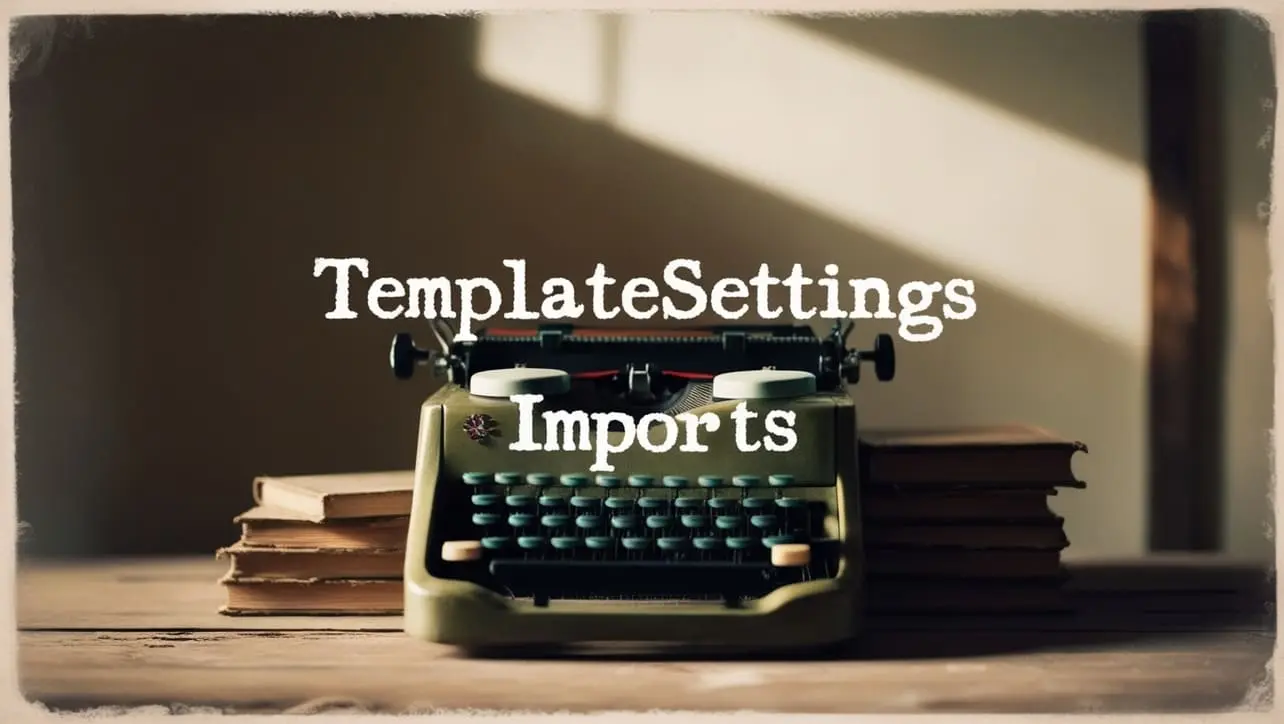
Lodash _.chain() Seq Method
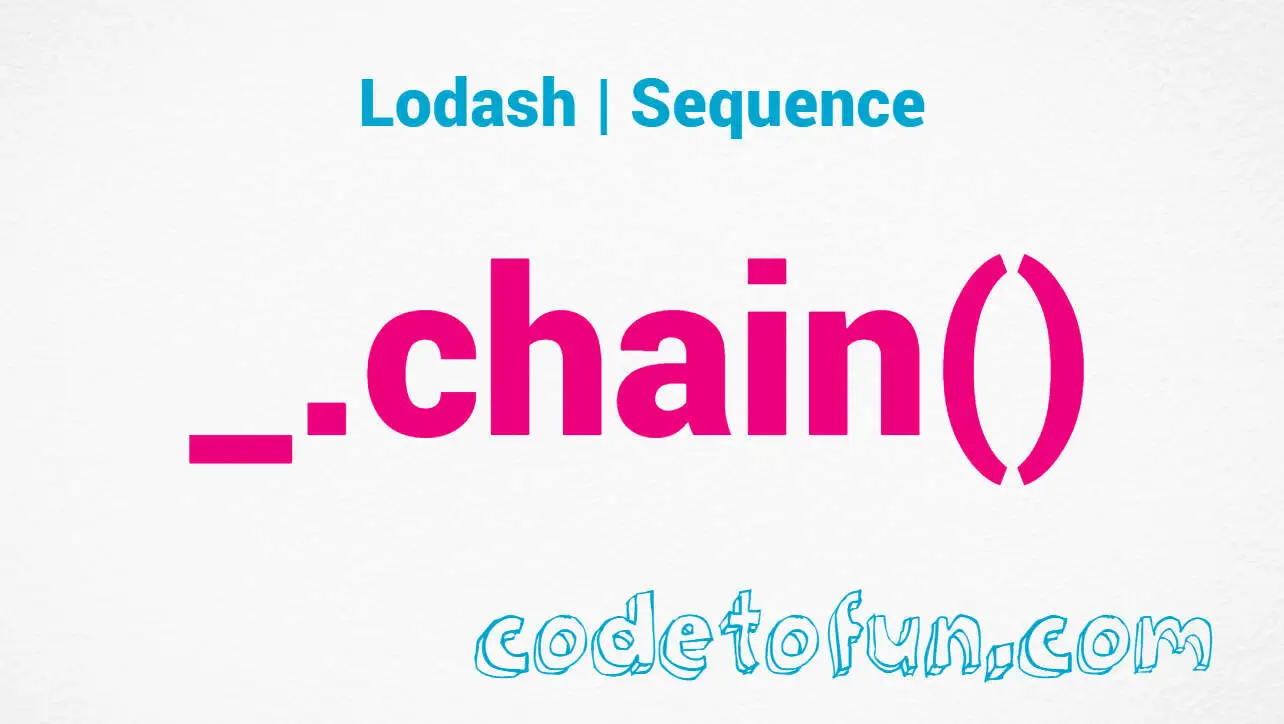
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, efficient data manipulation is crucial for building robust applications. Lodash, a widely-used utility library, provides a variety of methods to streamline common programming tasks. Among these methods is _.chain()
, a powerful function that allows developers to create a chainable sequence of operations on arrays, objects, or other values.
This method enables cleaner and more concise code by facilitating method chaining, making complex data transformations a breeze.
🧠 Understanding _.chain() Method
The _.chain()
method in Lodash creates a chainable sequence of operations on a given value. This allows you to perform a series of transformations or computations in a fluent and concise manner, enhancing code readability and maintainability.
💡 Syntax
The syntax for the _.chain()
method is straightforward:
_.chain(value)
- value: The initial value to start the chain with.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.chain()
method:
const _ = require('lodash');
const data = [1, 2, 3, 4, 5];
const result = _.chain(data)
.map(x => x * 2)
.filter(x => x > 5)
.value();
console.log(result);
// Output: [6, 8, 10]
In this example, the _.chain()
method creates a chainable sequence on the data array. Subsequent map() and filter() operations are applied to the data, resulting in a new array containing the transformed values.
🏆 Best Practices
When working with the _.chain()
method, consider the following best practices:
Understand Method Chaining:
Familiarize yourself with method chaining concepts to leverage the full potential of
_.chain()
. Method chaining allows you to perform multiple operations on a value in a single, fluent sequence.example.jsCopiedconst chainedResult = _.chain(data) .map(x => x * 2) .filter(x => x > 5) .value();
Use Value() to Retrieve Results:
Remember to use the value() method to retrieve the final result of the chain. This step is essential for terminating the chain and obtaining the transformed value.
example.jsCopiedconst result = _.chain(data) .map(x => x * 2) .filter(x => x > 5) .value(); console.log(result);
Keep Chains Concise and Readable:
Maintain clarity and readability by keeping your chain sequences concise. Avoid overly long chains that may become difficult to understand or debug.
example.jsCopiedconst conciseResult = _.chain(data) .map(x => x * 2) .take(3) // Limit the result to the first three elements .value();
📚 Use Cases
Data Transformation:
_.chain()
is ideal for performing complex data transformations, such as mapping, filtering, and sorting, in a single chain sequence.example.jsCopiedconst userData = /* ...fetch user data from API or elsewhere... */ ; const processedData = _.chain(userData) .filter(user => user.age > 18) .sortBy('lastName') .map(user => ({ fullName: `${user.firstName} ${user.lastName}`, age: user.age })) .value(); console.log(processedData);
Fluent API Design:
Leverage
_.chain()
to create fluent APIs for custom objects or libraries, allowing users to perform chained operations on data structures or custom classes.example.jsCopiedclass CustomDataProcessor { constructor(data) { this.data = data; } filterBy(condition) { this.data = this.data.filter(condition); return this; // Return instance for method chaining } sortBy(property) { this.data.sort((a, b) => a[property] - b[property]); return this; // Return instance for method chaining } getData() { return this.data; } } const customProcessor = new CustomDataProcessor(userData); const result = customProcessor .filterBy(user => user.age > 18) .sortBy('lastName') .getData(); console.log(result);
Data Analysis and Aggregation:
Perform data analysis and aggregation tasks by chaining together operations such as grouping, counting, and summarizing data elements.
example.jsCopiedconst salesData = /* ...fetch sales data from API or elsewhere... */ ; const summary = _.chain(salesData) .groupBy('product') .mapValues(products => ({ totalSales: _.sumBy(products, 'quantity'), averagePrice: _.meanBy(products, 'price') })) .value(); console.log(summary);
🎉 Conclusion
The _.chain()
method in Lodash empowers developers to create fluent and concise chains of operations on arrays, objects, or other values. By leveraging method chaining, you can streamline data manipulation tasks, enhance code readability, and design fluent APIs. Whether you're performing complex data transformations, designing custom libraries, or analyzing datasets, _.chain()
provides a versatile and powerful tool for JavaScript development.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.chain()
method in your Lodash projects.
👨💻 Join our Community:
Author
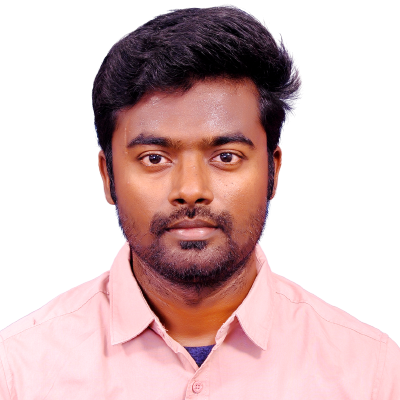
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
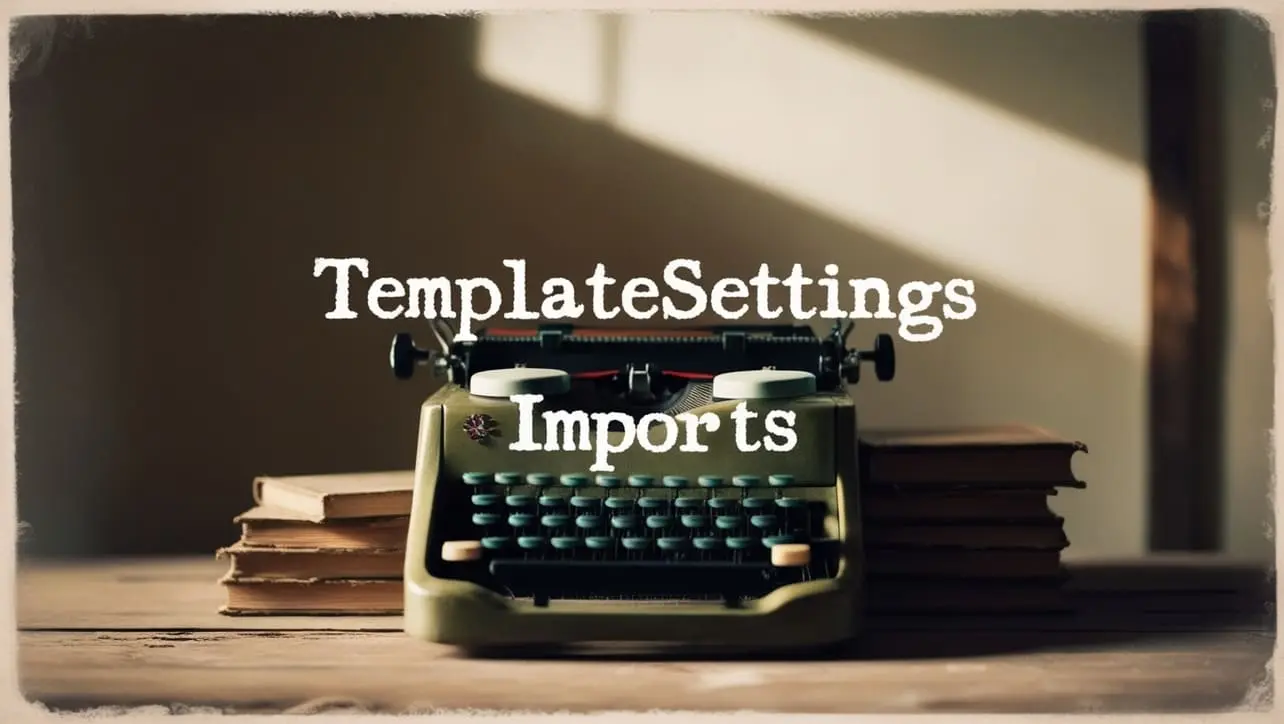
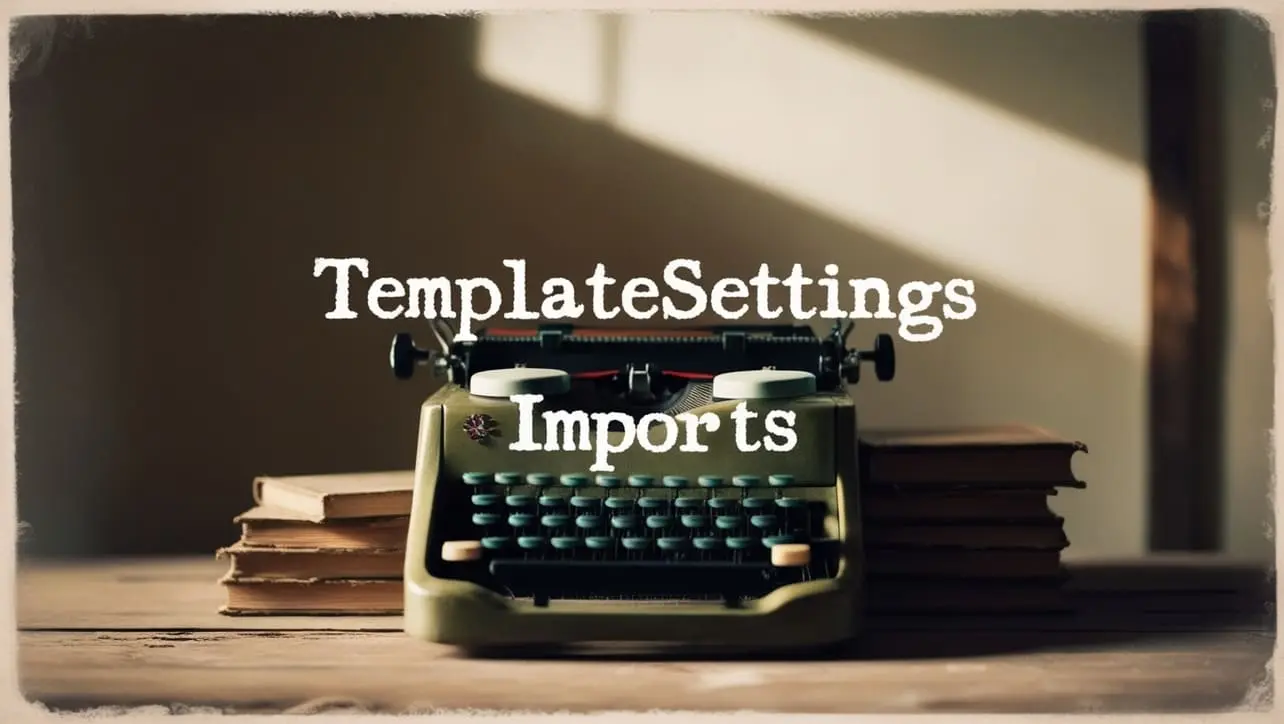
Lodash _.templateSettings.imports Property
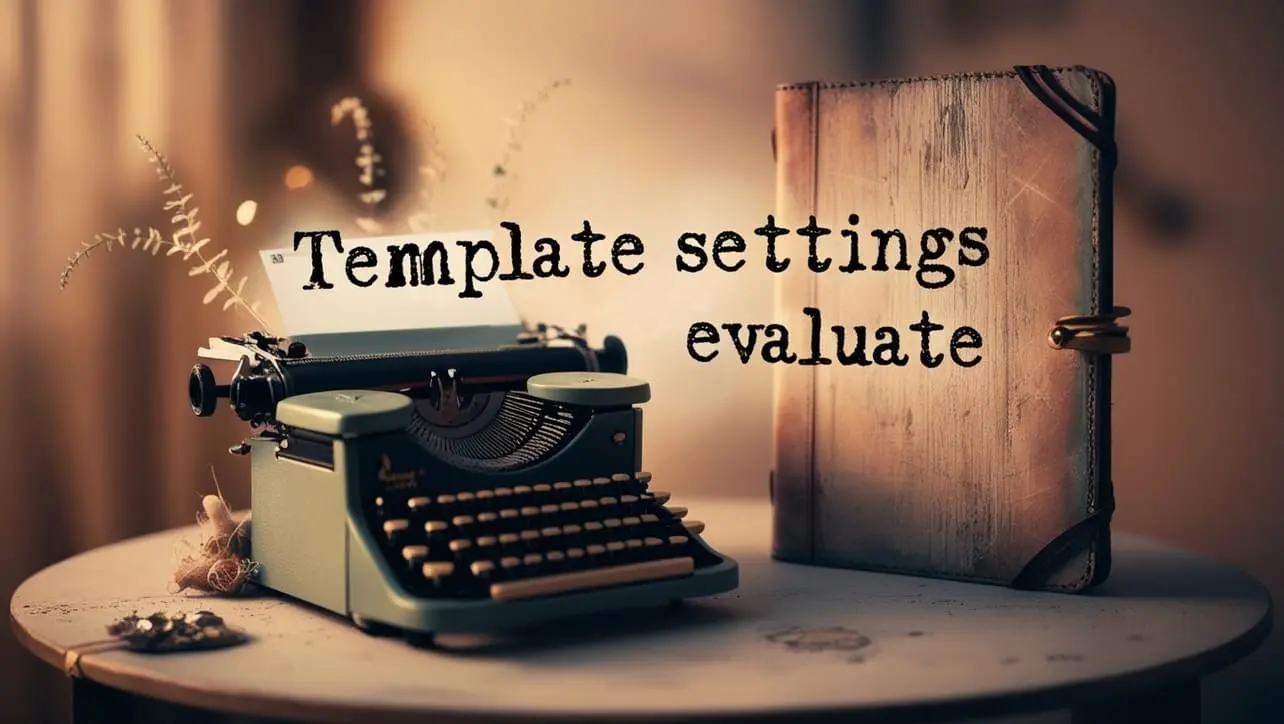
Lodash _.templateSettings.evaluate Property
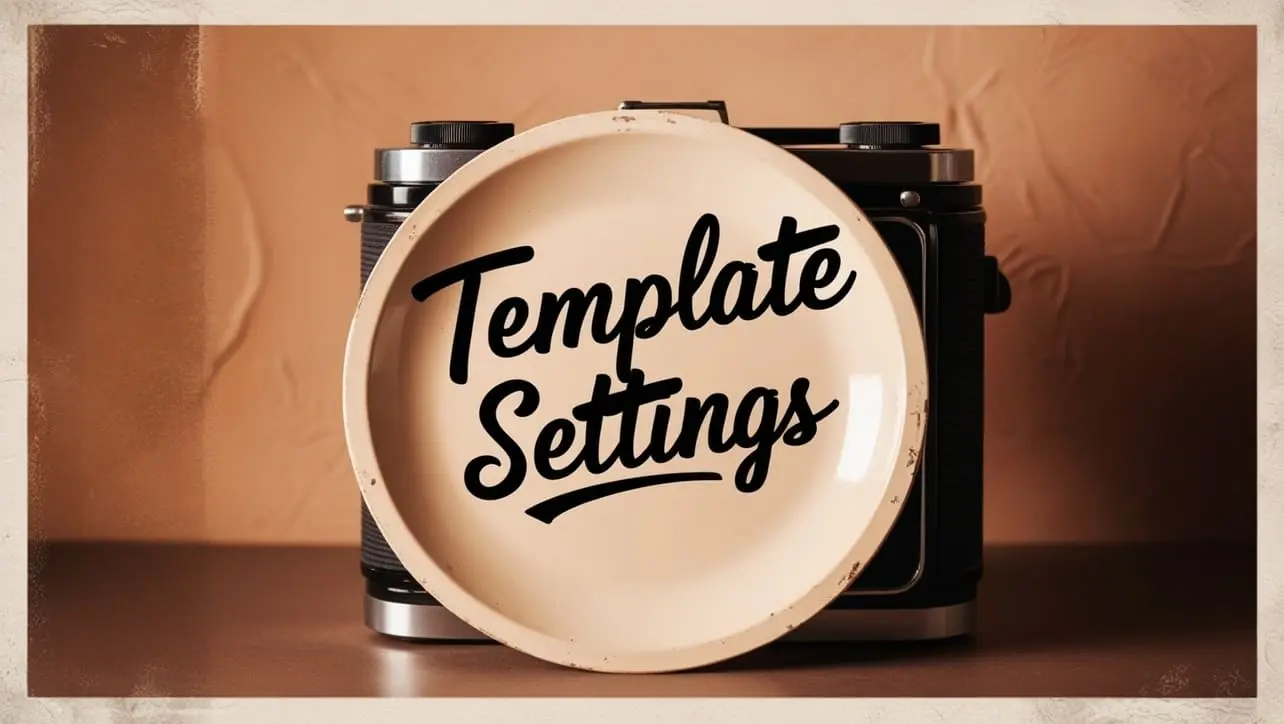
Lodash _.templateSettings Property
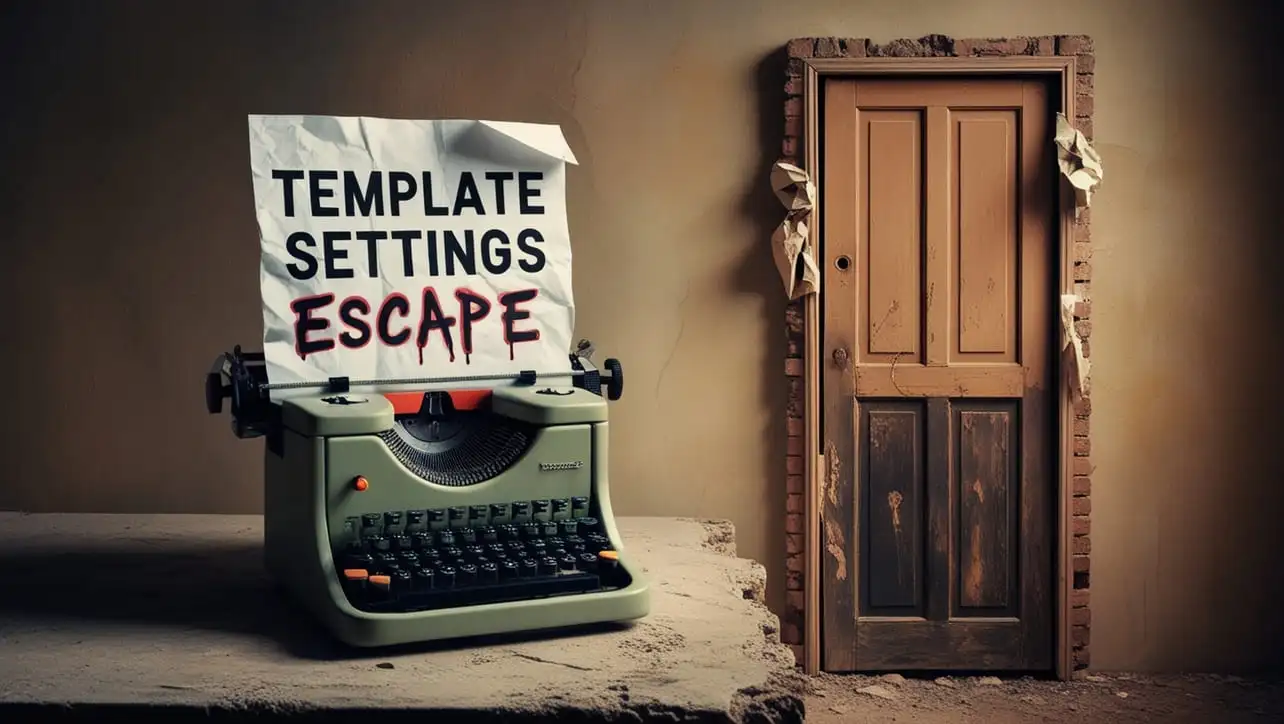
Lodash _.templateSettings.escape Property
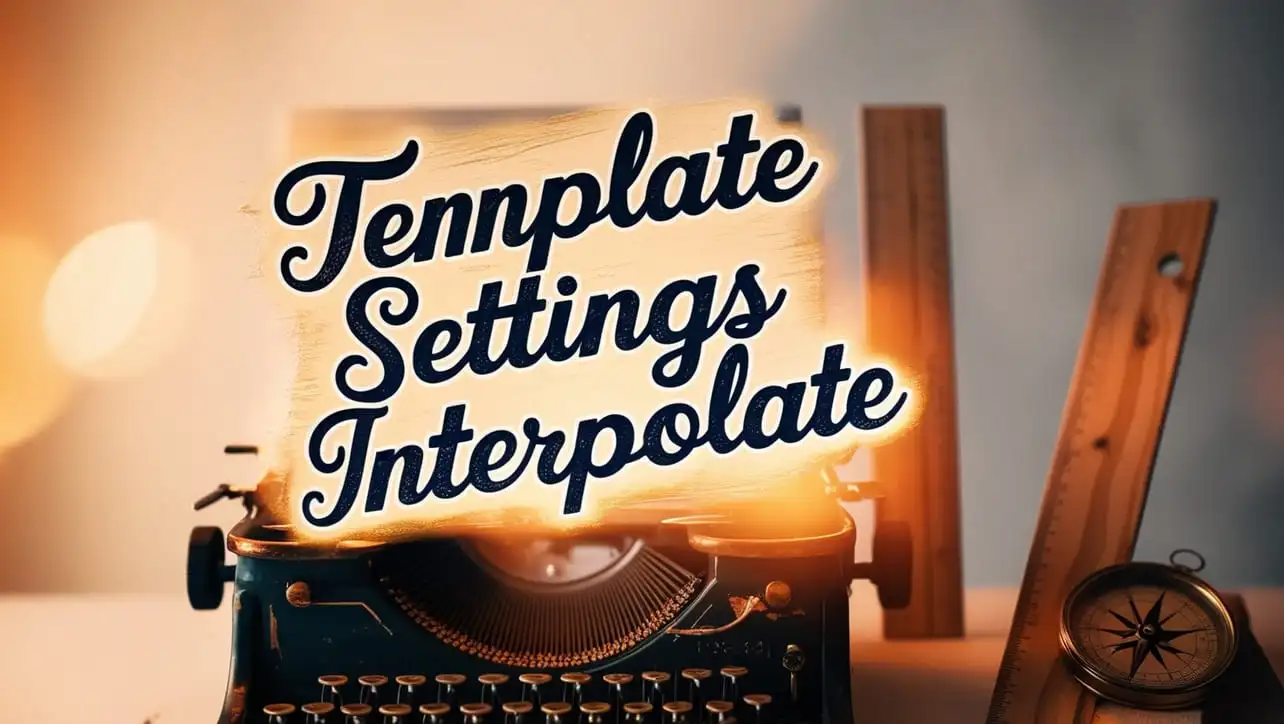
Lodash _.templateSettings.interpolate Property
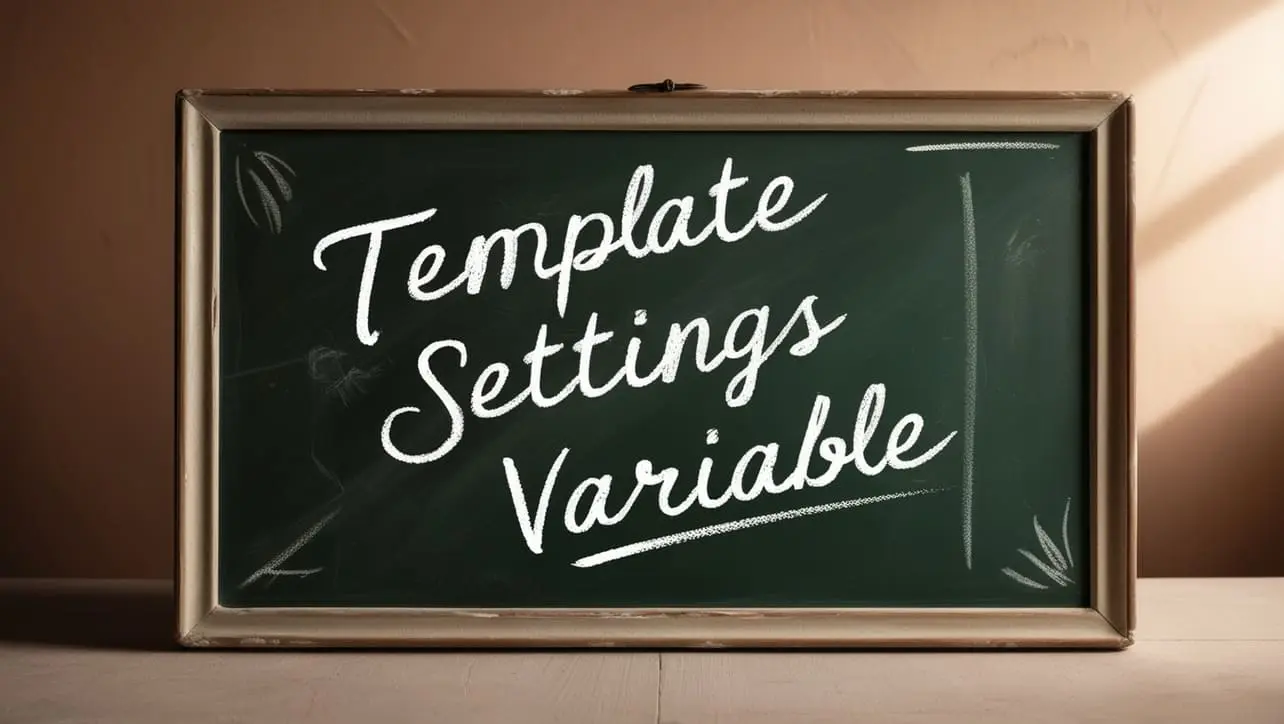
If you have any doubts regarding this article (Lodash _.chain() Seq Method), please comment here. I will help you immediately.