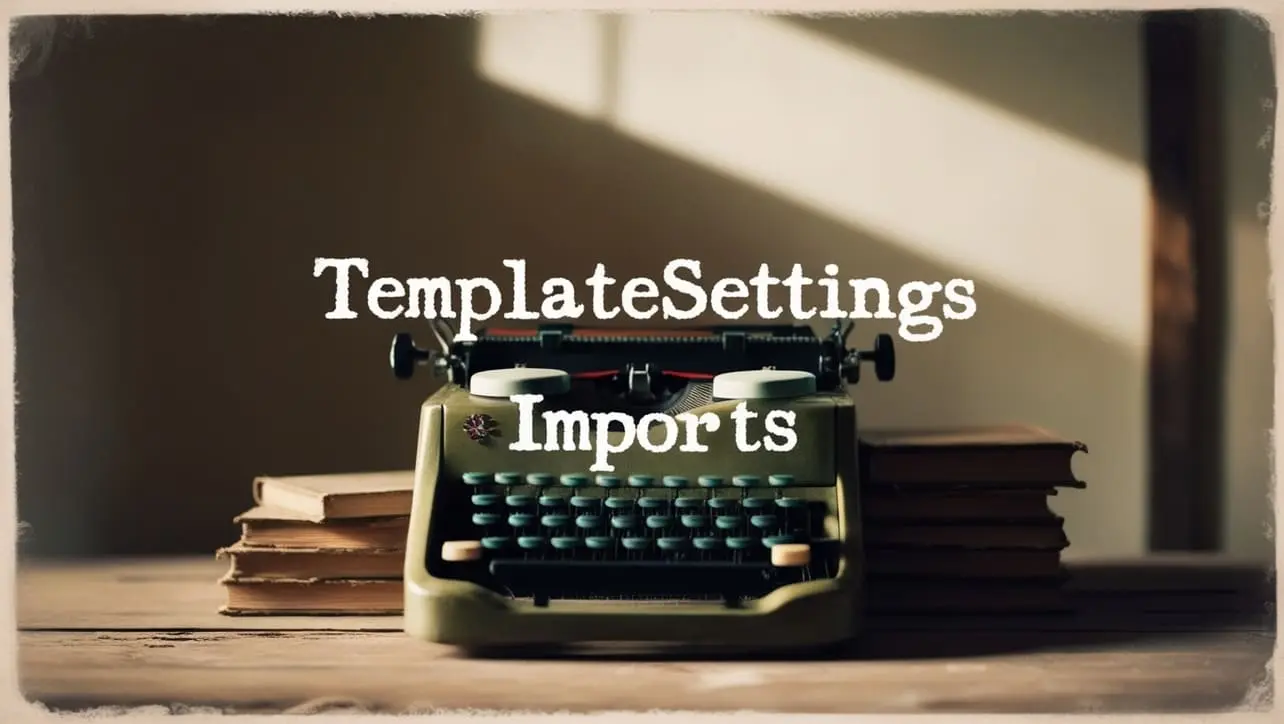
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.update() Object Method
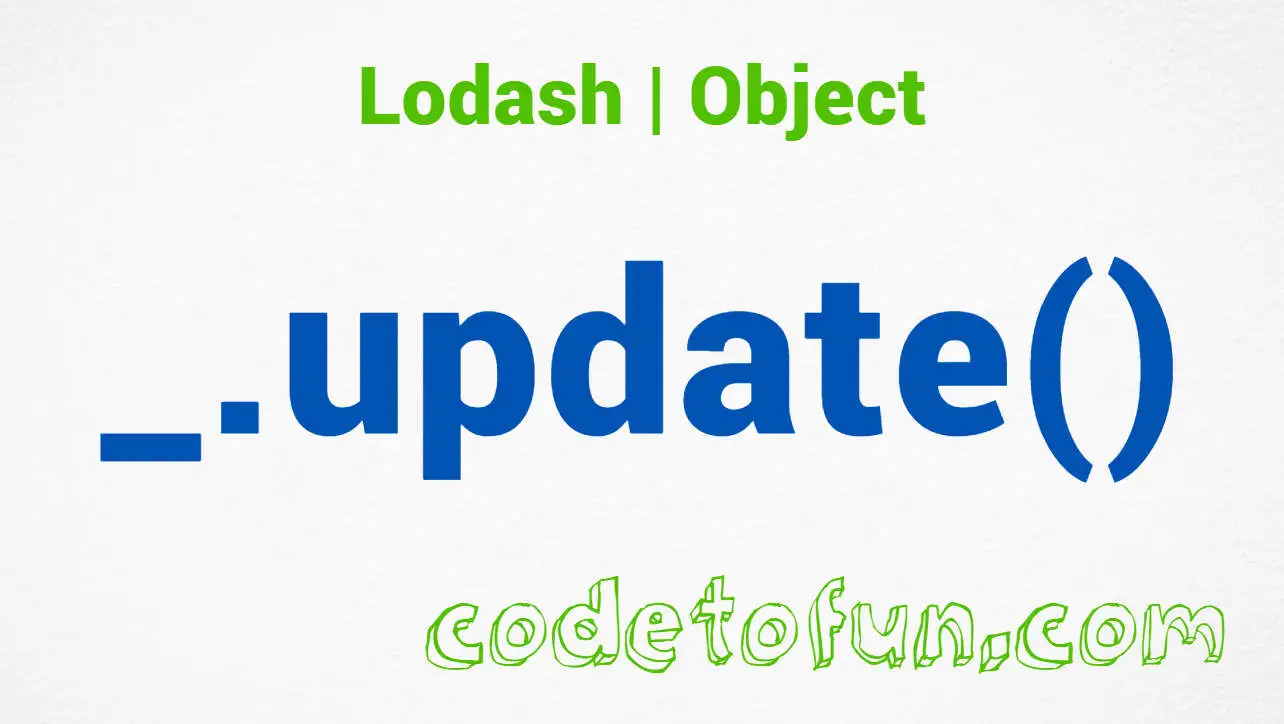
Photo Credit to CodeToFun
🙋 Introduction
Manipulating objects in JavaScript often requires a flexible and efficient approach. Lodash, a popular utility library, provides a multitude of functions to streamline object manipulation tasks. Among these functions is the _.update()
method, which offers a convenient way to update nested properties within an object.
This method enhances code readability and simplifies complex object transformations, making it invaluable for JavaScript developers.
🧠 Understanding _.update() Method
The _.update()
method in Lodash facilitates the modification of nested properties within an object. It allows you to specify a path to the property to be updated and provides various update functions to perform the desired transformation. Whether you need to set a new value, apply a function, or even remove a property, _.update()
offers a versatile solution for object manipulation.
💡 Syntax
The syntax for the _.update()
method is straightforward:
_.update(object, path, updater)
- object: The object to update.
- path: The path to the property to update.
- updater: The function used to modify the property value.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.update()
method:
const _ = require('lodash');
let user = {
name: 'John',
address: {
city: 'New York',
country: 'USA'
}
};
_.update(user, 'address.city', city => city.toUpperCase());
console.log(user);
// Output: { name: 'John', address: { city: 'NEW YORK', country: 'USA' } }
In this example, the address.city property of the user object is updated to its uppercase version using the _.update()
method.
🏆 Best Practices
When working with the _.update()
method, consider the following best practices:
Validate Input Object:
Before applying
_.update()
, ensure that the input object is valid and contains the expected structure. This helps prevent runtime errors and ensures the smooth execution of the update operation.example.jsCopiedif(!_.isPlainObject(user)) { console.error('Invalid input object'); return; } _.update(user, 'address.city', city => city.toUpperCase()); console.log(user);
Handle Nonexistent Paths:
Handle scenarios where the specified path does not exist within the object. Depending on your use case, you may choose to create missing properties, skip the update, or implement custom error handling logic.
example.jsCopied// Ensure the 'address' property exists before updating nested properties if(_.has(user, 'address')) { _.update(user, 'address.city', city => city.toUpperCase()); } else { console.error('Address property does not exist'); }
Utilize Custom Updaters:
Leverage custom updater functions to perform complex transformations or validations when updating object properties. This allows for greater flexibility and customization in your update operations.
example.jsCopied// Custom updater function to capitalize the first letter of the city const capitalizeFirstLetter = city => city.charAt(0).toUpperCase() + city.slice(1); _.update(user, 'address.city', capitalizeFirstLetter); console.log(user);
📚 Use Cases
Configurations and Settings:
_.update()
is useful for dynamically modifying configuration objects or application settings. It allows for easy adjustment of specific properties without affecting the overall structure of the configuration.example.jsCopiedlet config = { theme: 'light', fontSize: 14, options: { darkMode: false, animation: true } }; _.update(config, 'options.darkMode', mode => !mode); console.log(config);
State Management:
In state management scenarios,
_.update()
can be employed to update nested state objects in frameworks like React or Redux. It simplifies the process of immutably updating state properties, ensuring predictable state changes.example.jsCopiedlet state = { user: { name: 'Alice', isLoggedIn: true } }; _.update(state, 'user.isLoggedIn', isLoggedIn => !isLoggedIn); console.log(state);
Data Transformation:
When transforming data structures or performing data migrations,
_.update()
provides a convenient mechanism for updating nested properties based on specific criteria. This simplifies data manipulation tasks and improves code maintainability.example.jsCopiedlet data = { users: [ { id: 1, name: 'John', isAdmin: false }, { id: 2, name: 'Alice', isAdmin: true }, { id: 3, name: 'Bob', isAdmin: false }] }; _.update(data, 'users', users => users.filter(user => user.isAdmin)); console.log(data);
🎉 Conclusion
The _.update()
method in Lodash offers a versatile and efficient solution for updating nested properties within JavaScript objects. Whether you need to modify configuration settings, manage application state, or transform data structures, _.update()
simplifies the process and enhances code maintainability.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.update()
method in your Lodash projects.
👨💻 Join our Community:
Author
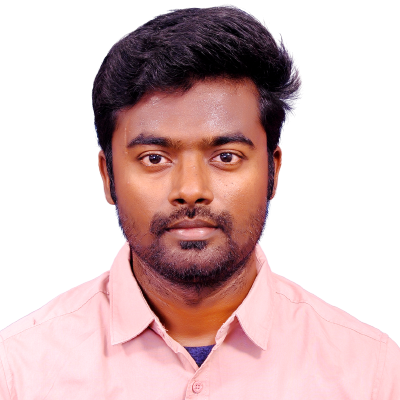
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
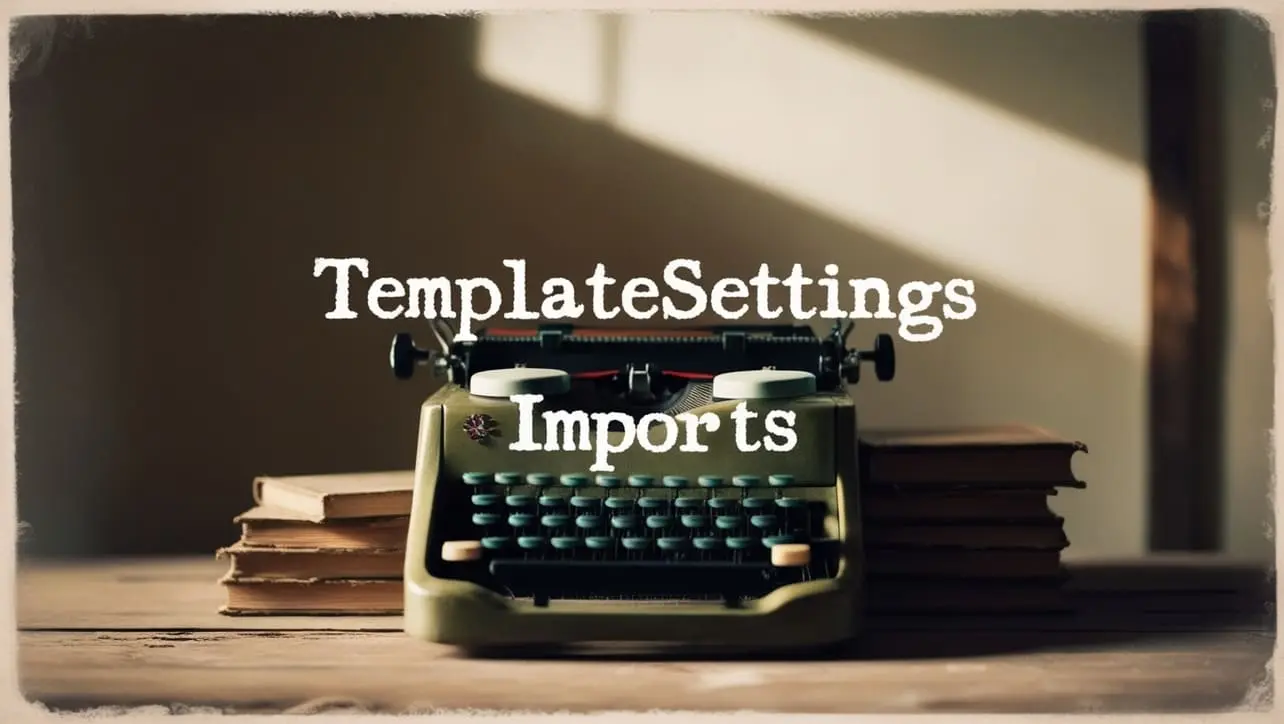
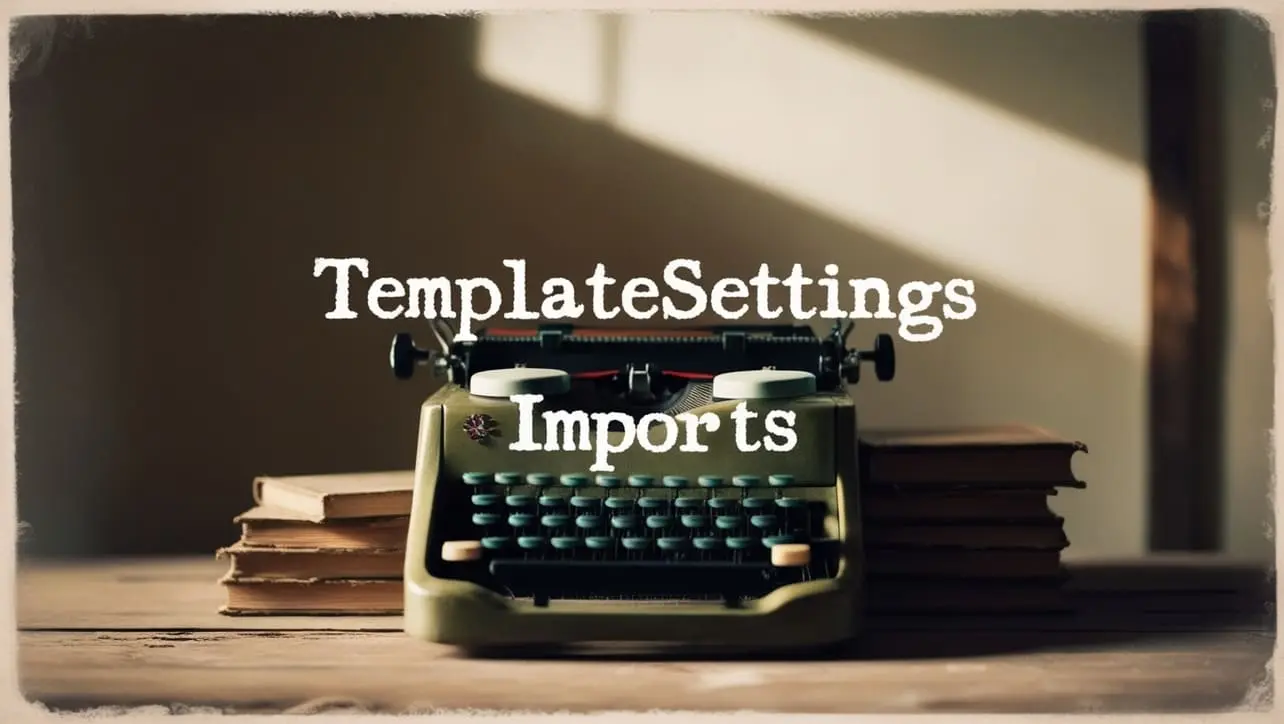
Lodash _.templateSettings.imports Property
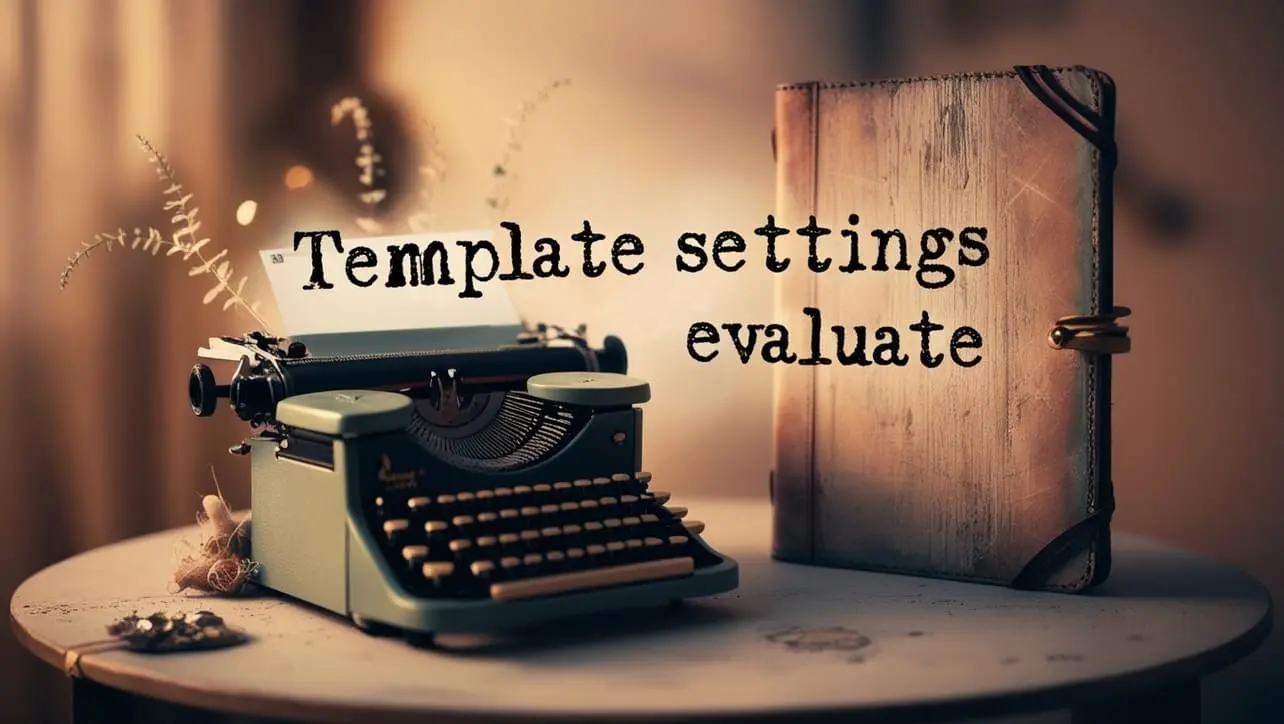
Lodash _.templateSettings.evaluate Property
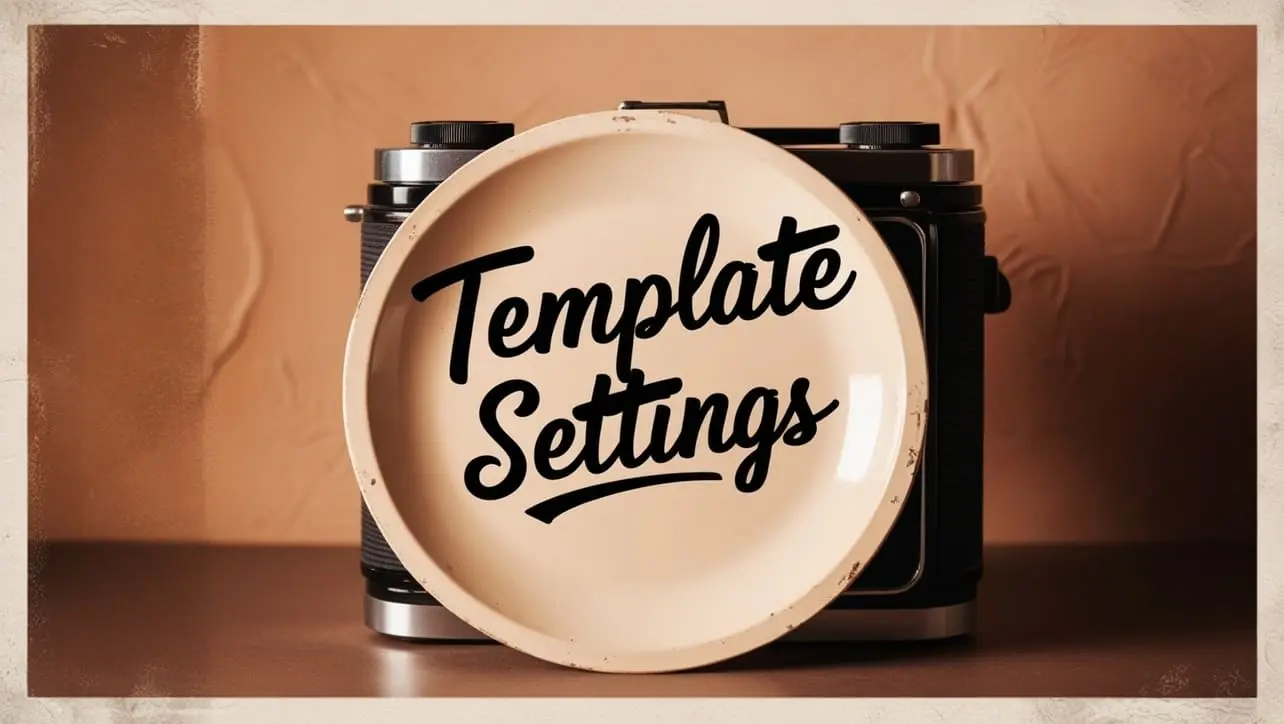
Lodash _.templateSettings Property
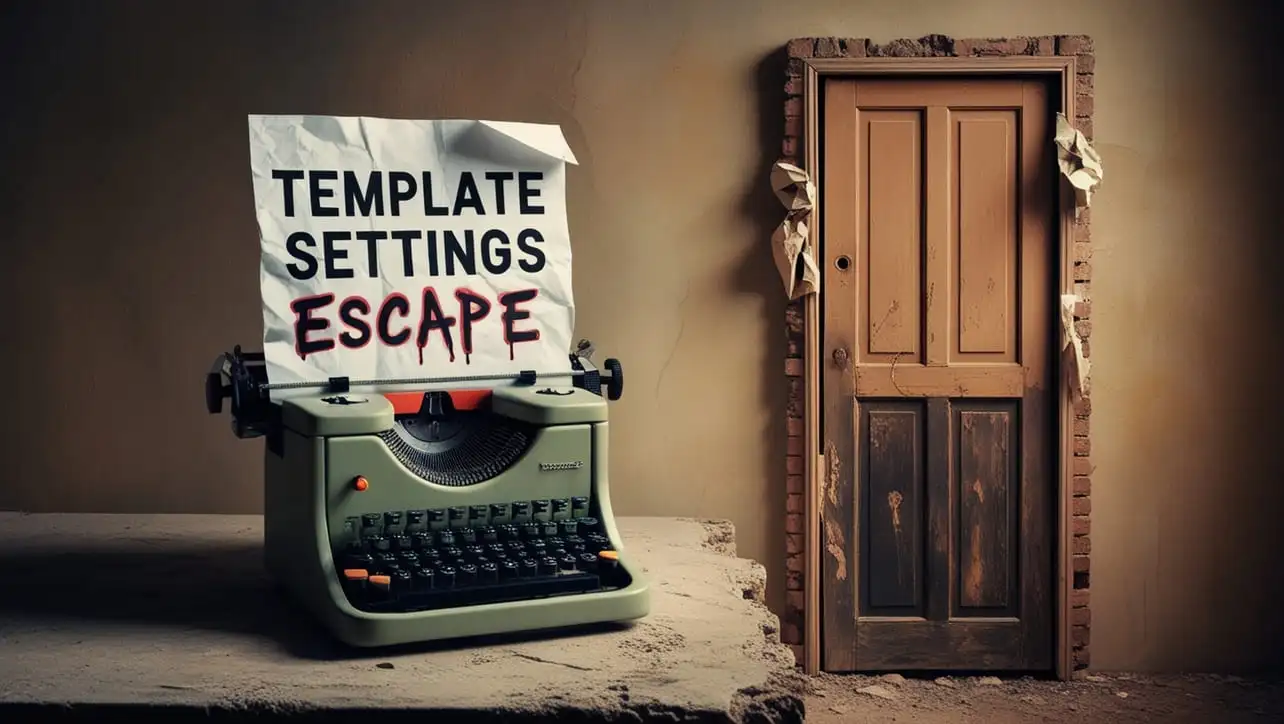
Lodash _.templateSettings.escape Property
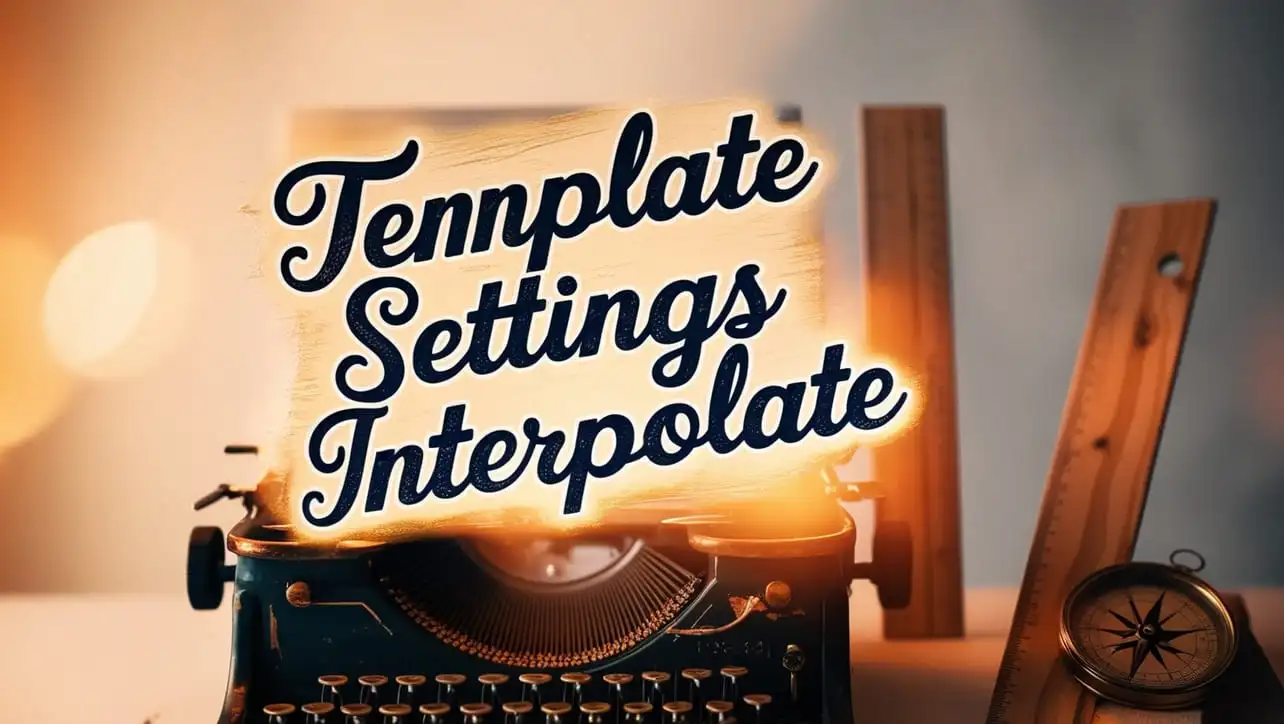
Lodash _.templateSettings.interpolate Property
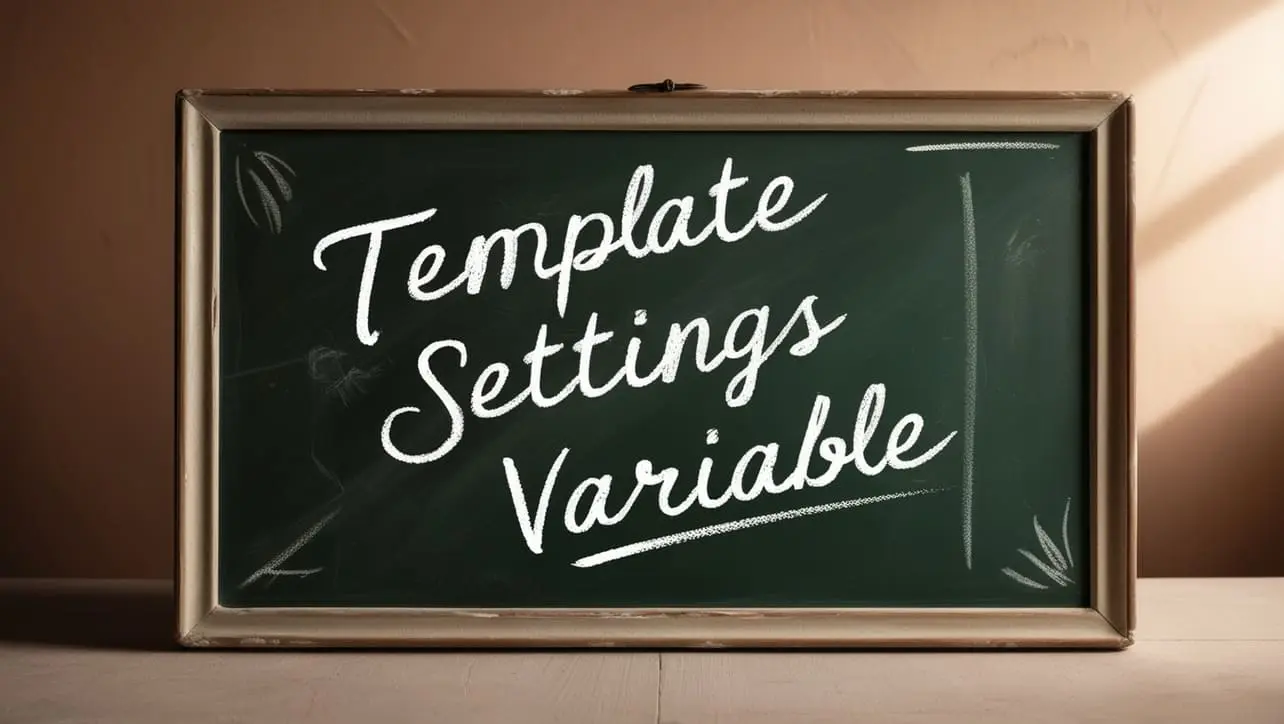
If you have any doubts regarding this article (Lodash _.update() Object Method), please comment here. I will help you immediately.