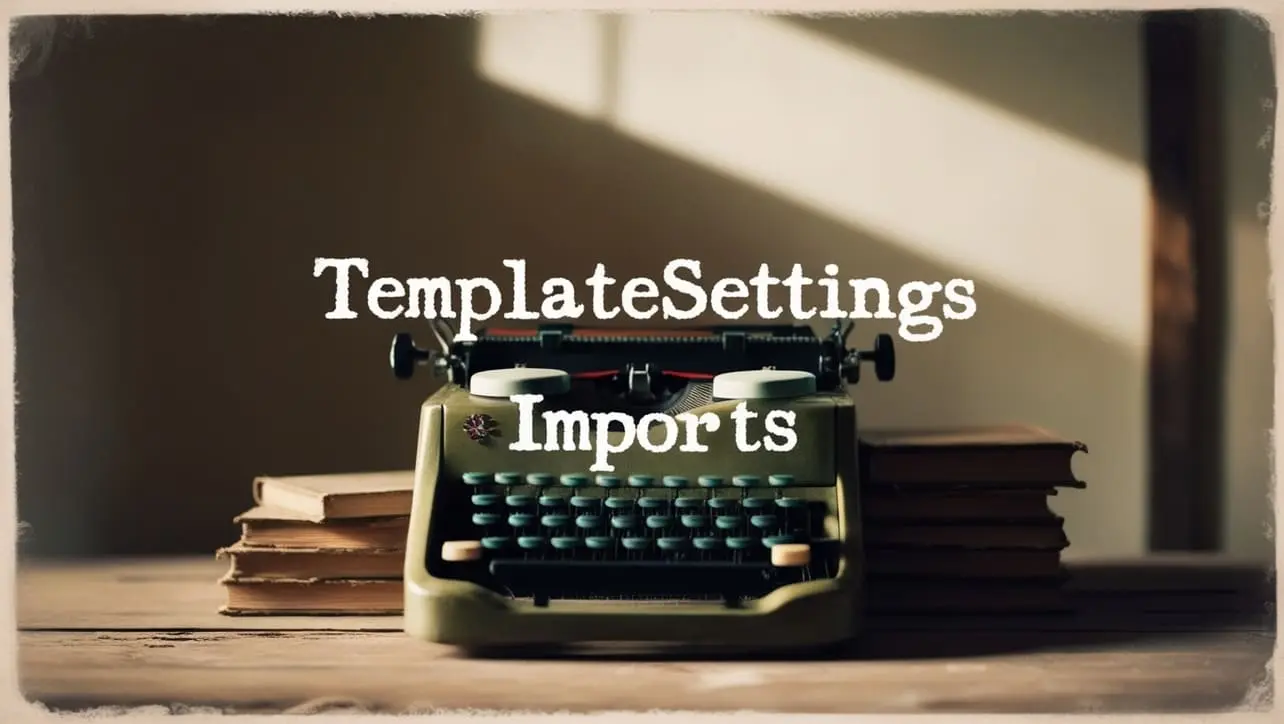
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- Lodash Seq
- Lodash String
- _.camelCase
- _.capitalize
- _.deburr
- _.endsWith
- _.escape
- _.escapeRegExp
- _.kebabCase
- _.lowerCase
- _.lowerFirst
- _.pad
- _.padEnd
- _.padStart
- _.parseInt
- _.repeat
- _.replace
- _.snakeCase
- _.split
- _.startCase
- _.startsWith
- _.template
- _.toLower
- _.toUpper
- _.trim
- _.trimEnd
- _.trimStart
- _.truncate
- _.unescape
- _.upperCase
- _.upperFirst
- _.words
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.camelCase() String Method
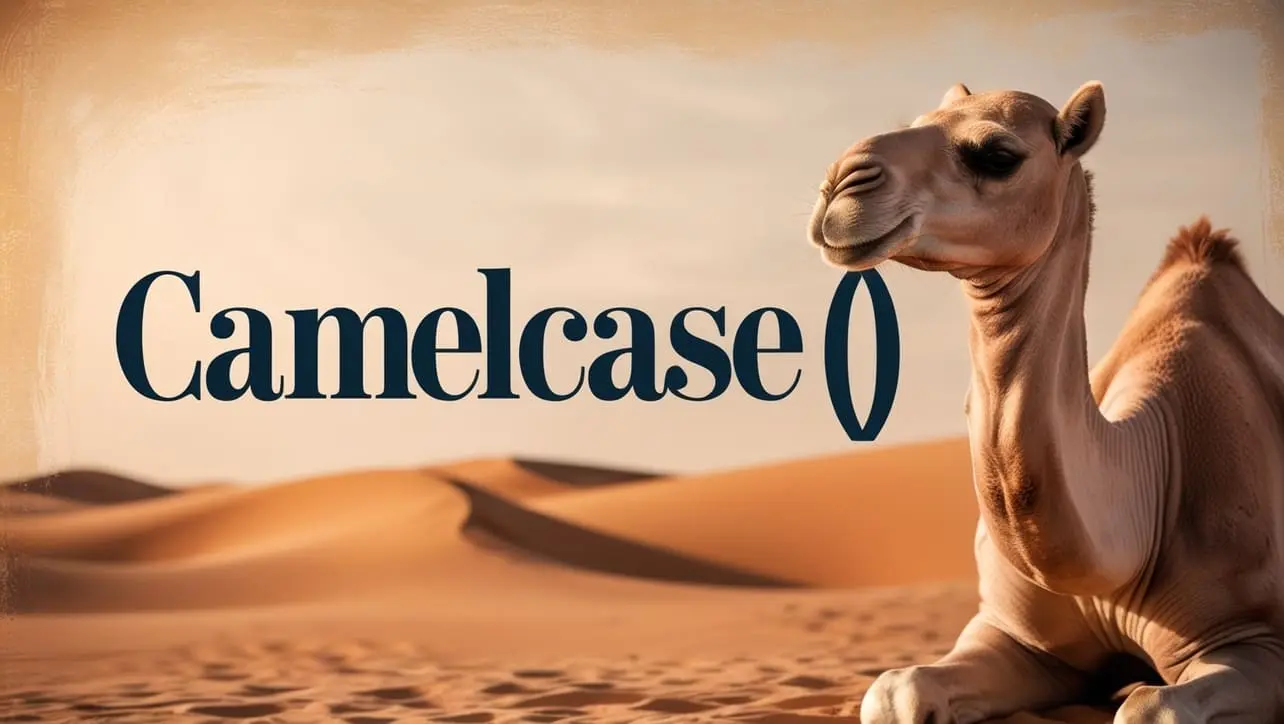
Photo Credit to CodeToFun
🙋 Introduction
String manipulation is a common task in JavaScript development, and having tools to streamline this process can greatly enhance productivity. Lodash, a popular utility library, offers a wide range of functions for simplifying various programming tasks. Among these functions is _.camelCase()
, which is particularly useful for converting strings to camel case format.
This method aids in standardizing naming conventions and improving code readability.
🧠 Understanding _.camelCase() Method
The _.camelCase()
method in Lodash converts a string to camel case format by removing non-alphanumeric characters and capitalizing subsequent words. This is a common convention used in JavaScript for naming variables, properties, and functions.
💡 Syntax
The syntax for the _.camelCase()
method is straightforward:
_.camelCase([string=''])
- string: The string to convert to camel case format.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.camelCase()
method:
const _ = require('lodash');
const kebabCaseString = 'hello-world';
const camelCaseString = _.camelCase(kebabCaseString);
console.log(camelCaseString);
// Output: helloWorld
In this example, the kebabCaseString is converted to camel case format using _.camelCase()
.
🏆 Best Practices
When working with the _.camelCase()
method, consider the following best practices:
Consistent Naming Conventions:
Maintain consistency in naming conventions throughout your codebase. Use
_.camelCase()
consistently to ensure uniformity in variable, property, and function names.example.jsCopiedconst snake_case_variable = 'value'; const camelCaseVariable = _.camelCase(snake_case_variable);
Handling Special Characters:
Be mindful of special characters and their treatment by
_.camelCase()
. Non-alphanumeric characters are removed, and subsequent words are capitalized. Ensure that this behavior aligns with your naming conventions.example.jsCopiedconst stringWithSpecialChars = 'my_variable-name'; const camelCaseString = _.camelCase(stringWithSpecialChars); console.log(camelCaseString); // Output: myVariableName
Performance Considerations:
Consider the performance implications of using
_.camelCase()
on large volumes of data or within performance-sensitive code paths. While generally efficient, excessive use of string manipulation functions may impact performance.example.jsCopiedconst largeDataset = /* ...fetch data from API or elsewhere... */; const transformedData = largeDataset.map(item => _.camelCase(item)); console.log(transformedData);
📚 Use Cases
Data Transformation:
_.camelCase()
is commonly used for transforming data fetched from external sources, such as APIs or databases, into a format consistent with JavaScript naming conventions.example.jsCopiedconst responseData = /* ...fetch data from API... */; const camelCaseData = responseData.map(item => _.camelCase(item)); console.log(camelCaseData);
Property Access:
When working with objects retrieved from external sources,
_.camelCase()
can be used to convert property names to camel case format, facilitating easier access and manipulation.example.jsCopiedconst apiResponse = /* ...fetch data from API... */; const camelCaseResponse = {}; for (const key in apiResponse) { camelCaseResponse[_.camelCase(key)] = apiResponse[key]; } console.log(camelCaseResponse);
Code Refactoring:
During code refactoring,
_.camelCase()
can be employed to standardize variable and function names, improving code readability and maintainability.example.jsCopiedconst OLD_CONST = 42; const newConst = _.camelCase('new_const');
🎉 Conclusion
The _.camelCase()
method in Lodash offers a convenient solution for converting strings to camel case format, facilitating consistent naming conventions and enhancing code readability. Whether you're transforming data, accessing object properties, or refactoring code, _.camelCase()
provides a versatile tool for string manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.camelCase()
method in your Lodash projects.
👨💻 Join our Community:
Author
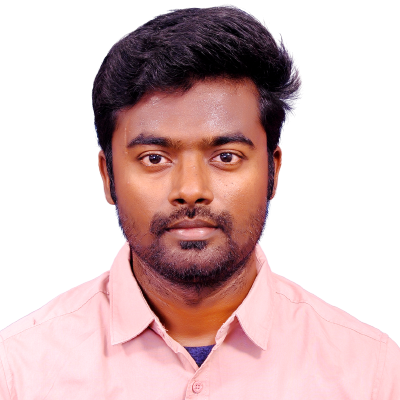
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
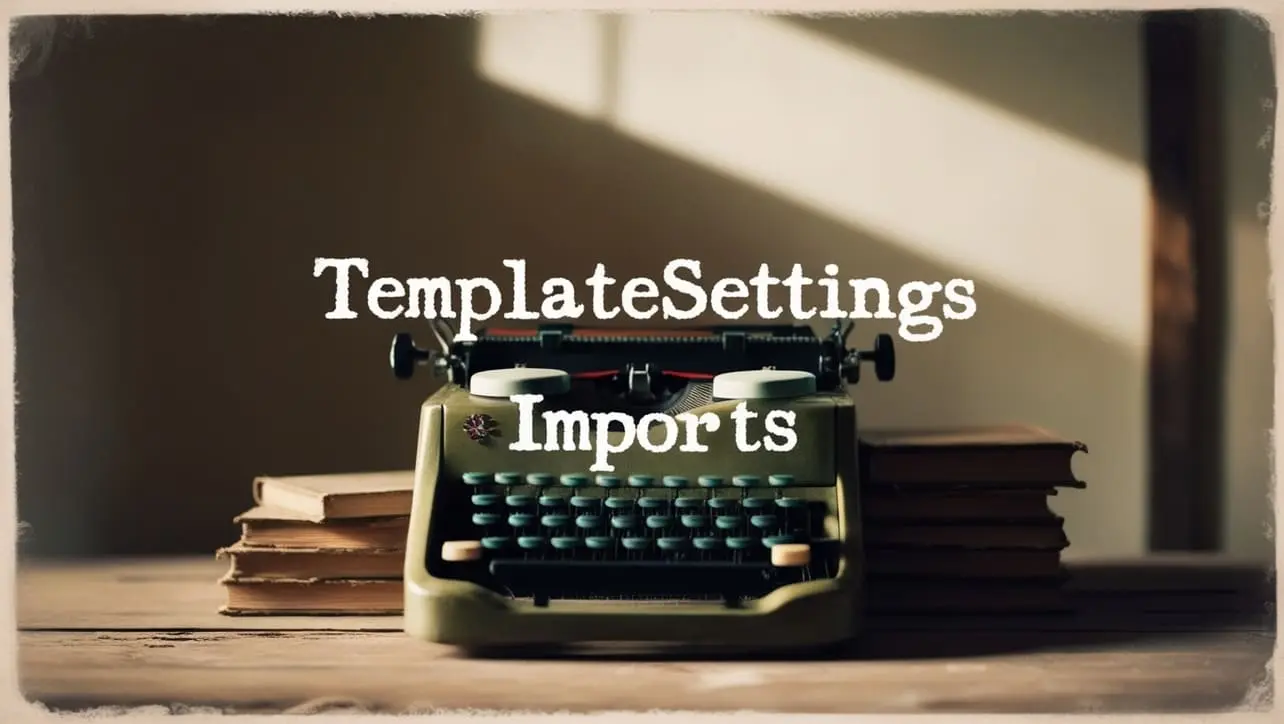
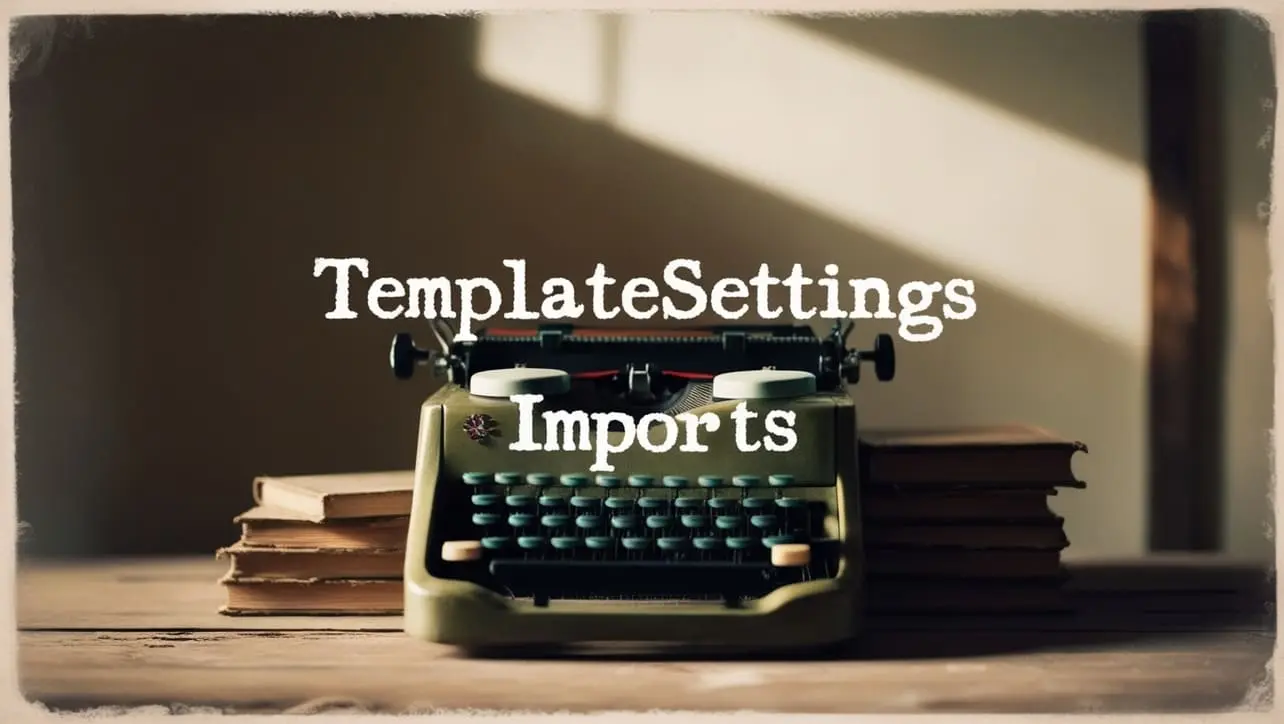
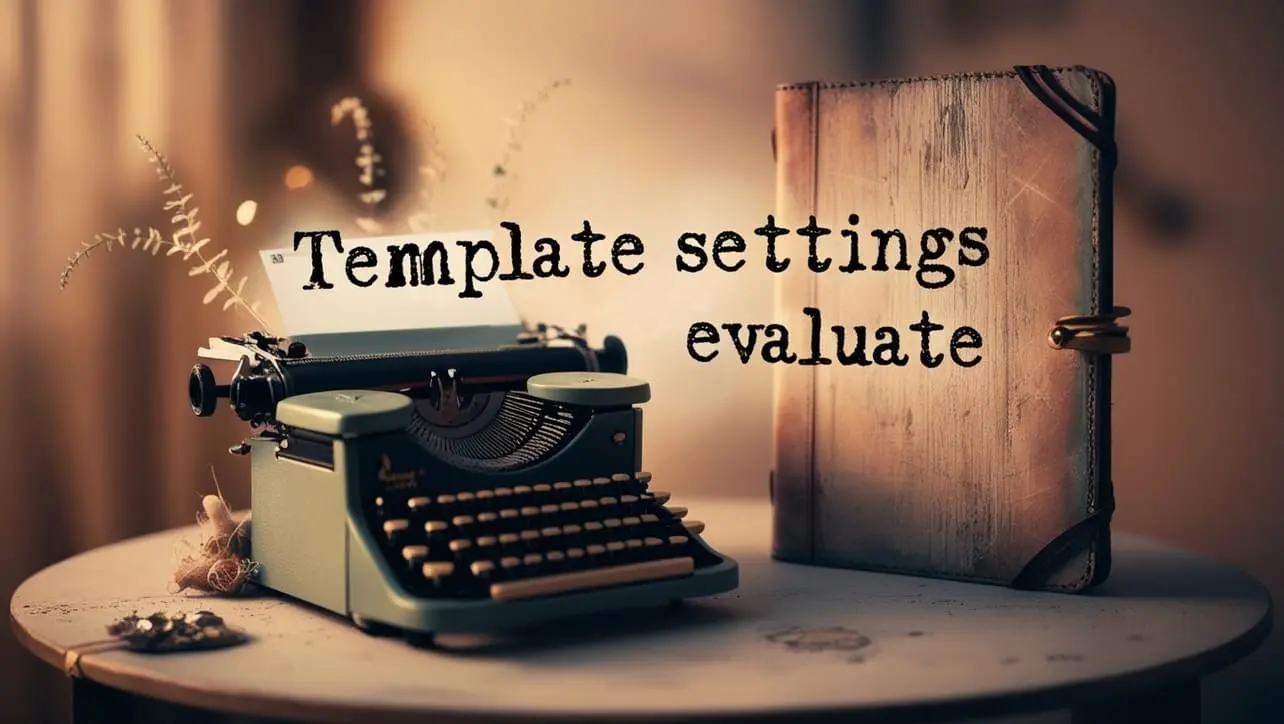
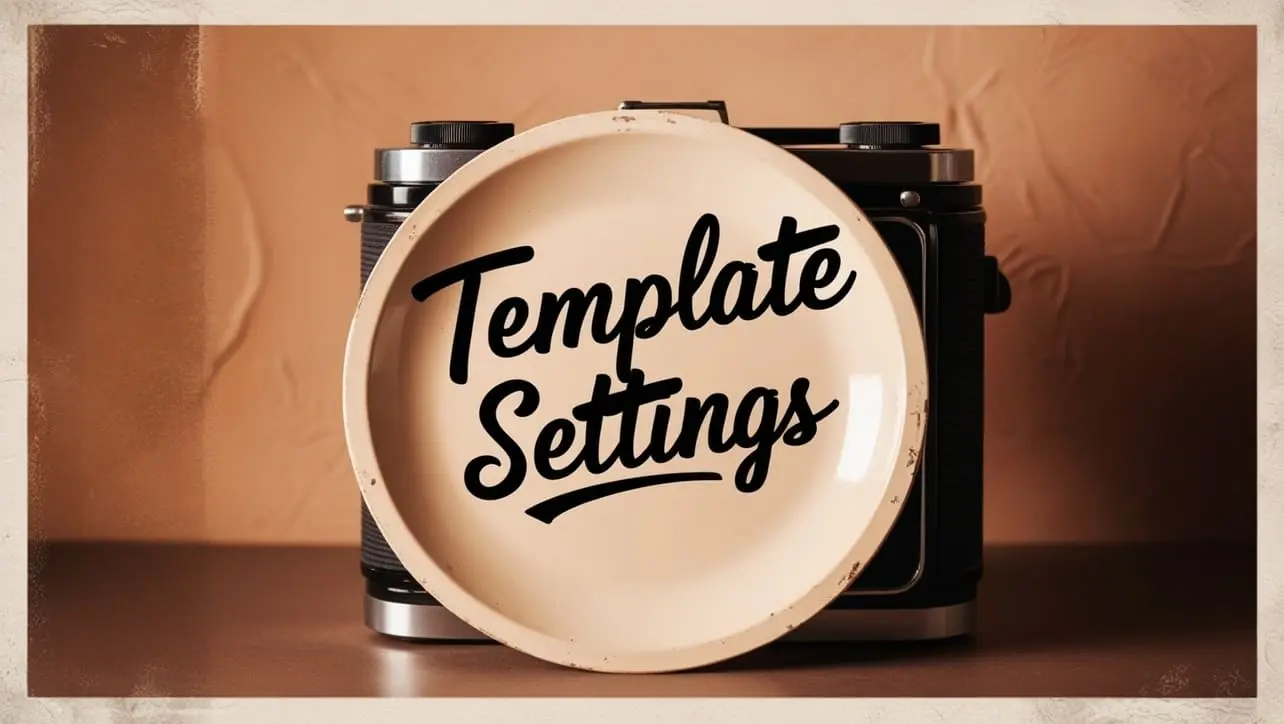
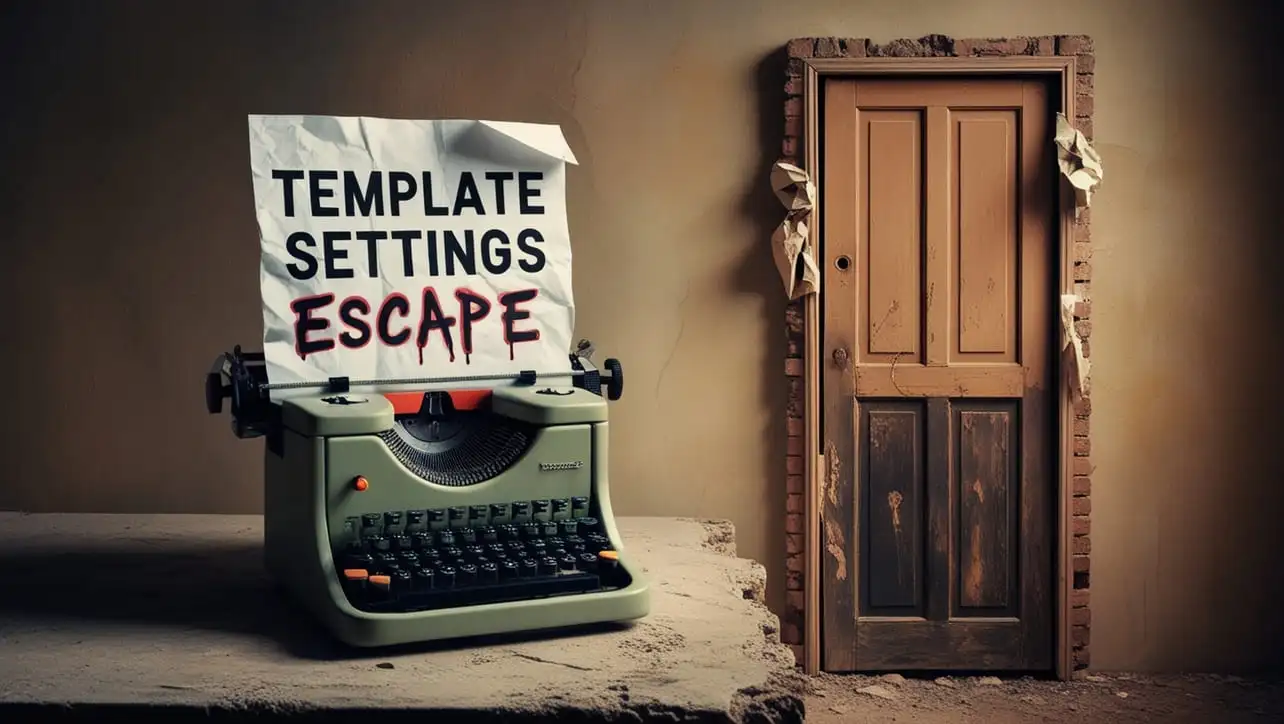
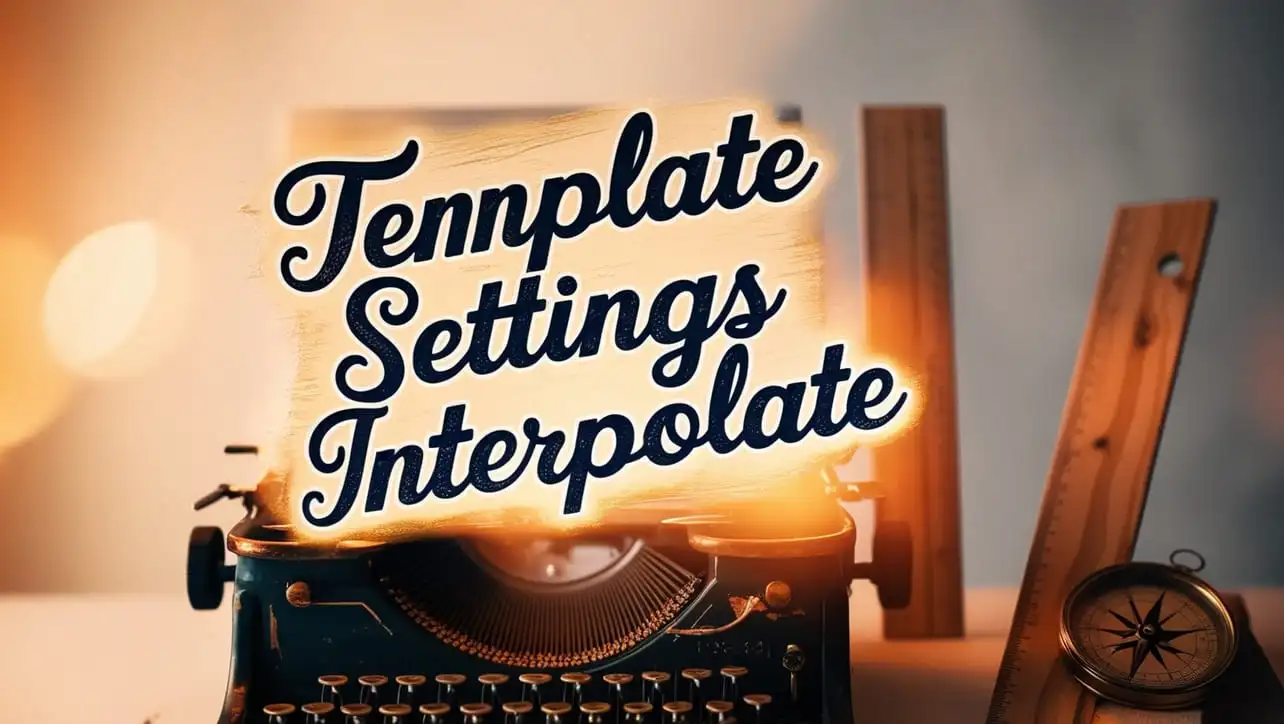
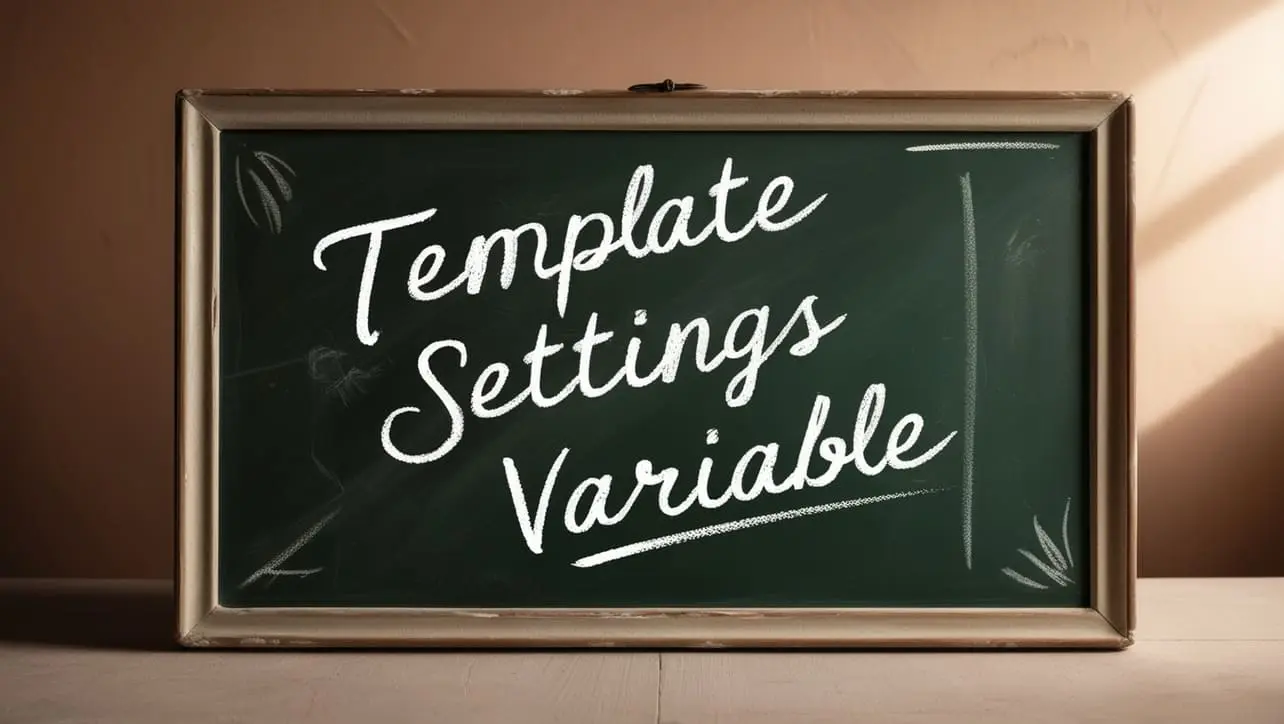
If you have any doubts regarding this article (Lodash _.camelCase() String Method), please comment here. I will help you immediately.