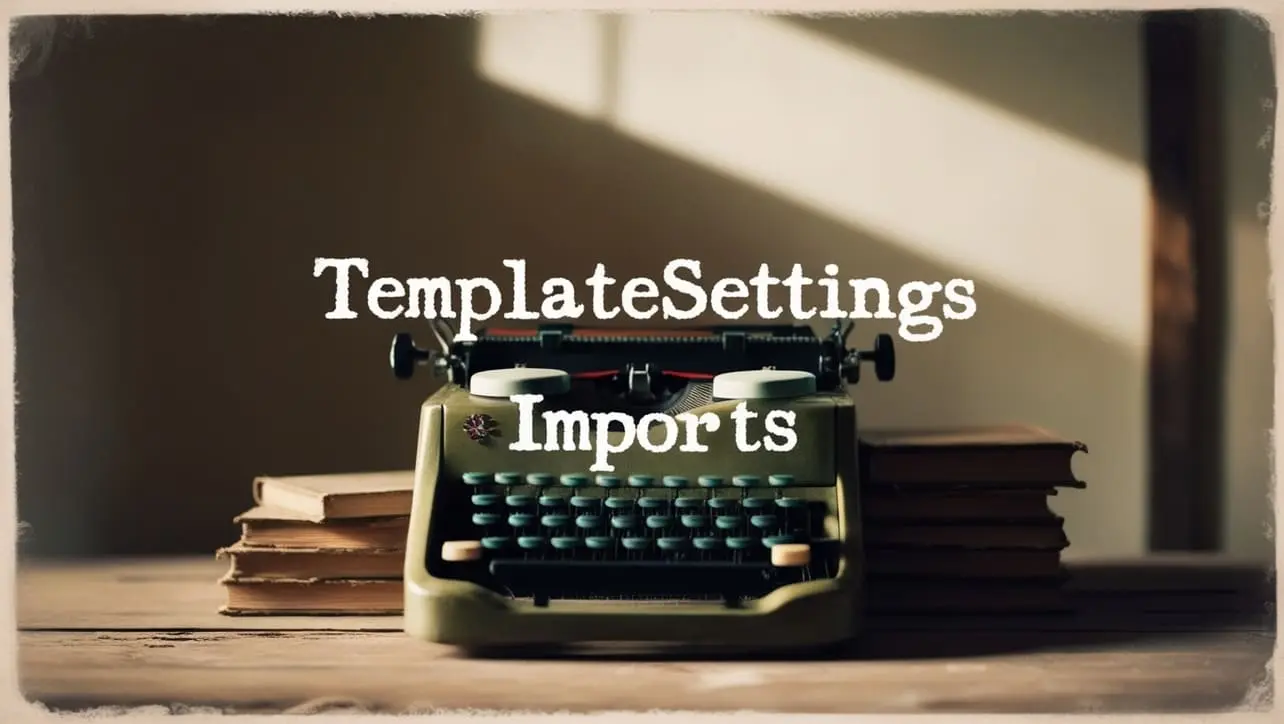
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.defaults() Object Method
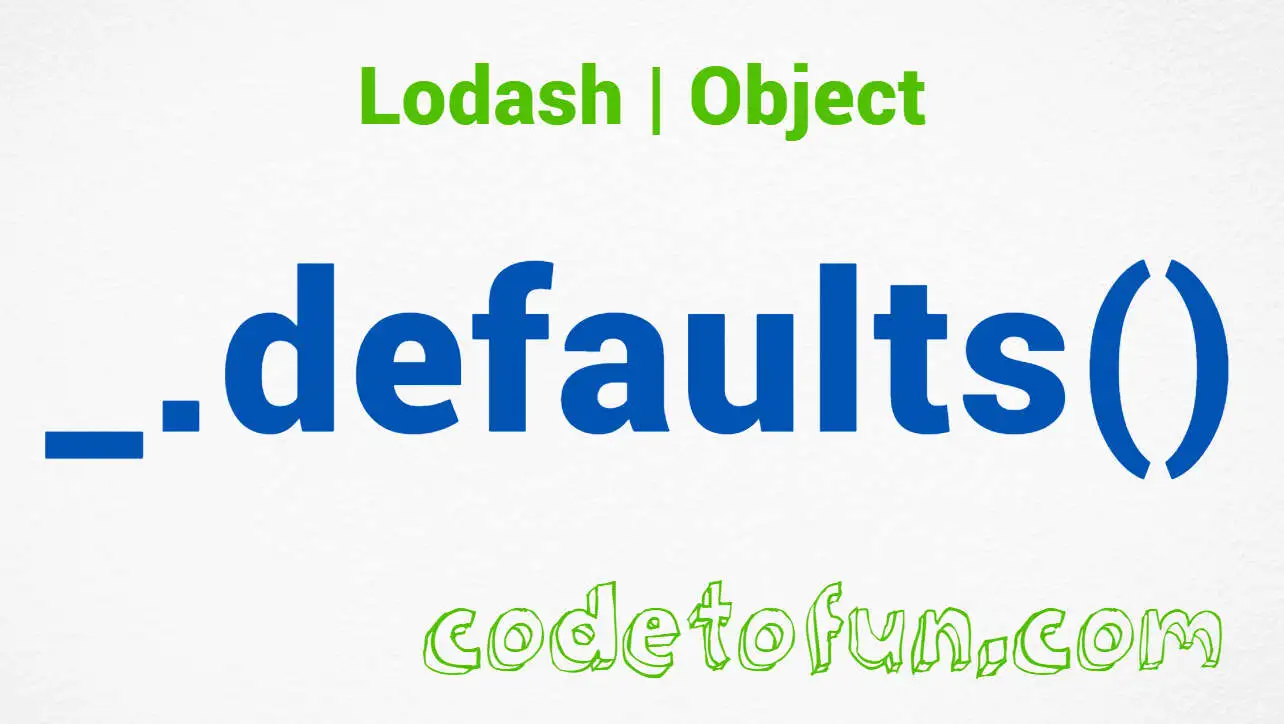
Photo Credit to CodeToFun
🙋 Introduction
In the landscape of JavaScript development, effective handling of objects is fundamental. Lodash, a powerful utility library, offers a range of functions to streamline object manipulation. Among these functions is the _.defaults()
method, a versatile tool for merging default values into an object.
This method enhances code flexibility and maintainability, making it an invaluable asset for developers dealing with complex object structures.
🧠 Understanding _.defaults() Method
The _.defaults()
method in Lodash is designed to populate undefined properties in an object with corresponding values from a defaults object. This ensures that the target object contains all the necessary properties, with the option to provide default values for missing ones.
💡 Syntax
The syntax for the _.defaults()
method is straightforward:
_.defaults(object, [defaults])
- object: The target object to populate.
- defaults: The object with default values.
📝 Example
Let's dive into a simple example to illustrate the usage of the _.defaults()
method:
const _ = require('lodash');
const targetObject = { a: 1, b: 2 };
const defaultsObject = { b: 3, c: 4 };
const mergedObject = _.defaults(targetObject, defaultsObject);
console.log(mergedObject);
// Output: { a: 1, b: 2, c: 4 }
In this example, the targetObject is merged with defaultsObject using _.defaults()
, resulting in a new object with default values applied to undefined properties.
🏆 Best Practices
When working with the _.defaults()
method, consider the following best practices:
Understand Object Structure:
Before applying
_.defaults()
, have a clear understanding of the structure of both the target object and the defaults object. This ensures accurate merging of properties.example.jsCopiedconst userSettings = { username: 'JohnDoe', theme: 'light' }; const defaultSettings = { theme: 'dark', language: 'en' }; const mergedSettings = _.defaults(userSettings, defaultSettings); console.log(mergedSettings); // Output: { username: 'JohnDoe', theme: 'light', language: 'en' }
Avoid Mutating Original Object:
If you want to keep the original object unaltered, create a new object and apply
_.defaults()
to it. This helps maintain immutability in your code.example.jsCopiedconst originalObject = { key1: 'value1' }; const defaults = { key2: 'value2' }; const mergedObject = _.defaults({}, originalObject, defaults); console.log(mergedObject); // Output: { key1: 'value1', key2: 'value2' } console.log(originalObject); // Output: { key1: 'value1' }
Combine with ES6 Spread Operator:
For a more modern approach, consider using the ES6 spread operator to achieve a similar result. This provides a concise syntax for merging objects.
example.jsCopiedconst targetObject = { a: 1, b: 2 }; const defaultsObject = { b: 3, c: 4 }; const mergedObject = { ...defaultsObject, ...targetObject }; console.log(mergedObject); // Output: { a: 1, b: 2, c: 4 }
📚 Use Cases
Configurable Options:
_.defaults()
is particularly useful when working with configurable options. It allows developers to set default values for various options while providing users the flexibility to customize settings.example.jsCopiedconst userOptions = { theme: 'dark', fontSize: 16 }; const defaultOptions = { theme: 'light', fontSize: 14, language: 'en' }; const finalOptions = _.defaults(userOptions, defaultOptions); console.log(finalOptions); // Output: { theme: 'dark', fontSize: 16, language: 'en' }
Merging User Preferences:
When dealing with user preferences or settings,
_.defaults()
simplifies the process of merging user-provided values with default configurations.example.jsCopiedconst userPreferences = { showNotifications: true, darkMode: true }; const defaultPreferences = { showNotifications: false, darkMode: false, fontSize: 14 }; const mergedPreferences = _.defaults(userPreferences, defaultPreferences); console.log(mergedPreferences); // Output: { showNotifications: true, darkMode: true, fontSize: 14 }
API Request Configuration:
In scenarios where you need to configure API requests,
_.defaults()
can be used to merge user-defined headers or parameters with default values.example.jsCopiedconst userHeaders = { Authorization: 'Bearer Token123' }; const defaultHeaders = { ContentType: 'application/json', Timeout: 5000 }; const mergedHeaders = _.defaults(userHeaders, defaultHeaders); console.log(mergedHeaders); // Output: { Authorization: 'Bearer Token123', ContentType: 'application/json', Timeout: 5000 }
🎉 Conclusion
The _.defaults()
method in Lodash provides an efficient way to merge default values into objects, offering enhanced flexibility and maintainability in your JavaScript code. Whether you're configuring options, managing preferences, or handling API requests, _.defaults()
is a valuable tool for ensuring that your objects have the necessary properties with sensible default values.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.defaults()
method in your Lodash projects.
👨💻 Join our Community:
Author
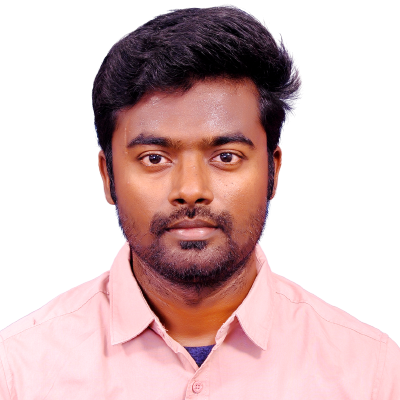
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
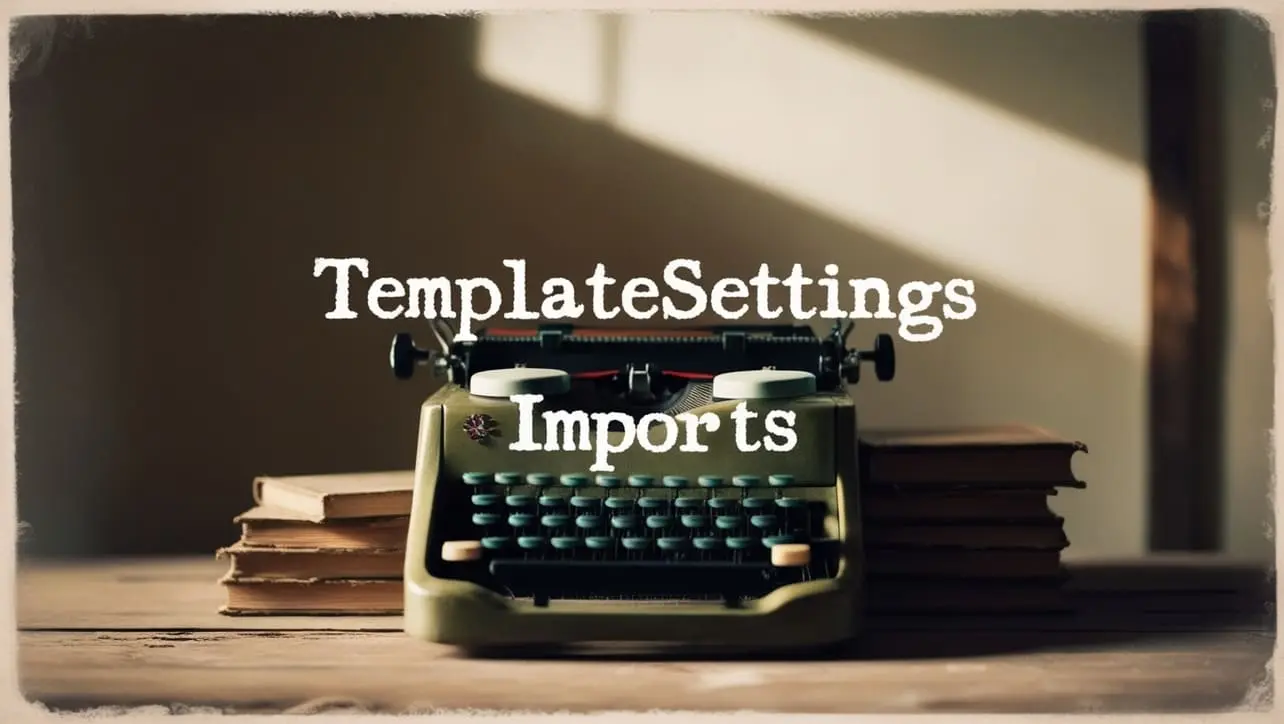
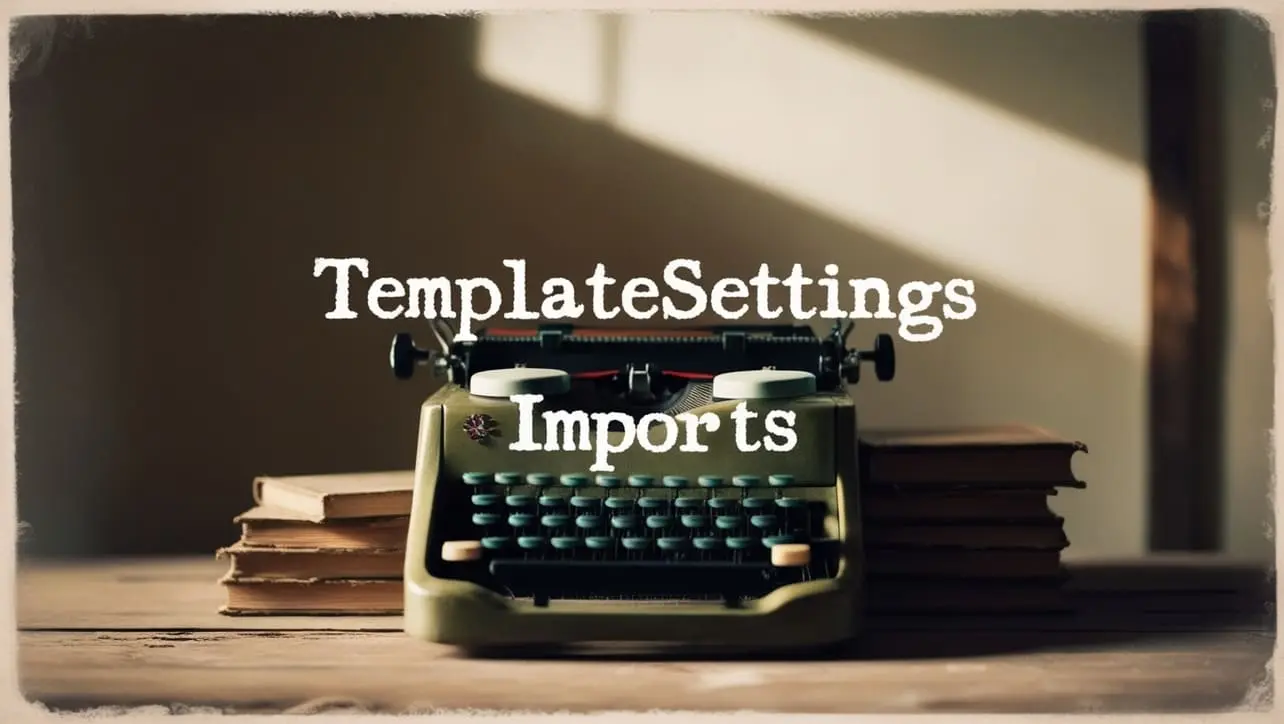
Lodash _.templateSettings.imports Property
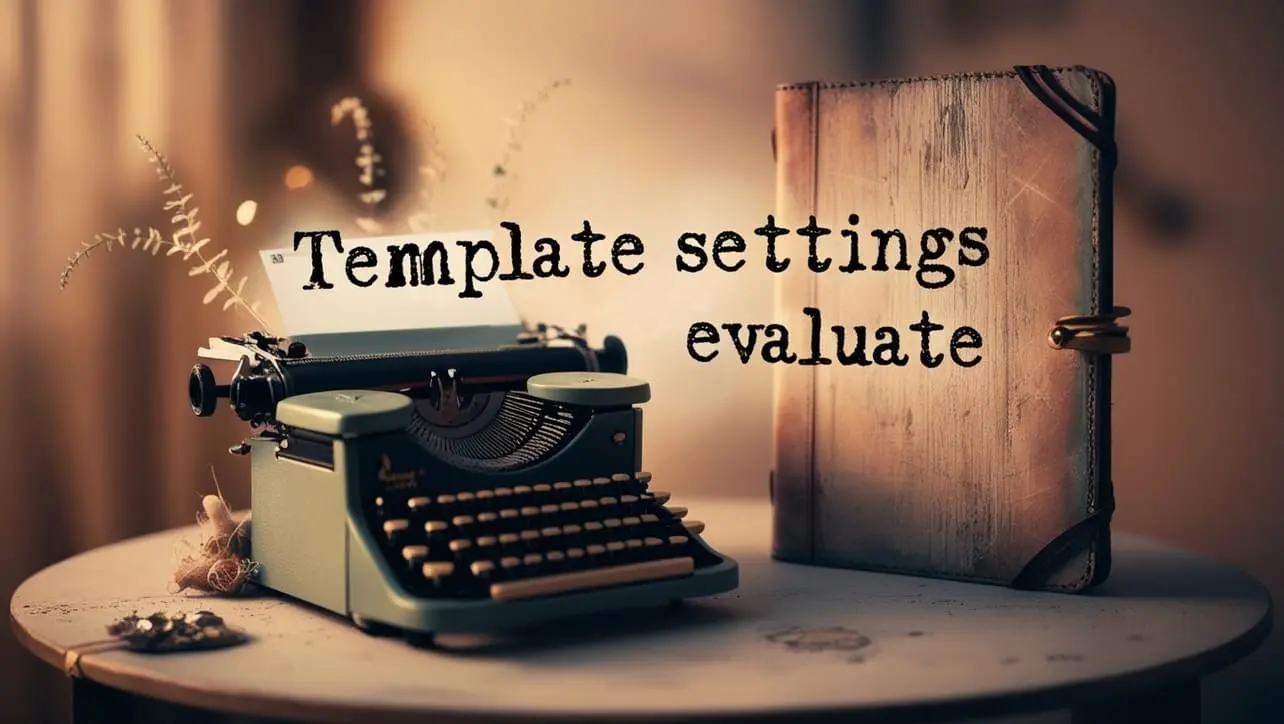
Lodash _.templateSettings.evaluate Property
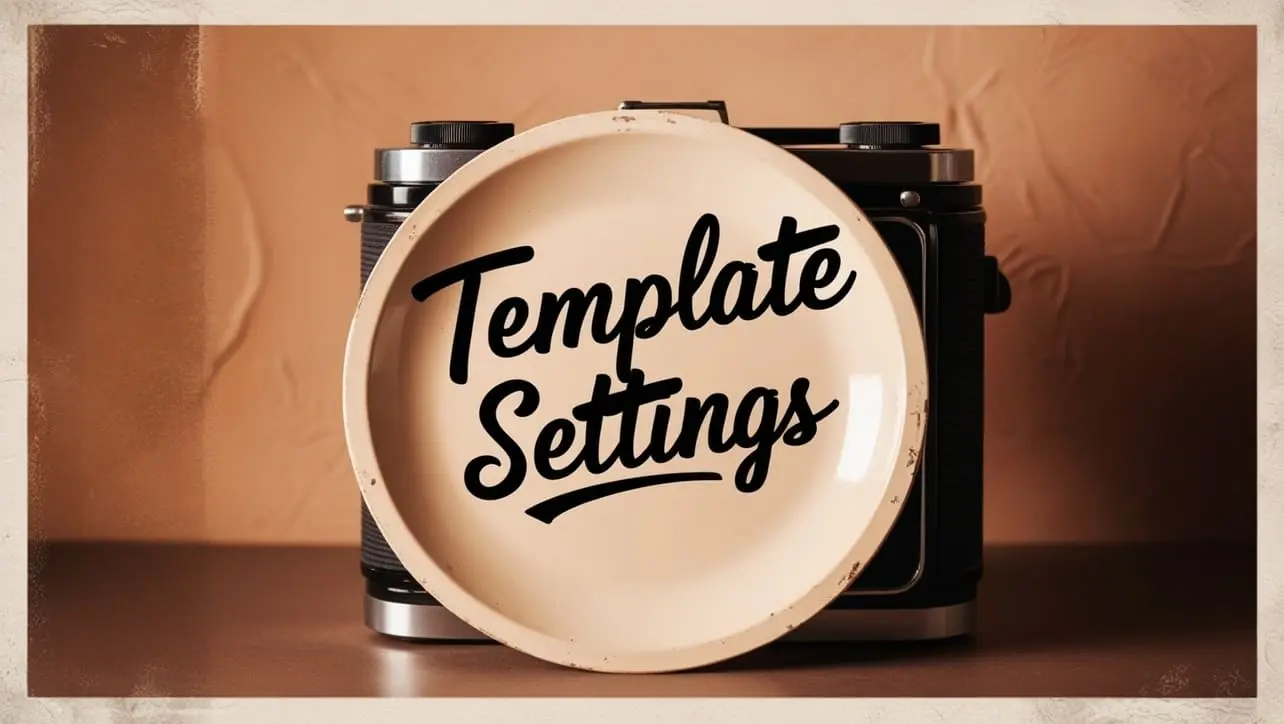
Lodash _.templateSettings Property
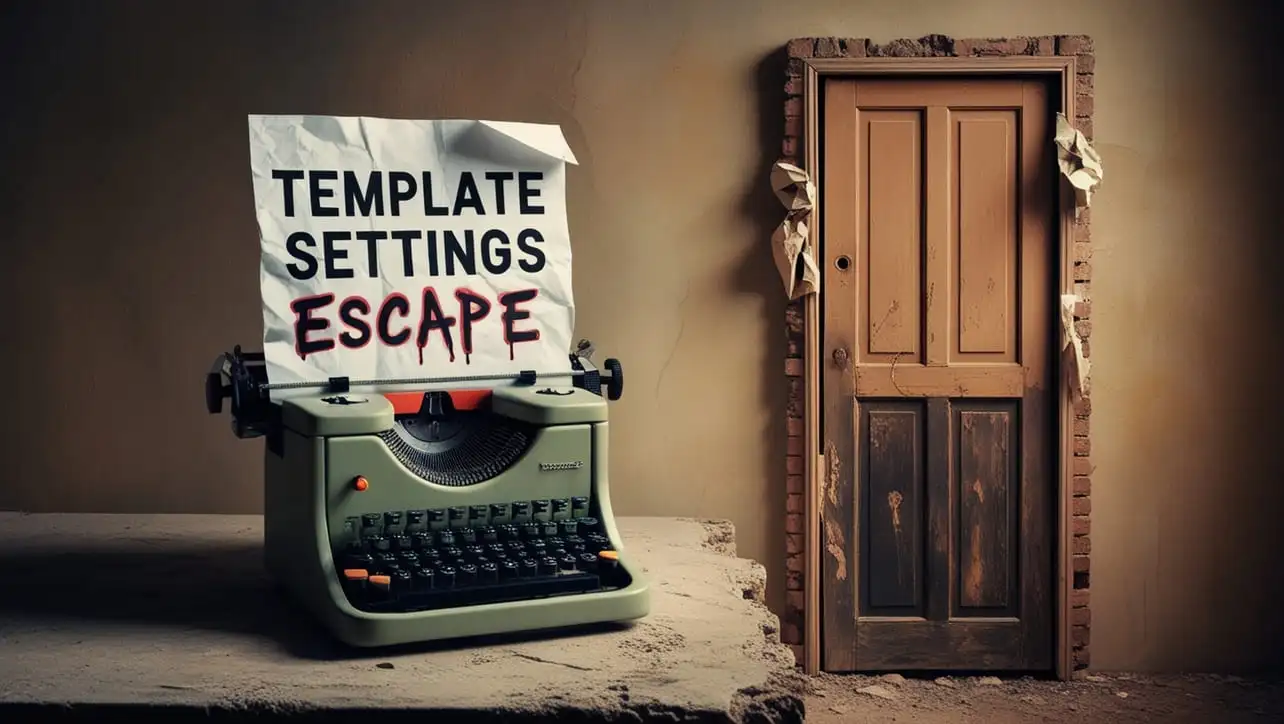
Lodash _.templateSettings.escape Property
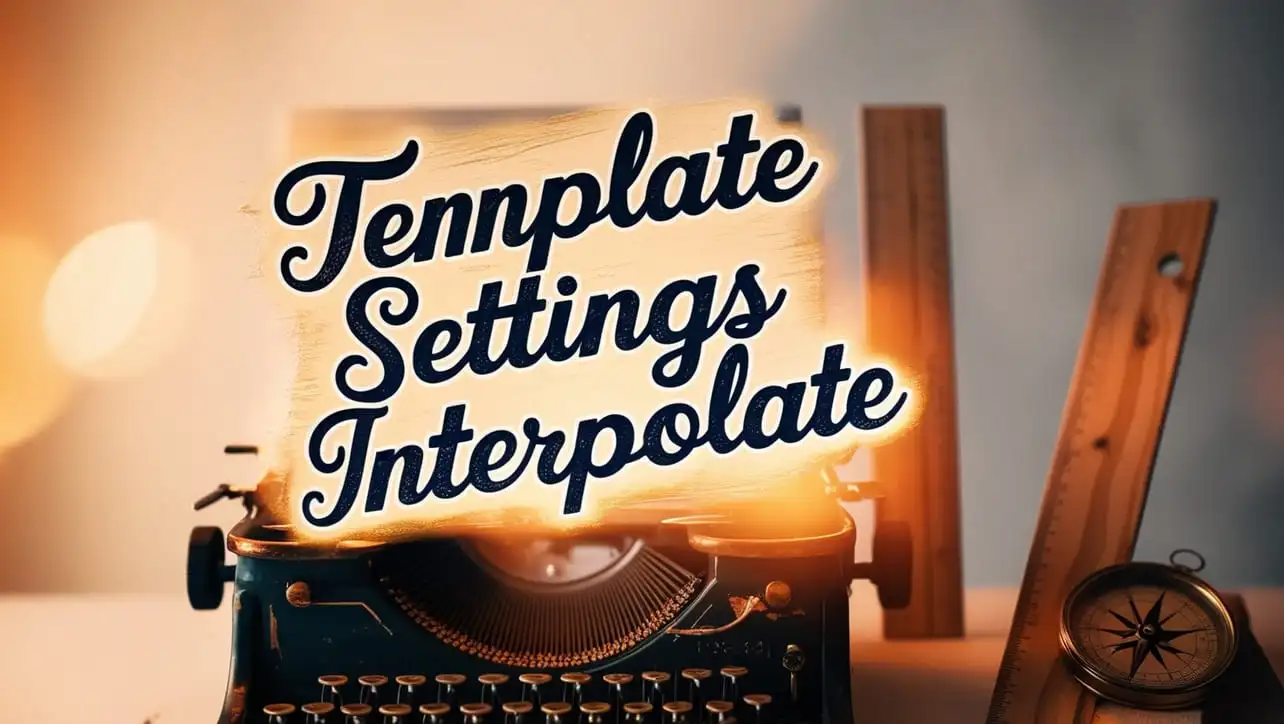
Lodash _.templateSettings.interpolate Property
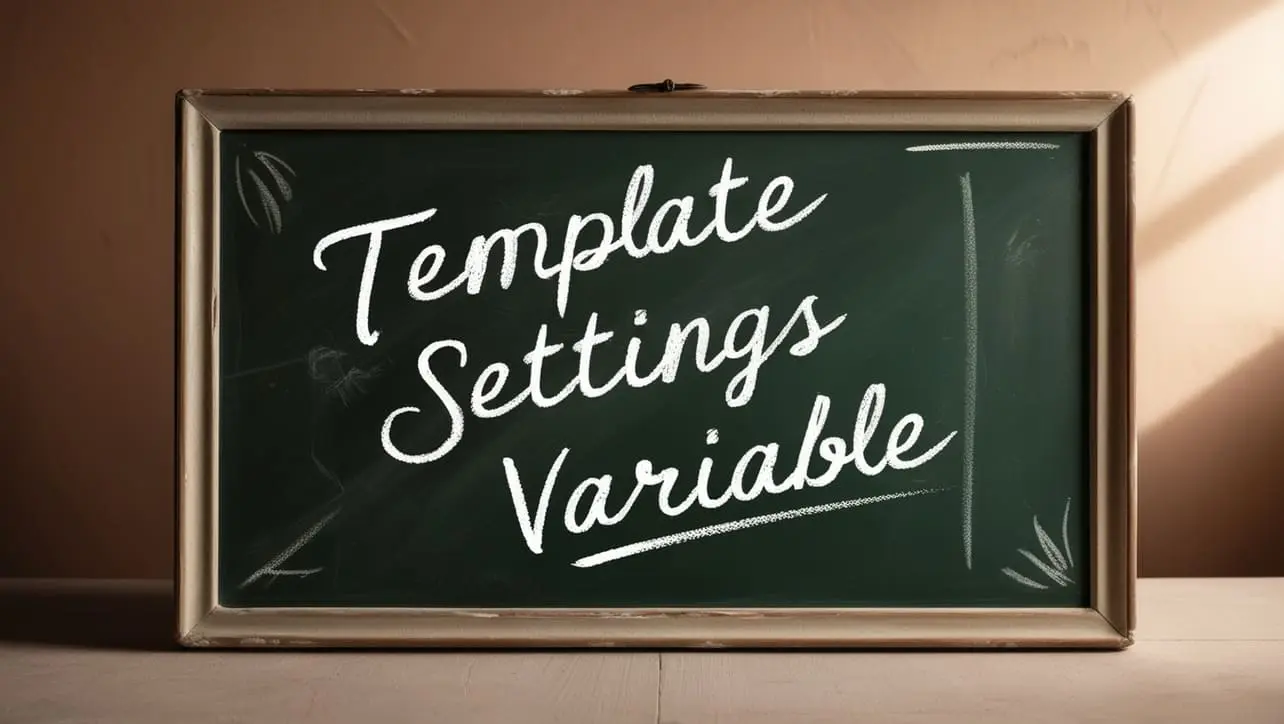
If you have any doubts regarding this article (Lodash _.defaults() Object Method), please comment here. I will help you immediately.