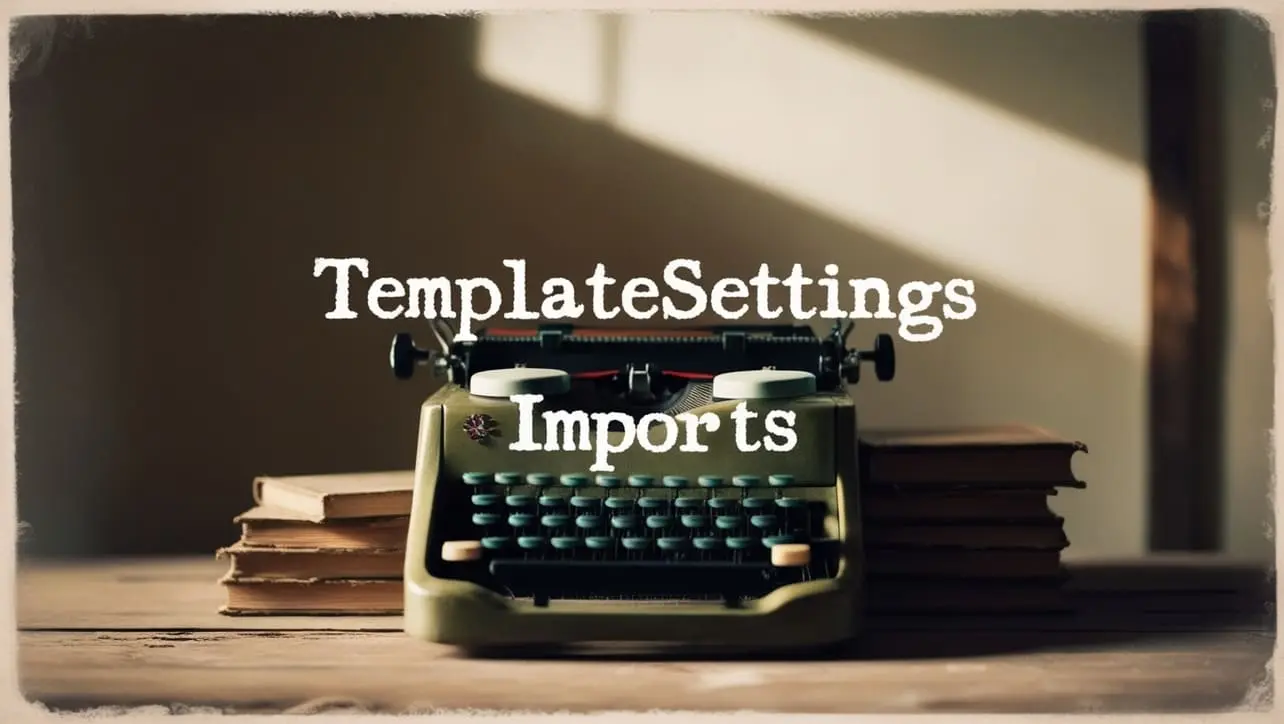
Lodash Home
- Lodash Intro
- Lodash Array
- Lodash Collection
- Lodash Date
- Lodash Function
- Lodash Lang
- Lodash Math
- Lodash Number
- Lodash Object
- _.assign
- _.assignIn
- _.assignInWith
- _.assignWith
- _.at
- _.create
- _.defaults
- _.defaultsDeep
- _.findKey
- _.findLastKey
- _.forIn
- _.forInRight
- _.forOwn
- _.forOwnRight
- _.functions
- _.functionsIn
- _.get
- _.has
- _.hasIn
- _.invert
- _.invertBy
- _.invoke
- _.keys
- _.keysIn
- _.mapKeys
- _.mapValues
- _.merge
- _.mergeWith
- _.omit
- _.omitBy
- _.pick
- _.pickBy
- _.result
- _.set
- _.setWith
- _.toPairs
- _.toPairsIn
- _.transform
- _.unset
- _.update
- _.updateWith
- _.values
- _.valuesIn
- Lodash Seq
- Lodash String
- Lodash Util
- Lodash Properties
- Lodash Methods
Lodash _.updateWith() Object Method
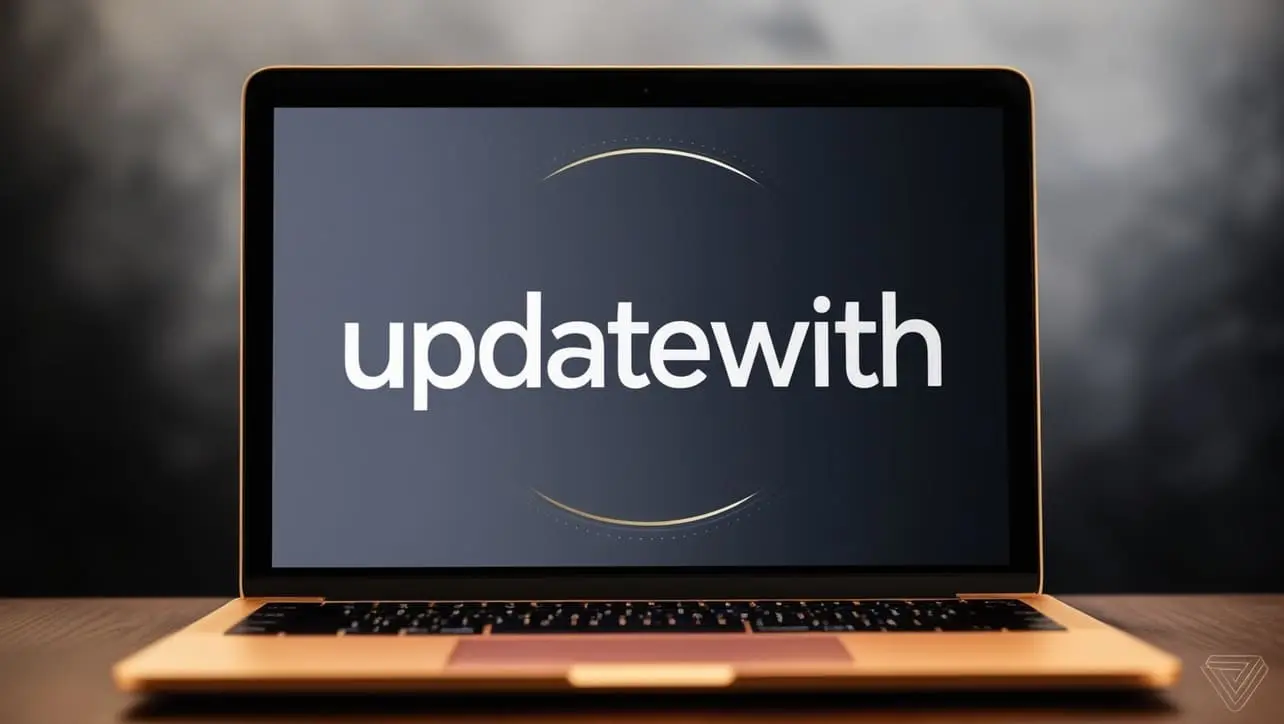
Photo Credit to CodeToFun
🙋 Introduction
In JavaScript development, managing complex nested objects often requires careful handling to ensure data integrity and maintainability. Lodash, a popular utility library, offers a wealth of functions to streamline object manipulation tasks. Among these functions is _.updateWith()
, a versatile method designed to facilitate deep updates within nested objects.
This method empowers developers to modify object properties with ease, enhancing code flexibility and readability.
🧠 Understanding _.updateWith() Method
The _.updateWith()
method in Lodash provides a powerful mechanism for updating nested object properties while offering extensive customization options. Whether you need to modify existing values, insert new properties, or apply complex transformations, _.updateWith()
equips you with the tools to tackle a wide range of object manipulation scenarios.
💡 Syntax
The syntax for the _.updateWith()
method is straightforward:
_.updateWith(object, path, updater, [customizer])
- object: The object to modify.
- path: The path of the property to update.
- updater: The function used to produce the updated value.
- customizer (Optional): The function to customize assigned values
📝 Example
Let's dive into a simple example to illustrate the usage of the _.updateWith()
method:
const _ = require('lodash');
const nestedObject = {
user: {
name: 'John',
age: 30,
address: {
city: 'New York',
country: 'USA'
}
}
};
_.updateWith(nestedObject, 'user.age', value => value + 1);
console.log(nestedObject.user.age);
// Output: 31
In this example, the user.age property within the nestedObject is incremented by 1 using the _.updateWith()
method.
🏆 Best Practices
When working with the _.updateWith()
method, consider the following best practices:
Understanding Property Paths:
Ensure clarity and accuracy when specifying property paths. Precise path definitions are essential for targeting the intended properties within nested objects.
example.jsCopied_.updateWith(nestedObject, 'user.address.city', () => 'Los Angeles');
Customizing Update Logic:
Leverage the updater function to tailor update logic according to your specific requirements. This allows for dynamic and context-aware property modifications.
example.jsCopied_.updateWith(nestedObject, 'user.name', value => value.toUpperCase());
Handling Undefined Properties:
Consider handling cases where target properties might be undefined to prevent unexpected errors. Utilize customizers or conditional checks to ensure robustness in property updates.
example.jsCopied_.updateWith(nestedObject, 'user.phoneNumber', () => 'N/A', (objValue, srcValue) => { if (objValue === undefined) { return srcValue; } });
📚 Use Cases
Updating User Profiles:
_.updateWith()
can be used to update user profile information stored within nested objects. This includes modifying user details such as name, age, address, and other relevant data.example.jsCopied_.updateWith(userProfile, 'personalInfo.email', () => 'newemail@example.com');
Configuring Application Settings:
When managing application settings,
_.updateWith()
enables dynamic updates to configuration objects. This facilitates runtime adjustments to various parameters and preferences.example.jsCopied_.updateWith(appConfig, 'notifications.email.enabled', () => true);
Modifying Nested Data Structures:
For complex data structures like trees or graphs,
_.updateWith()
offers a convenient means of updating nested properties. This is particularly useful in scenarios involving hierarchical data representations.example.jsCopied_.updateWith(dataTree, 'nodes[0].children[2].value', () => 'newValue');
🎉 Conclusion
The _.updateWith()
method in Lodash empowers developers to perform deep updates within nested objects with ease and precision. By providing flexible customization options and robust handling of nested structures, _.updateWith()
facilitates efficient object manipulation in JavaScript applications.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the _.updateWith()
method in your Lodash projects.
👨💻 Join our Community:
Author
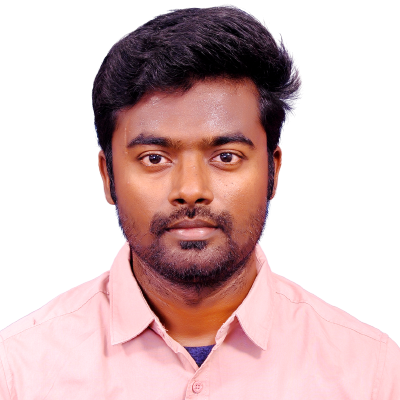
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in lodash
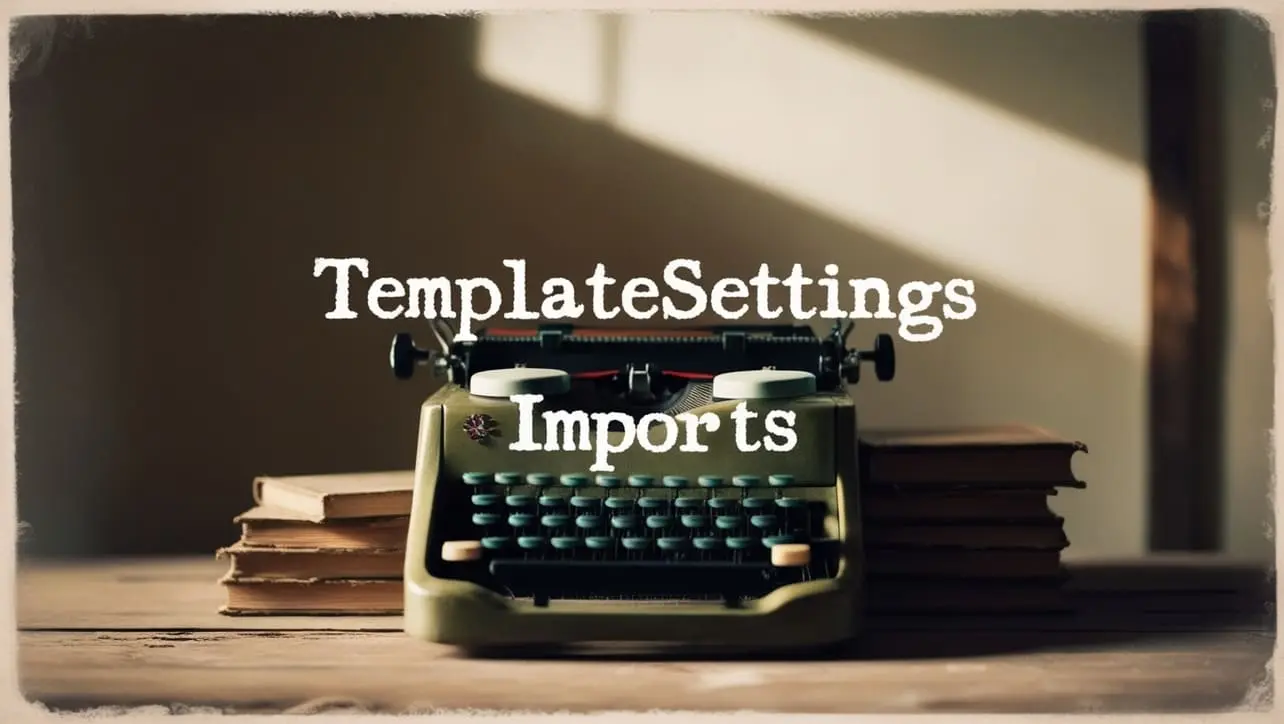
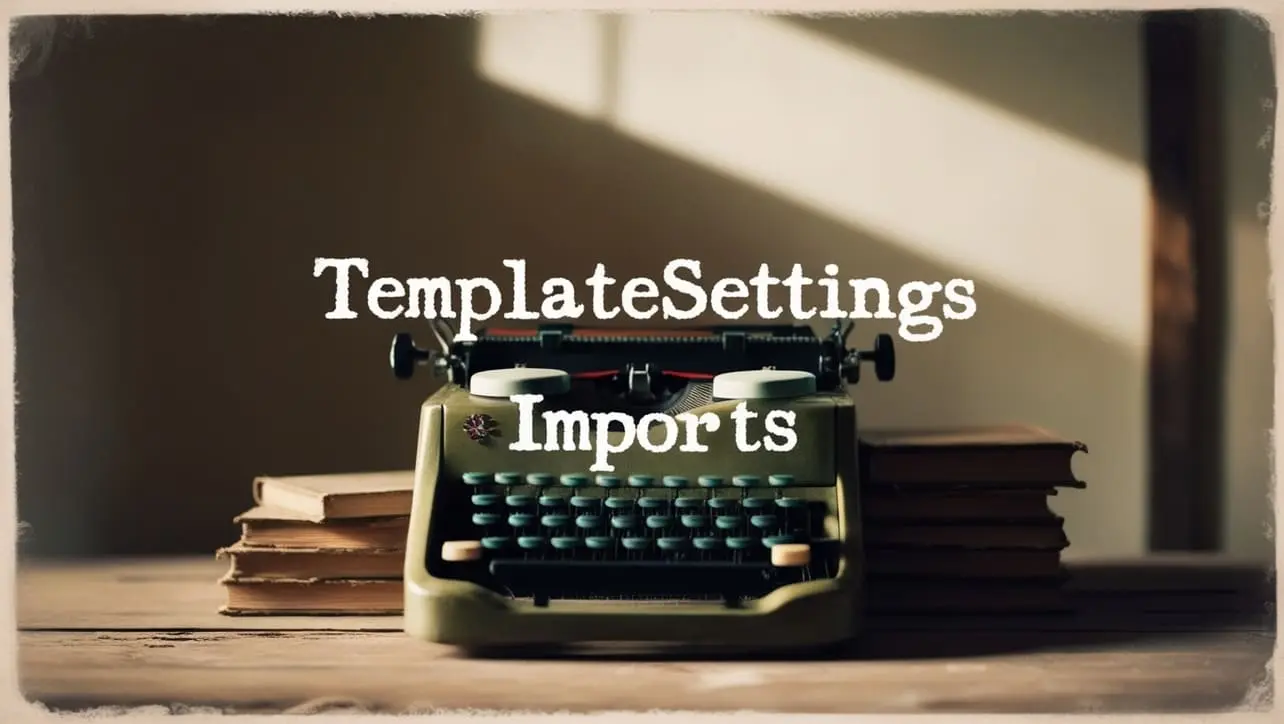
Lodash _.templateSettings.imports Property
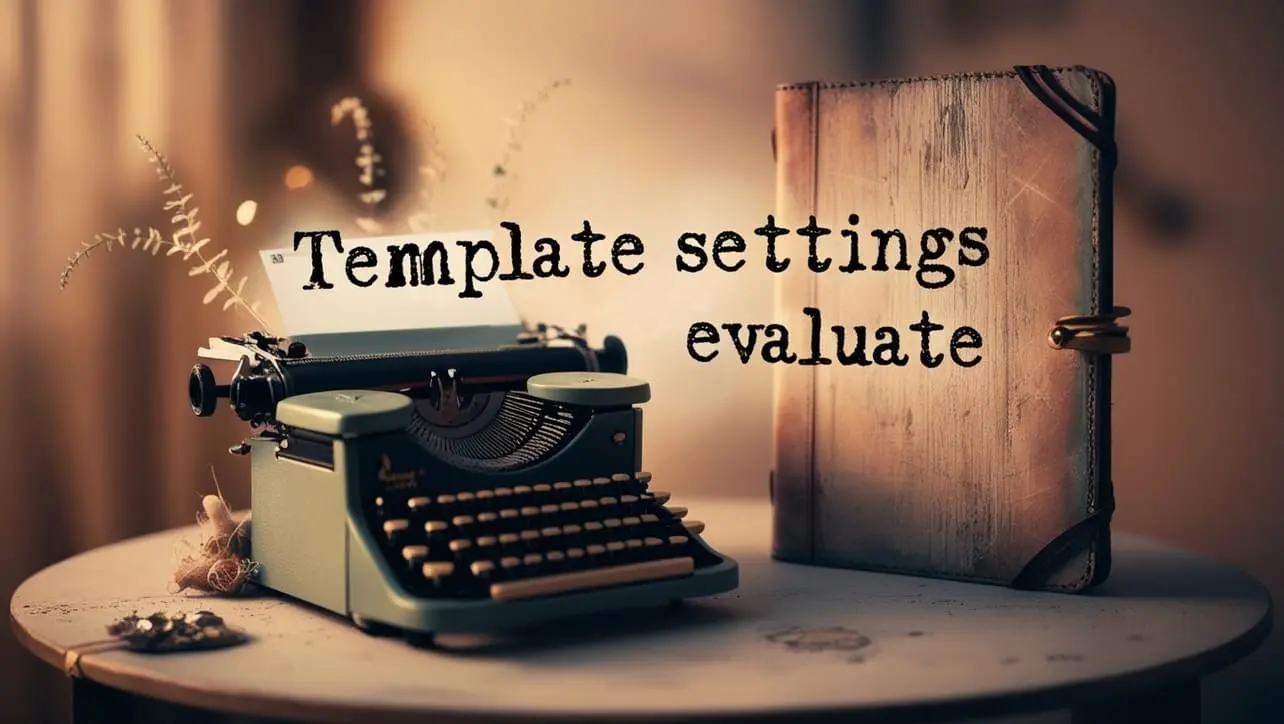
Lodash _.templateSettings.evaluate Property
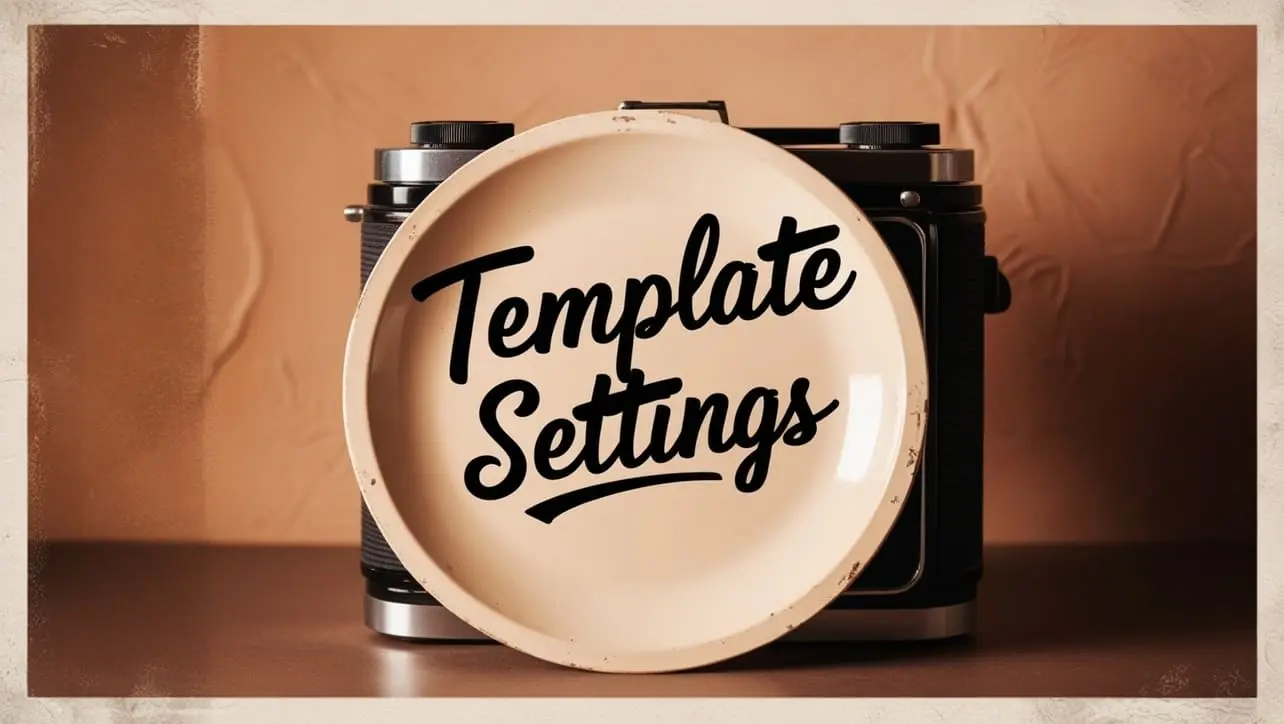
Lodash _.templateSettings Property
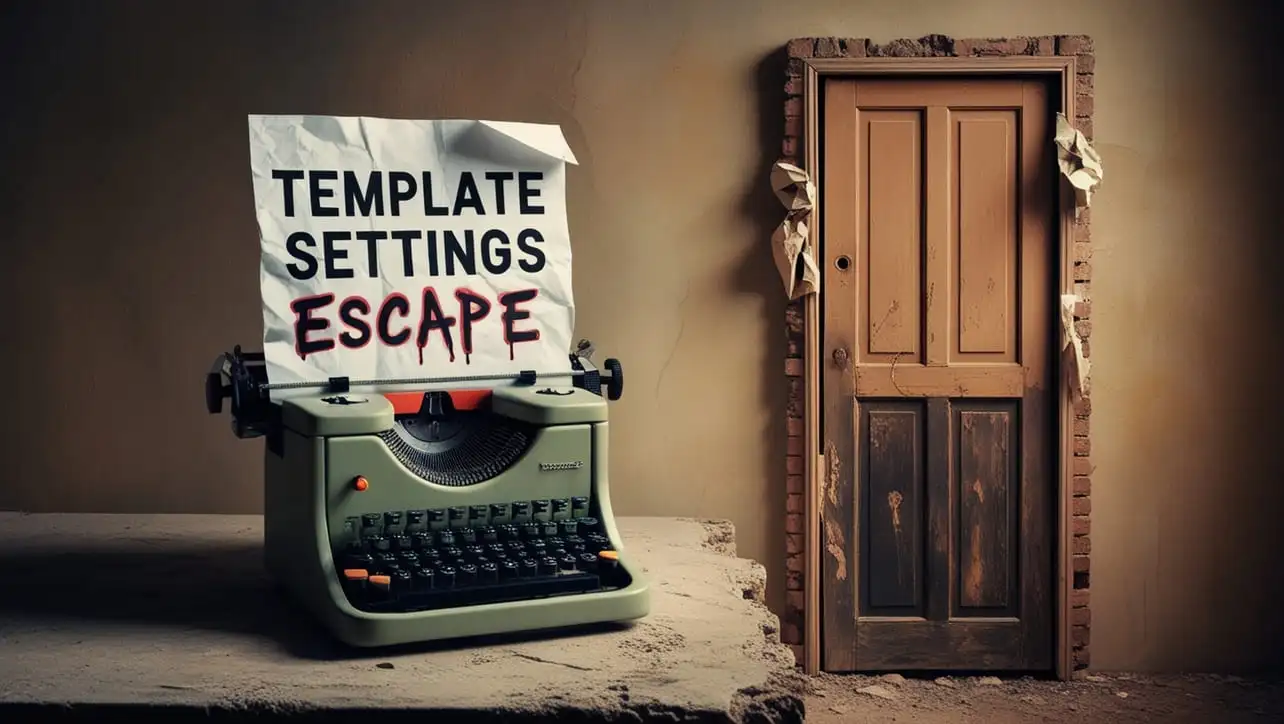
Lodash _.templateSettings.escape Property
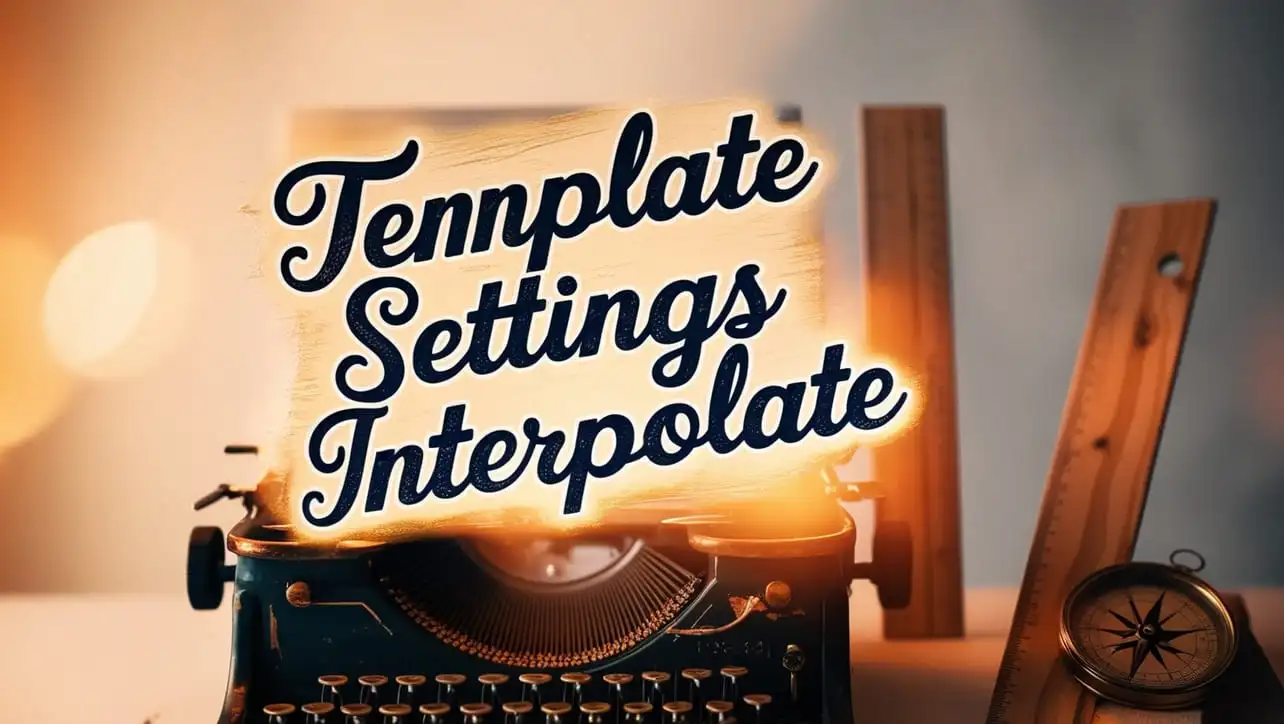
Lodash _.templateSettings.interpolate Property
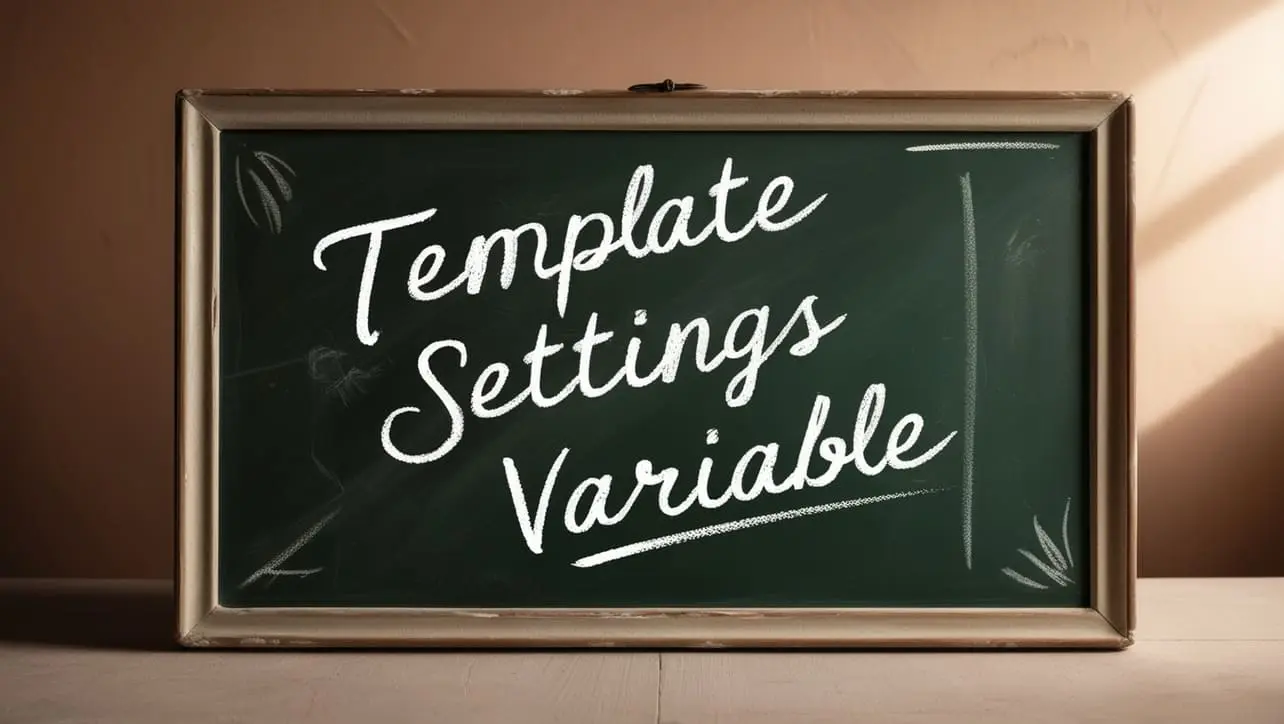
If you have any doubts regarding this article (Lodash _.updateWith() Object Method), please comment here. I will help you immediately.