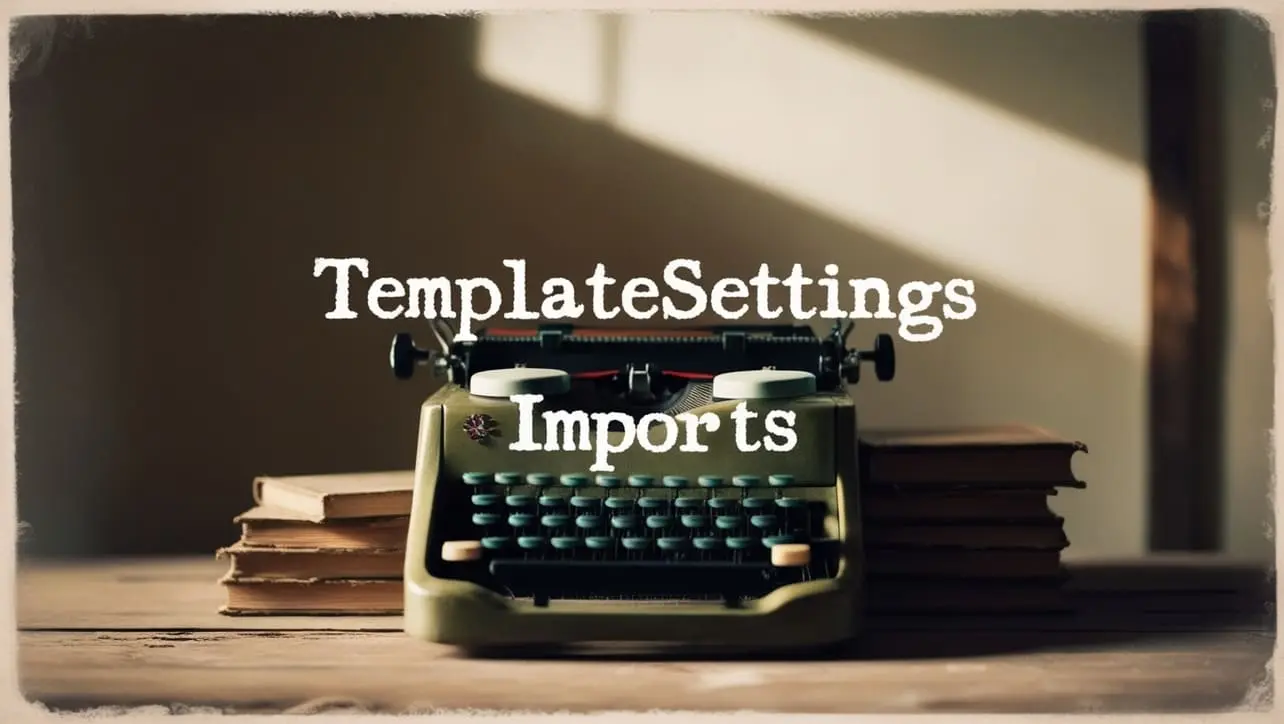
Lodash _.prototype.reverse() Seq Method
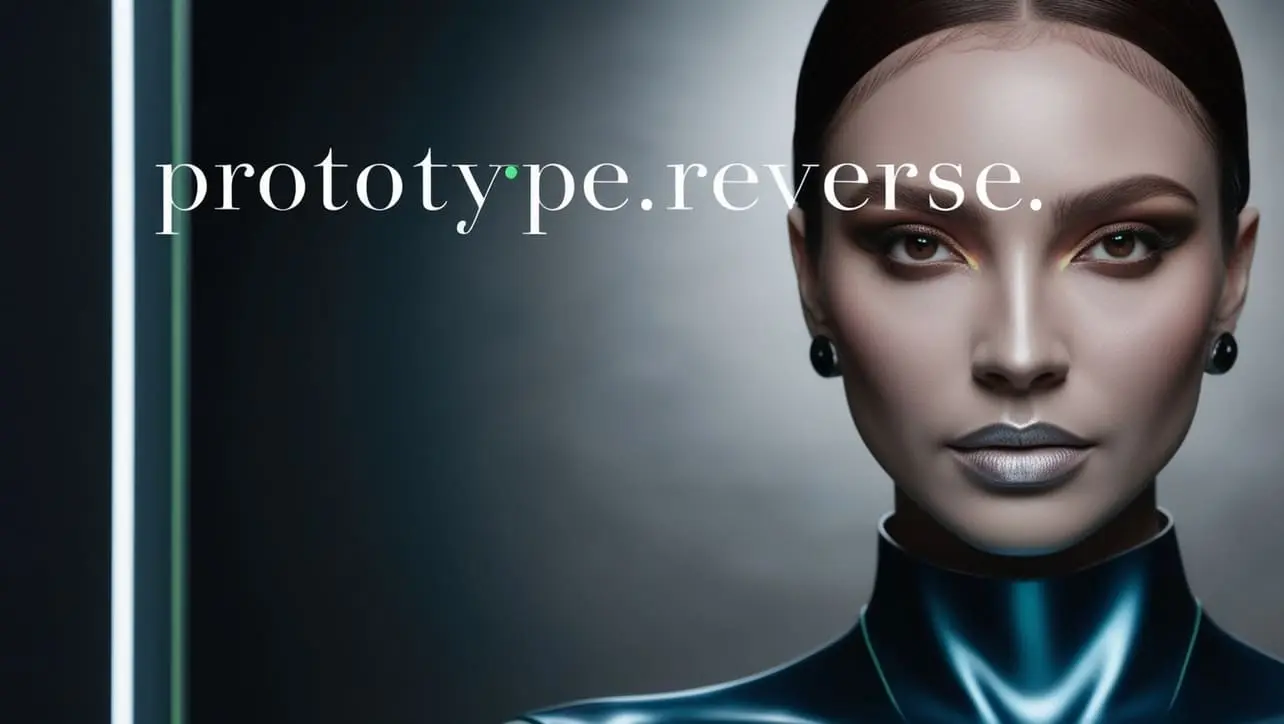
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, efficient manipulation of arrays is crucial for building robust applications. Lodash, a popular utility library, provides a variety of methods to simplify array operations. One such method is the _.prototype.reverse()
Seq method, which enables developers to reverse the order of elements in an array while using a chainable syntax.
Understanding how to utilize this method effectively can enhance code readability and streamline array transformations.
🧠 Understanding _.prototype.reverse() Method
The _.prototype.reverse()
Seq method in Lodash is designed to reverse the order of elements in an array. Unlike the standard Array.reverse() method, _.prototype.reverse()
operates within a Lodash sequence, allowing for seamless integration with other Lodash methods.
💡 Syntax
The syntax for the _.prototype.reverse()
method is straightforward:
_(array).reverse().value()
- array: The array to reverse.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.prototype.reverse()
method:
const _ = require('lodash');
const array = [1, 2, 3];
const reversedArray = _(array).reverse().value();
console.log(reversedArray);
// Output: [3, 2, 1]
console.log(array);
// Output: [3, 2, 1]
In this example, the array is reversed using _.prototype.reverse()
, and the reversed array is returned and logged. Notably, the original array is also modified in place.
🏆 Best Practices
When working with the _.prototype.reverse()
method, consider the following best practices:
Chainable Syntax:
Take advantage of the chainable syntax offered by
_.prototype.reverse()
. By chaining this method with other Lodash functions, you can construct concise and expressive sequences for array manipulation.example.jsCopiedconst array = [1, 2, 3, 4, 5]; const modifiedArray = _(array) .map(value => value * 2) .reverse() .value(); console.log(modifiedArray); // Output: [10, 8, 6, 4, 2]
Immutable Operations:
Be mindful of the potential side effects when using
_.prototype.reverse()
within a sequence. If immutability is desired, consider creating a copy of the original array before applying the reverse operation.example.jsCopiedconst originalArray = [1, 2, 3]; const reversedArray = _(originalArray.slice()) // Create a shallow copy .reverse() .value(); console.log(reversedArray); // Output: [3, 2, 1] console.log(originalArray); // Output: [1, 2, 3]
Performance Considerations:
While Lodash strives for performance optimization, keep in mind the overhead associated with using sequences. For performance-critical applications, evaluate the trade-offs between using sequences and native array methods.
📚 Use Cases
Reversing Array Order:
The primary use case of
_.prototype.reverse()
is to reverse the order of elements in an array. This can be helpful when presenting data in a different orientation or when processing arrays in a specific order.example.jsCopiedconst data = [10, 20, 30, 40, 50]; const reversedData = _(data).reverse().value(); console.log(reversedData); // Output: [50, 40, 30, 20, 10]
Chained Array Transformations:
_.prototype.reverse()
can be seamlessly integrated into chained sequences for complex array transformations. This allows for efficient and expressive data manipulation workflows.example.jsCopiedconst numbers = [1, 2, 3, 4, 5]; const transformedData = _(numbers) .map(value => value * 2) .filter(value => value > 5) .reverse() .value(); console.log(transformedData); // Output: [10, 8, 6]
🎉 Conclusion
The _.prototype.reverse()
Seq method in Lodash provides a convenient way to reverse the order of elements in an array within a chainable sequence. By leveraging this method alongside other Lodash functions, developers can streamline array transformations and enhance code readability. Whether you're reversing array order or constructing complex data pipelines, _.prototype.reverse()
offers a versatile solution for array manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.prototype.reverse()
method in your Lodash projects.
👨💻 Join our Community:
Author
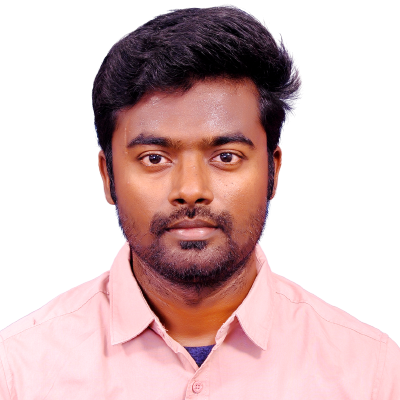
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
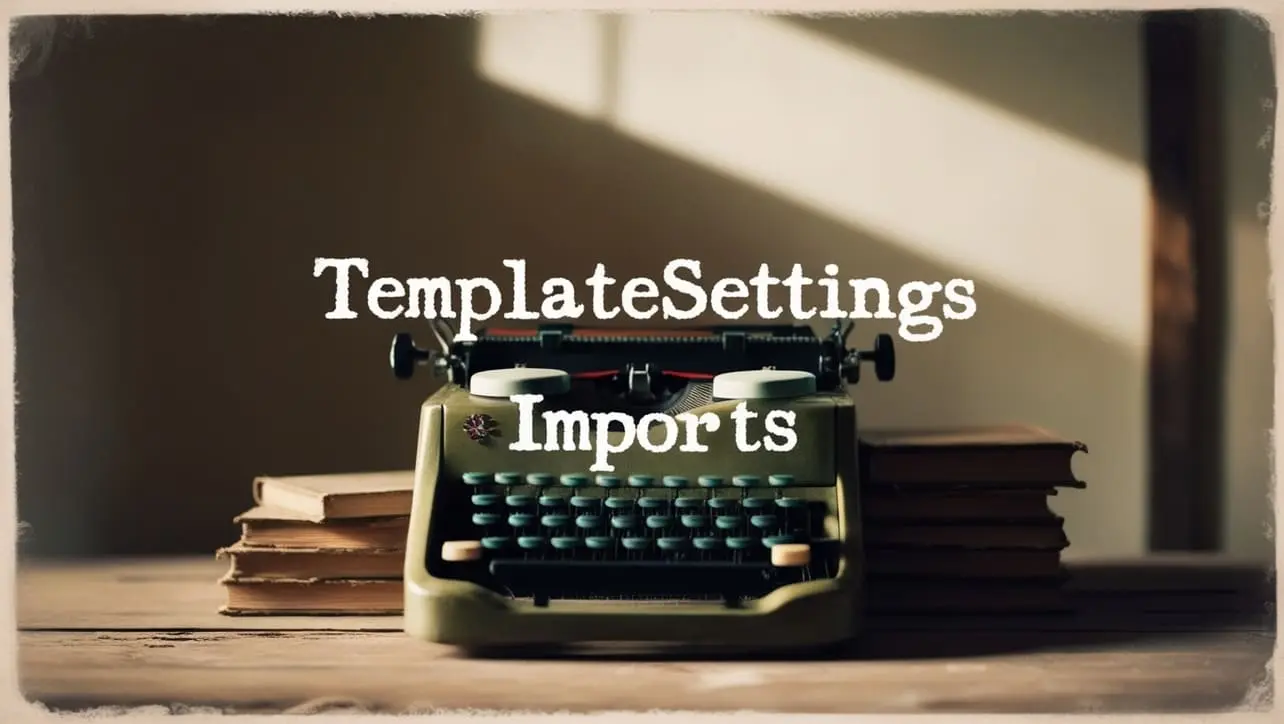
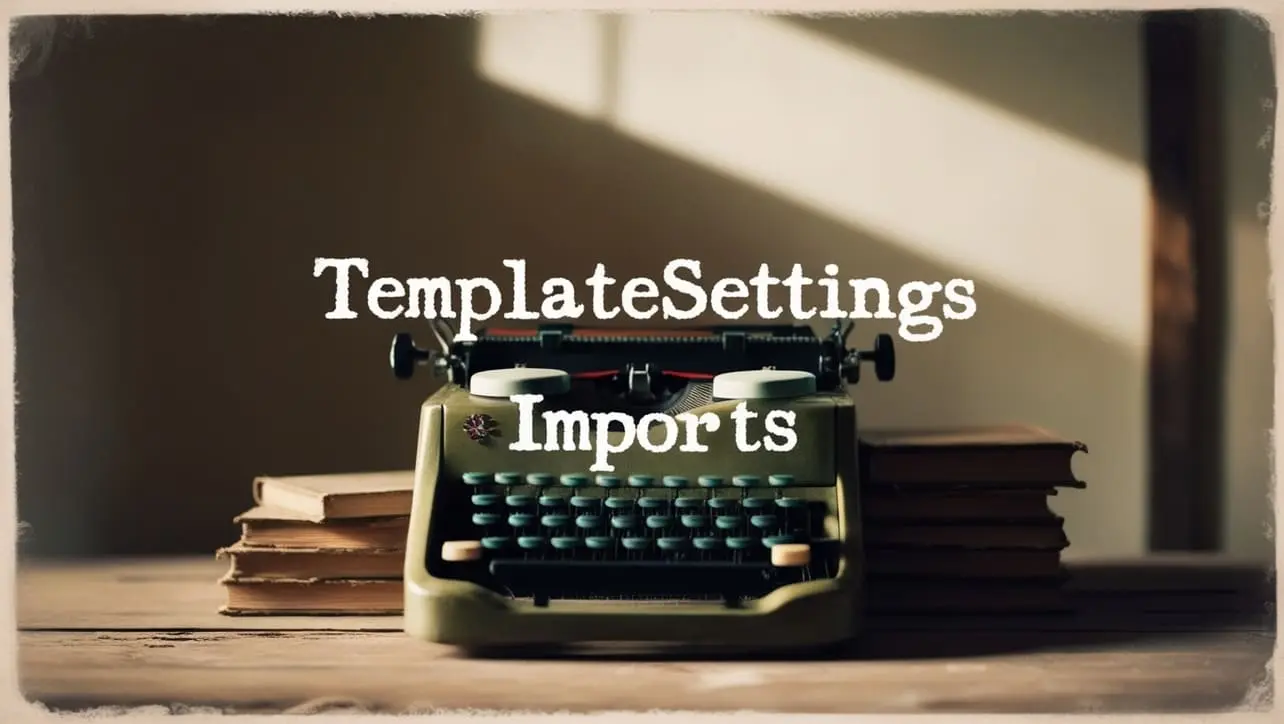
Lodash _.templateSettings.imports Property
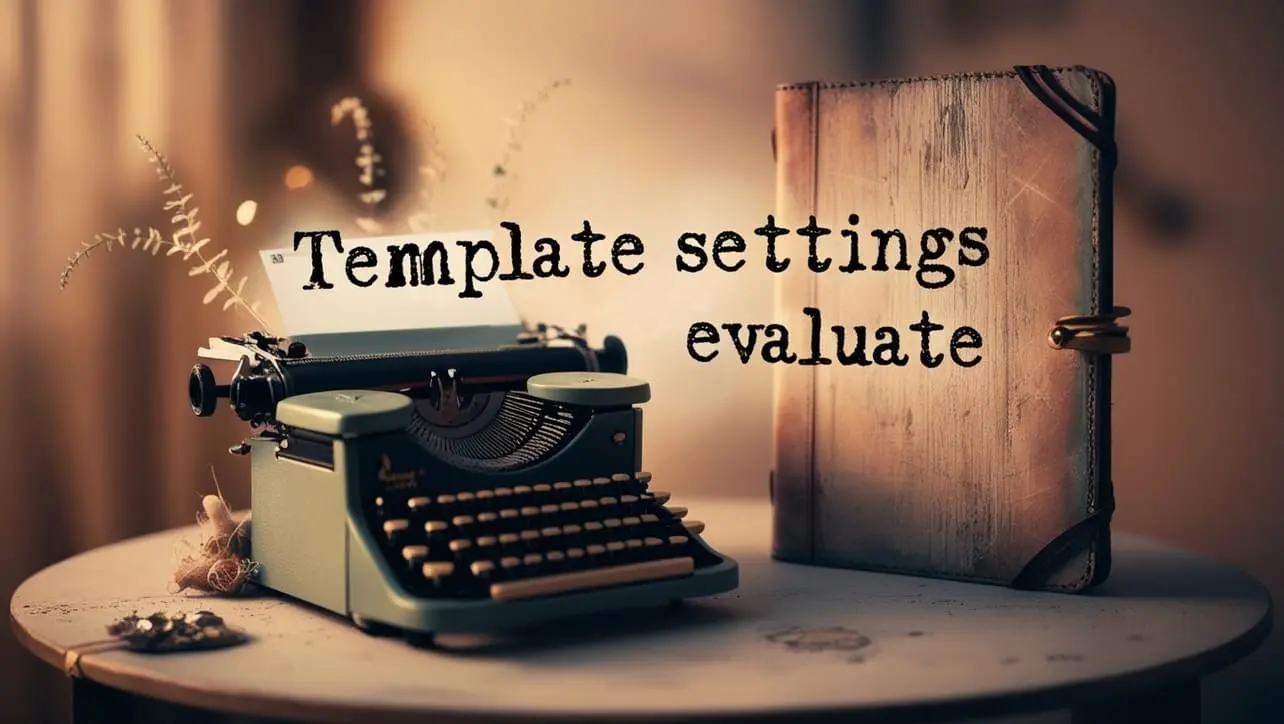
Lodash _.templateSettings.evaluate Property
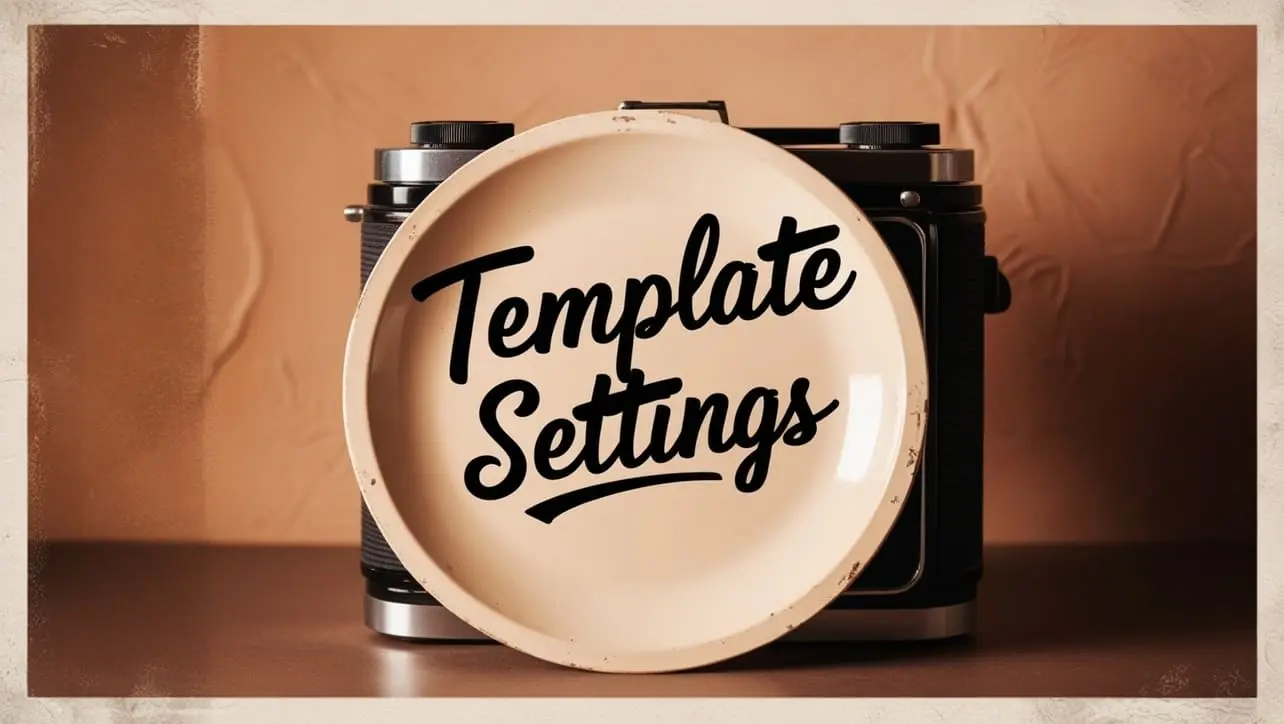
Lodash _.templateSettings Property
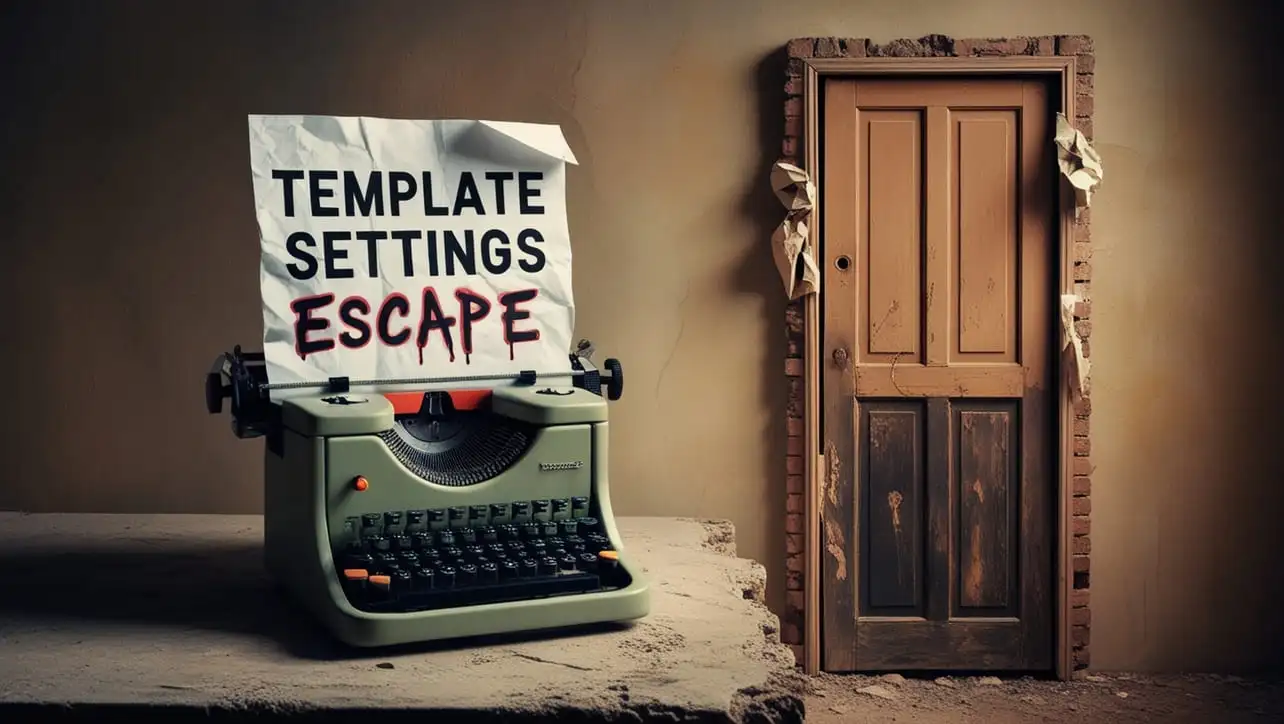
Lodash _.templateSettings.escape Property
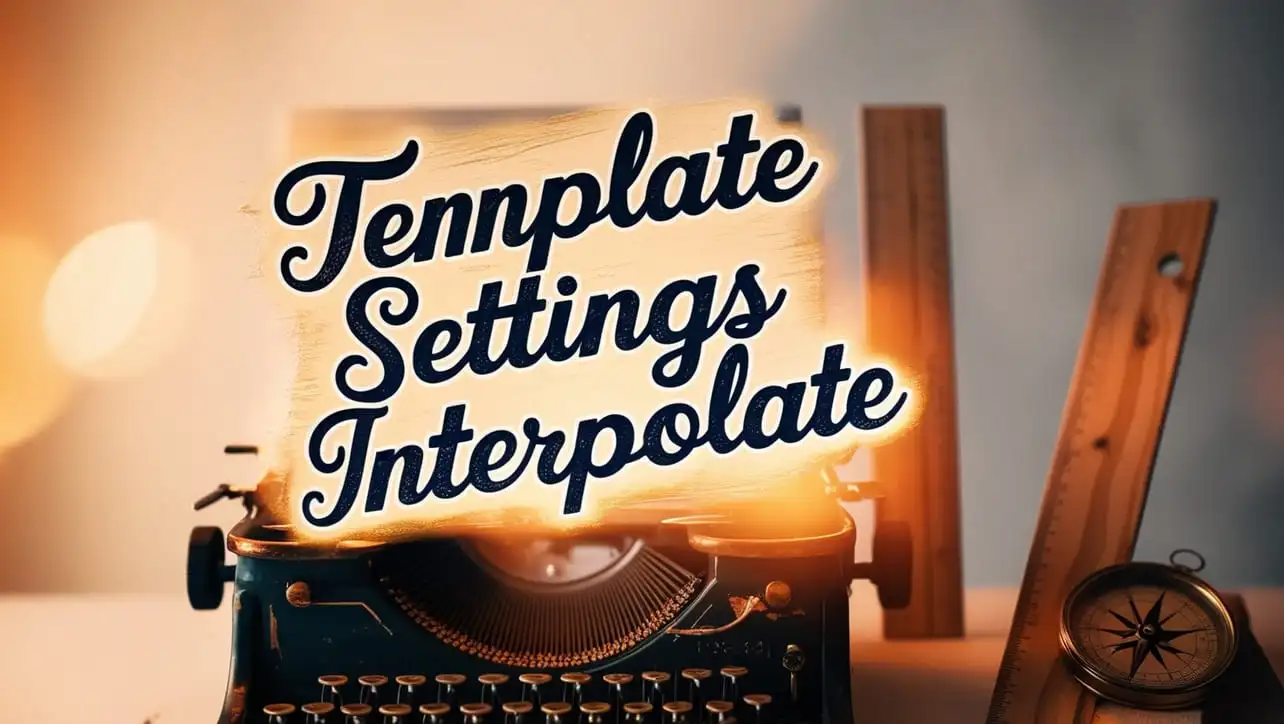
Lodash _.templateSettings.interpolate Property
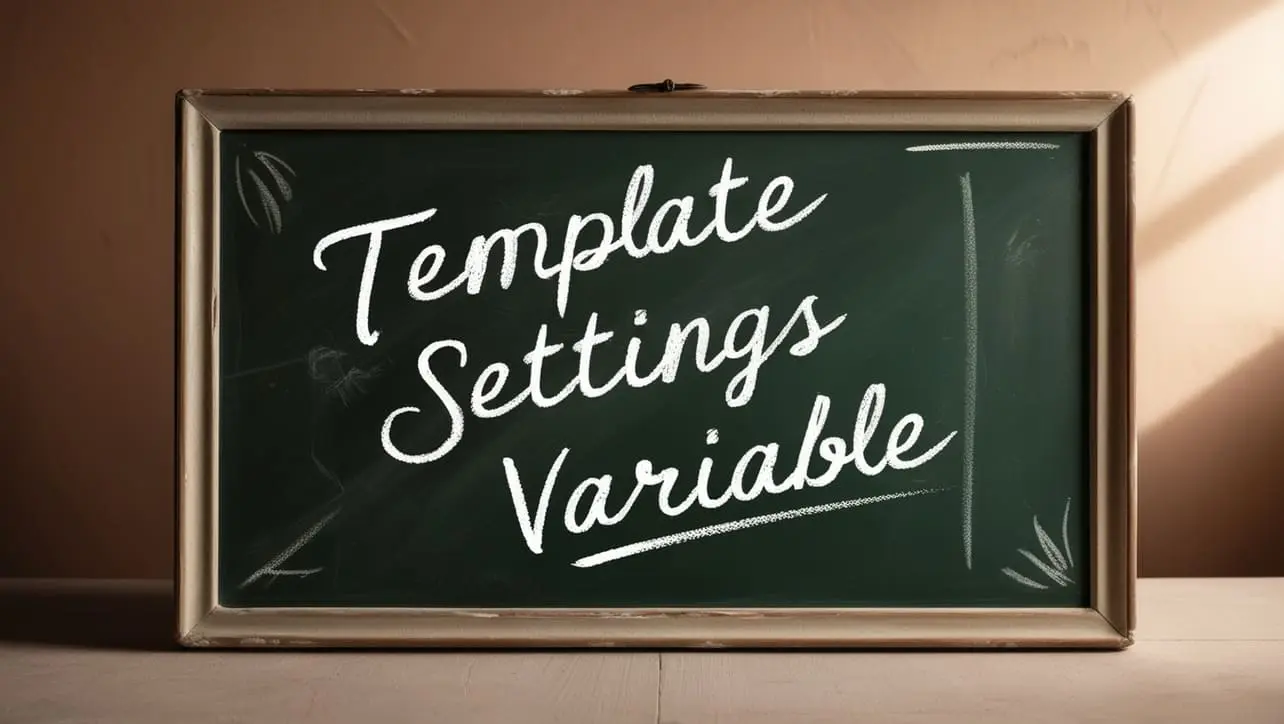
If you have any doubts regarding this article (Lodash _.prototype.reverse() Seq Method), please comment here. I will help you immediately.