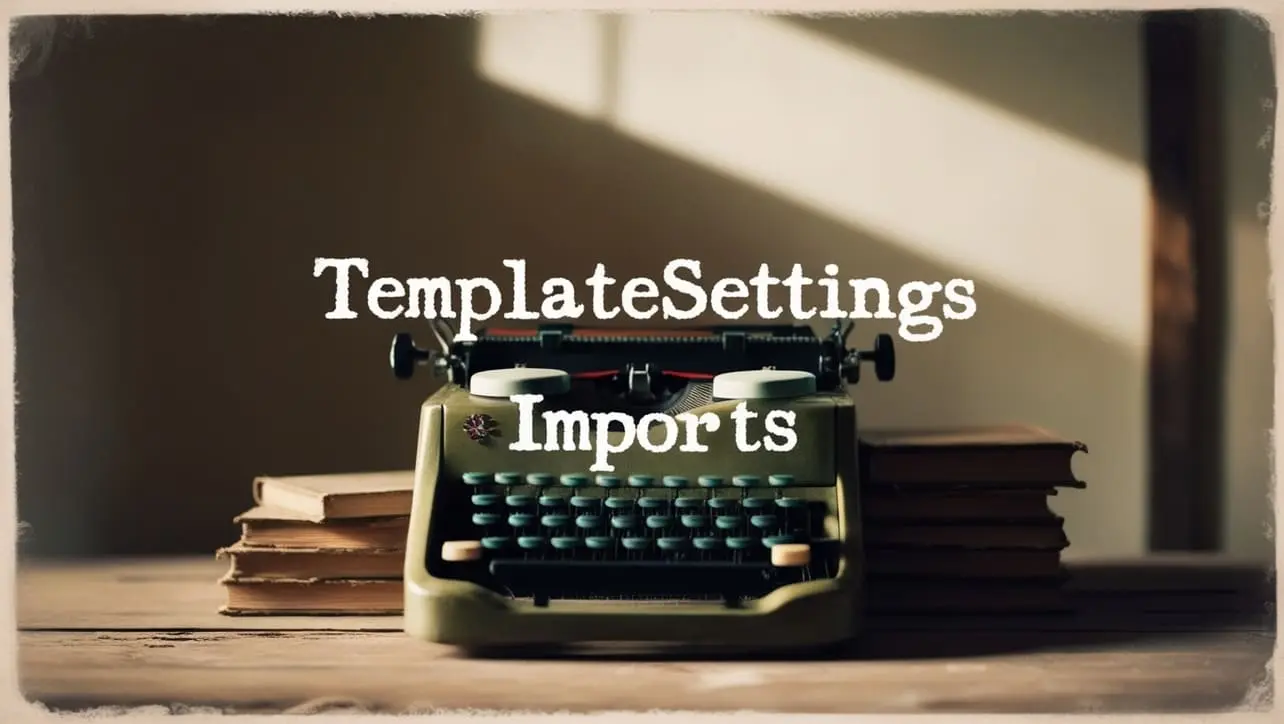
Lodash _.prototype.at() Seq Method
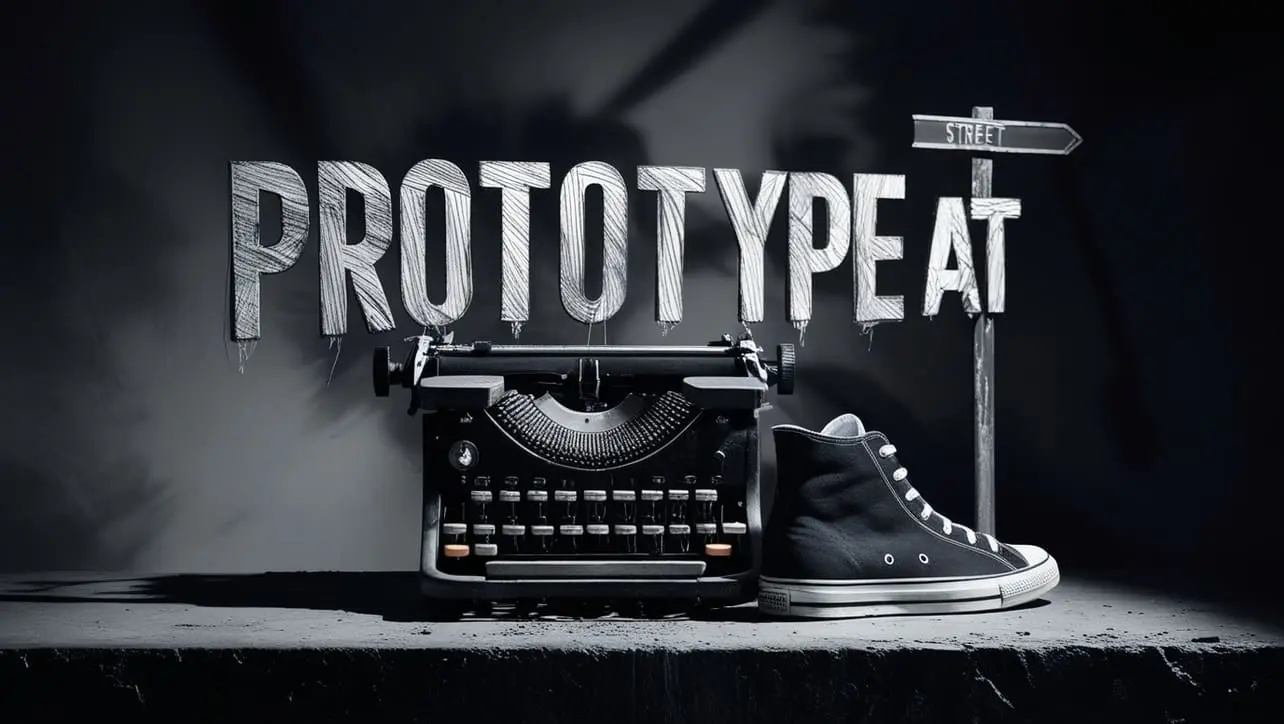
Photo Credit to CodeToFun
🙋 Introduction
Lodash is renowned for its array and object manipulation utilities, but it also offers powerful sequence methods for chaining and transforming data. Among these methods is _.prototype.at()
, a versatile tool for extracting values from nested objects or arrays based on specified paths.
This method simplifies data retrieval and enhances code readability, making it invaluable for developers dealing with complex data structures.
🧠 Understanding _.prototype.at() Method
The _.prototype.at()
method in Lodash is designed to extract values from nested objects or arrays based on specified paths. By providing an array of paths, developers can efficiently retrieve multiple values from a single source, streamlining data access and manipulation.
💡 Syntax
The syntax for the _.prototype.at()
method is straightforward:
_(value).at(paths)
- value: The source object or array.
- paths: An array of paths to extract values from.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.prototype.at()
method:
const _ = require('lodash');
const object = { 'a': [{ 'b': { 'c': 3 } }, 4] };
const extractedValues = _(object).at(['a[0].b.c', 'a[1]']).value();
console.log(extractedValues);
// Output: [3, 4]
In this example, _.prototype.at()
is used to extract values from the nested object object based on the provided paths.
🏆 Best Practices
When working with the _.prototype.at()
method, consider the following best practices:
Understand Path Syntax:
Familiarize yourself with the path syntax supported by
_.prototype.at()
. This includes dot notation for object properties and array bracket notation for array elements.example.jsCopiedconst nestedObject = { 'a': { 'b': { 'c': 123 } } }; const value = _(nestedObject).at('a.b.c').value(); console.log(value); // Output: [123]
Handle Missing Paths:
Account for missing paths by providing default values or implementing error handling mechanisms. This ensures robustness in data extraction operations.
example.jsCopiedconst nestedObject = { 'a': { 'b': { 'c': 123 } } }; const defaultValue = 'N/A'; const value = _(nestedObject).at('x.y.z').default(defaultValue).value(); console.log(value); // Output: ['N/A']
Streamline Data Access:
Leverage
_.prototype.at()
to streamline data access in your applications, especially when dealing with deeply nested data structures or complex object graphs.example.jsCopiedconst data = /* ...fetch data from API or elsewhere... */; const requiredFields = ['name', 'email', 'address.city', 'address.zipCode']; const extractedData = _(data).at(requiredFields).value(); console.log(extractedData);
📚 Use Cases
Extracting Data from API Responses:
_.prototype.at()
is particularly useful for extracting specific fields from API responses, allowing developers to focus on relevant data without unnecessary parsing.example.jsCopiedconst apiResponse = /* ...fetch data from API... */; const requiredFields = ['data.name', 'data.email', 'data.address.city']; const extractedData = _(apiResponse).at(requiredFields).value(); console.log(extractedData);
Form Data Population:
When populating form fields with data from a backend source,
_.prototype.at()
can efficiently extract the required values, simplifying the data binding process.example.jsCopiedconst formData = /* ...fetch form data from backend... */; const formFields = ['name', 'email', 'address.city']; const extractedFormData = _(formData).at(formFields).value(); console.log(extractedFormData);
Configuration Parsing:
In configuration parsing tasks,
_.prototype.at()
facilitates the extraction of specific parameters or settings, enabling seamless integration with application logic.example.jsCopiedconst configuration = /* ...load configuration settings... */; const requiredSettings = ['server.host', 'server.port', 'database.name']; const extractedSettings = _(configuration).at(requiredSettings).value(); console.log(extractedSettings);
🎉 Conclusion
The _.prototype.at()
method in Lodash offers a convenient and efficient solution for extracting values from nested objects or arrays based on specified paths. Whether you're fetching data from API responses, populating form fields, or parsing configuration settings, this method provides a versatile tool for data extraction and manipulation in JavaScript applications.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.prototype.at()
method in your Lodash projects.
👨💻 Join our Community:
Author
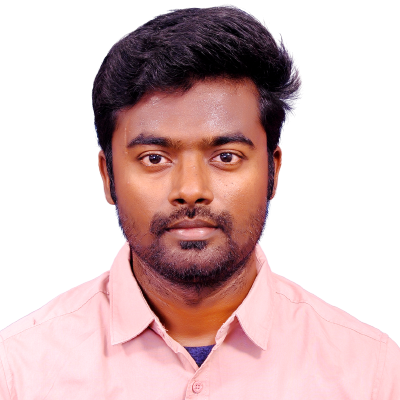
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
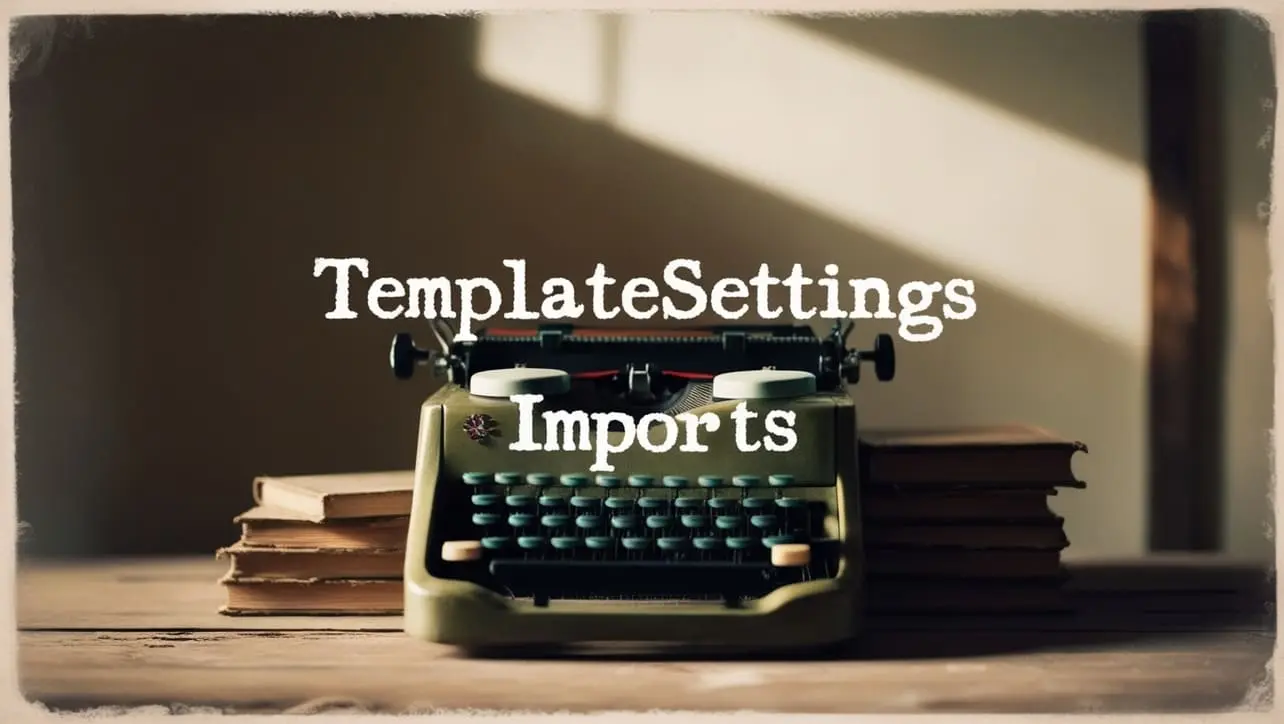
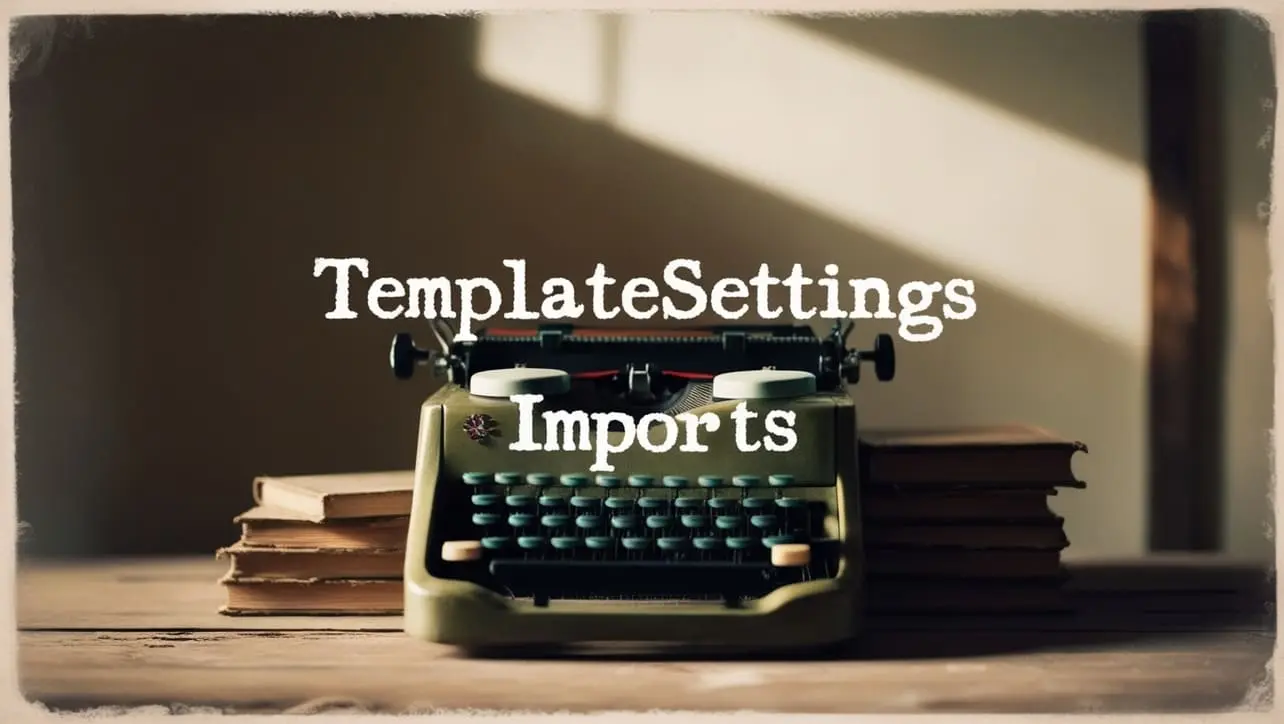
Lodash _.templateSettings.imports Property
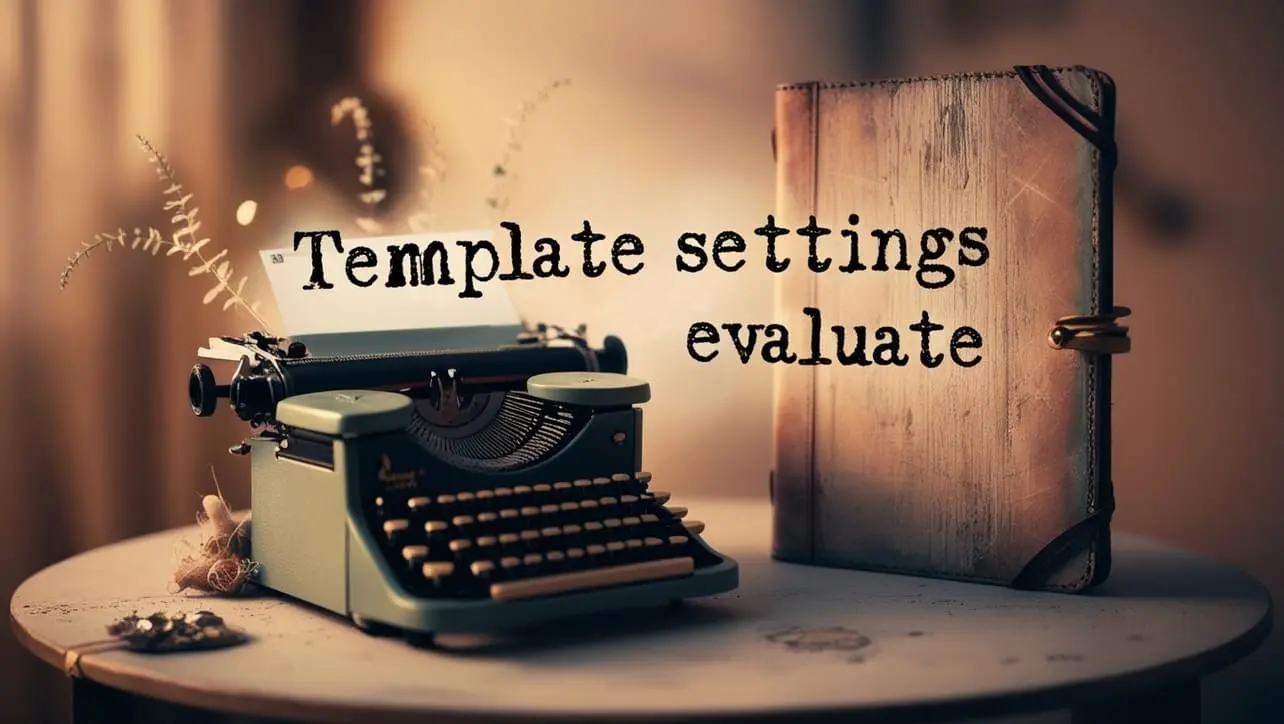
Lodash _.templateSettings.evaluate Property
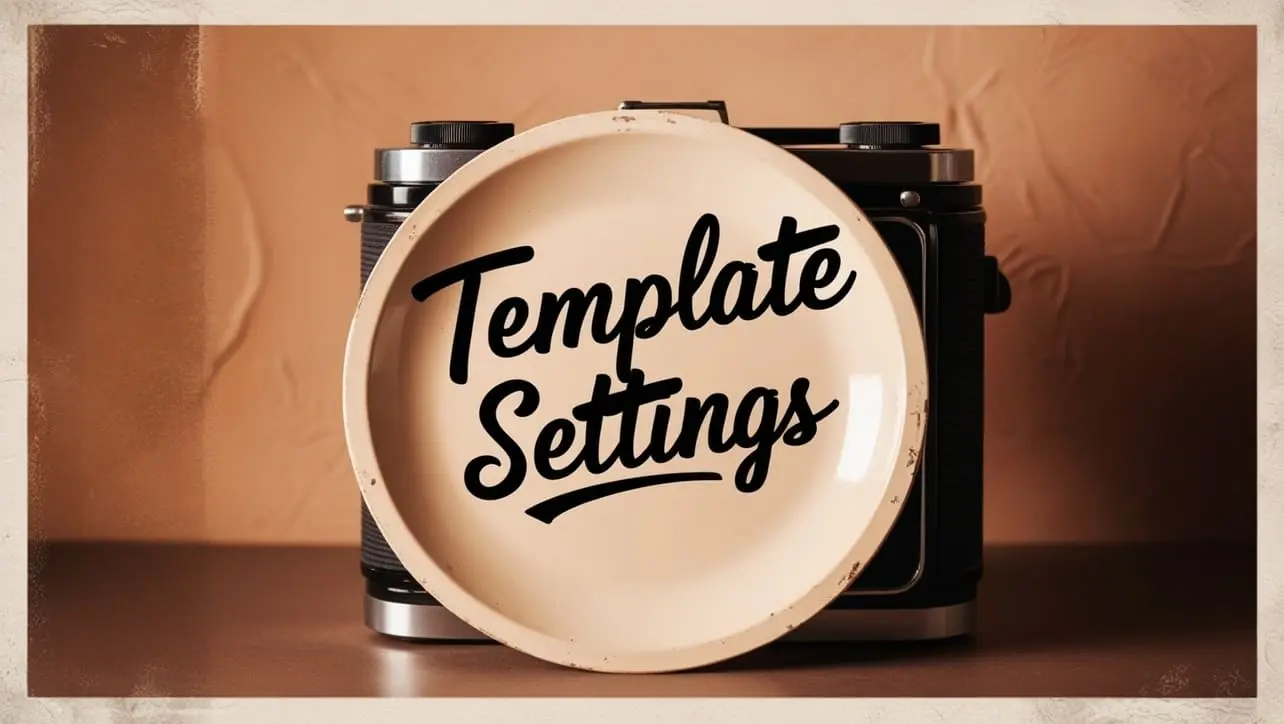
Lodash _.templateSettings Property
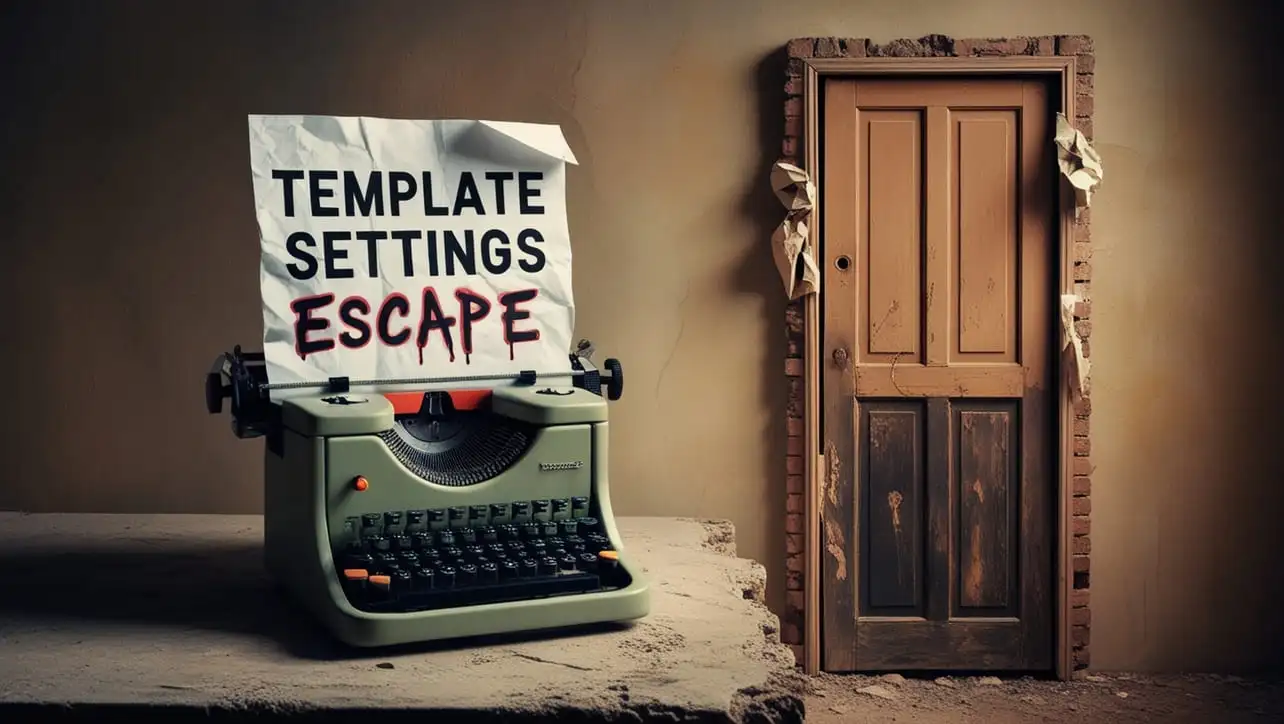
Lodash _.templateSettings.escape Property
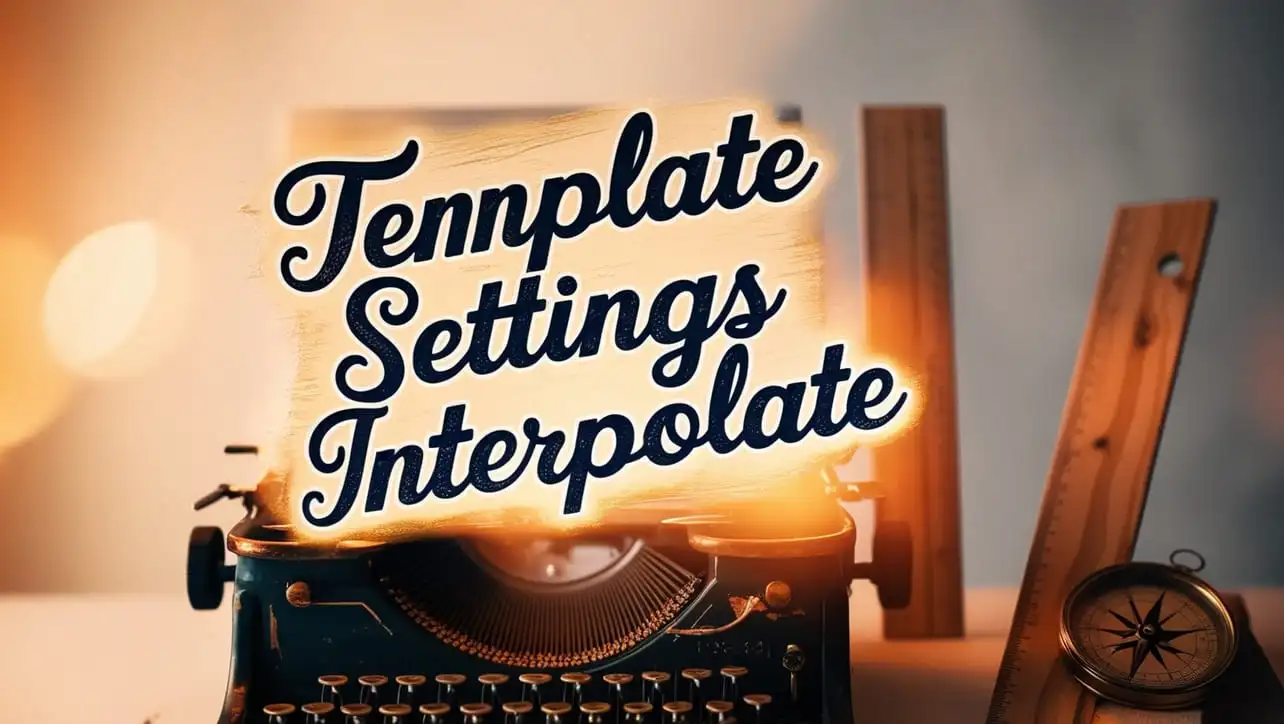
Lodash _.templateSettings.interpolate Property
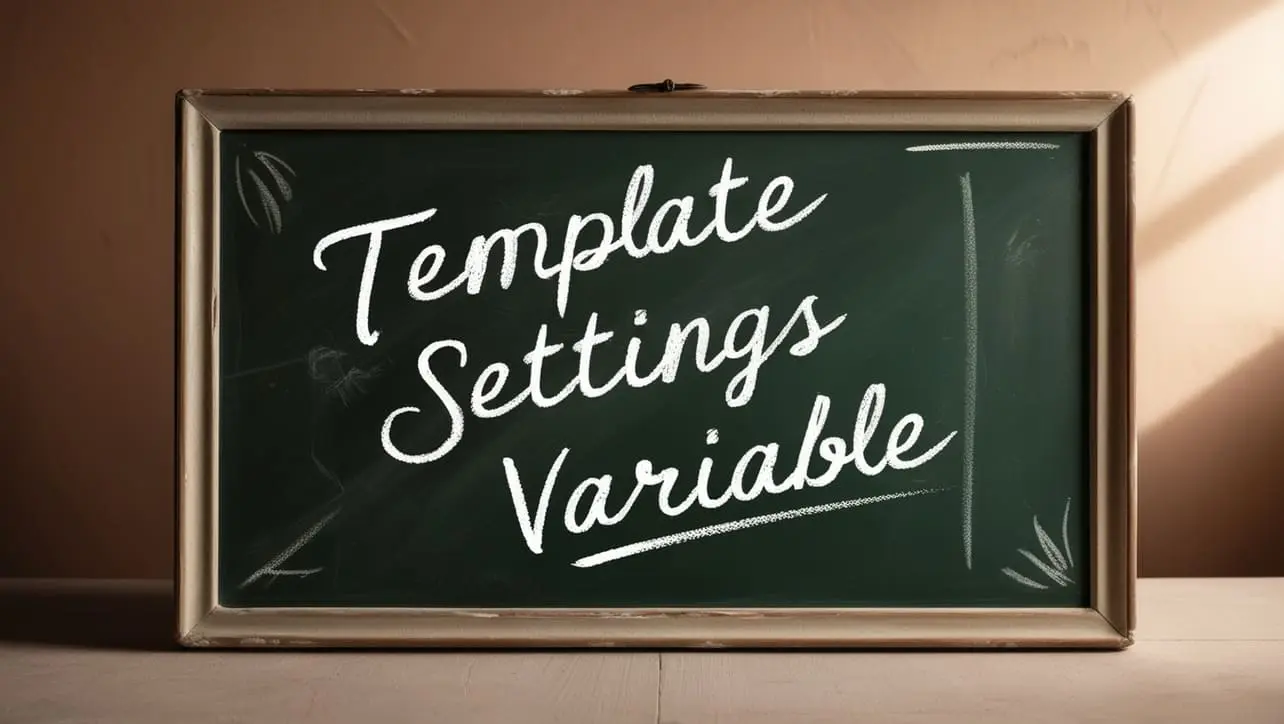
If you have any doubts regarding this article (Lodash _.prototype.at() Seq Method), please comment here. I will help you immediately.