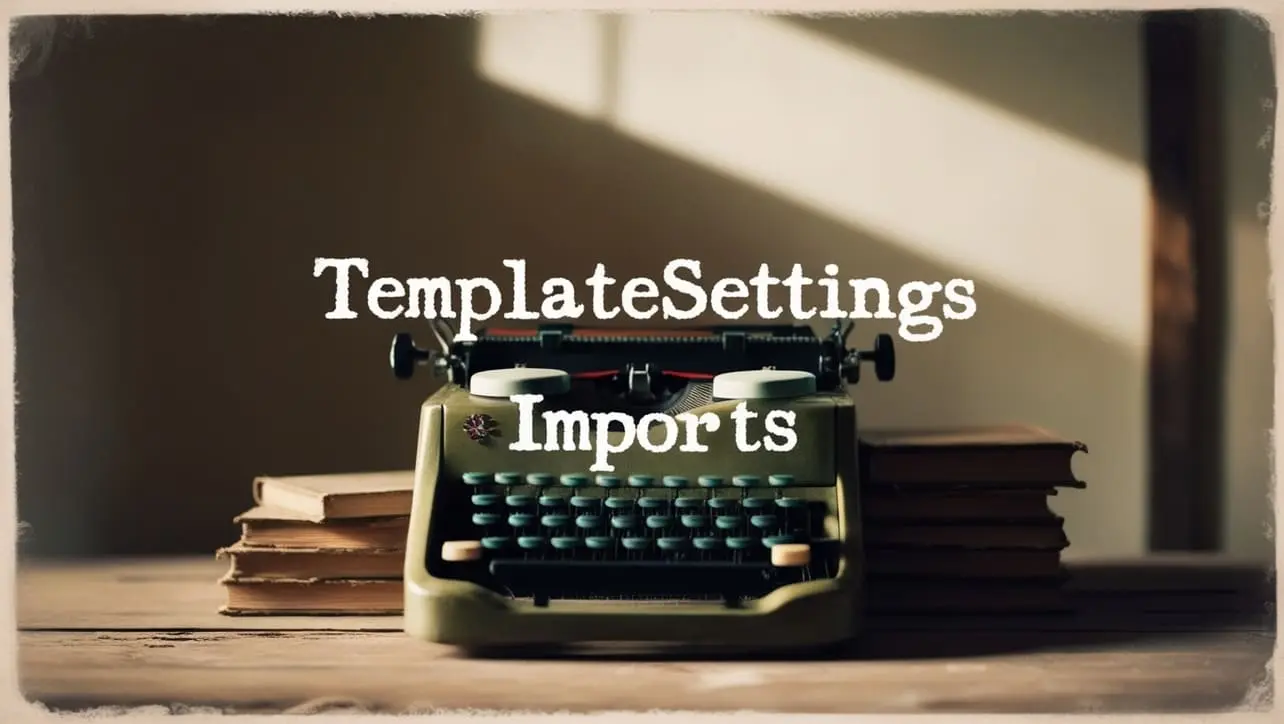
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.invertBy() Object Method
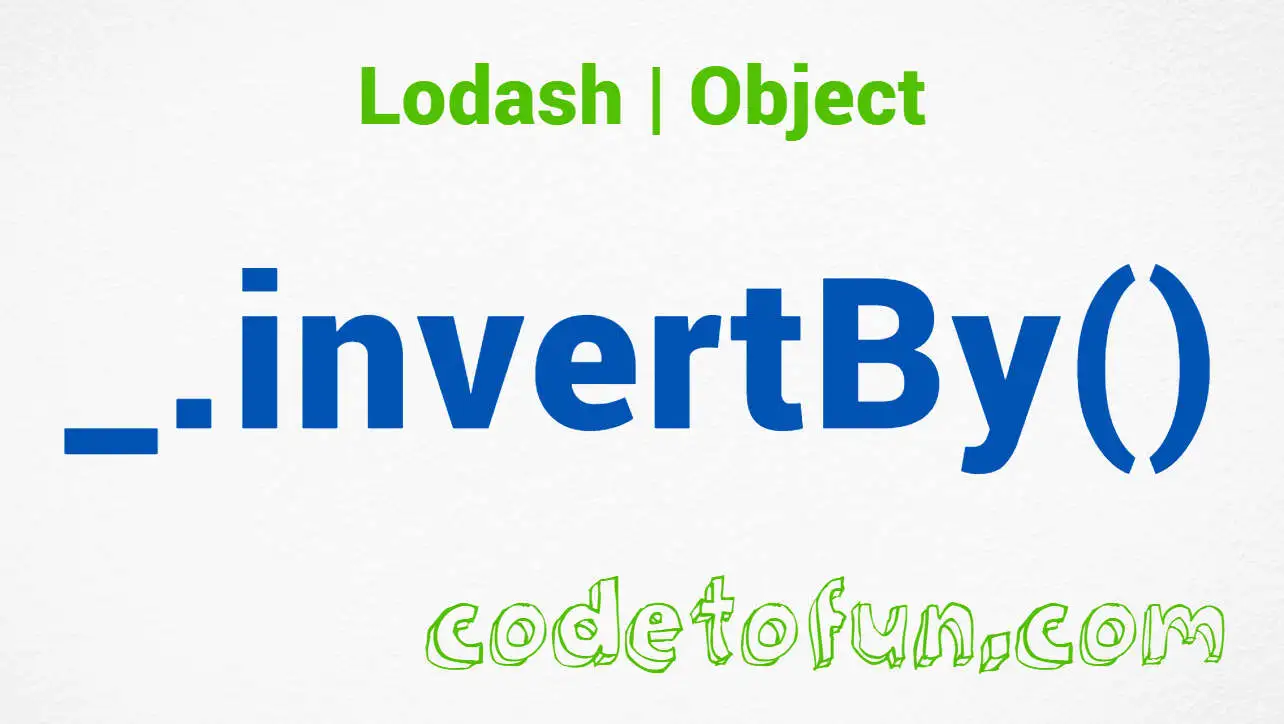
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, manipulating objects is a common task, and having efficient tools to handle them can greatly simplify coding tasks. Lodash, a popular utility library, offers a plethora of functions to streamline object manipulation, and one such function is _.invertBy()
.
This method is particularly useful for reorganizing object keys based on their corresponding values, providing a versatile tool for data transformation and analysis.
🧠 Understanding _.invertBy() Method
The _.invertBy()
method in Lodash is designed to invert the keys and values of an object, grouping the keys by their corresponding values. This can be especially handy when dealing with datasets where values are unique and act as identifiers. By flipping the structure of the object, _.invertBy()
facilitates efficient lookup and analysis of data.
💡 Syntax
The syntax for the _.invertBy()
method is straightforward:
_.invertBy(object, [iteratee])
- object: The object to invert.
- iteratee (Optional): The function invoked per iteration.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.invertBy()
method:
const _ = require('lodash');
const originalObject = {
a: 1,
b: 2,
c: 2,
d: 3,
};
const invertedObject = _.invertBy(originalObject);
console.log(invertedObject);
// Output: { '1': ['a'], '2': ['b', 'c'], '3': ['d'] }
In this example, the originalObject is inverted using _.invertBy()
, resulting in a new object where keys are values from the original object, and values are arrays of keys sharing the same value.
🏆 Best Practices
When working with the _.invertBy()
method, consider the following best practices:
Understand Object Structure:
Before applying
_.invertBy()
, ensure that the structure of the object aligns with your expectations. Understanding the keys and values within the object is crucial for interpreting the results accurately.example.jsCopiedconst originalObject = { apple: 'fruit', banana: 'fruit', carrot: 'vegetable', }; const invertedObject = _.invertBy(originalObject); console.log(invertedObject); // Output: { fruit: ['apple', 'banana'], vegetable: ['carrot'] }
Handle Value Conflicts:
Be mindful of potential conflicts when inverting an object, where multiple keys may map to the same value.
_.invertBy()
groups keys with the same value into arrays, providing a mechanism to handle such conflicts.example.jsCopiedconst originalObject = { apple: 'fruit', orange: 'fruit', banana: 'fruit', }; const invertedObject = _.invertBy(originalObject); console.log(invertedObject); // Output: { fruit: ['apple', 'orange', 'banana'] }
Utilize Iteratee Function:
The iteratee function allows you to customize the inversion process, enabling more advanced transformations based on specific criteria. Leveraging this functionality can enhance the versatility of
_.invertBy()
.example.jsCopiedconst originalObject = { 1: 'apple', 2: 'banana', 3: 'orange', }; const invertedObject = _.invertBy(originalObject, value => value.length); console.log(invertedObject); // Output: { 5: ['apple'], 6: ['banana', 'orange'] }
📚 Use Cases
Grouping Data:
_.invertBy()
is invaluable for grouping data based on common attributes or values, simplifying data analysis and organization tasks.example.jsCopiedconst salesData = { '2022-01-01': 100, '2022-01-02': 150, '2022-01-03': 100, '2022-01-04': 200, }; const invertedSalesData = _.invertBy(salesData); console.log(invertedSalesData);
Reorganizing Data Structures:
When dealing with complex data structures,
_.invertBy()
offers a straightforward way to reorganize data for easier access and manipulation.example.jsCopiedconst originalData = { alice: { id: 1, age: 25 }, bob: { id: 2, age: 30 }, charlie: { id: 3, age: 25 }, }; const invertedData = _.invertBy(originalData, obj => obj.age); console.log(invertedData);
Value-based Lookup:
For scenarios where value-based lookup is required,
_.invertBy()
provides a convenient solution by flipping the object's structure.example.jsCopiedconst phoneBook = { alice: '555-1234', bob: '555-5678', charlie: '555-9101', }; const invertedPhoneBook = _.invertBy(phoneBook); console.log(invertedPhoneBook);
🎉 Conclusion
The _.invertBy()
method in Lodash offers a versatile solution for reorganizing object keys based on their corresponding values. Whether you need to group data, restructure objects, or facilitate value-based lookup, _.invertBy()
provides a robust and efficient mechanism for object manipulation in JavaScript.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.invertBy()
method in your Lodash projects.
👨💻 Join our Community:
Author
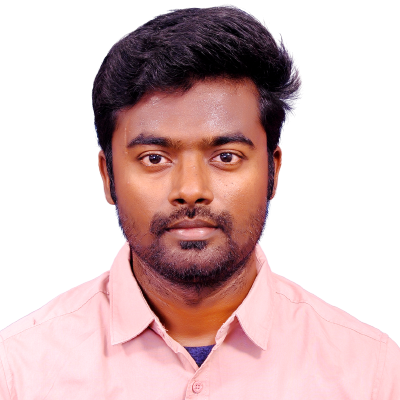
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
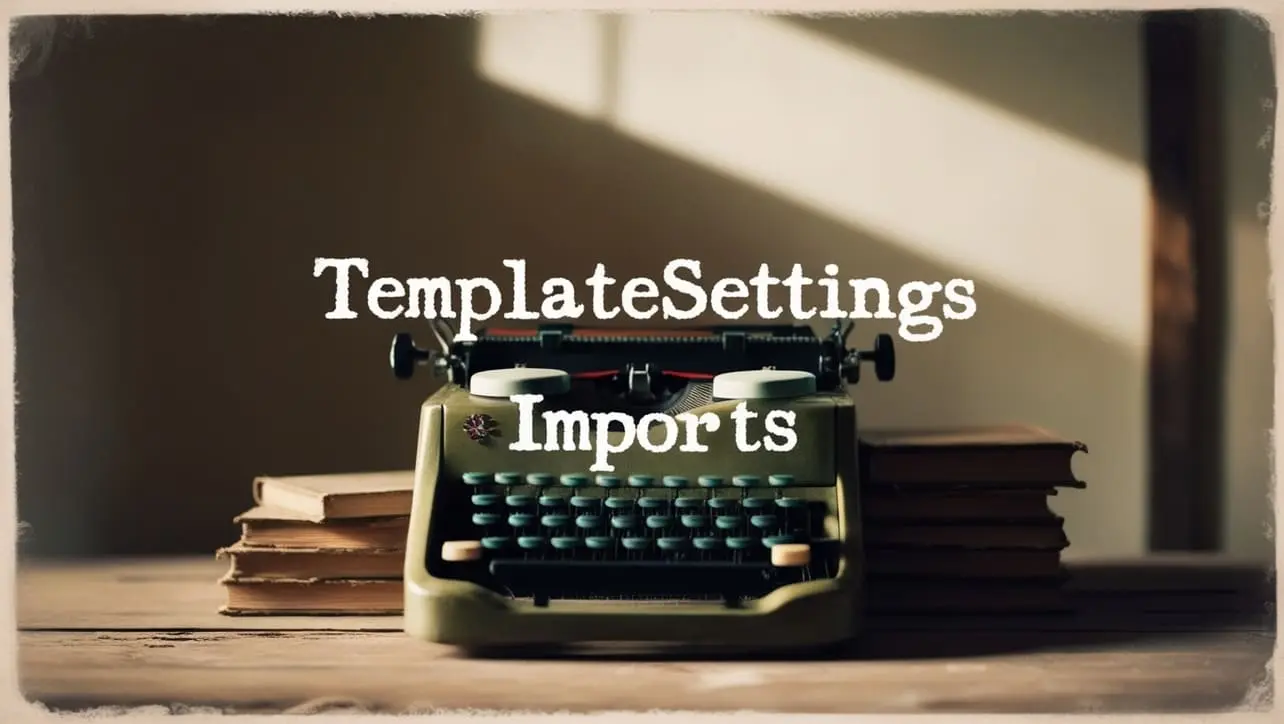
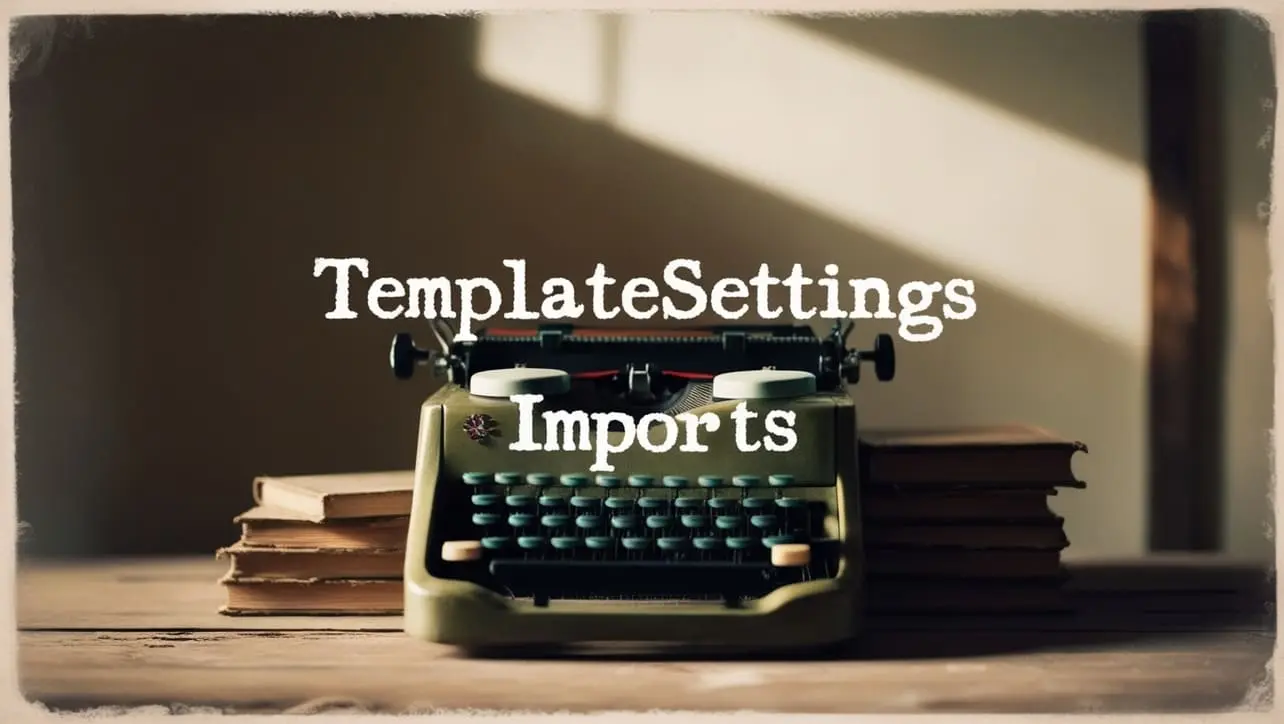
Lodash _.templateSettings.imports Property
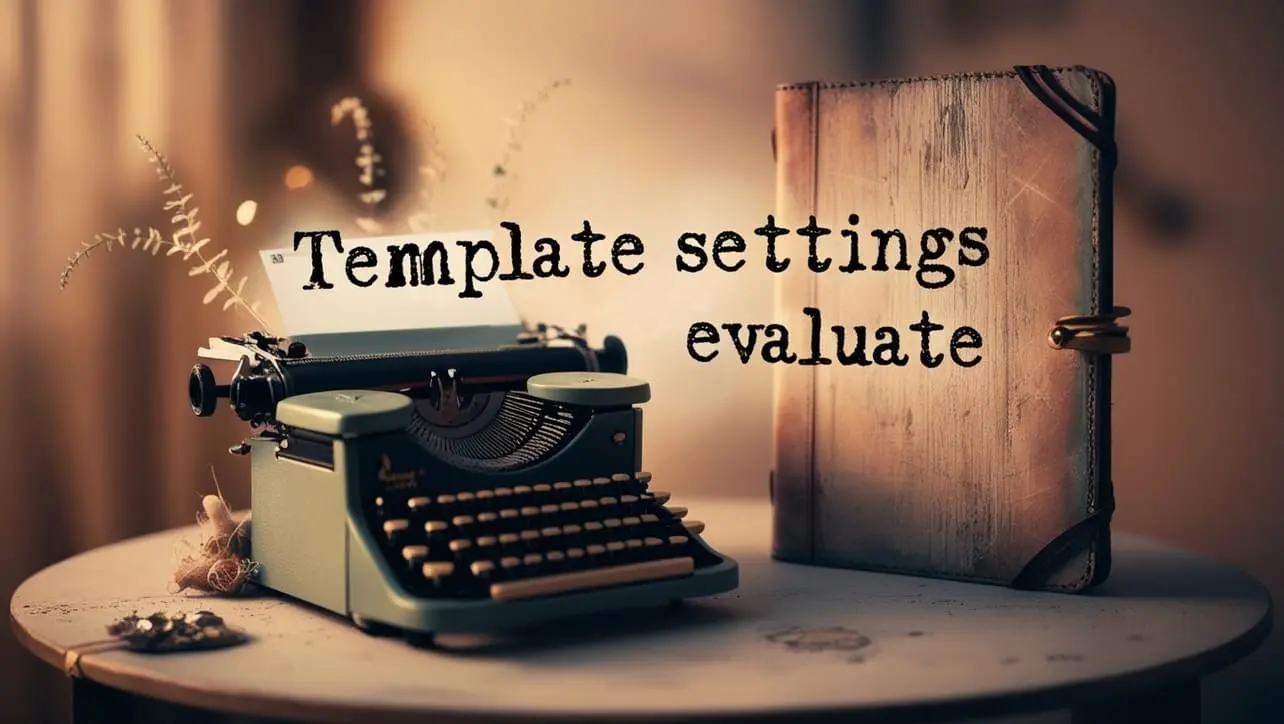
Lodash _.templateSettings.evaluate Property
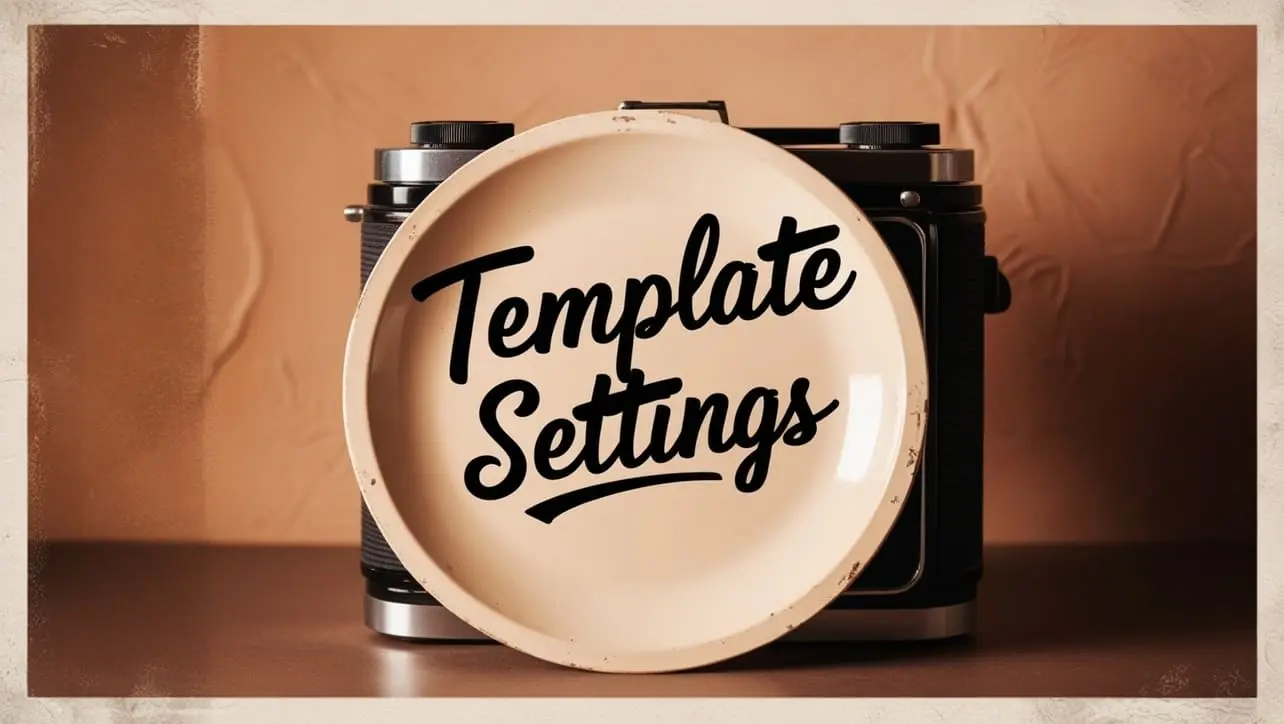
Lodash _.templateSettings Property
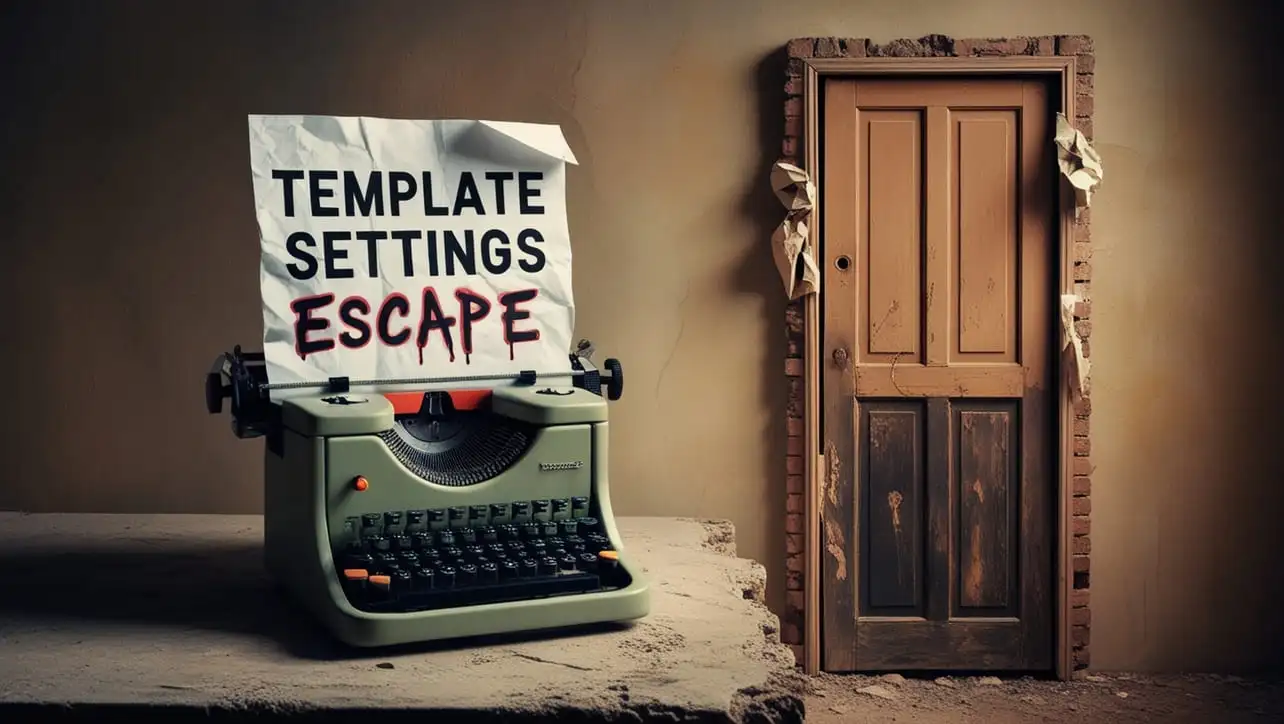
Lodash _.templateSettings.escape Property
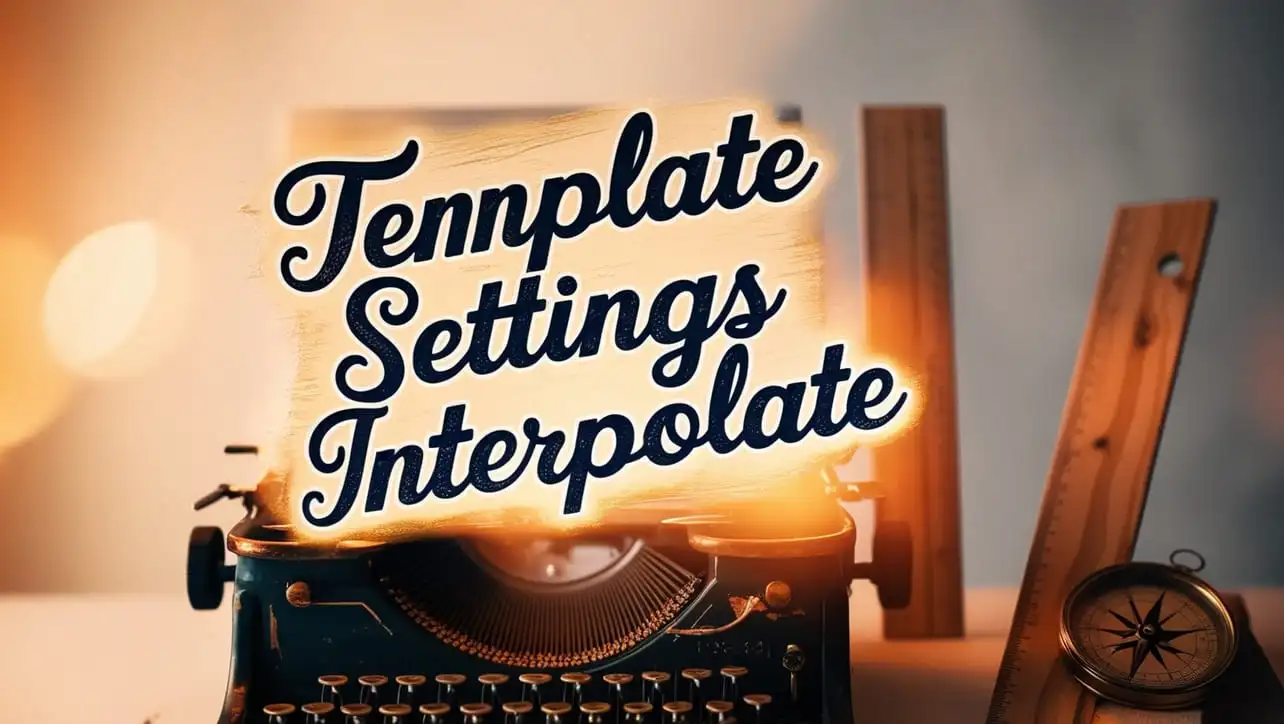
Lodash _.templateSettings.interpolate Property
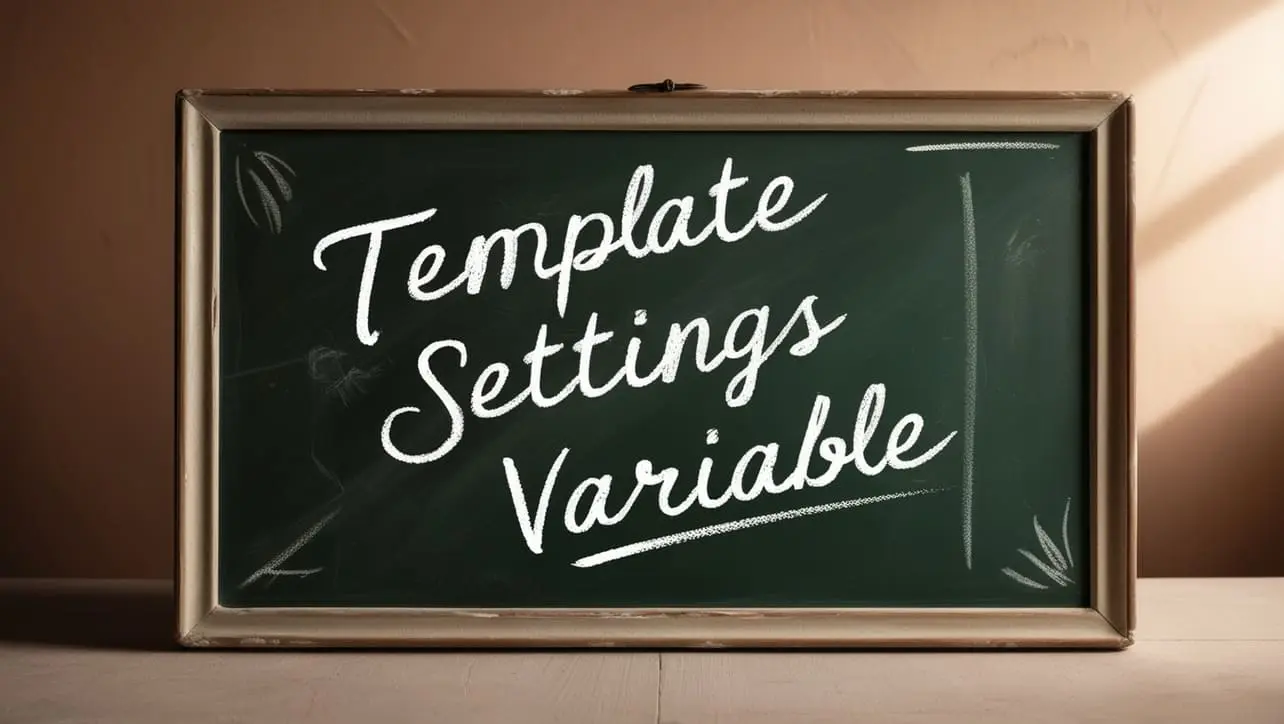
If you have any doubts regarding this article (Lodash _.invertBy() Object Method), please comment here. I will help you immediately.