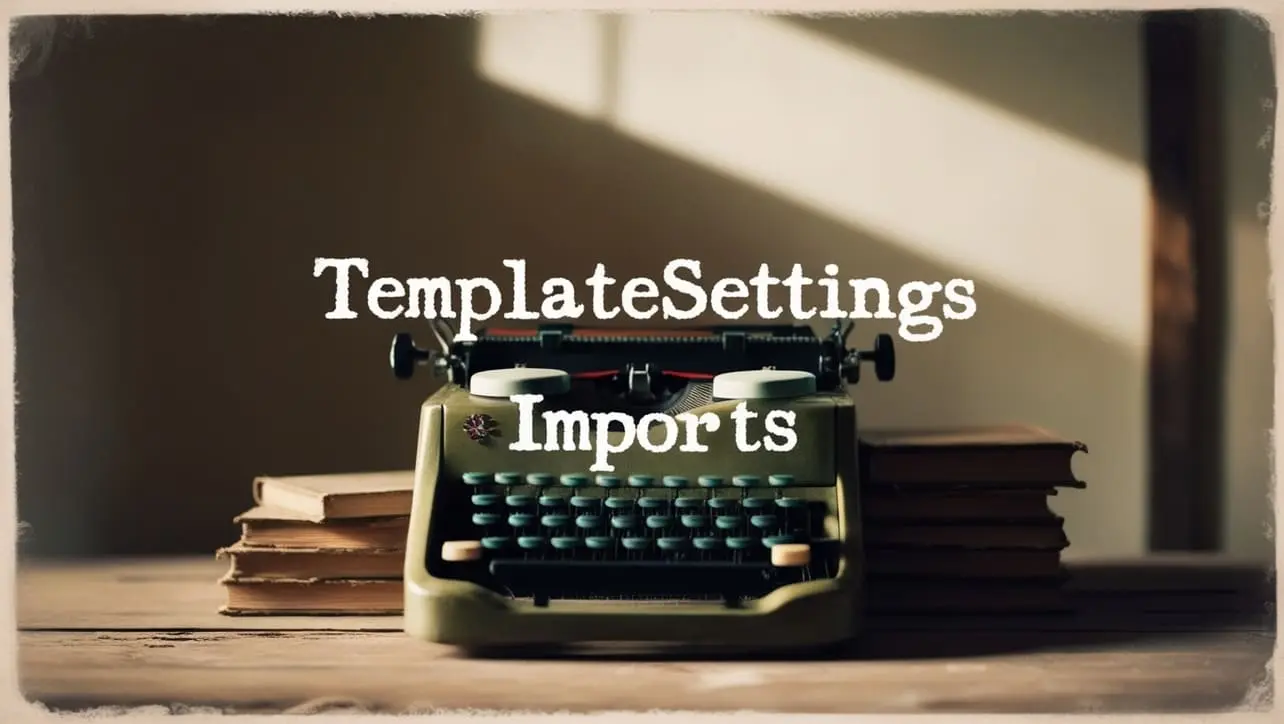
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.padStart() String Method
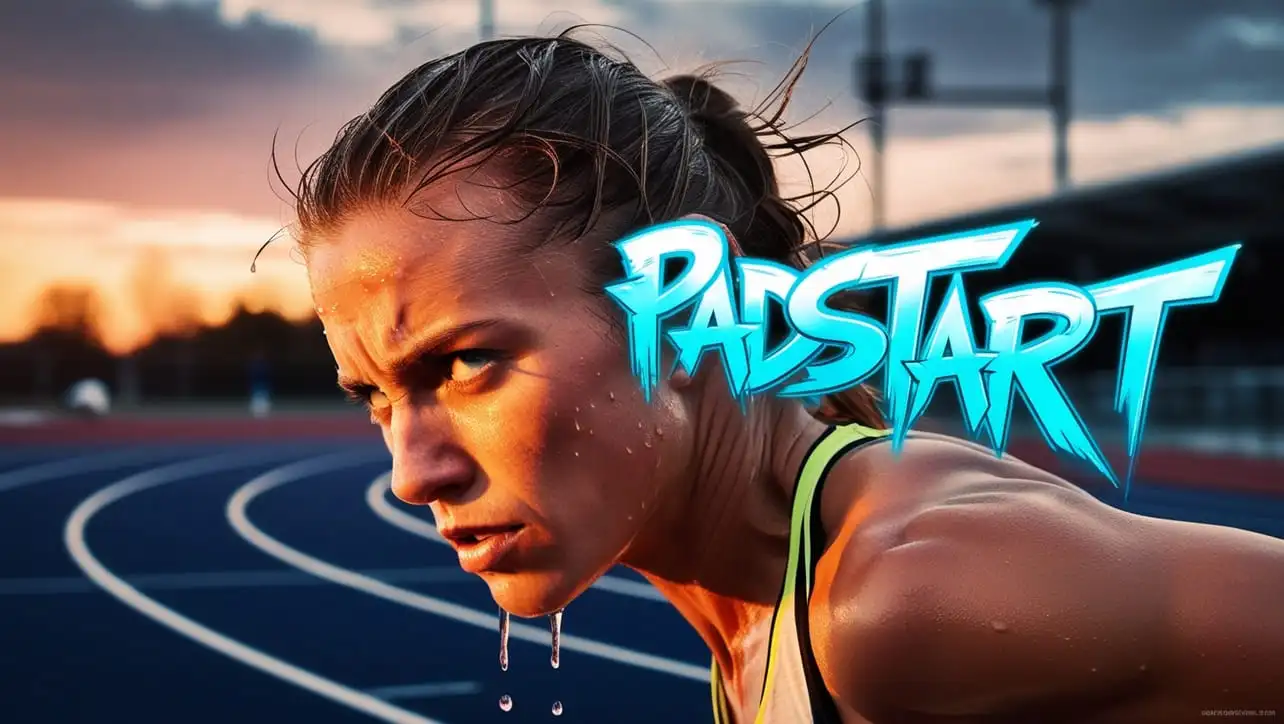
Photo Credit to CodeToFun
🙋 Introduction
String manipulation is a fundamental aspect of JavaScript programming, and Lodash offers a plethora of utility functions to streamline such tasks. Among these functions lies the _.padStart()
method, a versatile tool for padding the beginning of a string to a specified length.
Whether you're formatting text for display or aligning data in a table, _.padStart()
provides an elegant solution to ensure consistency and readability.
🧠 Understanding _.padStart() Method
The _.padStart()
method in Lodash enables you to pad the beginning of a string with characters until it reaches the desired length. This is particularly useful when aligning text in columns or ensuring uniform display formatting across your application.
💡 Syntax
The syntax for the _.padStart()
method is straightforward:
_.padStart(string, [length=0], [chars=' '])
- string: The string to pad.
- length: The target length of the padded string.
- chars (Optional) : The characters to use for padding (default is whitespace).
📝 Example
Let's dive into a simple example to illustrate the usage of the _.padStart()
method:
const _ = require('lodash');
const originalString = '123';
const paddedString = _.padStart(originalString, 5, '0');
console.log(paddedString);
// Output: "00123"
In this example, the originalString is padded with leading zeros until it reaches a length of 5 characters.
🏆 Best Practices
When working with the _.padStart()
method, consider the following best practices:
Specify Padding Length:
Always specify the desired length when using
_.padStart()
. This ensures consistency in formatting and prevents unexpected behavior.example.jsCopiedconst originalString = 'hello'; const paddedString = _.padStart(originalString, 10); console.log(paddedString); // Output: " hello"
Choose Appropriate Padding Characters:
Select padding characters that are suitable for your use case. Whether it's whitespace, zeros, or any other character, ensure that the padding aligns with your desired formatting.
example.jsCopiedconst originalString = 'world'; const paddedString = _.padStart(originalString, 10, '-'); console.log(paddedString); // Output: "-----world"
Consider Use Cases:
Evaluate your specific use cases to determine the most effective way to utilize
_.padStart()
. Whether it's formatting dates, aligning text in tables, or padding numbers, tailor your approach accordingly.example.jsCopiedconst phoneNumber = '1234567890'; const formattedPhoneNumber = `(${_.padStart(phoneNumber.slice(0, 3), 3, '0')}) ${_.padStart(phoneNumber.slice(3, 6), 3, '0')}-${phoneNumber.slice(6)}`; console.log(formattedPhoneNumber); // Output: "(123) 456-7890"
📚 Use Cases
Formatting Text:
_.padStart()
is invaluable for formatting text to achieve consistent alignment and presentation, particularly in scenarios such as generating reports or displaying tabular data.example.jsCopiedconst itemNames = ['Apple', 'Banana', 'Cherry']; const maxLength = Math.max(...itemNames.map(name => name.length)); const formattedItems = itemNames.map(name => _.padStart(name, maxLength)); console.log(formattedItems); // Output: [" Apple", "Banana", "Cherry"]
Data Alignment:
When working with structured data,
_.padStart()
can ensure uniform alignment of values, improving readability and aesthetics.example.jsCopiedconst data = [ { name: 'John', age: 25, city: 'New York' }, { name: 'Alice', age: 30, city: 'Los Angeles' }, ]; const alignedData = data.map(entry => ({ name: _.padStart(entry.name, 10), age: _.padStart(entry.age.toString(), 5), city: _.padStart(entry.city, 15), })); console.table(alignedData);
Numeric Padding:
In cases where numeric values need padding,
_.padStart()
can be used to ensure consistent length, facilitating comparison and readability.example.jsCopiedconst numbers = [10, 100, 1000, 10000]; const paddedNumbers = numbers.map(num => _.padStart(num.toString(), 6, '0')); console.log(paddedNumbers); // Output: ["000010", "000100", "01000", "10000"]
🎉 Conclusion
The _.padStart()
method in Lodash provides a convenient and efficient solution for padding the beginning of strings to achieve desired lengths. Whether you're formatting text for display, aligning data in tables, or padding numeric values, _.padStart()
offers versatility and ease of use in string manipulation tasks.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.padStart()
method in your Lodash projects.
👨💻 Join our Community:
Author
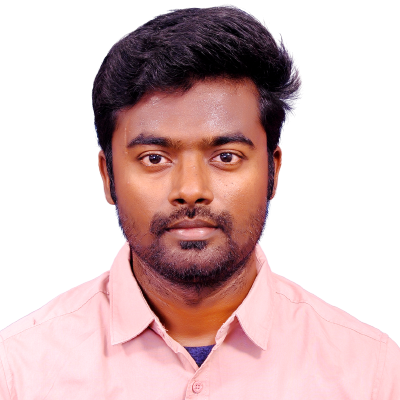
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
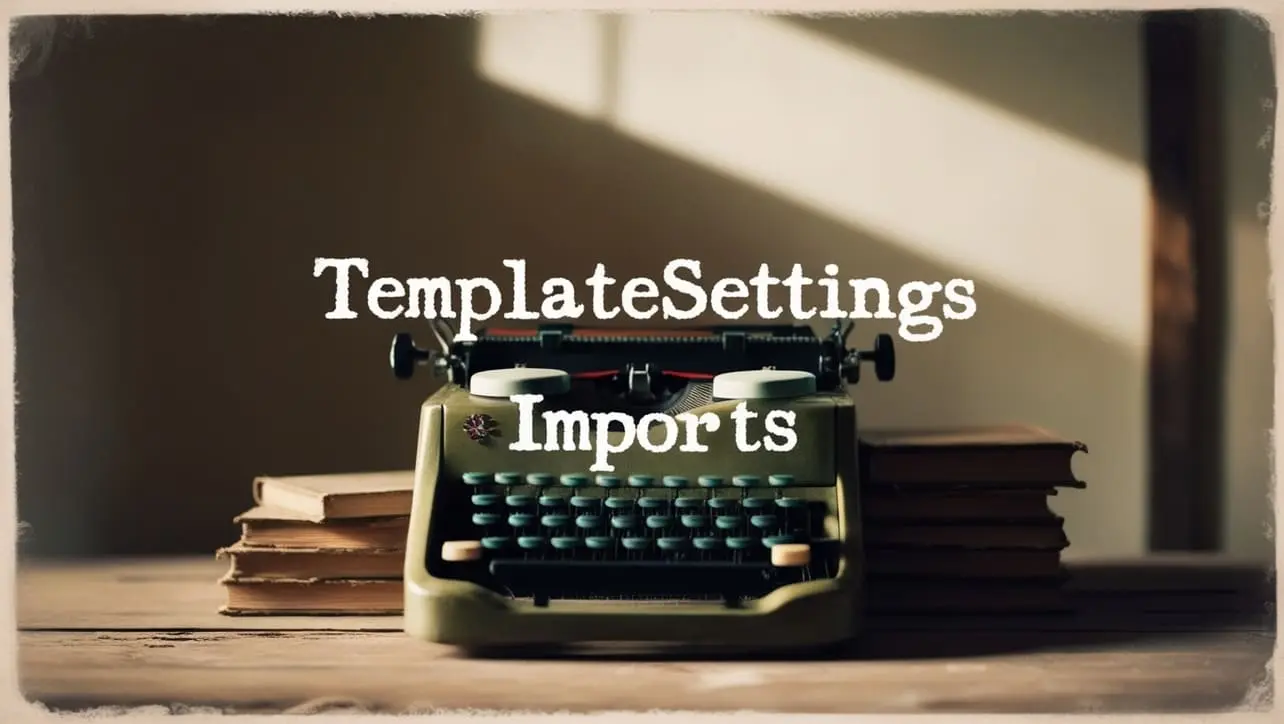
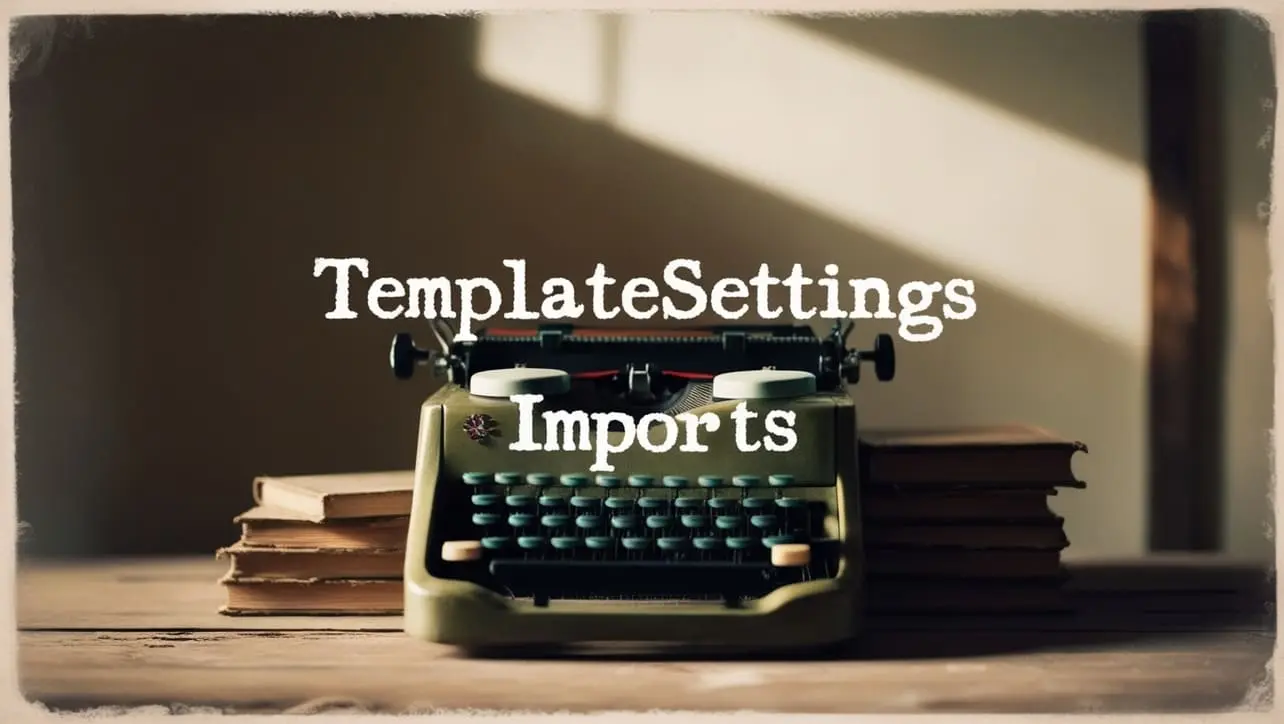
Lodash _.templateSettings.imports Property
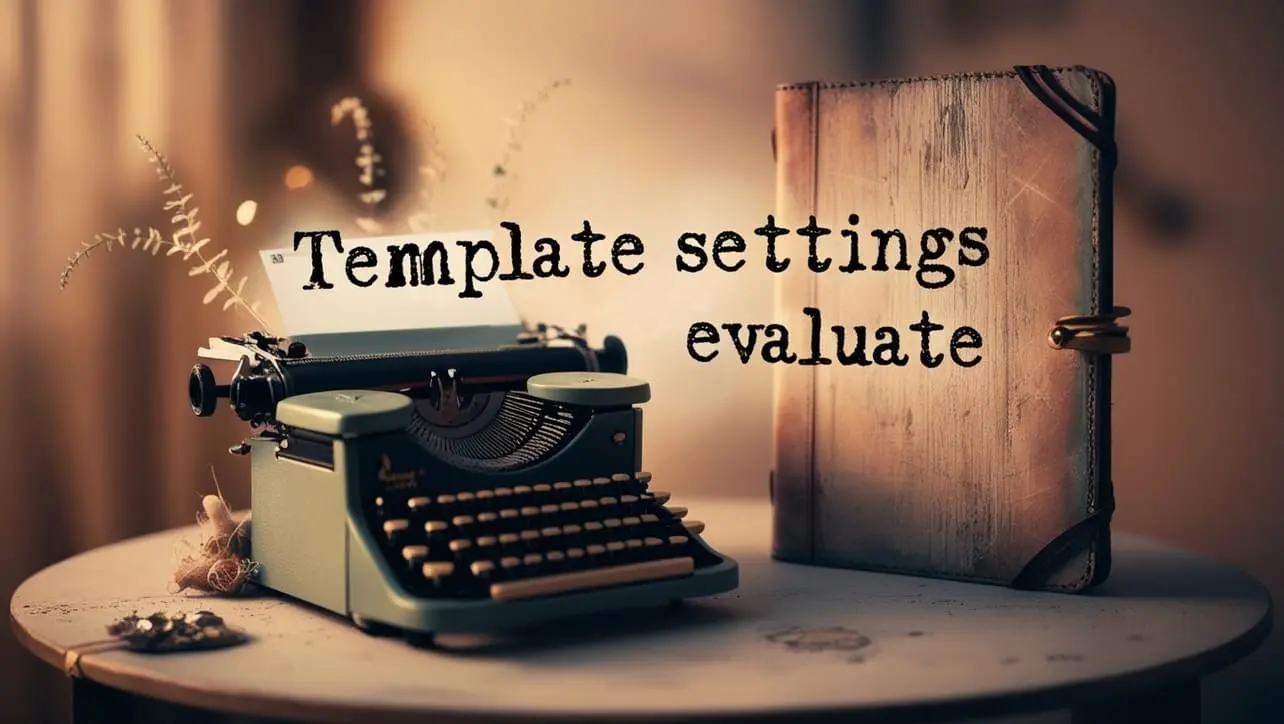
Lodash _.templateSettings.evaluate Property
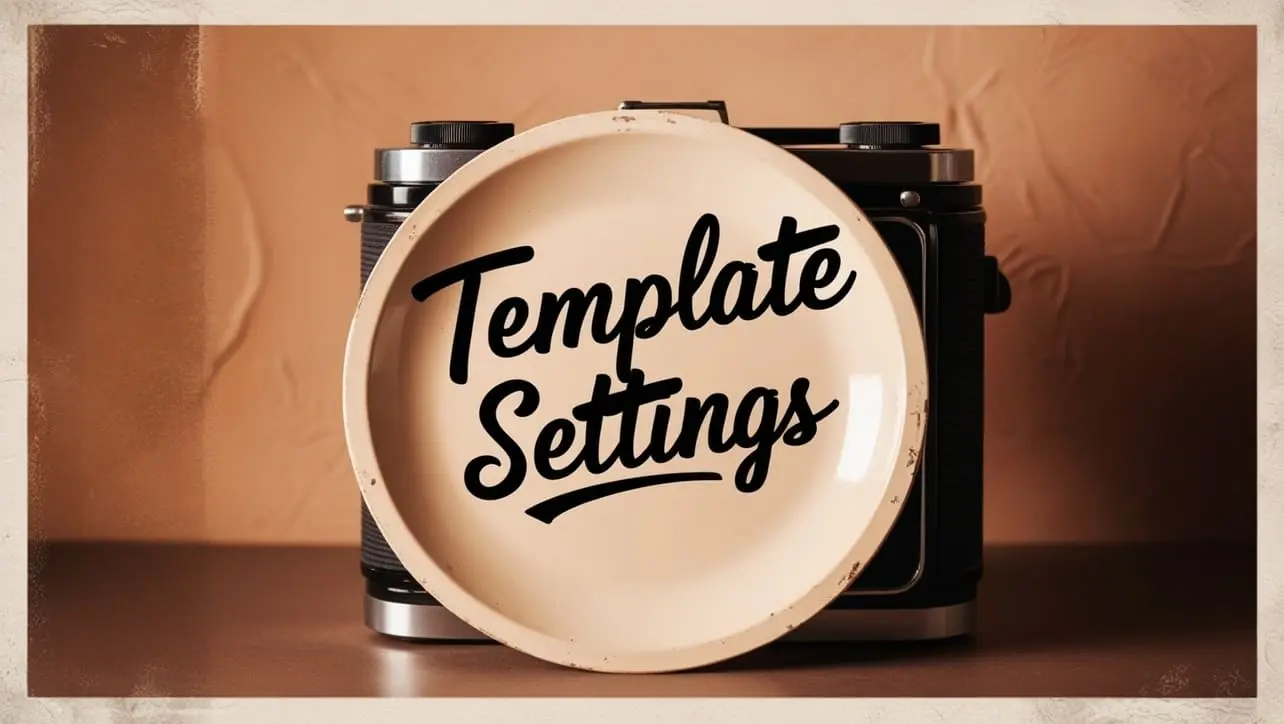
Lodash _.templateSettings Property
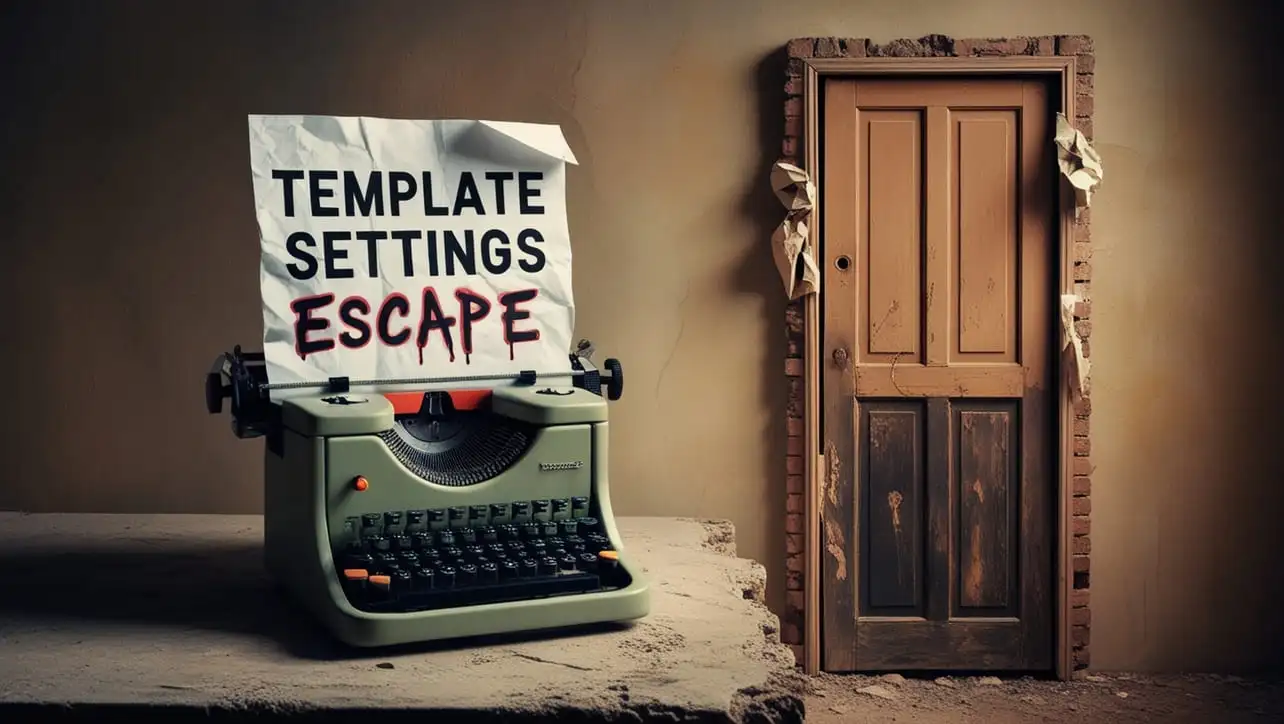
Lodash _.templateSettings.escape Property
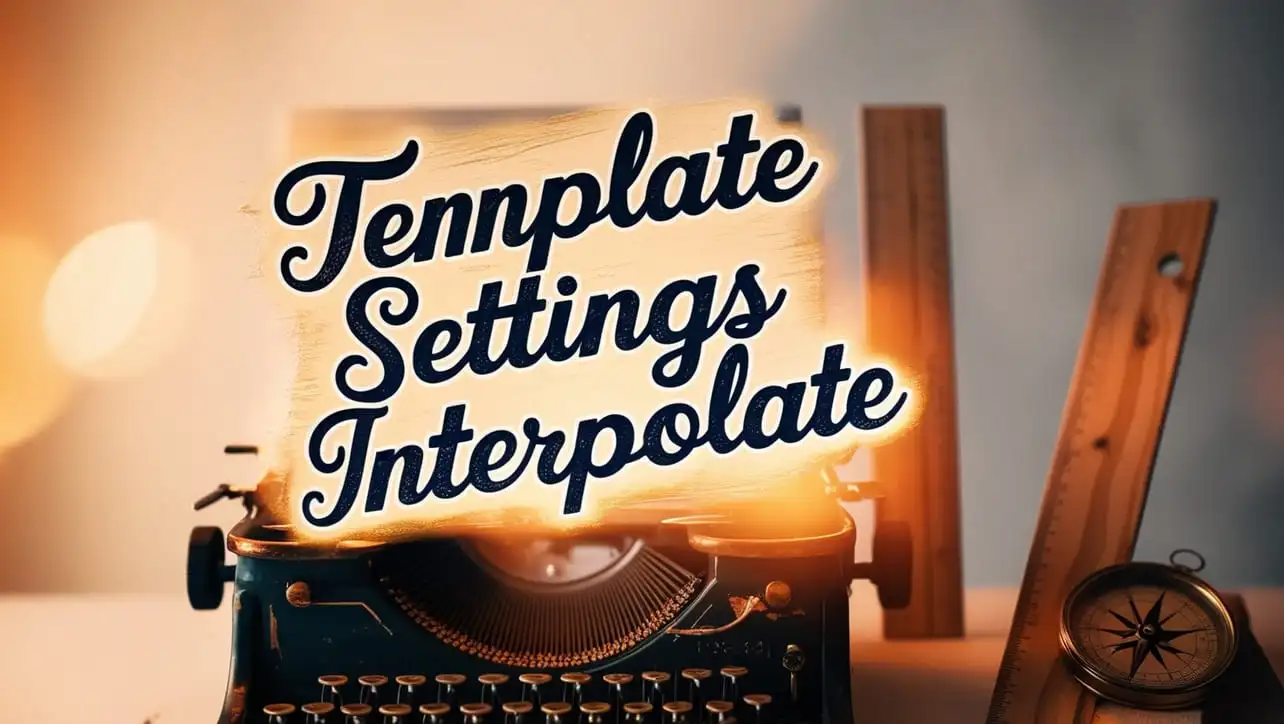
Lodash _.templateSettings.interpolate Property
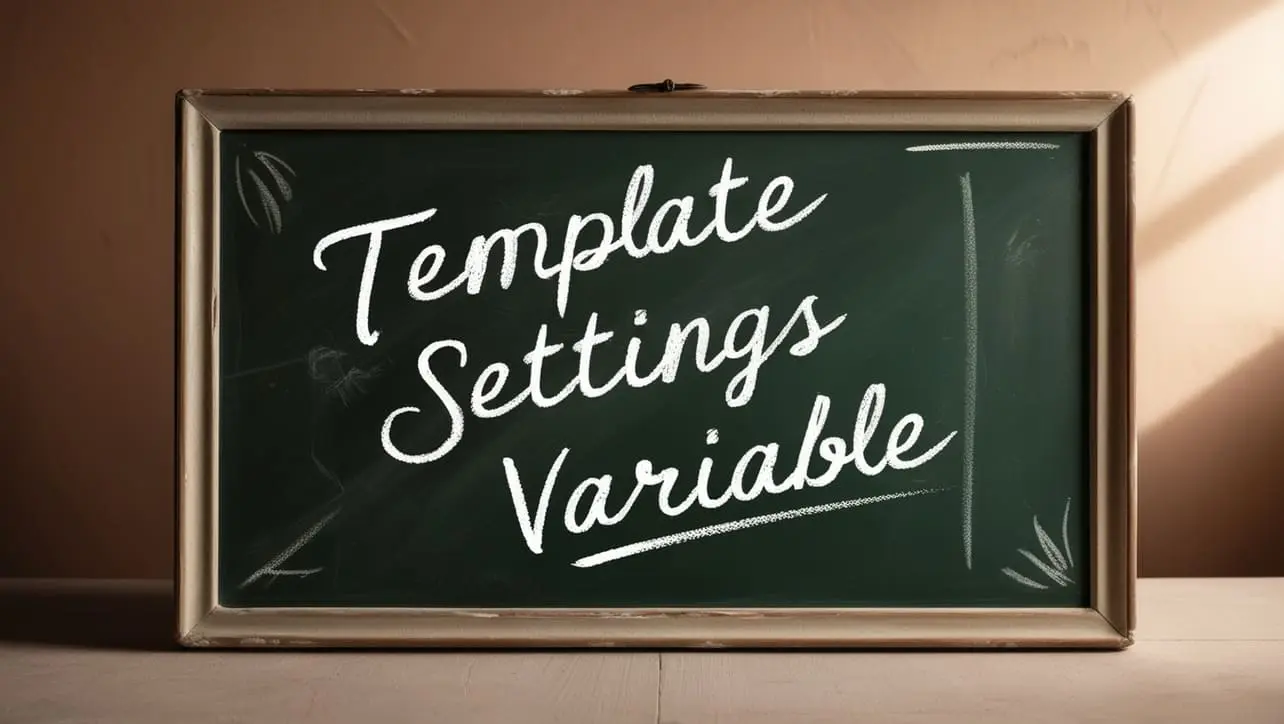
If you have any doubts regarding this article (Lodash _.padStart() String Method), please comment here. I will help you immediately.