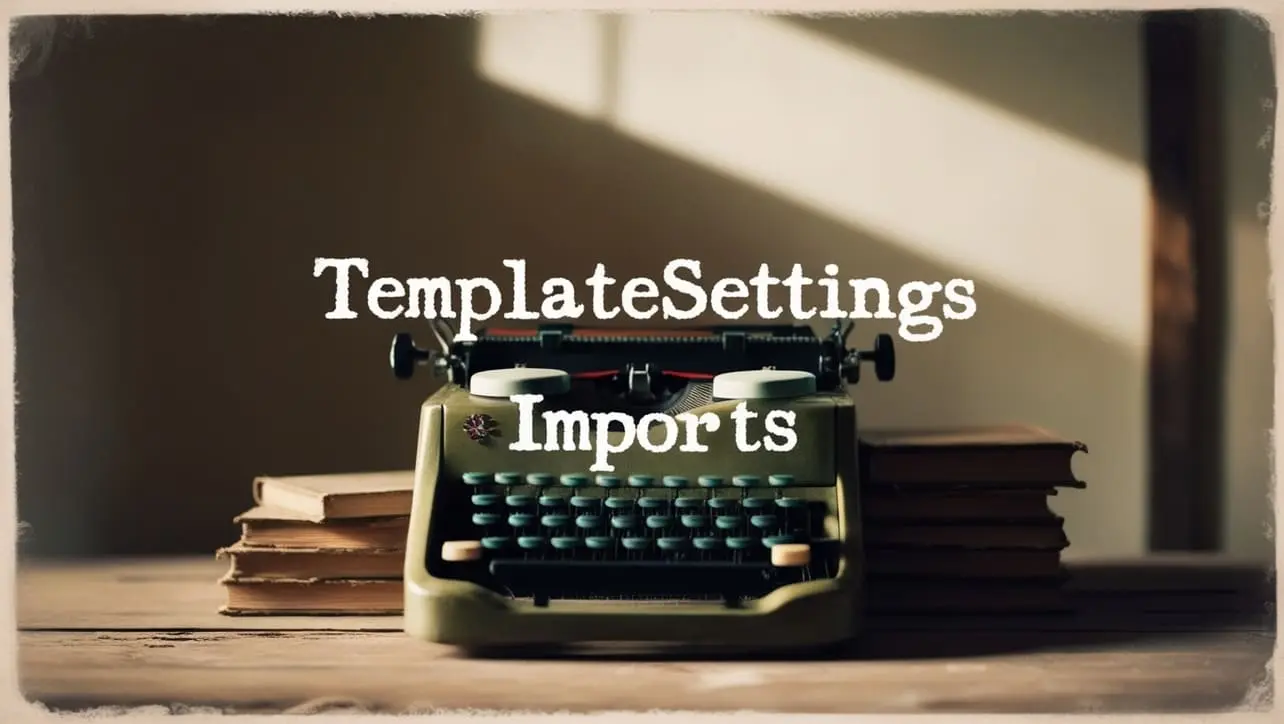
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.setWith() Object Method
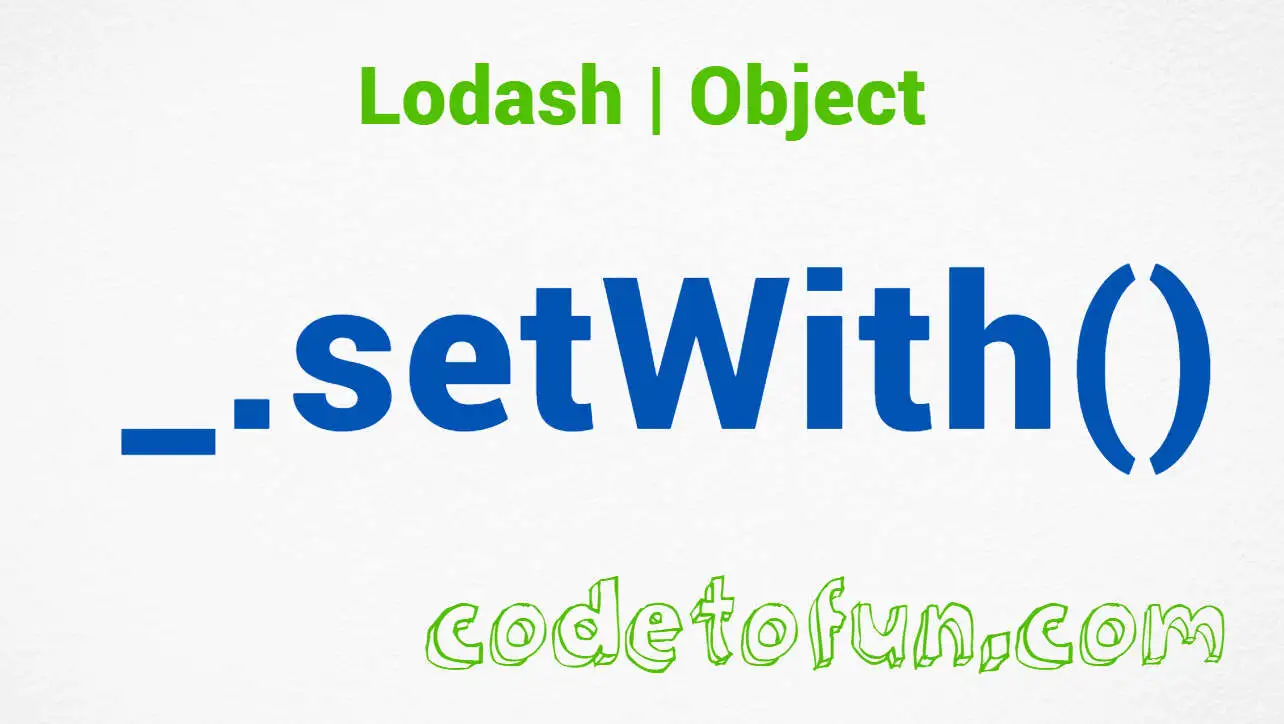
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript development, managing complex nested objects efficiently is crucial. Lodash, a popular utility library, provides a wide array of functions to simplify object manipulation tasks. Among these functions, the _.setWith()
method stands out as a powerful tool for setting nested properties within objects while allowing customizer functions for precise control.
This method enhances code clarity and flexibility, making it invaluable for developers working with nested data structures.
🧠 Understanding _.setWith() Method
The _.setWith()
method in Lodash facilitates the setting of nested properties within objects, with the option to customize the assignment behavior using a provided customizer function. This empowers developers to tailor the setting process according to specific requirements, enhancing the versatility and utility of the method.
💡 Syntax
The syntax for the _.setWith()
method is straightforward:
_.setWith(object, path, value, [customizer])
- object: The object to modify.
- path: The path of the property to set.
- value: The value to set.
- customizer (Optional): The function invoked to customize assigned values.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.setWith()
method:
const _ = require('lodash');
const object = {};
_.setWith(object, 'a[0].b.c', 42, Object);
console.log(object);
// Output: { a: [ { b: { c: 42 } } ] }
In this example, the _.setWith()
method is used to set a deeply nested property a[0].b.c within the object with the value 42.
🏆 Best Practices
When working with the _.setWith()
method, consider the following best practices:
Handle Custom Assignments:
Utilize the customizer function to customize the assignment behavior when setting nested properties. This allows you to implement complex logic or handle edge cases during the setting process.
example.jsCopiedconst object = {}; const customizer = (value, key, obj) => { if(_.isArray(obj)) { obj.push(value); } else { obj[key] = value; } }; _.setWith(object, 'a[0].b.c', 42, customizer); console.log(object); // Output: { a: [ { b: { c: 42 } } ] }
Error Handling:
Implement error handling mechanisms to gracefully handle scenarios where setting a property encounters issues, such as invalid paths or conflicts with existing properties.
example.jsCopiedconst object = { a: { b: { c: 42 } } }; try { _.setWith(object, 'a.b.c', 42, Object); } catch (error) { console.error('Error setting property:', error.message); } console.log(object); // Output: { a: { b: { c: 42 } } }
Performance Optimization:
Consider the performance implications, especially when dealing with deeply nested objects or frequent setting operations. Optimize your code and utilize
_.setWith()
judiciously to maintain optimal performance.example.jsCopiedconst object = {}; const path = 'a.b.c'; const value = 42; console.time('setWith'); _.setWith(object, path, value); console.timeEnd('setWith'); console.log(object);
📚 Use Cases
Dynamic Object Creation:
_.setWith()
is useful for dynamically creating nested objects with specified properties and values. This is particularly handy when dealing with data structures that evolve or are constructed dynamically.example.jsCopiedconst createNestedObject = (path, value) => { const object = {}; _.setWith(object, path, value); return object; }; const nestedObject = createNestedObject('a.b.c', 42); console.log(nestedObject); // Output: { a: { b: { c: 42 } } }
Deep Object Modification:
When you need to modify deeply nested properties within existing objects,
_.setWith()
provides a convenient and reliable solution, allowing you to precisely target and update specific properties.example.jsCopiedconst object = { a: { b: { c: 42 } } }; _.setWith(object, 'a.b.c', 99, Object); console.log(object); // Output: { a: { b: { c: 99 } } }
Custom Property Handling:
For scenarios requiring custom property handling logic, such as special formatting or validation,
_.setWith()
offers the flexibility to integrate customizer functions, enabling tailored property assignments.example.jsCopiedconst object = {}; const customizer = (value, key, obj) => { if(_.isNumber(value)) { obj[key] = value * 2; } else { obj[key] = value; } }; _.setWith(object, 'a.b.c', 21, customizer); console.log(object); // Output: { a: { b: { c: 42 } } }
🎉 Conclusion
The _.setWith()
method in Lodash empowers developers to efficiently set nested properties within objects, with customizable behavior to handle diverse use cases. Whether you're dynamically constructing objects, modifying existing data structures, or implementing custom property assignments, _.setWith()
provides a versatile and reliable solution for object manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.setWith()
method in your Lodash projects.
👨💻 Join our Community:
Author
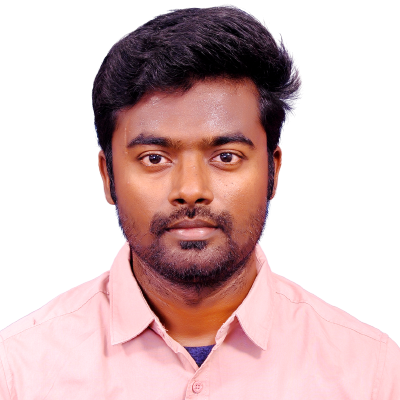
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
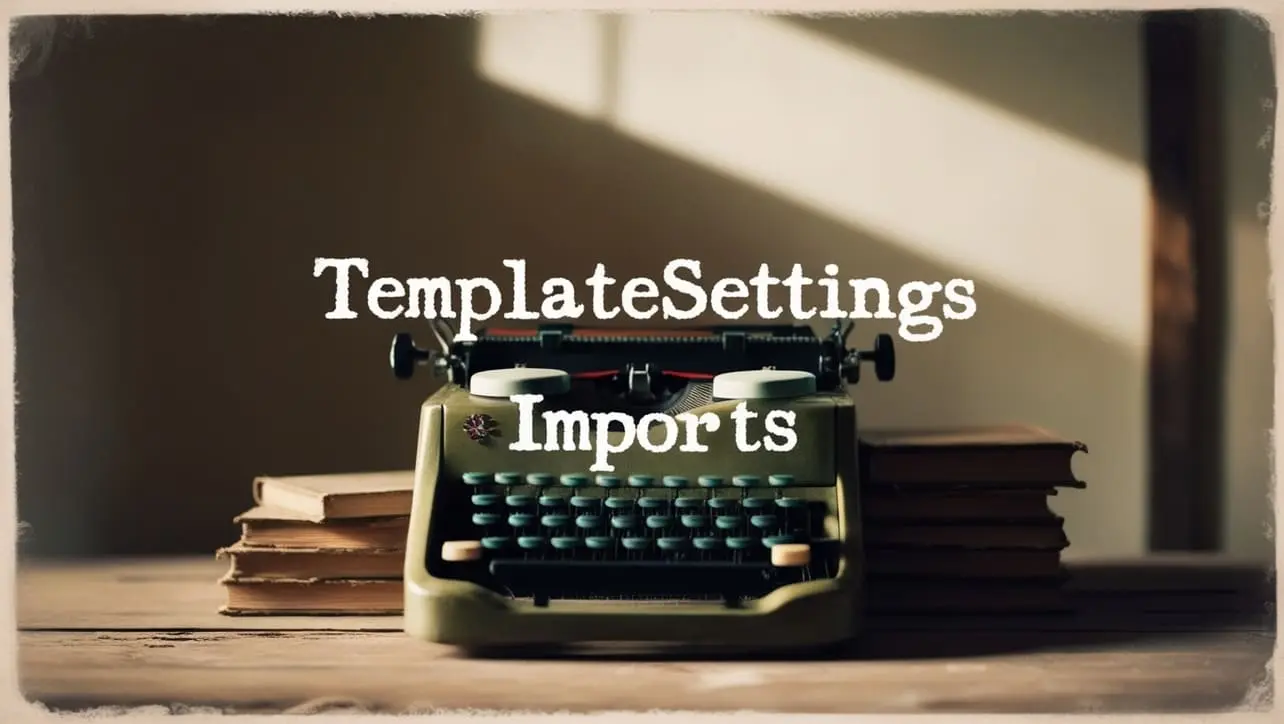
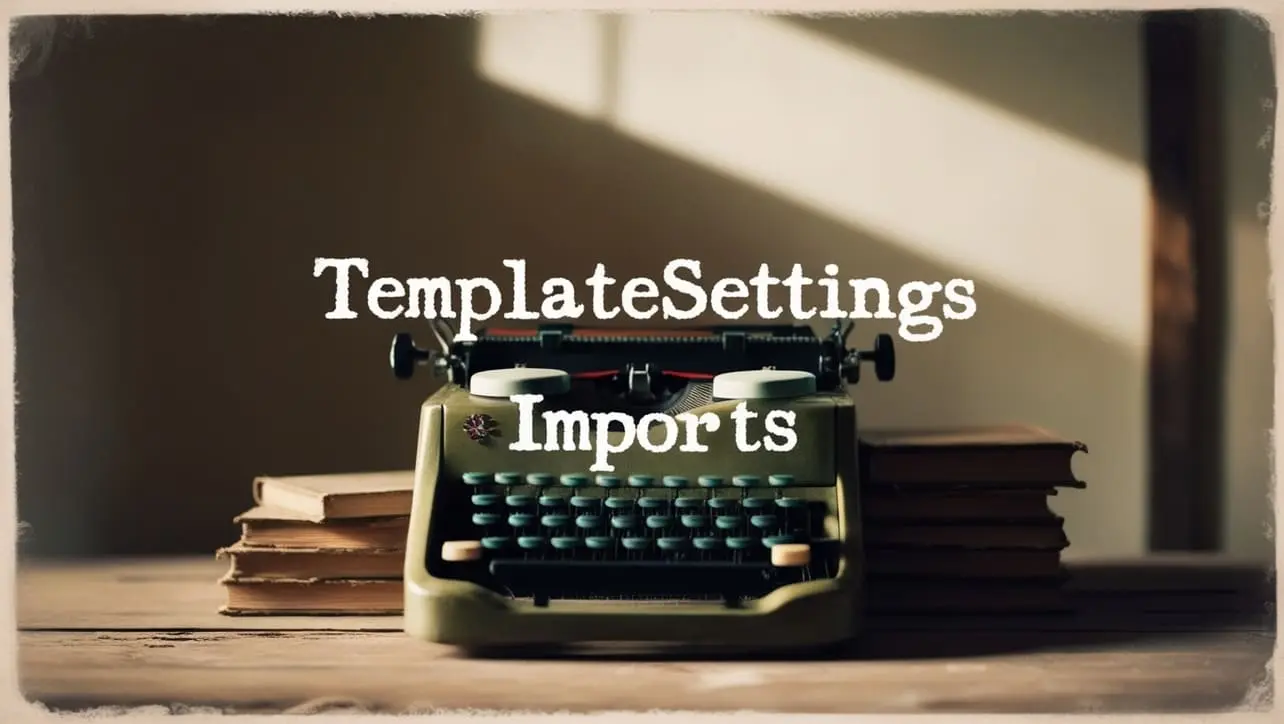
Lodash _.templateSettings.imports Property
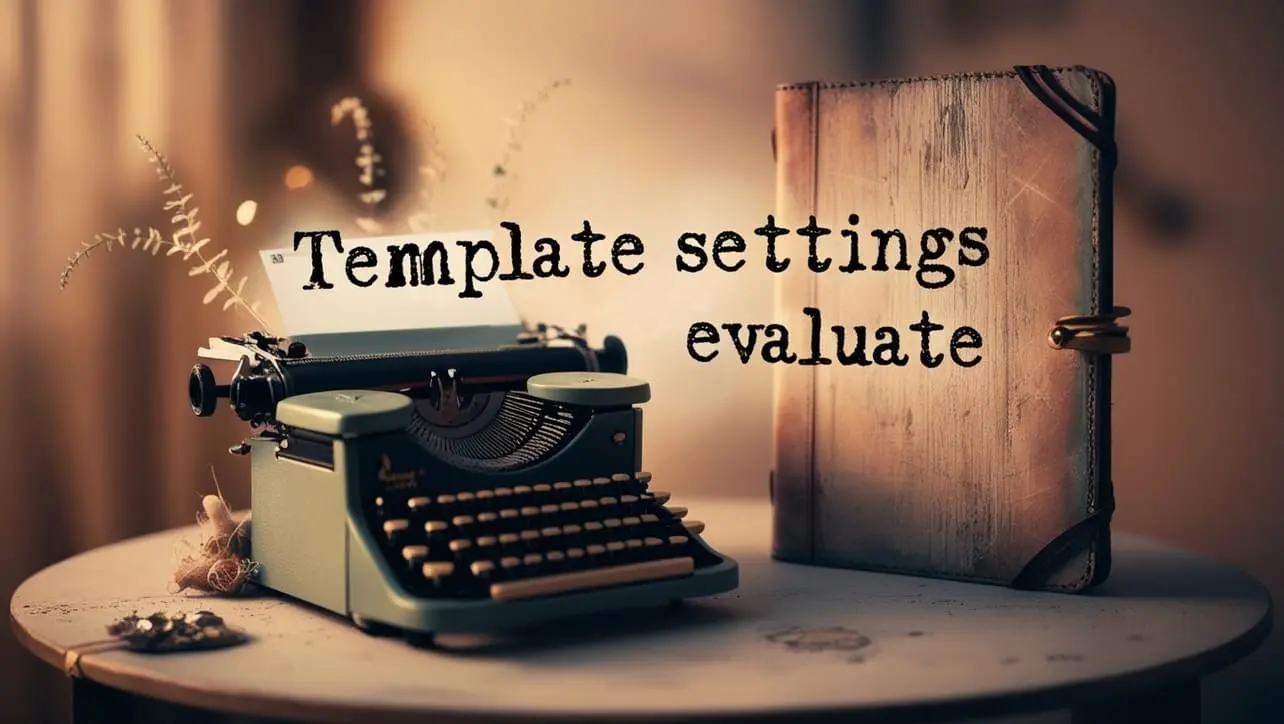
Lodash _.templateSettings.evaluate Property
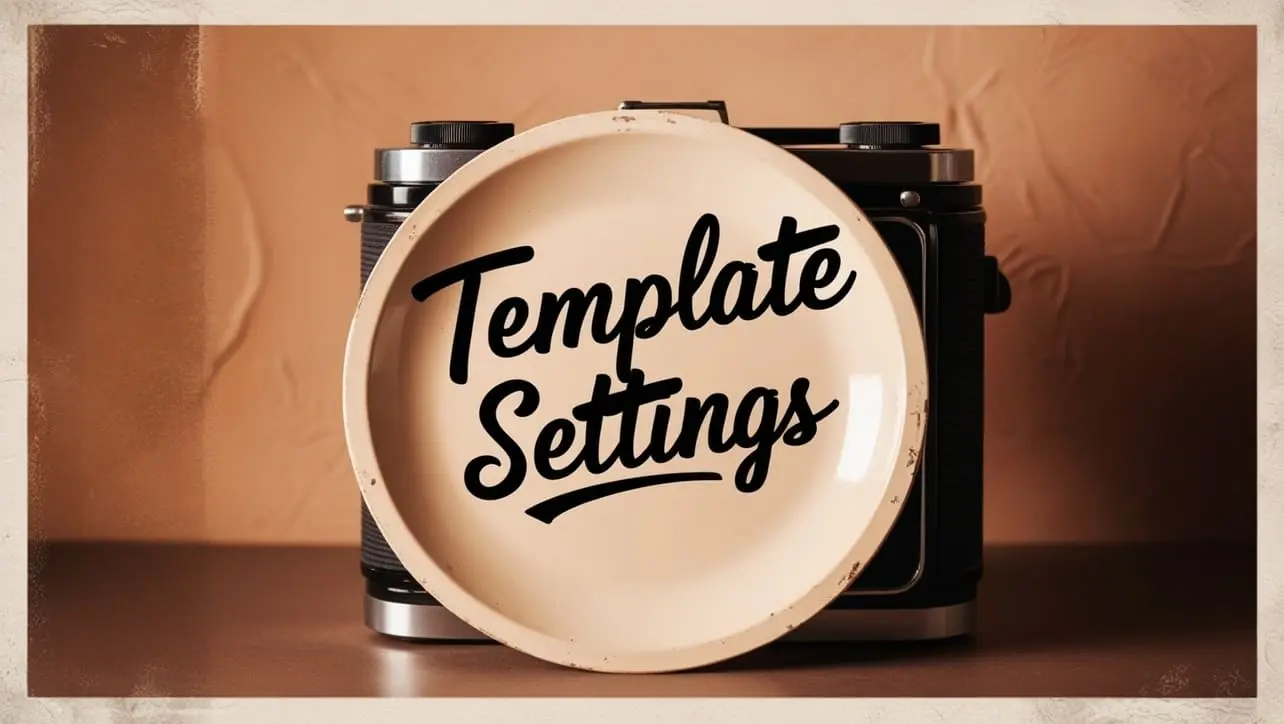
Lodash _.templateSettings Property
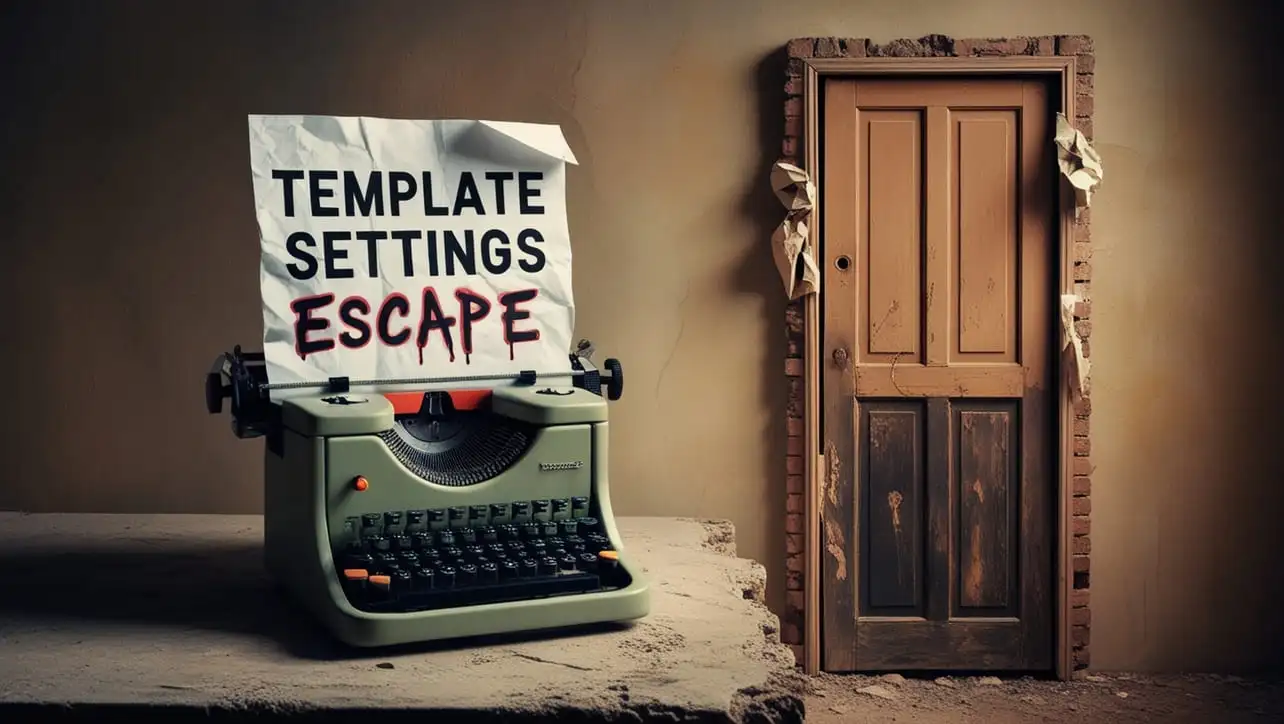
Lodash _.templateSettings.escape Property
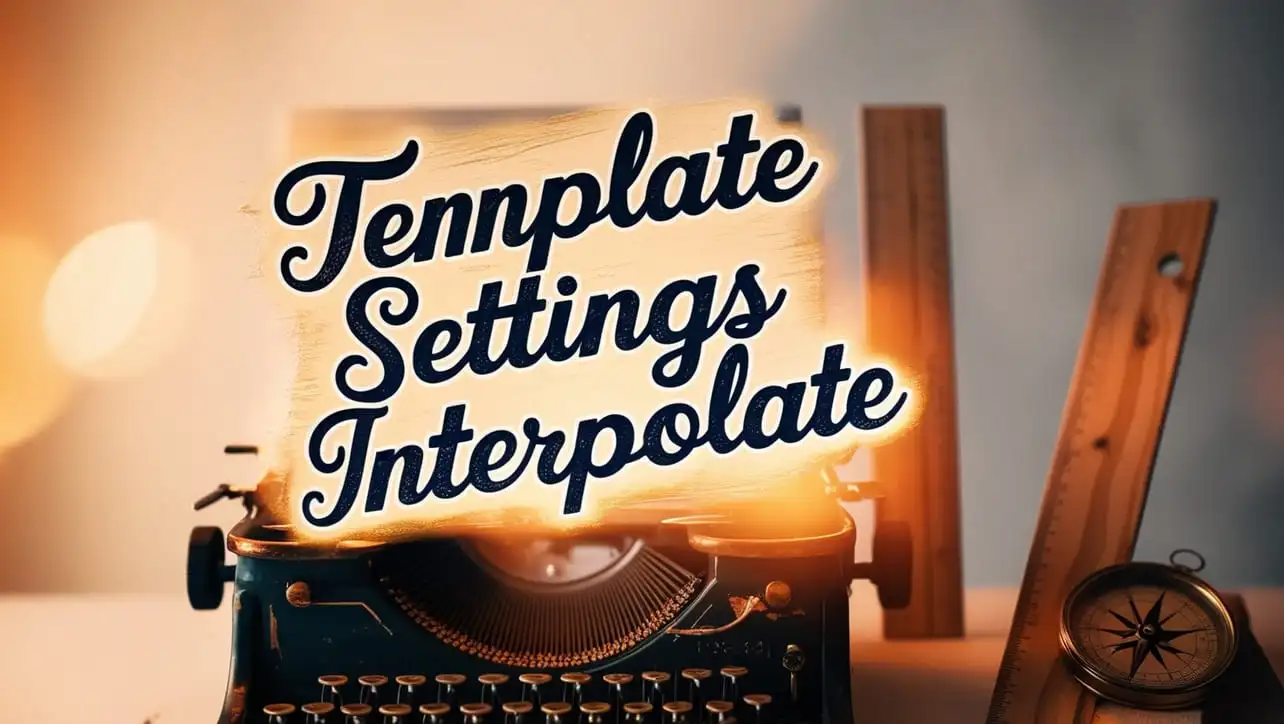
Lodash _.templateSettings.interpolate Property
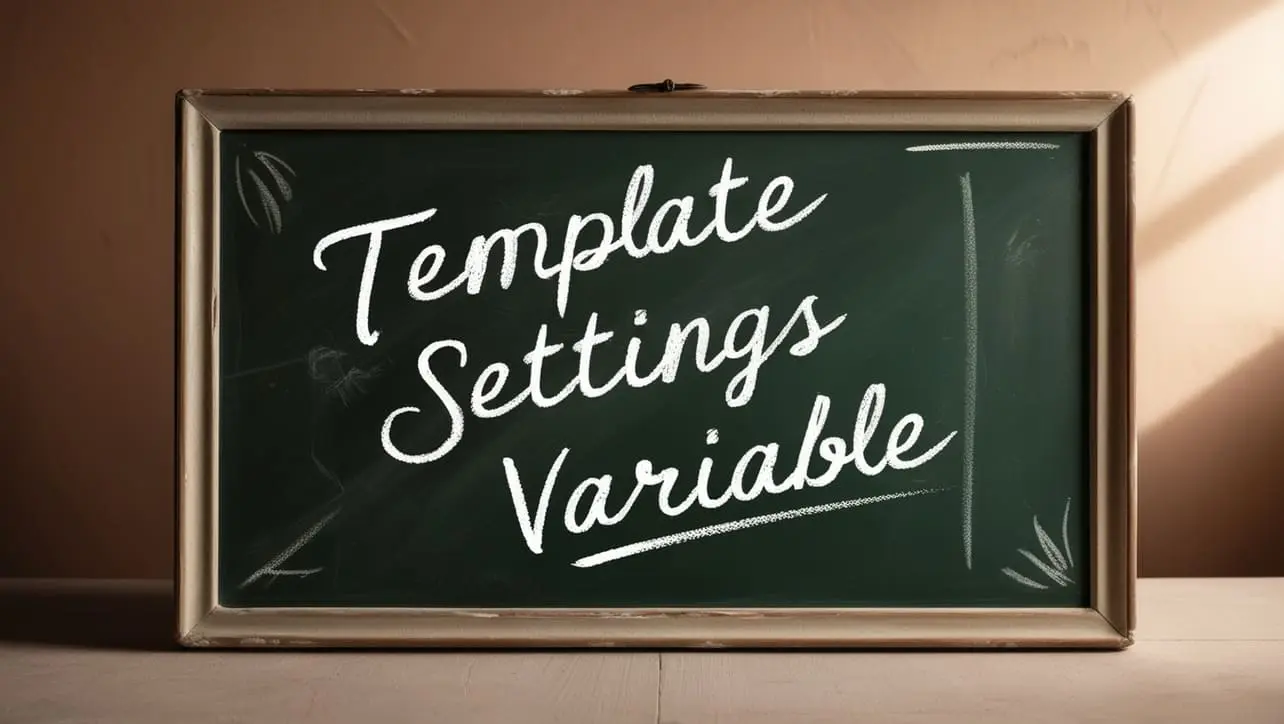
If you have any doubts regarding this article (Lodash _.setWith() Object Method), please comment here. I will help you immediately.