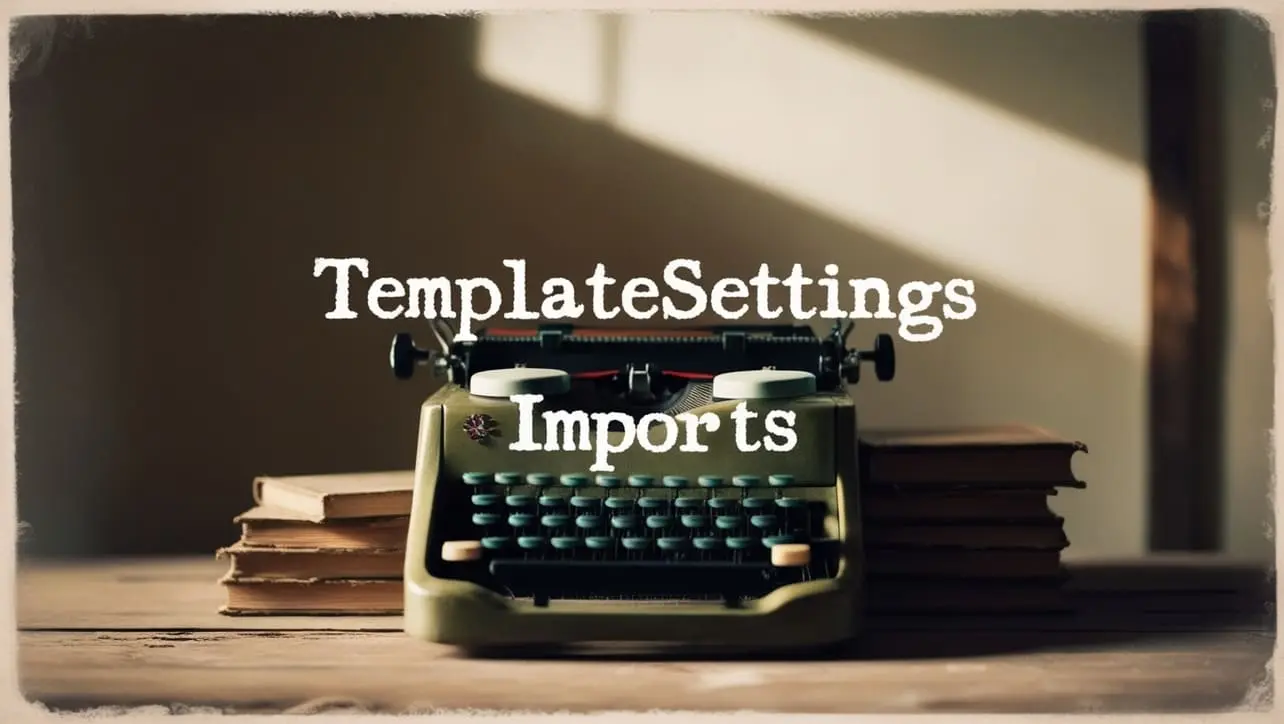
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.padEnd() String Method
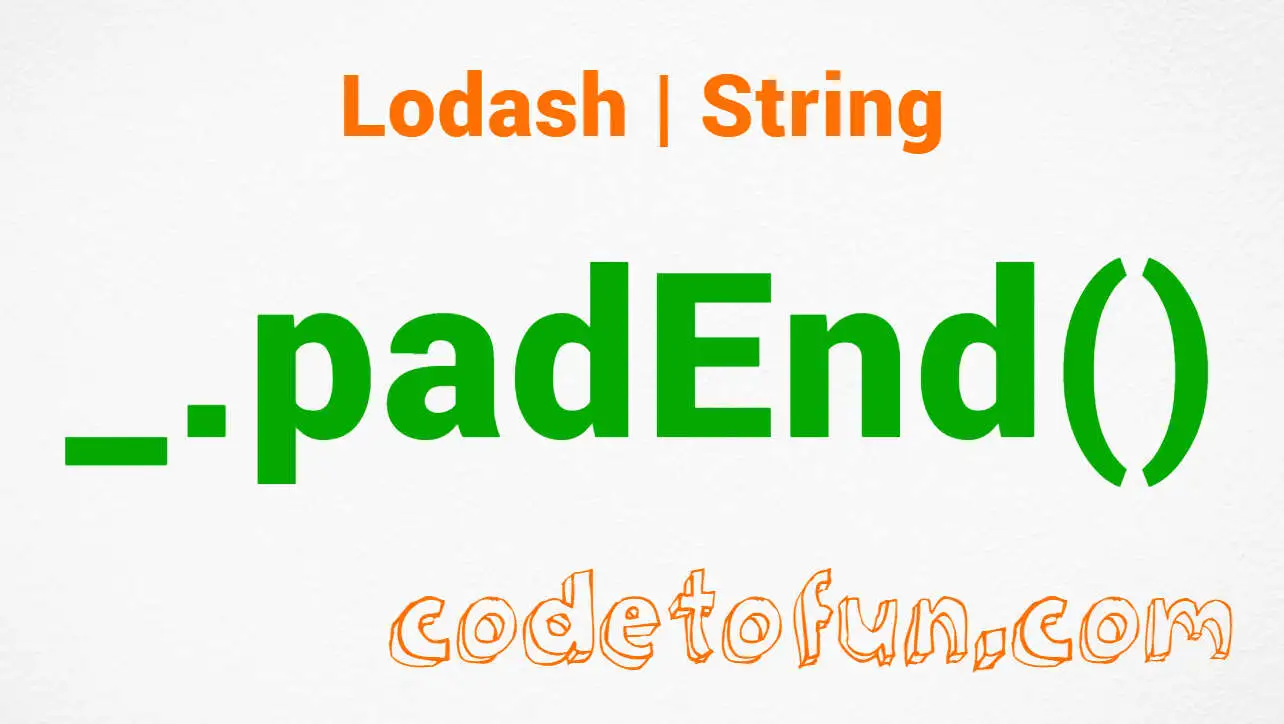
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript, formatting strings to achieve consistent spacing or alignment is a common task, especially when dealing with tabular data or textual output. Lodash, a popular utility library, provides the _.padEnd()
method to simplify this process.
This method enables developers to add padding characters to the end of a string, ensuring a specified length and enhancing the visual presentation of text-based content.
🧠 Understanding _.padEnd() Method
The _.padEnd()
method in Lodash extends the length of a string by appending padding characters to its end until it reaches the desired length. This functionality is particularly useful for aligning text within fixed-width columns or ensuring uniformity in textual output.
💡 Syntax
The syntax for the _.padEnd()
method is straightforward:
_.padEnd(string, length, [chars=' '])
- string: The string to pad.
- length: The target length of the padded string.
- chars (Optional): The padding characters to append (default is a space).
📝 Example
Let's dive into a simple example to illustrate the usage of the _.padEnd()
method:
const _ = require('lodash');
const originalString = 'Hello';
const paddedString = _.padEnd(originalString, 10);
console.log(paddedString);
// Output: 'Hello '
In this example, the originalString is padded with spaces to achieve a total length of 10 characters.
🏆 Best Practices
When working with the _.padEnd()
method, consider the following best practices:
Understanding Padding Characters:
Be mindful of the padding characters used with
_.padEnd()
. By default, spaces are appended to the end of the string, but you can customize this behavior by specifying alternative padding characters.example.jsCopiedconst paddedString = _.padEnd('Hello', 10, '-'); console.log(paddedString); // Output: 'Hello-----'
Target Length Considerations:
Ensure that the target length specified for padding meets your requirements. Padding beyond the desired length may introduce unnecessary whitespace, while insufficient padding could compromise alignment.
example.jsCopiedconst originalString = 'Hello'; const paddedString = _.padEnd(originalString, 3); console.log(paddedString); // Output: 'Hello'
Dynamic Padding:
Utilize variables or dynamic expressions to determine the padding length or characters dynamically based on runtime conditions.
example.jsCopiedconst dynamicPaddingLength = 10; const dynamicPaddingCharacter = '*'; const paddedString = _.padEnd('Hello', dynamicPaddingLength, dynamicPaddingCharacter); console.log(paddedString); // Output: 'Hello*****'
📚 Use Cases
Text Alignment:
_.padEnd()
is commonly used for aligning text within fixed-width columns, ensuring consistent spacing and visual alignment in tabular data or textual output.example.jsCopiedconst data = [ { name: 'John', age: 30 }, { name: 'Alice', age: 25 }, { name: 'Bob', age: 35 } ]; data.forEach(({ name, age }) => { const paddedName = _.padEnd(name, 10); const paddedAge = _.padEnd(age.toString(), 5); console.log(`${paddedName} | ${paddedAge}`); });
String Formatting:
When generating formatted strings for display or logging purposes,
_.padEnd()
can ensure consistent spacing and enhance readability.example.jsCopiedconst formattedString = [ _.padEnd('Name:', 10), _.padEnd('Age:', 5) ].join(' '); console.log(formattedString); // Output: 'Name: Age: '
🎉 Conclusion
The _.padEnd()
method in Lodash simplifies string formatting by enabling developers to add padding characters to the end of a string, ensuring a specified length and enhancing visual presentation. Whether aligning text within columns or formatting textual output, _.padEnd()
offers a versatile solution for string manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.padEnd()
method in your Lodash projects.
👨💻 Join our Community:
Author
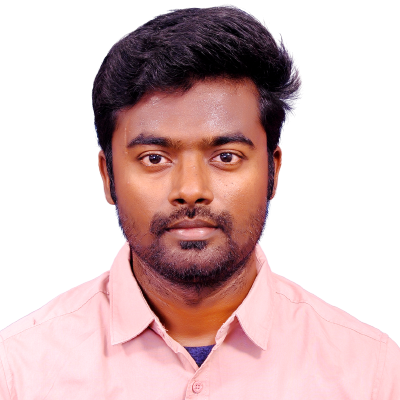
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
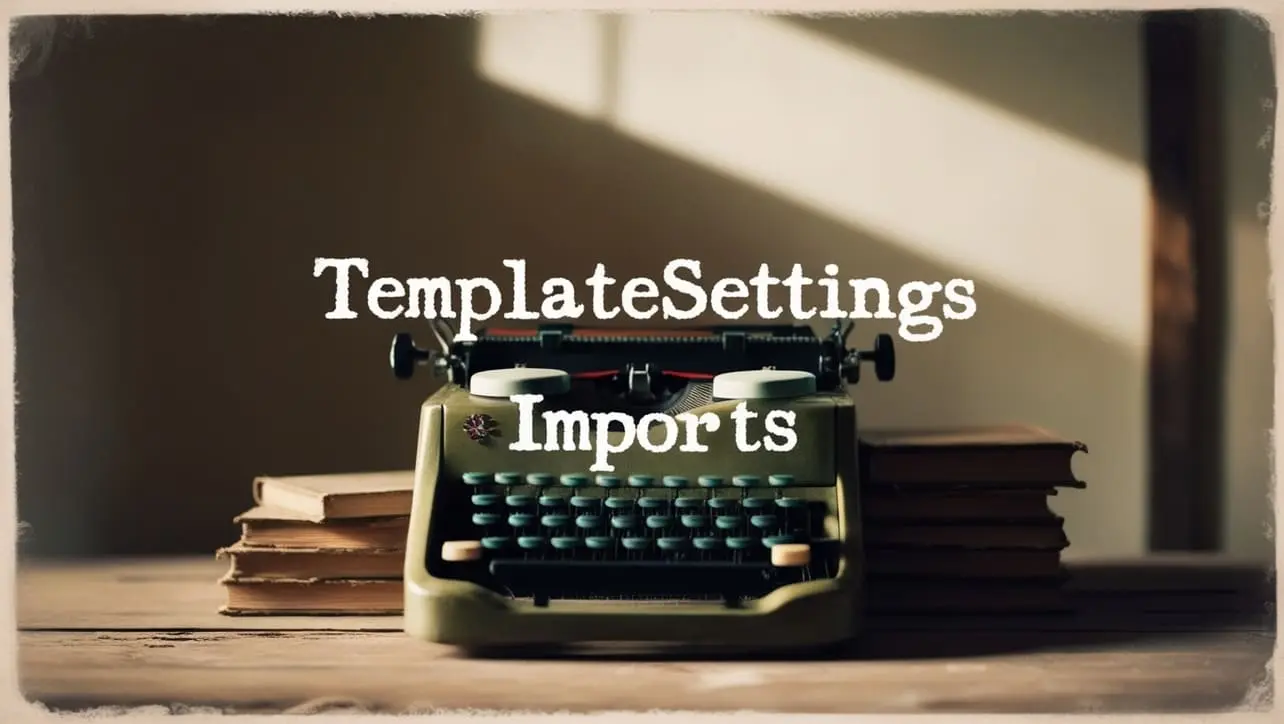
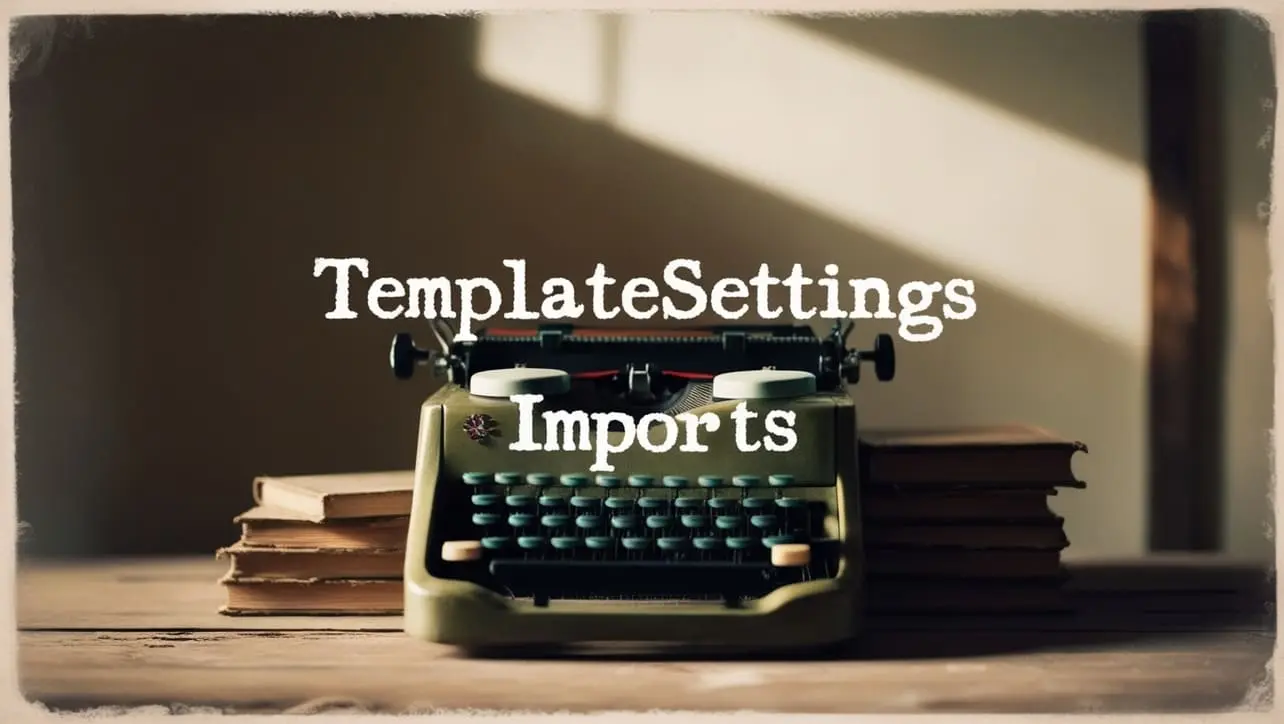
Lodash _.templateSettings.imports Property
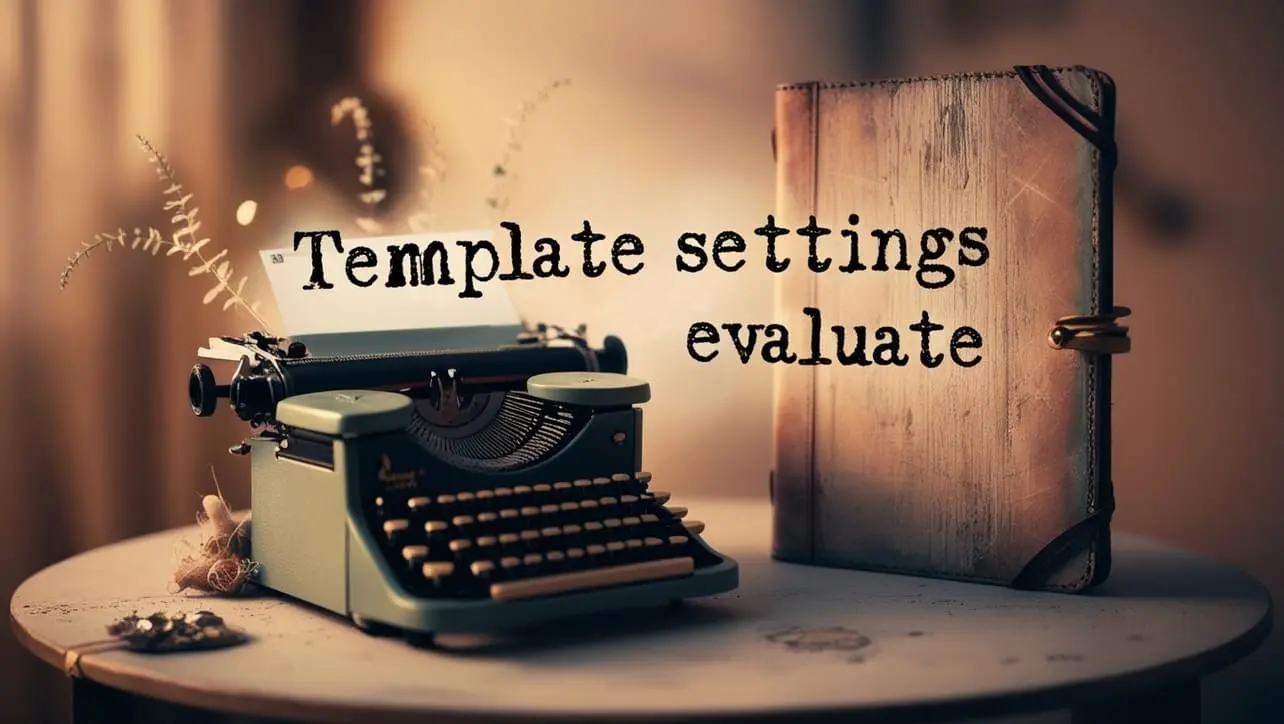
Lodash _.templateSettings.evaluate Property
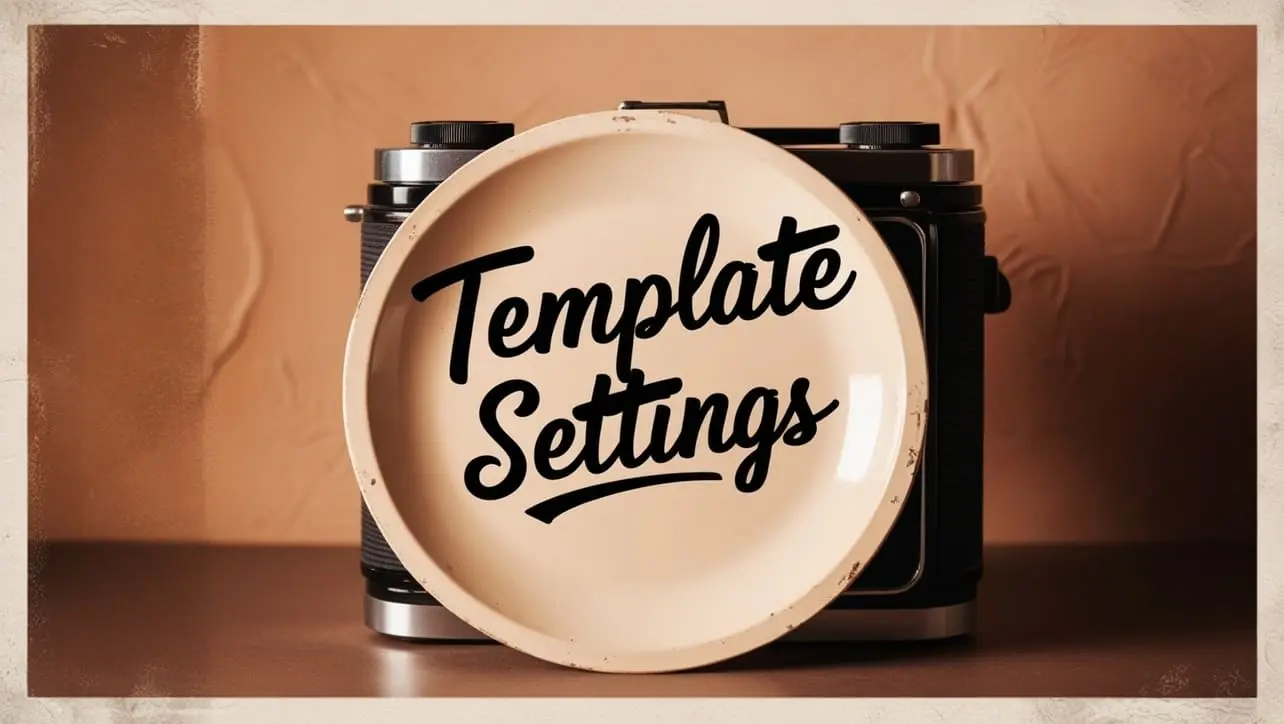
Lodash _.templateSettings Property
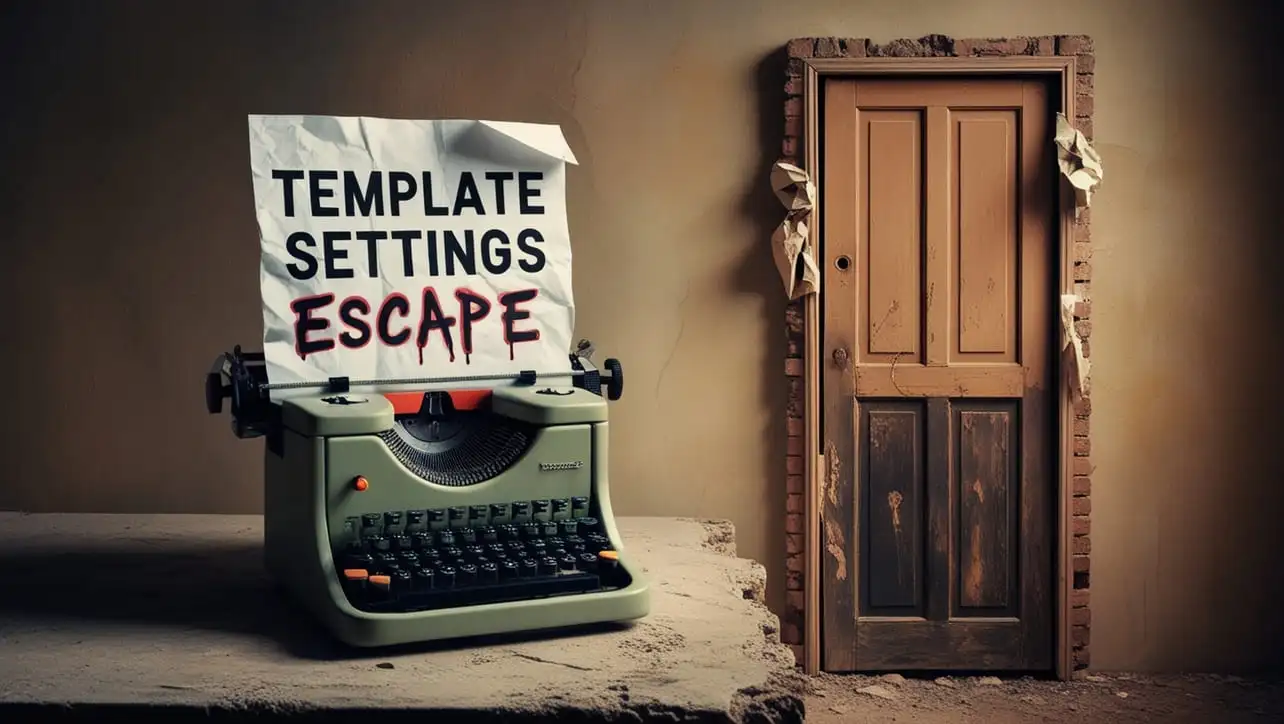
Lodash _.templateSettings.escape Property
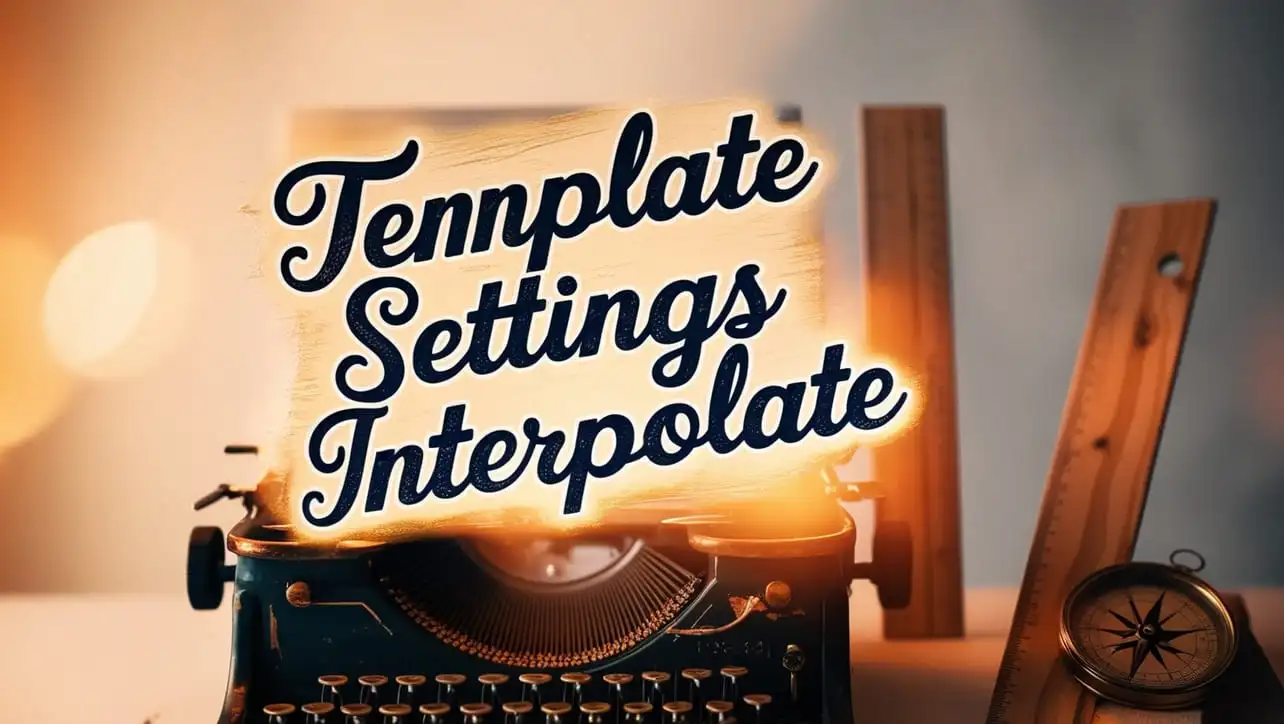
Lodash _.templateSettings.interpolate Property
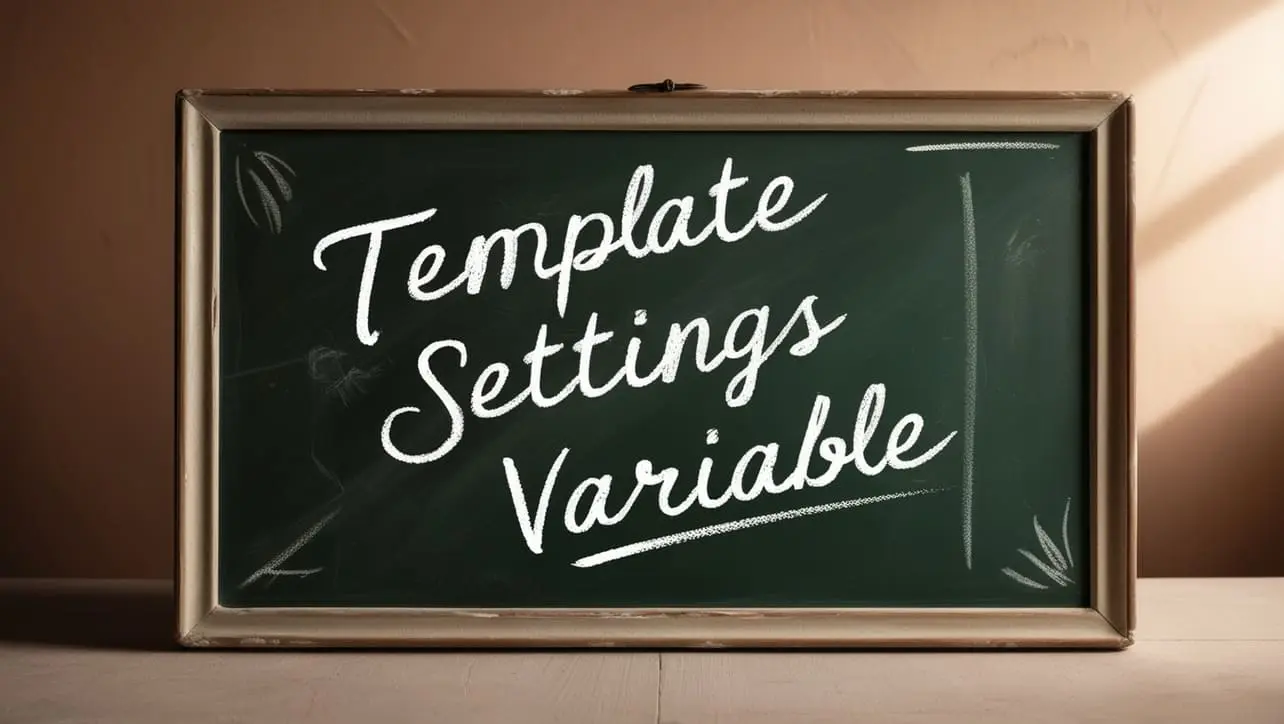
If you have any doubts regarding this article (Lodash _.padEnd() String Method), please comment here. I will help you immediately.