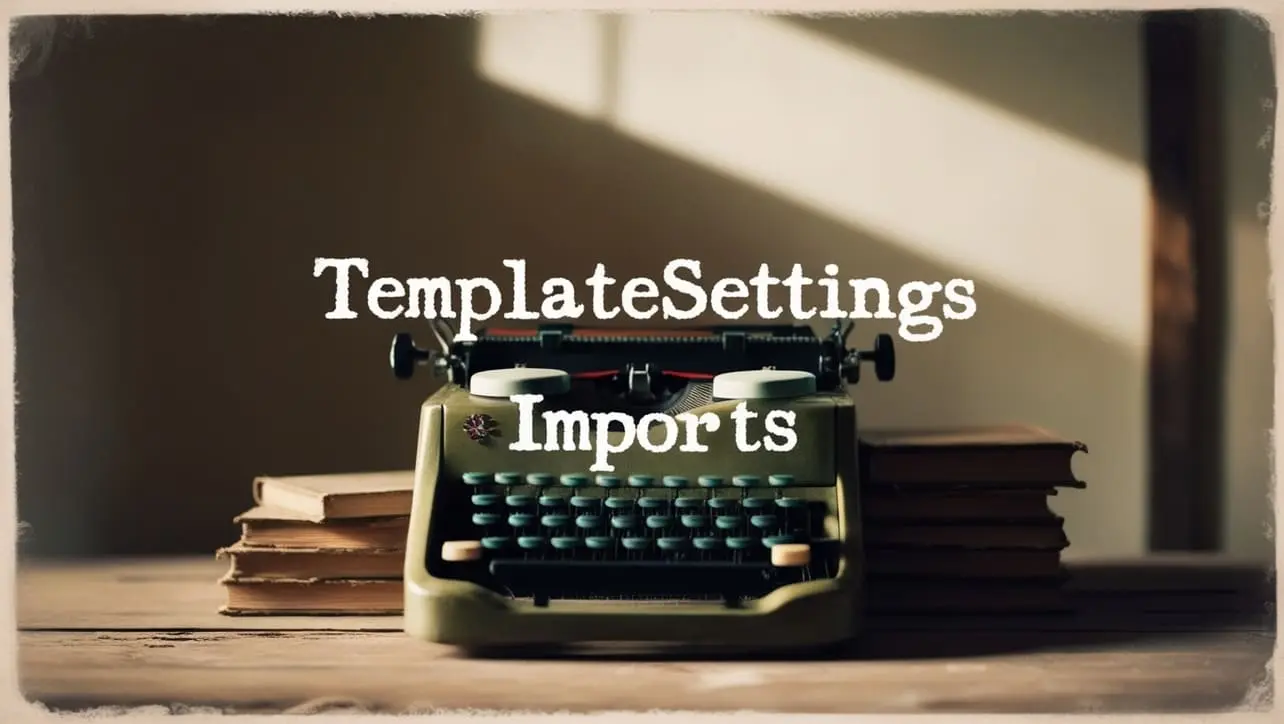
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.parseInt() String Method
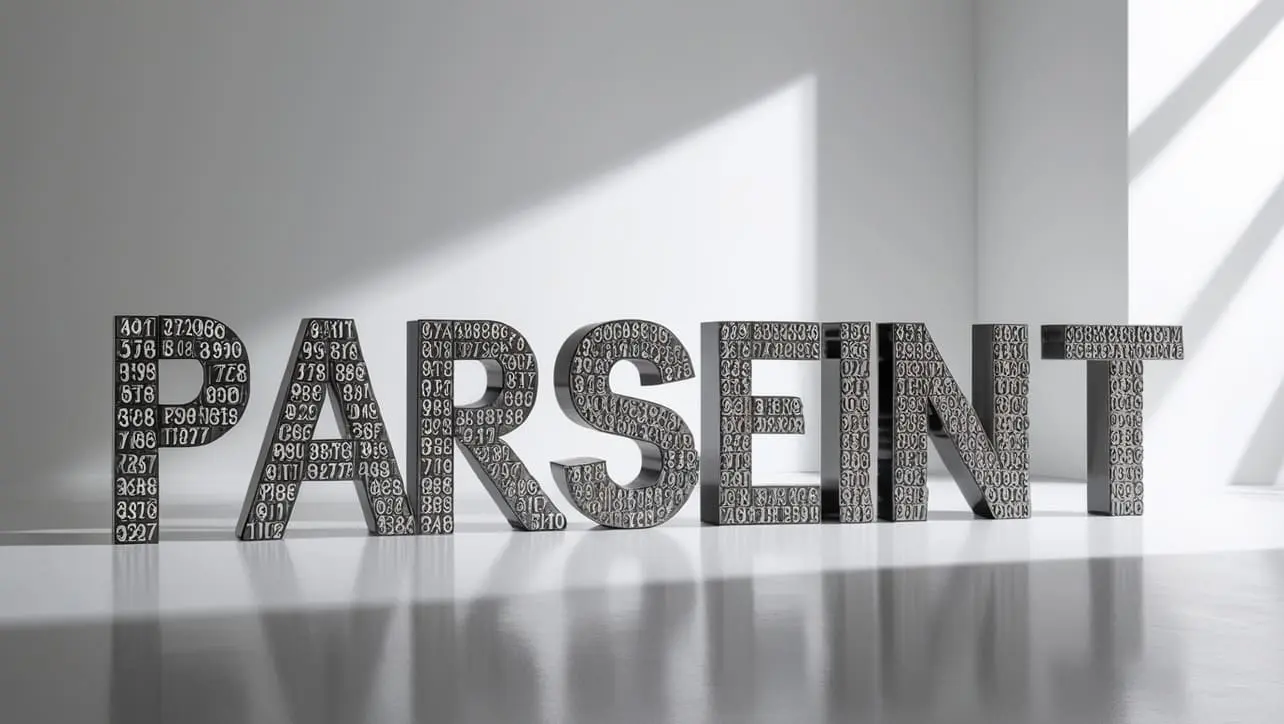
Photo Credit to CodeToFun
🙋 Introduction
Parsing strings to integers is a common task in JavaScript development. While JavaScript provides the parseInt() function, the Lodash utility library offers an enhanced version with the _.parseInt()
method.
This method provides additional features and robustness, making it a valuable tool for developers working with string manipulation.
🧠 Understanding _.parseInt() Method
The _.parseInt()
method in Lodash is designed to parse a string into an integer while providing customizable behavior and handling edge cases more gracefully than the native parseInt() function.
💡 Syntax
The syntax for the _.parseInt()
method is straightforward:
_.parseInt(string, [radix])
- string: The string to parse.
- radix (Optional): The base to use for parsing (default is 10).
📝 Example
Let's dive into a simple example to illustrate the usage of the _.parseInt()
method:
const _ = require('lodash');
const stringValue = '42';
const parsedValue = _.parseInt(stringValue);
console.log(parsedValue);
// Output: 42
In this example, the string 42 is parsed into an integer using _.parseInt()
.
🏆 Best Practices
When working with the _.parseInt()
method, consider the following best practices:
Specify Radix for Clarity:
Always specify the radix parameter to avoid confusion and ensure consistent behavior across different environments.
example.jsCopiedconst hexadecimalValue = '0xFF'; const parsedHexValue = _.parseInt(hexadecimalValue, 16); console.log(parsedHexValue); // Output: 255
Handle Invalid Inputs Gracefully:
Be prepared to handle cases where the input string cannot be parsed into a valid integer. Utilize error handling mechanisms to gracefully manage such scenarios.
example.jsCopiedconst invalidValue = 'abc'; const parsedInvalidValue = _.parseInt(invalidValue); if (isNaN(parsedInvalidValue)) { console.error('Invalid input string'); } else { console.log(parsedInvalidValue); }
Use With Functional Programming:
Leverage the functional programming capabilities of Lodash to integrate
_.parseInt()
seamlessly into your codebase, promoting code readability and maintainability.example.jsCopiedconst arrayOfStrings = ['10', '20', '30']; const parsedArray = _.map(arrayOfStrings, _.parseInt); console.log(parsedArray); // Output: [10, 20, 30]
📚 Use Cases
Data Transformation:
_.parseInt()
is ideal for transforming string data into integers, enabling seamless integration with numerical operations and calculations.example.jsCopiedconst priceString = '$50'; const price = _.parseInt(priceString.replace('$', '')); console.log(price); // Output: 50
Form Input Processing:
When processing form inputs,
_.parseInt()
can be used to extract numerical values from user-provided strings, ensuring data consistency and accuracy.example.jsCopiedconst userInput = document.getElementById('quantityInput').value; const quantity = _.parseInt(userInput); console.log(quantity);
Data Validation:
In scenarios where input validation is required,
_.parseInt()
can help validate and sanitize numerical input strings, reducing the risk of errors and vulnerabilities.example.jsCopiedconst userInput = '123'; if (_.isInteger(_.parseInt(userInput))) { console.log('Valid integer input'); } else { console.log('Invalid input'); }
🎉 Conclusion
The _.parseInt()
method in Lodash offers a robust and versatile solution for parsing strings into integers in JavaScript. Whether you're handling user input, transforming data, or validating numerical values, this method provides a reliable and flexible tool for string manipulation.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.parseInt()
method in your Lodash projects.
👨💻 Join our Community:
Author
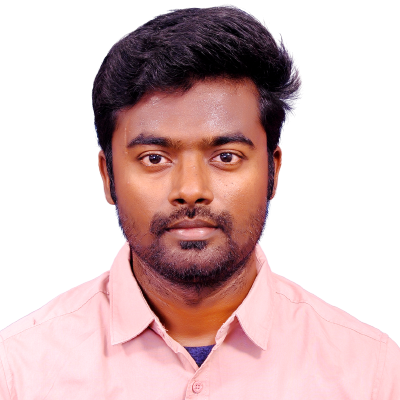
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
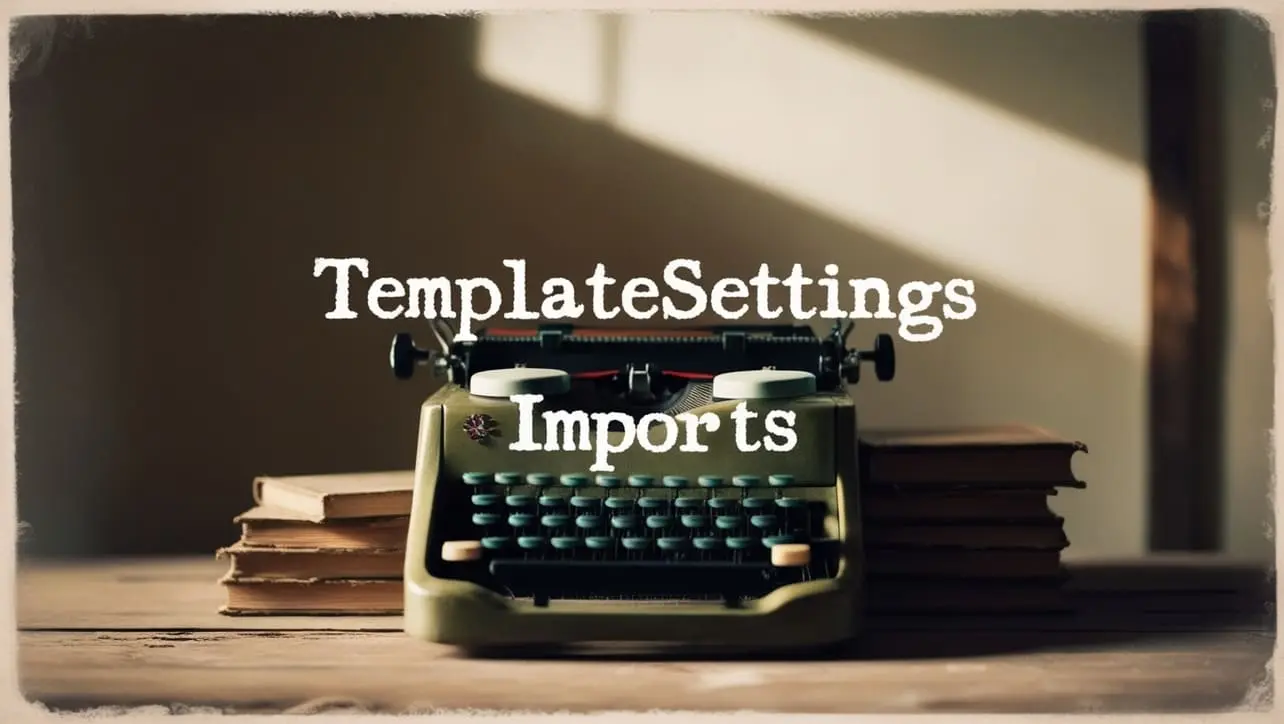
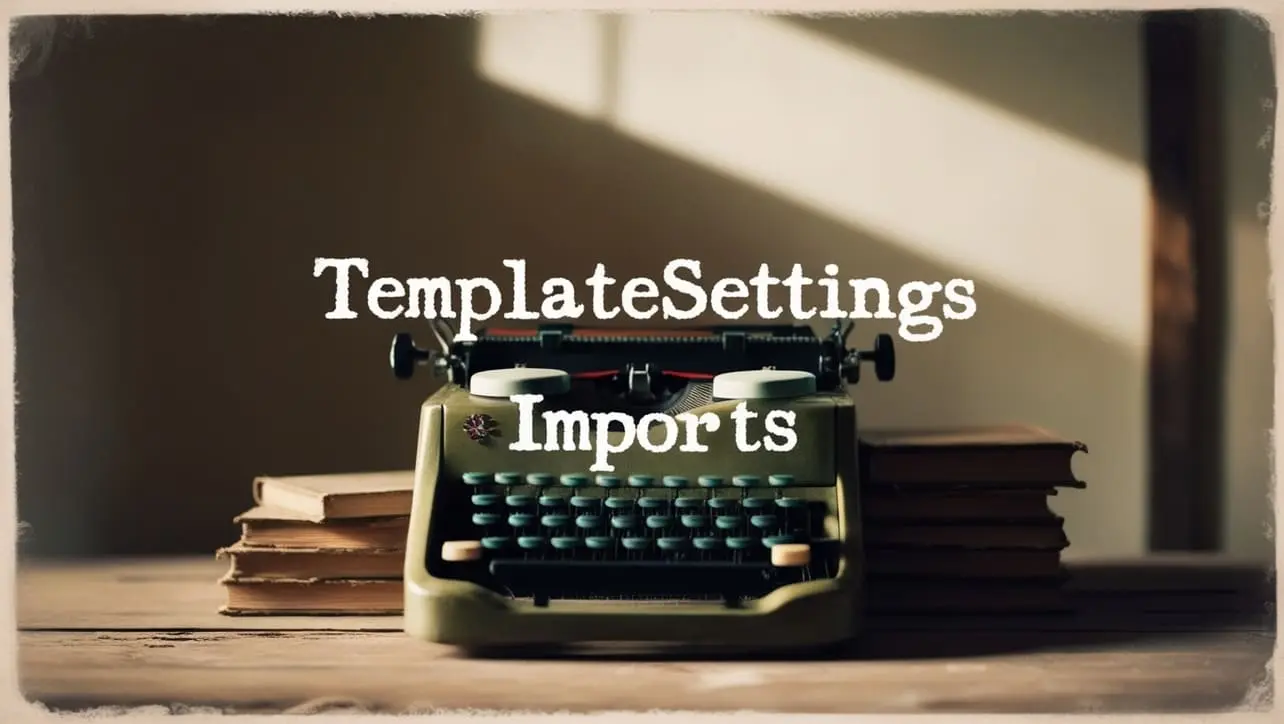
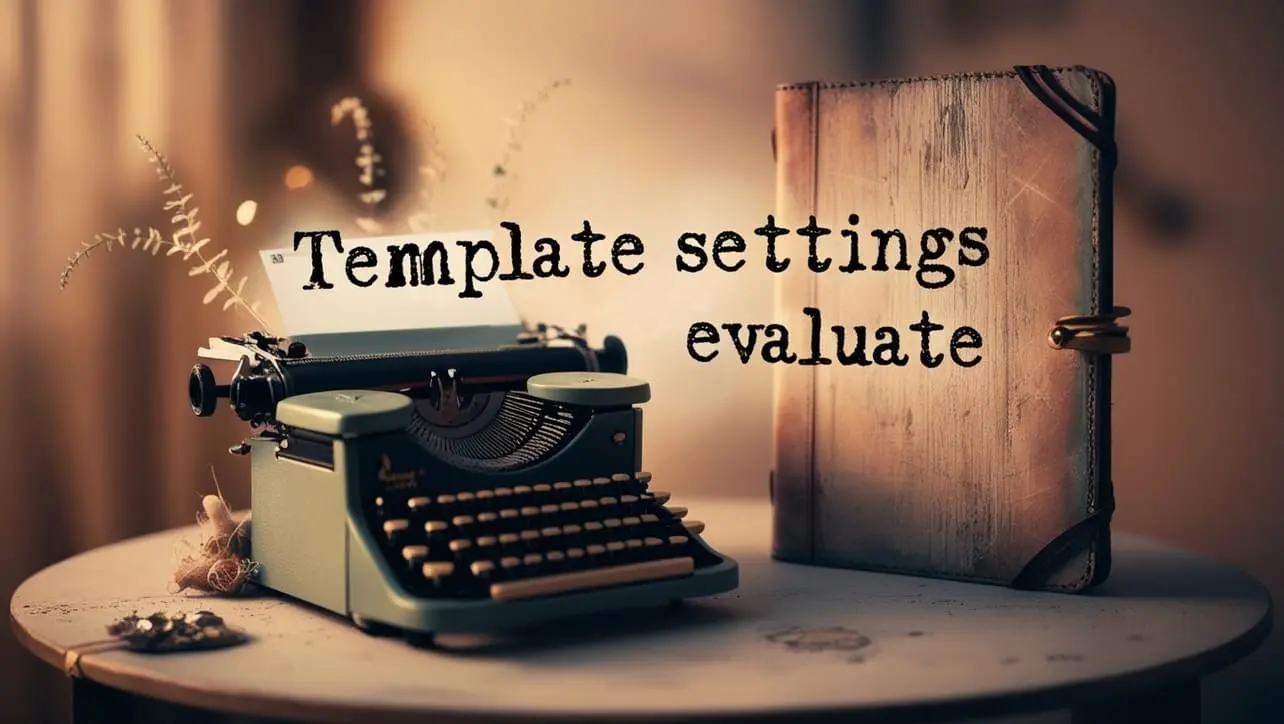
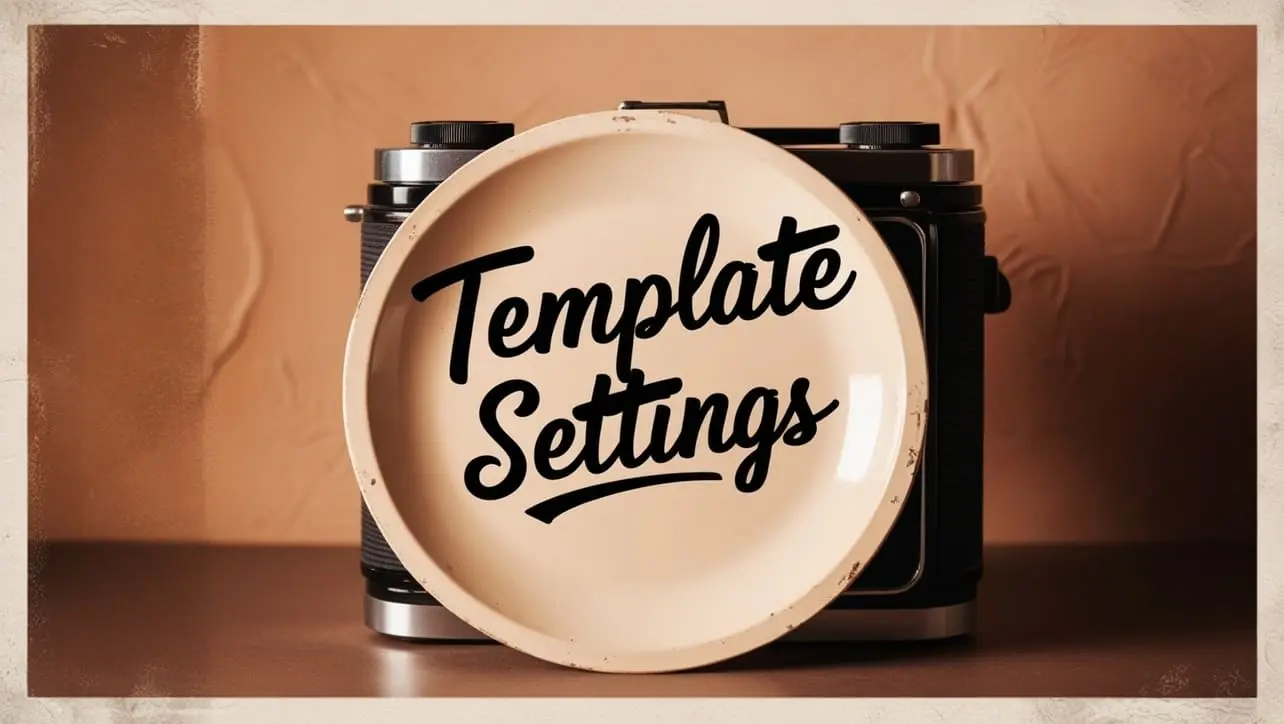
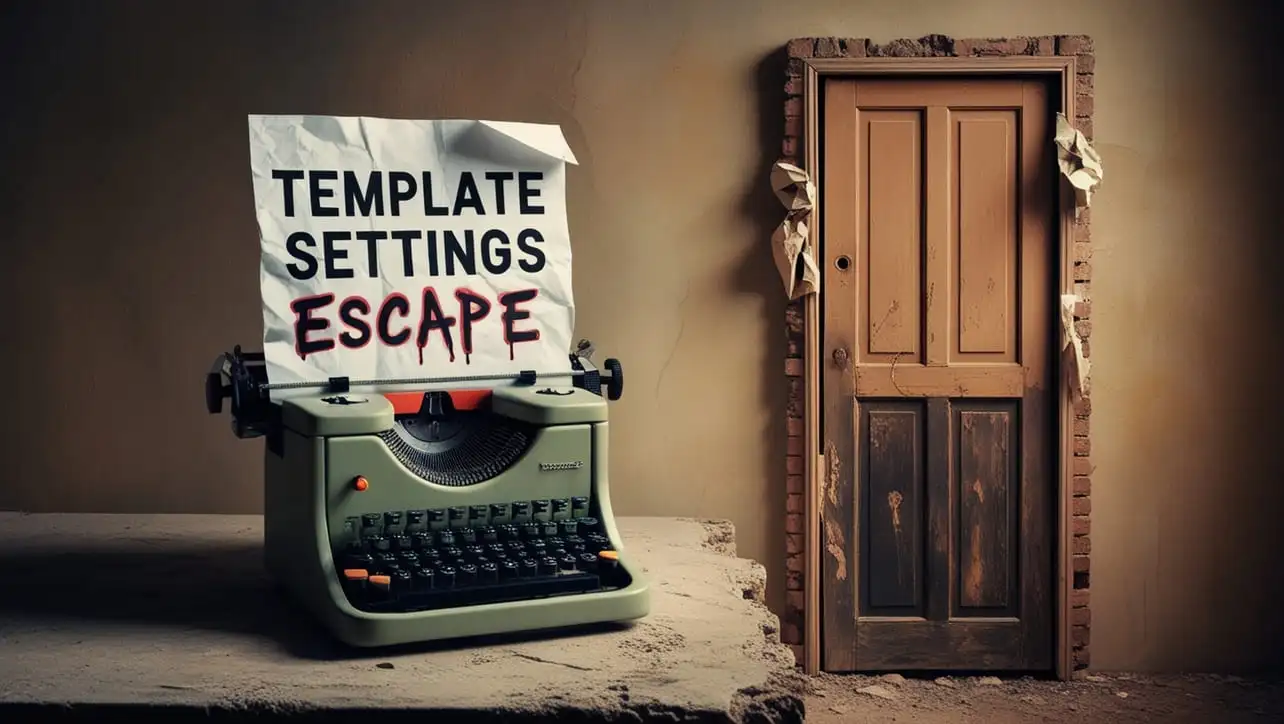
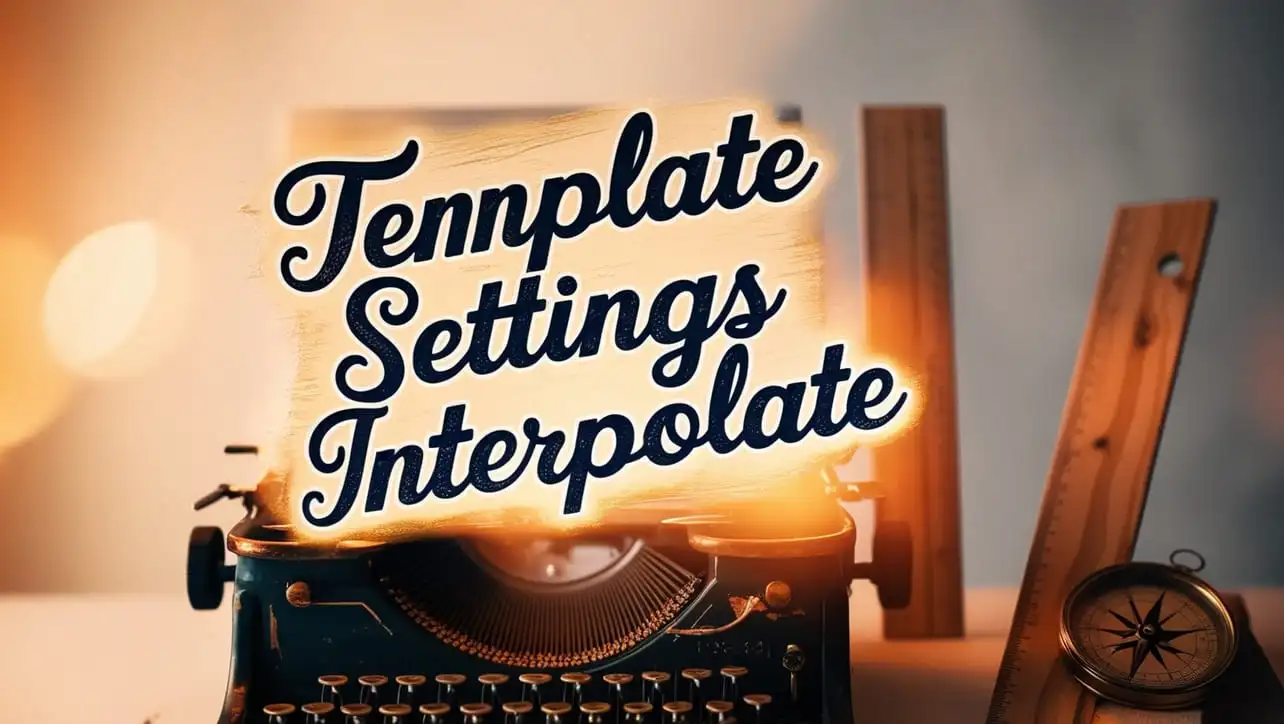
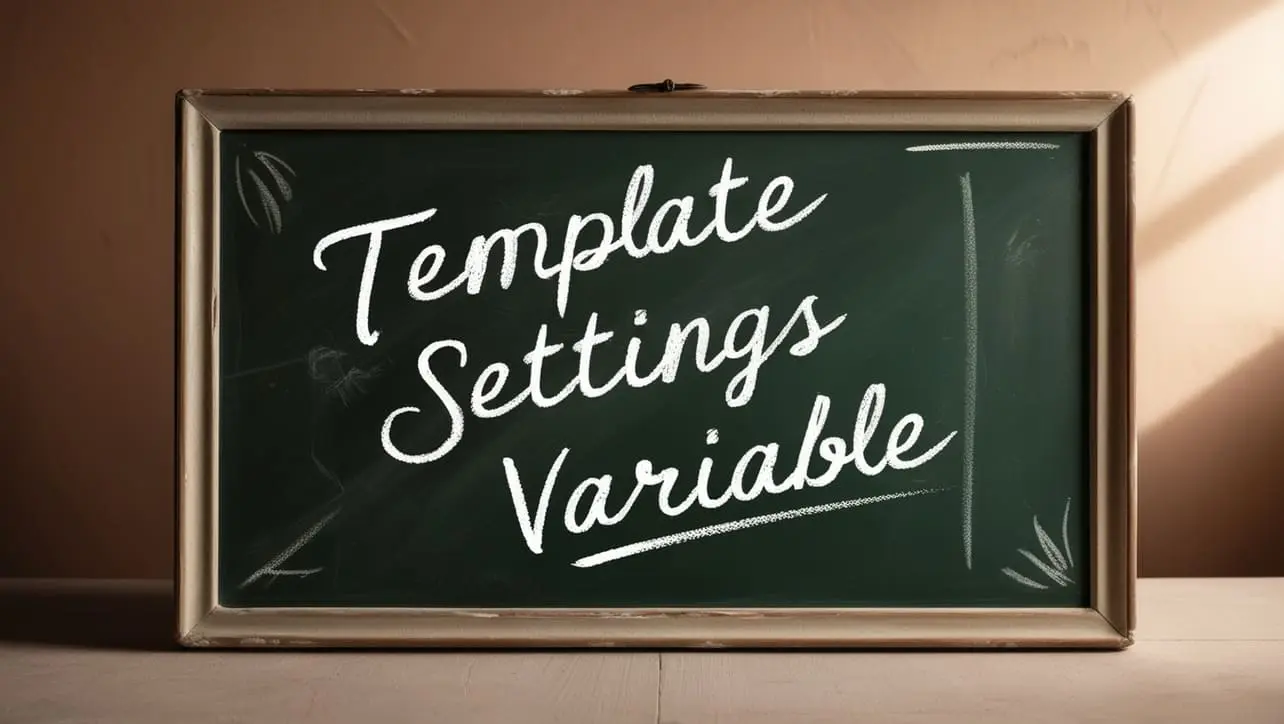
If you have any doubts regarding this article (Lodash _.parseInt() String Method), please comment here. I will help you immediately.