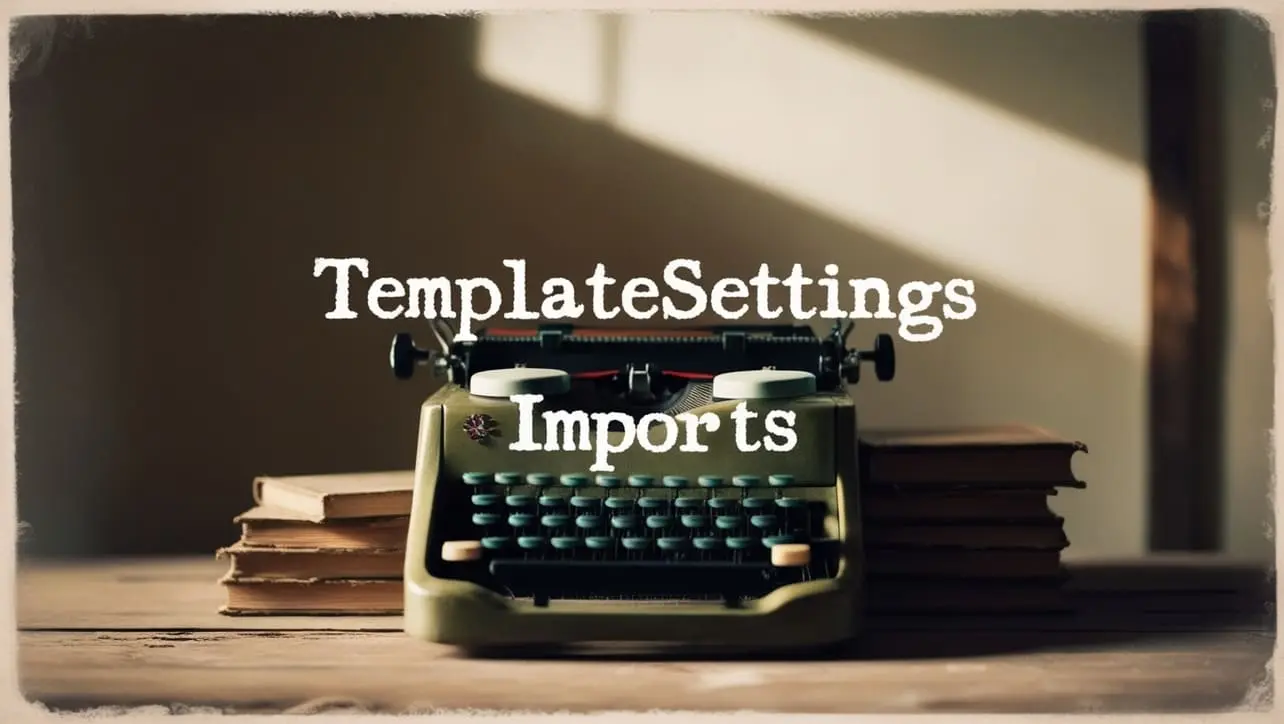
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.pick() Object Method
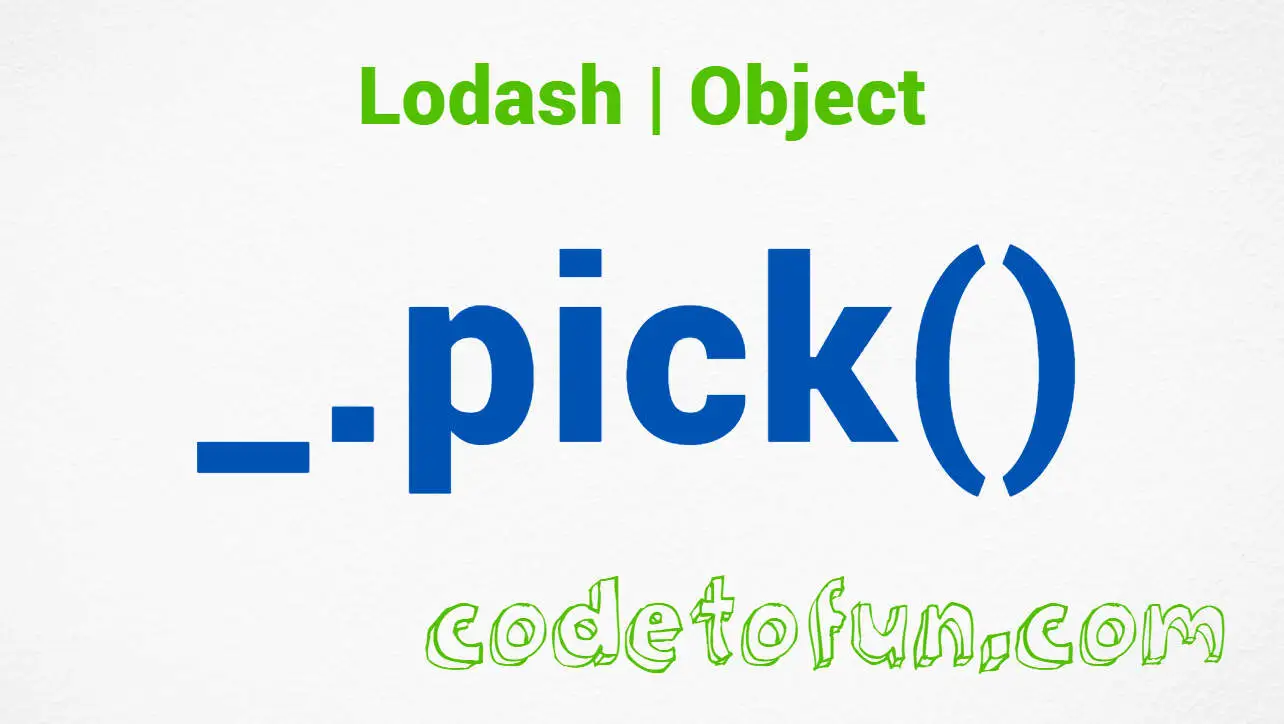
Photo Credit to CodeToFun
🙋 Introduction
In the realm of JavaScript programming, working with objects is a common task. However, dealing with large or complex objects often requires extracting specific properties of interest. This is where the _.pick()
method from the Lodash library comes into play.
_.pick()
enables developers to select and extract only the desired properties from an object, streamlining data manipulation and enhancing code clarity.
🧠 Understanding _.pick() Method
The _.pick()
method in Lodash allows you to extract specified properties from an object, creating a new object with only those properties included. This selective extraction simplifies data handling, reducing complexity and improving readability in your code.
💡 Syntax
The syntax for the _.pick()
method is straightforward:
_.pick(object, [props])
- object: The source object from which properties are to be picked.
- props: The property names to pick.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.pick()
method:
const _ = require('lodash');
const sourceObject = {
name: 'John Doe',
age: 30,
email: 'john@example.com',
isAdmin: true,
};
const pickedProperties = _.pick(sourceObject, ['name', 'email']);
console.log(pickedProperties);
// Output: { name: 'John Doe', email: 'john@example.com' }
In this example, the sourceObject is filtered using _.pick()
to create a new object containing only the specified properties.
🏆 Best Practices
When working with the _.pick()
method, consider the following best practices:
Specify Target Properties:
Be explicit about which properties you want to extract using
_.pick()
. This ensures clarity in your code and prevents unintended inclusion or exclusion of properties.example.jsCopiedconst user = { name: 'Alice', age: 25, email: 'alice@example.com', isAdmin: false, }; const filteredUser = _.pick(user, ['name', 'email']); console.log(filteredUser); // Output: { name: 'Alice', email: 'alice@example.com' }
Handle Non-existent Properties:
Ensure that the properties you intend to pick exist in the source object. Handling non-existent properties gracefully prevents errors and maintains code robustness.
example.jsCopiedconst user = { name: 'Bob', age: 30, }; const pickedUser = _.pick(user, ['name', 'email']); // 'email' property does not exist console.log(pickedUser); // Output: { name: 'Bob' }
Avoid Mutating Source Object:
Remember that
_.pick()
does not modify the source object; it creates a new object with the selected properties. Avoid relying on side effects and ensure that your source object remains unchanged.example.jsCopiedconst originalObject = { prop1: 'value1', prop2: 'value2', }; const pickedObject = _.pick(originalObject, ['prop1']); console.log(originalObject); // Output: { prop1: 'value1', prop2: 'value2' }
📚 Use Cases
Data Filtering:
_.pick()
is invaluable for filtering out unnecessary data properties, especially when dealing with large or nested objects. This streamlines data processing and optimizes memory usage.example.jsCopiedconst userData = { id: '123', name: 'John Doe', email: 'john@example.com', password: 'hashedpassword', isAdmin: true, // Other properties... }; const filteredUserData = _.pick(userData, ['id', 'name', 'email']); console.log(filteredUserData);
Object Transformation:
When transforming objects for specific purposes,
_.pick()
allows you to select only the relevant properties, simplifying the transformation process and improving code maintainability.example.jsCopiedconst rawApiResponse = { status: 'success', data: { id: '456', name: 'Jane Doe', email: 'jane@example.com', // Other properties... }, }; const transformedResponse = { status: rawApiResponse.status, userData: _.pick(rawApiResponse.data, ['id', 'name', 'email']), }; console.log(transformedResponse);
API Responses:
In API development,
_.pick()
can be used to tailor response objects, sending only the necessary data to clients. This reduces bandwidth usage and enhances API performance.example.jsCopiedapp.get('/user/:id', (req, res) => { const userId = req.params.id; const user = /* ...fetch user data from database or elsewhere... */; const responseData = _.pick(user, ['id', 'name', 'email']); res.json(responseData); });
🎉 Conclusion
The _.pick()
method in Lodash provides a convenient solution for extracting specific properties from objects in JavaScript. Whether you're filtering data, transforming objects, or customizing API responses, _.pick()
empowers you to efficiently manipulate object data with precision and clarity.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.pick()
method in your Lodash projects.
👨💻 Join our Community:
Author
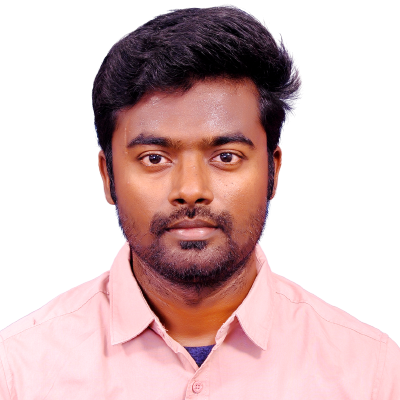
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
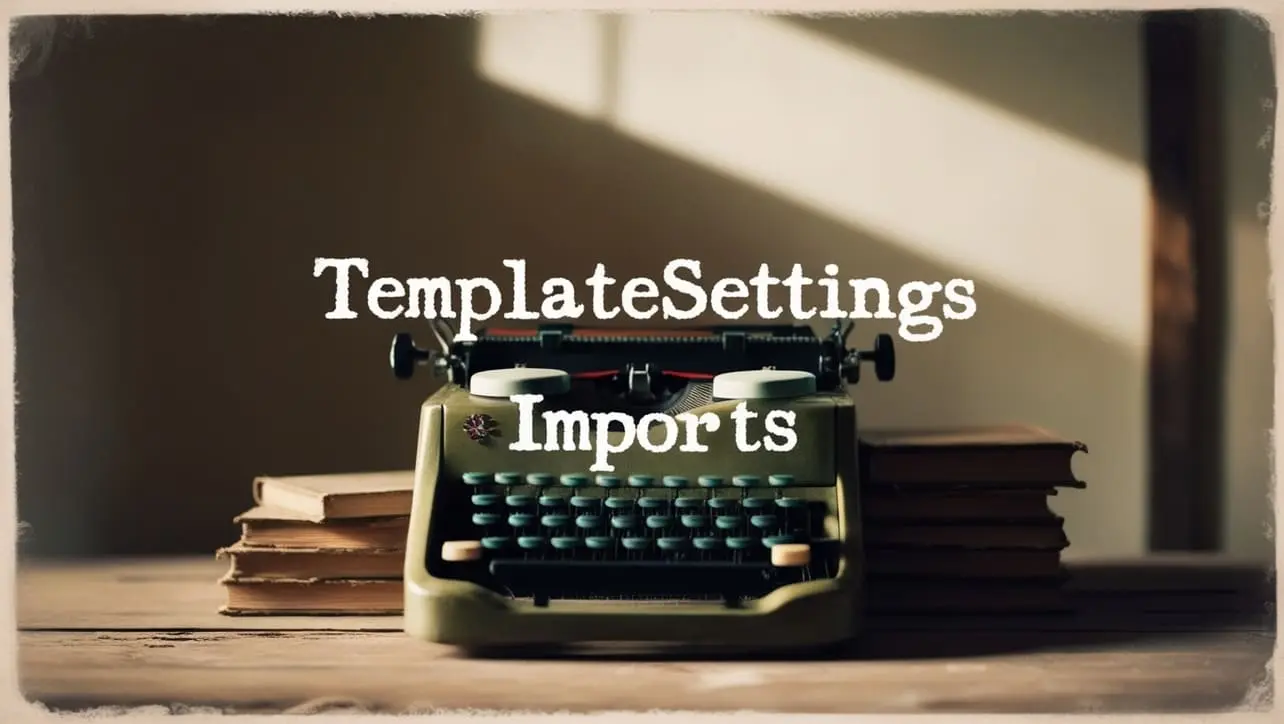
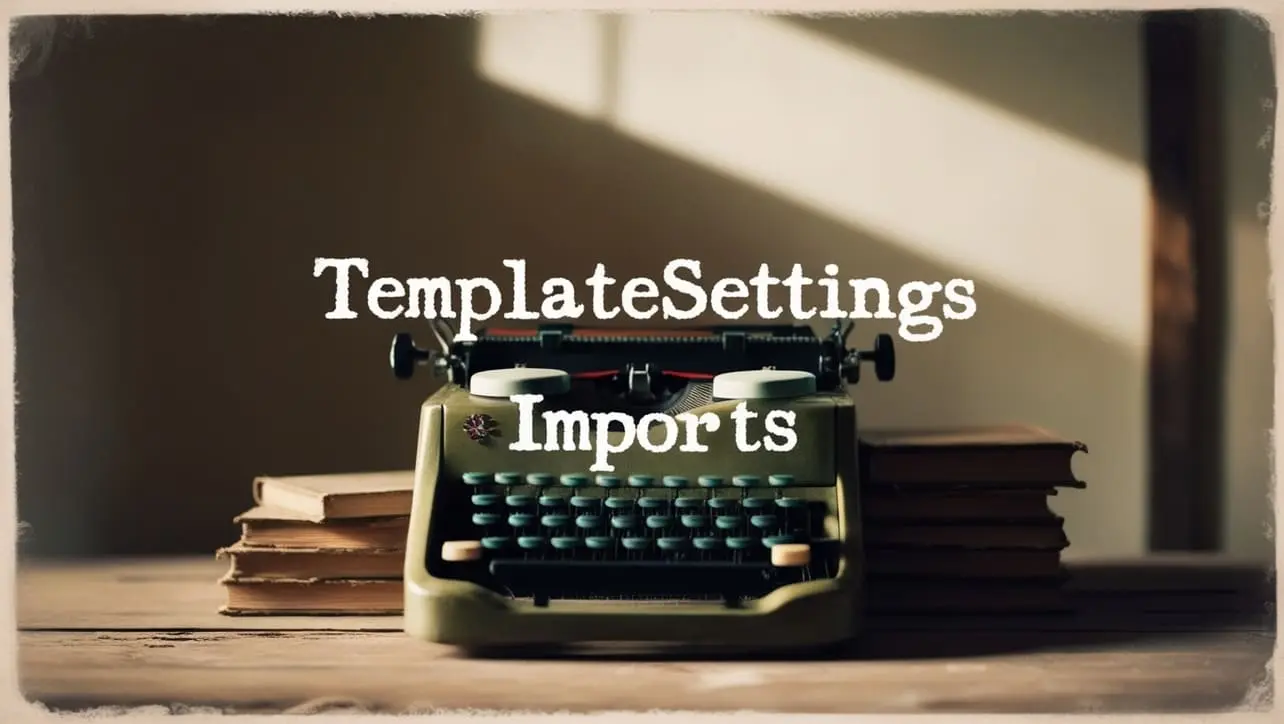
Lodash _.templateSettings.imports Property
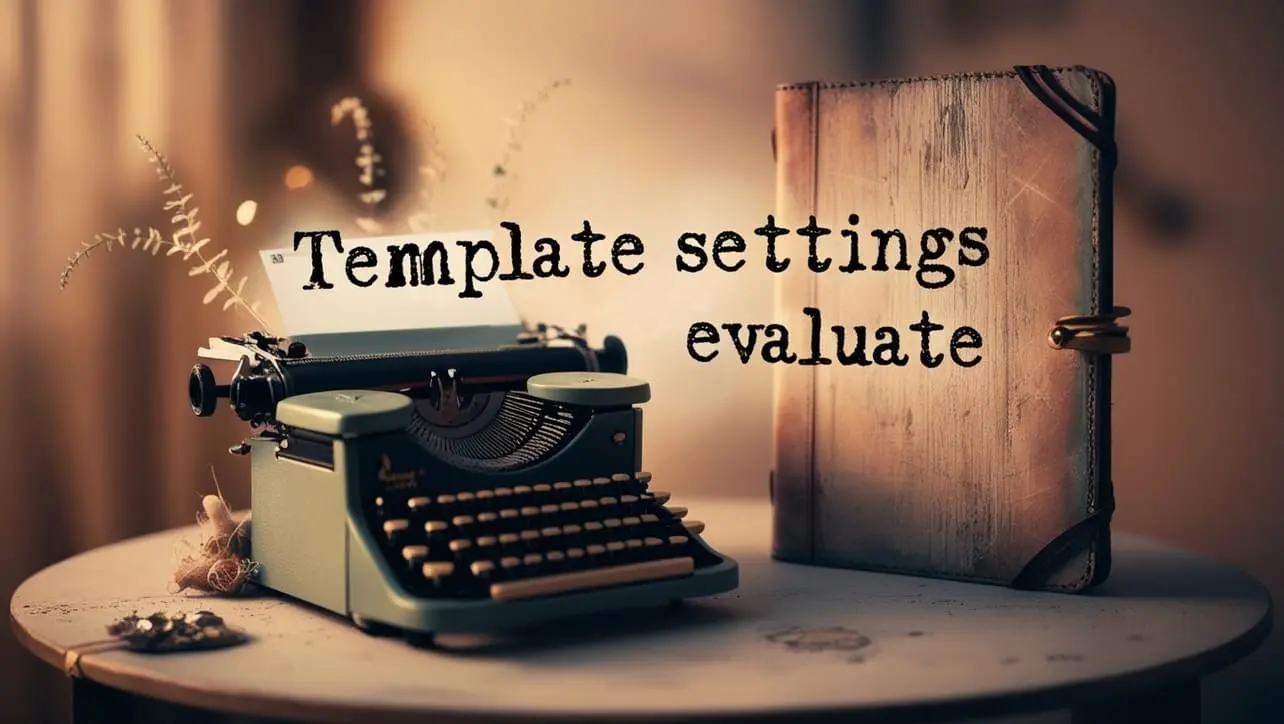
Lodash _.templateSettings.evaluate Property
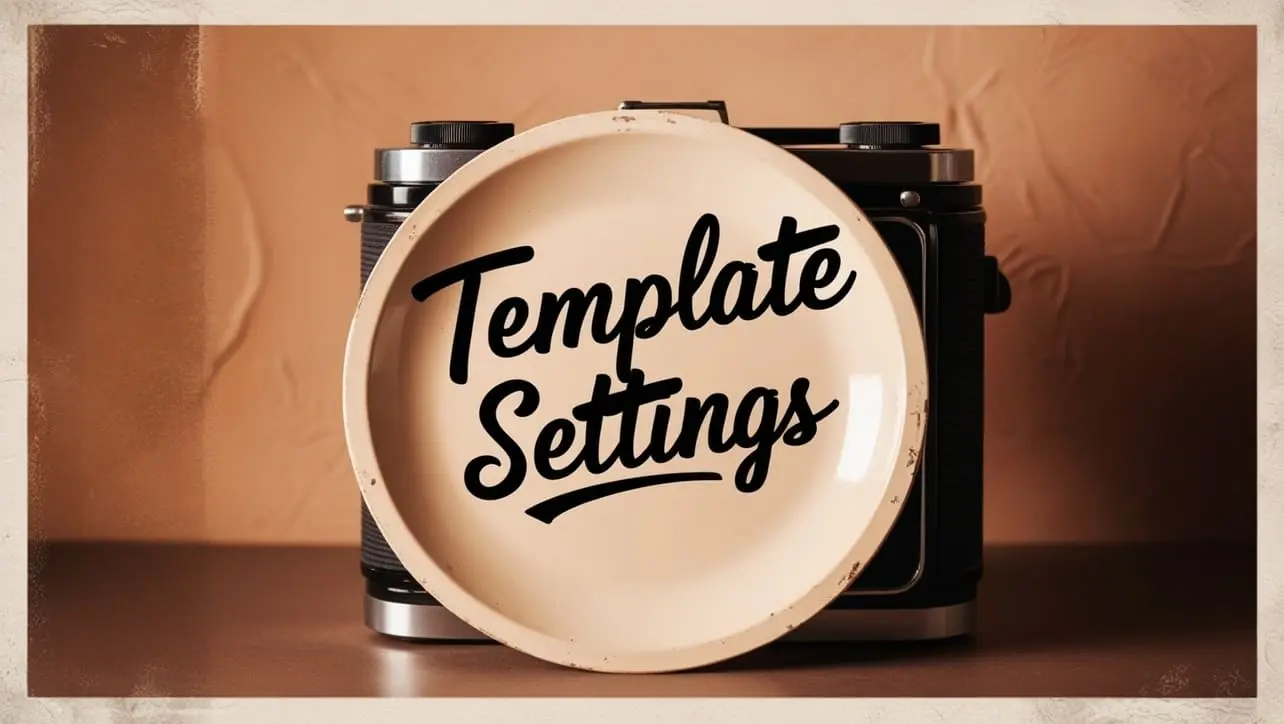
Lodash _.templateSettings Property
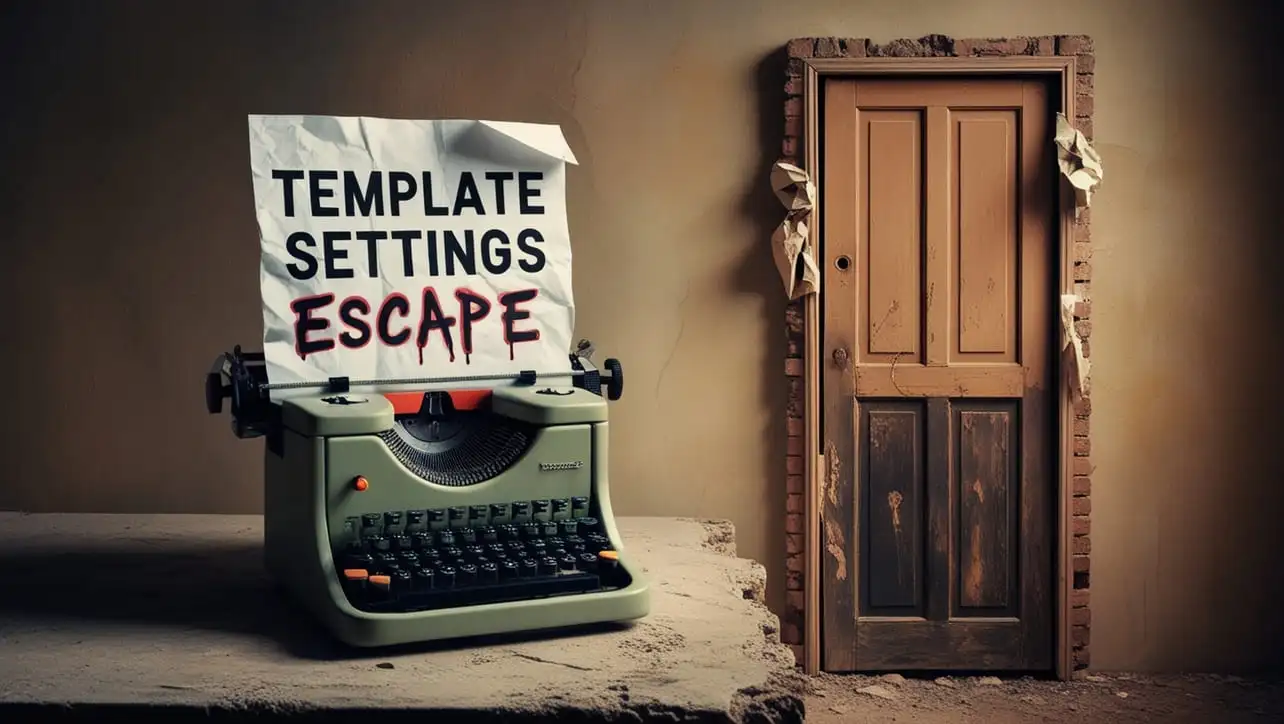
Lodash _.templateSettings.escape Property
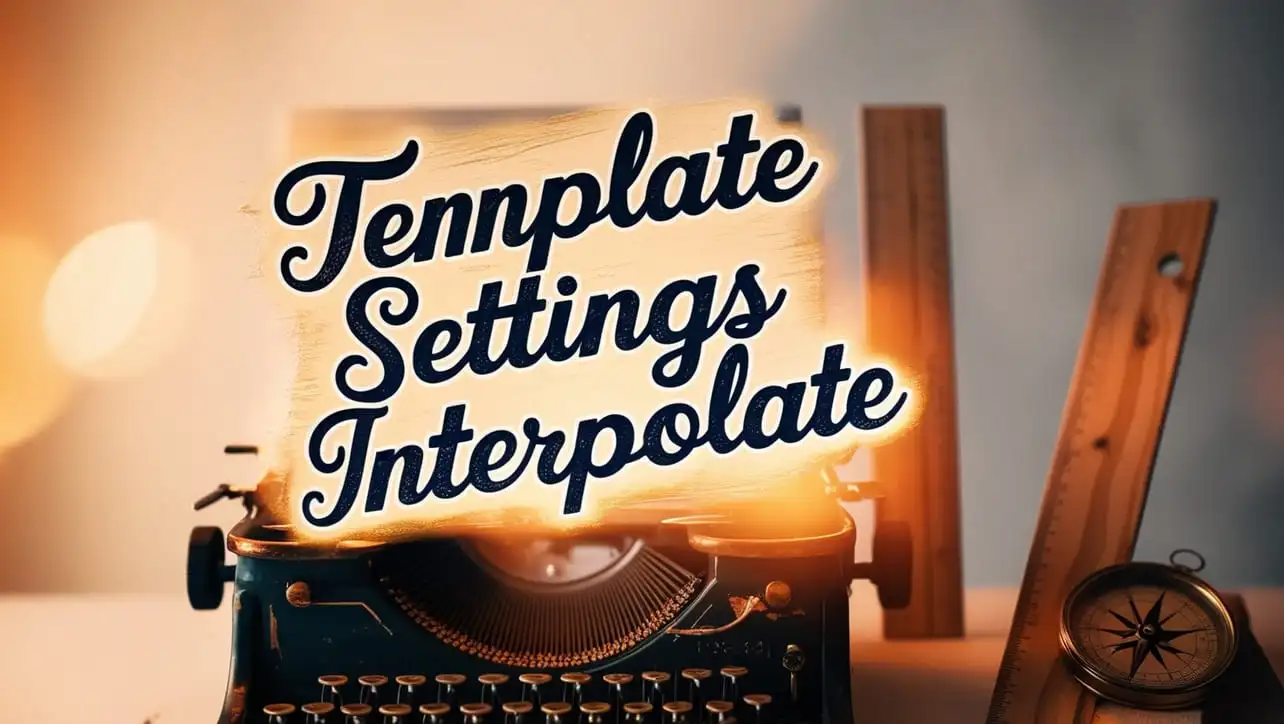
Lodash _.templateSettings.interpolate Property
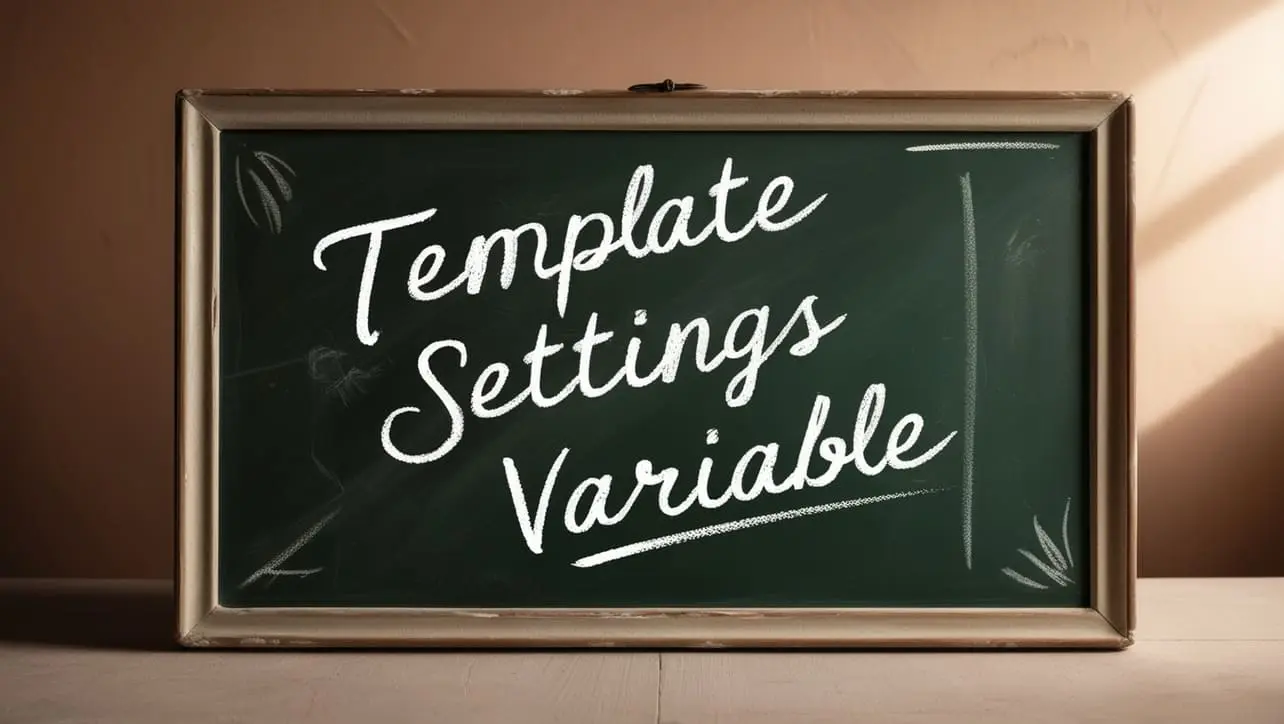
If you have any doubts regarding this article (Lodash _.pick() Object Method), please comment here. I will help you immediately.