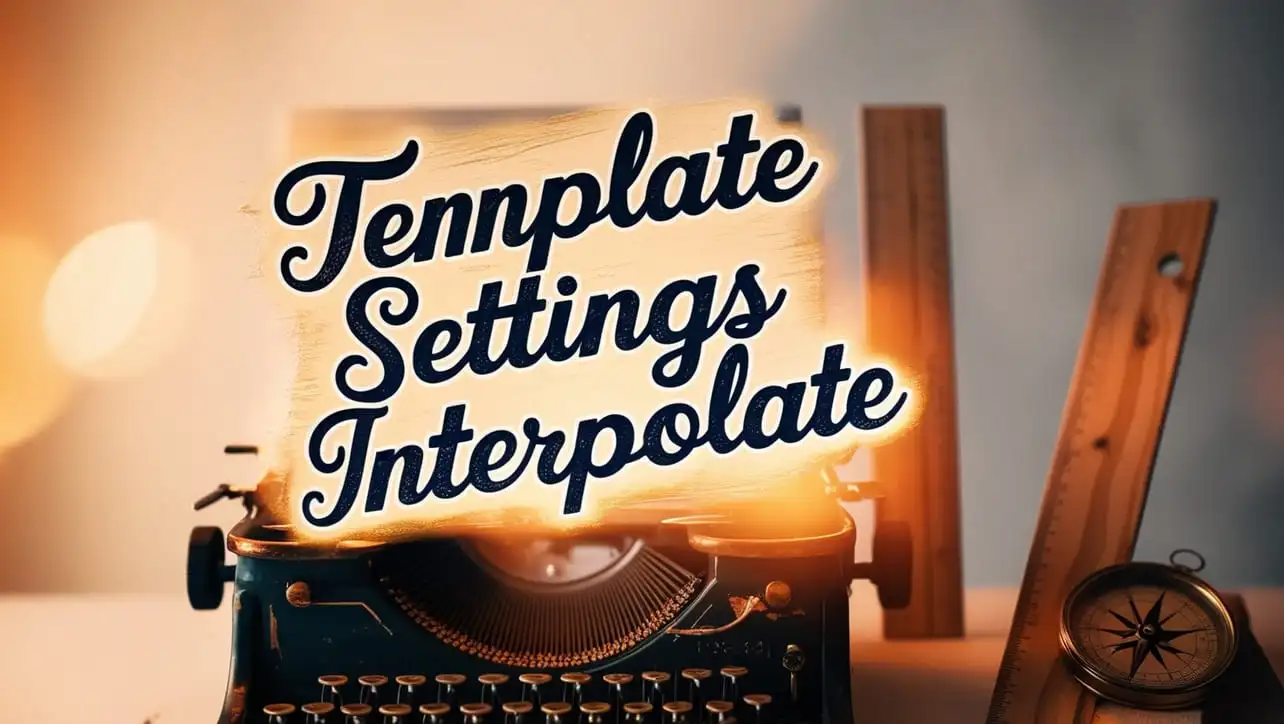
Lodash _.templateSettings.interpolate Property
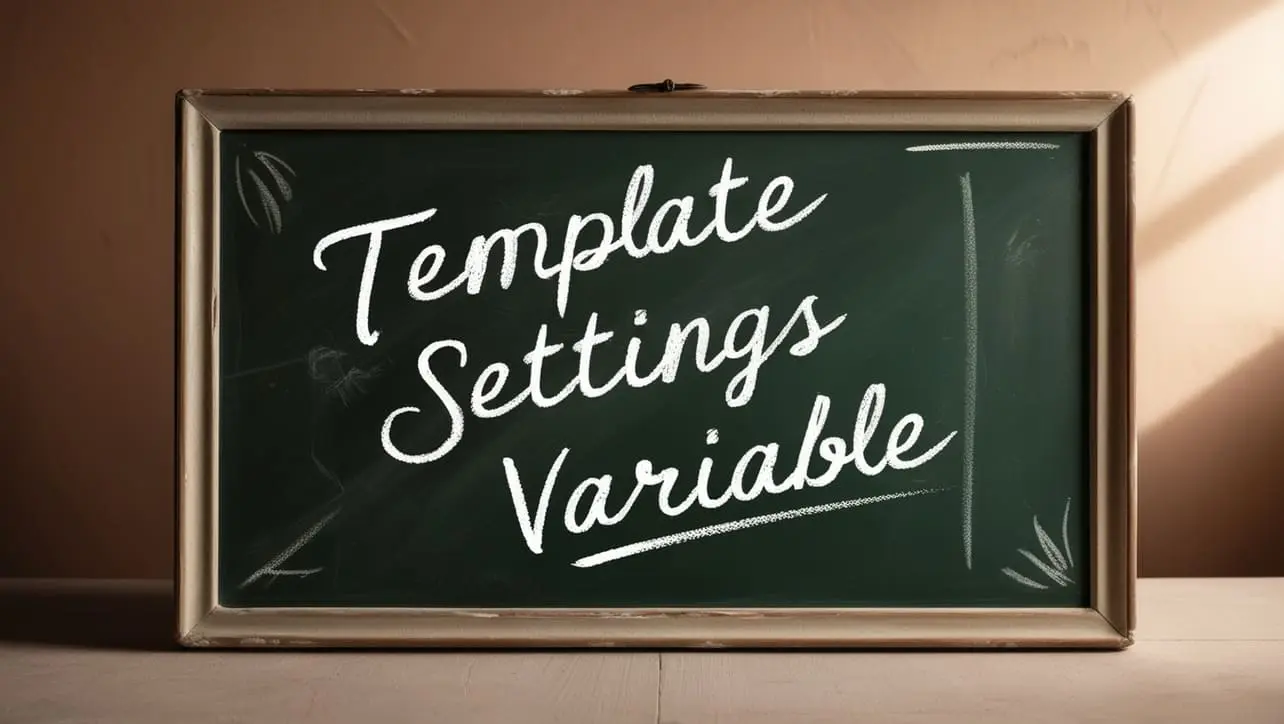
If you have any doubts regarding this article (Lodash _.snakeCase() String Method), please comment here. I will help you immediately.