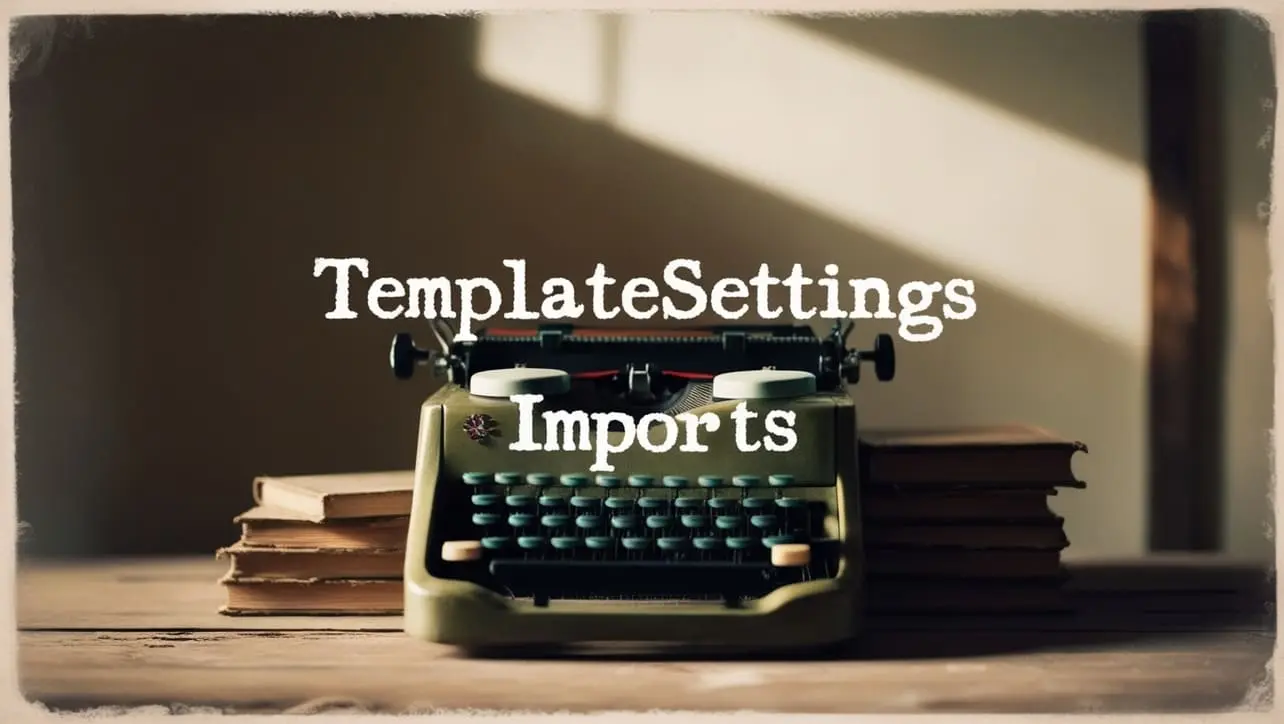
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.kebabCase() String Method
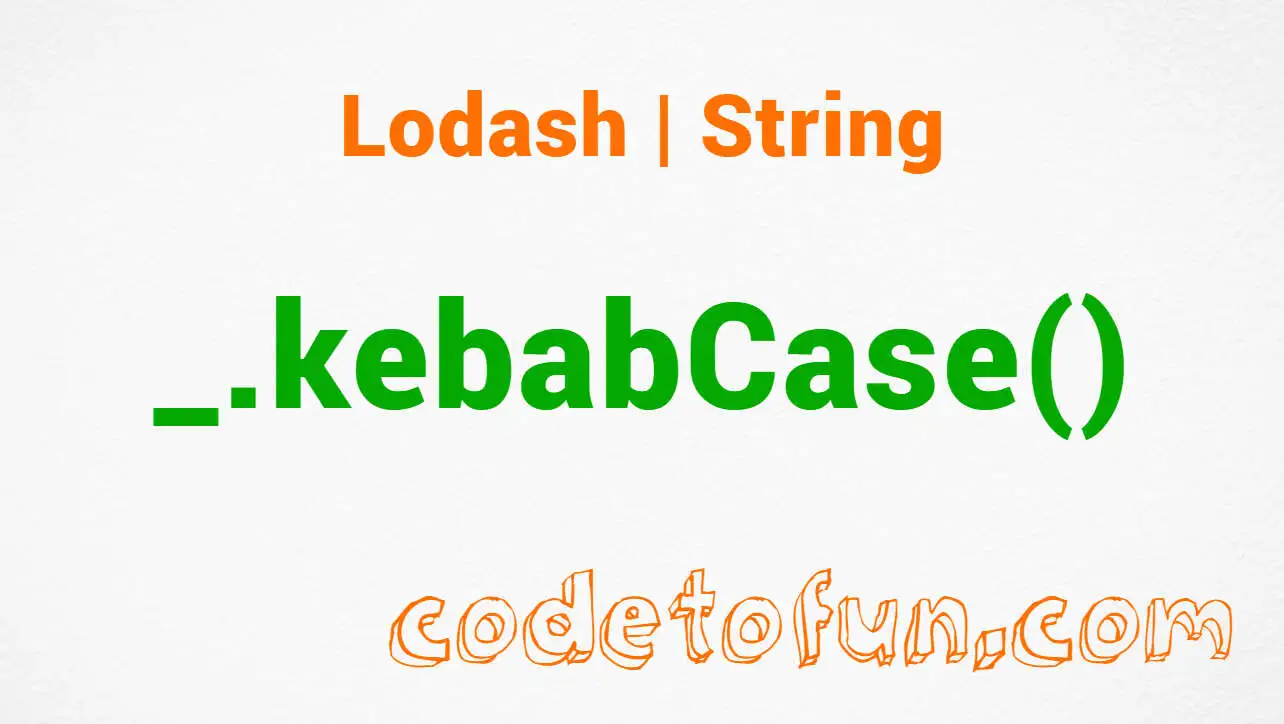
Photo Credit to CodeToFun
π Introduction
String manipulation is a common task in JavaScript development, especially when dealing with user inputs or data formatting. Lodash, a popular utility library, offers a plethora of functions to simplify such tasks. Among these functions is _.kebabCase()
, which converts a string to kebab case by replacing spaces and capital letters with hyphens.
This method is invaluable for ensuring consistency in data formatting and URL creation.
π§ Understanding _.kebabCase() Method
The _.kebabCase()
method in Lodash transforms a string into kebab case, also known as spinal case, where each word is separated by a hyphen ("-"). This is particularly useful for creating SEO-friendly URLs, CSS classes, and other identifiers.
π‘ Syntax
The syntax for the _.kebabCase()
method is straightforward:
_.kebabCase([string=''])
- string: The string to convert to kebab case.
π Example
Let's dive into a simple example to illustrate the usage of the _.kebabCase()
method:
const _ = require('lodash');
const inputString = 'Hello World!';
const kebabCaseString = _.kebabCase(inputString);
console.log(kebabCaseString);
// Output: hello-world
In this example, the inputString is converted to kebab case using _.kebabCase()
, resulting in a new string with hyphens separating words.
π Best Practices
When working with the _.kebabCase()
method, consider the following best practices:
Handle Special Characters:
Be mindful of special characters or punctuation marks in the input string. Ensure that they are appropriately handled or removed to maintain the integrity of the kebab case format.
example.jsCopiedconst inputString = 'Hello, World!'; const kebabCaseString = _.kebabCase(inputString); console.log(kebabCaseString); // Output: hello-world
Unicode Support:
Consider the Unicode support provided by
_.kebabCase()
when working with international or non-English characters. This ensures consistent conversion across various languages.example.jsCopiedconst inputString = 'ΠΡΠΈΠ²Π΅Ρ ΠΠΈΡ'; const kebabCaseString = _.kebabCase(inputString); console.log(kebabCaseString); // Output: ΠΏΡΠΈΠ²Π΅Ρ-ΠΌΠΈΡ
Consistent Formatting:
Maintain consistency in string formatting throughout your application by utilizing
_.kebabCase()
consistently, especially when generating URLs, CSS classes, or identifiers.example.jsCopiedconst userInput = 'User Profile'; const formattedURL = '/users/' + _.kebabCase(userInput); console.log(formattedURL); // Output: /users/user-profile
π Use Cases
URL Creation:
_.kebabCase()
is ideal for generating SEO-friendly URLs by converting user-provided titles or identifiers into kebab case format.example.jsCopiedconst pageTitle = 'Lodash _.kebabCase() String Method Explained'; const pageURL = '/articles/' + _.kebabCase(pageTitle); console.log(pageURL); // Output: /articles/lodash-kebab-case-string-method-explained
CSS Class Naming:
When defining CSS classes dynamically based on user inputs or data,
_.kebabCase()
ensures consistent and readable class names.example.jsCopiedconst componentName = 'Header Component'; const cssClassName = _.kebabCase(componentName); console.log(cssClassName); // Output: header-component
Identifier Formatting:
Use
_.kebabCase()
to format identifiers or keys in APIs, databases, or configuration files for better readability and consistency.example.jsCopiedconst apiKey = 'API_KEY'; const formattedKey = _.kebabCase(apiKey); console.log(formattedKey); // Output: api-key
π Conclusion
The _.kebabCase()
method in Lodash provides a simple yet powerful solution for converting strings to kebab case format. Whether you're creating SEO-friendly URLs, defining CSS classes, or formatting identifiers, _.kebabCase()
ensures consistency and readability in your codebase.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.kebabCase()
method in your Lodash projects.
π¨βπ» Join our Community:
Author
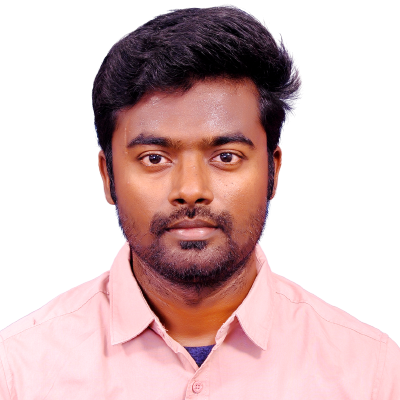
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
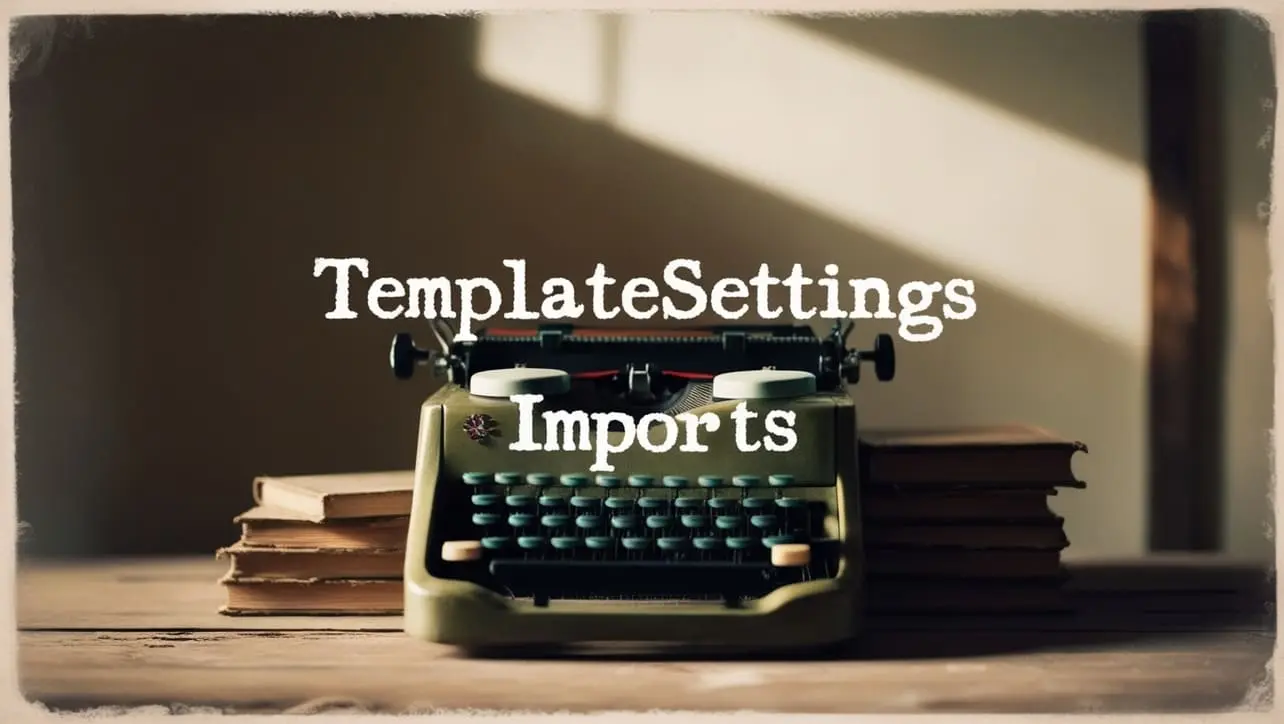
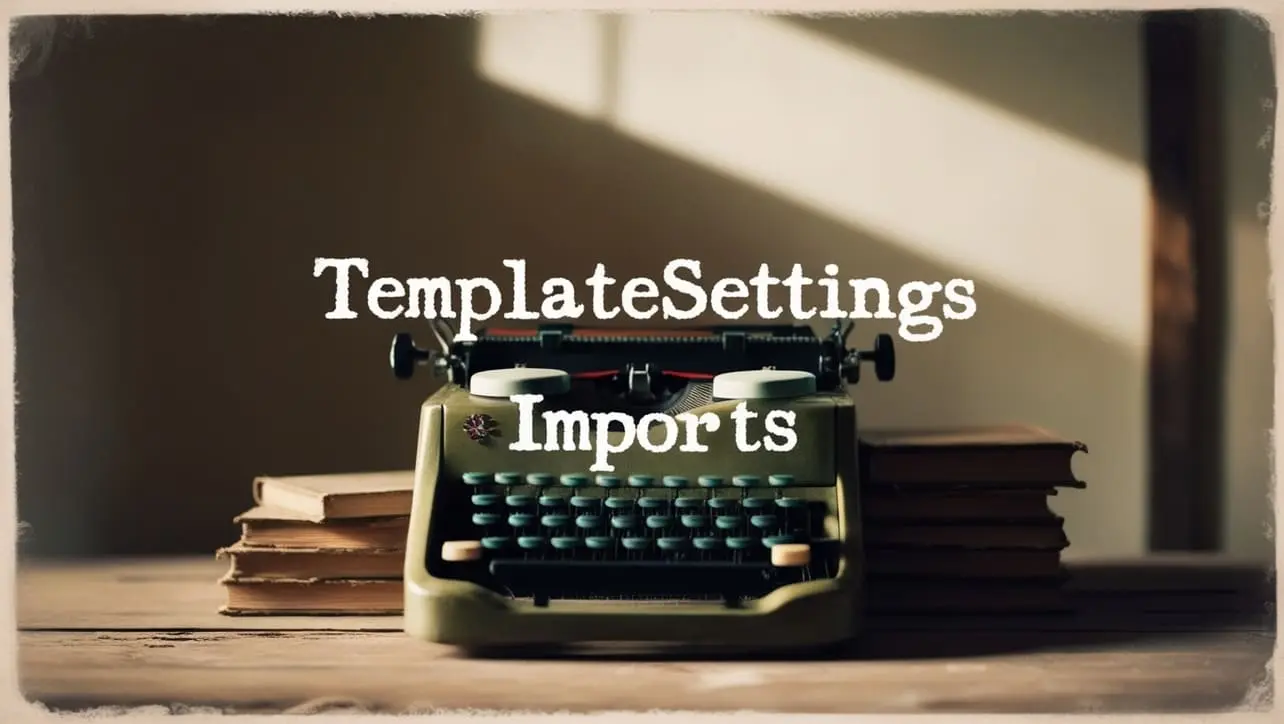
Lodash _.templateSettings.imports Property
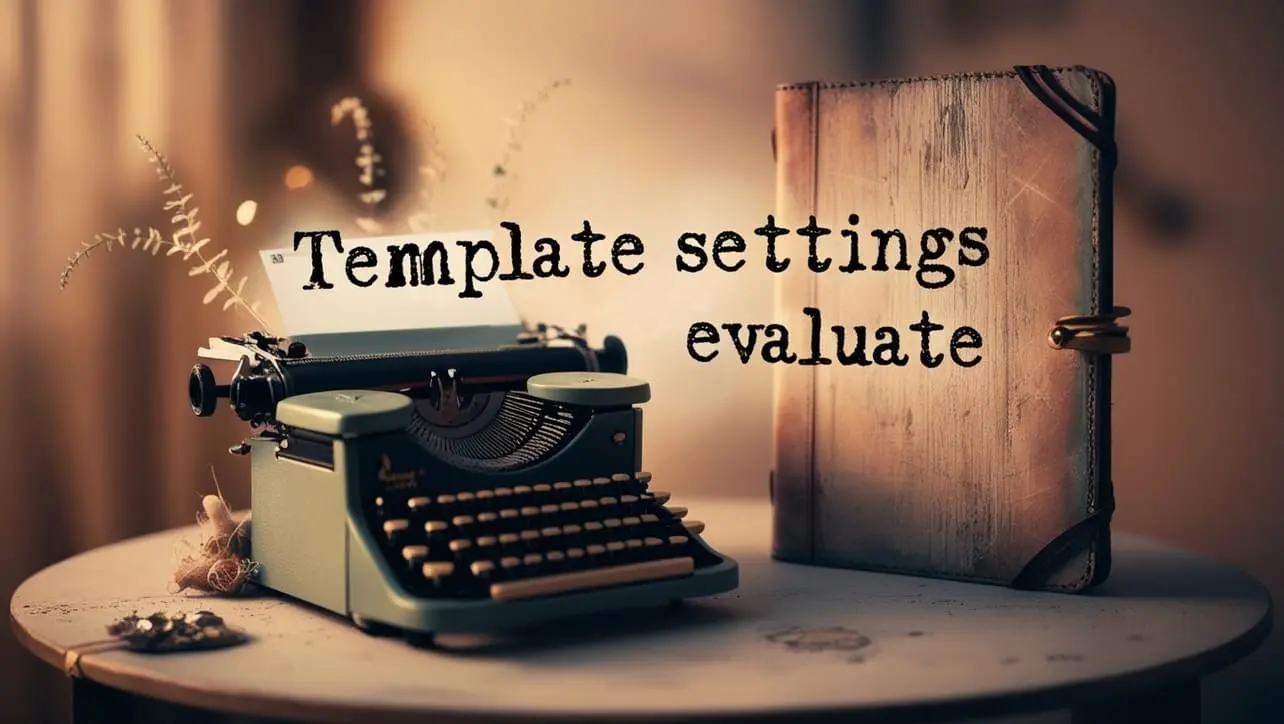
Lodash _.templateSettings.evaluate Property
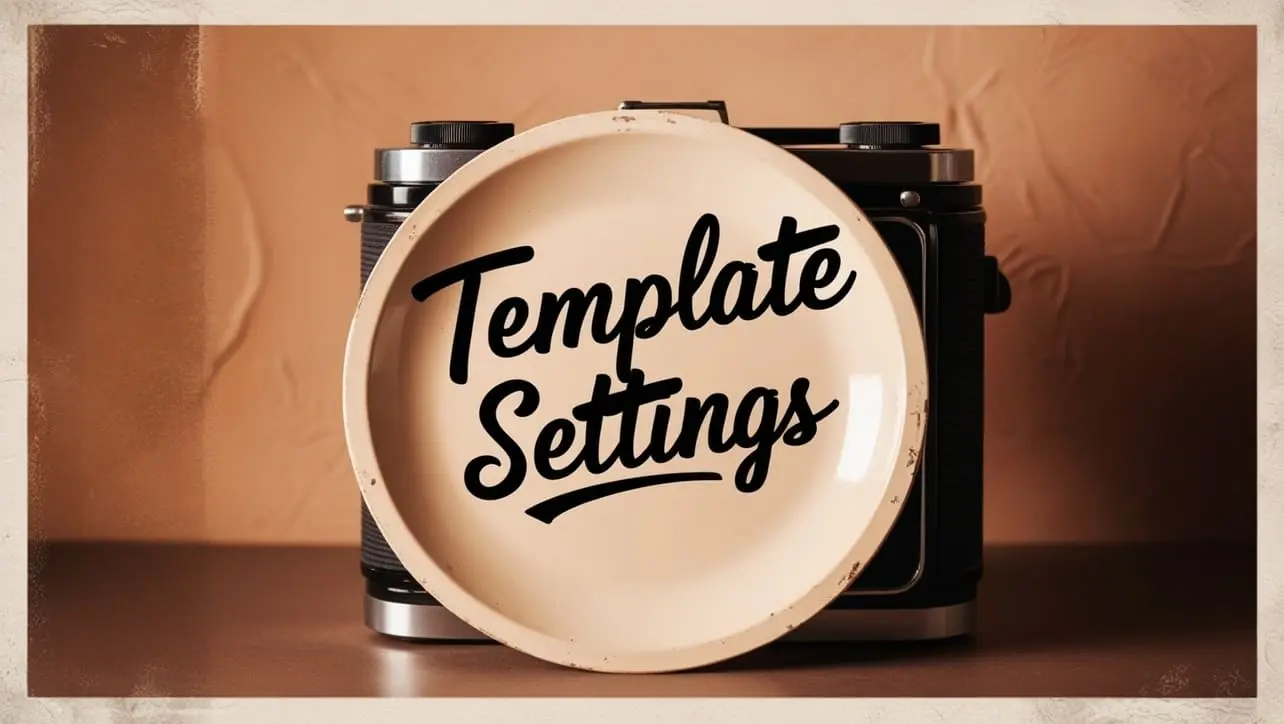
Lodash _.templateSettings Property
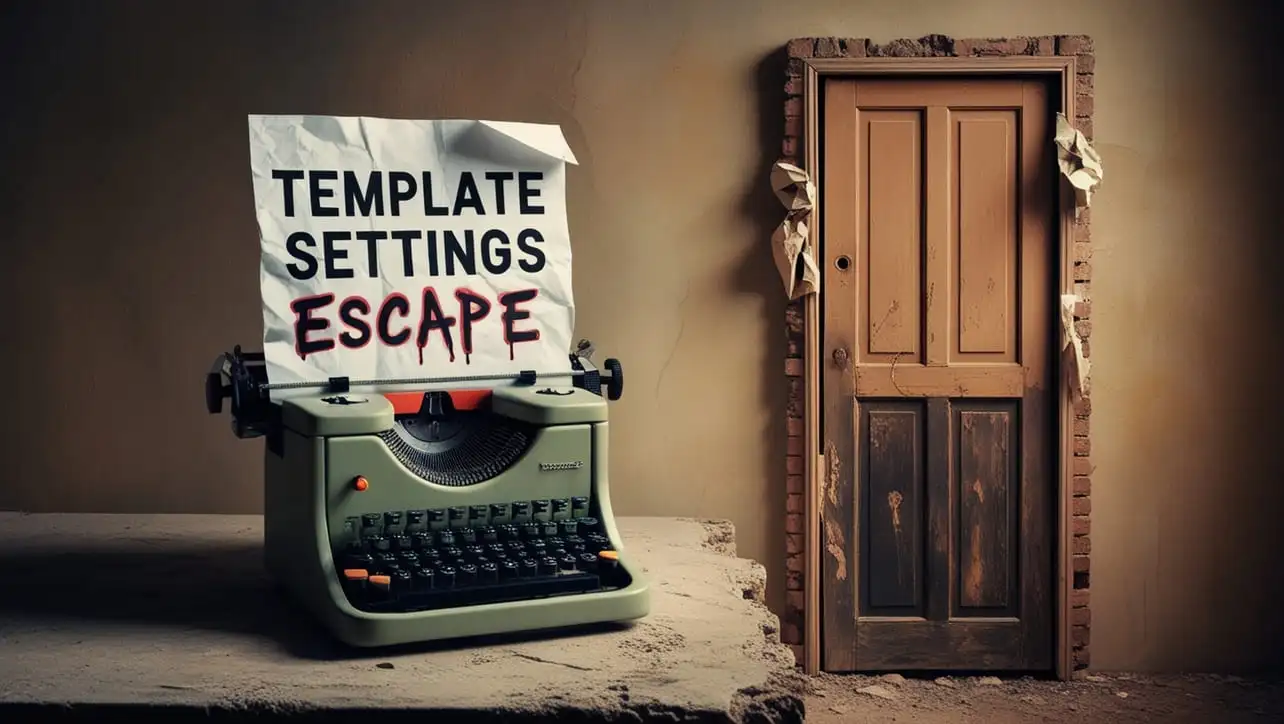
Lodash _.templateSettings.escape Property
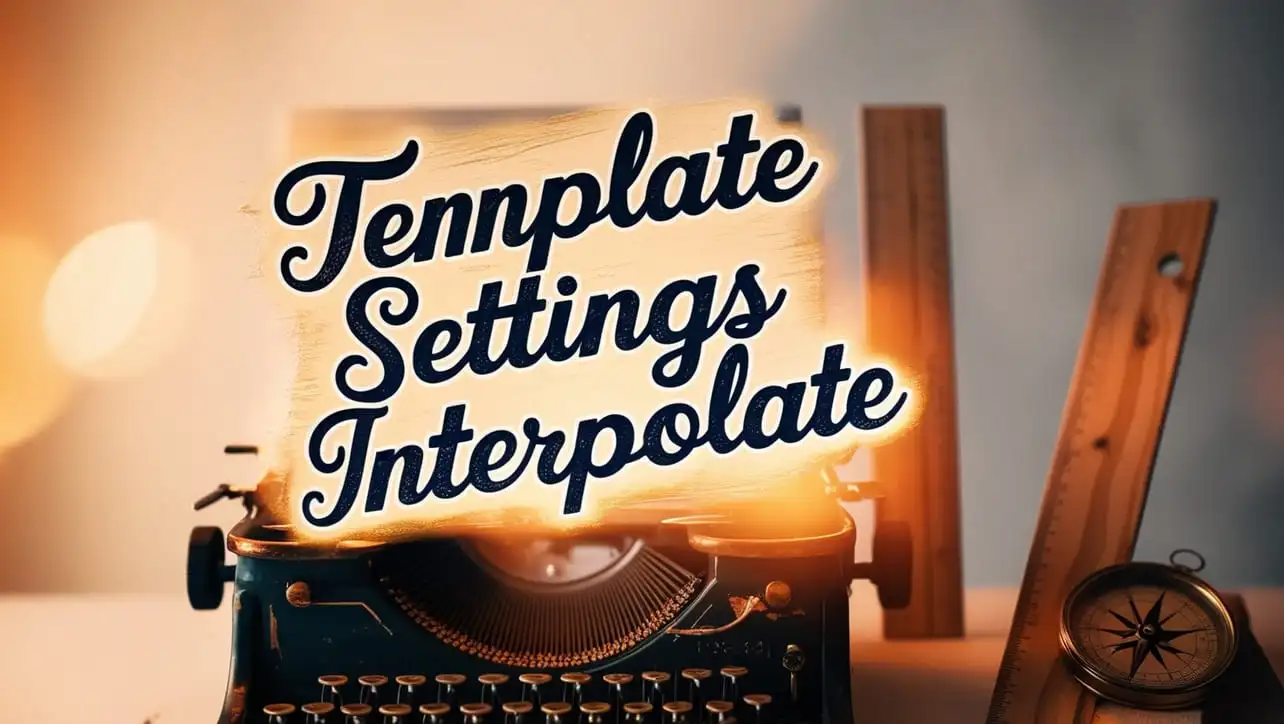
Lodash _.templateSettings.interpolate Property
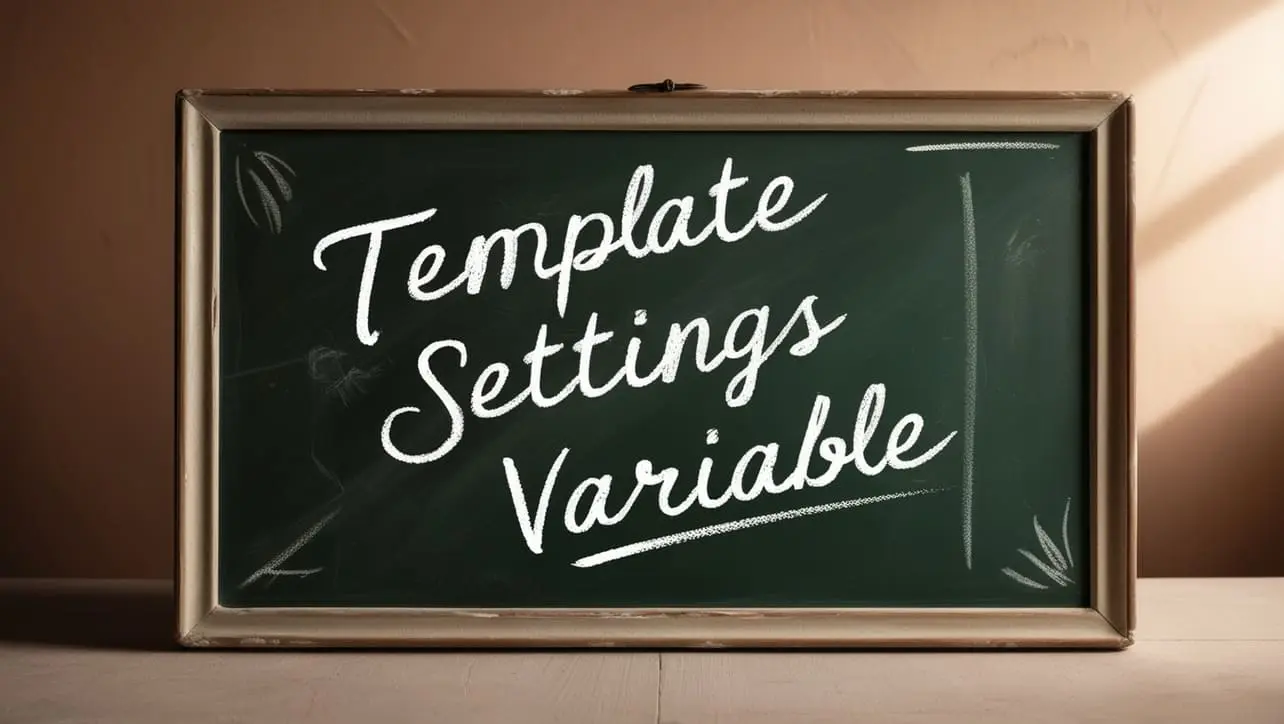
If you have any doubts regarding this article (Lodash _.kebabCase() String Method), please comment here. I will help you immediately.