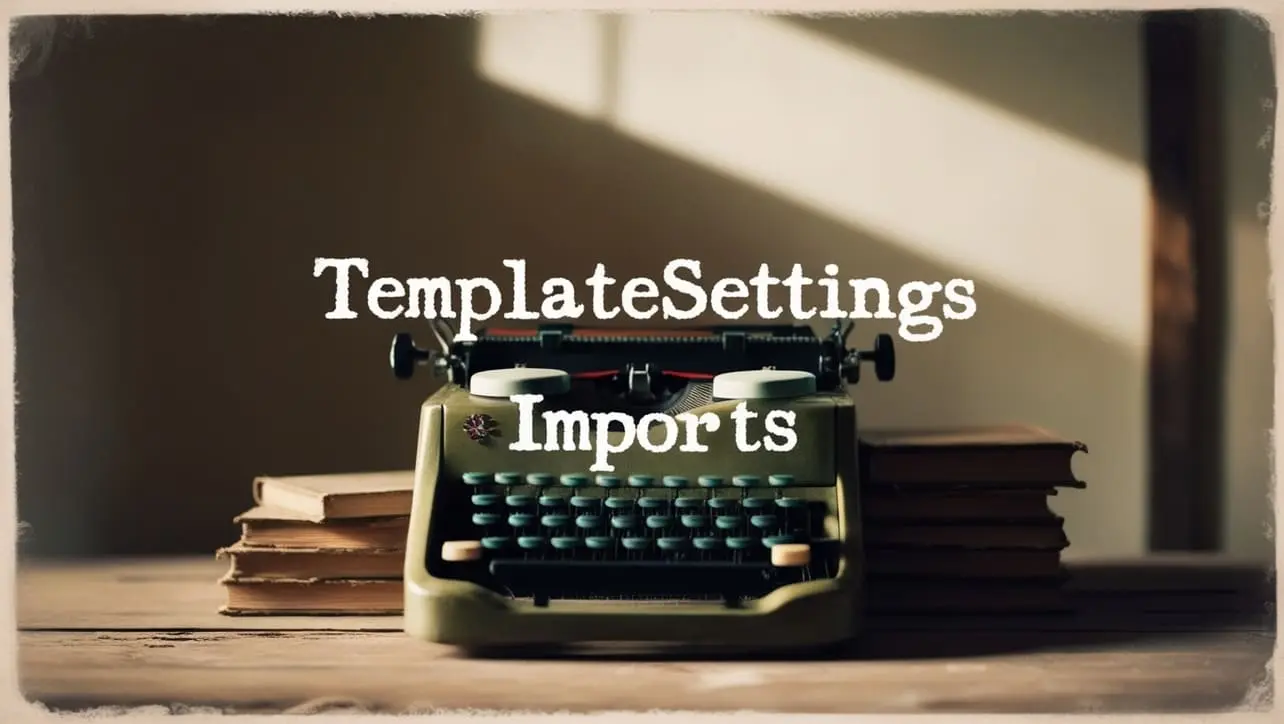
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.defaultsDeep() Object Method
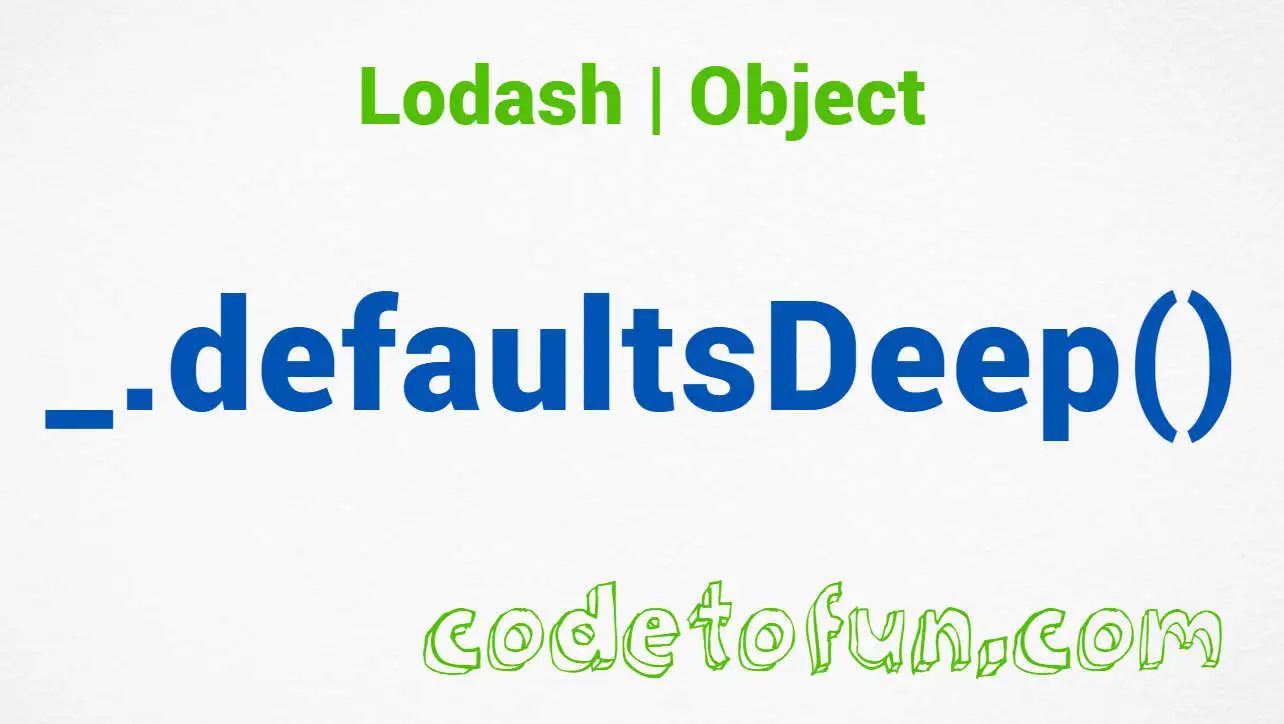
Photo Credit to CodeToFun
🙋 Introduction
In the vast landscape of JavaScript development, managing and configuring objects is a common task. Lodash, a feature-rich utility library, offers a plethora of functions to simplify these operations. Among these functions is the _.defaultsDeep()
method, a powerful tool for merging objects deeply, providing default values for undefined properties.
This method is invaluable for ensuring configuration objects have the necessary settings while preserving existing values.
🧠 Understanding _.defaultsDeep() Method
The _.defaultsDeep()
method in Lodash facilitates the deep merging of multiple objects, where properties with undefined values are populated with default values from subsequent objects. This ensures that existing properties are retained while incorporating missing or undefined properties from other objects.
💡 Syntax
The syntax for the _.defaultsDeep()
method is straightforward:
_.defaultsDeep(object, ...sources)
- object: The destination object.
- sources: The source objects to merge into the destination object.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.defaultsDeep()
method:
const _ = require('lodash');
const config = {
server: {
host: 'localhost',
port: 3000,
},
database: {
username: 'admin',
password: 'admin123',
},
};
const customConfig = {
server: {
port: 5000,
},
database: {
databaseName: 'mydatabase',
},
};
const mergedConfig = _.defaultsDeep(config, customConfig);
console.log(mergedConfig);
In this example, config and customConfig are merged using _.defaultsDeep()
, creating a new object where missing properties in config are populated with values from customConfig.
🏆 Best Practices
When working with the _.defaultsDeep()
method, consider the following best practices:
Understanding Deep Merging:
_.defaultsDeep()
excels at deep merging, preserving nested structures. Understand that it operates deeply within objects, ensuring that nested properties are merged without losing the existing structure.example.jsCopiedconst originalObject = { a: { b: { c: 1 } } }; const newValues = { a: { b: { d: 2 } } }; const mergedObject = _.defaultsDeep(originalObject, newValues); console.log(mergedObject); // Output: { a: { b: { c: 1, d: 2 } } }
Handling Arrays:
When working with arrays, be aware that
_.defaultsDeep()
treats arrays as values rather than merging them. If array merging is required, consider implementing custom logic based on your specific use case.example.jsCopiedconst originalObject = { arrayProp: [1, 2, 3] }; const newValues = { arrayProp: [4, 5] }; const mergedObject = _.defaultsDeep(originalObject, newValues); console.log(mergedObject); // Output: { arrayProp: [1, 2, 3] }
Use Cases with Nested Configurations:
_.defaultsDeep()
is particularly useful when dealing with configuration objects. It allows you to provide default configuration settings while allowing users to customize specific values.example.jsCopiedconst defaultConfig = { theme: 'light', font: { size: 14, family: 'Arial', }, }; const userConfig = { theme: 'dark', font: { size: 16, }, }; const finalConfig = _.defaultsDeep(defaultConfig, userConfig); console.log(finalConfig); // Output: { theme: 'dark', font: { size: 16, family: 'Arial' } }
📚 Use Cases
Configuration Objects:
_.defaultsDeep()
is a powerful tool for managing configuration objects, allowing developers to provide default settings while enabling users to customize specific values.example.jsCopiedconst defaultConfig = { apiUrl: 'https://api.example.com', timeout: 5000, headers: { Authorization: 'Bearer token', 'Content-Type': 'application/json', }, }; const userConfig = { timeout: 8000, headers: { 'Content-Type': 'application/xml', }, }; const mergedConfig = _.defaultsDeep(defaultConfig, userConfig); console.log(mergedConfig);
Merging Nested Data:
When working with deeply nested data structures,
_.defaultsDeep()
simplifies the process of merging multiple objects, ensuring that missing or undefined properties are populated with default values.example.jsCopiedconst defaultData = { user: { name: 'Guest', preferences: { theme: 'light', language: 'en', }, }, }; const customData = { user: { preferences: { theme: 'dark', }, }, }; const mergedData = _.defaultsDeep(defaultData, customData); console.log(mergedData);
Preserving Existing Values:
_.defaultsDeep()
excels at preserving existing values while incorporating defaults. This is particularly beneficial when updating application settings without losing user-specific configurations.example.jsCopiedconst currentSettings = { colors: { background: 'white', text: 'black', }, fontSize: 16, }; const defaultSettings = { colors: { background: 'lightgray', }, fontFamily: 'Arial', }; const updatedSettings = _.defaultsDeep(currentSettings, defaultSettings); console.log(updatedSettings);
🎉 Conclusion
The _.defaultsDeep()
method in Lodash provides an elegant solution for merging objects deeply, offering default values for undefined properties. Whether you're managing configuration objects, merging nested data, or preserving existing values, this method proves to be a versatile tool in the JavaScript developer's toolkit.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.defaultsDeep()
method in your Lodash projects.
👨💻 Join our Community:
Author
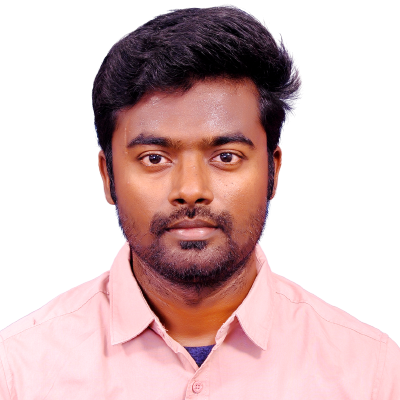
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
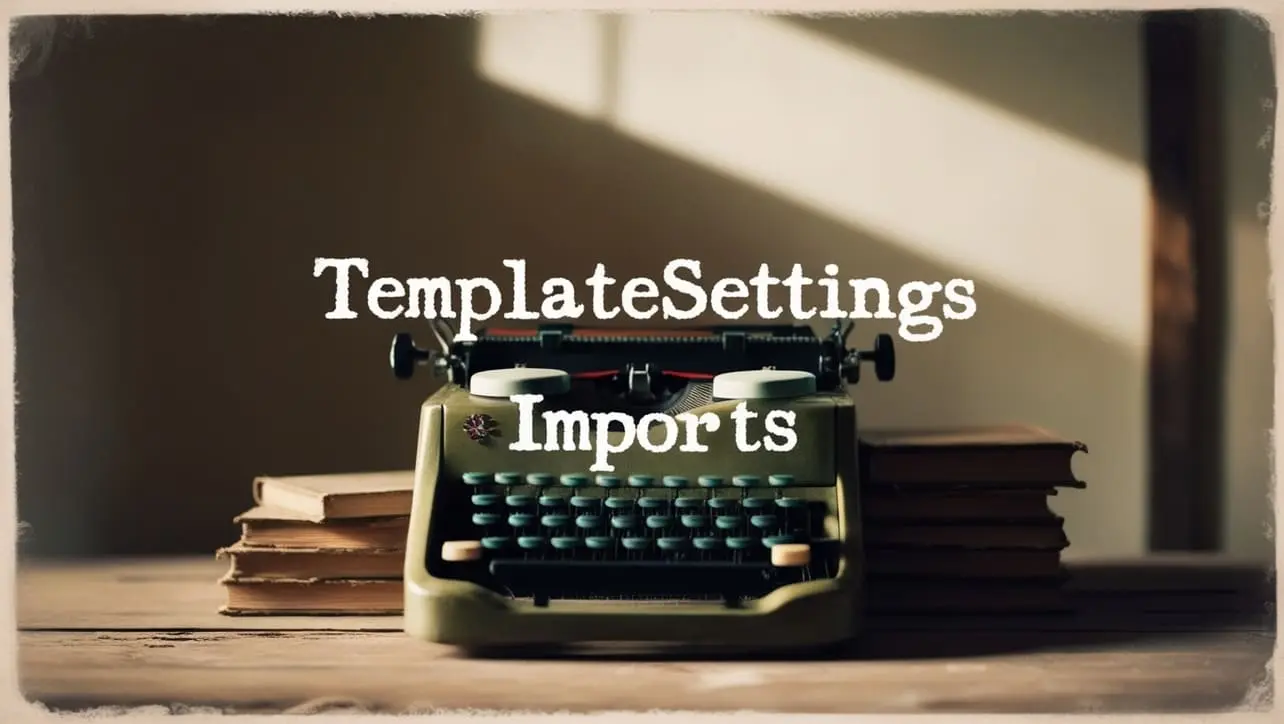
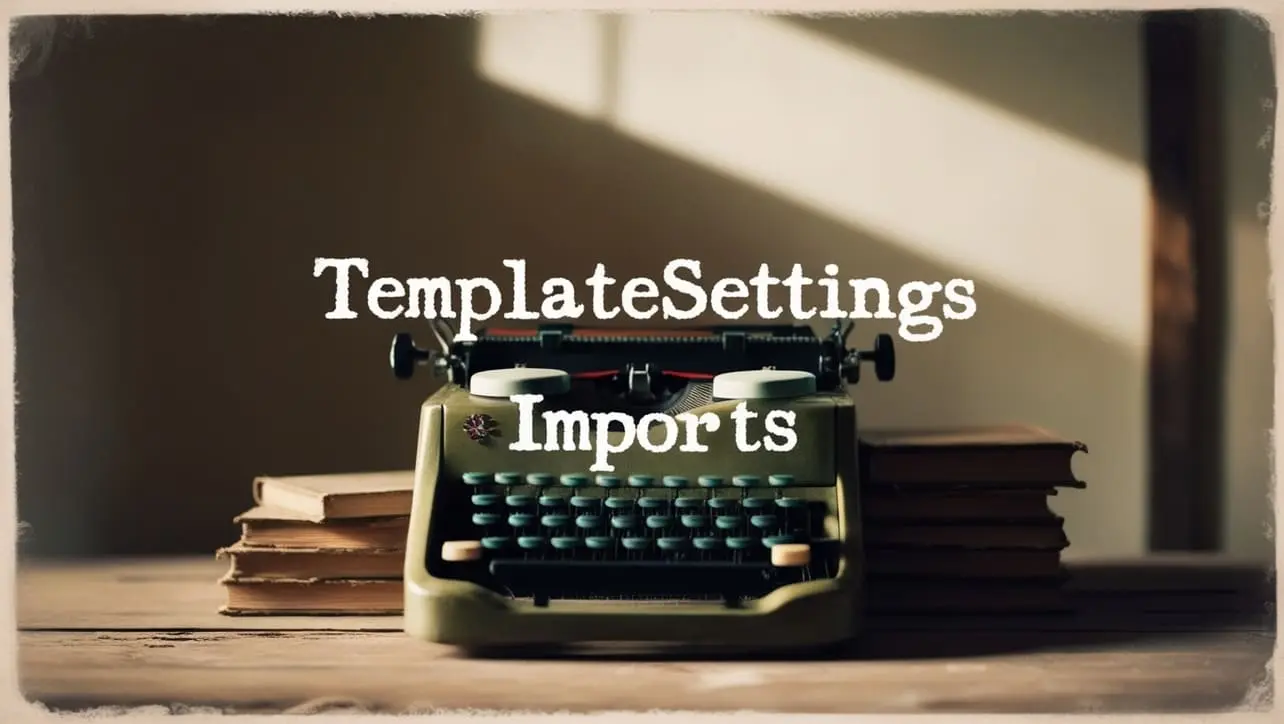
Lodash _.templateSettings.imports Property
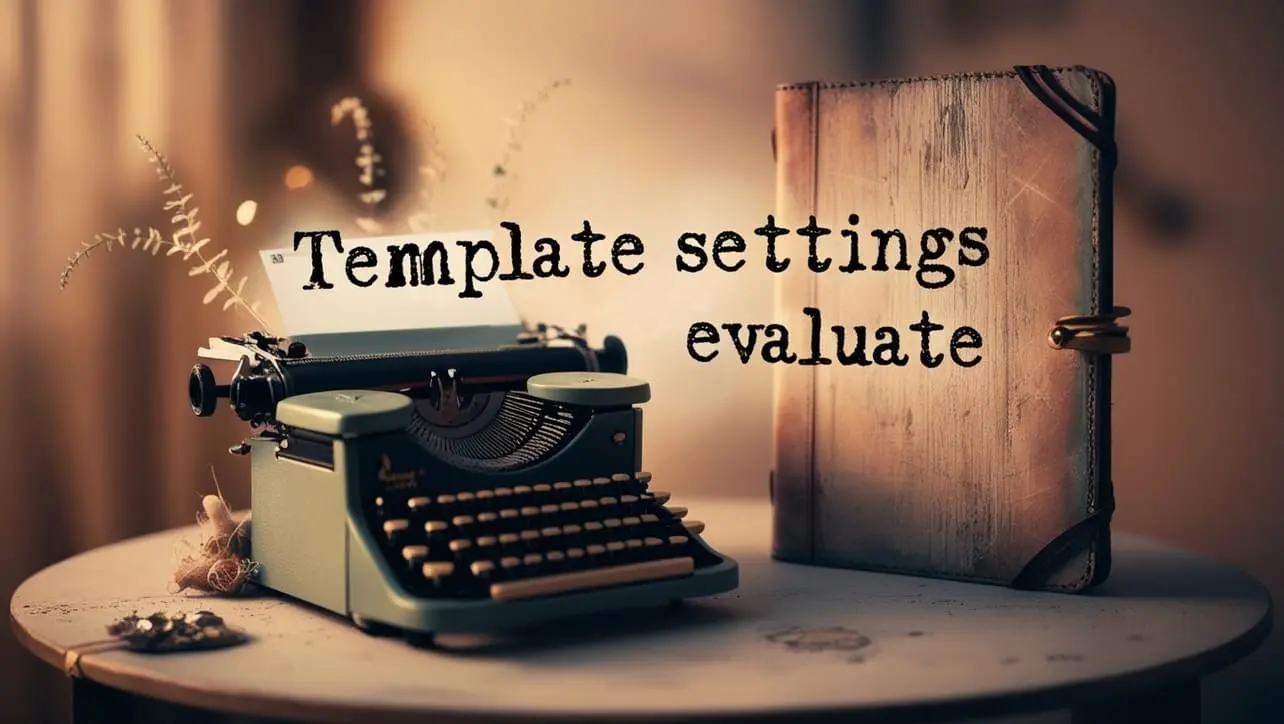
Lodash _.templateSettings.evaluate Property
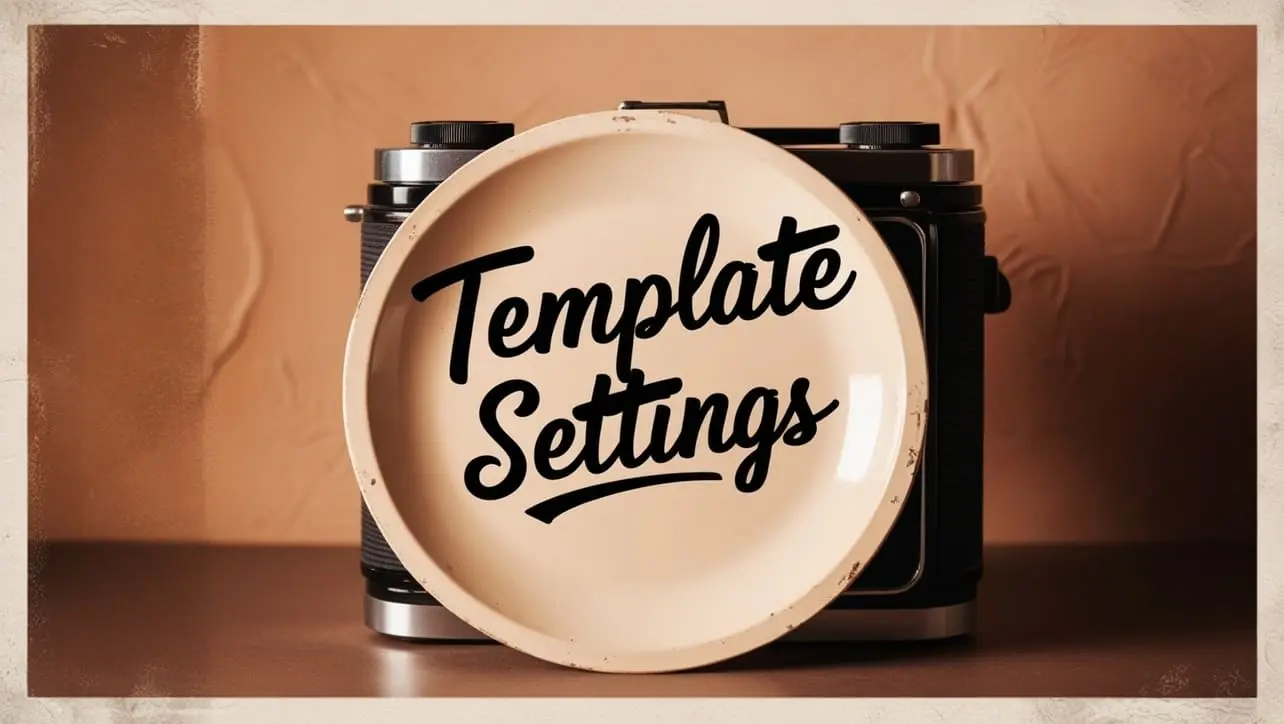
Lodash _.templateSettings Property
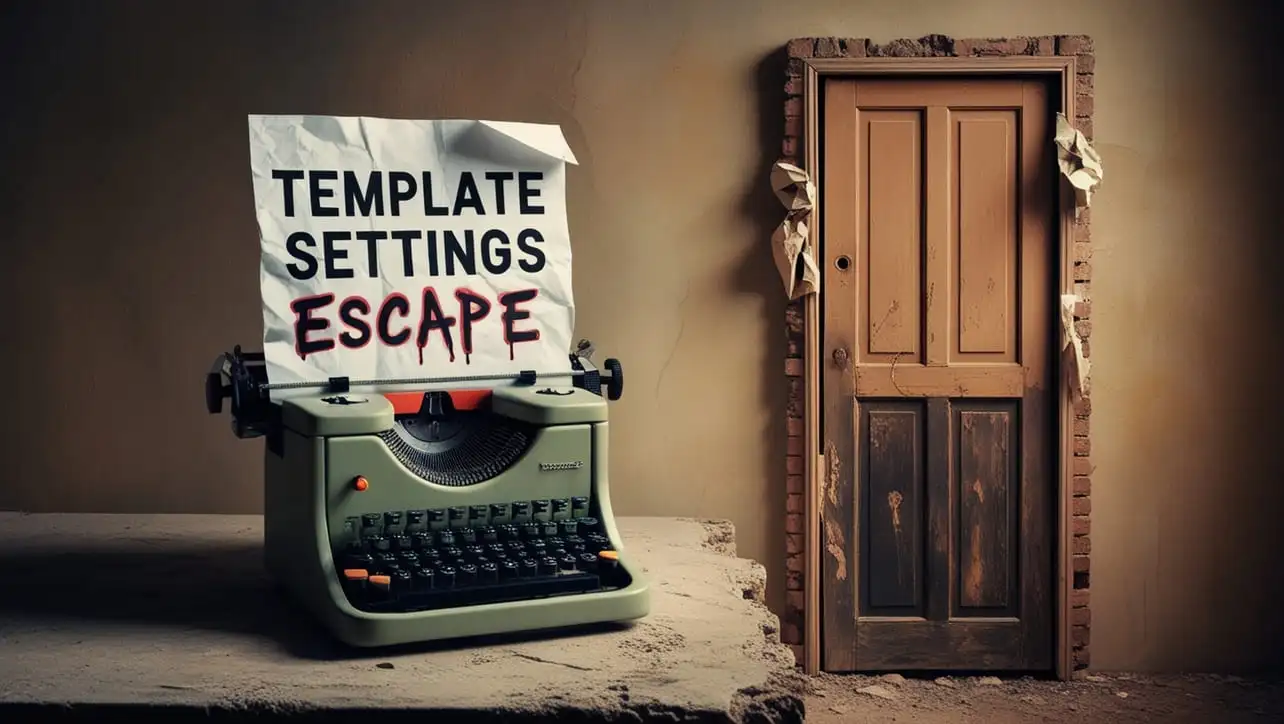
Lodash _.templateSettings.escape Property
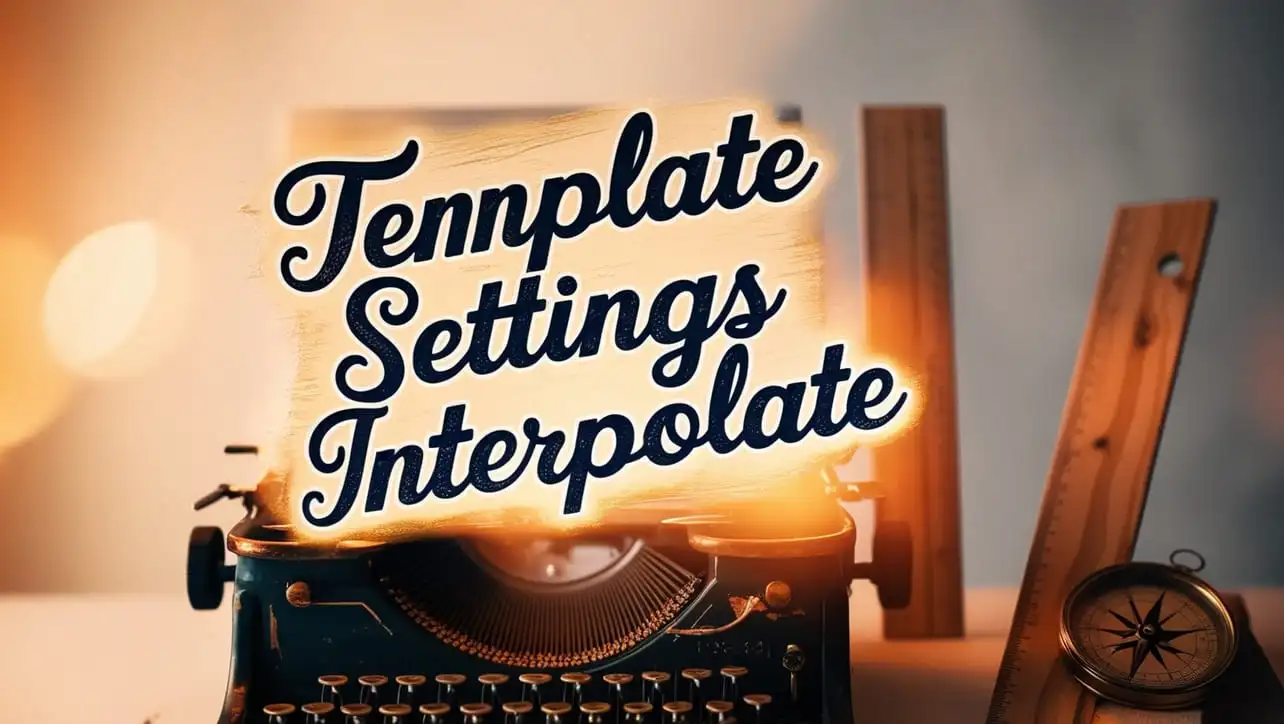
Lodash _.templateSettings.interpolate Property
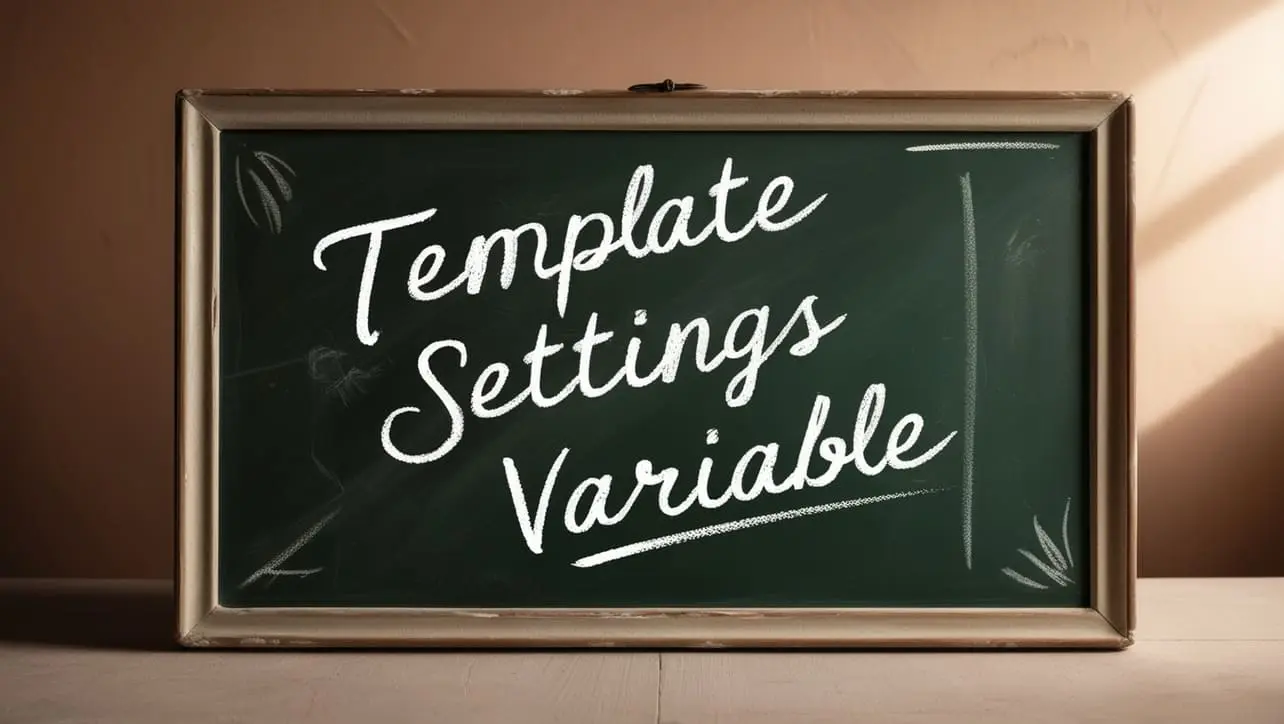
If you have any doubts regarding this article (Lodash _.defaultsDeep() Object Method), please comment here. I will help you immediately.