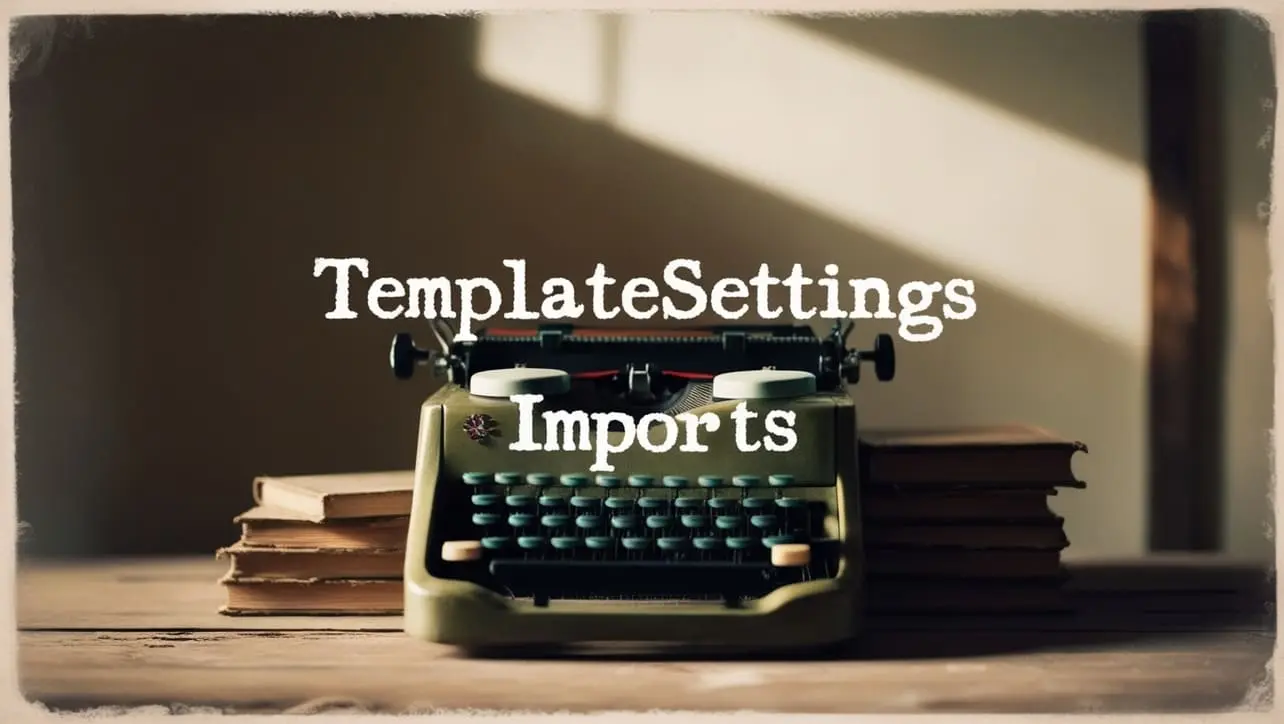
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.toPairsIn() Object Method
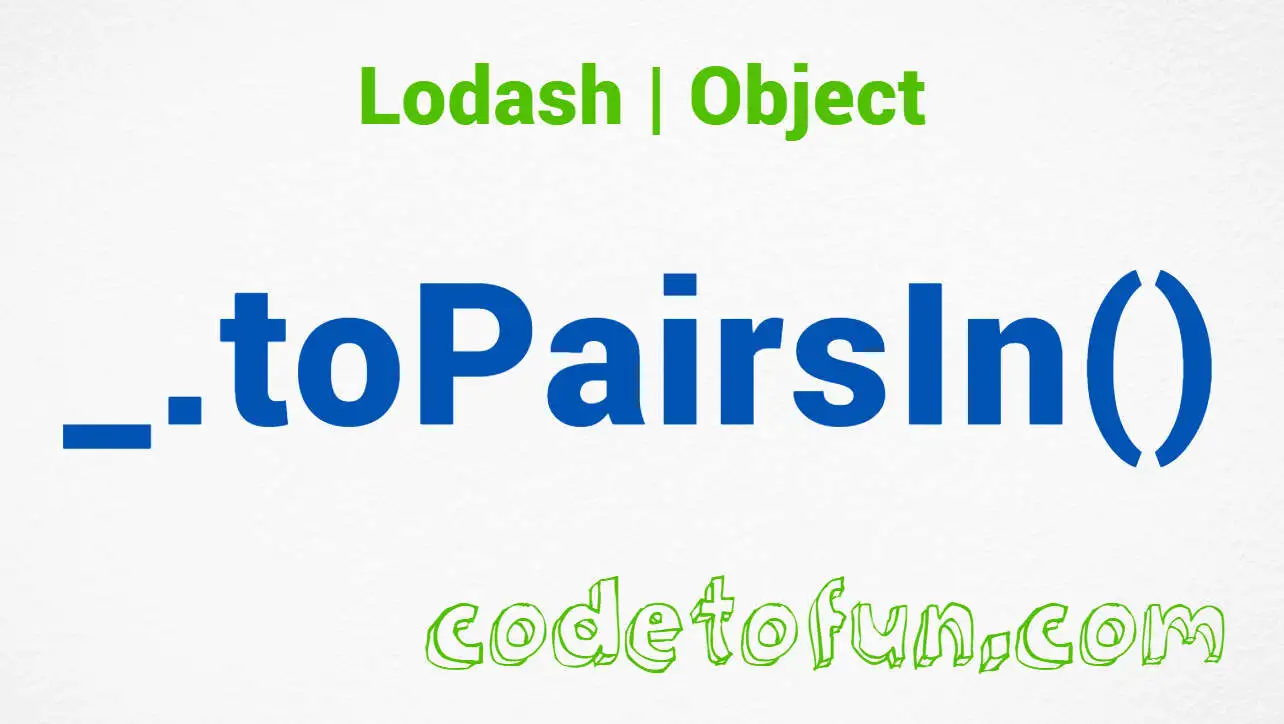
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, working with objects is fundamental. Lodash, a powerful utility library, provides developers with a plethora of functions to simplify object manipulation. Among these functions is the _.toPairsIn()
method, a versatile tool for converting objects into arrays of key-value pairs, including inherited properties.
This method enhances code flexibility and readability, making it indispensable for developers dealing with complex object structures.
🧠 Understanding _.toPairsIn() Method
The _.toPairsIn()
method in Lodash allows you to transform objects into arrays of key-value pairs, including both own and inherited properties. This provides a comprehensive view of the object's structure, facilitating various data manipulation tasks.
💡 Syntax
The syntax for the _.toPairsIn()
method is straightforward:
_.toPairsIn(object)
- object: The object to convert into key-value pairs.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.toPairsIn()
method:
const _ = require('lodash');
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(`${this.name} makes a sound.`);
};
const dog = new Animal('Dog');
const animalPairs = _.toPairsIn(dog);
console.log(animalPairs);
/*
Output:
[
['name', 'Dog'],
['speak', function() { console.log(`${this.name} makes a sound.`); }]
]
*/
In this example, the dog object, including its inherited property speak, is converted into an array of key-value pairs by _.toPairsIn()
.
🏆 Best Practices
When working with the _.toPairsIn()
method, consider the following best practices:
Consider Inherited Properties:
Be aware that
_.toPairsIn()
includes inherited properties when converting objects into key-value pairs. Ensure that this behavior aligns with your intended use case to avoid unexpected results.example.jsCopiedconst parentObject = { parentProp: 'parentValue' }; const childObject = Object.create(parentObject, { childProp: { value: 'childValue' } }); const childPairs = _.toPairsIn(childObject); console.log(childPairs); // Output: [['childProp', 'childValue'], ['parentProp', 'parentValue']]
Handle Circular References:
Exercise caution when dealing with objects containing circular references, as
_.toPairsIn()
may enter an infinite loop in such scenarios. Implement appropriate checks or handle circular references manually to prevent unintended behavior.example.jsCopiedconst circularObj = {}; circularObj.circularRef = circularObj; try { const circularPairs = _.toPairsIn(circularObj); console.log(circularPairs); } catch (error) { console.error(error.message); }
Use Case-Specific Iteration:
Consider whether
_.toPairsIn()
is the most suitable method for your use case. Depending on your requirements, other Lodash methods or native JavaScript techniques may offer more efficient or tailored solutions for object manipulation.example.jsCopiedconst objectToIterate = { a: 1, b: 2, c: 3 }; // Using _.toPairsIn() const pairs = _.toPairsIn(objectToIterate); console.log(pairs); // Equivalent native JavaScript approach const nativePairs = Object.entries(objectToIterate); console.log(nativePairs);
📚 Use Cases
Object Inspection:
_.toPairsIn()
is particularly useful for inspecting object properties, including inherited ones. This can aid in debugging and understanding complex object structures.example.jsCopiedconst complexObject = /* ...create or retrieve a complex object... */; const objectPairs = _.toPairsIn(complexObject); console.log(objectPairs);
Property Enumeration:
When you need to enumerate over an object's properties, including inherited ones,
_.toPairsIn()
provides a convenient way to iterate and manipulate key-value pairs.example.jsCopiedconst user = { name: 'John', age: 30, role: 'admin' }; _.toPairsIn(user).forEach(([key, value]) => { console.log(`${key}: ${value}`); });
Object Transformation:
In scenarios where you need to transform an object into a different format, such as preparing data for serialization or storage, _.toPairsIn() can be used to convert the object into an array of key-value pairs for further processing.
example.jsCopiedconst dataObject = /* ...retrieve data object from an external source... */; const transformedData = transformData(_.toPairsIn(dataObject)); console.log(transformedData);
🎉 Conclusion
The _.toPairsIn()
method in Lodash offers a versatile solution for converting objects into arrays of key-value pairs, including inherited properties. Whether you're inspecting object structures, iterating over properties, or transforming data, this method provides a flexible tool for object manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.toPairsIn()
method in your Lodash projects.
👨💻 Join our Community:
Author
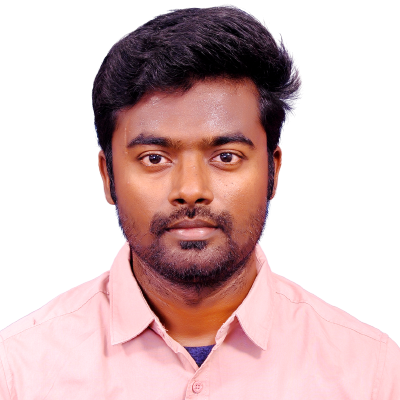
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
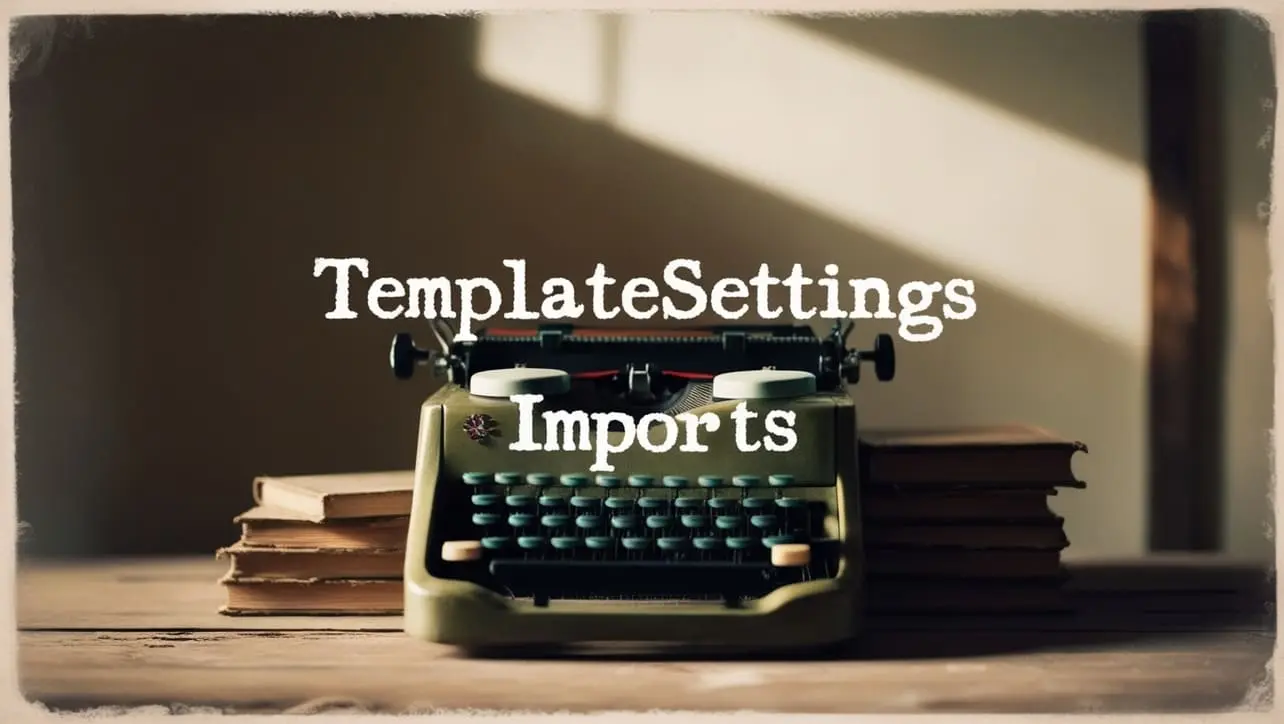
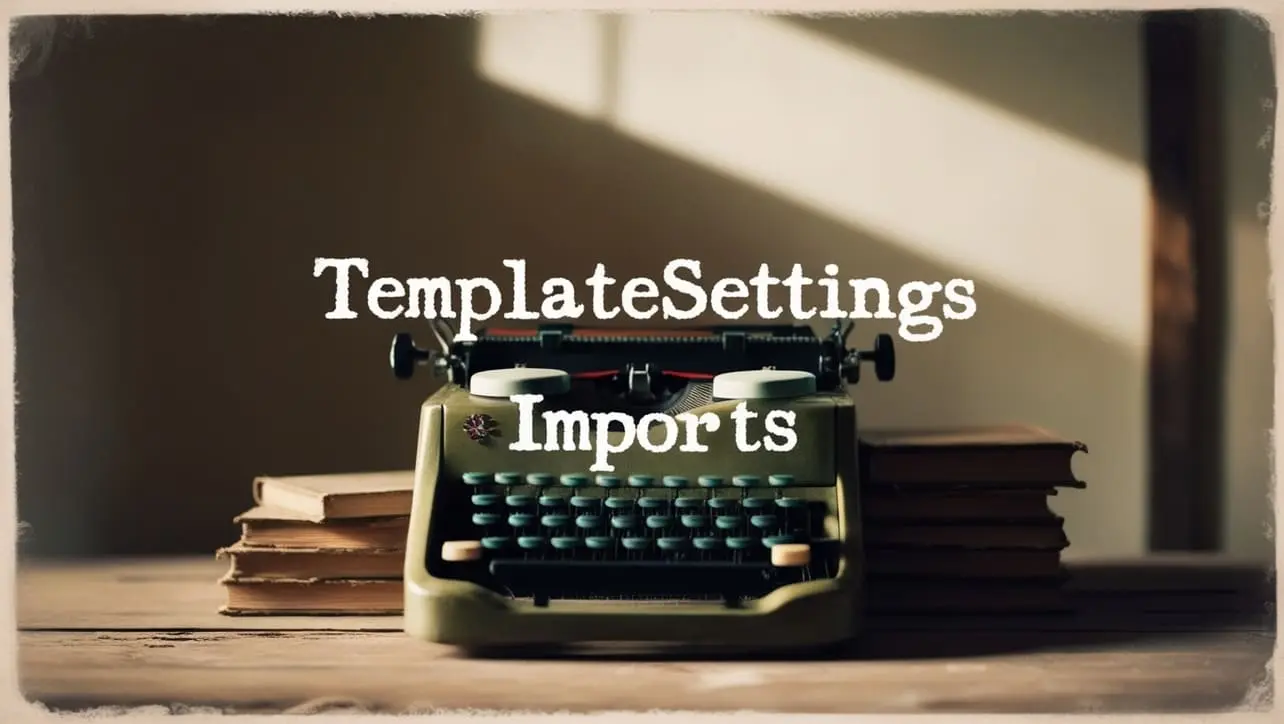
Lodash _.templateSettings.imports Property
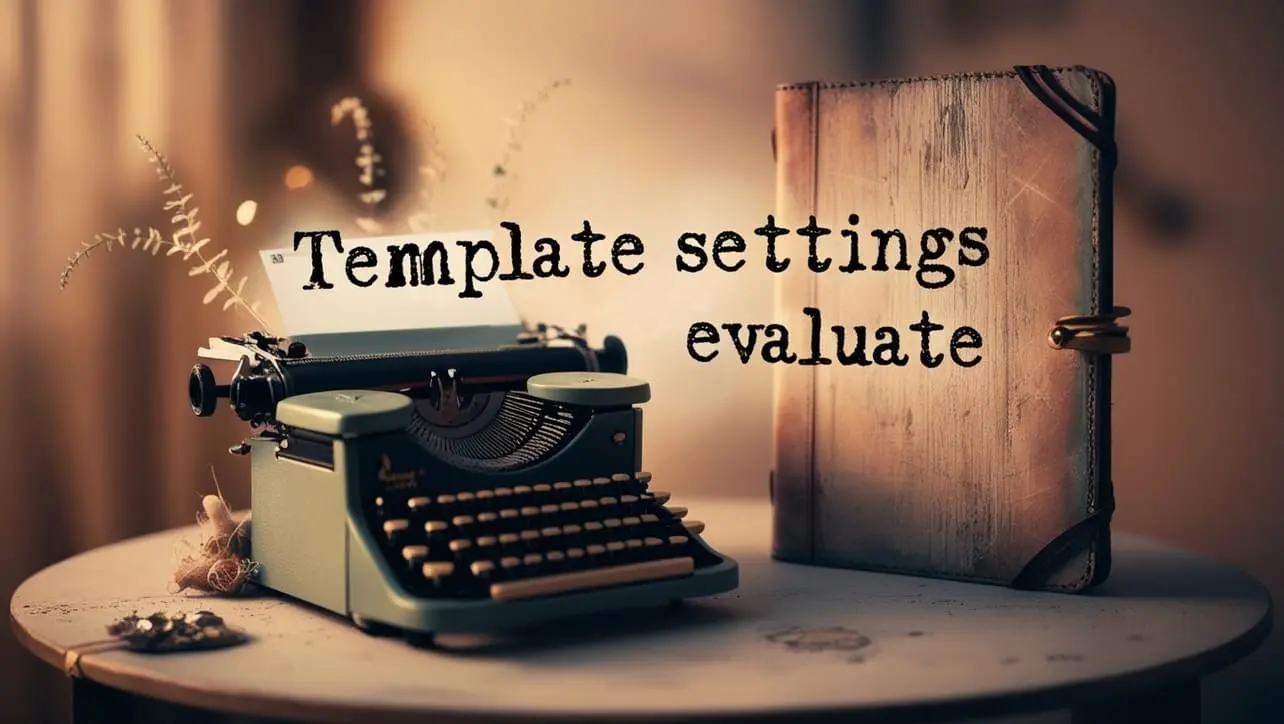
Lodash _.templateSettings.evaluate Property
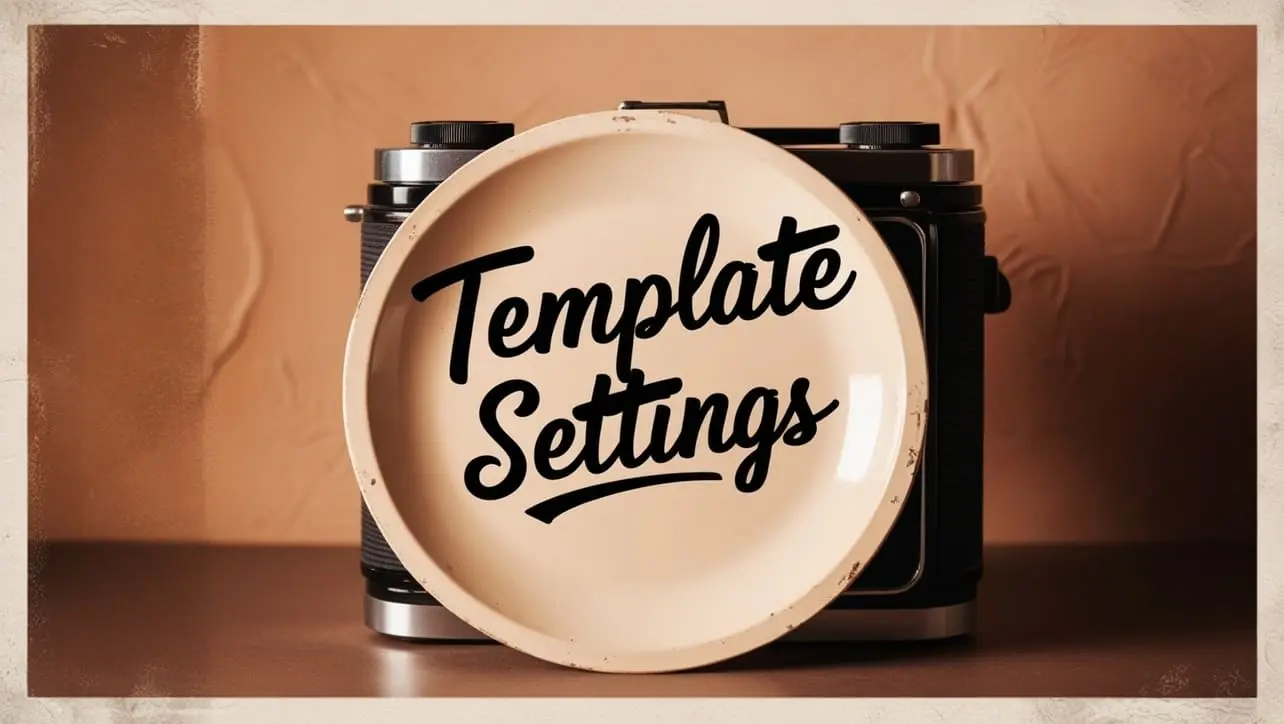
Lodash _.templateSettings Property
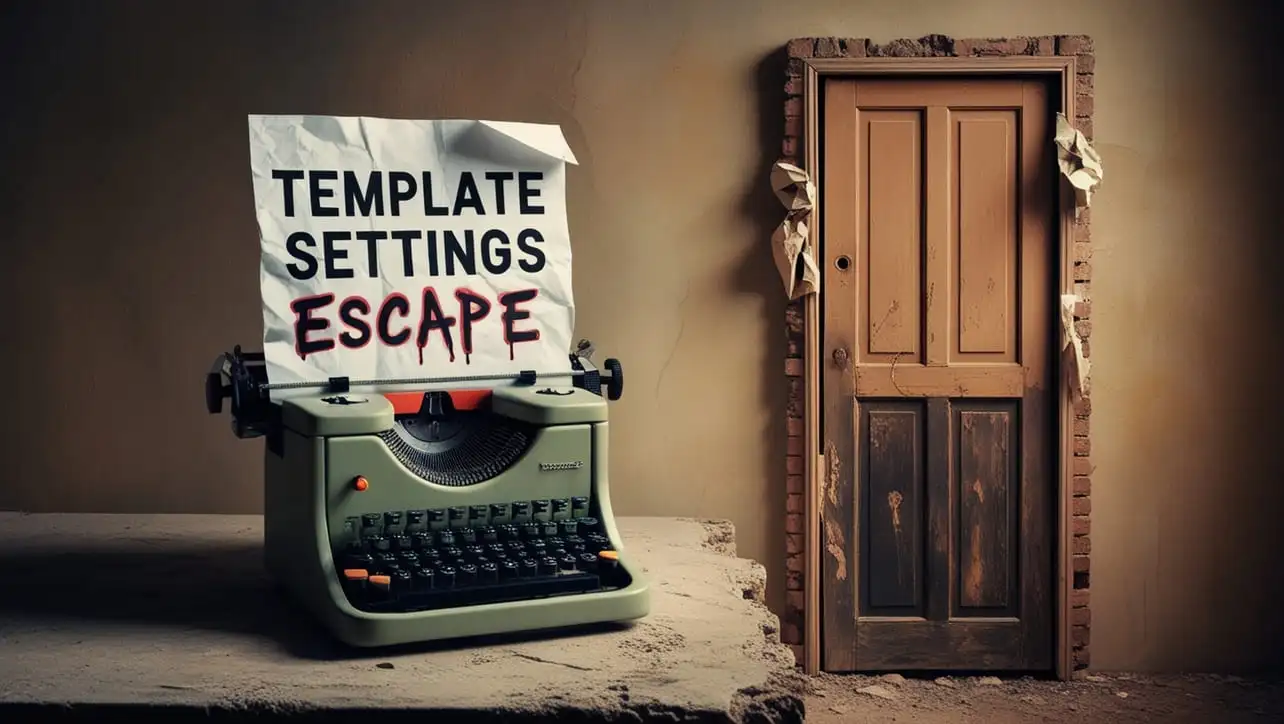
Lodash _.templateSettings.escape Property
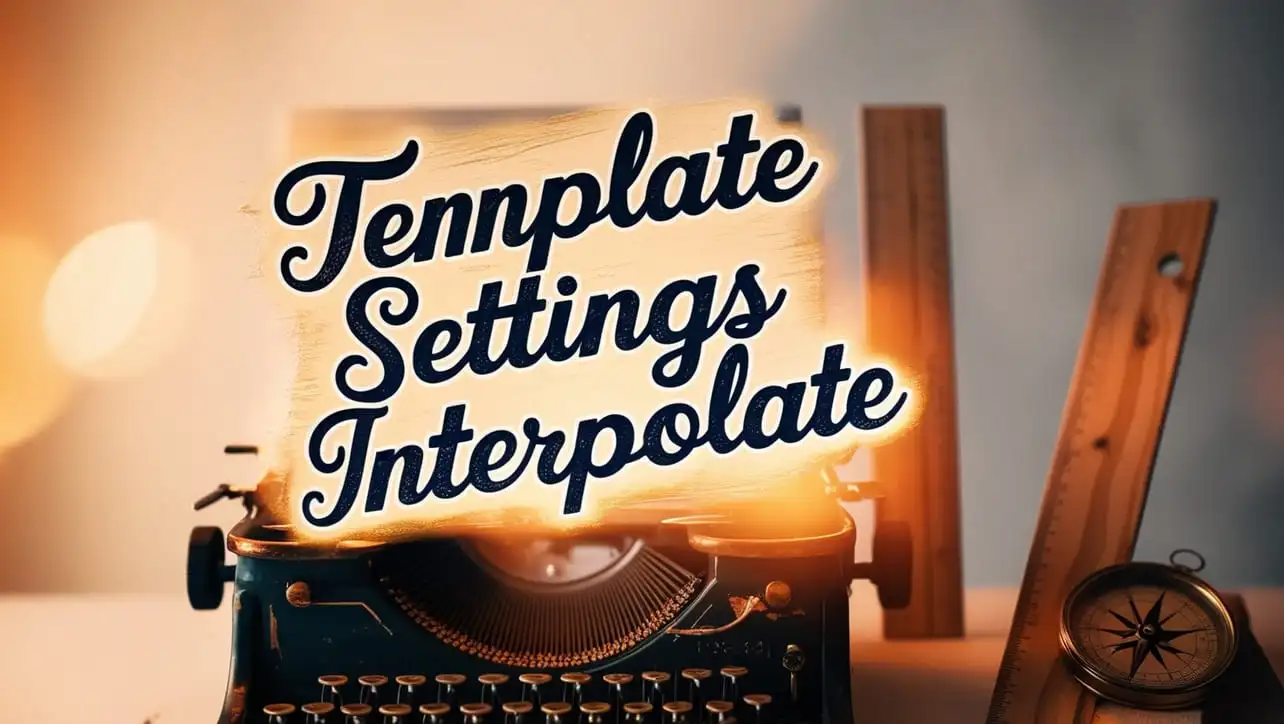
Lodash _.templateSettings.interpolate Property
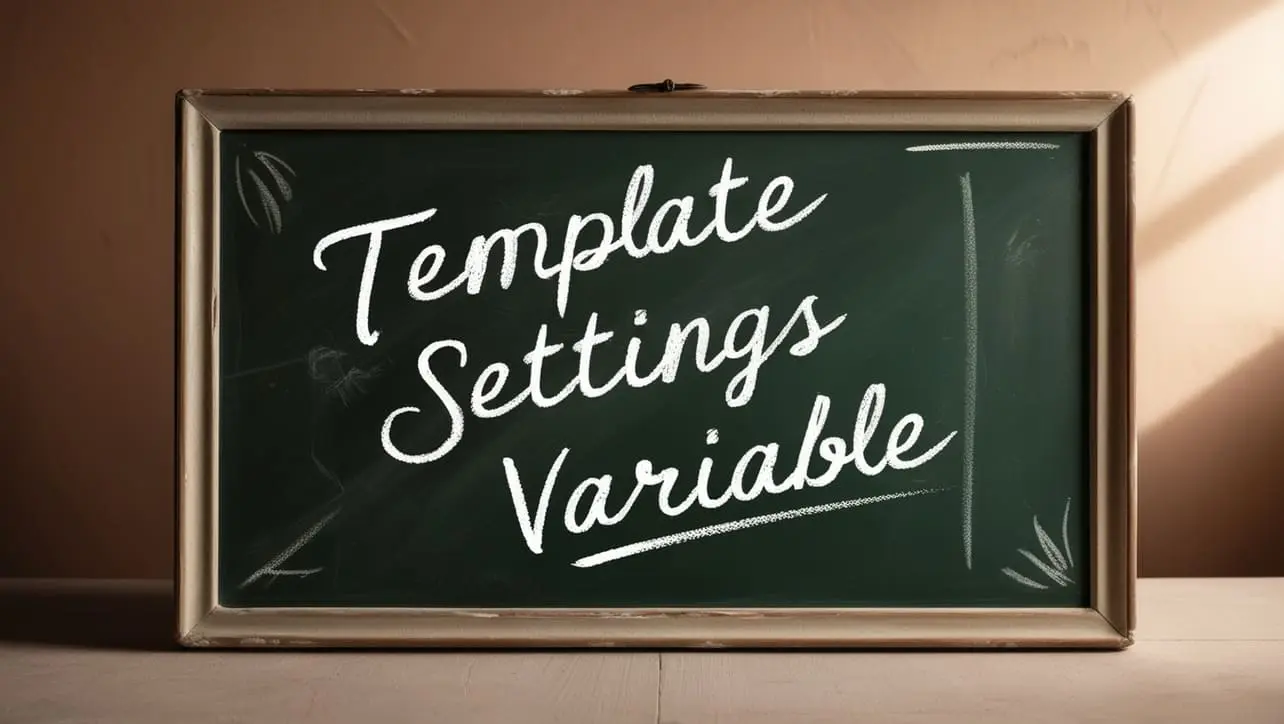
If you have any doubts regarding this article (Lodash _.toPairsIn() Object Method), please comment here. I will help you immediately.